Version Control in Git: A Developer's Proven Guide to Code Management
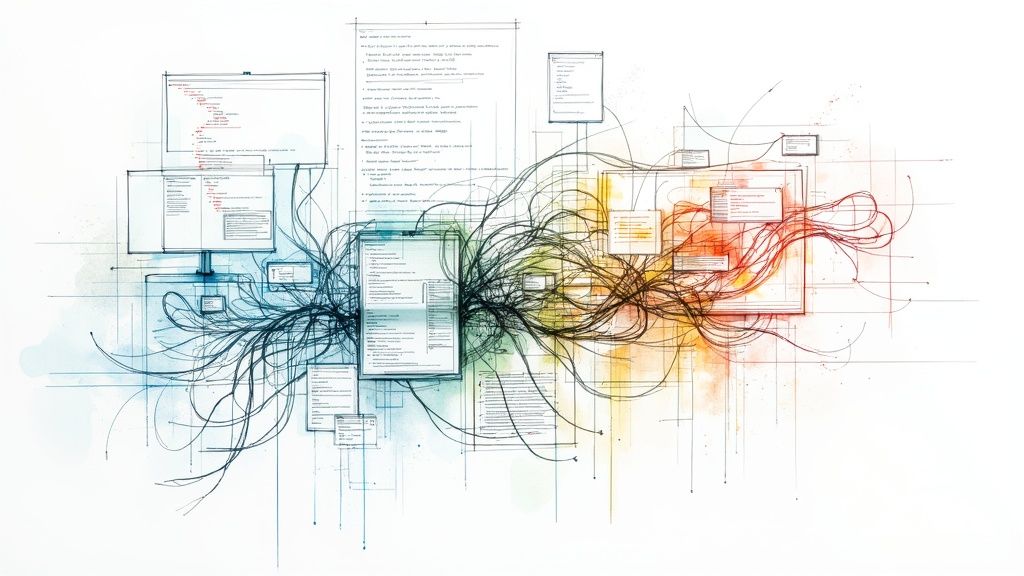
Understanding Version Control and Git's Evolution
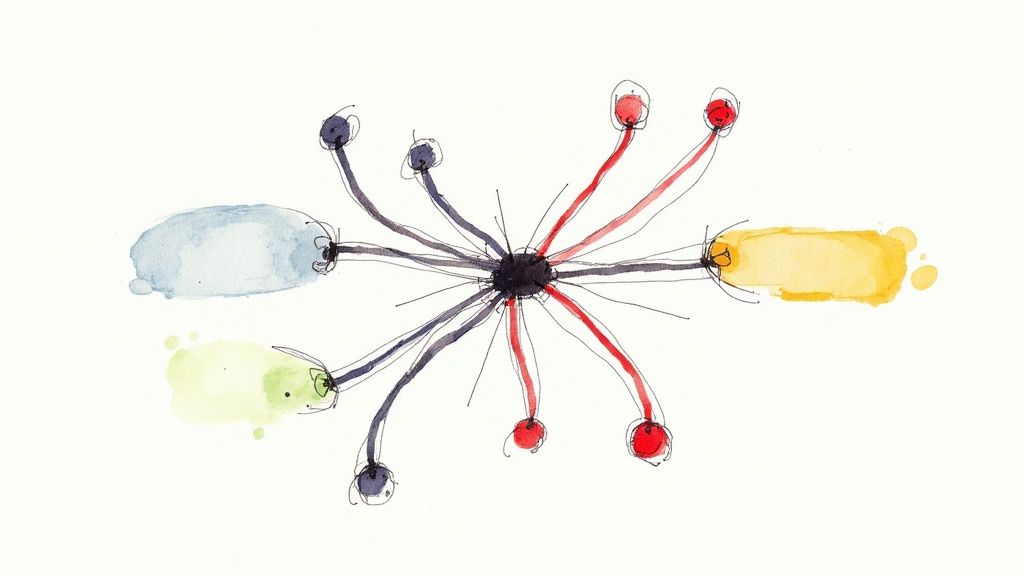
Version control is essential in modern software development - it's how teams track changes and work together effectively. This system helps developers roll back to earlier versions when needed, test new features safely, and collaborate without stepping on each other's toes. Multiple developers can work simultaneously while maintaining code quality and avoiding conflicts.
The Rise of Distributed Version Control
Early centralized version control systems (CVCS) had a major weakness - they relied entirely on a single server. When that server went down, work ground to a halt since developers couldn't access code or collaborate. This limitation led to the development of distributed version control systems (DVCS) like Git. The key difference? Every developer gets their own complete copy of the code and its history. This means faster performance, better reliability, and the ability to work offline.
Git's Origin Story
Git's creation story shows how necessity drives innovation. In 2005, the Linux kernel project - one of the largest open-source efforts at the time - lost access to their version control system BitKeeper when its free license was revoked. This crisis prompted Linux creator Linus Torvalds to build something new. In just a few weeks, Torvalds and his team developed Git's first version, releasing it on April 3, 2005. By June, Git was already managing Linux kernel version 2.6.12, proving it could handle major projects. Learn more about Git's history and capabilities.
Key Architectural Decisions in Git
Git's design includes several smart features that set it apart. The staging area acts as a middle step between your working files and the repository, letting developers carefully choose which changes to include in each commit. This helps keep the project history clean and organized. Git also makes branching simple and efficient - creating, merging, and removing branches happens quickly without bogging down the system.
Why Understanding Git is Crucial
For developers today, knowing Git isn't optional - it's a must-have skill. Git powers collaboration across the software industry, from small teams to massive open-source projects. Tools like Mergify build on Git's foundation to make development workflows even better. When you understand Git, you can join open-source projects, contribute to large codebases, and use powerful automation tools that boost productivity. This knowledge helps both individual developers and teams create better software more efficiently.
Mastering Essential Git Commands and Workflows
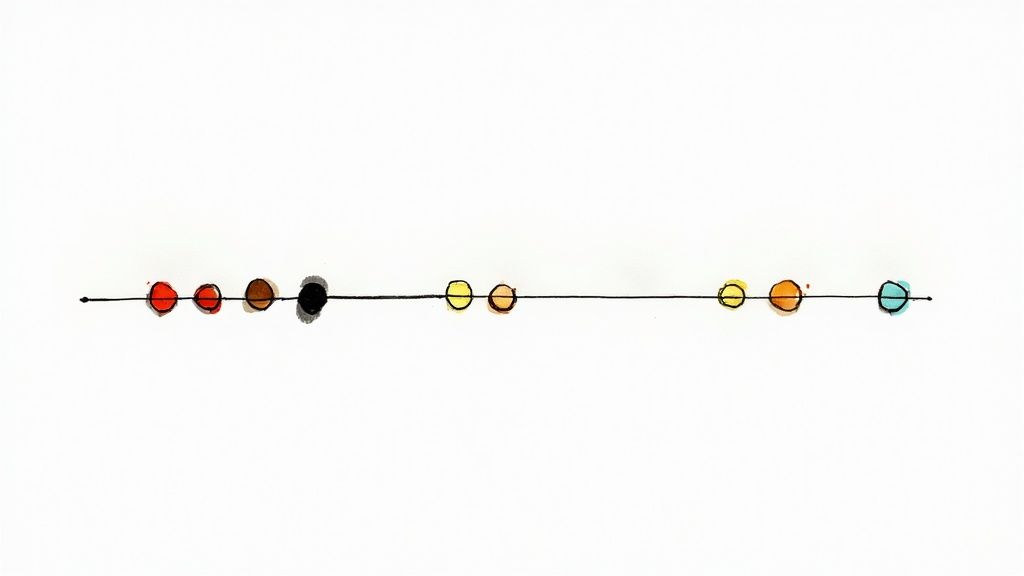
Understanding Git commands and workflows is crucial for any developer. Let's explore how to use these tools effectively to maintain clean and organized codebases. We'll focus on the practical aspects of Git - not just memorizing commands, but learning when and why to use them.
Core Git Commands: Building Blocks of Version Control
The foundation of Git rests on a few key commands that you'll use daily. Mastering these basics will make version control smoother and more intuitive.
git init
: Sets up a new Git repository in your project foldergit clone
: Makes a copy of an existing repository on your local machinegit add
: Prepares your changes to be saved by moving them to the staging areagit commit
: Takes a snapshot of your staged changes with a message explaining what you didgit status
: Shows which files have changed and what's ready to be committedgit push
: Sends your committed changes to a remote repositorygit pull
: Gets the latest changes from a remote repository and updates your local copy
Working with Branches: Managing Parallel Development
Branches let you work on different parts of your project without affecting the main code. This is perfect for developing new features or fixing bugs safely.
git branch
: Shows all branches in your repositorygit checkout -b <branch_name>
: Creates and switches to a new branchgit checkout <branch_name>
: Moves you to an existing branchgit merge <branch_name>
: Combines changes from one branch into another
Handling Merge Conflicts: Resolving Code Clashes
When multiple developers change the same code, conflicts can happen. While tools like Mergify can help automate conflict resolution, knowing how to handle conflicts manually is essential. Git marks conflicting sections in your code, letting you choose which changes to keep or how to combine them.
Recent studies show the value of version control: developers using systems like Git see a 30% drop in coding errors and teams experience a 20% boost in productivity. For more details, check out the Git development statistics.
Establishing Efficient Workflows: Best Practices for Teams
Good teamwork depends on consistent processes. Many teams use a feature branch workflow, where new features are built on separate branches. This keeps the main branch stable and makes code reviews easier.
Other common approaches include Gitflow for larger projects and the Forking workflow for open-source collaboration. Whatever method you choose, stick to clear commit messages, regular code reviews, and automated checks to keep your codebase clean and manageable.
Strategic Branching and Merging Techniques
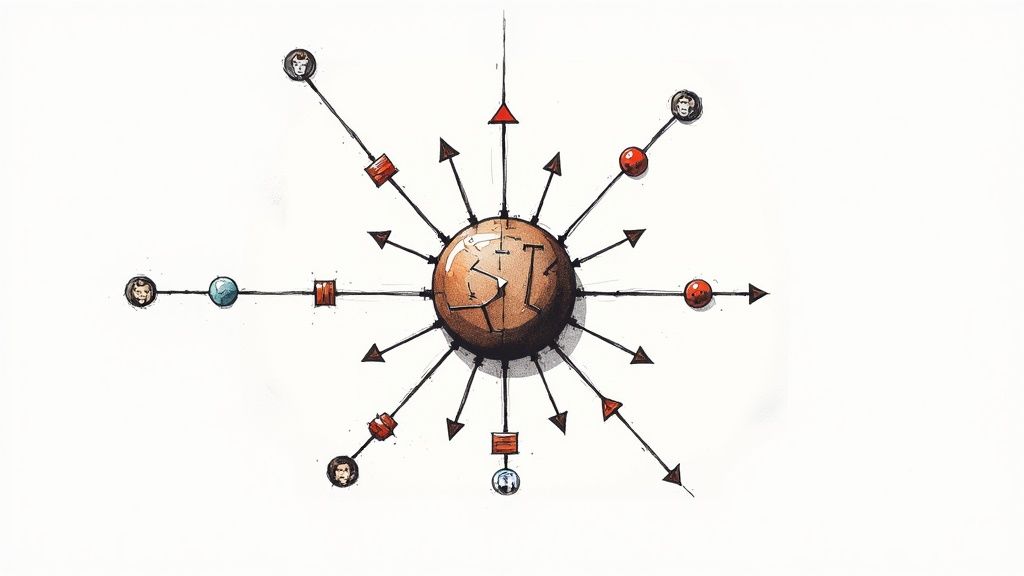
Good branching and merging practices form the backbone of Git version control. These core techniques let teams work simultaneously on features, fixes, and releases without disrupting the main code. Let's explore the key patterns and practices that make this possible.
Common Branching Patterns
Teams can choose from several proven branching models based on their specific needs and structure:
- Feature Branching: Create a dedicated branch for each new feature or bug fix. This keeps changes separate, makes code reviews clearer, and prevents errors from reaching the main branch.
- Release Branching: Set up branches specifically for preparing new versions. This provides a stable space for final testing and fixes before deployment, while other development continues normally.
- Hotfix Branching: For urgent fixes that can't wait for the normal release cycle, create a hotfix branch from production code. After deploying the fix, merge those changes back into development.
- Gitflow: Larger projects often benefit from this structured approach that defines specific branch types and rules for development, releases, and fixes.
These core strategies give teams a solid foundation for organizing their code and working together smoothly.
Rebasing vs. Merging: Choosing the Right Strategy
When combining changes between branches, you have two main options - each with its own strengths:
- Merging: Keeps a complete record of your project's history, including every merge point. While this provides an accurate timeline, it can make the history harder to follow.
- Rebasing: Places your branch's changes at the end of the target branch, as if they happened in sequence. This creates a simpler history but changes the original order of events.
Picking the right approach depends on what matters most to your team - preserving exact history or maintaining a clean, linear record.
Handling Merge Conflicts
When multiple developers change the same code, conflicts are bound to happen. Tools like Mergify can help automate conflict resolution, but sometimes you need to step in manually. Git clearly marks conflicting sections, letting you review the differences, choose which changes to keep, and commit the final version.
The widespread use of Git makes conflict resolution an essential skill. According to a 2018 Stack Overflow survey of 74,298 developers, 88.4% used Git as their version control system. Nearly 90% of developers have dealt with merge conflicts, showing just how common this challenge is. More details are available here.
Best Practices for Branching and Merging
Follow these key guidelines to improve your branching and merging workflow:
- Keep Branches Short-Lived: Merge branches back quickly to reduce complexity and avoid conflicts
- Use Clear Branch Names: Name branches to show their purpose, like "feature/user-authentication" or "bugfix/login-error"
- Stay Updated: Pull changes from the main branch often to catch conflicts early
- Review Code Thoroughly: Check all code changes carefully before merging to catch issues and maintain quality
These practices help teams work efficiently while keeping their Git repository clean and well-organized. This creates a strong base for teamwork and quality code.
Building Effective Remote Repository Strategies
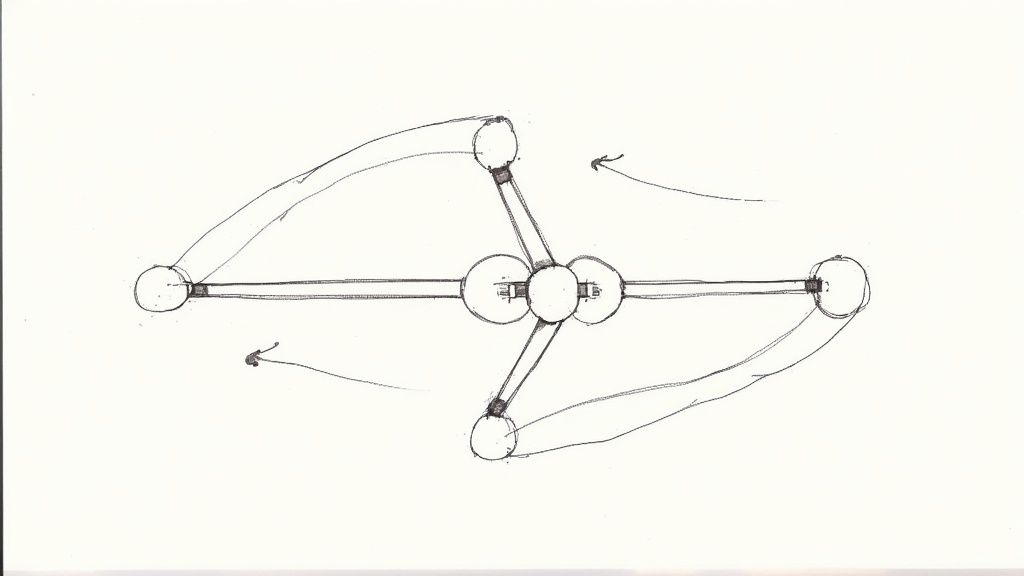
When teams work together from different locations, having a solid plan for managing shared code is essential. Git's remote repository features provide the foundation for smooth collaboration, efficient code reviews, and organized project management. Let's explore practical strategies for making the most of these capabilities.
Managing Remote Repositories in Git
At its core, Git remote collaboration centers on remote repositories - copies of your project that live on a server where team members can share and sync their work. The basic workflow involves three main commands: git clone
to download a complete copy of the remote repository to your computer, git push
to send your local changes back to the remote, and git pull
to get the latest updates from the remote to your local copy.
For instance, when starting work on an existing project, you'll first use git clone
to get your own working copy. As you make changes, you'll use git push
to share them with the team, and git pull
regularly to stay in sync with others' contributions.
Working with Multiple Remotes
Sometimes you'll need to work with several remote repositories at once. This is common in open-source work or when dealing with project forks. Git makes it simple to set up and manage connections to different remotes, so you can easily contribute to various project branches or versions simultaneously.
Streamlining Code Review Workflows
Code reviews become much easier with remote repositories. Popular platforms like GitHub and GitLab build on Git's features to create structured review processes. Tools like Mergify can help automate these workflows, saving time and effort. Pull requests are particularly useful here, giving teams a clear way to discuss changes and ensure code quality.
Maintaining Security with Proper Permissions
When working with shared code, security needs careful attention. Git provides ways to control who can do what - from basic read/write access to specific branch protections. Setting up the right permissions helps prevent mistakes and keeps your code safe.
By combining these security controls with Git's branching features, teams can work together effectively while maintaining proper oversight. This balanced approach works well even when team members are spread across different locations, ensuring both productivity and code safety.
Advanced Git Tools and Techniques
This section explores powerful Git methods used by skilled developers to enhance their version control practices. By mastering these advanced capabilities, teams can boost collaboration and manage code more effectively.
Interactive Rebasing
Interactive rebasing puts you in control of your commit history. Think of it like editing a document - you can fix old commit messages, combine related small changes into one logical update, or restructure your history for clarity. For example, if you made several small formatting commits, you could combine them into a single "Code formatting updates" commit. This keeps your project timeline clean and easy to follow.
Cherry-Picking
Cherry-picking lets you copy specific commits between branches with surgical precision. This comes in handy when you need to apply an urgent bug fix to multiple branches. For instance, if your team discovers a security vulnerability while preparing a release, you can cherry-pick just that fix from development into the release branch. This focused approach gives you exact control over which changes go where.
Git Hooks
Git hooks are automated scripts that run at key points in your Git workflow. They act like quality control checkpoints for your code. You can set up hooks to:
- Run code formatting checks before commits
- Verify commit message formatting
- Execute test suites automatically
- Block commits that don't meet standards
This automation helps catch issues early and keeps code quality consistent across the team.
Aliases and Helper Tools
Custom aliases and specialized tools can speed up your daily Git work significantly. Git aliases work like keyboard shortcuts - they let you create simple commands for complex operations. For example, instead of typing git checkout -b new-branch
, you could use git cb new-branch
. Third-party tools like Mergify add features like automated merging and branch protection that make managing large projects easier.
Debugging and Maintenance
Even experienced teams run into Git challenges occasionally. That's why knowing how to troubleshoot issues and keep your repository organized is crucial. Tools like git reflog
track all your repository changes, helping you recover from mistakes. The git bisect
command helps pinpoint problematic commits by using a binary search process. Regular use of these debugging tools helps maintain a clean, efficient codebase and makes it easier to resolve conflicts when they arise.
Implementing Enterprise-Grade Git Workflows
Managing Git effectively across large teams requires thoughtful planning and well-structured processes. When organizations grow to dozens or hundreds of contributors, it becomes increasingly important to establish consistent practices. Here's how to create and scale reliable Git workflows with real-world examples and techniques.
Standardized Commit Messages: Ensuring Clarity and Traceability
Good commit messages help everyone understand what changed and why. A clear messaging system makes it much easier to review code, track down changes, and maintain clean project history.
Here's what makes commit messages effective:
- Keep summaries brief: Your first line should be around 50 characters - think of it like an email subject line
- Add helpful details: Use the message body to explain your reasoning and approach, not just what you changed
- Connect to issues: Include references like "Fixes #123" to link commits with your issue tracking system
- Follow conventions: Use an established format like Conventional Commits to enable automation
These simple practices make a big difference in keeping your Git history clean and useful.
Repository Organization: Structuring for Scalability
The way you organize your codebase has a huge impact on how easily teams can work together. Good structure makes it simple to find code, manage dependencies, and grow sustainably.
Key organization principles:
- Break into modules: Split large codebases into smaller, focused packages that teams can work on independently
- Handle dependencies well: Use Git submodules or subtrees to cleanly manage external code
- Pick clear branching: Choose a branching strategy (like Gitflow or feature branches) that matches how your team works
Version Tagging: Marking Key Milestones
Version tags help teams track releases and roll back changes when needed. They act as markers for important points in your project's timeline.
Best practices for version tagging:
- Use semantic versions: Follow the MAJOR.MINOR.PATCH format to clearly show the scale of each change
- Tag every release: Create version tags to mark completed releases for easy reference
- Set up automation: Let your CI/CD system handle tagging as part of successful builds
This approach creates clear project history that's easy to navigate and maintain.
Onboarding and Documentation
Getting new team members up to speed quickly is crucial for growing teams. Clear guidance on Git practices helps everyone contribute effectively from day one.
Essential onboarding elements:
- Write a style guide: Document your team's Git conventions and practices in one central place
- Create learning resources: Give new members practical examples and guides for common Git tasks
- Build team support: Help team members learn from each other through code reviews and mentoring
Good onboarding materials and documentation keep practices consistent as teams grow and change.
Mergify helps teams work together more smoothly by automating merge processes, managing pull requests, and making collaboration easier.