Using pytest: Boost Your Python Testing Skills
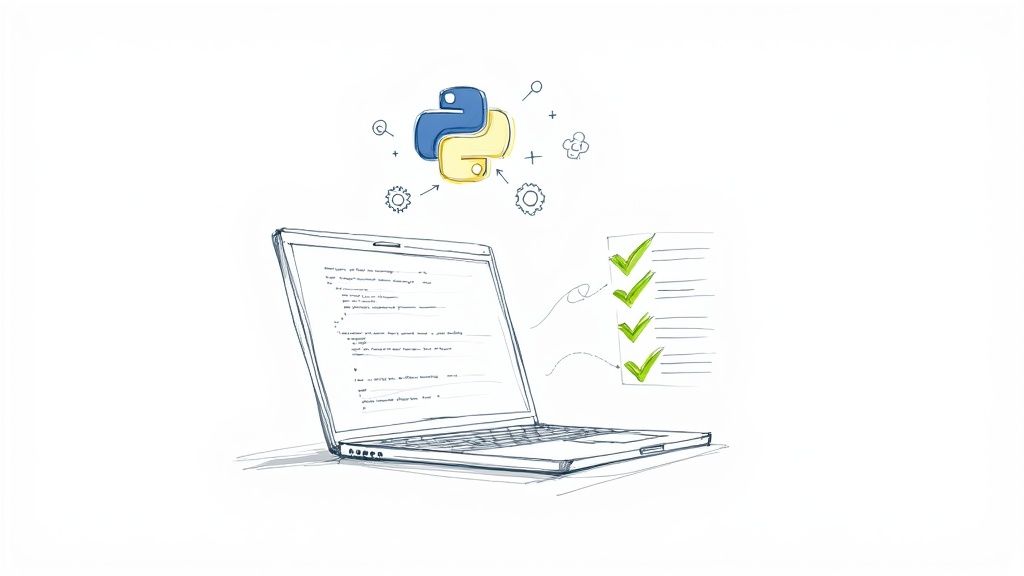
Getting Started With Using Pytest: First Steps To Success
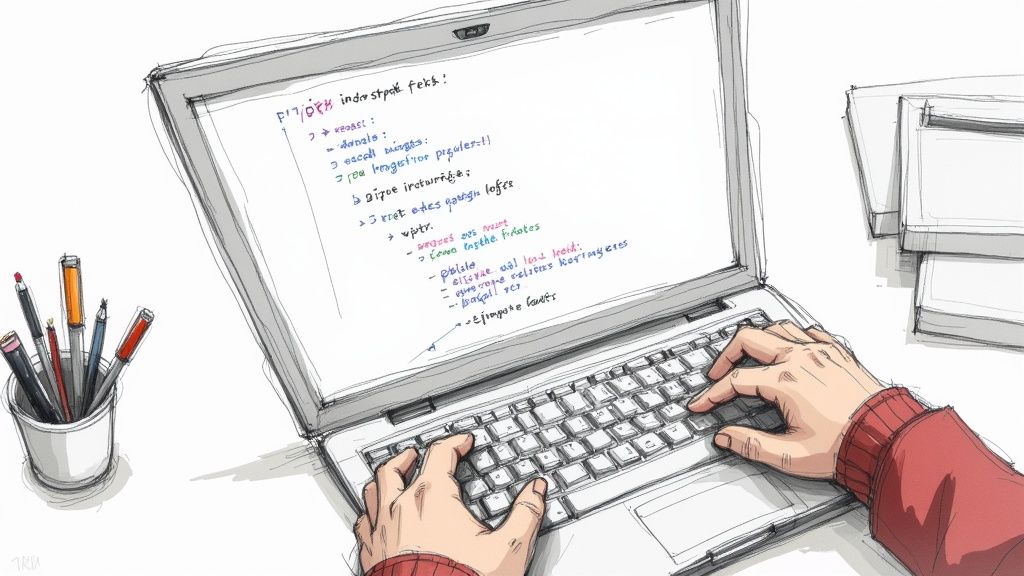
Starting your testing journey with pytest is a smooth experience, especially compared to frameworks like unittest. One of the first things you'll notice is how pytest simplifies the process. It significantly reduces the amount of boilerplate code, allowing you to write clean and concise tests.
This streamlined approach means faster test creation and less time spent on complex setups. It's a big part of why pytest is such a popular choice for Python developers.
Installation and Configuration
Installing pytest is easy. Just use pip
, Python's package installer: pip install pytest
. This simple command makes pytest readily available. Even better, the configuration is minimal. You can start writing tests almost immediately after installation.
Writing Your First Tests With Pytest
Pytest allows you to write tests in a natural and intuitive way. Its assertion capabilities simplify how you verify expected outcomes. You can use standard Python assert
statements to check for conditions without needing specialized assertion methods.
This clear syntax makes your tests easy to read and understand. It also makes pytest easier to learn, especially for developers new to testing. For a more detailed guide, check out this helpful resource: How to use pytest.
Pytest's Global Adoption
Pytest’s popularity has grown significantly. As of early 2025, over 7,300 companies in 81 countries were using it. This widespread adoption is a testament to its simple yet powerful design.
Compared to Python’s built-in unittest
framework, pytest requires far less boilerplate code. This efficiency is a major contributor to its global popularity. Pytest also boasts advanced features such as fixtures, parameterized testing, and rich assertion introspection, enabling developers to create comprehensive test suites. Plus, its extensible plugin architecture, supported by over 800 external plugins, allows it to adapt to various development needs. Learn more about pytest's rising popularity and features. This establishes pytest as a mature and globally recognized testing tool, emphasizing its commitment to integrating testing seamlessly into the development process.
Key Advantages of Using Pytest
- Simplicity: Pytest's intuitive syntax makes writing tests straightforward and minimizes boilerplate code.
- Flexibility: Its powerful features and plugin architecture adapt to various testing needs.
- Efficiency: Faster test creation and execution, combined with informative error reports, speeds up the entire testing process.
This accessibility and robustness make pytest a valuable tool for any Python developer looking to improve code quality and software reliability.
Harnessing the Power of Pytest Fixtures
Fixtures are key to writing efficient, maintainable tests with pytest. They manage the setup and teardown of resources for your tests, preventing code duplication and ensuring consistency. Think of fixtures as setting the stage for a play: each fixture arranges the necessary props and backdrops so every scene (test) begins with the right environment.
Understanding Fixture Scopes
Pytest fixtures offer various scopes, controlling how often a fixture runs. This flexibility helps optimize resource management based on your testing needs.
- Function: The default scope. The fixture is invoked for every test function, providing complete isolation between tests.
- Class: The fixture runs once per test class. Useful for setting up resources shared by tests within the same class.
- Module: Executed once per module that contains test functions. Suitable for module-level setup and teardown.
- Session: The fixture runs only once per test session. Ideal for resources that are expensive to create, such as database connections.
For a more detailed explanation, check out this helpful resource: How to master pytest fixtures
Leveraging Fixtures in Real-World Scenarios
Experienced developers use fixtures to streamline complex test scenarios. For instance, when testing a web application, you could create a fixture to set up a test database, populate it with sample data, and then tear it down after the tests finish. This prevents each individual test from repeating these steps.
Another common use case is managing connections to external dependencies, like a third-party API. A fixture can handle the connection and disconnection process, ensuring tests consistently interact with the external service in a controlled manner.
To better understand fixture usage, let's introduce a comparison table. This table outlines the different fixture scopes in pytest, highlighting when each scope is created and destroyed, along with ideal use cases.
Pytest Fixture Scopes Comparison
Scope | Creation Time | Teardown Time | Best Used For | Performance Impact |
---|---|---|---|---|
Function | Before each test | After each test | Test-specific resources, ensuring isolation | Highest overhead |
Class | Before the first test in a class | After the last test in a class | Resources shared by tests within a class | Moderate overhead |
Module | Before the first test in a module | After the last test in a module | Module-level setup, like database schemas | Lower overhead |
Session | Before the first test session | After the last test session | Expensive resources, like database connections | Lowest overhead |
As the table shows, the choice of scope significantly impacts performance. While function scope offers the most isolation, it also incurs the highest overhead. Session scope minimizes overhead but reduces isolation. Choosing the right scope is crucial for balancing performance and isolation needs.
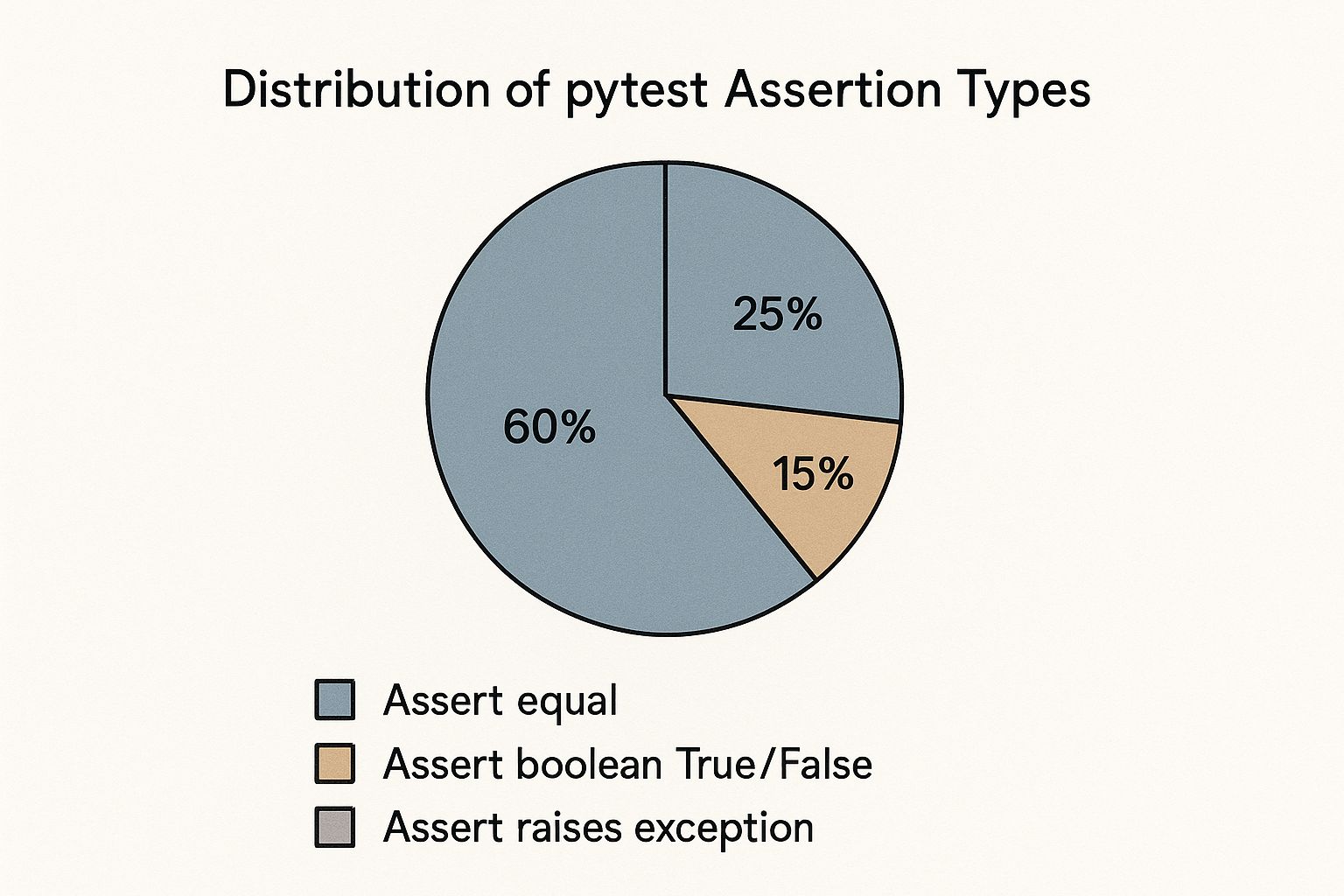
The pie chart above displays the distribution of assertion types frequently used in pytest. 60% of checks are assertions for equality, followed by 25% for boolean assertions, and 15% for verifying exceptions. This data emphasizes the importance of understanding and effectively applying different assertion methods for thorough test coverage.
Advanced Fixture Techniques
Pytest offers advanced techniques to create reusable and flexible tests.
- Parameterized Fixtures: Create multiple fixture variations by passing different parameters. This is beneficial for testing different configurations or input values.
- Factory Fixtures: Return factory functions that generate objects on demand. Particularly useful for creating unique objects for each test, further enhancing isolation.
- Fixture Composition: Combine multiple fixtures to build more complex setups, allowing you to create a hierarchy of fixtures where each is responsible for a specific part of the test environment.
By mastering these techniques, you can transform repetitive setup tasks into reusable components, improving test maintainability and reducing errors. This allows you to focus on the core logic of your tests and build more robust software.
Advanced Testing Techniques Using Pytest
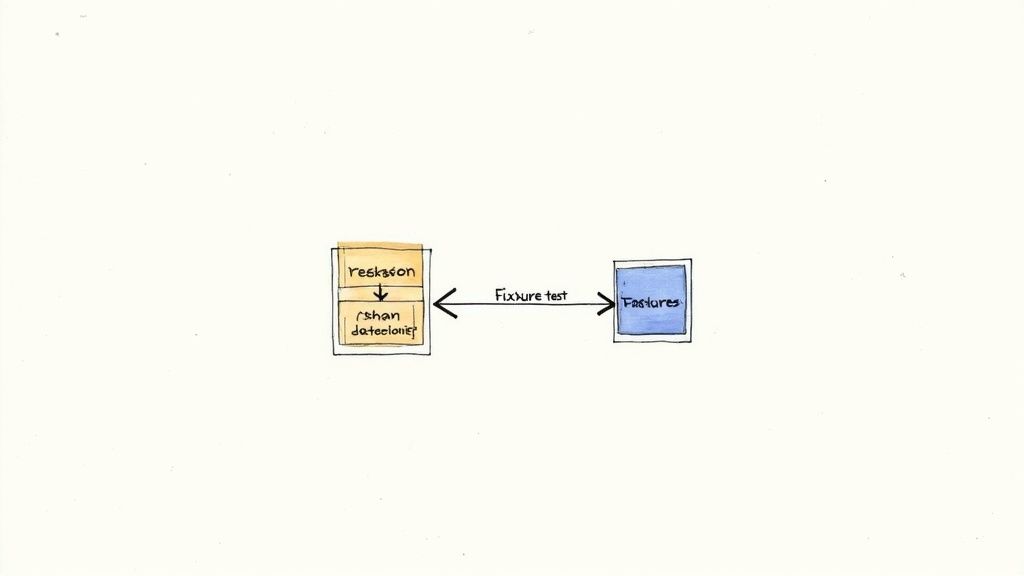
Building on the basics of Pytest, let's explore some advanced features. These techniques empower experienced developers to build incredibly robust test suites, catching those tricky bugs before they impact users. This proactive approach is essential for maintaining high-quality software.
Parameterized Testing for Increased Coverage
One of Pytest's most powerful features is parameterized testing. This lets you run the same test logic against multiple inputs, significantly increasing your test coverage without duplicating code.
Imagine testing an email validation function. Instead of separate tests for each valid and invalid format, parameterized testing allows you to provide a list of inputs and expected outputs. This not only streamlines your code but simplifies adding or modifying test cases.
Test Markers for Flexible Categorization
Test markers offer a way to categorize and select tests. You can group tests based on criteria like functionality, environment, or performance. This allows you to run specific subsets of tests during different development stages.
For example, you could mark long-running tests as "slow" and exclude them from regular runs, executing them only during nightly builds. This targeted approach optimizes your workflow and reduces feedback time.
Assertion Introspection for Effective Debugging
When tests fail, clear error messages are key for fast debugging. Pytest shines in this area with its assertion introspection. It provides detailed information about the expected and actual values, often pinpointing the problematic line of code. This detail significantly speeds up debugging.
Handling Exceptions and Asynchronous Code
Testing exception handling is crucial for robust code. Pytest provides mechanisms to verify that your functions raise the expected exceptions under specific conditions.
Asynchronous code presents unique testing challenges. Pytest offers strong support for testing asynchronous functions and coroutines, letting you reliably verify their behavior.
The widespread use of Python across different applications, from web development to machine learning, demands strong testing solutions. In fact, the global Python developer community, representing about 51% of developers worldwide as of 2025, relies heavily on test automation frameworks like Pytest. This underscores Pytest’s importance in ensuring software quality. Learn more about the global adoption of pytest.
Testing Complex Integrations
Testing individual software components is important, but testing how they interact is equally crucial. Pytest provides ways to simulate dependencies and external systems, making it easier to test complex integrations.
By mocking or stubbing external services, you can isolate the behavior of your code under test and simulate various scenarios, including errors and edge cases. This allows you to confirm your system functions correctly as a whole. By mastering these advanced Pytest techniques, you can transform testing from a chore into a powerful tool for development speed and code reliability.
Accelerating Test Performance With Pytest
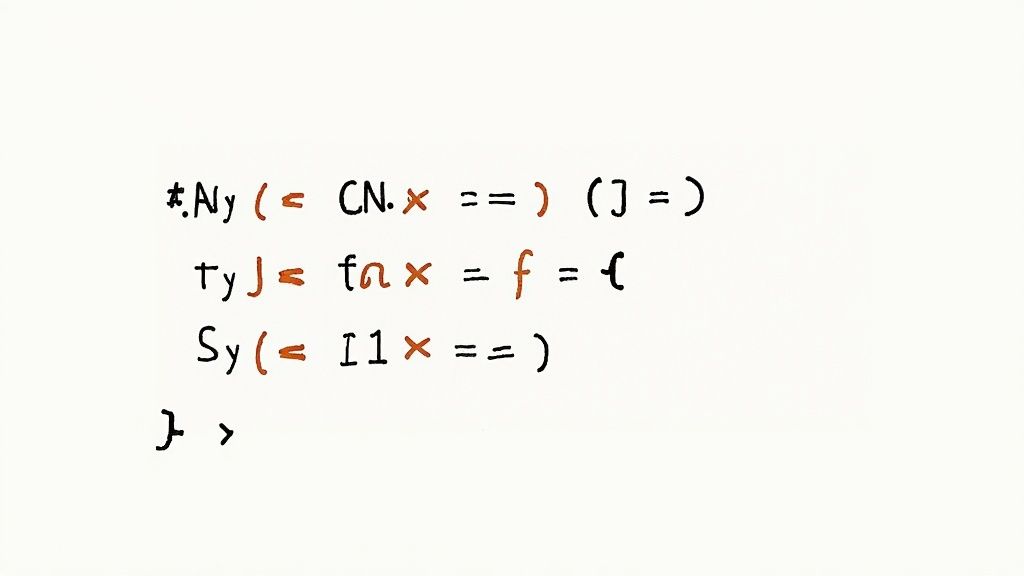
Slow tests can significantly drag down a development team's productivity. This section explores how to optimize your pytest suite for faster feedback without compromising thorough testing. Finding this balance is key for a smooth and efficient development process.
Parallel Testing With pytest-xdist
One of the most effective ways to boost your test suite's speed is parallel testing. The pytest-xdist
plugin distributes your tests across multiple CPU cores, potentially slashing execution time, especially for larger suites. Think of it as turning minutes of waiting into mere seconds.
For instance, on a four-core machine, pytest-xdist
could theoretically divide your execution time by four. This kind of performance boost can dramatically improve your workflow.
Selective Test Execution
Running your entire test suite for every single code change is often overkill. Pytest allows you to selectively execute specific tests based on different criteria. This targeted approach is crucial for a streamlined development cycle.
- Using Keywords: Tag your tests with keywords and use the
-k
option to run only those matching specific keywords. - By Test Name: Execute tests by providing their file path and name directly to pytest.
- By Node ID: Use precise node IDs to pinpoint and run individual tests within a module.
By running only the necessary tests, you get faster feedback and focus your testing efforts where they matter most, optimizing resource utilization.
Structuring Tests for Optimal Performance
The organization of your test files and the structure of your fixtures heavily influence performance. Thoughtful fixture scoping is essential to minimize overhead. This careful planning has a significant impact on overall speed.
Keep tests that share fixtures within the same module or class. This encourages fixture reuse, cutting down on setup and teardown times. Also, splitting large test modules into smaller, more focused files can improve parallelization and further accelerate the process.
Identifying and Addressing Bottlenecks
Even with parallelization and selective execution, bottlenecks can still crop up. Profiling your tests helps reveal these performance hotspots. Tools like Python's built-in cProfile
can pinpoint areas ripe for optimization. This data-driven approach to improvement is essential for peak testing efficiency.
Consider the real-world case of PyPI, the Python Package Index. PyPI relies extensively on pytest for testing. As of April 2025, its test suite, comprising 4,734 tests and achieving 100% branch coverage, executed in just 30 seconds. This is a dramatic improvement from the 161 seconds recorded in March 2024, demonstrating the power of continuous optimization. Check out this PyPI performance case study for insights into how a major project leverages pytest effectively.
By implementing these strategies, pytest becomes more than just a quality assurance tool; it becomes a driver of speed and efficiency in your development workflow. This translates to a better developer experience and faster release cycles.
Expanding Testing Capabilities With Pytest Plugins
The true power of Pytest comes from its extensibility through plugins. These plugins transform Pytest from a basic framework into a robust testing powerhouse. Let's explore how these plugins can elevate your testing strategy.
Essential Pytest Plugins for Enhanced Testing
Several key Pytest plugins are frequently used by developers to address various testing needs, improving both efficiency and quality.
pytest-cov
: This plugin integrates with Coverage.py to measure code coverage. By understanding how much of your code is tested, you can identify gaps and enhance overall quality. For more detailed information, check out this blog post: How to master pytest-cov.pytest-xdist
: As projects grow, test execution time can become a bottleneck.pytest-xdist
enables parallel testing, distributing tests across multiple CPU cores to significantly reduce testing time.pytest-mock
: Isolating tests from external dependencies is essential, and mocking plays a key role.pytest-mock
provides a simple way to create mocks and stubs, leading to more predictable and reliable tests.pytest-html
: Generating clear and visually appealing test reports is simplified with this plugin. The HTML reports detail test results, making analysis and communication more effective.pytest-rerunfailures
: Flaky tests can be a major source of frustration. This plugin automatically reruns failed tests, helping pinpoint intermittent issues and improve stability.
To help you choose the right plugins for your needs, the following table provides a quick overview of these essential tools.
Essential Pytest Plugins
Plugin Name | Primary Function | When To Use | Installation Command | Popularity |
---|---|---|---|---|
pytest-cov |
Code Coverage Analysis | Measure how much of your code is tested | pip install pytest-cov |
Very High |
pytest-xdist |
Parallel Testing | Reduce test execution time for large projects | pip install pytest-xdist |
High |
pytest-mock |
Mocking and Stubbing | Isolate tests from external dependencies | pip install pytest-mock |
Very High |
pytest-html |
HTML Report Generation | Create visually informative test reports | pip install pytest-html |
High |
pytest-rerunfailures |
Rerunning Failed Tests | Identify and address flaky tests | pip install pytest-rerunfailures |
Medium |
This table highlights the core functionality of each plugin, along with common use cases and installation commands. The popularity column provides a general indication of how widely used each plugin is within the Pytest community.
Choosing the Right Pytest Plugins
With numerous Pytest plugins available, selecting the right ones for your project requires careful consideration. Here are some key factors to evaluate:
- Functionality: Does the plugin directly address a specific testing need or challenge in your project?
- Maintenance: Is the plugin actively maintained with regular updates and bug fixes?
- Community Support: Is there a vibrant community and comprehensive documentation available for the plugin?
- Integration: Does the plugin integrate seamlessly with your existing tools and workflows?
Developing Custom Plugins
Sometimes, existing plugins may not fully satisfy your unique requirements. Pytest allows you to develop custom plugins, tailoring its functionality to your specific testing needs.
Creating a custom plugin involves writing Python code that interacts with Pytest's internal hooks. These hooks provide access to different stages of the testing process, allowing customization and the addition of new features. This advanced capability ensures Pytest can adapt to even highly specialized testing scenarios.
Integrating Plugins for Specialized Testing
Pytest plugins can effectively handle various specialized testing requirements, such as API validation, database interactions, and UI automation.
For API testing, plugins like pytest-requests
simplify making HTTP requests and validating responses. For database interactions, dedicated plugins provide fixtures and utilities for managing test databases, ensuring data integrity. Pytest can even integrate with UI testing frameworks like Selenium through specialized plugins to automate browser interactions. These integrations demonstrate Pytest's versatility in handling a wide range of testing contexts.
Integrating Pytest Into Your Development Workflow
Integrating testing seamlessly into your development process is crucial for building robust and reliable software. Using pytest effectively makes testing an integral part of your workflow, rather than a chore. Let's explore how to achieve this integration.
Organizing Your Tests
Maintaining a clear separation between test and production code is essential for project organization. A common practice is to create a dedicated tests
directory within your project's root. Inside this directory, mirror the structure of your application's source code. This mirrored structure simplifies locating tests for specific modules.
For example, if your application has a module at src/my_app/utils.py
, the corresponding test file would be at tests/src/my_app/test_utils.py
. This organized approach enhances discoverability and maintainability.
Naming Conventions and Documentation
Consistent naming conventions and thorough documentation make tests easier to understand and maintain. Prefixing test files with test_
is a standard practice that helps pytest automatically discover them. Within the test files, name your test functions descriptively to clearly communicate their purpose, such as test_function_with_valid_input
or test_function_handles_exceptions
.
Also, add docstrings to explain each test's purpose, expected behavior, and any relevant context. This practice creates self-documenting tests, reducing the need for separate documentation.
Achieving Meaningful Test Coverage
While aiming for high test coverage is good, solely focusing on arbitrary metrics can be counterproductive. Meaningful test coverage emphasizes testing the critical paths and logic of your application. Prioritize testing complex logic, edge cases, and user interactions. This strategic approach ensures that the most important parts of your application are thoroughly validated.
For more information on integrating pytest into a DevOps workflow, resources on DevOps automation tools can be helpful.
Managing Test Data and Dependencies
Effective test data and external dependency management simplifies testing and improves reliability. Use fixtures to set up and tear down test data, ensuring consistent starting conditions. For external dependencies like databases or APIs, utilize mocking or stubbing to isolate tests from these external systems. This ensures predictable test runs regardless of external factors.
Transforming Legacy Code Through Testing
Adding tests to legacy code can be challenging. A practical approach is to start by adding tests for frequently modified parts of the codebase. When making changes or adding features, write tests concurrently. This gradual process improves overall test coverage without requiring a complete rewrite.
By integrating pytest into your workflow through these approaches, you foster a culture of quality, reduce bugs, and accelerate development. Tools like Mergify can further enhance your workflow with features like Merge Queue and Merge Protections to automate pull requests and improve CI efficiency.