Boost Code Quality with testing frameworks java
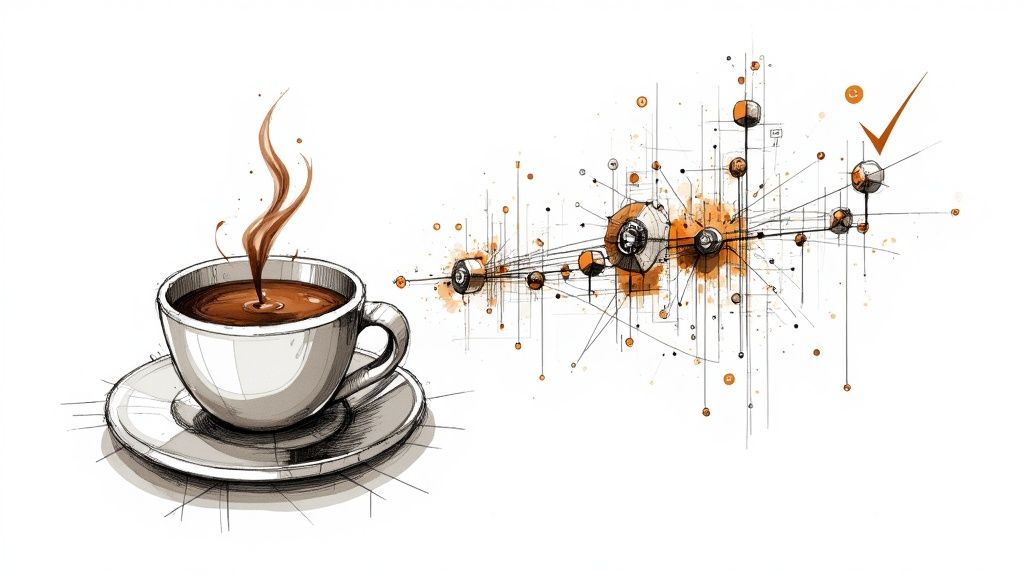
Java Testing Frameworks: An Overview
In the world of software development, thorough testing is essential for building high-quality applications. For Java developers, this means choosing the right testing framework is crucial. With so many options available, selecting the best fit for your project can be challenging.
This article explores the top 8 Java testing frameworks, providing you with the information you need to make an informed decision. We'll examine how these frameworks help address different testing needs, from basic unit tests to complex system-wide tests.
Key Considerations When Choosing a Framework
Several factors influence the choice of a testing framework. These include ease of use, integration with existing CI/CD pipelines, community support, and licensing. We’ll also discuss essential framework qualities, such as detailed reporting, mocking capabilities, and support for various testing methodologies. Whether you're a seasoned DevOps engineer or a QA specialist, understanding these frameworks is essential for delivering reliable software.
Top 8 Java Testing Frameworks
Here's a brief overview of the top 8 Java testing frameworks:
- JUnit: A widely used framework for unit testing Java code. Known for its simplicity and ease of use.
- TestNG: A powerful framework inspired by JUnit and NUnit, offering advanced features like annotations and parallel test execution.
- Mockito: A popular mocking framework that simplifies the creation of test doubles (mocks, stubs, etc.) for isolating units of code during testing.
- PowerMock: A framework that extends Mockito, providing capabilities for mocking static methods, constructors, and final classes.
- JBehave: A framework for Behavior-Driven Development (BDD) that helps bridge the gap between business stakeholders and technical teams.
- Cucumber: Another BDD framework that allows writing tests in a natural language format, making them more understandable for non-technical stakeholders.
- Spock: A testing and specification framework for Java and Groovy applications. Its expressive syntax enhances test readability.
- Arquillian: A framework designed for integration and functional testing of Java EE applications. It allows testing within the actual application server environment.
Making the Right Choice
By the end of this article, you’ll be equipped to choose the best Java testing framework for your specific needs. Consider project requirements, team expertise, and integration with existing tools when making your decision. Selecting the right framework is a vital step towards building robust, reliable, and maintainable Java applications.
1. JUnit 5
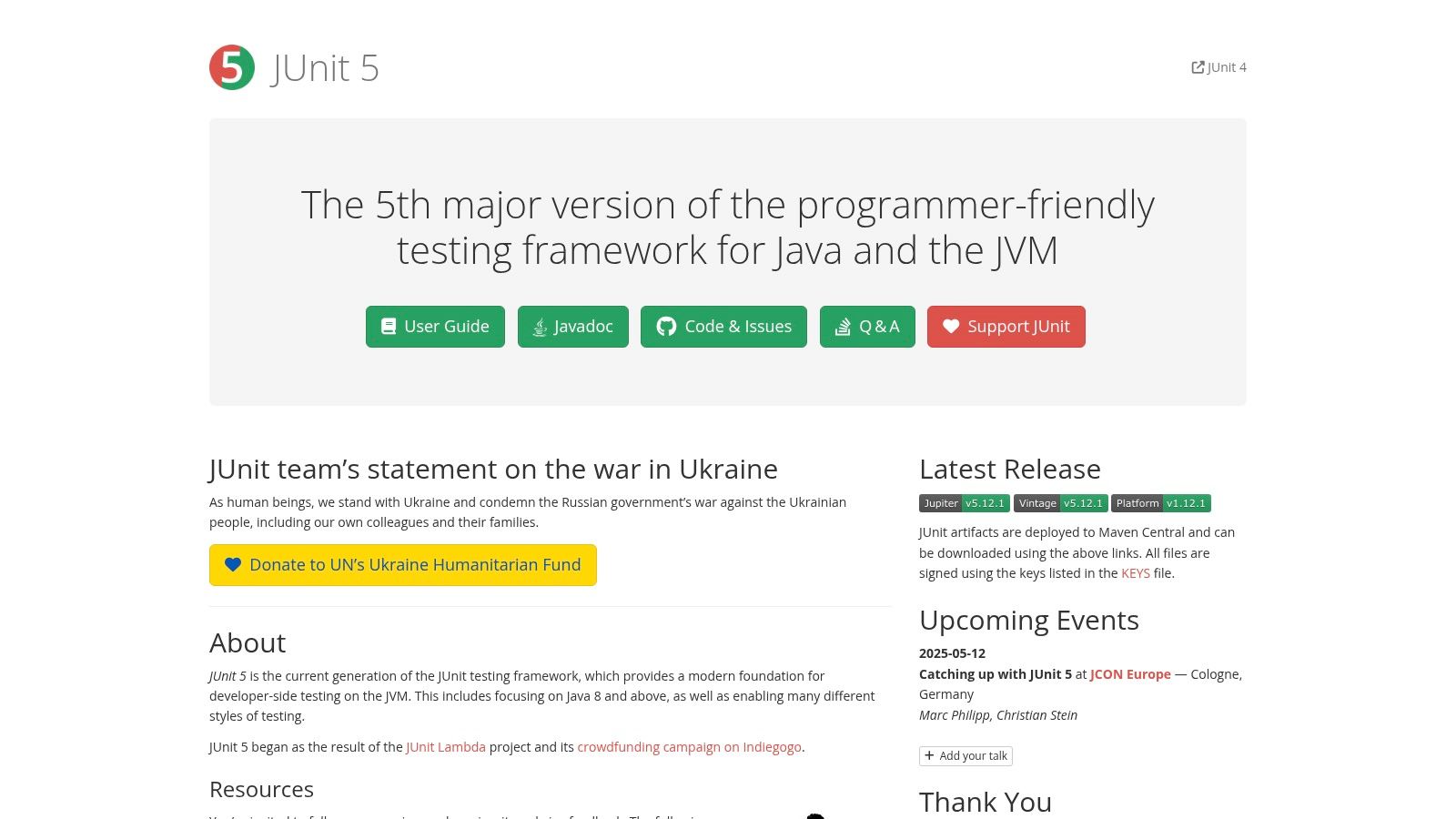
JUnit 5 is the current standard for unit testing Java applications. Its widespread adoption, robust features, and seamless IDE integration make it a top choice. Moving beyond JUnit 4's limitations, JUnit 5 provides a modernized framework designed to work with Java 8 and later versions.
It's not a single library, but a set of modules: JUnit Platform, JUnit Jupiter, and JUnit Vintage. The Platform serves as the foundation for running testing frameworks on the JVM. Jupiter provides the new programming and extension model for writing tests, and Vintage offers backward compatibility, allowing you to run older JUnit 3 and 4 tests alongside your JUnit 5 tests.
A key advantage of JUnit 5 is its embrace of Java 8 features like lambda expressions and method references, resulting in more concise test code. For example, assertions are enhanced with features like assertAll()
, letting you group multiple assertions together. This displays all failures at once instead of halting on the first error.
Parameterized Tests and Extensibility
Parameterized tests are more powerful in JUnit 5, pulling data from various sources such as CSV files, methods, or enums. The extensible architecture, using the @ExtendWith
annotation, simplifies integration with other tools, providing more flexibility in customizing your testing process.
Consider the challenge of testing a service with multiple dependencies. JUnit 5's nested test classes help structure tests to reflect the different facets of your service, improving code organization and readability. Imagine testing database interactions nested within a "Persistence Layer Tests" group. Further, conditional test execution, based on factors like environment variables, lets you precisely manage tests in environments like development, staging, or production. You might find valuable insights in resources like the Mergify Blog Posts on enhancing your development workflow.
Features:
- Support for Java 8 lambda expressions and method references
- Nested test classes and enhanced assertions
- Parameterized tests with diverse data sources
- Extension model for customization
- Conditional test execution
Pros:
- Industry-standard for Java testing
- Strong IDE integration
- Extensive ecosystem of extensions and plugins
- Comprehensive documentation and community support
Cons:
- Potential verbosity compared to newer frameworks
- Learning curve for migrating from JUnit 4
- Possible need for additional dependencies for some advanced features
Website: https://junit.org/junit5/
Implementation Advice
- Begin by adding the necessary JUnit 5 dependencies to your project's build file, whether you are using Maven or Gradle.
- Use the
@DisplayName
annotation to create descriptive names for your test classes and methods for improved reporting. - Explore the various available JUnit 5 extensions, including community-provided ones. The MockitoExtension, for instance, allows easy integration with the Mockito mocking framework.
JUnit 5's status as a core testing framework is strengthened by its comprehensive features, backward compatibility, and broad community support. The transition from JUnit 4 offers benefits such as better code organization, stronger assertions, and greater extensibility, making it valuable for modern Java development.
2. TestNG
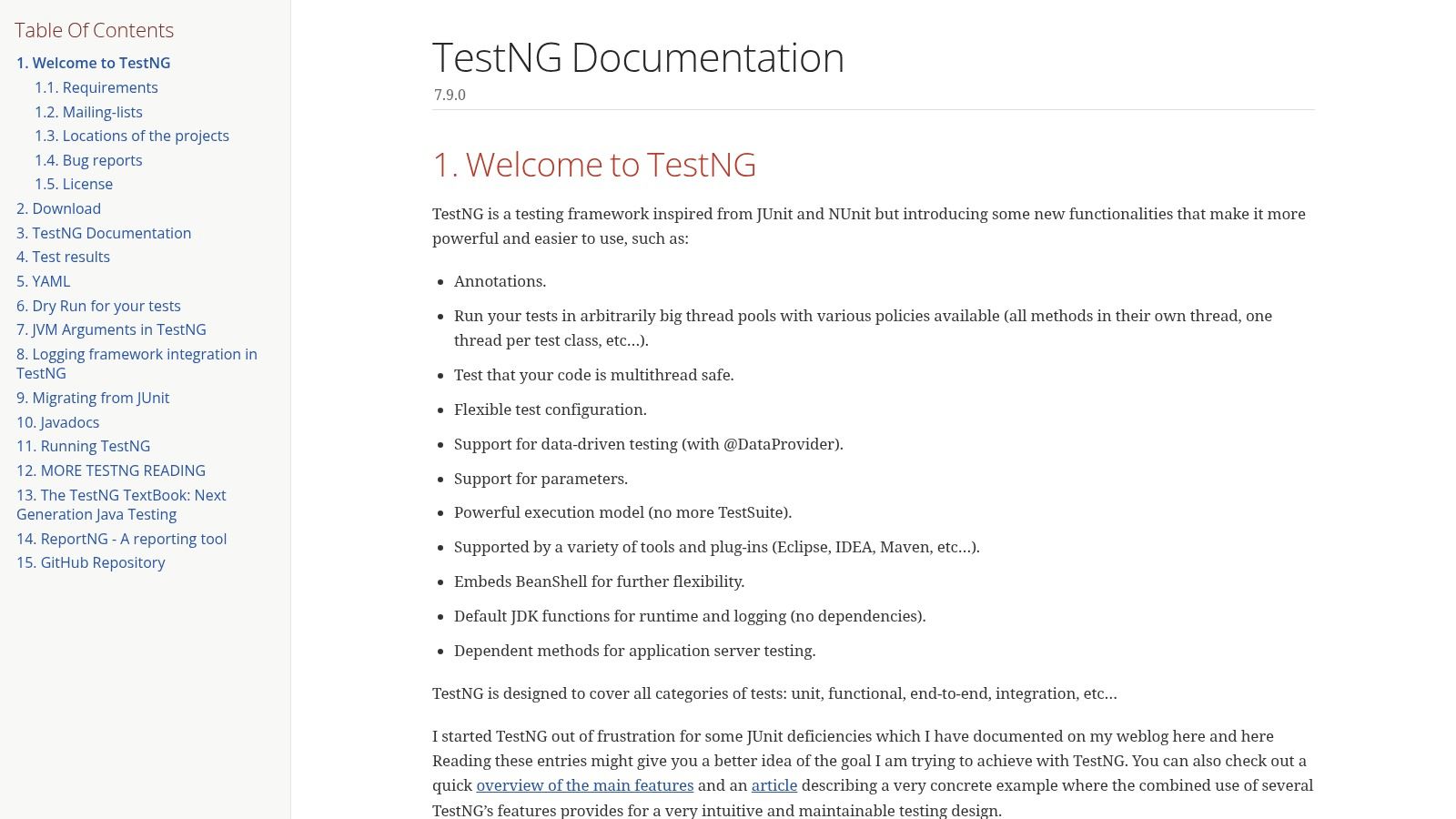
TestNG is a powerful testing framework, especially useful for complex integration and system tests. Drawing inspiration from JUnit and NUnit, it expands on their core functionalities with features designed for intricate applications. If your team works with interconnected modules, complex dependencies, or large datasets, TestNG offers distinct advantages.
TestNG is designed to handle all types of tests: from unit and functional testing to end-to-end and integration testing. Its main strength lies in managing complex scenarios using features like dependency testing and grouping. For example, if step B in a multi-step test depends on step A completing successfully, TestNG lets you define that dependency. This ensures step B runs only if step A passes, making complex testing workflows much easier to manage.
Key Features & Benefits
TestNG offers several key features and benefits that enhance the testing process. Here's a quick rundown:
- Annotations-Based Configuration: Annotations like
@Test
,@BeforeTest
, and@AfterTest
offer a clean and readable way to configure tests and their execution sequence. This minimizes boilerplate code and makes tests easier to maintain. - Dependent Methods and Groups: Using the
dependsOnMethods
andgroups
attributes within the@Test
annotation lets you establish dependencies between test methods and group related tests, improving organization and control. - Parallel Test Execution: TestNG allows parallel test execution, which significantly reduces testing time, particularly for large suites. This feature is highly valuable in CI/CD pipelines.
- Built-In Data-Driven Testing: The
@DataProvider
annotation streamlines data-driven testing by allowing you to supply various datasets to your tests without repetitive code. This is essential for testing different inputs and scenarios. - Flexible Test Configuration with XML: While sometimes verbose for simple tests, XML configuration provides extensive flexibility for complex suites, including parameterization, dependencies, and parallel execution settings.
Pros and Cons
Like any tool, TestNG has its strengths and weaknesses.
Pros:
- Ideal for complex integration and system tests due to its support for dependencies, grouping, and parallel execution.
- Superior handling of test dependencies compared to JUnit, providing a more organized approach.
- Powerful reporting features offer detailed results, including successes, failures, and skipped tests.
- Comprehensive documentation and a well-established API make learning and usage easier.
Cons:
- Configuration can be overly complex for simple tests where the added flexibility becomes overhead.
- XML configuration, while powerful, can become lengthy and challenging to manage for large suites.
- A smaller community than JUnit, which may sometimes mean less readily available support.
Implementation/Setup Tips
Here are a few tips for getting started with TestNG:
- Organize and structure your tests using annotations effectively.
- Use
@DataProvider
for data-driven testing to maximize efficiency. - Begin with basic configurations and gradually explore advanced features as needed.
- Consider using a build tool like Maven or Gradle for dependency management and build simplification.
Pricing and Requirements
TestNG is open-source and free to use. The primary technical requirement is the Java Development Kit (JDK).
Conclusion
TestNG provides robust tools for tackling the complexities of software system testing. While there's a learning curve, especially with the XML configuration, the benefits of organized testing, dependency management, and parallel execution make it a valuable asset. For projects that go beyond simple unit tests and involve integration or system testing, TestNG is an excellent choice. You can find more information on their official website.
3. Mockito
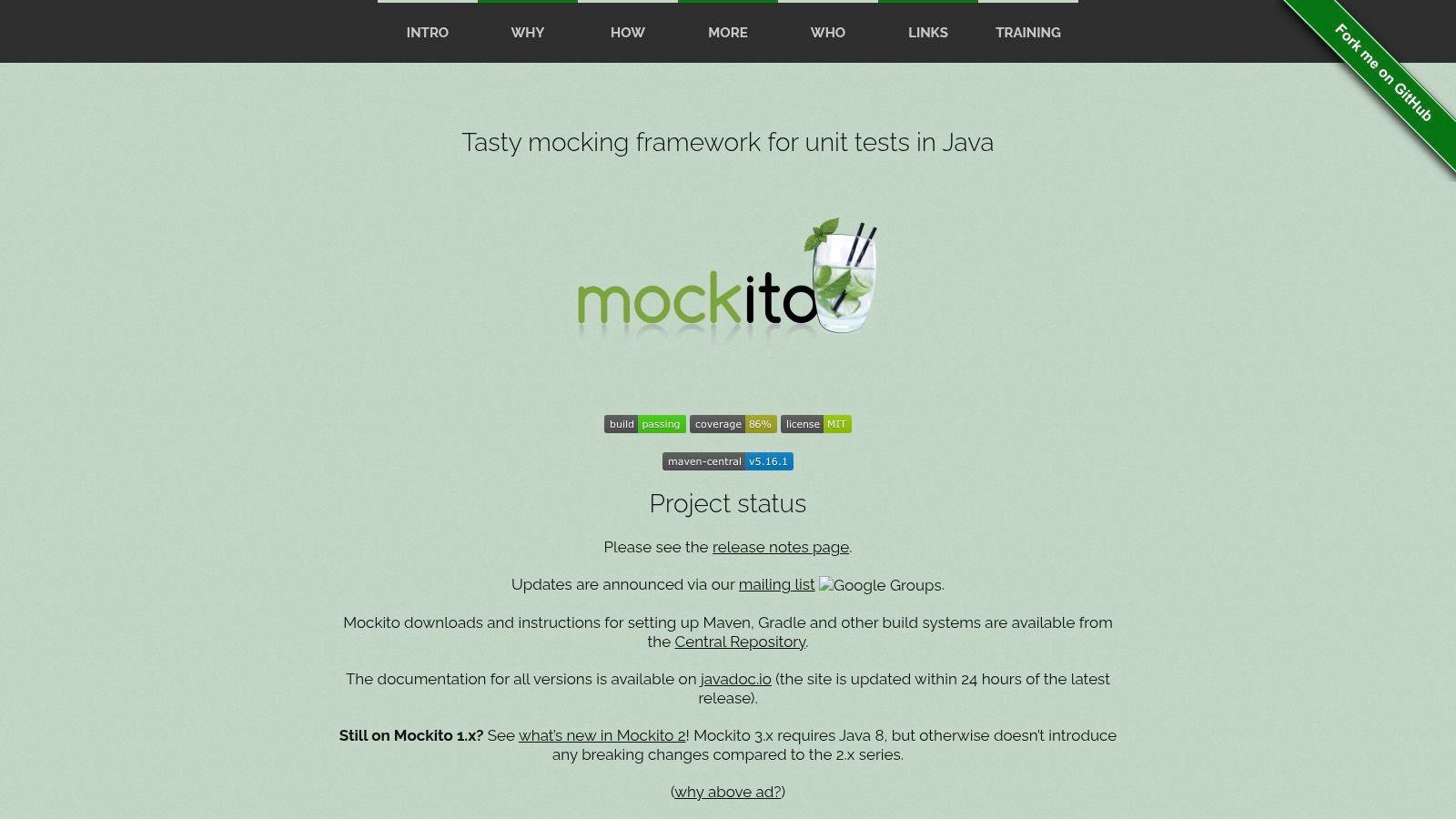
Mockito is a prominent mocking framework for Java, making clean and effective unit tests easier to create. Its focus on a clean API and readability makes it a popular choice for developers looking to isolate units of code and verify behavior without external dependencies.
Imagine building a service that interacts with both a database and an external API. This kind of scenario is where Mockito truly shines. You can mock both of these dependencies, ensuring that your tests concentrate solely on the core service logic. This level of isolation results in faster tests and helps pinpoint errors within the service itself, not in its supporting components.
Features and Benefits
Mockito offers a range of features that streamline the testing process:
- Simple and Readable API: Creating mocks and stubs is straightforward, resulting in tests that are easier to write and maintain. Mocking a database call, for example, becomes a simple task accomplished with minimal code.
- Verification of Mock Interactions: Mockito allows developers to precisely verify the interactions between the unit under test and its mocked dependencies. You can check the number of calls to a method, the arguments used in those calls, and even the order of interactions.
- Support for Spy Objects (Partial Mocking): Spy objects enable mocking specific methods of a real object while leaving other methods untouched. This is particularly helpful for testing a majority of a class's actual functionality while controlling specific aspects for your test scenario.
- Flexible Argument Matching With Custom Matchers: Mockito handles argument matching with flexibility. You can even define custom matchers to manage complex scenarios, providing fine-grained control over your test setup.
- Integration With JUnit (and TestNG): Mockito smoothly integrates with testing frameworks like JUnit, creating a cohesive testing environment and simplified reporting.
Pros
- Intuitive API: Developers can quickly pick up Mockito and begin writing effective tests without a steep learning curve.
- Seamless Integration: Mockito works effectively with JUnit and TestNG, fitting comfortably into established testing workflows.
- Detailed Failure Messages: When tests fail, Mockito provides detailed error messages that make debugging and troubleshooting easier.
- Active Development: Mockito is actively maintained and updated, offering compatibility with current Java versions and reflecting community input.
Cons
- Mocking Limitations: Mocking final classes and methods typically requires extensions like Mockito Inline, adding an extra layer of configuration. While recent updates have improved support for static methods, some constraints remain compared to mocking instance methods.
- Debugging Complex Setups: Debugging interactions between multiple mocks can be tricky in intricate test scenarios.
You might be interested in: Our guide on Mergify site pages
Implementation and Setup Tips
Integrating Mockito into your project is straightforward using dependency management tools like Maven or Gradle. Once added, you can utilize Mockito annotations like @Mock
, @InjectMocks
, and @Spy
to create and manage mocks. Key methods to understand include when()
, thenReturn()
, and verify()
.
Pricing
Mockito is open-source and free to use.
Website
4. Spock Framework
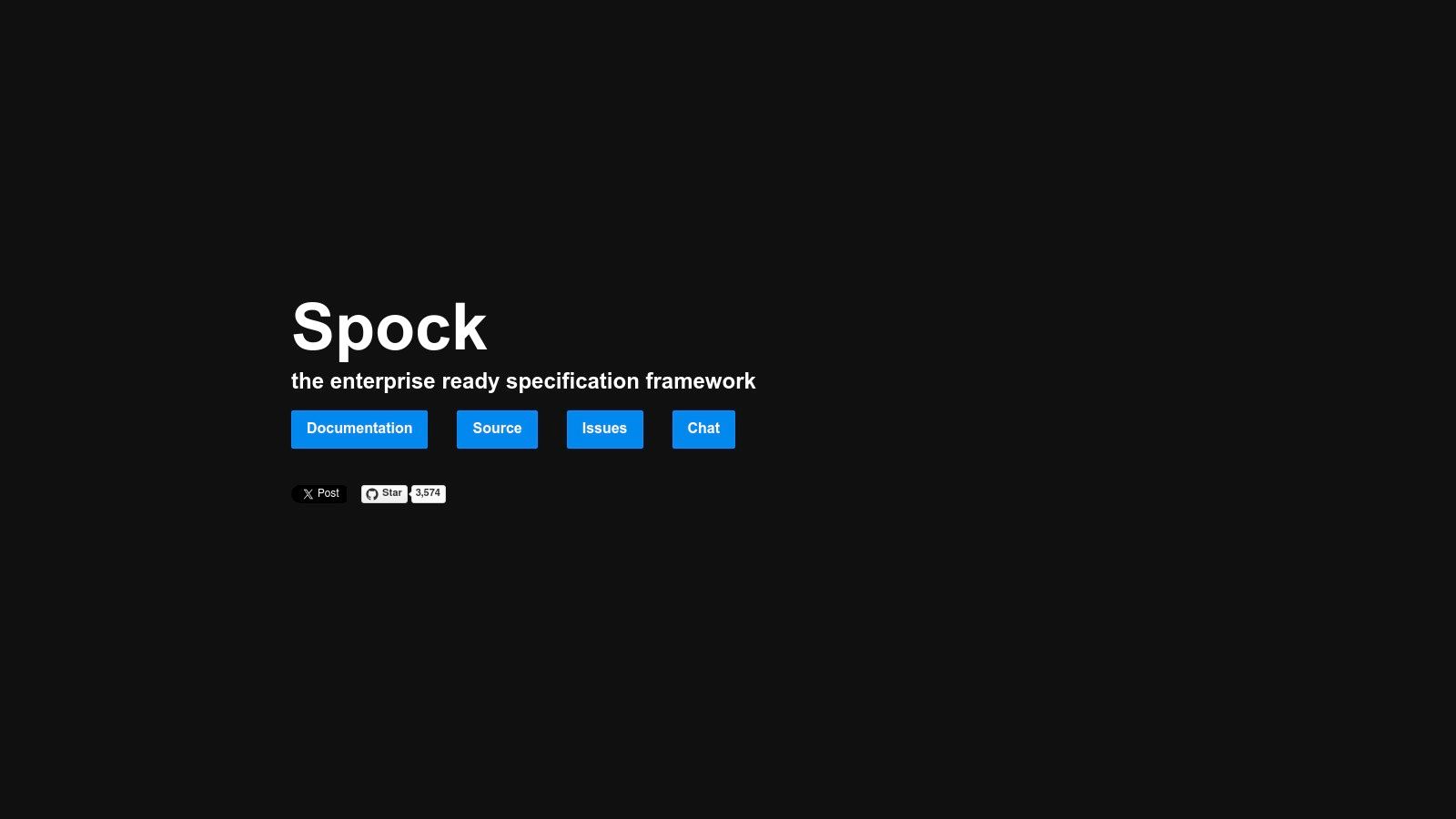
Spock stands out among testing frameworks thanks to its potent combination of powerful features, an expressive syntax, and a strong emphasis on readability. Built on Groovy, it integrates smoothly with Java projects, providing a welcome alternative to traditional Java testing frameworks. Spock truly shines in creating easily maintained and understood tests, a significant advantage when dealing with complex business logic and interactions.
Spock is specifically designed for testing Java and Groovy applications. It uses Groovy's dynamic nature to offer a concise and expressive syntax. Unlike typical Java testing frameworks, Spock encourages a Behavior-Driven Development (BDD) approach. Its unique given-when-then
blocks structure tests for improved clarity and understanding. It effectively combines the strengths of popular tools like JUnit and Mockito into one cohesive package.
Key Features and Benefits
Spock offers a range of features that make it a compelling choice for developers:
- BDD-Style Testing: The
given-when-then
blocks clearly separate setup, actions, and expected outcomes, making tests remarkably clear and easy to maintain. - Built-in Mocking: Spock handles mocking internally, eliminating the need for external libraries. This simplifies dependency management and streamlines your code.
- Data-Driven Testing: Spock excels at data-driven testing using data tables and data pipes. You can run the same test with multiple inputs and expected outputs, ideal for boundary testing and scenario exploration.
- Expressive Assertions: Spock's assertions are more expressive and readable than standard JUnit assertions, leading to better diagnostics when tests fail.
- Java and Groovy Compatibility: While built with Groovy, Spock works seamlessly with Java projects, allowing you to use it with your existing Java codebase.
Practical Applications and Use Cases
Spock's versatility makes it suitable for a wide range of testing scenarios:
- Unit Testing: Ideal for testing complex business logic thanks to its clear structure and expressive syntax.
- Integration Testing: Built-in mocking simplifies testing interactions between components without complicated setups.
- API Testing: Spock’s data-driven testing features are extremely efficient for testing APIs with varied inputs and validating responses.
- UI Testing: When combined with Geb, a Groovy-based browser automation solution, Spock becomes a powerful UI testing framework.
Pros and Cons
Like any tool, Spock has its advantages and disadvantages:
Pros:
- Readability: BDD style and Groovy syntax contribute to highly readable test code.
- Integrated Mocking: Built-in mocking simplifies dependency management.
- Detailed Reports: Provides powerful failure reports for easier debugging.
- Reduced Boilerplate: Requires less code than traditional Java testing frameworks.
Cons:
- Groovy Knowledge: Requires basic Groovy knowledge, though it’s generally easy to learn.
- Compilation Speed: Groovy compilation can be slower than Java.
- IDE Support: While improving, IDE support for Spock might not be as comprehensive as JUnit in some cases.
Implementation and Setup
Getting started with Spock is straightforward:
- Add the Spock dependency to your project's build file (Maven or Gradle).
- Ensure Groovy support is enabled in your IDE.
- Begin with simple tests using the
given-when-then
structure. - Explore Spock's mocking capabilities to isolate units under test.
- Use data tables for effective data-driven testing.
Pricing and Technical Requirements
Spock is open-source and free to use. It requires a Java Development Kit (JDK) and a Groovy environment.
Website
5. AssertJ
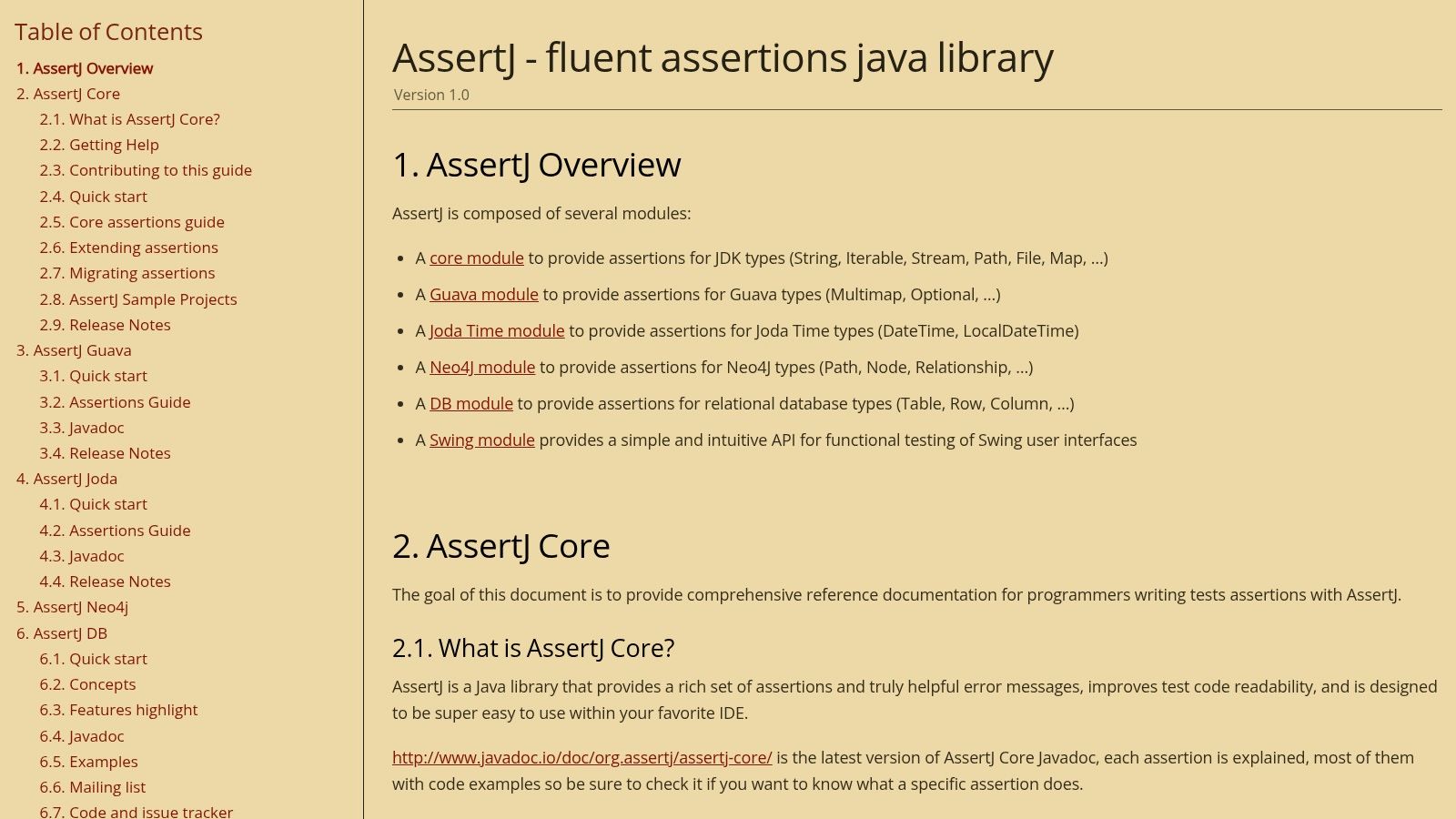
AssertJ stands out as a powerful Java testing tool, thanks to its fluent API. This API dramatically improves how readable and maintainable your test code is. While not a full-blown framework like JUnit or TestNG, it integrates seamlessly with them to enhance assertions. This is especially useful in complex projects where clear test failures are essential for efficient debugging.
AssertJ’s strength lies in its human-readable assertions. Instead of traditional JUnit like assertEquals(expected, actual)
, AssertJ uses a fluent API that reads naturally: assertThat(actual).isEqualTo(expected)
. This seemingly small change significantly improves readability, especially in tests with numerous assertions.
Key Features and Benefits
- Fluent Assertion API: The fluent API is the heart of AssertJ, creating assertions that are easy to understand and maintain. For example, comparing strings becomes a breeze:
assertThat(resultString).startsWith("Hello").endsWith("World!");
This is considerably more intuitive than nestedassertTrue
calls. - Rich Set of Assertions: AssertJ provides a wide-ranging library of assertions for many Java types, including Strings, Collections, and Maps. Specialized assertions are available for conditions such as emptiness, size, and specific element checks.
- Detailed Failure Messages: When assertions fail, AssertJ gives detailed error messages pinpointing the problem. This significantly reduces debugging time. Instead of a simple "values are not equal," AssertJ shows expected and actual values, highlighting the differences.
- Custom Assertions: You can create custom assertions for objects specific to your application. This leads to greater consistency and less boilerplate code.
- Java 8 Support: AssertJ seamlessly supports Java 8 features, such as lambdas and streams, allowing for expressive and concise assertions in modern Java code.
Pros
- The fluent API's readability makes tests easier to understand and maintain.
- The comprehensive set of assertions covers a wide range of Java types, minimizing the need for custom assertion logic.
- Descriptive failure messages make debugging quicker.
- Excellent IDE support simplifies test development.
Cons
- It's not a complete testing framework and needs JUnit or TestNG.
- The vast number of assertions can initially seem overwhelming.
- The fluent API can occasionally be a bit more verbose than traditional methods for very basic assertions.
Implementation/Setup Tips
Adding AssertJ is straightforward. Include the dependency for your build tool (Maven, Gradle). For Maven, add the following to your pom.xml
:
org.assertjassertj-core3.24.2test
Comparison
While libraries like Hamcrest offer comparable functionality, AssertJ's fluent API is more readable. Its Java focus and comprehensive assertions make it a good choice for most Java projects. Hamcrest's multi-language flexibility can be less intuitive for Java-specific cases.
Website
https://assertj.github.io/doc/
AssertJ is a valuable tool for any Java testing project. The fluent API improves readability, the range of assertions reduces boilerplate, and detailed error messages speed up debugging. Despite a small learning curve, the benefits for test code quality and maintainability make it a worthwhile addition.
6. Cucumber JVM
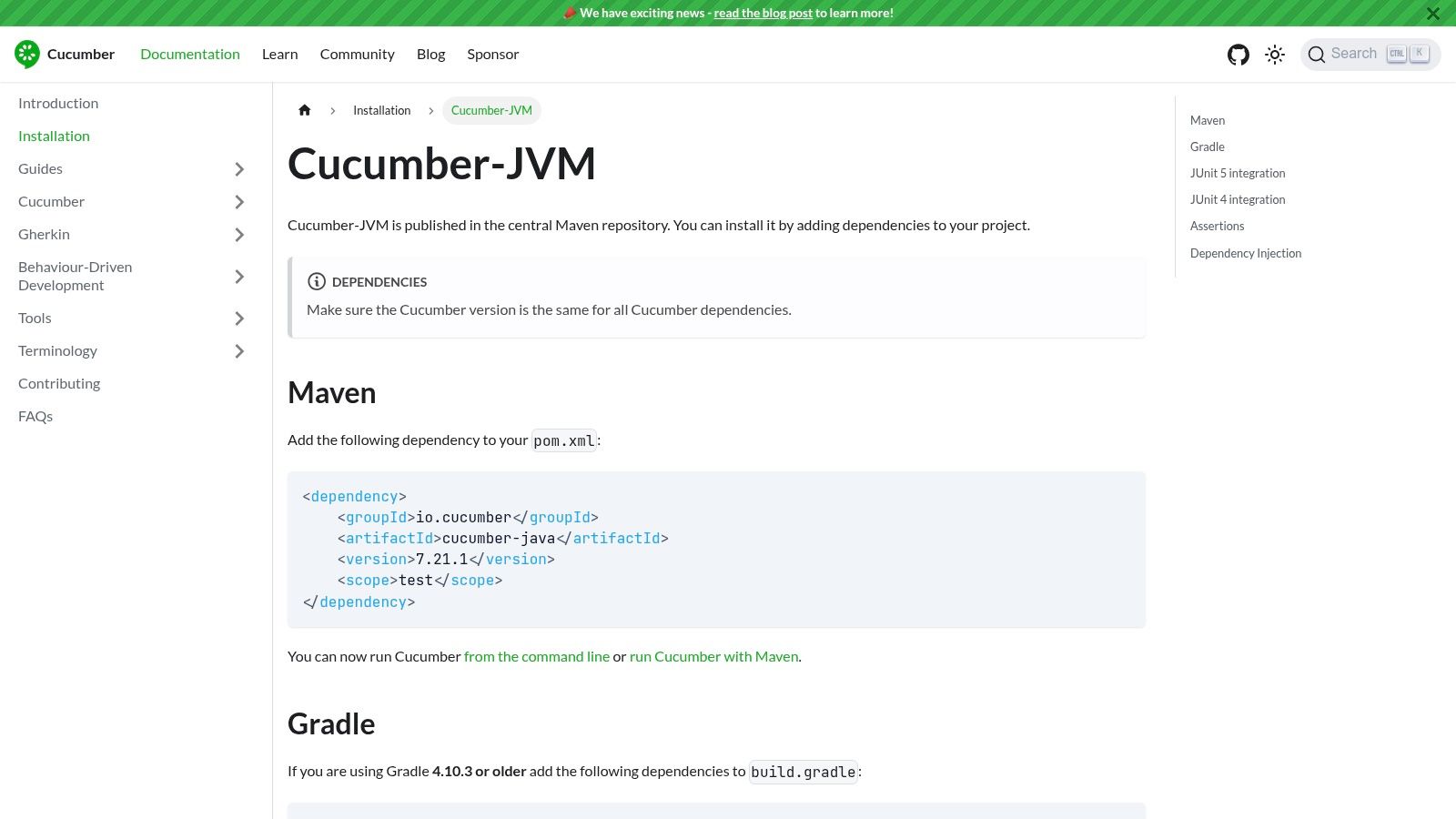
Cucumber JVM is a valuable tool for bridging the gap between technical implementation and business needs. It uses a Behavior-Driven Development (BDD) approach. This allows teams to describe how software should behave in plain language, making it accessible to both technical and non-technical team members. This shared understanding helps improve teamwork and minimize misunderstandings.
As the Java implementation of Cucumber, Cucumber JVM utilizes Gherkin, a human-readable language for writing feature scenarios. These scenarios then become executable specifications, tested directly against the Java code. Teams can efficiently implement and maintain automated acceptance tests. Key features include reusable step definitions with regular expressions, integration with Java testing frameworks like JUnit, and support for data tables and scenario outlines.
Features
- Executable Specifications: Gherkin scenarios are live tests, not just static documents.
- BDD Facilitation: Promotes collaboration and a shared understanding of project requirements.
- Reusable Step Definitions: Regular expressions enable flexible and reusable test code.
- JUnit Integration: Works seamlessly with existing Java testing processes.
- Data-Driven Testing: Data tables and scenario outlines allow efficient testing with various inputs.
Pros
- Improved Collaboration: Reduces communication barriers between business and development teams.
- Living Documentation: Feature scenarios act as always up-to-date documentation, mirroring system behavior.
- BDD Best Practices: Encourages a focus on the desired outcomes through a behavior-driven development approach.
- Comprehensive Reporting: Provides detailed reports on test execution results, offering valuable insights.
Cons
- Added Complexity: Introduces an additional layer to the testing process compared to basic unit testing.
- Step Definition Maintenance: Managing step definitions can become difficult in larger projects.
- Performance Overhead: Running scenarios through Cucumber JVM can be slower than direct unit tests.
- Learning Curve: Requires a good grasp of BDD principles and Gherkin syntax for effective use.
You might be interested in: Mergify Authors for additional insights into development workflows.
Implementation Tips
- Begin with a solid understanding of the BDD process.
- Organize your project logically to manage feature files and step definitions efficiently.
- Use regular expressions carefully in step definitions to find a balance between flexibility and maintainability.
- Integrate Cucumber JVM with your CI/CD pipeline for automatic execution of acceptance tests.
Cucumber JVM is an open-source tool, free to use. Technical requirements include Java and a build tool such as Maven or Gradle. While other BDD tools exist, like SpecFlow (.NET) and Behat (PHP), Cucumber JVM remains a popular choice for Java-based projects thanks to its mature development and active community support. It's especially suited for projects that value collaboration and aim to maintain living documentation that is tightly integrated with the codebase. If your team wants to adopt BDD principles and improve communication throughout the software development lifecycle, consider Cucumber JVM. More information and documentation can be found on the official website: Cucumber JVM Installation.
7. JMeter
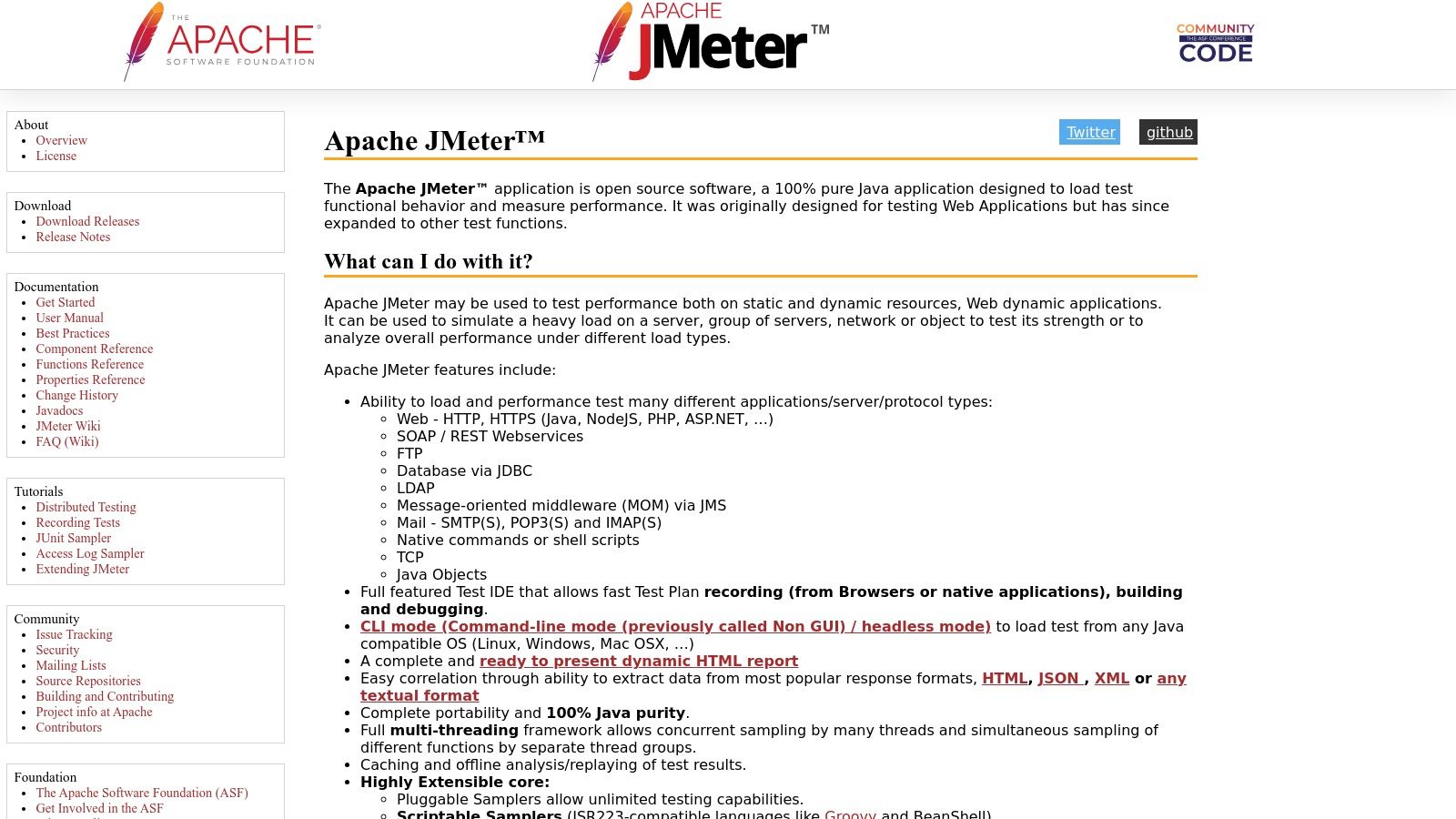
Apache JMeter is a powerful open-source tool primarily used for performance and load testing. It's also capable of functional testing of web services. Its extensibility and broad protocol support make it a valuable asset for testing, especially in Java-centric environments. JMeter helps you simulate heavy user traffic to identify and address performance bottlenecks, ensuring your applications can handle the anticipated load.
Description:
JMeter simulates user behavior to thoroughly test web applications, databases, and other services. It generates detailed reports on key performance indicators (KPIs) like response times, throughput, and error rates. This information helps pinpoint and diagnose performance issues. While its primary focus is performance, JMeter can also be used for functional testing of APIs and web services by sending requests and validating responses.
Key Features:
- Protocol Support: JMeter handles a variety of protocols, including HTTP, HTTPS, SOAP, REST, FTP, JDBC, LDAP, and JMS, making it suitable for testing a wide spectrum of applications.
- Scriptable Samplers: JMeter offers flexibility for complex test scenarios using JSR223-compatible languages such as Groovy, enabling advanced logic and data manipulation.
- Extensible Core: A highly extensible core, achieved through plugins, allows for customization and integration with other tools.
- Dynamic Testing: Built-in functions and variables support dynamic parameterization and data-driven testing.
- Distributed Testing: JMeter facilitates distributed testing across multiple machines, simulating massive user loads.
Pros:
- Comprehensive Performance Testing: JMeter provides a rich feature set for various types of performance testing, including load testing, stress testing, and endurance testing.
- Functional Testing Capabilities: It can be used to test the functionality of web services and APIs.
- Highly Configurable and Extensible: JMeter boasts extensive configuration options and a large library of plugins for tailoring tests.
- Cross-Platform and Browserless: It runs on any platform with Java and doesn't require a browser for web service testing.
- Open-Source and Free: JMeter eliminates licensing costs.
Cons:
- Steep Learning Curve: It can be challenging for beginners, especially for complex scripting scenarios.
- Limited JavaScript Rendering: JMeter doesn't fully render JavaScript like a browser, potentially affecting test accuracy for client-side heavy applications.
- Resource Intensive: It can consume substantial resources, particularly during large-scale distributed tests.
- Dated UI: The user interface may feel less modern compared to some newer tools.
Use Cases:
- Load Testing Web Applications: Simulate thousands of concurrent users to identify bottlenecks.
- API Testing: Test the functionality and performance of RESTful and SOAP APIs.
- Database Testing: Stress-test databases under heavy load conditions.
- Continuous Integration: Integrate JMeter into your CI/CD pipeline to automate performance testing.
Implementation/Setup Tips:
- Begin with a simple test plan and gradually increase complexity.
- Use the JMeter Proxy Server to record user interactions for creating test scripts.
- Utilize Groovy scripting for advanced test logic and data manipulation.
- Monitor server resources during tests to identify performance bottlenecks.
- Consider cloud-based testing platforms for distributed testing.
Comparison With Similar Tools:
JMeter is often compared to tools like Gatling and k6. While Gatling might offer better performance for extremely high loads due to its asynchronous architecture, JMeter's wider protocol support and extensive plugin ecosystem make it more versatile. k6 excels with its developer-friendly JavaScript scripting, but JMeter's mature feature set and robust distributed testing capabilities are attractive for large-scale testing.
Pricing and Technical Requirements:
JMeter is open-source and free. It requires Java.
Website: https://jmeter.apache.org/
JMeter's versatility and extensibility make it a powerful tool for ensuring the performance and reliability of your Java applications and web services. While there's a learning curve, its comprehensive testing capabilities make it a worthwhile investment. Its open-source nature and active community solidify its value in the Java testing landscape.
8. Selenide: Concise and Elegant UI Testing for Java
Selenide stands out as a simple and efficient tool for UI testing in Java. Building upon Selenium WebDriver, it addresses common UI testing challenges by reducing boilerplate code and automating tasks like waiting for elements and taking screenshots when tests fail. This leads to more manageable tests, boosting productivity for QA and development teams.
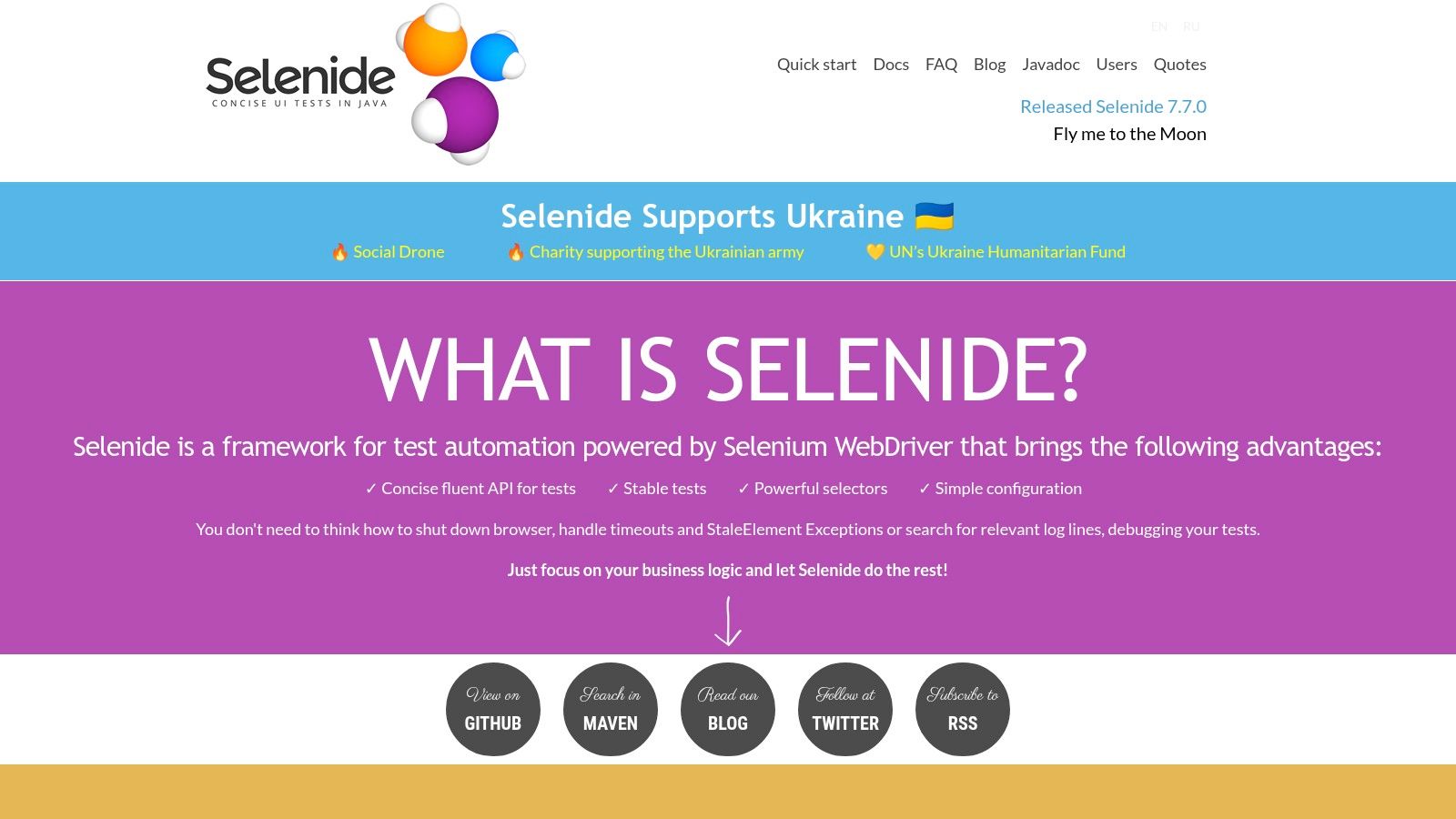
Practical Applications and Use Cases
- Rapid UI Testing: Perfect for quickly developing and running UI tests for web applications, a necessity in fast-paced agile development.
- Cross-Browser Testing: Selenide supports various browsers, ensuring your application works consistently across different platforms.
- Regression Testing: Streamlines regression test maintenance and execution, preventing new code from introducing UI bugs.
- Continuous Integration/Continuous Delivery (CI/CD): Selenide’s clear syntax and seamless integration make it ideal for automated testing in CI/CD pipelines.
Features and Benefits
- Concise and Readable API: Selenide uses a fluent API that makes tests easier to understand and simplifies code compared to plain Selenium. For example, instead of:
WebElement element = driver.findElement(By.id("myElement")); WebDriverWait wait = new WebDriverWait(driver, 10); wait.until(ExpectedConditions.visibilityOf(element)); element.click();
You can write:
$("#myElement").shouldBe(visible).click();
- Automatic Waits: Selenide intelligently manages waits, removing the need for explicit
WebDriverWait
, thus improving test stability. - Automatic Screenshots on Failure: Selenide captures screenshots automatically when tests fail, providing useful debugging information.
- Simplified Ajax Support: Built-in functions simplify the testing of asynchronous operations and dynamic web content.
- Fluent Element Interactions: Chainable methods for selecting and interacting with elements enhance code readability.
Pros
- Significantly less boilerplate code compared to using Selenium directly.
- Automatic management of timing issues.
- Simple setup and configuration.
- Excellent documentation and many examples.
Cons
- Less flexibility than raw Selenium for certain complex scenarios.
- Can potentially obscure underlying Selenium problems.
- Still requires a basic understanding of web technologies for creating effective tests.
- Primarily for UI testing; not suitable for other types like API testing.
Technical Requirements and Setup
Selenide requires Java and a build tool like Maven or Gradle. Adding Selenide to a project is easily done through dependency management:
com.codeborneselenideLatest Version
Comparison with Similar Tools
While similar to Selenium, Selenide prioritizes ease of use and developer experience. Other Java UI testing frameworks like Serenity BDD provide more detailed reporting and integration with BDD tools. The best tool depends on individual project requirements.
Website
Selenide is a valuable tool for Java UI testing, simplifying both test development and upkeep. Its concise API, automatic waits, and helpful features enable teams to create robust and reliable UI tests efficiently. For a more user-friendly approach to using Selenium, Selenide is worth exploring.
Top 8 Java Testing Frameworks: Feature Comparison
Tool | Core Features ✨ | User Experience ★ | Value Proposition 💰 | Target Audience 👥 |
---|---|---|---|---|
JUnit 5 | Java 8+ support, modular design, nested & parameterized tests | Widely adopted, excellent IDE integration | Industry standard reducing migration hassles | Java developers & enterprise teams |
TestNG | Annotation configuration, dependency groups, parallel execution | Mature API for complex integration testing | Flexible framework with robust reporting | Integration & system testers |
Mockito | Simple mock creation, spy support, custom argument matching | Intuitive API with clear, detailed error messages | Enhances test reliability and speeds up debugging | Unit testers and Java developers |
Spock Framework | BDD-style given/when/then, built-in mocking, data-driven testing | Highly readable with less boilerplate code | Streamlines specs for Java & Groovy while offering rich diagnostics | Developers open to Groovy and agile teams |
AssertJ | Fluent assertions, extensive Java type support, detailed failure messages | Very intuitive with descriptive error reports | Improves readability and complements other testing frameworks | Test developers focusing on assertion clarity |
Cucumber JVM | Gherkin-based specs, reusable step definitions, integration with JUnit | Bridges business & tech with living documentation | Promotes collaboration through behavior-driven development | Cross-functional teams and agile stakeholders |
JMeter | Multi-protocol testing, scriptable samplers, distributed load testing | Robust performance testing with high configurability | Cost-effective tool for optimizing load and performance tests | Performance testers and web service analysts |
Selenide | Concise API for browser automation, auto-waiting, screenshot capture on failure | Simplifies Selenium with minimal boilerplate | Enhances UI test efficiency through stable, automated testing | UI testers and web automation engineers |
Choosing the Right Java Testing Framework
Choosing the right Java testing framework is a crucial decision that depends heavily on your project's specific needs. Several factors come into play when making this choice. For unit testing, two popular options are JUnit and TestNG. JUnit is often favored for its simplicity and widespread use, making it an easy starting point for many projects. TestNG, however, provides more advanced features, including parameterized tests and the ability to run tests in parallel.
These frameworks can be further enhanced with supporting tools. Mockito is excellent for creating mocks, which simulate the behavior of real objects and isolate units of code for testing. AssertJ provides a fluent API for writing assertions, making your test code more readable and easier to maintain.
Beyond unit testing, other frameworks cater to different testing needs. For integration and behavior-driven development (BDD), Spock and Cucumber JVM are strong contenders. Spock, with its Groovy-inspired syntax, offers a concise and expressive way to write tests. Cucumber JVM, on the other hand, uses the Gherkin language, which helps bridge communication gaps between technical and non-technical stakeholders.
Considering Different Testing Types
Different types of testing require specialized tools. JMeter is a powerful platform for performance testing, allowing you to simulate heavy loads and analyze your application's behavior under stress. For UI testing, Selenide builds upon Selenium to provide a more streamlined and developer-friendly experience. It simplifies common UI testing tasks and handles many of the complexities of working with web browsers.
Implementing these frameworks is generally straightforward. Most offer comprehensive documentation and practical examples to guide you. The typical process involves adding the necessary dependencies to your project, creating test classes, and writing test methods. Remember to make the most of each framework’s specific features to thoroughly test various aspects of your application.
Budget, Resources, and Compatibility
Budget and resource considerations also play a role in framework selection. While many popular frameworks are open-source, integrating them into a CI/CD pipeline might require specific tools or infrastructure. It's essential to consider the learning curve for each framework and allocate time for team training.
Integration and compatibility are also critical. The chosen framework should seamlessly integrate with your existing development environment, including build tools like Maven or Gradle, and your CI/CD platform. A framework's maturity and community support are also significant factors, as they influence the availability of resources and assistance when troubleshooting issues.
In short, selecting a Java testing framework involves balancing project requirements, team expertise, and budget constraints. Consider the type of testing required (unit, integration, performance, UI), application complexity, and the level of integration needed with existing tools. By thoughtfully evaluating these factors, you can choose the right framework to ensure the quality and reliability of your Java applications.