What is RSpec? Learn How It Transforms Ruby Testing
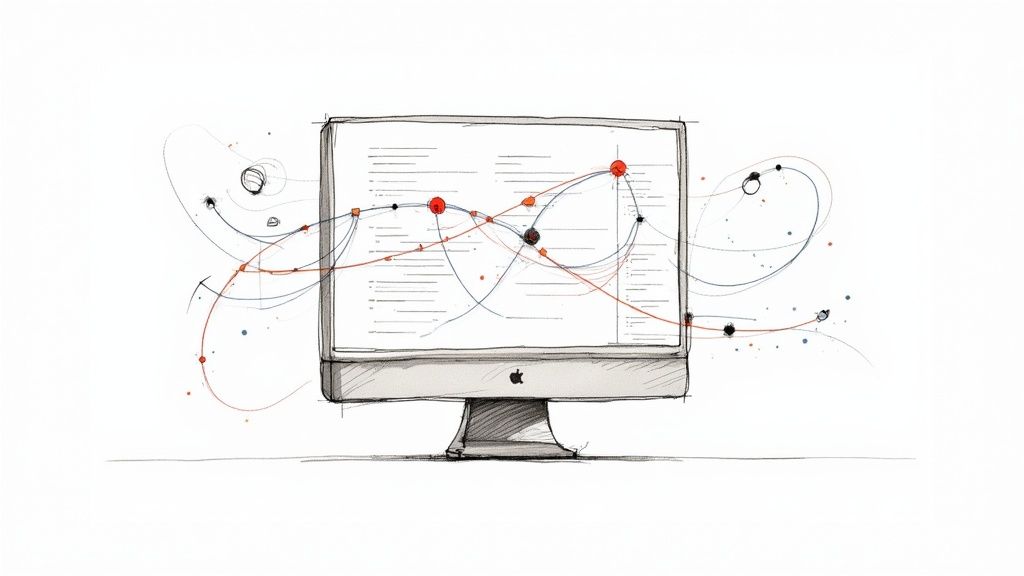
What Is RSpec: Beyond Just Another Testing Tool
RSpec isn't simply a testing tool; it's a domain-specific language (DSL) that describes your Ruby code's behavior in a readable format. This behavior-driven development (BDD) approach changes how developers approach testing, turning it from a chore into a design tool. Instead of verifying how the code is implemented, RSpec encourages you to describe what your code should do, making tests clearer and more useful. This means tests become living documentation, illustrating your application's intended behavior.
This approach encourages collaboration. RSpec's readable syntax allows developers, product managers, and even non-technical stakeholders to understand the specifications, improving communication and shared understanding. Imagine describing a user login process. With RSpec, you can write tests that read like plain English, explaining the system's behavior in different scenarios, like successful logins, incorrect passwords, or locked accounts.
Interestingly, RSpec began as a teaching tool in 2005, created by Steven Baker. Initially designed to clarify testing concepts, it quickly gained popularity for its BDD approach. By 2014, RSpec reached version 3.0. Now at version 3.12, RSpec shows its continued evolution and adaptation to Ruby's testing needs. Learn more at Mergify's blog post about RSpec. This ongoing development keeps RSpec effective in the changing world of software development.
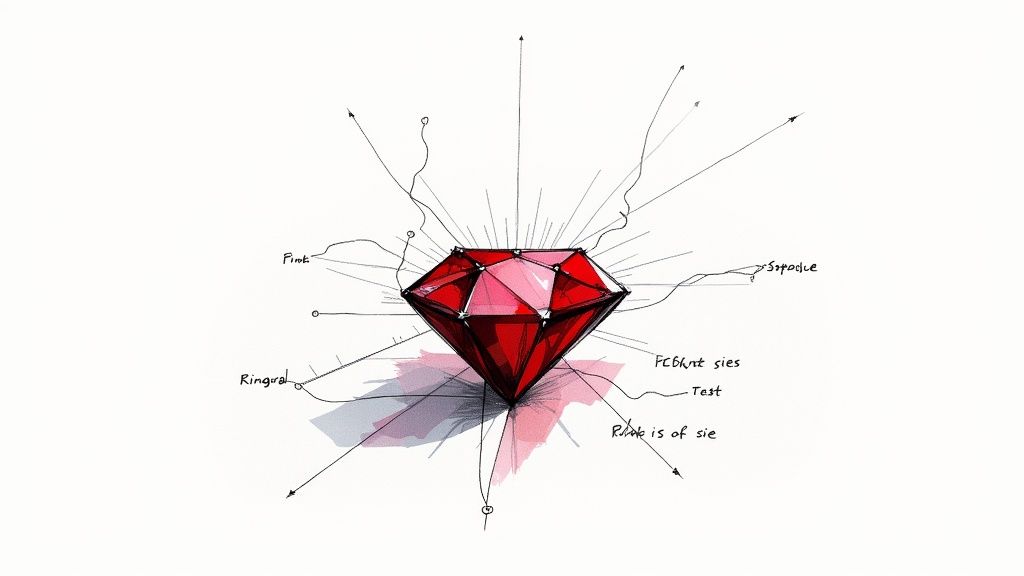
Core Components of RSpec
RSpec's modular design makes it flexible and powerful. Its core components work together, letting you use what's needed for each testing situation. These key parts include:
- rspec-core: This component manages the testing process, forming the base of your test suite.
- rspec-expectations: This provides many "matchers," letting you clearly and concisely state your expectations for your code's behavior.
- rspec-mocks: This allows the creation of test doubles (mocks, stubs, and spies) to precisely control interactions between parts of your code during testing.
- rspec-rails: This integrates RSpec with Rails applications, offering specific helpers and matchers to simplify testing Rails features like controllers, models, and views.
This modularity allows teams to adapt their testing strategies to their needs. This is vital for maintaining code quality without slowing down development. As projects change and grow, teams can adjust their testing by adding or extending specific RSpec components, keeping testing focused and efficient.
Why 90% of Rails Developers Choose RSpec
RSpec's widespread popularity in the Ruby on Rails community is no accident. It comes down to real advantages that make it the go-to testing framework for so many. One major factor is RSpec's expressive syntax, turning what could be complex test scenarios into readable, maintainable code. This readability isn't just nice to have; it genuinely helps teams collaborate and understand each other's work better.
RSpec's describe/context/it structure mirrors how developers naturally think about software behavior. This pattern helps organize tests logically by describing actions under various conditions. For example, you might describe a user model, set a context for a "valid user," and then check if it "should be able to log in." This structure adds clarity and makes tests easier to write, understand, and maintain over time.
RSpec also provides a rich matcher library. Matchers are specialized methods that let you express expectations about your code's behavior clearly and concisely. They remove unnecessary code, making tests shorter and easier to read. And when tests fail, RSpec gives detailed error messages that pinpoint the problem. This significantly cuts down debugging time and speeds up development.
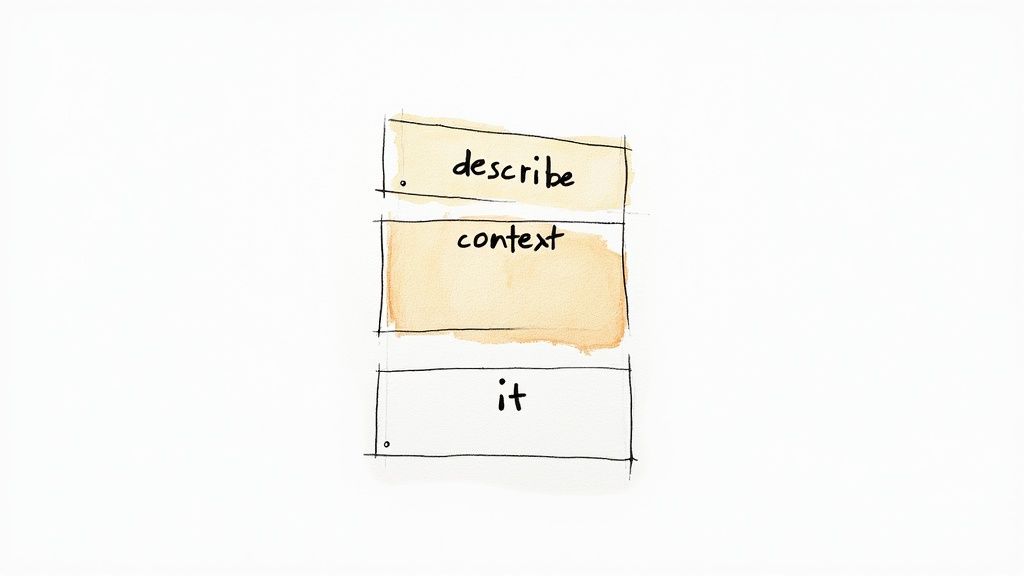
This widespread use shows how well RSpec integrates with other tools. Up to 90% of new Rails projects use RSpec, a strong sign it's preferred over options like Minitest. This popularity highlights how effective and easy it is to use within the Rails ecosystem. Explore this topic further. The rspec-rails
gem, designed to integrate RSpec with Rails, offers seamless testing tools and generators.
Comparing RSpec with Minitest
While Minitest is a viable option, RSpec has distinct advantages that explain its popularity. The following table summarizes key differences.
To understand these differences better, let's look at a comparison table:
RSpec vs. Minitest Comparison: A side-by-side comparison of RSpec and Minitest highlighting key differences in syntax, features, and community adoption.
Feature | RSpec | Minitest |
---|---|---|
Syntax | Domain-Specific Language (DSL) | More traditional Ruby syntax |
Readability | Highly readable | Can be less descriptive |
Matchers | Extensive library of built-in matchers | Fewer built-in matchers |
Flexibility | Highly flexible and extendable | Less flexible |
Community Support | Larger and more active community | Smaller community |
This table clearly illustrates RSpec's focus on describing behavior, not just implementation details. This makes your test suites more robust and adaptable. As your code changes, your tests are less likely to break due to internal tweaks if the external behavior stays the same. This promotes more sustainable and maintainable codebases over the long term.
Inside RSpec: The Components That Power Your Tests
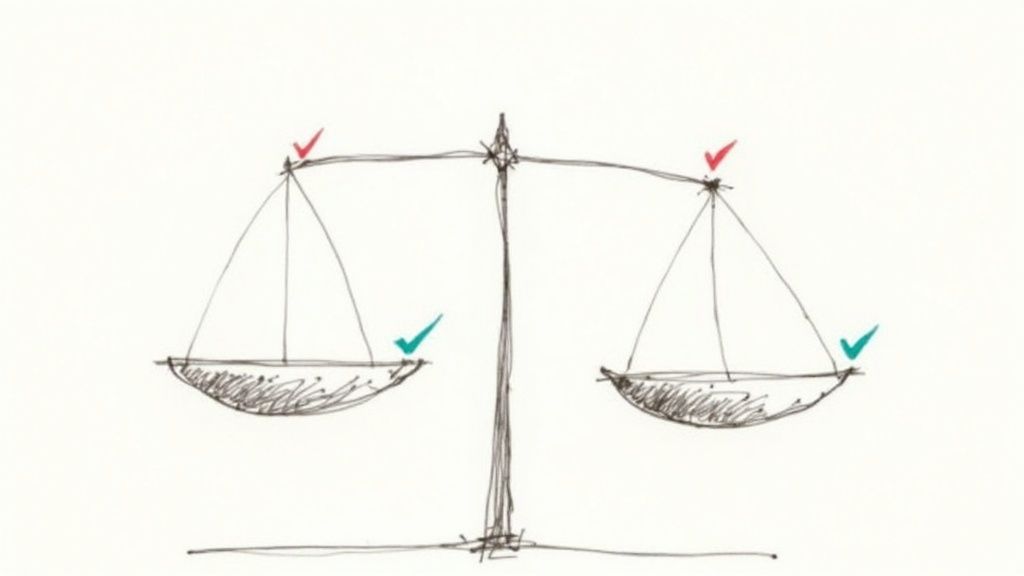
RSpec provides a smooth testing experience thanks to its modular design. This section explores the core components that work together, offering flexibility in your testing approach. We'll see how these individual parts interact and contribute to the overall power of RSpec.
RSpec-Core: The Foundation
At the heart of RSpec is rspec-core. This core component provides the foundational structure, orchestrating the execution of your test suite. It loads your tests, ensuring they run in the correct order, and then reports the results.
Think of rspec-core as the conductor of an orchestra. It brings all the elements together, ensuring a harmonious execution of your tests. It's the backbone of your testing process.
RSpec-Expectations: Clear Assertions
RSpec-expectations helps you define the intended behavior of your code. It uses "matchers" which are methods that express expected outcomes in a clear and concise way.
For example, expect(user.valid?).to be_truthy
verifies the validity of a user object. This readable syntax not only clarifies your tests but also makes them easier to maintain as your code changes.
RSpec-Mocks: Isolating Units of Code
RSpec-mocks allows you to isolate parts of your code by controlling their interactions. You can create "test doubles"—mocks, stubs, and spies—which mimic real objects.
These doubles allow you to specify the exact behavior of these objects during tests. This isolation makes tests more predictable and focused on specific functionalities.
RSpec-Rails: Seamless Rails Integration
For Rails developers, rspec-rails is a key component. It provides helpers and matchers tailored for testing Rails elements such as models, controllers, and views.
This integration streamlines the setup and writing of tests within the Rails environment. By leveraging Rails' conventions, rspec-rails makes your tests feel like a natural extension of your Rails application.
The Benefits of Modularity
These components work together to create a robust and adaptable testing environment. Teams can choose the components they need for each testing scenario, ensuring efficient use of resources and minimizing unnecessary overhead.
This means you have the right tools at your fingertips for every testing situation. As your application scales, this modularity allows your testing strategy to adapt and maintain code quality without hindering development speed.
This flexible, modular approach is a significant strength of RSpec. It supports a variety of testing styles and allows you to create a testing strategy that best fits your application and your team's needs. This ultimately contributes to a more efficient process and a higher quality product.
Mastering RSpec Test Types for Rails Applications
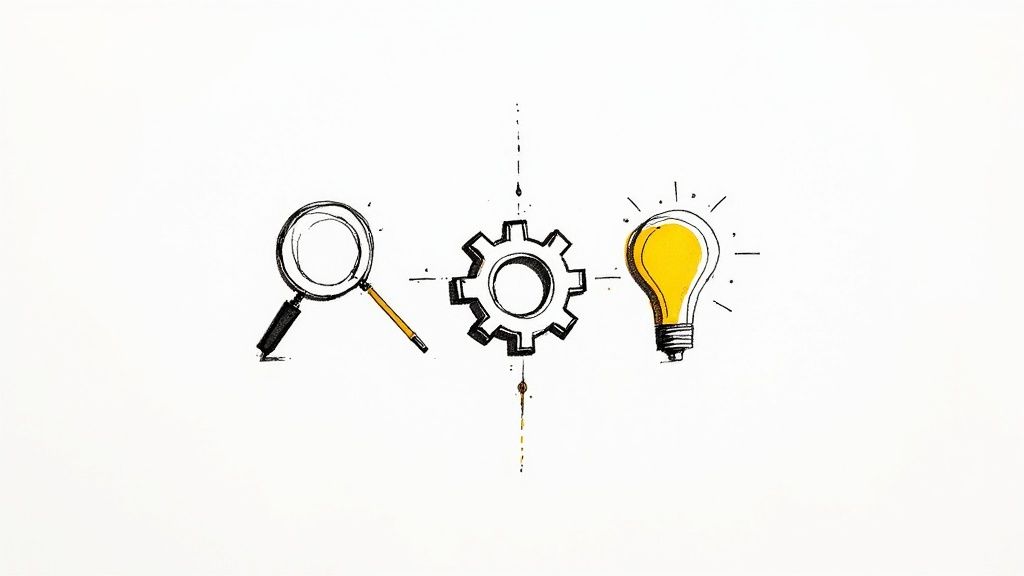
Building robust and reliable Rails applications demands a comprehensive testing strategy. Understanding the various test types offered by RSpec, a powerful testing framework for Ruby, is key to this process. Selecting the right test type for each scenario ensures thorough testing while maintaining efficiency.
Unit Tests: Verifying Individual Components
Unit tests are the bedrock of a solid test suite. They examine the smallest pieces of your application – individual methods or classes – in isolation. This isolation allows developers to quickly pinpoint and address issues at the source. Think of it as checking individual parts of a machine before assembly. Unit tests are renowned for their speed, making them perfect for continuous integration pipelines where rapid feedback is essential.
Request Specs: Testing API Endpoints
For applications with API endpoints, request specs verify that your application's interfaces behave as expected. They examine the HTTP interactions, concentrating on the requests sent to your application and the responses received. This confirms proper data handling and status codes. For example, a request spec would verify that a POST request to create a user correctly creates the record and returns the appropriate HTTP status code.
Model Specs: Validating Business Logic
Model specs examine the core of your application: the business logic within your models. They verify data integrity, relationships, and any custom methods. For instance, a model spec might check that a user's email address is both unique and properly formatted before being saved to the database. This protects against data corruption and ensures core functions work as designed.
System Specs: End-to-End Testing
System specs, introduced in Rails 5.1, provide crucial end-to-end validation. They simulate real user interactions, ensuring all parts of your application work harmoniously. System specs are essential for testing complex user flows, like a user adding items to a shopping cart and completing the checkout process. A system spec would test this entire flow, from adding items to final purchase confirmation.
RSpec supports various testing types including system specs, feature specs (which serve a similar purpose with additional setup capabilities), and request specs. This allows developers to choose the appropriate tool for any testing scenario.
Balancing Test Types: Speed and Coverage
Experienced Rails developers recognize the trade-offs between test coverage and execution speed. While thorough testing is vital, lengthy test suites can hinder development. Leading Ruby teams strategically employ different RSpec test types, often focusing on fast unit tests for core components and strategically using system specs for critical user flows.
The following table provides a helpful overview of the different RSpec test types and their usage:
RSpec Test Types in Rails Applications
Test Type | Purpose | When To Use | Speed |
---|---|---|---|
Unit Tests | Test individual methods or classes in isolation | Testing small, independent units of code | Fastest |
Request Specs | Test API endpoints and HTTP interactions | Testing API responses and data handling | Fast |
Model Specs | Validate business logic and data integrity within models | Testing model validations, relationships, and custom methods | Moderate |
System Specs | Simulate real user interactions and test end-to-end flows | Testing complex user flows and interactions across multiple components | Slowest |
This table summarizes the different RSpec test types available in Rails applications, providing a clear understanding of their purposes, appropriate usage, and relative speeds.
Structuring Your Test Suite for Success
A well-structured test suite, with clearly defined purposes for each test type, is essential for maintainability and effectiveness as your application grows. Each type of test plays a specific role in verifying different aspects of your application’s behavior. This structured approach allows your testing efforts to scale alongside your application's increasing complexity.
How RSpec Changed Testing Beyond the Ruby World
RSpec's influence extends far beyond its Ruby origins. Its innovative behavior-driven development (BDD) approach has fundamentally changed how developers across various languages approach testing. This section explores how RSpec's core principles have permeated testing frameworks in languages ranging from JavaScript to Python and PHP.
One of RSpec's key contributions is its emphasis on describing behavior. This shifts the focus from testing internal implementation details to specifying desired outcomes. For instance, instead of testing the inner workings of a user login function, RSpec encourages defining how the system should behave under different scenarios: correct credentials, incorrect credentials, or a locked account. This leads to tests that are more readable, easier to maintain, and ultimately contribute to more resilient code.
The Spread of BDD and Readable Syntax
Central to RSpec's BDD approach is its human-readable syntax, a characteristic widely adopted by other testing frameworks. Structured around terms like describe
, context
, and it
, this syntax allows tests to be written in a way that resembles natural language. This increased readability benefits not only developers but also non-technical stakeholders, improving communication and collaboration across teams. This bridging of the communication gap between technical and non-technical team members has played a key role in BDD's broader adoption.
This influence is evident in JavaScript testing frameworks like Jasmine and Mocha, both of which utilize a similar descriptive syntax. Likewise, Python's Behave and PHP's Codeception frameworks have embraced BDD methodologies, highlighting the widespread recognition of RSpec's impact. The move toward more readable test suites has made testing more accessible and less tedious for developers across various tech stacks. Furthermore, RSpec's integration with continuous integration platforms like Semaphore streamlines automated test runs and CI/CD pipeline integration, boosting productivity and ensuring consistent quality. Learn more about CI/CD with RSpec.
From Specification by Example to Living Documentation
RSpec's focus on specifying behavior naturally results in tests that act as living documentation. These tests become executable specifications, offering a clear and current view of the application's intended functionality. This ensures that code and documentation stay in sync, minimizing inconsistencies and enhancing project maintainability.
The practice of test-driven development (TDD), writing tests before the actual code, has also gained considerable traction, frequently alongside BDD. This approach promotes writing code with testability in mind, resulting in more modular and well-defined components, further contributing to robust and maintainable software.
Beyond Specific Frameworks: A Change in Mindset
RSpec's influence goes beyond inspiring the creation of similar frameworks. It marks a shift in the developer mindset toward a more collaborative and behavior-focused approach to testing. Understanding RSpec's philosophy benefits developers regardless of their chosen language, fostering better communication, clearer specifications, and ultimately, more robust and maintainable code.
Your First RSpec Test: From Setup to Success
This section offers a practical walkthrough for creating your first RSpec tests. We'll explore the initial setup and configuration process for both standalone Ruby projects and Rails applications, addressing any potential hiccups along the way. We'll then dive into RSpec's core structure and syntax, providing you with a solid foundation to begin testing your own Ruby code effectively.
Setting Up RSpec
The initial step involves installing the rspec
gem. For standalone Ruby projects, simply use the command gem install rspec
. If you're working within a Rails application, add gem 'rspec-rails'
to your Gemfile and then execute bundle install
. This installs the necessary libraries and tools required for writing and running your RSpec tests.
After installing the gem, you need to initialize RSpec within your project. For standalone projects, execute the command rspec --init
. This generates the spec
directory and the crucial spec_helper.rb
file, which configures the RSpec environment. For Rails applications, run rails generate rspec:install
. This sets up a similar configuration tailored specifically for Rails, creating all the necessary helpers and configuration files.
Structuring Your Tests: Describe-Context-It
RSpec employs a describe-context-it structure for organizing tests. This pattern significantly enhances readability and organization.
describe
: This defines a test suite for a specific class or module. For example,describe User
would encapsulate all tests related to theUser
class.context
: This specifies different scenarios or conditions within adescribe
block. For instance,context "with valid attributes"
sets the stage for tests focused on a user object with valid attributes.it
: This represents an individual test case. Inside anit
block, you write your assertions using RSpec'sexpect
syntax to verify the expected behavior. For example,it "should be valid"
would contain the assertion to check the validity of a user.
This structure allows tests to resemble natural language descriptions of the code's intended behavior. This clarity improves understanding of the tests and also serves as valuable documentation.
Writing Your First Test
Let's illustrate with a simple example. Imagine you have a User
class with a valid?
method. A corresponding RSpec test might look like this:
describe User do context "with valid attributes" do let(:user) { User.new(name: "John Doe", email: "john.doe@example.com") }
it "should be valid" do
expect(user.valid?).to be_truthy
end
end
context "with invalid attributes" do let(:user) { User.new(name: nil, email: nil) }
it "should not be valid" do
expect(user.valid?).to be_falsy
end
end end
In this example, we're describing the User
class and defining two contexts: one with valid attributes and another with invalid attributes. Within each context, an individual test (it
block) asserts the user's validity using RSpec's expect
syntax. This structured approach ensures your tests remain organized and easy to understand as your project grows.
Interpreting RSpec Output
After running your tests (using rspec spec
), RSpec provides detailed output indicating whether each test passed or failed. This feedback is crucial for identifying and addressing issues quickly. RSpec’s output format can be customized to include more detailed information for debugging, as explored in various blog posts. One technique involves injecting browser logs directly into failure messages, providing insight into any front-end errors directly within your test results.
Matchers: Expressing Expectations Clearly
RSpec's matchers are methods like be_truthy
, be_falsy
, eq
, include
, and many others. They are used to express expectations about your code's behavior clearly and concisely. For example, expect(user.name).to eq("John Doe")
asserts that the user's name is "John Doe".
RSpec offers a wide array of matchers to cover a multitude of scenarios. These matchers enhance readability and efficiency when writing tests.
Ready to optimize your development workflow and significantly reduce CI costs? Discover how Mergify can help your team.