Ruby Minitest: Transform Your Testing Approach
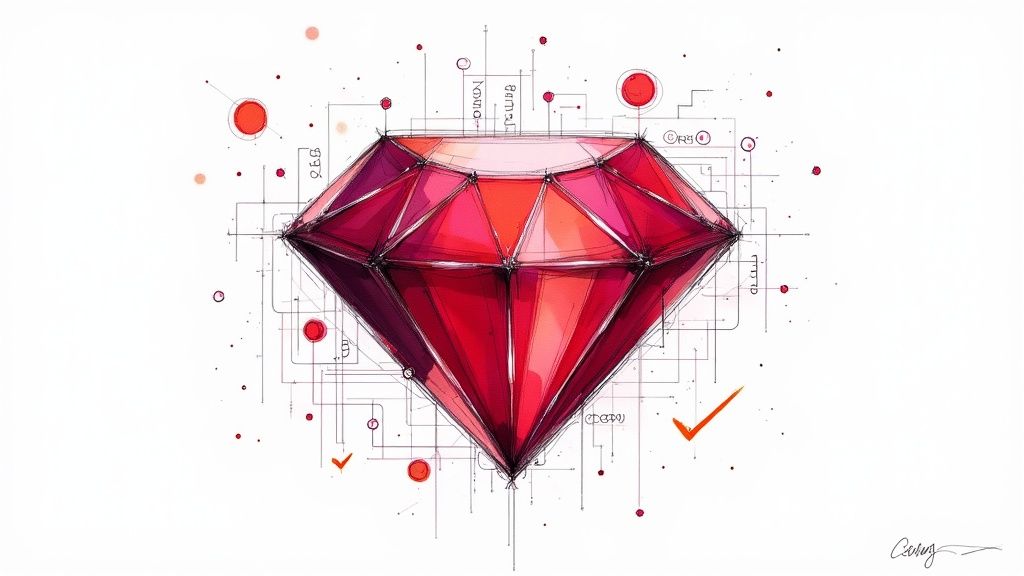
Why Ruby Minitest Is Your Testing Secret Weapon
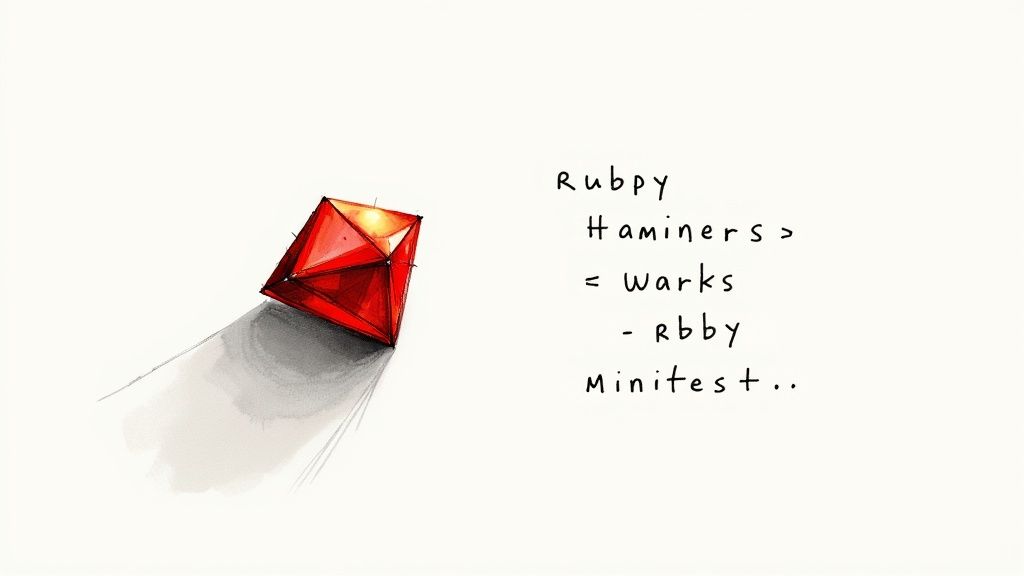
Picking the right testing framework can be a real headache in the fast-paced world of Ruby development. While many options are available, Ruby Minitest stands out with its compelling blend of simplicity and power. This lightweight framework offers surprising flexibility without the extra baggage of some alternatives, making it a secret weapon for efficient and effective testing.
Minitest's efficiency comes from its minimalist design. It focuses on providing core testing functionalities without unnecessary extras, resulting in a smaller footprint and faster execution speeds. This allows developers to concentrate on writing tests instead of getting bogged down in complex configurations or slow test runs.
Minitest's clear syntax and minimal learning curve also make it easier for teams to adopt and maintain a consistent testing strategy. This streamlined approach boosts productivity and ensures that testing remains an integral part of the development process.
Minitest integrates seamlessly with the Ruby ecosystem. Its Ruby-native feel fosters a natural workflow that complements Ruby's elegant and practical nature. This integration is especially evident in Ruby on Rails projects.
Why Teams Choose Ruby Minitest
Minitest has been a bundled feature of Rails since its early versions, and it's still one of the most popular testing suites for Rails applications. As of Rails 8.0, Minitest is supported alongside Ruby versions as recent as Ruby 3.2. This integration has made Minitest a go-to choice for developers who prefer a lightweight testing solution.
With Minitest included in Rails, developers can enjoy simple, fast testing without installing additional dependencies. Minitest's minimalist approach provides a clean and efficient way to write tests, fitting well with the agile methodologies often used in Ruby and Rails development. You can explore this further at the Minitest GitHub repository.
Many leading Ruby teams use Minitest for several key reasons:
- Speed: Minitest's lightweight architecture allows for faster test execution, providing quicker feedback during development. This contributes to a more agile and responsive development process.
- Ease of Use: Minitest is known for its minimal learning curve. Its intuitive syntax and straightforward approach allow developers to write effective tests quickly, even with limited experience.
- Ruby-Native Integration: Minitest feels like a natural extension of Ruby, improving code readability and reducing the mental overhead of switching between testing and application code.
This combination of speed, simplicity, and seamless integration makes Ruby Minitest a great choice for both seasoned Ruby developers and those new to the language. By choosing Minitest, teams empower themselves to write more robust, reliable, and maintainable code. This helps improve code quality, enhance collaboration, and increase overall development velocity by focusing on what matters most: building great software.
Setting Up Ruby Minitest: From Zero to Testing Hero
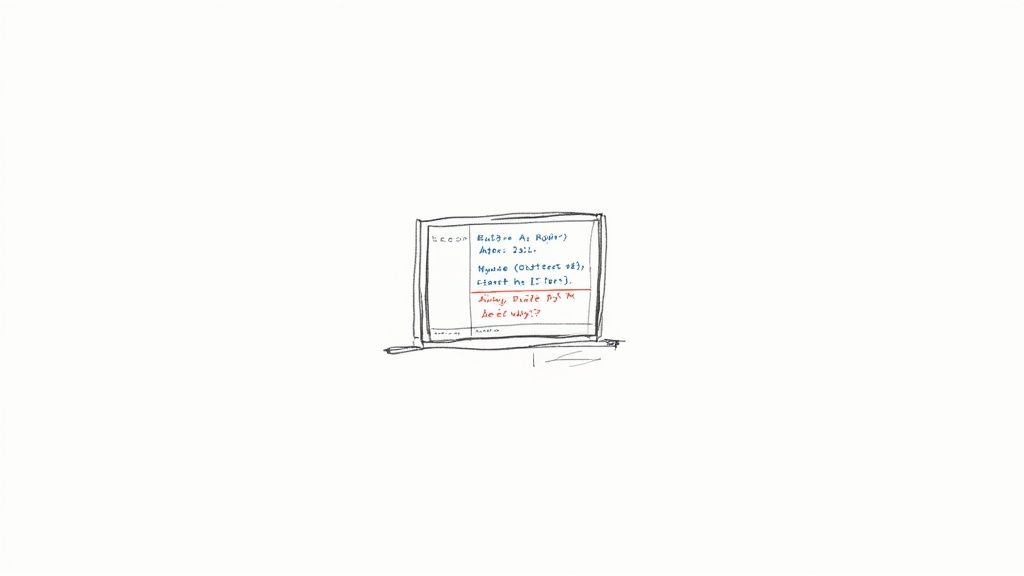
This section helps you clear any initial testing hurdles. We’ll walk you through practical Ruby Minitest implementations, covering both new and existing projects. Our focus is on approaches that are effective in real-world scenarios, getting your tests running quickly and efficiently.
Structuring Your Test Directory
Organizing your tests is essential for maintaining a healthy project. A well-defined structure makes managing your tests significantly easier as your project scales. This also simplifies locating and updating individual tests. A typical approach for Ruby Minitest involves creating a test
directory at your project's root.
Inside this directory, you can organize tests by type (unit, integration, system) or by the application module they pertain to.
- Unit Tests: These typically reside under
test/unit
. - Integration Tests: Place these under
test/integration
. - System Tests: If you're using system tests, the convention is
test/system
.
Configuring Ruby Minitest for Your Workflow
Minitest offers a variety of configuration options to fit your team's workflow. You can adjust the output format, set default reporters, and even add custom plugins. Customizing Minitest improves the readability of your test results and your overall testing efficiency.
For instance, if you need a more detailed output, you can configure Minitest to show comprehensive information for each test run.
Integrating Ruby Minitest in Different Environments
Minitest seamlessly integrates with different Ruby project types. Whether you're working with a standalone Ruby application or a more complex Rails project, Minitest can be easily incorporated. In Rails, Minitest is the default testing framework, simplifying its use in your Rails testing process.
For standalone applications, remember to include the minitest
gem in your project's Gemfile
.
Automating Tests With Rake Tasks
Rake tasks provide a way to automate the execution of your Ruby Minitest suite. Creating separate tasks for different test types or subsets of your tests allows you to run specific tests easily. This saves valuable development time and promotes focused testing during development.
A simple Rake task for running all your tests might look like this:
Rakefile
namespace :test do task :all do ruby 'test/**/*_test.rb' end end
This setup is easily adaptable to various Ruby projects and CI/CD pipelines. Services like Mergify can automate your CI further, triggering tests automatically and reporting results on pull request updates. This integration significantly improves team productivity and shortens feedback cycles.
Troubleshooting Common Issues
While Ruby Minitest is generally user-friendly, you might occasionally encounter some hiccups. A common issue is tests failing unexpectedly due to test dependencies or problems with setup and teardown procedures.
Using techniques like mocking and stubbing, along with a solid understanding of Minitest's lifecycle hooks, can help address these problems effectively. Tools like FactoryDefault
from the test-prof
gem are valuable for managing complex factory cascades in tests, which can often slow down test suite execution. These optimization techniques help your tests run quickly and produce reliable results, even as your project expands.
Crafting Ruby Minitest Tests That Actually Make Sense
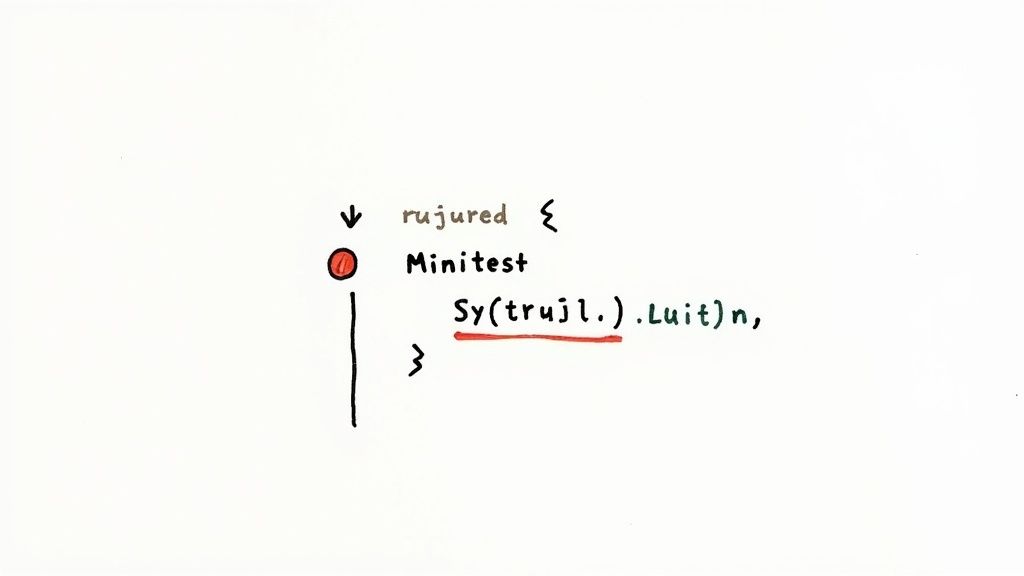
Writing effective tests is more than just making simple assertions. It's about creating tests that illuminate your code, expose hidden bugs, and simplify future maintenance. Ruby Minitest gives you the tools to create a robust and easy-to-understand test suite. This section explores how to structure your Minitest tests for maximum impact.
Understanding Minitest Testing Styles
Minitest offers two main testing styles: assertion-style and spec-style. Understanding each style is key to writing clear and maintainable tests.
Assertion-style tests, descended from the xUnit framework family, use a traditional approach. Methods like assert_equal
verify expected outcomes. This style is often favored for its straightforward simplicity.
Spec-style tests, inspired by frameworks like RSpec, take a more descriptive, behavior-driven approach. Tests are grouped within describe
blocks, and keywords like it
and should
define expected behaviors. This style can improve readability, especially in complex test scenarios.
A key advantage of Minitest is its flexibility. It supports both assertion-style and spec-style, letting developers choose the best fit for their project or preference. Developers familiar with RSpec can use spec-style in Minitest. Plus, Minitest provides features like mocking and stubbing, which are essential for isolating dependencies and ensuring reliable tests, particularly in complex applications. Learn more about different testing approaches with Minitest here.
Structuring Tests for Clarity
High-performing Ruby teams often use consistent patterns when structuring their Minitest tests. Meaningful test names are essential. A good test name clearly explains the scenario being tested, making it easy to grasp the purpose without digging into the code. Organizing tests by feature or module improves code organization, simplifying locating and updating specific tests.
Leveraging Minitest's Assertion Library
Minitest provides a comprehensive assertion library, offering a wide range of tools for clearly expressing test expectations. Assertions like assert_equal
, assert_nil
, and assert_match
allow for precise validation of values, ensuring your code functions as expected. This expressiveness allows your tests to act as living documentation, clearly demonstrating the expected behavior of your code.
Streamlining Setup and Teardown
Consistent setup and teardown processes are crucial for efficient testing. Setting up necessary test data and dependencies before each test guarantees a clean testing environment. Proper teardown after each test prevents leftover data from affecting subsequent tests. This practice saves valuable debugging time, especially when requirements evolve.
Assertion-Style vs. Spec-Style Syntax
The following table compares assertion-style and spec-style syntax in Ruby Minitest, providing examples and explaining when each style might be most suitable:
Feature | Assertion-Style | Spec-Style | When to Use |
---|---|---|---|
Structure | Uses class and def for test definition |
Uses describe and it blocks |
Spec-style for behavior-driven tests, assertion-style for simpler cases |
Assertions | assert_equal(expected, actual) , assert_nil(value) , etc. |
expect(actual).must_equal expected , expect(value).must_be_nil , etc. |
Choose based on project conventions and personal preference |
Readability | Can be concise but less descriptive | More descriptive and easier to understand for complex scenarios | Spec-style for larger teams and complex logic, assertion-style for smaller projects |
By understanding each style's strengths and applying consistent practices, you can build Ruby Minitest tests that enhance code quality and streamline your development process. This proactive approach to testing minimizes the chance of regressions, accelerates debugging, and ensures your application stays robust and reliable.
Advanced Ruby Minitest: Beyond the Basics
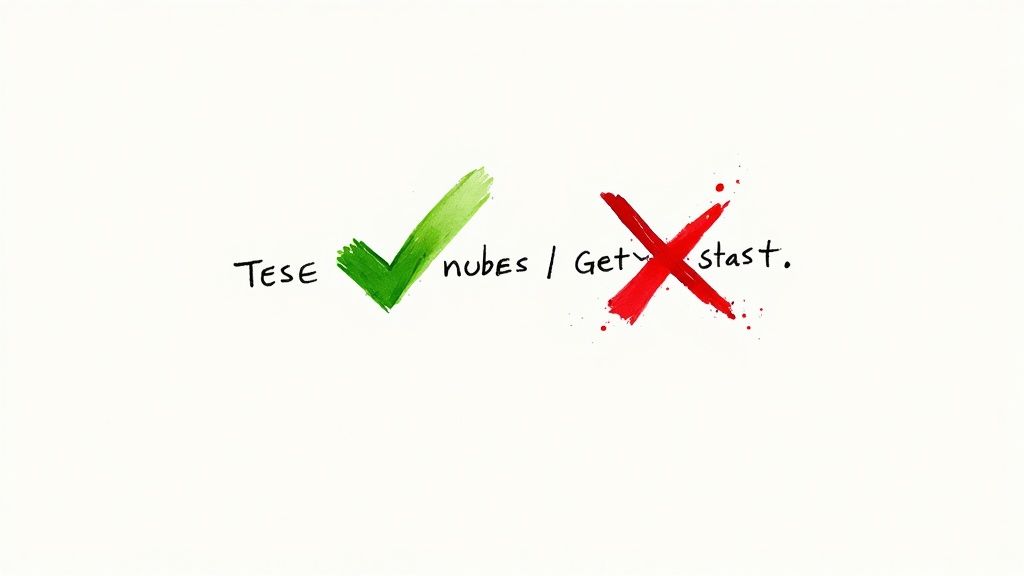
Let's explore techniques that can elevate your Ruby Minitest skills. This section covers practical strategies for mocking and stubbing, handling asynchronous operations, and optimizing performance – all essential for building robust and efficient test suites.
Mocking and Stubbing for Isolation
Mocking and stubbing are key techniques for isolating the code you're testing. Mocking creates a stand-in object that mimics real object behavior, while stubbing replaces a method with a predetermined return value. This prevents brittle tests by focusing on specific logic without relying on external dependencies.
Imagine testing a class interacting with an external API. Instead of making live API calls, you can mock the API client and stub its methods. This returns predefined responses, making tests faster and more reliable by eliminating network issues or API changes.
Testing Asynchronous Operations
Asynchronous operations can be tricky to test. Minitest, however, provides tools to manage these scenarios effectively. Libraries like Mocha or minitest-stub_any_instance
help stub methods, letting tests run synchronously while verifying asynchronous calls.
For complex multi-threaded scenarios, consider exploring Concurrent-Ruby, a gem offering advanced control over concurrency.
Database Interactions and External API Dependencies
Testing code that interacts with databases or external APIs requires special attention. For databases, transactional fixtures maintain a clean state for each test. Database Cleaner further assists by efficiently cleaning up between tests, particularly useful with multiple connections or database types.
When dealing with external APIs, mocking and stubbing are essential for isolation. Mocking the API client and stubbing its methods with predefined responses safeguards tests from external fluctuations.
Handling Complex Testing Scenarios
Minitest is equipped to handle complex scenarios, including file operations, multi-threaded code, and error conditions. Use temporary files or mock the file system to isolate file operation tests. concurrent-ruby
provides finer control for multi-threaded testing, simplifying concurrency management.
Performance Optimization for Your Test Suite
As your test suite expands, performance becomes crucial. Ruby Minitest offers tools to streamline execution time. Parallel testing, facilitated by gems like parallel_tests
, can significantly reduce overall test runtime.
Focus on core test logic and consider tools like FactoryDefault
from the test-prof
gem to manage complex factory cascades that can slow down test execution.
Testing Edge Cases and Private Methods
Minitest allows comprehensive testing, including edge cases. When necessary, you can even access private methods for testing using Ruby's reflection capabilities. Employ setup and teardown methods to maintain consistent test states across complex scenarios.
By applying these advanced Ruby Minitest techniques, you can create comprehensive and efficient test suites, improving code quality and streamlining your development workflow. A well-structured and optimized Minitest suite ensures robust and maintainable code. Consider integrating your testing with platforms like Mergify for automated CI/CD.
Supercharging Ruby Minitest With Strategic Extensions
Extending Ruby Minitest can greatly improve your testing workflow while keeping its simplicity. This section explores key plugins used by Ruby developers, offering practical examples and advice on selecting the right extensions.
Key Plugins for Enhanced Testing
Several plugins boost Minitest, tackling common testing issues. Three particularly helpful extensions are minitest-reporters
, minitest-focus
, and minitest-hooks
.
minitest-reporters
: This plugin provides better test output formatting. The standard Minitest output can be hard to read, especially in large projects.minitest-reporters
offers several reporters, likeSpecReporter
orJUnitReporter
, creating clearer, more structured output. This simplifies finding failing tests and understanding results.minitest-focus
: When developing, you often need to run only certain tests.minitest-focus
lets you tag specific tests and run only those tagged tests or exclude them. This speeds up the feedback loop by focusing on tests related to the code you're working on. Addfocus
to the tests you want to run, like this:focus def test_my_feature; ...; end
.minitest-hooks
: This plugin adds advanced setup and teardown options. It provides hooks such asbefore_all
,after_all
,around
, andaround_all
, allowing for more complex test lifecycle management. This is especially useful for setting up external dependencies or managing shared state across tests.
The following table summarizes these key plugins:
Plugin Name | Core Functionality | Installation | Best Use Case |
---|---|---|---|
minitest-reporters |
Clearer Test Output | gem install minitest-reporters |
Large test suites, CI integration |
minitest-focus |
Targeted Test Runs | gem install minitest-focus |
Faster feedback during development |
minitest-hooks |
Sophisticated Setup/Teardown | gem install minitest-hooks |
Managing complex test dependencies |
This table offers a quick overview of each plugin's core function, how to install it, and its ideal use case.
Evaluating and Implementing Extensions
When choosing a Minitest extension, consider its quality, activity, and compatibility with your current testing setup. Actively maintained plugins with good documentation are usually better choices. Test any new plugin thoroughly in a staging environment before adding it to your main workflow.
Adding these plugins usually involves adding them to your Gemfile
and requiring them in your test helper file. For example, to use minitest-reporters
, add gem 'minitest-reporters'
to your Gemfile
, run bundle install
, and then add require 'minitest/reporters'
to your test_helper.rb
file.
Extending vs. Custom Solutions
Sometimes, extending Minitest is better than building custom solutions. Plugins often offer a quicker, more maintainable approach to common testing problems. However, for very specific needs, a custom solution might be required. Always consider the benefits and drawbacks of using a plugin versus a custom solution based on your project's needs and the long-term maintainability of your tests. Consider tools like Mergify to automate your CI process, as efficient testing is crucial for rapid development. Mergify's automation features, like automatic pull request updates and merge queue management, allow developers to focus on writing effective tests. A strong Ruby Minitest suite, enhanced with well-chosen extensions, is a valuable asset for building reliable and maintainable Ruby applications.
Ruby Minitest in Rails: A Perfect Testing Partnership
Rails and Ruby Minitest make a great team for testing. This built-in pairing simplifies testing your entire Rails application without adding extra complexity. Let's explore how this synergy makes testing various aspects of your Rails projects easier.
Testing Models, Controllers, and Integrations
Effective Rails testing needs to cover models, controllers, and how they connect. Ruby Minitest provides a clean, efficient way to do this. For example, when testing models with complex relationships, Minitest lets you easily set up test data using fixtures or factories. You can then verify the integrity of those relationships.
When testing controllers with nested actions, Minitest lets you simulate user requests and check the responses. This ensures your routing and logic work as expected. For integrations between different components, Minitest lets you test the entire interaction flow, validating data consistency and proper communication throughout your application.
Leveraging Rails-Specific Testing Helpers
Rails provides helpful tools designed to simplify testing common Rails features. Ruby Minitest works perfectly with these helpers. This allows you to, for instance, simulate user logins to test authentication workflows. You can also use built-in helpers for testing background jobs, ensuring they run correctly and handle various situations. This integration cuts down on complicated setup or custom test utilities.
Testing Authentication, Authorization, and Background Jobs
Testing security features like authentication and authorization is essential for web applications. Ruby Minitest, combined with Rails' testing helpers, makes it easy to thoroughly test these features. You can simulate different user roles and permissions, check access control logic, and ensure protected resources stay secure. You can also simulate requests to restricted routes and verify correct authorization processes are used.
Background jobs, crucial for handling long tasks outside the request-response cycle, are easily tested with Minitest. Using Rails' testing tools, you can check that jobs are queued correctly, execute as expected, and handle both successful completions and errors. This helps keep your application reliable and responsive.
Managing Test Growth and Maintaining Speed
As your Rails application grows, so will your tests. This creates challenges in managing complexity and keeping the test suite fast. Minitest helps you implement organizational patterns to handle a large number of tests. For example, grouping tests by features or modules improves readability and maintainability. This structure makes managing a large suite less overwhelming and makes it easier to find related tests.
Techniques like parallel testing can further improve your Minitest suite’s speed. This speeds up development and shortens the feedback loop. You can use tools like parallel_tests
and the test-prof
gem, specifically FactoryDefault
. This gem helps deal with factory cascades, a common issue caused by nested associations and callbacks that can slow down tests. FactoryDefault
creates default records for associations, reducing database overhead and improving performance. These strategies help maintain a fast and efficient test suite as your application scales.
By combining Ruby Minitest and Rails, you can create a strong, maintainable, and efficient testing strategy. This approach empowers you to test your entire application, improve code quality, and boost productivity while minimizing the risk of regressions. Integrations with tools like Mergify can further automate your CI/CD pipeline, automatically triggering tests and presenting results clearly, making your testing even more efficient.
Ruby Minitest Best Practices From the Trenches
After exploring the intricacies of Ruby Minitest, let's discuss practical advice from experienced Ruby developers. These experts have built and maintained substantial Minitest suites, and their insights can help you avoid common pitfalls and write robust, maintainable tests.
Organizing Your Minitest Tests
A well-organized test suite is essential for maintainability, especially as your project grows. A clear structure simplifies locating, updating, and understanding individual tests. Many developers prefer a modular approach, grouping tests by feature or domain.
For example, user authentication tests might reside in test/users/authentication_test.rb
, while product management tests could live in test/products_test.rb
. This logical grouping simplifies navigation and helps pinpoint tests related to specific areas of your application.
Naming Conventions for Clarity
Descriptive test names are vital. A good name clearly communicates the scenario and expected outcome. test_user_login_success
instantly conveys the test's purpose. In contrast, a vague name like test_user_1
offers little insight.
Clear naming conventions save time and reduce the risk of misinterpreting results. They allow you to grasp each test's purpose without digging into the implementation.
Preventing Test Brittleness With Isolation Techniques
Test brittleness, where tests break due to unrelated code changes, is a common problem. Isolation techniques like mocking and stubbing minimize this. By isolating the unit under test from its dependencies, tests only fail when the core logic has a problem.
This focused approach makes tests more reliable and reduces debugging time. You can focus on fixing genuine issues instead of chasing phantom failures caused by peripheral changes.
Balancing Test Coverage and Development Velocity
Comprehensive test coverage is important, but it shouldn't come at the expense of development speed. Writing tests for every single edge case can slow you down. Instead, focus on core functionalities and critical user flows.
As your project grows, you can strategically increase coverage based on risk and usage patterns. This pragmatic approach maximizes the value of your tests without hindering progress. Tools like Mergify can help streamline your Continuous Integration (CI) process.
Automating tasks like pull request merging and updating keeps development fast even with a growing test suite. Mergify's emphasis on CI cost reduction and improved developer experience complements the goal of efficient, reliable testing with Ruby Minitest.