Ruby Test Essentials for Modern Developers
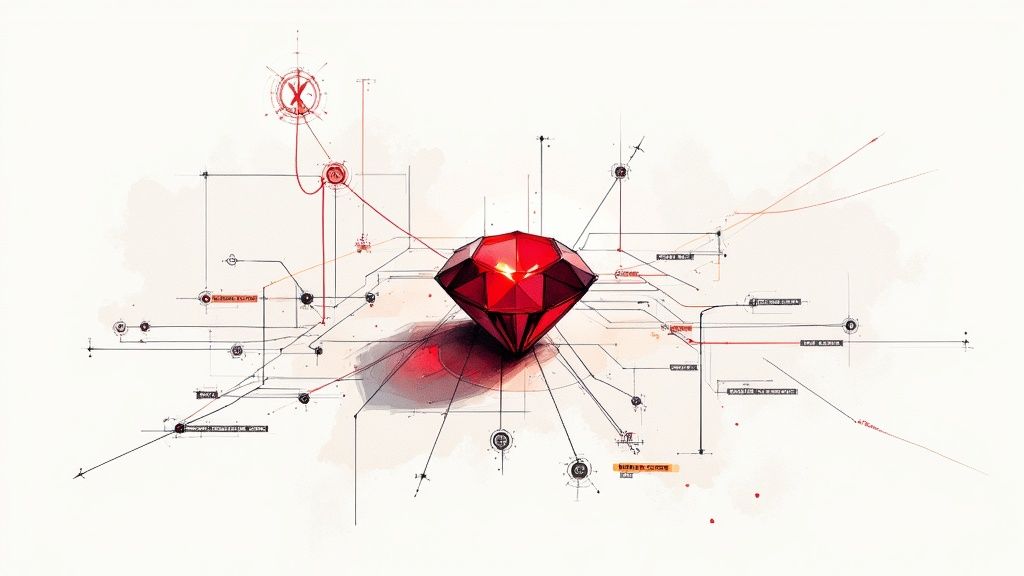
Why Ruby Test Excellence Matters In Modern Development
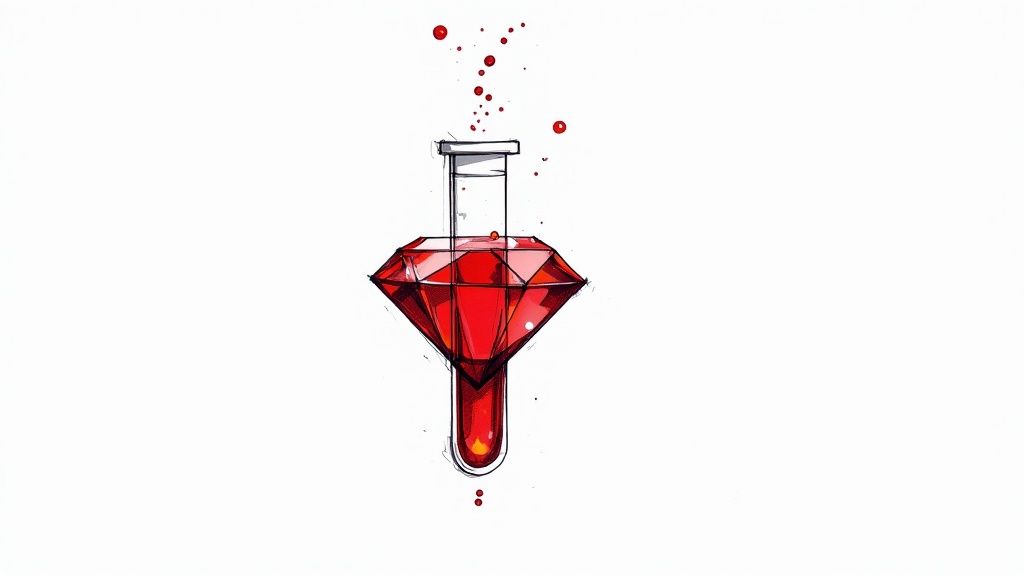
In the ever-shifting world of software development, reliable testing is essential. This is particularly true for Ruby, known for its flexibility and expressiveness. Effective Ruby testing practices are crucial for creating dependable and maintainable applications. This results in fewer bugs in production and more confidence when making changes or adding features.
The Impact of Thorough Testing
Comprehensive Ruby test suites act as a safety net. They empower developers to modify code without worrying about introducing regressions. This is vital in agile environments with frequent releases and quick iterations. For example, thorough tests can quickly identify the root of problems. This drastically reduces debugging time and speeds up the development cycle. Well-written tests also act as up-to-date documentation, clarifying how the code should behave.
Ruby's Unique Testing Philosophy
Ruby's testing philosophy prioritizes developer satisfaction and productivity. This is evident in popular testing frameworks like Test::Unit and RSpec, which promote clear, concise tests. This differs from some languages where testing can feel burdensome. Ruby's approach makes testing a more integrated part of development.
The emphasis on readable and maintainable tests facilitates collaboration and knowledge sharing within teams. This contributes to a more resilient and robust codebase over time. This focus on developer experience makes testing less of a chore and more of a valuable asset in the development process.
Ruby Usage Trends
The Ruby language continues to adapt to modern development needs. Ruby's popularity and usage have shifted over time. As of 2023, Ruby 3.2 is the most used version, adopted by 43% of developers, surpassing Ruby 2.7. In 2021, Ruby 2.7 held the top spot at 51%. The shift to newer versions demonstrates the adoption of modern features and improvements. Learn more about these trends here.
Choosing The Right Approach
Not all testing methods are equal. Some common practices, like relying too heavily on mocking or neglecting integration tests, can decrease productivity and create fragile tests. Developers must carefully choose testing strategies that align with project needs and long-term goals.
By prioritizing meaningful tests that cover essential features and various scenarios, teams can maximize their testing investment. Embracing a culture of Ruby test excellence leads to more stable, reliable, and maintainable applications that can adapt to the changing demands of software development.
Practical Test::Unit: Building a Robust Ruby Codebase
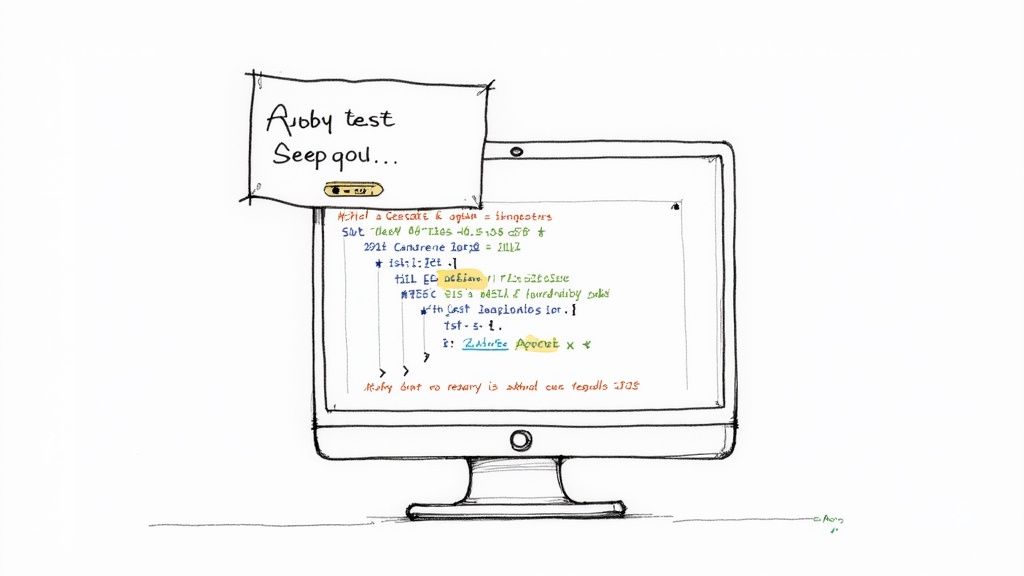
Let's move past theory and delve into the practical application of Test::Unit for improving your Ruby code. This isn't just about achieving high test coverage, but about crafting tests that uncover real-world problems. Think of it like constructing a building: you wouldn't simply count the bricks, but ensure they're assembled correctly and the structure is sound.
Structuring Tests for Maintainability
Maintaining a growing test suite can be challenging. Disorganized tests often break with each code change, hindering development. However, adopting proven organizational patterns can prevent this.
One effective strategy is mirroring your application's structure within your test directory. This simplifies locating tests related to specific code sections.
Selecting the Right Assertions
The assertions you choose directly impact test readability and debugging speed. Think of assertions as your debugging magnifying glass. A well-chosen assertion clearly highlights what went wrong, making problems easier to identify.
For example, instead of simply checking if a value is true or false, use assert_equal
to specify the expected value. This improves test clarity and accelerates debugging. Adding custom error messages within assertions provides even more context.
To help you choose the best assertion for your needs, we've compiled a handy table summarizing common Test::Unit assertions. This table provides a quick reference guide, outlining each assertion's purpose and offering practical usage examples.
Common Test::Unit Assertions: A Quick Reference Guide
Assertion Method | Purpose | Example Usage |
---|---|---|
assert_equal |
Checks for equality | assert_equal 5, result |
assert_not_equal |
Checks for inequality | assert_not_equal 0, result |
assert_nil |
Checks if a value is nil | assert_nil value |
assert_not_nil |
Checks if a value is not nil | assert_not_nil value |
assert_true |
Checks if a value is true | assert_true condition |
assert_false |
Checks if a value is false | assert_false condition |
This table provides a quick overview of frequently used assertions, aiding in writing clearer and more effective tests. By using the appropriate assertion, you can pinpoint issues more quickly and ensure your tests are easy to understand.
Mastering the Command Line for Ruby Tests
Using command-line options effectively is vital for targeted testing. Experienced Ruby developers use specific commands to focus on problematic areas, optimizing test execution.
Running tests for a single file or directory saves significant debugging time, allowing for effective issue isolation. Ruby developers often use Test::Unit, part of the Ruby Standard Library. This framework offers specialized methods like assert_dom_equal
for testing DOM equality in web development. While Ruby's testing ecosystem is robust, it's been relatively stable recently, focusing on these established frameworks. Learn more in this Ruby Cookbook. Understanding test output and deciphering error messages is also crucial for efficient debugging.
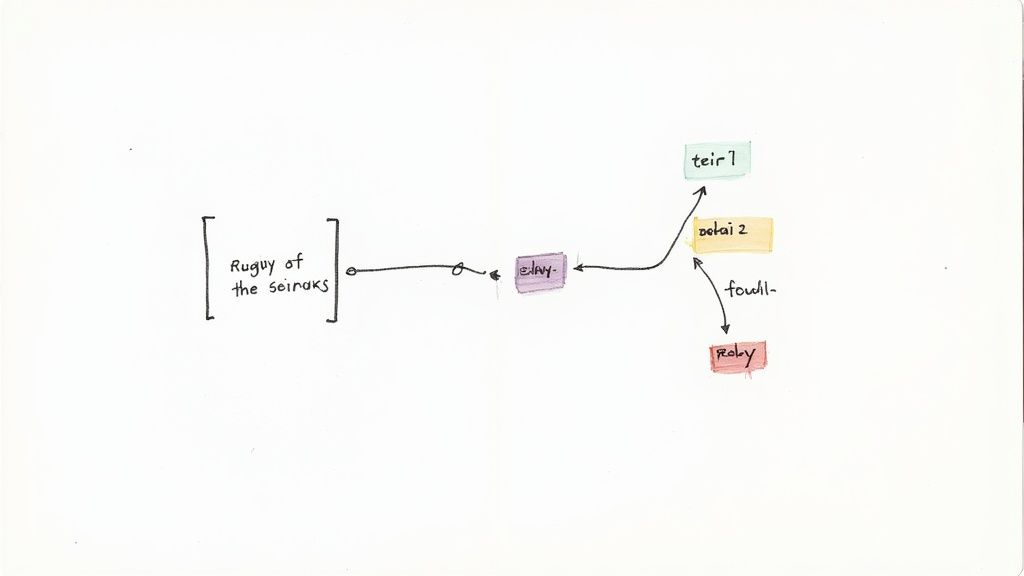
RSpec truly elevates Ruby testing. It helps create maintainable and readable test suites. It's more than a framework; it empowers teams to build better software. Unlocking its full potential lets you craft tests that act as living documentation.
Writing Clear Tests With RSpec
Top Ruby teams leverage RSpec's expressive syntax. This makes tests easier for everyone to understand, not just developers. This shared understanding boosts collaboration and reduces time spent deciphering test logic.
For example, descriptive describe
and it
blocks clearly define the behavior under test. This allows even non-technical team members to understand the tested functionality.
Structuring Tests for Clarity and Efficiency
Organizing tests effectively within RSpec is crucial for efficient debugging and maintenance. Think of a large, cluttered house. Finding anything is a nightmare. Similarly, organizing tests into logical contexts makes it easier to pinpoint and fix issues.
This clarity helps you quickly locate failing tests. It also helps you understand the scope of their impact. Plus, it aids in maintaining test suite organization as the project grows. Well-structured tests save valuable time and reduce debugging frustration.
Mocking Effectively in RSpec
Mocking in RSpec isolates units of code for focused testing. But, over-mocking can create fragile tests that break easily during refactoring. Finding a balance between isolation and flexibility is key.
RSpec offers robust mocking capabilities. This allows you to isolate code units effectively. Think of mocks like stunt doubles in movies. They stand in for the real actors in specific scenes, allowing for controlled filming. Similarly, mocks replace real dependencies in tests, enabling isolated and predictable testing scenarios.
Using Advanced RSpec Features
RSpec offers features like shared examples and custom matchers to increase efficiency. Shared examples remove redundant code, making tests concise and easier to update. They're like reusable blueprints for common testing scenarios.
Custom matchers let you create domain-specific assertions. This expresses complex conditions clearly. For example, a custom matcher can verify that a user is "logged in" without checking multiple attributes individually. This clarifies test intent and improves maintainability.
Real-world projects using these techniques see substantial improvements in maintainability. Once chaotic, test suites become valuable assets, supporting confident code changes and higher product quality. This thoughtful approach to Ruby testing with RSpec ensures your test suite remains a reliable and efficient tool for your team.
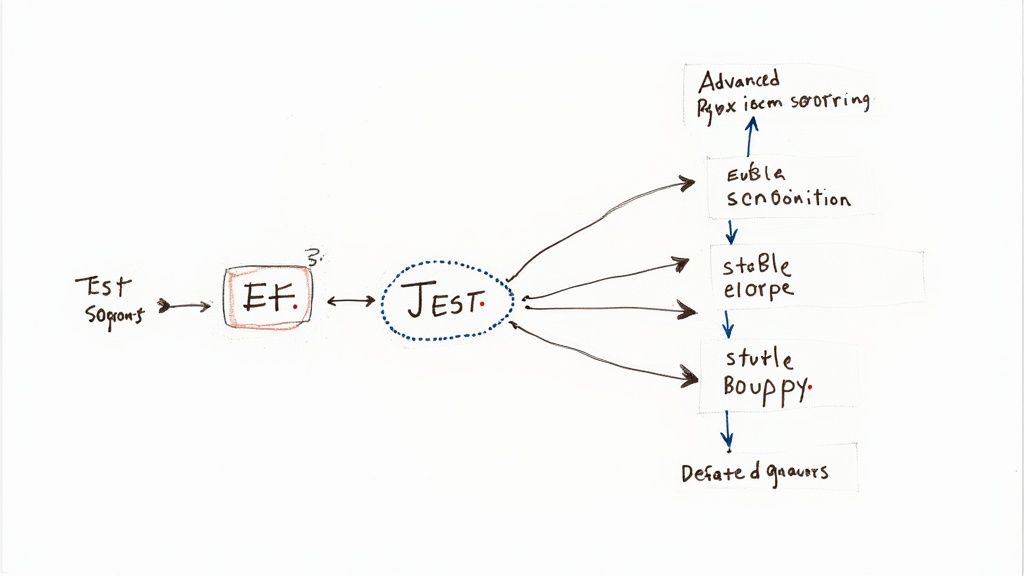
Testing Rails applications effectively, especially as they grow, requires a well-defined strategy. Simply having tests isn't enough. We need tests that are fast, comprehensive, and easy to maintain. This means understanding the different types of tests and knowing when to use each one.
Balancing Test Types for Optimal Coverage
Successful Rails development teams understand the importance of balancing unit, integration, and system tests. Unit tests, which focus on individual components, are generally fast and precise. They help catch errors early in the development process. Integration tests verify the interactions between different components, ensuring they work together seamlessly.
System tests, while slower, ensure the application functions correctly from the user's perspective. Finding the right balance between these three types of tests is key to a healthy and efficient test suite. Too many slow system tests can bog down your testing process, while too few might leave gaps in your coverage.
Testing Complex Rails Features
Testing Rails features like callbacks, validations, and background jobs can be particularly challenging. Callbacks and validations, core parts of the Rails framework, should be thoroughly tested. However, it’s important to keep the test scenarios manageable. Consider using shared examples for common validation logic across different models.
Background jobs present another testing hurdle. Tests need to verify correct execution without slowing down the entire test suite. Tools like the Sidekiq testing API can help isolate background jobs for more efficient testing.
Choosing the Right Tools
Rails offers built-in testing tools, but gems like RSpec and Minitest provide additional functionality. Choosing the right tool depends on the project's needs. Rails' built-in tools might suffice for smaller applications. However, for larger projects, RSpec's structure and expressiveness can improve maintainability. Features like shared examples and custom matchers can make your tests more readable and easier to manage.
The following table summarizes the different types of tests available in Rails:
Types of Tests in Rails Applications
Test Type | Purpose | Testing Speed | When to Use |
---|---|---|---|
Unit | Tests individual components in isolation | Fast | Testing models, controllers, services, or other isolated logic |
Integration | Tests interactions between multiple components | Moderate | Testing interactions between controllers and models |
System | Tests the entire application from a user perspective | Slow | Testing user flows through the entire application |
This table provides a quick reference to help you decide which type of test is most appropriate for different scenarios. Choosing the right tool and the right type of test is crucial for building a robust and maintainable application.
Refactoring Legacy Test Suites
Legacy test suites can become difficult to maintain. Slow, brittle tests often discourage regular execution, leading to undetected bugs and slower development cycles. Refactoring these test suites, while challenging, offers significant long-term benefits.
Profiling tools, like those in the TestProf gem, can help identify bottlenecks. Strategies like switching from create
to build_stubbed
with FactoryBot can drastically reduce database interaction and speed up tests. Optimizing background job testing and ensuring jobs aren’t run inline unnecessarily are also key improvements.
As of 2021, Rails was used by 86% of Ruby developers, solidifying its position as the most popular Ruby framework. Learn more about Ruby usage. This widespread adoption further emphasizes the importance of efficient testing practices.
Mastering Test Doubles: Beyond Basic Mocking
Many Ruby developers overuse test doubles, resulting in fragile tests that break with minor code changes. Instead of providing a safety net, these tests become a maintenance headache. This section explores how effective teams strategically employ mocks, stubs, fakes, and spies for more robust and maintainable Ruby test suites.
Understanding the Different Types of Test Doubles
Understanding the distinct roles of test doubles is the first step towards mastery. Consider them specialized actors in a movie:
- Mocks: These verify interactions. Like a demanding director, mocks ensure methods are called with the correct arguments in the proper order. They're useful for testing complex object interactions.
- Stubs: These control return values. Similar to a script, stubs provide predetermined responses to method calls. This isolates the code being tested from external dependencies.
- Fakes: These simulate real implementations. Like stand-in actors, fakes provide a simplified version of a dependency for testing.
- Spies: These monitor behavior. Spies record which methods are called and with what arguments, like behind-the-scenes observers. This verifies interactions without controlling return values.
Selecting the right test double is crucial. Overusing mocks, for example, can tightly couple tests to implementation details, causing breakage with every refactor.
Avoiding Implementation Coupling
Effective test doubles avoid implementation coupling. Tests should focus on the behavior of the code, not its internal workings. This means minimizing mocks and prioritizing stubs and fakes.
For instance, when testing a class interacting with an external API, use a stub to control the API response instead of mocking the interaction. This allows internal changes without breaking tests.
Testing Challenging Dependencies
Testing dependencies like APIs and databases requires specific strategies. For APIs, fakes simulate responses without network calls, making tests faster and more reliable.
For databases, use a dedicated test database, cleaning it after each test run for isolation and consistent results.
Time-Dependent and Asynchronous Testing
Time-dependent tests can be tricky. Use tools like Timecop to control time flow within your tests, preventing unexpected failures.
For asynchronous code, ensure tests wait for asynchronous operations to finish before assertions. This avoids intermittent failures and maintains test suite reliability. Mastering test doubles and these techniques transforms problematic test suites into valuable assets, ensuring robust and maintainable Ruby projects.
Identifying Bottlenecks With Profiling Tools
Slow test suites can significantly hinder productivity, often leading developers to skip tests altogether, ultimately compromising code quality. Before attempting any optimization, it's crucial to identify the actual bottlenecks. Don't rely on guesswork. Profiling tools offer concrete data, revealing where your suite spends the most time.
Tools like Stackprof and RubyProf can pinpoint frequently called methods, highlighting areas ripe for optimization. Furthermore, TestProf allows granular profiling of different test types, identifying slowdowns within specific areas like controllers or models. This targeted approach focuses your optimization efforts where they'll have the biggest impact.
Parallelization Techniques That Work
Parallelization can drastically reduce test execution time. However, simply adding more hardware isn't always the solution. Effective parallelization requires careful management of dependencies between tests and shared resources. parallel_tests distributes your suite across multiple processes or machines, significantly reducing overall runtime.
Remember to address any shared state issues between parallel tests to maintain accuracy and reliability. This ensures your tests remain independent and produce consistent results, even when run concurrently.
Managing Test Data Effectively
Database interactions are a frequent source of slowdowns in Ruby tests. Minimize these interactions by strategically using test doubles like mocks and stubs. FactoryBot streamlines test data creation, but overuse of create
can lead to unnecessary database calls.
Instead, prefer build_stubbed
when database persistence isn't required. This creates lighter-weight objects, significantly speeding up test execution. Using transactions effectively within your tests keeps the database clean and maintains test isolation. This ensures each test runs in a pristine environment, preventing unintended side effects.
Balancing Unit and Integrated Tests
Finding the right balance between unit and integrated tests is essential. Unit tests offer speed and precision, while integrated tests ensure different application parts work together correctly. Prioritize fast, focused unit tests for core logic and use integration tests strategically to verify critical interactions between components.
This balanced approach provides comprehensive coverage without sacrificing speed. As discussed in this article about the continuing relevance of Ruby, When Will Ruby Finally Die, regional adoption of the language varies. By carefully evaluating the need for each test type, you can optimize your suite for both speed and thoroughness.
Optimizing your Ruby test suite isn't just about faster tests; it's about improving the entire development workflow. By implementing these strategies, you can empower your team to embrace testing as a valuable tool, leading to higher quality code and a more efficient development process.
Strategically Structuring Test Runs
Effective Continuous Integration (CI) transforms Ruby tests into a constant safety net for your code. Instead of occasional checks, CI provides continuous feedback, catching bugs before they impact users. By looking at how successful Ruby teams structure their CI pipelines, we can learn how to build robust systems that boost team confidence and identify problems early.
One key strategy is structuring your test runs effectively. Running every test for each commit can be slow, particularly for larger projects. A tiered approach is often more efficient. Run quick unit tests with every commit to catch basic errors immediately. Then, schedule more time-consuming integration and system tests less frequently, perhaps before major releases.
Ensuring compatibility across different Ruby versions is also crucial. Running your tests across strategically chosen versions, especially those most used by your audience, lets you identify and address compatibility problems early without slowing down the entire CI process.
Optimizing CI Execution Time
Fast CI feedback is vital for a smooth development process. Long build times can disrupt workflow and delay releases. Caching dependencies, like frequently used libraries and gems, prevents redundant downloads, drastically cutting down build time.
Parallelizing your Ruby tests is another powerful optimization. By distributing test runs across multiple CI runners, you can significantly decrease overall execution time. Tools like parallel_tests can help streamline this process, maximizing the use of your CI resources. This faster feedback loop enables your team to address issues quickly, keeping the development cycle moving.
Integrating Code Coverage and Quality Metrics
Integrating code coverage analysis into your CI pipeline gives you a continuous view of how thoroughly your tests exercise your codebase. Tools like SimpleCov provide reports that track coverage over time, helping pinpoint gaps and enhance test quality.
Including other quality metrics, such as code complexity and style checks, can help maintain consistent coding standards and reduce the accumulation of technical debt. However, it’s important to choose metrics that genuinely add value and align with your team's goals, keeping your CI process efficient and focused.
By implementing these practical CI strategies, you can create a development workflow that's both efficient and dependable. Your Ruby tests become an invaluable tool, ensuring code quality, catching problems early, and empowering your team to build better software. Ready to boost your Ruby CI/CD? Mergify offers automation and optimization tools to streamline your process and save valuable time. Explore how Mergify can help.