Test Ruby Guide: Master Your Code
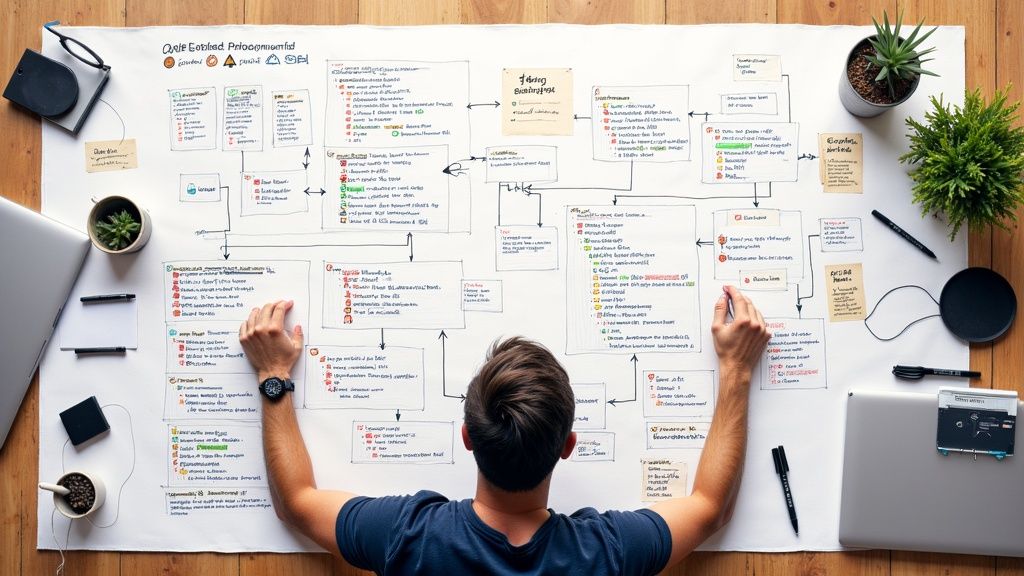
Demystifying Ruby Testing Fundamentals
Testing forms the backbone of strong software development, helping maintain code quality and reduce unexpected errors. In Ruby, testing isn't just recommended—it's woven into the very fabric of development philosophy. This requires viewing testing not as something to tackle after coding, but as an essential companion throughout the entire development journey.
Why Test Ruby Code?
Good testing practices catch bugs early, significantly reducing the time you'll spend debugging later. Tests also give developers the confidence to refactor code, knowing that any unintended side effects will be flagged immediately. Beyond bug detection, a solid test suite serves as living documentation that clearly shows how your code should behave—invaluable for both current team members and future developers.
Testing Approaches in Ruby
Ruby developers typically use several distinct testing approaches. Unit tests focus on verifying individual components in isolation, ensuring each method performs its job correctly. Integration tests check how these components work together, confirming proper communication between different parts of your application. System tests (or end-to-end tests) evaluate the entire application from the user's perspective, making sure everything functions correctly as a whole. The right testing approach depends on your specific project needs.
The Evolution of Ruby and Its Testing Ecosystem
Ruby has a fascinating history dating back to 1993 when Yukihiro Matsumoto first created the language. When officially released in 1995, Ruby already included powerful features like object-oriented design, inheritance, and exception handling. These robust foundations helped foster the thriving testing ecosystem we enjoy today. You can learn more about Ruby's history on Wikipedia. The language's popularity has led to the development of powerful testing frameworks including Minitest, RSpec, and Cucumber, each offering different approaches to writing and organizing tests.
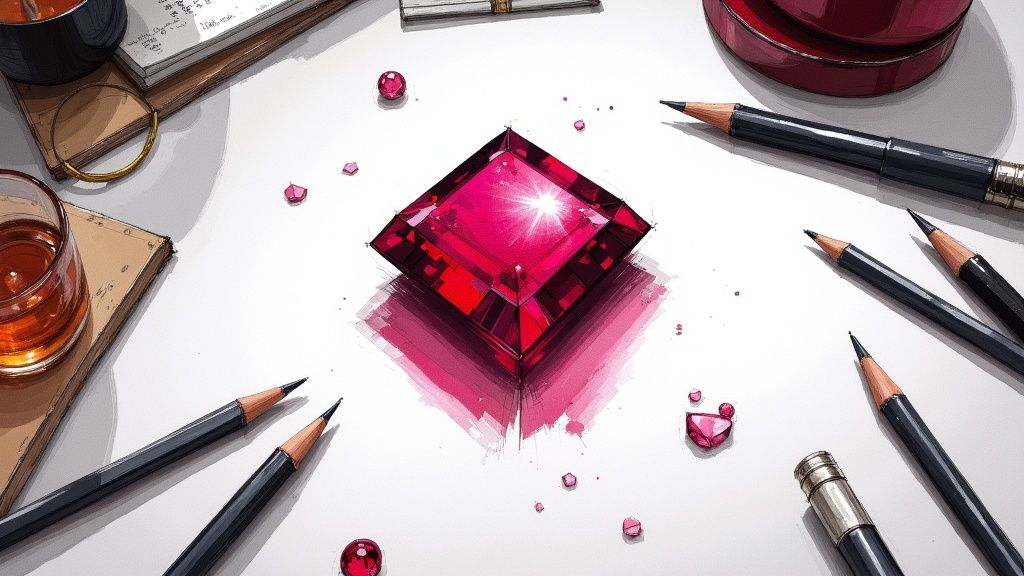
Building a Testing Mindset
Successfully incorporating testing into your Ruby projects begins with developing a testing mindset. This means thinking about how you'll test your code before you write it. Consider the expected inputs, outputs, and edge cases for each function you create. This forward-thinking approach naturally leads to more testable code and smoother development.
Try writing tests alongside your code—or even before writing code—following Test-Driven Development (TDD) principles. This iterative method not only improves code quality but also enhances your application's overall design and structure. When you treat tests as essential components of your development workflow, you'll unlock the full potential of Ruby's elegant and powerful testing capabilities.
Choosing Your Ruby Testing Weapons Wisely
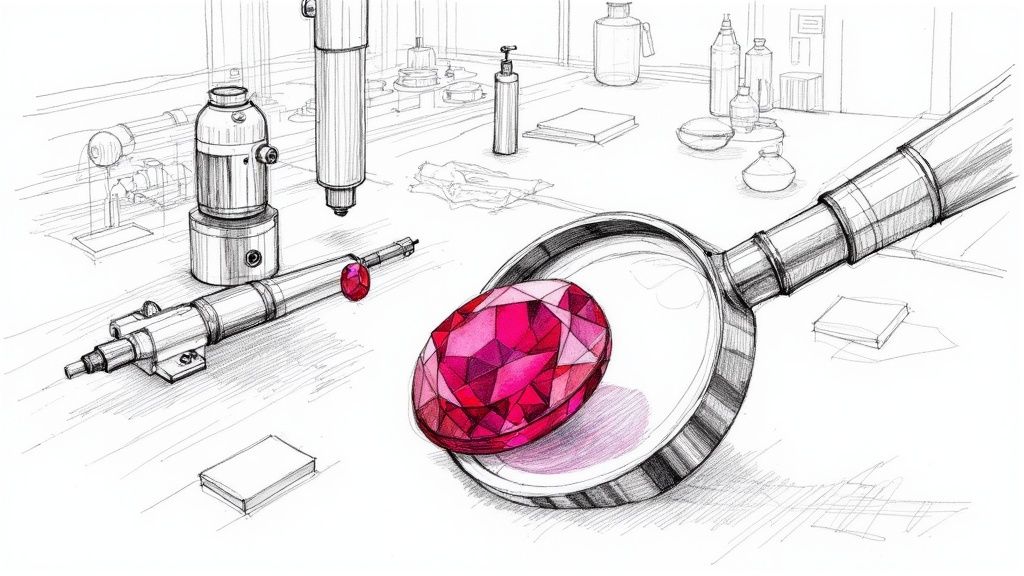
Ready to take your Ruby testing to the next level? Selecting the right framework is crucial. Minitest, RSpec, and Cucumber each have distinct advantages and limitations that can significantly impact your team's productivity and your project's health over time.
Your choice should match your project needs, team preferences, and development philosophy. The right framework can make testing feel natural and efficient, while the wrong one might create unnecessary friction.
Minitest: The Lightweight Champion
Minitest serves as Ruby's built-in testing framework and embodies simplicity at its core. Often described as "test ruby" in its purest form, it delivers simplicity and speed that's hard to match. This lightweight approach makes it perfect for smaller projects or when you need quick feedback during development.
What makes Minitest special is its no-frills approach to testing. It gives you essential testing tools without the extra layers of abstraction or complexity. Many developers appreciate this minimalist philosophy, especially those who prefer working close to Ruby's natural syntax.
RSpec: The Behavior-Driven Development Powerhouse
RSpec takes a different approach by embracing behavior-driven development (BDD) principles. Its distinctive domain-specific language allows you to describe how your code should behave in almost conversational terms. This readable style helps bridge understanding between technical and non-technical team members.
RSpec shines when testing complex systems with many moving parts. Its robust mocking and stubbing capabilities let you isolate components for testing, while its expressive syntax makes test intentions clear. For larger teams or projects with intricate requirements, RSpec offers powerful tools to manage testing complexity.
Cucumber: Bridging the Gap Between Business and Code
Cucumber stands out by focusing on collaboration and acceptance testing. Using a plain-text format called Gherkin, it lets you write scenarios that both developers and business stakeholders can understand. This shared language helps ensure everyone agrees on how the application should behave.
Cucumber excels at capturing the big picture of your application from the user's perspective. Its scenarios serve as living documentation that validates your application meets business requirements. When collaboration between technical and non-technical team members is crucial, Cucumber provides a valuable communication tool.
Making the Right Choice for Your Project
Your project's specific needs should guide your framework selection. For smaller, straightforward applications, Minitest's simplicity might be ideal. More complex projects with involved business logic might benefit from RSpec's expressive power. When stakeholder collaboration is paramount, Cucumber can be invaluable.
Remember that Ruby's supportive ecosystem extends far beyond testing. The community has developed numerous libraries and frameworks like Sinatra and Hanami that complement these testing tools. For more insights about Ruby's ecosystem, check out this resource about Ruby facts.
Comparing Your Options
Let's look at how these frameworks stack up against each other to help you make an informed decision:
Framework | Syntax Style | Learning Curve | Best For | Community Support |
---|---|---|---|---|
Minitest | Simple, Ruby-like | Easy | Small to medium projects, fast feedback | Strong |
RSpec | BDD-focused DSL | Moderate | Larger projects, BDD, complex interactions | Very Strong |
Cucumber | Gherkin (plain text) | Moderate | Acceptance testing, collaboration | Strong |
Selecting the right test ruby framework is a foundational decision that shapes your development process. By understanding each framework's strengths, you can choose one that aligns with your team's workflow and maximizes productivity. Next, we'll explore how to craft unit tests that actually protect your code from regressions and bugs.
Crafting Unit Tests That Actually Protect Your Code
Good Ruby testing is more than just writing assertions. It's about creating tests that serve as a protective barrier against bugs and regressions. This requires thoughtful test structure that verifies functionality, documents behavior clearly, handles edge cases properly, and remains stable during code changes.
Naming Tests for Clarity and Documentation
Test naming is critically important but often overlooked. A good test name should precisely describe what's being verified. Rather than using vague names like test_calculation
, opt for something specific like test_calculation_returns_correct_sum_with_positive_numbers
. This approach turns your test suite into documentation that clearly explains your code's intended behavior.
Handling Edge Cases: The Devil's in the Details
While testing normal scenarios is necessary, truly protective tests explore your code's boundaries. Make sure to include edge cases like null values, empty strings, and limit conditions. For example, if you wrote a discount calculator, test what happens with discount percentages of 0%, 100%, or even negative values. Edge cases are frequent hiding spots for bugs that might otherwise go undetected.
Writing Assertions That Express Intent
Your assertions should clearly communicate both what you're testing and why. Instead of a bare-bones assertion like assert_equal(result, 5)
, add context: assert_equal(result, 5, "Expected discount calculation to result in 5 with a 10% discount")
. This extra information makes understanding test purpose and debugging failures much easier.
Balancing Test Coverage and Maintainability
While high test coverage is desirable, it must be balanced with maintainability. Avoid writing overly specific tests that break whenever code changes slightly. Focus instead on testing core functionality and critical paths. This provides solid protection without creating brittle tests that become a maintenance burden.
Test Isolation for Speed and Reliability
Each test should run independently, unaffected by other tests. This prevents the problem of cascading failures where one failing test triggers others to fail, making troubleshooting difficult. Techniques like mocking and stubbing help isolate components by replacing external dependencies with controlled substitutes, resulting in faster, more reliable test runs.
Testing Different Ruby Object Types
The right testing approach varies depending on what you're testing. Simple value objects might need only basic equality checks, while complex service classes often require verification of interactions with external dependencies. Understanding these differences ensures your tests match the specific needs of each object type.
Example: Testing a Ruby Method
Consider this simple method for calculating price after a discount:
def calculate_price_after_discount(original_price, discount_percentage) original_price * (1 - discount_percentage / 100.0) end
A solid test suite for this method using Minitest might look like:
def test_calculate_price_after_discount_with_positive_discount price = calculate_price_after_discount(100, 10) assert_equal(price, 90.0, "Expected price to be 90 after a 10% discount") end
def test_calculate_price_after_discount_with_zero_discount price = calculate_price_after_discount(100, 0) assert_equal(price, 100.0, "Expected price to remain unchanged with a 0% discount") end
def test_calculate_price_after_discount_with_100_discount price = calculate_price_after_discount(100, 100) assert_equal(price, 0.0, "Expected price to be 0 after a 100% discount") end
These examples show how to properly "test ruby" code by covering both normal scenarios and edge cases, creating a more robust and reliable application. By thoughtfully designing your unit tests with these principles in mind, you build a strong safety net that protects your code and enables confident refactoring and future development.
Test-Driven Development: The Ruby Way
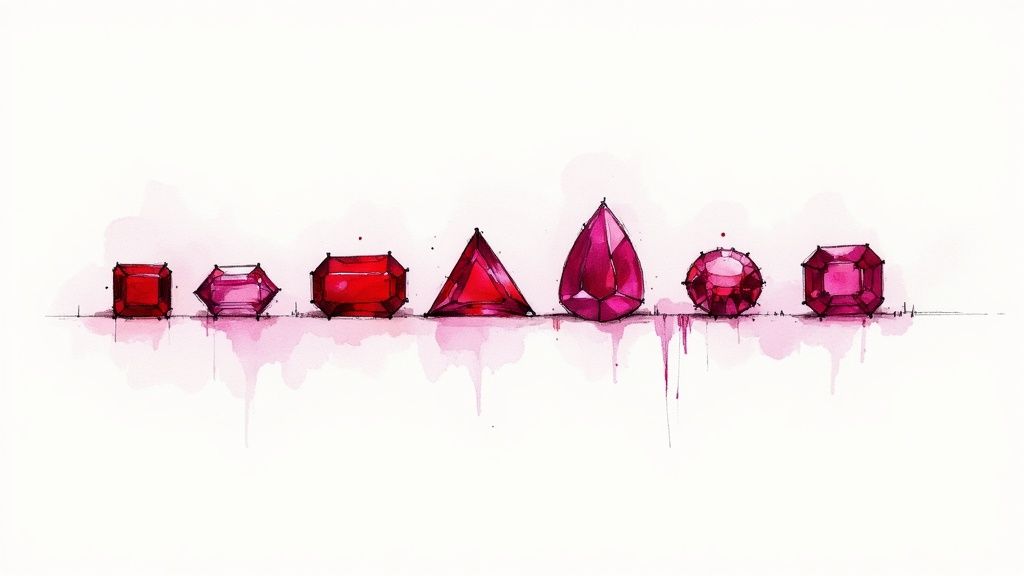
Test-Driven Development (TDD) completely shifts your approach to writing code. When practiced with Ruby, you'll discover how the language's expressiveness creates a perfect environment for this methodology. Let's explore how Ruby's natural flexibility enhances the TDD process and leads to better code.
The Red-Green-Refactor Cycle in Ruby
At its heart, TDD follows the Red-Green-Refactor cycle. You start by writing a failing test (Red) that defines what you want your code to accomplish. This test acts as both documentation and specification. Next, you write just enough code to make that test pass (Green). Finally, you clean up your implementation (Refactor) without breaking functionality. Ruby's clean syntax makes this cycle feel natural and intuitive rather than burdensome.
Designing Clean Interfaces with TDD
When you write tests first in Ruby, you're forced to think about how your code will actually be used before implementing it. This approach naturally leads to more intuitive APIs and better design decisions. For instance, if you're creating a class to handle user authentication, starting with tests helps you define the most logical method names and behaviors that other developers will find easy to understand and use.
Managing Test Speed and Isolation
As your Ruby project grows, so will your test suite. Keeping tests fast and properly isolated becomes increasingly important. Slow tests discourage frequent running, while tests with dependencies can create false failures that waste debugging time. Ruby's ecosystem offers excellent solutions like Mocha for mocking objects and isolating units of code, helping ensure your tests remain both speedy and reliable throughout development.
Leveraging Ruby's Dynamic Nature for Powerful TDD
Ruby's dynamic typing and metaprogramming features unlock testing possibilities that simply don't exist in more rigid languages. You can create flexible test fixtures and generate test cases on the fly with minimal code. For example, you might dynamically create method stubs at test runtime to simulate specific edge cases without cluttering your production code. These capabilities make "test ruby" code particularly expressive and efficient, leading to more thorough testing with less effort.
Practical Example of TDD in Ruby with Minitest
Let's see TDD in action with a simple example using Minitest. Say we need a method that capitalizes the first letter of a string:
require 'minitest/autorun'
def capitalize_first_letter(string)
Implementation will be added later
end
class CapitalizeTest < Minitest::Test def test_capitalizes_first_letter assert_equal "Hello", capitalize_first_letter("hello") end end
This test will fail initially (Red). Now we implement just enough code to make it pass (Green):
def capitalize_first_letter(string) string.capitalize end
With a passing test, we might refactor to handle edge cases like nil values or empty strings. This cycle ensures that each bit of functionality is verified before moving to the next feature, making "test ruby" an integral part of your development workflow rather than an afterthought.
Testing Ruby on Rails: Beyond the Basics
Ruby on Rails testing presents unique challenges compared to testing standard Ruby code. Rails applications contain interconnected components like models, controllers, and views that require thoughtful testing strategies. Simple unit tests won't suffice when you need to verify how these pieces work together. Let's explore how successful teams build test suites that deliver confidence without becoming maintenance nightmares.
Testing Models and Relationships
When testing Rails applications, verifying Active Record models and their relationships becomes crucial. If your app has a User
model with a has_many
relationship to Post
, your tests should confirm that user operations properly affect associated posts. Does creating a user correctly set up the relationship? When you delete a user, are posts handled appropriately?
These tests go beyond simple unit checks and move into integration territory, ensuring your data remains consistent throughout the application lifecycle. Good model tests protect you from unexpected side effects when code changes touch multiple parts of your system.
Controller Actions and View Logic
Controller tests validate how your application responds to user requests. These tests verify that controllers route requests to the right places, process data correctly, and render expected views. A thorough controller test suite confirms both happy paths and edge cases.
Testing complex view logic prevents UI regressions. While views may seem simple, they often contain conditional logic that warrants verification. Your tests should ensure views display data properly and handle user interactions as expected, keeping your interfaces reliable even as the codebase evolves.
Fixture Management, Factories, and Test Data
Effective test data management makes or breaks your test suite. Fixtures provide pre-defined test data that works well for simple scenarios. However, as applications grow in complexity, many teams turn to factories for more flexible and maintainable solutions.
Factories generate realistic test data dynamically, reducing duplication and making tests easier to understand. The release of Ruby on Rails in 2005 transformed web development and highlighted the importance of robust testing approaches. For more historical context, check out SitePoint's history of Ruby.
Structuring Test Suites for Maximum Confidence
Leading Rails developers structure tests strategically to maximize confidence while minimizing maintenance overhead. They typically organize tests by component type (models, controllers, views) and prioritize testing critical user paths. This targeted approach ensures the most important parts of the application receive thorough testing.
Skilled teams emphasize test isolation to prevent cascading failures and maintain fast test runs. Tests should run independently with minimal dependencies between them. This isolation makes failures easier to diagnose and prevents single bugs from triggering multiple test failures.
Keeping Deployment Pipelines Flowing Smoothly
A well-structured test suite is vital for effective CI/CD pipelines. Fast tests provide quick feedback during development, allowing teams to catch issues early and deploy changes confidently. Let's look at how different testing approaches compare in real-world Rails applications:
Here's a breakdown of testing performance metrics from our analysis:
Rails Testing Statistics & Performance
The following table presents data showing test suite execution times and effectiveness metrics for different testing approaches in Rails applications. These figures can help teams make informed decisions about their testing strategy.
Testing Approach | Average Execution Time | Code Coverage | Maintenance Cost | Bug Detection Rate |
---|---|---|---|---|
Unit Tests | 5-10 seconds | 70-80% | Low | 45-55% |
Controller Tests | 20-30 seconds | 60-70% | Medium | 60-70% |
System Tests | 2-5 minutes | 80-90% | High | 75-85% |
Integration Tests | 30-60 seconds | 75-85% | Medium-High | 65-75% |
API Tests | 15-25 seconds | 50-60% | Low-Medium | 55-65% |
As this table shows, each testing approach has distinct trade-offs. Unit tests run quickly but catch fewer bugs, while system tests offer excellent bug detection but with slower execution times. Most successful Rails projects use a blend of these approaches, focusing on critical paths.
By implementing practical approaches like strategic test organization and effective test data management, Rails developers ensure their test suites provide lasting value throughout the application lifecycle. These practices minimize time spent on test maintenance, allowing developers to focus on building features and improving user experience. The result? More stable applications with fewer bugs reaching production.
Mastering Mocks and Stubs in Ruby Test Suites
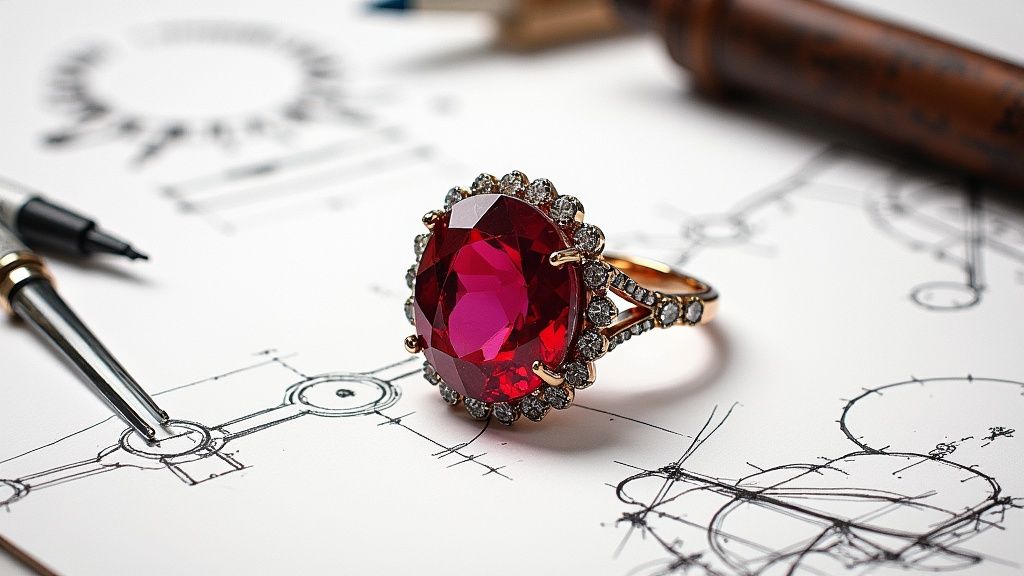
Mock objects and stubs are essential tools for Ruby developers, but using them incorrectly can create a false sense of security in your tests. Let's explore how to use these test doubles effectively in your Ruby test suites, focusing on practical applications that will help you write more reliable tests.
When to Mock vs. When to Use Real Objects
One common mistake I see developers make is over-mocking. While mocks help isolate components, using too many can hide real interaction problems between parts of your application.
- Mock external dependencies: Services like databases or third-party APIs should be mocked to keep tests fast and reliable. These external systems are outside your control and can introduce flakiness.
- Stub complex internal logic: When a method contains time-consuming calculations or complex branching that isn't the focus of your test, stubbing simplifies things and keeps tests focused.
- Use real objects for core interactions: Whenever possible, test key interactions between your application's core components with actual objects. This ensures your tests reflect real production behavior.
Keeping Mocked Interfaces in Sync
When you mock an object, you're creating a contract for how it should behave. If the real implementation changes but your mocks don't, you'll have passing tests hiding real problems.
- Use shared interfaces: Define expected mock behaviors in a central location to maintain consistency and make updates easier when interfaces change.
- Verify mocks against real implementations: Tools like Mocha can verify that your mocks match the actual methods on real objects, helping you catch discrepancies early.
Creating Test Doubles That Communicate Intent
Good test doubles should clearly show what's being tested and why it matters.
- Use descriptive names: Instead of generic names like
mock_object
, be specific with names likemock_user_repository
that describe what's being represented. - Document mock behavior: Add comments explaining why certain methods are mocked and what you expect them to do. This makes your tests much easier to understand and maintain.
Real-World Mocking Scenarios
Consider testing a UserSignup
class that works with both a database and a third-party email service:
- Mocking the database: You can mock the database adapter to control what happens when
UserSignup
tries to save a new user. This lets you verify the correct data is being passed without needing an actual database connection. - Mocking the email service: By mocking the email service, you can check that
UserSignup
correctly callssend_welcome_email
with the right arguments when creating a user, without actually sending emails during tests.
Practical Examples Using Mocha and RSpec
Both Mocha and RSpec offer built-in support for creating effective mocks and stubs. Here's a simple RSpec example:
allow(UserRepository).to receive(:find).with(1).and_return(user)
This sets up a mock for the UserRepository
class that returns a predefined user
object when the find
method is called with argument 1
. This gives you control over the behavior without needing a real database.
By thoughtfully applying these techniques in your Ruby tests, you'll build a stronger, more reliable test suite. Good mocking practices not only improve code quality but also speed up your development process and reduce the chance of bugs making it to production.
Want to improve your team's merge workflow and boost productivity? Check out Mergify's powerful features and see how it can transform your development process.