Testing with Ruby: Expert Insights & Techniques
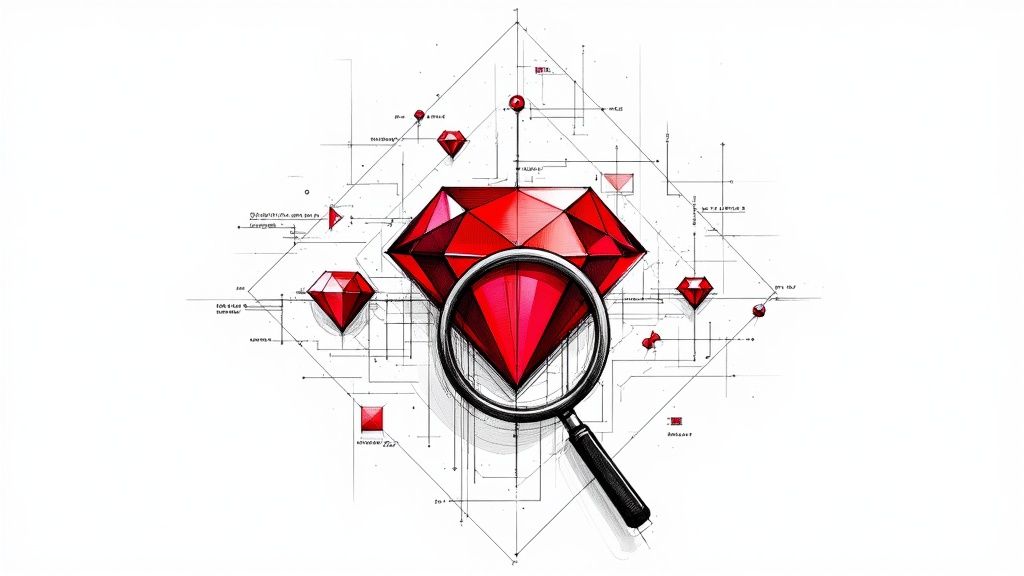
Why Ruby Excels as Your Testing Powerhouse
Choosing the right language for testing is crucial for a smooth development process. Ruby, with its elegant and readable syntax, simplifies complex tests into maintainable code. This enhanced readability promotes collaboration and reduces misinterpretations, leading to more efficient debugging and faster development cycles. The entire team can easily understand and contribute to the testing process.
This advantage streamlines the workflow and reduces the time spent on deciphering complicated test scripts.
Additionally, Ruby's dynamic nature allows developers to adopt flexible testing approaches. For example, it supports metaprogramming, enabling the creation of domain-specific testing languages tailored to your project. This flexibility is often difficult to achieve in more rigid languages, highlighting Ruby's adaptability in testing.
This means you can create tests that are not only effective but also highly expressive and easy to maintain.
The Power of Community and Ecosystem
Ruby boasts a vibrant and active community that continually contributes to a robust testing ecosystem. This supportive community fosters collaboration, knowledge sharing, and rapid problem-solving. The result is a constant stream of innovative tools and best practices.
This collaborative environment ensures that testers and developers have access to the latest resources and support.
Moreover, the availability of numerous specialized gems extends Ruby's testing capabilities. These readily available tools help developers address specific testing challenges, such as API validation and browser automation, without starting from scratch. Popular gems like rspec
and minitest
provide frameworks for writing and running tests, while others cater to specific needs like mocking or web scraping.
This rich ecosystem simplifies the testing process and allows developers to focus on writing effective tests rather than building infrastructure.
This community-driven approach to testing has led to a steady rise in Ruby's adoption in the QA world. As of recent years, Ruby 3.2 has become increasingly popular, used by 43% of developers, surpassing older versions like Ruby 2.7. This trend underscores Ruby’s continued relevance and growing adoption within the testing community. Explore this topic further
This growth demonstrates the value Ruby brings to testing teams, particularly its readability, dynamic nature, and strong community backing.
Building Intuitive and Robust Test Suites
Ruby's expressive capabilities facilitate the creation of intuitive test suites. Its clear syntax allows developers to write tests that resemble natural language, making them easier to understand and maintain. This is particularly helpful when working with complex business logic, where tests need to clearly communicate the intended behavior.
Well-written tests improve code quality and reduce the cost of future maintenance.
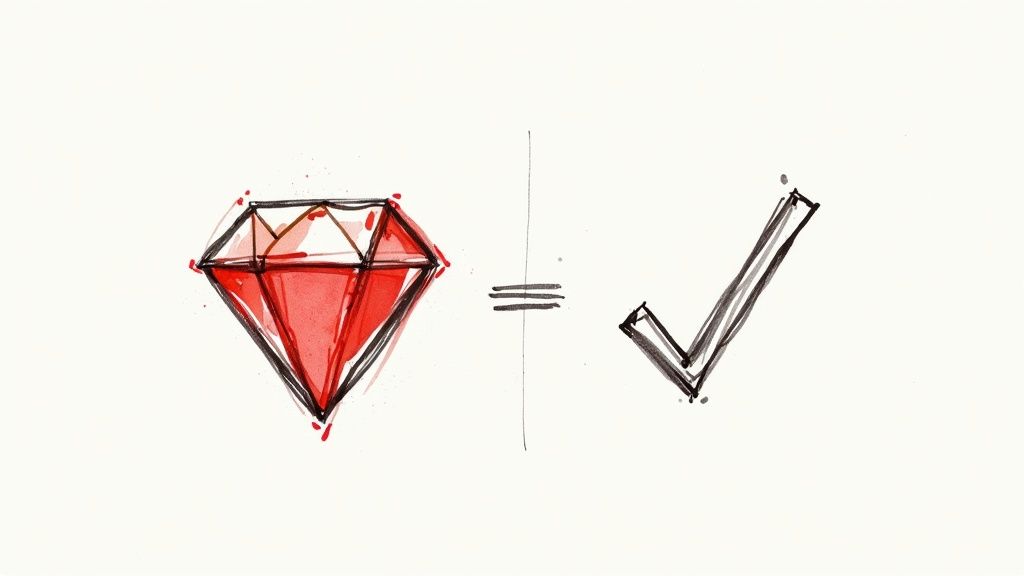
However, effective Ruby testing goes beyond simple functionality. It's about crafting tests that thoroughly document your code's behavior and design. By leveraging Ruby's strengths, you can create living documentation that evolves with your codebase and provides valuable insights for current and future team members.
This approach leads to better code quality, reduced maintenance costs, and an improved overall development experience.
Navigating Ruby's Testing Framework Landscape
Picking the right testing framework is crucial for any Ruby project. With several options available, the decision can be daunting. Understanding each framework's strengths makes the process much clearer. This section explores the leading Ruby testing frameworks: RSpec, Minitest, and Cucumber, helping you find the perfect fit.
RSpec: The Behavior-Driven Development Champion
RSpec is known for its behavior-driven development (BDD) focus. It emphasizes describing how your code should behave in a readable, almost conversational way. This approach makes tests easily understandable for both technical and non-technical team members. RSpec also encourages separating different parts of your tests, making them easier to maintain over time.
For example, an RSpec test might read: "it 'should return the sum of two numbers'". This clearly explains the expected behavior.
Minitest: The Lightweight and Pragmatic Choice
Minitest offers a leaner, minimalist testing approach. It's built into the Ruby standard library, readily available without extra installations. Minitest prioritizes speed and simplicity, ideal for performance-sensitive projects. It’s often preferred by developers who appreciate a straightforward testing process.
Minitest's compact syntax reduces the verbosity sometimes found in other frameworks. The result is concise and focused test suites.
Cucumber: Bridging the Gap Between Business and Development
Cucumber promotes collaboration through plain-language test cases. Written in Gherkin, these specifications are understandable by everyone, from developers to product owners. Cucumber connects technical implementation with business needs, ensuring everyone is on the same page.
Cucumber's collaborative approach improves communication and reduces misinterpretations. This leads to more accurate and effective testing.
Integrating Frameworks Into Your Workflow
Choosing a framework is the first step. Integrating it effectively into your workflow is equally important. Consider factors like the learning curve, performance impact, and compatibility with other tools. Many teams find a combination of frameworks works best.
To help you decide, let’s take a closer look at the differences between these three frameworks:
Ruby Testing Framework Comparison
A comparison of major Ruby testing frameworks highlighting their strengths, weaknesses, and ideal use cases
Framework | Syntax Style | Learning Curve | Best For | Community Support |
---|---|---|---|---|
RSpec | Descriptive, BDD-focused | Moderate | Large projects, BDD, complex scenarios | Large, active community |
Minitest | Simple, concise | Easy | Small to medium projects, performance-sensitive applications, quick tests | Good, part of the Ruby standard library |
Cucumber | Gherkin (plain language) | Moderate | Projects requiring strong collaboration between technical and non-technical stakeholders | Active community |
This table highlights the distinct styles, complexity, and target use cases of each framework, enabling a more informed decision based on project needs and team dynamics.
Teams using testing frameworks in Ruby often see a substantial drop in production bugs. Some statistics report a 30% reduction. This not only improves application reliability but also boosts user satisfaction. Find more detailed statistics here. This success often comes from integrating tests with CI/CD pipelines, allowing for early bug detection and faster feedback.
Choosing The Right Tool For The Job
The best Ruby testing framework depends on your project. Consider team size, project complexity, and the level of BDD or TDD adoption.
For example, RSpec might be perfect for a large team using BDD, while Minitest could suit a smaller, speed-focused project. Cucumber is valuable when clear communication between technical and business teams is paramount. Combining frameworks can create a truly comprehensive testing strategy. The right approach ultimately catches bugs before they hit production, resulting in better software and happier users.
Crafting Tests That Tell Your Code's Story
Testing with Ruby isn't simply about checking if things work; it's about creating tests that explain the purpose and design of your code. Top development teams treat their test suites as living documentation that grows with the codebase. This approach boosts maintainability and helps new team members quickly understand the project. Let's explore how to write effective and communicative tests in Ruby.
Naming Conventions That Speak Volumes
Good test names clearly state the purpose of each test. Instead of a vague name like test_method_x
, opt for a descriptive name such as test_method_x_returns_correct_value_when_input_is_positive
. This makes it easy to understand what each test verifies, even without looking at the code. This detailed naming turns your test suite into a valuable resource for understanding your code's behavior.
Additionally, organizing tests logically with describes and contexts helps group related tests, improving the overall structure. This organization reflects your application's structure, making it easy to find tests for specific modules or features.
Writing Assertions That Provide Meaningful Feedback
Assertions should offer clear feedback when tests fail. Instead of a simple assert result
, be specific: assert_equal expected_value, result
. When a test fails with a specific assertion, debugging is much easier. It pinpoints the exact difference between expected and actual results, reducing troubleshooting time and making testing more efficient.
Refactoring Tests for Clarity and Maintainability
Refactoring isn't just for production code; it applies to tests as well. Consider this before-and-after example:
Before:
def test_complicated_logic
Several lines of setup and calculations
assert result < 10
end
After:
def test_complicated_logic_returns_value_less_than_10
setup_data
result = perform_calculation
assert_operator result, :<, 10
end
The refactored version separates setup and calculation logic into methods, making the test's purpose clear. The assert_operator
provides better context than just <
. This clarity improves understanding and simplifies future maintenance.
Managing Test Data and Prerequisites
Managing test data well is crucial for test reliability and independence. Ruby allows you to use factories or fixtures to create consistent test data. This ensures that each test begins with a known state and avoids unwanted side effects between tests. Isolating tests in this way helps pinpoint issues when a test fails without interference from previous tests. This isolation is key for reliable testing.
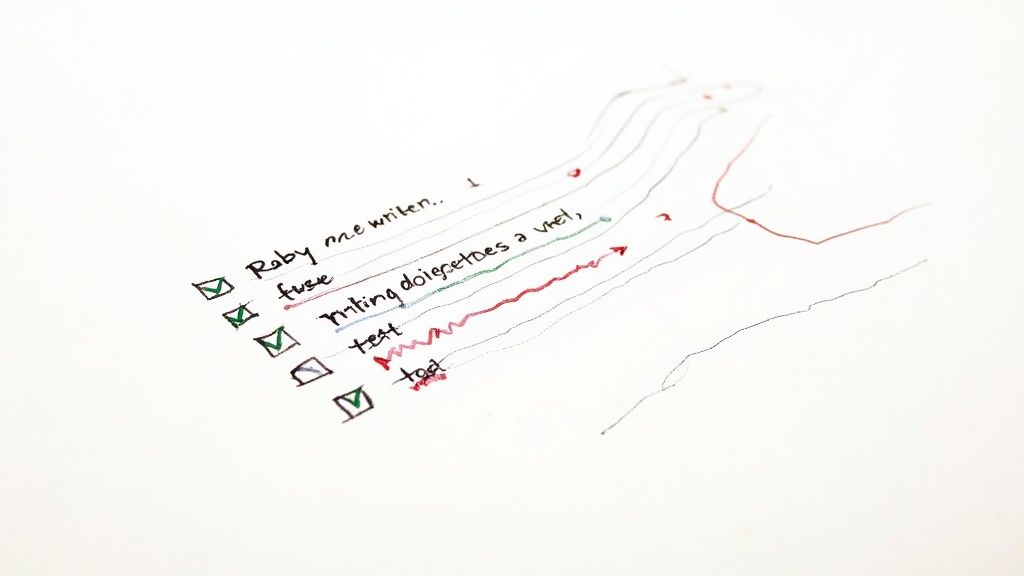
By following these practices, you transform tests from simple checks into valuable documentation that improves understanding, simplifies maintenance, and creates a more robust codebase. This thoughtful approach to testing in Ruby ultimately raises the quality of your software.
Optimizing Ruby Applications Through Performance Testing
Performance problems can severely impact even the most polished Ruby applications. This section explores how effective teams proactively identify and address these bottlenecks before they affect users. Through performance testing, development teams can ensure a smooth and responsive user experience.
Benchmarking: Establishing Performance Baselines
Benchmarking is fundamental to understanding your application's performance profile. Establishing clear baselines provides a crucial reference point for measuring the impact of future code changes. This helps you track performance improvements and quickly identify regressions.
For example, measuring the average response time of critical API endpoints establishes a starting point for optimization efforts. By setting realistic thresholds based on these baselines, you can prevent performance issues from escalating and impacting your users.
This targeted approach allows you to focus your optimization efforts where they'll have the greatest impact. Remember to consider factors like expected user load and system capacity when establishing benchmarks to ensure they reflect real-world conditions.
Interpreting Performance Metrics in Context
Raw performance metrics can be misleading without proper context. Consider factors like network latency, database performance, and external dependencies when analyzing your results. This holistic view prevents premature optimization by focusing on genuine bottlenecks, not just surface-level symptoms.
By understanding the bigger picture, you can target the root cause of performance issues, leading to more effective and long-lasting solutions.
Tools and Techniques for Performance Testing With Ruby
Several tools integrate seamlessly with Ruby, empowering developers to measure and improve application performance. The following table provides a breakdown of popular options:
To help you choose the right tool for your needs, we've compiled a table summarizing the key features and use cases of popular Ruby performance testing tools.
Ruby Performance Testing Tools Overview of popular Ruby performance testing tools with their key features and use cases
Tool | Primary Focus | Learning Curve | Integration Options | Best For |
---|---|---|---|---|
benchmark |
Micro-benchmarks, comparing code snippets | Easy | Built-in Ruby library | Isolating performance differences in small code blocks |
rspec-benchmark |
Benchmarking within RSpec tests | Easy | Integrates with RSpec | Combining performance testing with functional tests |
Benchmark::Trend |
Tracking performance trends over time | Moderate | Standalone gem | Identifying performance regressions and scaling issues |
ruby-prof |
Profiling code execution | Moderate | Standalone gem | Identifying performance bottlenecks and hotspots |
This table offers a quick overview of each tool's strengths, making it easier to select the one that best fits your specific project requirements.
These tools allow you to measure execution times, pinpoint bottlenecks, and understand how your Ruby code performs under various loads. This data informs optimization decisions and helps you achieve the desired performance outcomes. Tools like Benchmark::Trend
are particularly useful for assessing computational complexity. By running code with increasingly large inputs and measuring execution times, Benchmark::Trend
can help predict how your code will scale as your application grows. Discover more insights about Benchmark::Trend here. This proactive approach is crucial for identifying potential scaling issues early on.
Balancing Performance and Code Readability
While performance is paramount, it shouldn't come at the expense of readable code. Maintaining a balance between optimization and clean code is vital for long-term maintainability. Prioritize clear, concise code that's easy to understand and modify, even after optimizations have been applied.
This balanced approach ensures your application remains both performant and maintainable. By integrating performance testing into your workflow, you can effectively address performance issues while writing easy-to-understand Ruby code.
Supercharging Your Tests With Ruby's Gem Ecosystem
Why reinvent the wheel? Ruby's rich gem ecosystem offers a treasure trove of tools to enhance your testing process. Successful development teams frequently use these specialized gems to handle complex scenarios, from API validation to automating interactions within web browsers. This frees them to concentrate on crafting effective tests rather than building the underlying infrastructure, improving efficiency and ensuring tests stay current with evolving project needs.
Evaluating Gems for Production Use
Selecting the right gem requires thoughtful consideration. Production readiness, integration compatibility, and long-term maintenance are key factors for informed decision-making. Seek out gems with active communities, thorough documentation, and a consistent history of updates. This due diligence ensures the gem aligns with your project's long-term objectives.
Also, assess how well a gem integrates with your existing testing framework and other project dependencies.
For example, if your team uses RSpec, a gem that meshes well with it would likely be a better fit. This seamless integration can save considerable time and effort when setting up your testing environment.
Combining Gems for Cohesive Testing Solutions
The optimal approach often involves using several gems together. However, it's crucial to avoid dependency conflicts. Carefully plan how your chosen gems will interact and test thoroughly to ensure they don't interfere with one another. This collaborative integration can create a more robust and complete testing strategy than relying on a single gem. By managing your testing environment like any other part of your application's codebase, you maintain control and prevent unforeseen issues.
This means you can capitalize on the strengths of multiple tools without compromising stability, ultimately streamlining your process and significantly improving test coverage while simplifying maintenance.
Real-World Success Stories and Cautionary Tales
Many teams have dramatically increased test coverage and decreased code maintenance through the strategic use of gems. However, there are situations where custom solutions are more appropriate. For instance, if a gem doesn't completely meet your specific requirements or introduces excessive overhead, developing your own tool might be a better option. This targeted approach allows you to tailor solutions precisely to your needs.
Ruby's gem ecosystem has seen explosive growth. Downloads have increased significantly since 2019, rising from about 3.71 billion in July 2019 to over 137.53 billion by July 2023. This represents a surge of roughly 133.82 billion downloads in four years, compared to slower growth in prior years. This growth correlates with the increasing adoption of CI/CD pipelines and Ruby's rising popularity. Learn more about Ruby gem download trends here. Daily downloads have also more than doubled, from approximately 50 million in 2019 to over 127 million by 2023.
This highlights the expanding role of gems in Ruby development, especially in testing. By understanding when to use existing gems and when to build your own, you can maximize the efficiency and effectiveness of your Ruby testing efforts. This strategic approach contributes to a smoother development process and higher-quality code.
Mastering Test-Driven Development in Ruby
Test-Driven Development (TDD) is more than just a testing method; it's a design philosophy. Effective TDD leads to more modular, maintainable, and robust Ruby code. This section explores the practical application of TDD in a Ruby development workflow.
The Red-Green-Refactor Cycle in Ruby
At the heart of TDD is the red-green-refactor cycle. This iterative process begins with a failing test (red), followed by writing the minimum code necessary to pass the test (green). The final step is refactoring to improve code quality while keeping the test passing (refactor). Ruby's elegant syntax makes each phase of this cycle more intuitive.
Let's say you're creating a ShoppingCart
class. You'd start with a test asserting that the total
method returns 0 for an empty cart. Initially, this test would fail (red). You would then implement the total
method, ensuring it returns 0 for an empty cart (green). Finally, you could refactor the total
method for clarity and efficiency, while ensuring the test continues to pass.
Overcoming Common TDD Obstacles
While TDD offers numerous advantages, developers sometimes encounter challenges. Managing external dependencies, testing edge cases, and navigating complex object relationships can feel overwhelming. Fortunately, Ruby provides tools and techniques to address these issues.
- Managing External Dependencies: Mocking libraries, such as Mocha or RSpec Mocks, help isolate your code from external systems during testing. This improves both the speed and reliability of your tests.
- Testing Edge Cases: Ruby's flexible syntax simplifies the creation of parameterized tests, allowing you to cover a wide range of input values, including those tricky edge cases.
- Complex Object Relationships: Test setup can become complicated when dealing with interconnected objects. Factories, like Factory Bot, streamline the creation of test objects with predefined relationships, simplifying test preparation.
TDD and Design Decisions in Ruby
TDD has a significant impact on design. By prioritizing tests, you naturally design more modular and testable code. This approach promotes smaller, more focused methods and classes, which contributes to a cleaner, more maintainable codebase.
For example, when writing tests for a complex calculation, TDD might lead you to break down the logic into smaller, individually testable units. The result is more reusable and easier to understand code. This inherent modularity stems directly from the TDD process.
Practical Strategies for Existing Codebases
Integrating TDD into an existing project can be a challenge. A good strategy is to start with critical or frequently modified modules. Focus on writing tests for new features and bug fixes, gradually increasing test coverage over time. This incremental approach prevents overwhelming your team and demonstrates the benefits of TDD organically.
Tools like SimpleCov can help track test coverage. They provide valuable insights into areas of your codebase that need more attention. Focusing on these areas maximizes the impact on your code's stability and reliability. While 100% test coverage can be a goal, it's not always practical. Prioritize testing critical paths and complex logic.
TDD, when embraced effectively, transforms testing from a chore into a powerful design tool. By utilizing the resources within the Ruby ecosystem, developers can adopt TDD to create more robust, maintainable, and well-designed applications. This not only saves time and resources but also enhances user experience. It fosters a deeper understanding of the code's behavior and encourages a more proactive and confident approach to development through a continuous feedback loop between tests and implementation.
Elevating Your Testing With Advanced Ruby Patterns
As your Ruby applications grow, basic testing might not be enough. This section explores advanced patterns used by seasoned Ruby developers to handle tricky situations like testing concurrency, state machines, and event-driven systems. These areas often pose challenges for traditional testing methods.
Custom Matchers: Enhancing Test Readability
Custom matchers in RSpec boost the clarity and expressiveness of your tests. They let you package complex assertions into reusable parts, making your test code simpler and its purpose clearer. For example, if you frequently check email address formats in your tests, a custom matcher can handle this logic. This replaces multiple assertions with a single, descriptive matcher. This not only reduces redundancy but also improves understanding.
This enhanced readability makes grasping the purpose of each test easier, especially when dealing with complex logic.
Shared Contexts: Eliminating Redundancy
When multiple tests require similar setup steps, shared contexts in RSpec help avoid redundant code. Grouping common setup actions into a shared context lets you include it in multiple test suites, supporting DRY (Don't Repeat Yourself) principles. This simplifies test maintenance. For instance, if several tests need a logged-in user, the login steps can reside in a shared context and be included as needed. This keeps tests concise and avoids repetition.
Test Composition: Building Complex Tests from Simpler Ones
Test composition enables creating complex tests by combining simpler, focused tests. This technique increases code reusability and makes managing large test suites easier. Imagine a scenario with multiple sequential steps: each step can be its own test. A higher-level test can then combine these to verify the entire flow.
This modularity simplifies debugging and helps pinpoint issues more effectively. By breaking complex scenarios into smaller, testable units, test composition makes development and upkeep easier.
Testing Time-Dependent Behavior
Testing time-dependent code can be challenging. Ruby offers tools like Timecop for controlling time flow in your tests. This allows you to simulate specific time-based situations, such as scheduled tasks or timeouts. These tools are crucial for effectively testing time-sensitive application aspects.
Strategies for Complex Validations and Business Logic
Thorough testing of intricate business rules and validations requires careful planning. Techniques like data-driven testing help cover a wide range of input values and ensure your code performs correctly in different situations. Separating test data from test logic makes adding new test cases easy without altering code. This flexibility expands coverage and reduces error risk.
Decision Frameworks for Applying Advanced Patterns
While these patterns are powerful tools for Ruby testing, use them wisely. Overusing complex patterns can lead to tests that are hard to understand and maintain. Consider the code's complexity and the benefits of using a more advanced pattern. If a simpler approach suffices, choose that. Balance test effectiveness and maintainability for a healthy development cycle.
For example, a basic unit test might suffice for a simple method with few edge cases, rather than a complex data-driven test. However, critical components with complex business logic might require more advanced patterns to ensure comprehensive coverage and robustness.
Ready to streamline your merging process and improve your CI workflow? Check out Mergify today! Discover how Mergify can help your team.