Master Testing Ruby: Boost Your Code Quality
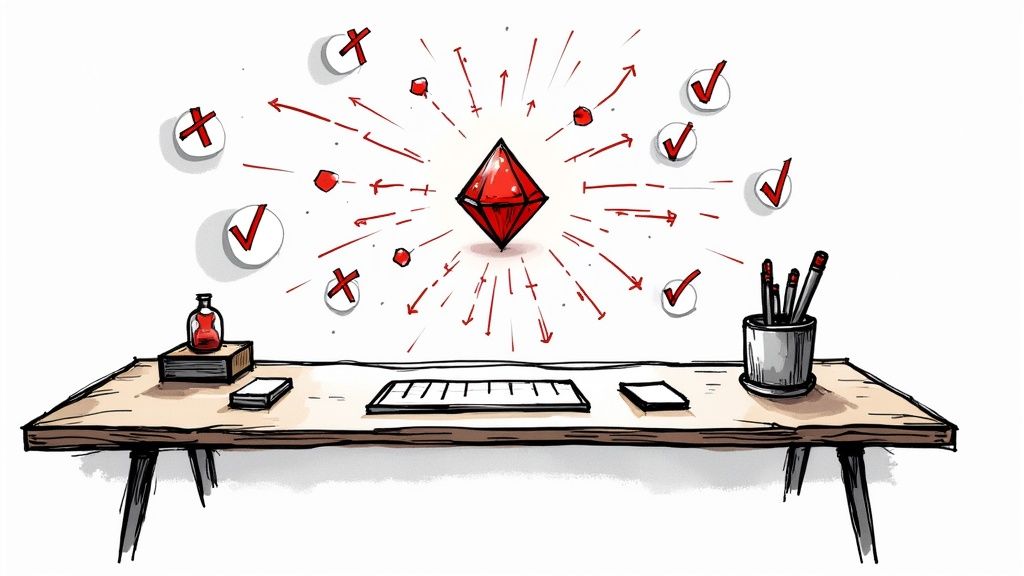
Why Testing Ruby Is Your Secret Weapon
Many developers view testing as an added task, something that slows down their progress. However, testing is much more than a simple checkbox on a to-do list. It's a vital tool that transforms a Ruby codebase from uncertain and fragile to dependable and robust. Think of it as the foundation of a house: essential for stability and future expansion.
Testing provides the structure needed to build with confidence. This confidence allows for bold refactoring and changes without the constant worry of introducing bugs. With a comprehensive suite of tests, you're free to explore new ideas and enhance your code. Plus, thorough testing prevents costly issues in production.
The Advantages of a Robust Test Suite
How does testing accelerate development? Simply put, the longer a bug remains hidden, the more expensive it becomes to fix. A minor oversight can quickly escalate into a major problem, requiring significant debugging and rework. A robust test suite acts as an early warning system, catching these issues before they spiral out of control. This saves valuable development time.
Well-written tests also act as live documentation for your code. They clearly explain the intended function of your application, which helps new team members understand the codebase and contribute more quickly. This shared understanding improves team productivity and reduces miscommunication. Ruby, known for its flexibility and dynamic nature, particularly benefits from the clarity that testing provides. As applications grow, the initial investment in testing pays off exponentially.
Ruby has a long history of evolving testing practices, utilizing robust frameworks. The language boasts a rich ecosystem of tools, and testing is a prime example. Ruby developers heavily rely on unit testing frameworks to guarantee code quality. This focus on testing is a key factor in the ongoing success of Ruby applications. Even with the growing popularity of type specification tools, many Ruby developers still prioritize thorough testing to maintain code integrity. Explore this topic further.
Making Testing Work For You
Effective testing is achievable even for small teams. It can scale seamlessly as projects expand. Starting with a core set of unit tests and gradually adding integration and system tests can provide extensive coverage. Testing doesn't have to be an all-or-nothing endeavor. Even a small effort can dramatically improve code quality. Focus on the most crucial aspects of your application and use appropriate tools to build a successful testing strategy.
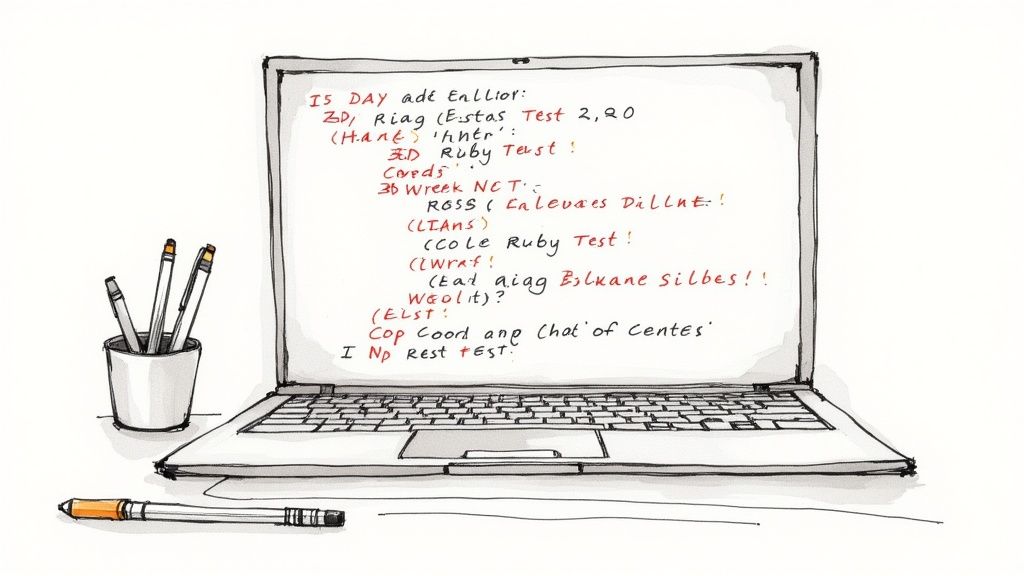
By embracing testing as a valuable resource, you can unlock its full power. Stop thinking of it as a burden and start using it as the secret weapon it is. The next section will explore specific testing tools in the Ruby ecosystem, helping you create the ideal testing toolkit for your projects.
Choosing The Right Ruby Testing Framework
Building a reliable testing strategy for your Ruby applications means picking the right tools. This involves understanding each testing framework’s strengths and how they can work together. Let’s explore the key players: MiniTest, RSpec, Cucumber, and Test::Unit.
MiniTest: Simplicity and Speed
MiniTest, included with Ruby, prioritizes simplicity and speed, making it great for quick, targeted tests. It uses a direct assertion-based approach. For example, assert_equal(expected, actual)
checks for matching values. This simplicity leads to faster test execution.
MiniTest's lightweight nature also reduces overhead. It's ideal for testing individual methods or classes. However, for complex scenarios, it might lack the expressiveness of other frameworks. So, MiniTest shines when speed and ease of use are paramount.
RSpec: Behavior-Driven Development
RSpec, in contrast, focuses on behavior-driven development (BDD). This promotes collaboration between developers, testers, and business stakeholders. RSpec uses a domain-specific language (DSL) that resembles natural language.
For instance, expect(user.name).to eq("Alice")
clearly expresses expected behavior. This improves communication and ensures everyone grasps the test’s purpose. However, RSpec's DSL adds complexity, potentially steepening the learning curve. Its behavior focus can sometimes obscure implementation details. Still, RSpec excels when collaboration and clear communication are crucial.
Cucumber: Bridging the Communication Gap
Cucumber takes BDD further, using plain-text scenarios to define acceptance criteria. These scenarios bridge the gap between technical and non-technical stakeholders, describing the system's behavior from a user's perspective. Cucumber links these scenarios to underlying Ruby code to execute tests.
This high-level approach simplifies communication and clarifies expectations. But, Cucumber can become cumbersome for low-level testing. Its strength is in defining and verifying complete user flows, not individual methods.
Test::Unit: The Default Option
Test::Unit, also included with Ruby, is a classic xUnit-style framework, providing a structured approach to unit testing. It offers various assertions and setup/teardown methods.
While lacking RSpec’s elegance or Cucumber’s high-level perspective, it’s a reliable foundation for thorough tests. Test::Unit is a practical choice for teams comfortable with traditional testing styles.
Combining Frameworks for Comprehensive Coverage
The best approach often involves combining frameworks. For instance, use MiniTest for rapid unit tests and RSpec for behavior-driven tests of more complex interactions. Then, add Cucumber to define and verify user acceptance criteria. This strategy provides broad coverage and leverages each framework’s strengths.
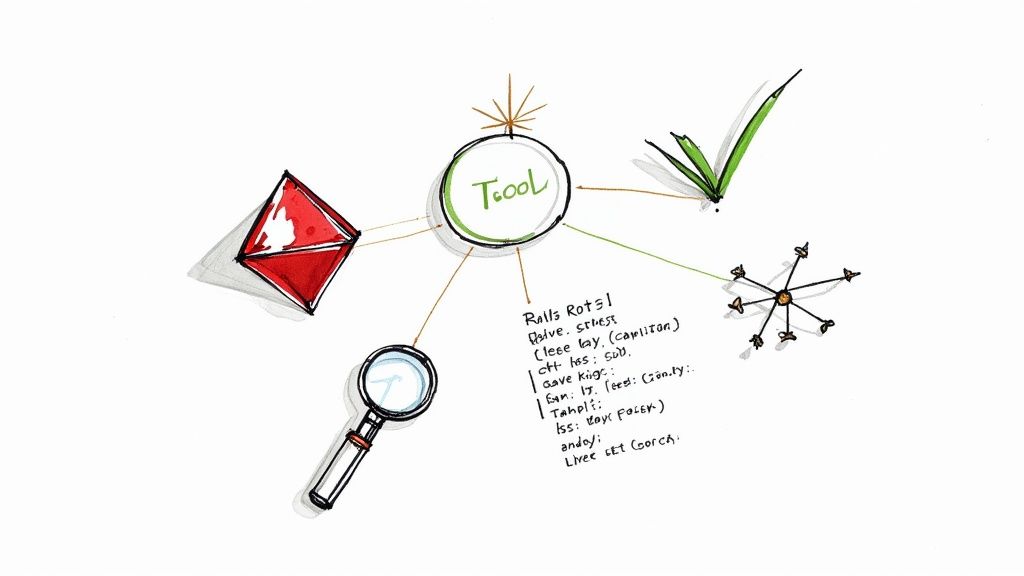
To help you choose the right framework for your needs, let's look at a comparison of their key features:
Ruby Testing Framework Comparison: A detailed comparison of the most popular Ruby testing frameworks, highlighting their key features, pros, cons, and ideal use cases.
Framework | Testing Style | Syntax Complexity | Learning Curve | Best For |
---|---|---|---|---|
MiniTest | Assertion-based | Simple | Easy | Fast, focused unit tests |
RSpec | Behavior-driven (BDD) | Moderate | Moderate | Collaboration, clear specifications |
Cucumber | Acceptance testing (BDD) | Simple (Gherkin) | Moderate | User acceptance criteria, high-level behavior |
Test::Unit | xUnit style | Simple | Easy | Traditional unit testing |
As you can see, each framework caters to different testing needs and styles. MiniTest shines in its simplicity and speed, while RSpec facilitates collaboration. Cucumber excels at bridging the communication gap between technical and non-technical team members. Test::Unit remains a solid choice for those comfortable with traditional unit testing approaches.
Choosing The Best Tool For Your Team
Selecting the best tool depends on your project and team culture. A small team on a fast-paced project might prefer MiniTest’s simplicity. A larger team working closely with business analysts might favor RSpec or Cucumber. Check out our article on How to master your sitemap for additional insights. Consider your team’s experience, project size, and testing goals. The right combination will empower you to build reliable and maintainable Ruby applications. Successfully testing Ruby applications becomes more attainable when your tools are tailored to your needs.
Unit Testing Ruby: Catching Real Bugs
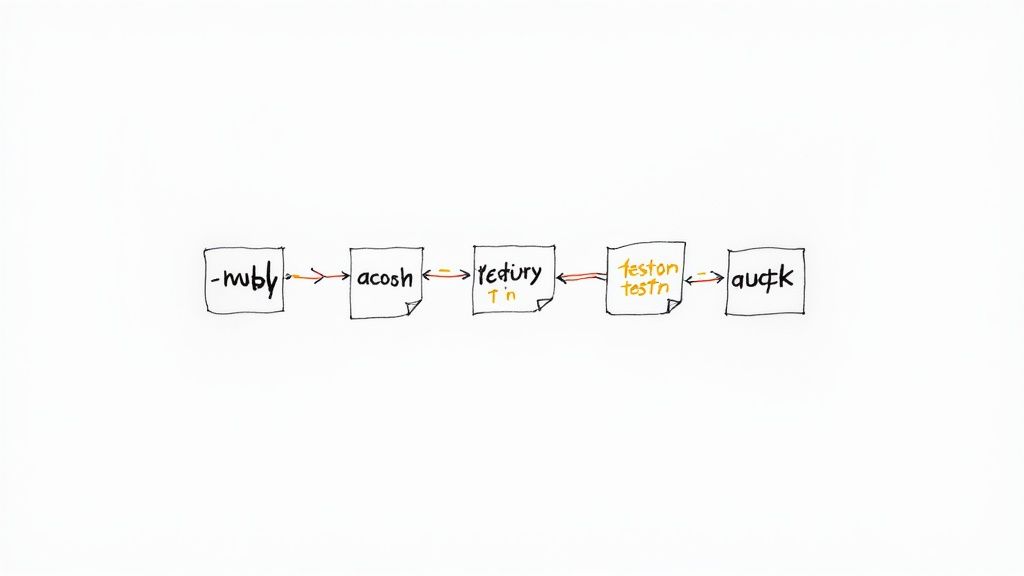
Truly effective Ruby unit tests go beyond simple assertions. Instead of just confirming expected values, they should proactively uncover hidden problems before they affect users. This involves strategically using stubs and mocks, especially when dealing with external dependencies.
Stubbing and Mocking: Isolating Your Code
Stubbing and mocking are essential techniques. They isolate the code being tested by simulating the behavior of its dependencies. For instance, if your method depends on an external API call, stubbing lets you return a predefined response. This ensures consistent test results. Mocking, on the other hand, verifies specific interactions with dependencies, confirming they occurred as expected.
Overusing mocks, however, can lead to brittle tests tightly coupled to implementation. The key is balance. Use stubs and mocks judiciously to isolate units effectively while still allowing for future code changes. Focus on testing behavior, not just implementation.
Testing the Edges: Beyond the Happy Path
Effective tests explore edge cases and boundary conditions. Test with empty inputs, invalid data, and extreme values. These scenarios often reveal hidden bugs that might otherwise go unnoticed.
Consider real-world scenarios, too. Mimic the data and interactions your code will encounter in production. This ensures your tests reflect actual usage and catch problems that simpler test cases might miss.
Maintaining Speed: Efficient Test Suites
As your test suite grows, maintaining speed is crucial. Slow tests discourage frequent testing and can hinder rapid development. Employ techniques like test caching and parallelization to boost performance. Ruby's emphasis on agility, exemplified by tools like Ruby on Rails, has always prioritized speed. This philosophy significantly influences Ruby testing best practices. Learn more about Ruby's history.
Minimize dependencies within your tests. Each dependency adds overhead. If a test doesn't truly need a specific component, stubbing or mocking can reduce execution time.
Avoiding Common Pitfalls: Writing Meaningful Tests
Many developers fall into common testing traps. One mistake is writing tests that only verify obvious behavior, offering little protection against regressions. Another is neglecting error handling. Your tests must cover what happens when things go wrong.
By avoiding these pitfalls and implementing the strategies outlined here, your unit tests become a valuable asset. They will catch real bugs, enhance code quality, and ultimately accelerate your development process.
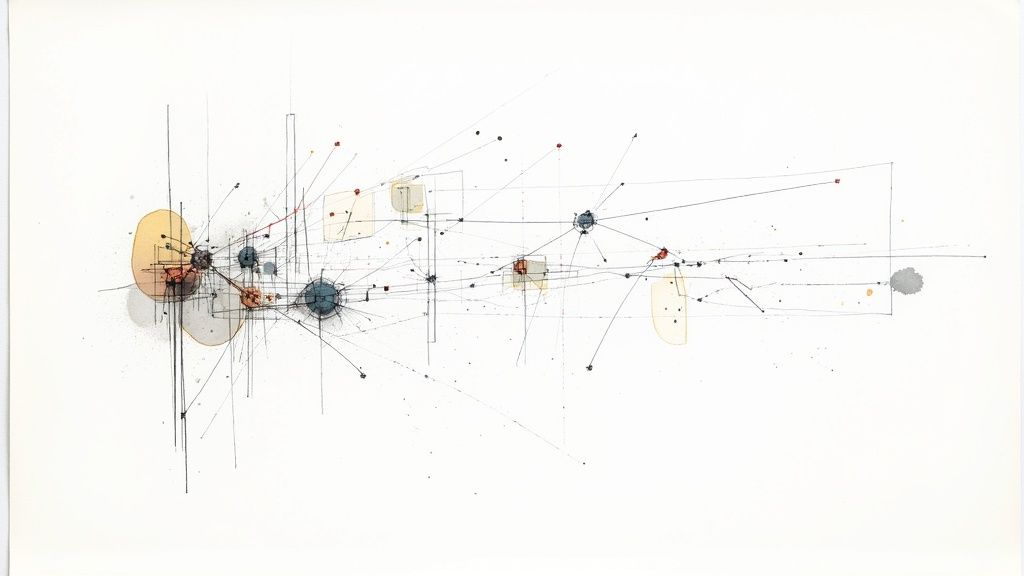
Unit tests are great for checking individual parts of your code. But to see how everything works together, you need integration tests. These tests show you how your Ruby application behaves in a real-world setting.
They ensure that all the pieces, from database interactions and API calls to connections with external services, function smoothly as a whole. This layered testing approach is crucial for finding hidden problems that unit tests might miss.
Managing Test Databases: Keeping Your Data Clean
Integration tests often involve databases. For reliable results, you need to manage these test databases effectively.
A few good practices can make a big difference. Using a separate test database instance, or using tools to reset the database to a known state before each test, can greatly improve the stability of your integration tests. This prevents leftover data from interfering with your test runs.
Realistic Test Scenarios: Mirroring Real-World Use
Creating realistic test scenarios is also crucial. Consider how users will actually interact with your application in production. Design your integration tests to mimic those interactions.
For example, if you're testing an API endpoint, send it the kinds of requests real users would send. This includes different input parameters, boundary conditions, and even error scenarios. You might find this resource helpful: How to master sitemaps related to blog authors.
Controlling Test Data Complexity: Finding The Right Balance
As your application grows, managing your test data can become a real challenge. Too much data can slow down your tests, while too little might not provide a realistic picture.
Finding the right balance is the key. Using factories to generate realistic data, or focusing on specific data subsets for certain tests, can help you manage this complexity. Efficient execution and thorough coverage are important priorities for successful Ruby teams. They value quick feedback to catch integration issues early. They also avoid overly complex tests that become hard to maintain and debug.
Avoiding Flaky Tests: Ensuring Reliable Results
Flaky tests, those that pass sometimes and fail others without code changes, can be a real headache. They erode confidence in your test suite and make it tough to identify real problems.
Properly managing test dependencies, having clear setup and teardown procedures, and minimizing external influences can help you avoid flaky tests.
Ruby maintains a consistent presence in web development. Despite a market share of 0.98% among programming languages, roughly 6.2% of websites still use Ruby on the server-side, proving its enduring value. For more detailed statistics, look here.
Structuring Test Suites: Locating Failures Quickly
The structure of your test suite impacts how quickly you can find and fix failures. Organizing tests logically by functionality or component makes it easier to pinpoint issues. Clear naming conventions and descriptive error messages also help with debugging.
Prioritizing maintainability and clarity creates a test suite that benefits your team in the long run. This approach ensures your integration tests accurately reflect how your Ruby application will perform in the real world.
Testing Ruby on Rails: Beyond the Basics
Testing a Rails application goes beyond basic Ruby testing. It requires a deep understanding of Rails-specific tools and their potential pitfalls. Let's explore some key aspects of effective Rails testing.
Speeding Up Your Rails Test Suite
Slow test suites are a common pain point in Rails development. As your application grows, slow tests can significantly hinder your development speed.
Fortunately, there are ways to combat this. Optimizing database interactions within your tests is crucial. Using fixtures or factories strategically can help minimize database queries. Leveraging caching mechanisms can also reduce redundant computations during test runs.
- Optimize Database Interactions
- Use Fixtures or Factories Judiciously
- Leverage Caching Mechanisms
Another effective strategy is parallelizing your tests. This distributes the workload across multiple cores or machines, drastically reducing execution time. Keeping your feedback loop rapid allows you to catch errors quickly and stay productive. For more insights on related topics, check out this article: How to master sitemaps related to blog posts.
Testing Models, Controllers, and Views Effectively
Testing Rails components requires a strategic approach. Avoid tight coupling between your tests and the underlying implementation. This makes your tests brittle and prone to breaking with minor code changes.
Focus on testing the behavior of your components. For models, ensure data integrity and proper database interactions. For controllers, verify they handle requests correctly and return expected responses. For views, focus on the rendered output and user interactions, not the internal rendering mechanics.
Fixtures vs. Factories: Choosing the Right Approach
Fixtures and factories are two approaches to managing test data. Fixtures define data statically in YAML files, suitable for simple scenarios. Factories generate data programmatically, offering more flexibility and customization.
To help illustrate the differences, let’s look at the following table:
Rails Testing Types and Tools
This table provides an overview of the differences between Fixtures and Factories in Rails testing:
Testing Type | Tools/Libraries | What to Test | Common Pitfalls |
---|---|---|---|
Model Testing with Fixtures | Fixtures (YAML files) | Data integrity, relationships, validations | Can become difficult to maintain for complex data |
Model Testing with Factories | FactoryBot | Data integrity, relationships, validations | Overly complex factories can obscure test logic |
Controller Testing | RSpec, Minitest | Request handling, response codes, redirects | Testing implementation details instead of behavior |
View Testing | RSpec, Minitest, Capybara | Rendered HTML, user interactions | Brittle tests tied to specific HTML structure |
System Testing | Capybara, Selenium | End-to-end user flows, JavaScript interactions | Slow test execution, browser compatibility issues |
Key takeaway: Factories are generally better for larger projects due to their flexibility and maintainability. Fixtures might suffice for smaller projects with simpler data needs.
System Tests and the Development Lifecycle
System tests, also known as end-to-end tests, verify the entire user interaction flow. These tests are crucial for catching critical issues that might not be apparent during unit or integration testing.
System tests simulate real user behavior, interacting with your application through the browser or API. Integrating them into your development lifecycle ensures high quality and confidence in your application's functionality. This is especially important for Rails applications where user experience is paramount.
Maintaining testing discipline throughout the development process – from initial development to ongoing maintenance – is vital for preventing regressions and delivering a robust product. Consistent testing practices will ensure your Rails applications are well-tested and function as expected.
Test-Driven Development: A Practical Ruby Approach
Test-driven development (TDD) often promises cleaner code and fewer bugs, but implementing it effectively can be tricky. Many Ruby developers find that rigidly following the red-green-refactor cycle can slow down progress. This article explores how experienced Ruby teams adapt TDD to real-world projects.
Practical TDD: Finding Balance
The core principle of TDD – writing a failing test, then making it pass, followed by refactoring – is still valuable. However, successful teams don’t treat it as an inflexible rule. Sometimes, it’s more efficient to write the code first, particularly for simpler components. The key is to be pragmatic and adapt your approach to the specific situation.
For complex algorithms, TDD shines by guiding design and ensuring correctness. For simpler tasks, like adding a getter method, writing the code first is often faster. Flexibility is key to efficient development.
Focusing TDD Efforts
Not every component benefits equally from TDD. Focus on areas with complex logic, high error potential, or critical functionality. This focused approach provides the best return on your testing investment. Ruby code interacting with external systems, performing complex calculations, or handling sensitive data are great candidates for TDD.
Integrating TDD with Legacy Code
Introducing TDD to existing codebases can feel overwhelming. Don’t attempt a complete overhaul at once. Start by adding tests for new features or during refactoring. Gradually increase your test coverage. This allows you to see the benefits of TDD without disrupting current development. As you add more tests, you’ll build confidence and find refactoring easier, improving overall code quality.
Designing With TDD, Avoiding Over-Engineering
Thoughtful TDD can positively influence code design. Writing tests first encourages testable and modular code, leading to better separation of concerns. However, avoid over-engineering. Don’t test every single line of code. Focus on testing the public interface and the core logic of your classes and modules to keep your test suite focused and efficient.
TDD, when applied thoughtfully, provides significant benefits to Ruby projects. It can guide better code design and empower you to make changes with confidence. By adapting TDD practices to your project’s needs, you gain flexibility and control over your development process.
Continuous Integration For Ruby Projects
Continuous Integration (CI) is a cornerstone of effective Ruby development. By automatically running tests with every code change, CI helps catch errors early. This promotes consistent code quality and gives developers immediate feedback. Popular CI providers like CircleCI, Travis CI, and GitHub Actions offer robust Ruby support, making setup relatively straightforward. A well-configured CI system acts like a safety net, preventing regressions and bolstering team confidence.
Many teams use a tiered CI approach. Unit tests run first for quick feedback. If these pass, slower integration tests follow. This strategy balances the need for speed with the importance of thorough testing, which is especially crucial for Ruby on Rails projects.
Interpreting Test Results: Beyond Pass/Fail
Simply seeing green checkmarks isn't enough. Understanding why tests fail is crucial for efficient debugging. Robust CI systems provide detailed output, including error messages, stack traces, and logs. Learning to effectively interpret these results significantly speeds up debugging.
Tracking test failures over time can also reveal patterns and identify areas prone to bugs. This data can guide refactoring efforts. Furthermore, this practice helps pinpoint flaky tests - tests that inconsistently pass or fail without code changes.
Maintaining Test Quality As Your Codebase Grows
Maintaining test quality becomes more challenging as a Ruby project expands. Tests can become slow, complex, and tightly coupled to implementation, making them brittle.
- Address Slow Tests: Regularly profile your tests and optimize slow database queries or external service calls. Explore test caching strategies.
- Improve Test Readability: Use clear naming conventions and structure your tests logically. Keep tests focused and concise.
- Reduce Test Brittleness: Decouple your tests from internal implementation details. Focus on testing the public interface and behavior.
Testing Legacy Code: A Gradual Approach
Adding tests to legacy Ruby code can seem daunting. A gradual approach is best. Start with tests for new features or during refactoring. Prioritize critical code areas. Over time, test coverage will gradually improve.
Dealing With Flaky Tests
Flaky tests erode confidence in the entire test suite. Identifying and addressing them is crucial. Common causes include:
- Test Order Dependencies: Ensure tests are independent and can run in any order.
- External Dependencies: Isolate tests from external systems using tools like VCR or WebMock.
- Asynchronous Behavior: Manage asynchronous code with appropriate testing techniques.
Measuring Test Coverage Meaningfully
Test coverage quantifies how much code is exercised by your tests. While high coverage is helpful, meaningful coverage is more important. Don’t chase arbitrary percentages; focus on testing critical and complex areas.
A robust testing workflow builds confidence, catches errors early, and prevents regressions. These practices enable scalable development and the delivery of high-quality Ruby applications.
Ready to streamline your team's Ruby development workflow and minimize CI costs? Learn how Mergify can transform your code integration process.