Ruby Testing Mastery: Boost Reliability
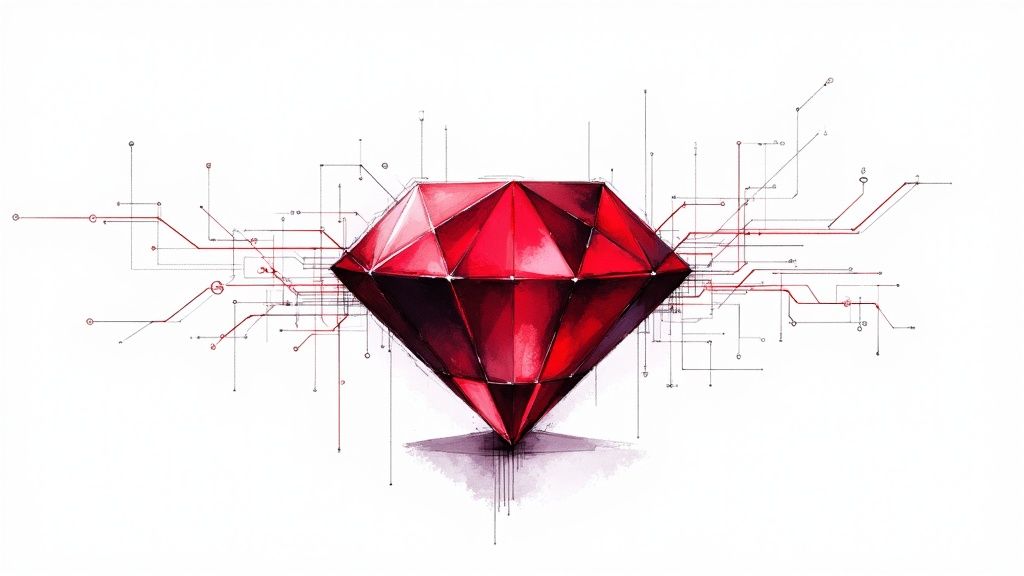
The Ruby Testing Mindset: Beyond the Basics
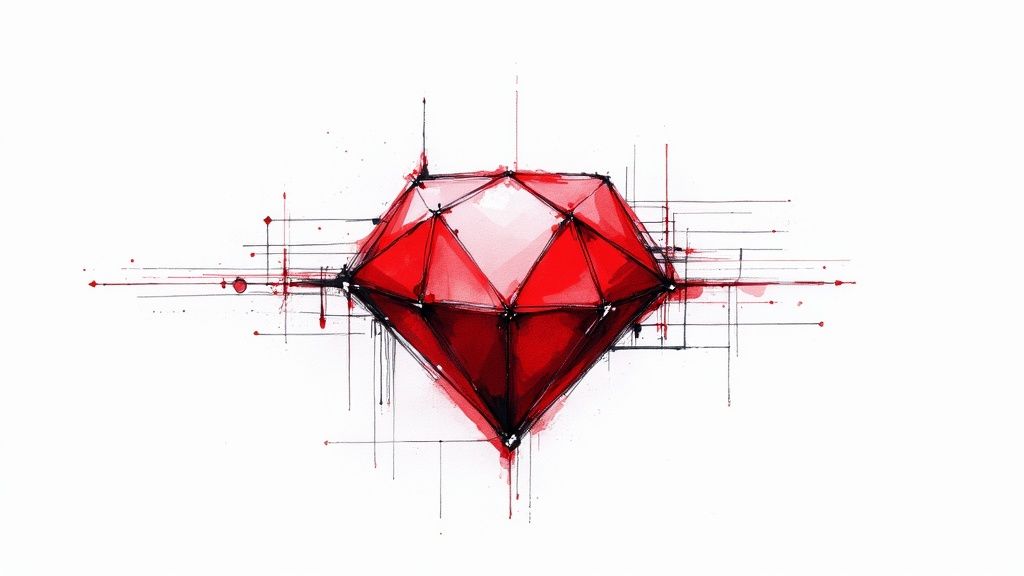
Effective Ruby testing goes beyond simply verifying functionality. It's integral to building robust and maintainable applications from the ground up. It's a core philosophy within the Ruby ecosystem. This mindset prioritizes designing testable code, proactively preventing bugs, and promoting clarity.
Embracing the Dynamic Nature of Ruby
Ruby's dynamic typing offers unique testing advantages. Unlike statically-typed languages that catch type errors during compilation, Ruby relies heavily on runtime testing. This makes testing crucial for identifying potential issues before they impact users. This flexibility allows for rapid development, but thorough testing is essential to mitigate unforeseen consequences.
Consider a method expecting an integer but receiving a string. A statically-typed language like Java would immediately flag this error. Ruby, however, would attempt execution, potentially leading to unexpected behavior. This highlights the vital role of well-designed Ruby tests.
These tests validate assumptions about data types, input ranges, and method interactions, ensuring predictable application behavior. They act as a safety net, catching errors that might otherwise slip through the cracks.
Essential Testing Vocabulary
Understanding key terminology is fundamental to effective Ruby testing. Unit tests are the foundation, verifying individual components in isolation. Integration tests examine how different parts of your application interact. System tests, sometimes called end-to-end tests, assess the entire application flow, including user interaction and database access.
This layered approach provides comprehensive coverage, pinpointing the source of any issues. A failing unit test indicates a problem within a specific method, while a failing integration test suggests a flaw in inter-module communication. This granularity is essential for quick debugging and confident development.
The Power of Community and Frameworks
Ruby's active community and robust frameworks significantly contribute to the language's testing capabilities. Ruby on Rails, known for its convention-over-configuration philosophy, provides a strong testing foundation. Rails boasts impressive statistics on GitHub: over 53,000 stars, 21,200 forks, and 4,700 contributors.
This vibrant community encourages collaboration, sharing best practices, and refining testing methodologies. Frameworks like RSpec and Minitest streamline the testing process. These frameworks offer structured approaches to writing tests, simplifying the management of complex test suites and ensuring consistent code quality. They empower developers to write concise, expressive tests covering a wide range of scenarios. This strong foundation sets the stage for mastering specific Ruby testing frameworks and techniques.
Choosing The Right Ruby Testing Framework
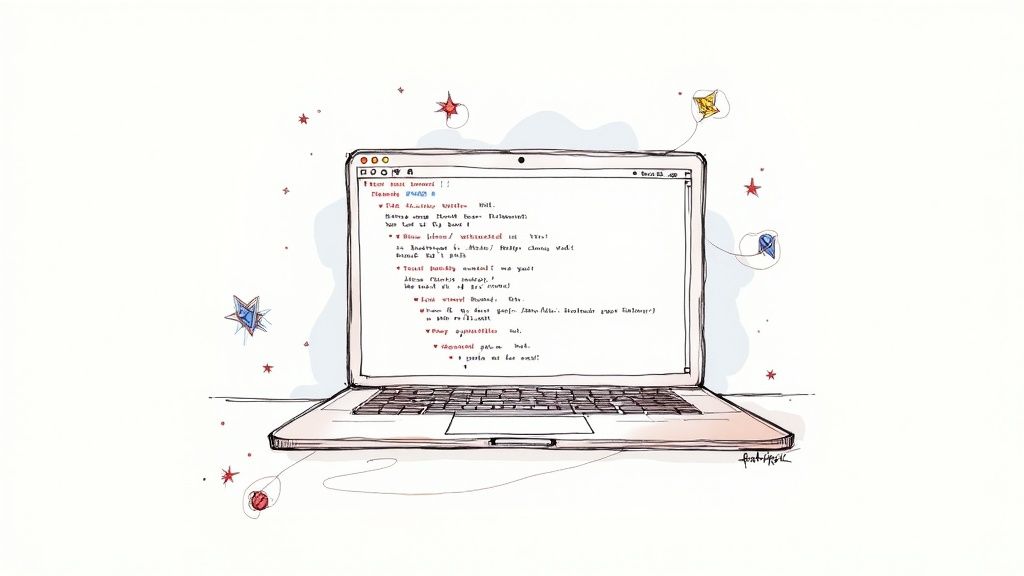
The Ruby ecosystem offers a diverse range of testing frameworks. Each framework brings its own advantages and disadvantages to the table. Picking the right one is a key factor in efficient and effective testing. This choice significantly impacts your team's productivity, how easy your code is to maintain, and the overall success of your testing efforts. Let's explore and compare some popular options: RSpec, Minitest, Cucumber, and Test::Unit.
RSpec: The Behavior-Driven Development Approach
RSpec is well-known for its behavior-driven development (BDD) approach and readable syntax. It excels at describing how your application should behave in a clear, almost narrative way. For example, a test case might read "it should return the correct user data."
This expressiveness makes the codebase easier to understand and simplifies team collaboration. However, this can sometimes lead to more verbose test suites.
Minitest: Simplicity and Performance
Minitest prioritizes simplicity and speed. It's a lightweight framework that prefers a more traditional test-driven development (TDD) style. This minimalist approach results in faster test execution and reduced overhead. It’s a good fit for projects where performance is a top priority.
Minitest's straightforward syntax also makes it easy to learn, allowing developers to get started quickly with Ruby testing. This makes it ideal for smaller teams or projects with tight deadlines.
Cucumber: Bridging The Gap Between Developers and Stakeholders
Cucumber focuses on collaboration between developers and non-technical stakeholders. It utilizes plain language descriptions of application behavior, known as Gherkin, enabling everyone to understand the tests.
This shared understanding simplifies identifying potential issues and ensures the application meets requirements. However, Cucumber can add complexity to smaller projects where this level of collaboration might not be necessary.
Test::Unit: Ruby’s Built-In Testing Solution
Test::Unit is included in Ruby's standard library, making it readily available without adding extra dependencies. It offers a basic but robust foundation for writing tests. This is particularly useful for projects that prioritize simplicity and minimal external libraries.
While Test::Unit is a good starting point for beginners, its features might be limited for larger, more complex projects.
Ruby Testing Frameworks Comparison
To summarize the key differences between these frameworks, let's review the following comparison table:
Framework | Syntax Style | Learning Curve | Best For | Community Support |
---|---|---|---|---|
RSpec | BDD, expressive | Moderate | Large projects, BDD practitioners | Large, active community |
Minitest | TDD, simple | Easy | Small to medium projects, fast testing | Active, growing community |
Cucumber | Plain language (Gherkin) | Moderate | Projects requiring strong stakeholder collaboration | Active community |
Test::Unit | xUnit style | Easy | Simple projects, minimal dependencies | Mature, stable community |
The table above provides a concise overview of each framework's strengths and weaknesses.
Choosing the right framework depends on several factors. Project size, team expertise, and the level of stakeholder involvement all play a part. Carefully considering these factors will help you make the best decision for your Ruby testing process. Understanding the strengths and weaknesses of each framework allows you to choose the one that best suits your project's specific needs. This ultimately leads to more effective testing and higher-quality Ruby code.
Test-Driven Development: The Ruby Way
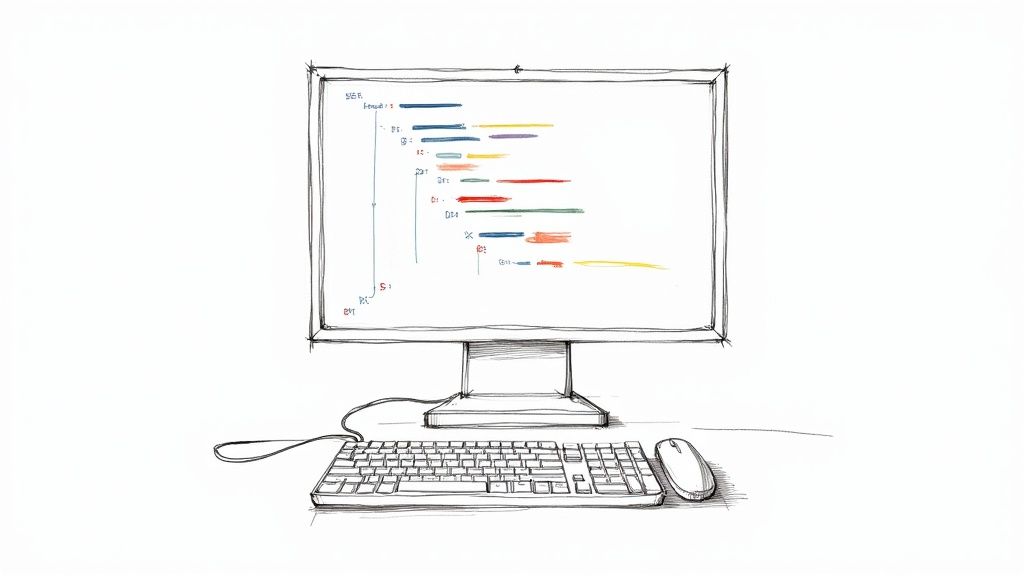
Ruby's elegant syntax makes Test-Driven Development (TDD) feel natural. TDD, where you write tests before the code, is more than just testing. It's a design philosophy. This approach results in cleaner code architecture. More importantly, it catches tricky edge cases you might otherwise miss.
The Red-Green-Refactor Cycle in Ruby
At the heart of TDD is the red-green-refactor cycle. This iterative process starts with a failing test (red). This test defines how a specific piece of functionality should behave.
You then write the minimum code needed to make the test pass (green). Finally, you refine the code, making it clean, efficient, and maintainable (refactor).
This continuous cycle ensures comprehensive test coverage, leading to robust and reliable software.
A Practical Example of TDD in Ruby
Let's say you're building a method to calculate discounts. First, you'd write a test that asserts the correct discounted price for a given input. Initially, this test would fail.
Next, you'd implement the discount calculation logic. You only write enough code to make the test pass. Lastly, refactor the code for clarity and efficiency, knowing your tests will catch any regressions.
Overcoming Ruby Testing Challenges
TDD offers many advantages. However, it's important to address Ruby-specific testing challenges. One challenge is managing dependencies in your test suite.
As your codebase grows, dependencies can become tangled. This can lead to slow and brittle tests. To address this, focus on isolating dependencies and using mocking.
By mocking external services or complex internal modules, your tests focus solely on the code under test. This improves test speed and makes debugging much easier. Well-structured tests are as important as well-structured code.
Another important aspect of Ruby testing is performance benchmarking. With over 1.3 million websites using Ruby on Rails, testing has become crucial. Tools like Benchmark::Trend help analyze computational complexity. Learn more about Ruby on Rails trends.
Practical Tips for Organizing Tests
Effective test organization is crucial as your project grows. A disorganized test suite becomes a maintenance nightmare. Mirror your code structure with your test structure.
For example, a User
module in your code should have a corresponding user_test.rb
file in your test directory. This simplifies locating and maintaining tests.
Also, use descriptive test names. Instead of test_1
, use names like test_calculate_discount_with_percentage
. This improves readability and clarifies the purpose of each test.
By following these practices, you can build a sustainable test suite. The initial investment in good tests pays off by catching bugs early and simplifying refactoring.
Advanced Ruby Testing Techniques That Actually Work
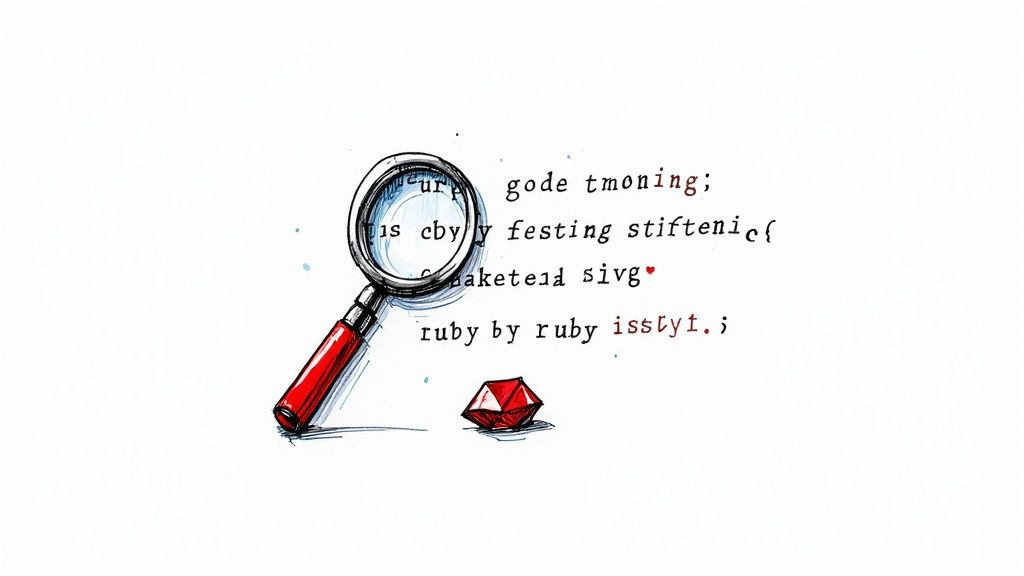
This section builds on the core principles of Test-Driven Development (TDD) and explores advanced Ruby testing strategies. These strategies are designed to boost the maintainability and effectiveness of your test suite, addressing the complex scenarios you'll encounter in real-world projects. They'll take your Ruby testing skills to the next level.
Metaprogramming For Maintainable Tests
Ruby's metaprogramming capabilities offer significant advantages for improving the maintainability of your tests. You can use metaprogramming to define common test setup or assertion logic once and apply it across multiple test cases.
This approach dramatically reduces code duplication. It also simplifies updating tests as your application grows and changes. The result? Less time spent on maintenance and more time building features.
Doubles and Stubs: Effective Test Isolation
Doubles and stubs are powerful tools for isolating the components you're testing. A double stands in for a real object, mimicking its behavior. A stub, a specialized type of double, replaces a method with a predefined response.
This isolation lets you test a component's behavior without dependencies on external systems or complex internal interactions. However, be cautious: overusing doubles and stubs can lead to brittle tests that are tightly coupled to implementation details. Finding the right balance is key for a robust test suite.
Tackling Asynchronous Code
Testing asynchronous code can present unique challenges. Using specialized libraries designed for testing asynchronous operations is crucial. Many popular Ruby testing frameworks offer these types of libraries.
These libraries provide mechanisms to wait for asynchronous operations to complete before assertions are made. This prevents race conditions and inconsistent test results, allowing for robust testing of asynchronous code.
Testing External Services
Testing code that interacts with external services brings its own set of difficulties. Directly interacting with these services during tests can be slow and unpredictable. Mocking or stubbing the behavior of the external service is a better approach.
This isolates your tests from network issues or changes in the external service's API, ensuring fast and reliable tests. Simulating these interactions creates a more predictable test environment.
Performance Testing in Ruby
Performance testing is vital for identifying bottlenecks. Tools like Benchmark and Benchmark::Trend provide mechanisms for measuring execution time. For performance-critical code, these tools help pinpoint areas for optimization.
By incorporating performance tests regularly, you can detect and address performance regressions early, before they impact users.
Bringing Legacy Code Under Test
Adding tests to a legacy Ruby codebase can seem daunting. Start with the most critical sections of your application. Focus on frequently modified code or areas with a history of bugs. Gradually expand test coverage from there.
This incremental approach provides immediate value while working towards comprehensive coverage. Even small steps towards testing legacy code can significantly improve confidence in its stability and maintainability. Prioritizing high-risk areas maximizes the impact of your testing efforts. These advanced techniques help handle even the most complex testing scenarios, ensuring the quality and maintainability of your Ruby code.
Automating Ruby Tests: From Local to CI/CD
Automating your Ruby tests is crucial for modern software development. It provides quick feedback, minimizes human error, and enables Continuous Integration and Continuous Deployment (CI/CD). This automation boosts your team's confidence in the code they release.
Setting Up Your CI/CD Pipeline for Ruby Testing
Many CI/CD platforms integrate smoothly with Ruby projects. Popular choices for Ruby teams include GitHub Actions, CircleCI, and Jenkins.
- GitHub Actions: Offers tight integration with GitHub repositories, simplifying workflow setup.
- CircleCI: Provides robust features for complex testing and parallel execution.
- Jenkins: A highly customizable and extensible option, suitable for diverse project needs.
Choosing the right platform depends on your team's workflow and project requirements. Consider factors like ease of use, scalability, and integration with your existing tools. The correct platform empowers your team to write high-quality code while automation handles the testing.
Parallelizing Your Ruby Test Suite
As your Ruby project expands, your test suite grows. This often leads to longer execution times. Parallelization, running tests concurrently, addresses this by significantly reducing feedback time. This allows developers to identify issues quickly.
However, parallelization can introduce complexity, particularly if tests share resources or state. Proper test isolation is vital for preventing inconsistent results.
Structuring Your Test Automation for Clear Feedback
Effective test automation provides clear, actionable feedback. When a test fails, you need to quickly understand what went wrong and why. This requires structuring your tests logically and using descriptive names.
For example, a test named test_user_signup
clearly indicates a problem with the user signup process if it fails. Further investigation, guided by the test output, pinpoints the specific error.
Consistent Test Environments
Maintaining consistency between local development and CI/CD environments is essential. This ensures tests behave identically in both, preventing unforeseen issues.
Tools like Docker help create reproducible test environments, eliminating discrepancies caused by differing dependencies or system configurations. This allows you to catch issues early in development.
The rise of CI/CD has significantly impacted Ruby testing. Total Ruby gem downloads have dramatically increased, from 3.71 billion in July 2019 to over 137 billion by July 2023. This demonstrates the growing importance of automated testing and deployment. Explore this topic further.
By implementing these strategies, you build a robust and reliable automated testing system. This promotes code quality and accelerates the development lifecycle, leading to faster releases and satisfied users.
Ruby Web Application Testing: Strategies For Growth
Testing Ruby web applications can be tricky. As your application expands, your testing methods need to keep up to stay efficient and effective. This article explores how successful teams build testing strategies that find bugs without creating a maintenance nightmare.
Layered Testing For Ruby Web Applications
Comprehensive coverage relies on a layered testing approach. This involves a balanced mix of unit tests, integration tests, and system tests.
- Unit Tests: These tests check the smallest parts of your application in isolation. They are quick to run and help identify bugs early on. Think of them as testing individual Lego bricks.
- Integration Tests: Integration tests look at how different parts of your application work together. They ensure your "Lego bricks" combine to create a solid structure. These are slower than unit tests but faster than system tests.
- System Tests (End-to-End Tests): System tests cover the whole application flow, mimicking user actions and external service integrations. They give you the highest level of confidence but are the slowest to execute. They test the completed Lego model.
This layered approach acts as a safety net during development. It helps you catch errors early and ensures the whole system functions correctly. The key is finding the right balance between these layers for a maintainable and effective test suite.
To illustrate this layered approach in more detail, let's look at a practical breakdown. The following table summarizes the key aspects of each testing layer:
Ruby Web Application Testing Layers
Testing Layer | Primary Focus | Recommended Tools | Ideal Coverage | Execution Speed |
---|---|---|---|---|
Unit Tests | Individual components/methods | Minitest, RSpec | High (70-90%) | Fast |
Integration Tests | Interactions between components/modules | Minitest, RSpec, Capybara | Medium (50-70%) | Moderate |
System Tests | End-to-end application flow | Capybara, Selenium | Medium (30-50%) | Slow |
This table provides a handy overview of each layer, its purpose, and the tools commonly used. Notice the trade-offs between coverage, speed, and the scope of each testing layer.
Practical Techniques For Scalable Testing
Several techniques contribute to scalable Ruby web application testing:
- Testing API Endpoints: Focus on clear results verification and avoid fragile tests that break easily with minor API changes. Use tools specifically designed for API testing to simplify the process.
- Managing Test Data: Use efficient strategies to create and manage test data. Consider using factories or fixtures to generate test data instead of relying on production data for consistency.
- Authentication Testing: Develop clear, maintainable testing strategies for authentication, avoiding overly complex setups.
- Browser Testing vs. Faster Alternatives: Evaluate when to use full browser testing versus faster options like headless browsers. Headless browsers speed up testing but full browser testing might be necessary for browser-specific behavior.
- Structuring Your Test Suite: Organize tests logically and use consistent naming conventions. A well-structured test suite makes it easier to find and maintain tests as your application grows.
These strategies create a testing framework that grows with your application, preventing testing from becoming a development bottleneck. This enables confident delivery of high-quality Ruby web applications.
Streamlining CI/CD With Mergify
Managing complex testing workflows and CI/CD pipelines can be a challenge. Mergify offers a robust solution to simplify code integration, lower CI costs, and boost developer productivity. Mergify's features, such as Merge Queue and Merge Protections, automate pull request updates and ensure stable code integration. Explore how Mergify can improve your team's management of complex development environments and enhance your CI workflow.