Ruby Unit Testing: Essential Developer Tips
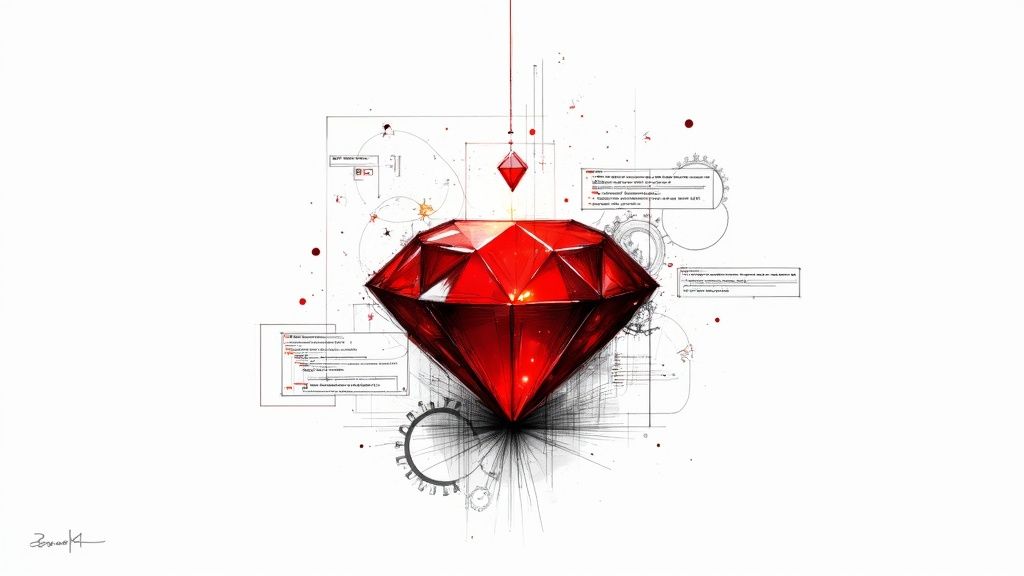
Breaking Into Ruby Unit Testing Without the Overwhelm
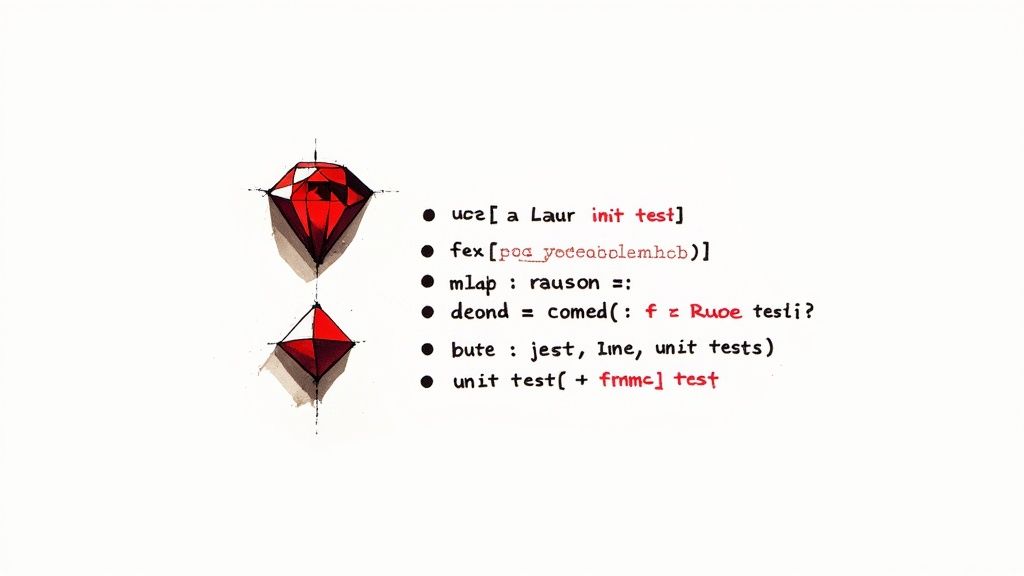
Starting with Ruby unit testing can feel intimidating, but for experienced Ruby developers, it's a core practice. It's all about building confidence incrementally, beginning with that very first test. This section will guide you through practical methods seasoned developers use, making testing an integral part of their workflow.
Overcoming Initial Testing Hurdles
Many developers struggle with testing anxieties at first. They may worry about writing "perfect" tests right away or feel daunted by the apparent complexity. The key is to start small and prioritize progress. Begin by testing a single method with a simple assertion. This helps you grasp the fundamentals before tackling more complex scenarios. Remember, tests are iterative. You can always refine and improve them as your code changes.
Choosing the Right Framework: Test::Unit
Selecting the right testing framework is crucial. Ruby unit testing has evolved, with robust frameworks like Test::Unit offering a comprehensive set of assertions and test suites that integrate seamlessly into Ruby applications. This allows developers to create maintainable and robust tests, ensuring software components function as expected. Test::Unit's prevalence in Ruby development is evident in many Ruby resources. You can learn more about the uses of Test::Unit and other Ruby testing practices here. Other frameworks like Minitest and RSpec offer different approaches, each with its advantages. The ideal choice depends on your project's needs and your team's preferences.
Building a Testing Routine That Sticks
Maintaining testing discipline can be tough, particularly when deadlines approach. A good strategy is to integrate testing directly into your development process. Consider Test-Driven Development (TDD), writing tests before the code. This ensures comprehensive test coverage and guides your design decisions. Make testing a habit by running your test suite regularly, ideally after every code change. This catches errors early, preventing them from becoming bigger issues.
Writing Effective Tests: Beyond the Basics
As you become more experienced, explore advanced techniques beyond basic assertions. This includes handling asynchronous code, mocking external services, and managing complex application states. These techniques enable robust testing of intricate interactions and ensure application reliability under various conditions. This means you can confidently handle challenging scenarios, knowing your tests are thoroughly evaluating your code. Mastering Ruby unit testing is a journey. By starting small, building good habits, and continuously learning, you can transform testing from a source of stress into a powerful tool for building high-quality Ruby applications.
Navigating Ruby's Testing Framework Ecosystem
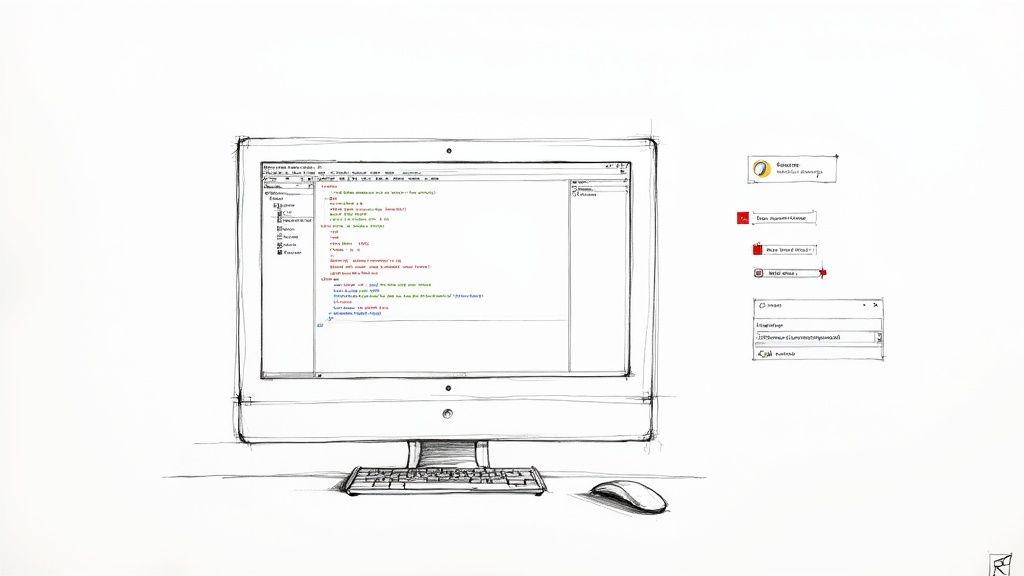
A deep understanding of Ruby's testing frameworks is crucial for efficient and effective Ruby unit testing. This exploration clarifies the differences between popular choices like Test::Unit, Minitest, and RSpec, providing insights for developers. We'll delve deeper than simple syntax comparisons, exploring each framework's strengths in specific scenarios and helping you choose the right one for your team.
Test::Unit: The Built-In Baseline
Test::Unit, often referred to as Test
, is Ruby's built-in testing framework. Its readily available nature, without requiring extra gems, makes it an attractive option. It prioritizes simplicity and minimal dependencies. Test::Unit follows a classic xUnit style, using assertions like assert_equal(expected, actual)
to verify outcomes. This straightforwardness makes it easy to begin testing.
Minitest: Compact Yet Powerful
Minitest also comes standard with Ruby and offers a modern, flexible approach. It supports both xUnit and Behavior-Driven Development (BDD) styles. This versatility allows developers to select the style that best fits their project or even combine them. Minitest is known for its speed and low overhead, making it ideal for fast-paced development.
RSpec: The BDD Champion
RSpec stands out with its focus on BDD. It promotes describing the desired behavior of code using a natural language-like syntax. For example, you might see expect(actual).to eq(expected)
. This readability can improve communication between developers and stakeholders. RSpec's extensive features, like mocking and stubbing, are well-suited for complex projects. However, there is a steeper learning curve.
Selecting the Ideal Framework
Choosing the right framework depends on several factors. Test::Unit or Minitest might be sufficient for smaller projects or those already using the standard library. Larger projects with complex interactions may benefit from RSpec's BDD approach and features. Team experience and existing codebases also significantly influence the decision. Switching frameworks mid-project can be costly.
Comparing Framework Features
To help you choose the best framework, the following table summarizes their key differences:
Framework | Syntax Style | Learning Curve | Mocking Support | Best For | Community Size |
---|---|---|---|---|---|
Test::Unit | xUnit | Easy | Basic | Small to medium projects, standard library users | Large |
Minitest | xUnit/BDD | Easy to Moderate | Moderate | Fast feedback, flexible style | Large |
RSpec | BDD | Moderate to Steep | Extensive | Complex projects, BDD practitioners | Very Large |
While these frameworks share similar objectives, their methodologies differ. Understanding these nuances helps you choose the best tool for your Ruby unit testing strategy. Experimenting with each can be invaluable. The best fit varies between teams. Consider project size, complexity, and team preferences for a smoother testing process and robust, maintainable Ruby applications.
Test-Driven Development That Actually Makes Sense
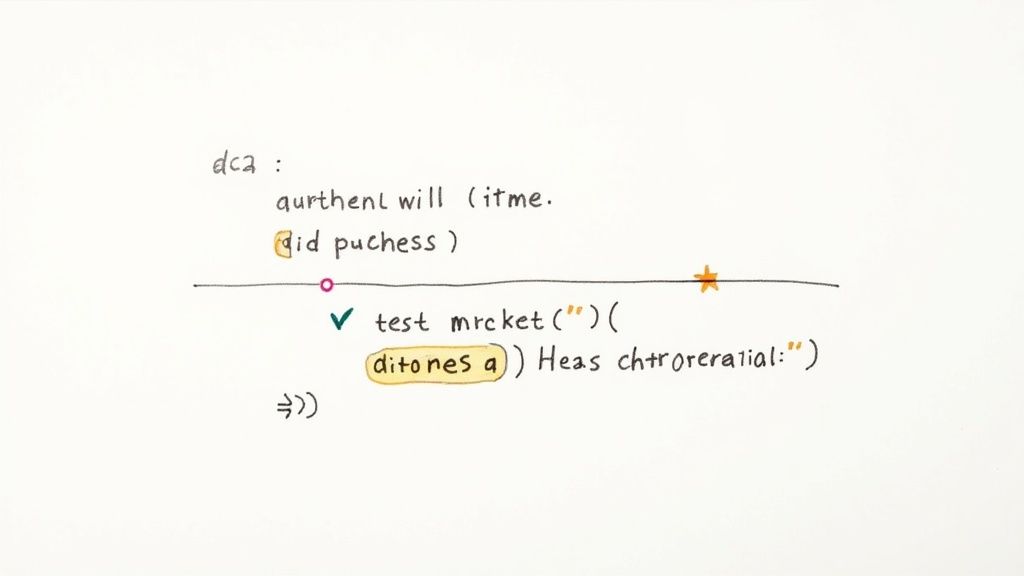
Beyond the theory, how does Test-Driven Development (TDD) truly benefit Ruby projects? This section breaks down the Red-Green-Refactor cycle with practical Ruby examples, demonstrating how writing tests first leads to cleaner, more maintainable code. You’ll see how TDD naturally guides better design decisions and helps prevent feature creep.
The Red-Green-Refactor Cycle in Action
TDD revolves around a simple, iterative cycle: Red, Green, Refactor. This process provides a clear path for Ruby unit testing.
First, write a failing test (Red). This test defines the desired behavior of a specific piece of code. Next, write the minimum code necessary to pass the test (Green). Finally, improve the code while ensuring the tests remain green (Refactor). This cycle repeats, building functionality incrementally and with confidence.
For example, imagine implementing a method to calculate the total price of items in a shopping cart. In TDD, you would first write a test that asserts the calculate_total
method returns 0 for an empty cart. This test will initially fail (Red).
Then, you’d implement the calculate_total
method, returning 0 if the cart is empty. Now the test passes (Green). Finally, you might refactor the method to handle non-empty carts, always ensuring the tests remain Green.
TDD and Better Design Decisions
TDD encourages modular and testable code. By writing tests before the code, you're forced to consider how the code will be used and how it interacts with other components. This leads to better design choices and promotes loose coupling.
Additionally, having a comprehensive suite of tests provides a safety net for refactoring. This allows you to improve the design without fear of breaking existing functionality. This means that TDD can improve code quality by focusing development on fulfilling specific requirements captured by tests.
By doing so, it naturally guides you toward well-structured code. This is especially helpful for large projects where maintaining design integrity is a continuous challenge.
Practical TDD in Ruby Projects
The practice of Test-Driven Development (TDD) is widely adopted in Ruby projects, including those built with Ruby on Rails. TDD involves writing tests before implementing code, ensuring that each component meets its specifications. Rails offers a robust testing framework that supports TDD, enabling developers to run tests in isolation using a dedicated test database.
This approach helps maintain high-quality codebases by preventing regressions. Explore this topic further here. However, successful Ruby teams recognize that TDD is not a dogma. They adapt TDD principles to different project types and needs.
For instance, they might use TDD extensively for core business logic while adopting a more pragmatic approach for UI testing.
Testing Challenging Scenarios
TDD is not without its challenges. Certain aspects of a codebase, such as interactions with external APIs or complex database queries, can be difficult to test. In these situations, focusing on testing the core logic of your code and using mocking techniques to isolate dependencies can streamline the process. Remember, even partial test coverage is better than none.
Building robust and maintainable Ruby applications requires a strong commitment to testing. TDD offers a powerful approach, fostering cleaner code, guiding better design decisions, and boosting developer confidence. By understanding and applying the Red-Green-Refactor cycle, Ruby developers can transform testing into an integral part of their workflow and deliver high-quality software consistently.
Advanced Ruby Testing Techniques That Solve Real Problems
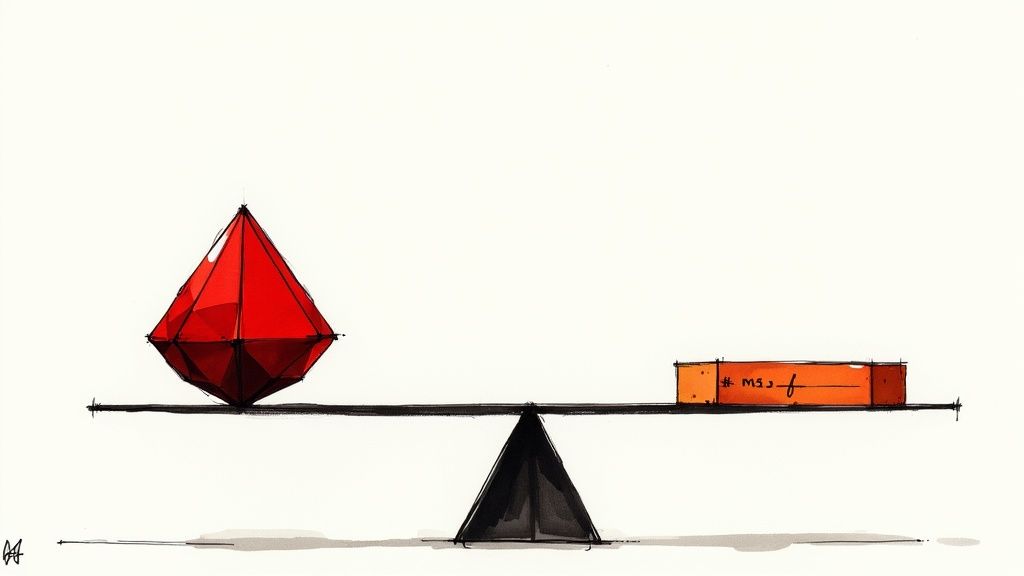
Mastering Ruby unit testing goes beyond the basics. It means tackling the truly complex scenarios. This section explores how seasoned Ruby developers approach testing in challenging situations, including asynchronous operations, external APIs, and complex application state. We'll dive into practical, real-world examples, showcasing effective testing strategies for everything from time-sensitive functions to database interactions and third-party service integrations. Our focus? Techniques that actually help you find and fix bugs.
Testing Asynchronous Code
Asynchronous operations are a staple of modern Ruby applications, but they present unique testing hurdles. Because these operations run outside the main thread, typical assertions can miss their behavior entirely. This is where tools like concurrent-ruby become invaluable. Concurrent-ruby provides mechanisms that simplify testing by allowing you to wait for asynchronous operations to complete before your tests make their assertions.
- Use
wait
methods to ensure asynchronous operations have finished. - Focus your tests on the final application state and any side effects.
- Consider mocking external dependencies to isolate the asynchronous behavior you're testing.
For example, imagine testing a background job that updates your database. You would use wait
to ensure the job completes before verifying the database changes. This guarantees your test accurately reflects the asynchronous workflow.
Stubbing and Mocking External APIs
Working with external APIs introduces dependencies that can slow down your tests and make them unreliable. Stubbing and mocking offer solutions by simulating these external services.
- Stubbing: This technique replaces a method with a predefined return value. It's particularly helpful for testing specific code paths without needing to call the actual API.
- Mocking: Mocking provides a more complete simulation. It lets you verify exactly how your code interacts with the API, checking that the correct methods were called with the expected arguments.
Suppose your code sends data to a third-party analytics service. Stubbing lets you bypass the API call and focus on testing your data formatting logic. Mocking, on the other hand, lets you confirm that the send_data
method was called with the correct data and API key.
Managing Complex State
Applications with intricate internal states demand careful test design. State changes can trigger unforeseen side effects, making thorough testing essential.
- Isolate your tests to prevent state from leaking between them.
- Use setup and teardown methods to reset the application state before each test.
- Consider using factories or fixtures to create consistent initial states for your tests.
Think of testing a game with various levels and player statistics. Each test should begin with a well-defined game state. This ensures reliable and predictable results by giving you control over the context of each test and preventing interference between different parts of the test suite.
Balancing Test Coverage and Performance
Comprehensive testing is paramount, but it’s also important to keep test execution time in check. Long test suites can hinder the development process. Optimizing test execution time has led to tools like test impact analysis. Datadog’s Intelligent Test Runner, for example, uses a Ruby library that can reduce testing time by up to 50%. This library leverages Ruby's built-in Coverage
module and TracePoint
API to efficiently track test impact, allowing developers to significantly speed up their test suites by skipping unnecessary tests and making continuous testing a more practical reality. Explore this topic further here.
Finding the balance between comprehensive test coverage and practical considerations is key to successful Ruby unit testing. Advanced techniques, such as those for testing asynchronous code, stubbing and mocking APIs, and managing complex application state, are invaluable for building robust and maintainable Ruby applications. While comprehensive coverage is the ultimate goal, practical concerns like test run time and maintenance overhead must also be addressed to create a truly efficient and effective testing strategy.
Supercharging Your Testing Workflow With Modern Tools
Effective Ruby unit testing isn't just about picking the right framework. It's about having the right tools, too. Top Ruby developers know a well-configured testing environment is key to boosting productivity. This exploration dives into practical setups that provide rapid feedback, intuitive debugging, and clear test visualizations, helping you create better tests, faster. We'll also discuss which editor extensions truly help and which ones just add unnecessary complexity.
IDE Integrations: A Game Changer for Ruby Unit Testing
Integrated Development Environments (IDEs) offer powerful tools to streamline testing. Features like built-in test runners, debuggers, and visual feedback significantly boost efficiency.
- Built-in Test Runners: Run tests directly within the IDE. No more external terminals or commands needed. This integrated approach means faster test execution and quicker feedback.
- Debuggers: Step through tests line by line to find and fix errors quickly. Interactive debugging helps pinpoint issues efficiently.
- Test Visualizations: Graphical displays of test results make understanding test coverage and spotting problems much easier.
RubyMine is a great example of an IDE with advanced features tailored for Ruby unit testing. Recently, RubyMine has improved the unit testing experience by adding features to view and explore results. The Run tool window displays progress and results, letting you navigate stack traces, filter successful tests, and sort tests by how long they take. This makes debugging and test analysis much more efficient. RubyMine can also export test results in formats like HTML or XML for easy sharing. Find more detailed statistics here.
Editor Extensions: Choosing the Right Helpers
While IDEs provide comprehensive tools, editor extensions can add even more to your testing workflow. However, choose wisely. Too many extensions can clutter your editor and slow it down.
- Test Snippets: Quickly create tests with snippets that generate common test structures. This helps avoid repetitive typing and keeps a consistent style.
- Linting for Tests: Use linters made for test code to catch errors and enforce best practices early on, keeping your test code clean.
- Test Coverage Visualizers: See test coverage results directly within your editor for instant feedback, helping you understand how well your tests cover your code.
Pick extensions that directly address your testing needs. Don't add tools just because. This helps you avoid a cluttered workspace and maintain an efficient workflow.
Building a Productive Testing Setup
The best testing environments combine IDE features, smart editor extensions, and external tools working together. This creates a smooth workflow that helps you write high-quality tests. This setup can change based on project size and team preferences.
- Start With a Strong IDE Foundation: A capable IDE like RubyMine is essential for an efficient testing process.
- Choose Editor Extensions Strategically: Pick extensions that address your specific needs, such as test snippets, linting, and coverage visualization.
- Integrate with External Tools: Connect your testing environment to tools like CI/CD pipelines for automated testing and reports.
By carefully choosing and setting up your testing environment, you create a productive setup that lets you deliver top-notch Ruby code consistently. Minimize friction and maximize the value of your Ruby unit testing. This allows you to write better tests, find bugs early, and maintain confidence in your codebase.
Making Ruby Tests Shine in Your CI/CD Pipeline
Successfully running Ruby unit tests locally is a great first step. But integrating them into your Continuous Integration/Continuous Deployment (CI/CD) pipeline is where real confidence comes from. This catches problems before they impact users. This section explores how to set up Ruby testing on popular CI/CD platforms like GitHub Actions, CircleCI, and GitLab CI, providing practical examples you can put to use right away. We'll also look at strategies for faster tests, running tests in parallel, and tackling those annoying intermittent failures that plague CI environments.
Integrating Ruby Tests With Popular CI/CD Platforms
Setting up Ruby unit testing in your CI/CD pipeline is easier than you might think. The general approach looks like this:
- Define a Testing Stage: Create a dedicated testing stage in your pipeline that runs after your build stage. Most CI/CD platforms support this.
- Install Dependencies: Make sure your CI environment has the right Ruby version and gems. Using a
Gemfile
andbundle install
keeps your dependencies consistent. - Run Your Test Suite: Execute your tests using your testing framework's command-line runner (e.g.,
rake test
,rspec
). - Report Results: Configure your CI/CD platform to clearly display the test results. This gives you quick feedback on whether your tests passed or failed.
Here’s a simple example of a .gitlab-ci.yml
file for GitLab CI:
test: image: ruby:latest script: - bundle install - rspec
This basic configuration shows how simple it is to add testing to your CI/CD workflow. More complex setups might need extra configuration, like setting up a database or using mocks for external services.
Optimizing Test Run Time in Your CI/CD Pipeline
Long test runs slow down your entire pipeline. Optimizing test performance is key for fast feedback and efficient deployments. Here's what you can do:
- Parallelization: Run tests at the same time across multiple machines or processes. This significantly cuts down on overall test time. Gems like parallel_tests can help.
- Targeted Testing: Run only the tests related to the code that has changed. This is sometimes called test impact analysis and requires tools that can track code changes and their corresponding tests.
- Caching: Cache dependencies and test results between pipeline runs. This avoids redundant work and speeds up future builds.
Handling Intermittent Failures in CI
Intermittent failures are a real headache in CI/CD. These are tests that sometimes pass and sometimes fail even when the code hasn't changed. They create uncertainty and make it hard to trust your test suite. Here are a few ways to address them:
- Retry Failed Tests: Set up a system to automatically retry failed tests a few times. This often catches temporary glitches.
- Isolate Problematic Tests: If you have tests that consistently fail intermittently, isolate them. This keeps them from blocking the entire pipeline while you investigate the cause.
- Improve Test Isolation: Ensure your tests are independent of each other. Shared state or external dependencies can cause unpredictable behavior.
Transforming Tests Into Quality Gates
Effective CI/CD pipelines treat tests as quality gates. If the tests fail, the pipeline stops automatically, preventing bad code from reaching production. This increases team confidence and reduces the risk of deploying bugs. Integrating unit testing within CI/CD provides significant benefits. For example, platforms like GitLab display unit test results directly within merge requests and pipeline details. This feature usually uses the JUnit format, which tools like the RSpec JUnit Formatter can easily generate. You can configure your .gitlab-ci.yml
file to include these reports, letting developers quickly see test failures without wading through job logs. Learn more about this here. By implementing strong Ruby unit testing in your CI/CD pipeline, you go beyond basic verification. You create a system that actively prevents bugs and ensures code quality. This transforms tests from potential roadblocks into valuable tools that guarantee a smoother, more reliable path to production.
Ruby Test Performance Metrics
This table presents typical performance improvements achieved through different testing optimization techniques.
Optimization Technique | Average Speed Improvement | Implementation Complexity | Best Suited For |
---|---|---|---|
Parallelization | 2x - 10x | Medium | Large test suites, CI environments |
Targeted Testing | 1.5x - 5x | High | Projects with good test coverage, frequent changes |
Caching | 1.2x - 3x | Low | Projects with stable dependencies |
The table above summarizes the potential benefits of various test optimization techniques. While parallelization offers the most significant speed improvements, it comes with moderate implementation complexity. Targeted testing can also be very effective, but it's more complex to set up. Caching is the easiest to implement and offers good gains, particularly for projects with stable dependencies.
Ruby Unit Testing Best Practices That Stand the Test of Time
Building a sustainable and effective Ruby unit testing suite goes beyond simply writing tests. It involves adopting practices that deliver long-term value. This section draws insights from successful, long-running Ruby projects to explore key elements in creating a robust and maintainable test suite. We'll cover practical approaches to organization, naming conventions that promote collaboration, and strategies for preventing cascading test failures.
Organizing Your Tests for Clarity and Maintainability
Well-organized tests are easier to understand, navigate, and maintain. A clear structure allows you to quickly locate specific tests, improving readability and simplifying updates.
- Mirror Your Code Structure: Organize tests to mirror the structure of your application code. This makes finding tests related to specific modules or classes straightforward. For example, a
ShoppingCart
class would have a correspondingshopping_cart_test.rb
test file. - Use Descriptive File Names: Choose file names that clearly indicate what's being tested. Avoid generic names like
test_helper.rb
unless the file genuinely contains helper functions used across multiple tests. - Group Related Tests: Within each test file, group related tests using
describe
blocks (RSpec) or custom modules (Minitest). This maintains organization and logical flow.
Naming Conventions That Foster Collaboration
Consistent naming conventions enhance team understanding of your tests. A unified naming style streamlines the process of finding and working with tests, promoting efficient collaboration.
- Descriptive Test Names: Use descriptive names that clearly communicate the purpose of each test. Avoid vague names like
test_1
ortest_method
. Opt for names that explain the specific scenario being tested.test_calculate_total_for_empty_cart
is far more informative thantest_total
. - Follow a Consistent Style: Choose a consistent naming style, whether underscores (
test_calculate_total
) or camel case (testCalculateTotal
), and adhere to it throughout the project. Consistency is paramount.
Isolating Tests to Prevent Cascading Failures
Cascading test failures, where one failing test triggers failures in other unrelated tests, complicate debugging. Isolating tests helps pinpoint the root cause of errors efficiently.
- Avoid Shared State: Ensure tests don't rely on shared state or data. Each test should begin with a clean slate and conclude without altering shared data that could impact other tests.
- Use Setup and Teardown Methods: Use setup methods (e.g.,
before
in RSpec orsetup
in Minitest) to initialize the test environment. Use teardown methods (after
orteardown
) for cleanup, ensuring a fresh start for subsequent tests. This is especially important for database interactions or external resources.
Refactoring Tests and Managing Legacy Code
Knowing when and how to refactor tests is vital for a healthy test suite. Overly complex tests require the same care as production code.
- Treat Tests Like Production Code: Apply the same clean code principles to your tests. If a test is difficult to understand, it likely needs refactoring.
- Refactor When Tests Become Brittle: Tests frequently breaking due to minor code changes suggests tight coupling to implementation details. Refactoring can increase their resilience.
- Balance Refactoring and Rewriting: Sometimes rewriting a test is more efficient than refactoring an overly complex one, especially if the test is outdated or the tested logic has significantly changed.
Working with legacy code presents unique challenges, often lacking comprehensive test coverage.
- Prioritize Testing Critical Paths: When dealing with untested legacy code, prioritize writing tests for the most critical parts of the codebase to maximize impact.
- Incrementally Add Tests During Refactoring: Introduce tests while refactoring legacy code. As you update the code, add tests for both new and existing functionalities to gradually improve coverage.
Measuring Testing ROI and Focusing on Value
Testing is an investment, and maximizing its return requires focusing on high-value areas.
- Prioritize Business-Critical Functionality: Concentrate on testing application parts essential for business operations to prevent severe issues.
- Balance Coverage with Practicality: While high test coverage is desirable, 100% coverage isn't always practical or necessary. Testing some edge cases may offer limited value compared to the cost.
- Monitor and Adjust Your Testing Strategy: Regularly evaluate your testing approach and make adjustments based on its effectiveness. Track metrics like bug rates and test execution time to optimize value.
By implementing these best practices, you can create a Ruby unit testing suite that not only catches bugs but also improves development efficiency. These strategies contribute to a sustainable testing approach, enabling teams to deliver high-quality Ruby code reliably.
Ready to optimize your merge workflow and CI/CD pipeline? Discover how Mergify can help your team enhance efficiency and reduce CI costs.