Master RSpec Tests: Boost Your Code Quality
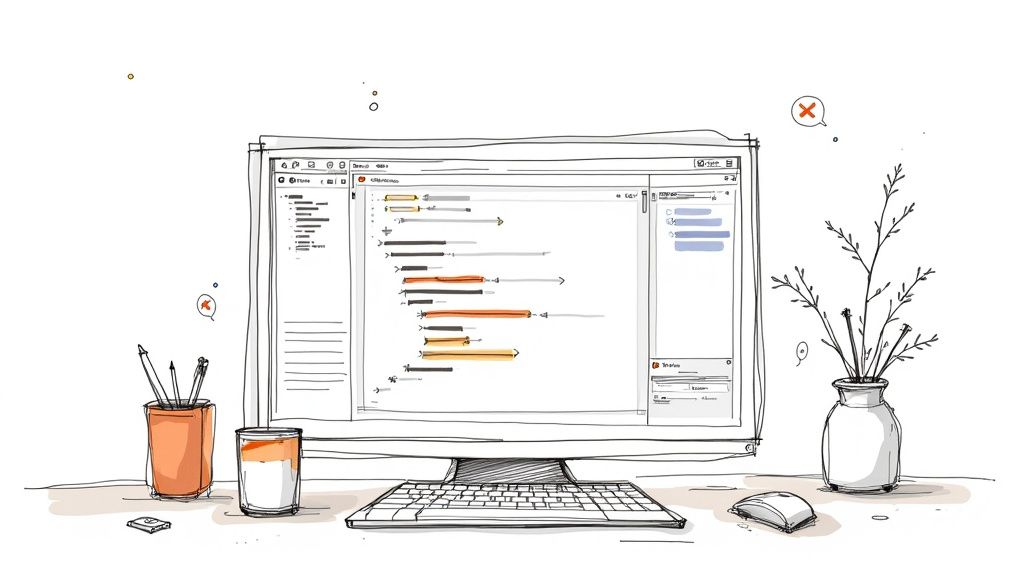
Mastering RSpec Tests Fundamentals
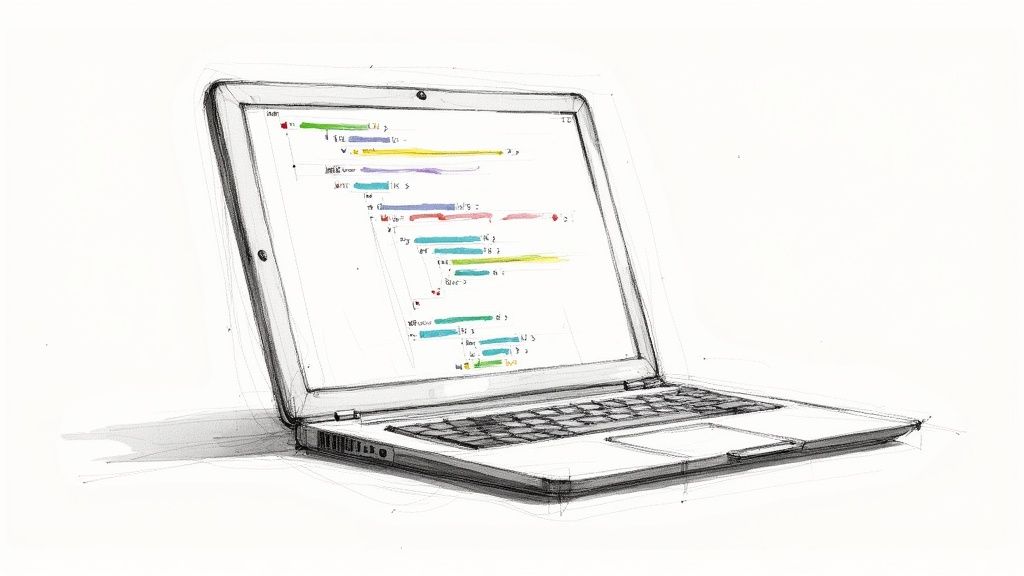
RSpec tests are essential for Ruby development. They provide a solid framework for behavior-driven development (BDD). Instead of testing how code functions, BDD focuses on what the code should accomplish.
This approach allows developers to describe their code's intended behavior clearly. The result is improved code quality and fewer bugs. This section explores the basics of writing effective RSpec tests.
Understanding the 'Describe' and 'It' Blocks
The foundation of RSpec tests lies in describe
and it
blocks. The describe
block establishes a context or a particular area of functionality. Think of it as grouping related tests.
For instance, when testing a User
model, you might have a describe
block dedicated to authentication methods. Inside each describe
block, it
blocks define individual test cases. Each it
block details a specific behavior or expectation.
describe User do describe '#authenticate' do it 'returns true with valid credentials' do # Test logic here end
it 'returns false with invalid credentials' do
# Test logic here
end
end end
This structure results in an organized and understandable test suite. It clearly shows which part of the codebase each test covers.
The Power of Expectations
Expectations are the core of behavior verification in RSpec. They specify the expected outcome of a particular code segment. RSpec offers a wide range of matchers to express these expectations clearly and expressively.
For example, expect(user.authenticate('correct_password')).to be_truthy
verifies that the authentication method returns a true value with the right credentials. On the other hand, expect(user.authenticate('wrong_password')).to be_falsey
checks for a false value with incorrect credentials. This clearly defines expected outcomes, improving test readability.
Focusing on Behavior, Not Implementation
Effective RSpec tests prioritize behavior over implementation. This means focusing on the functionality of specific features or associations, not the technical details of how they're implemented.
Consider a Classroom
model with a has_many :students
association. Instead of testing the association's existence, you should test the behavior it enables. For example, test calculating the average GPA of students in a classroom.
This approach creates robust tests and allows flexibility in changing implementations without disrupting your tests. Tests focused on behavior tend to have better code coverage and are more resilient to code changes. Learn more about this approach: Learn more about behavior-driven testing. This leads to less brittle tests and greater confidence during refactoring. Your tests will continue to reflect intended behavior, even after underlying code modifications.
Crafting RSpec Tests That Actually Work
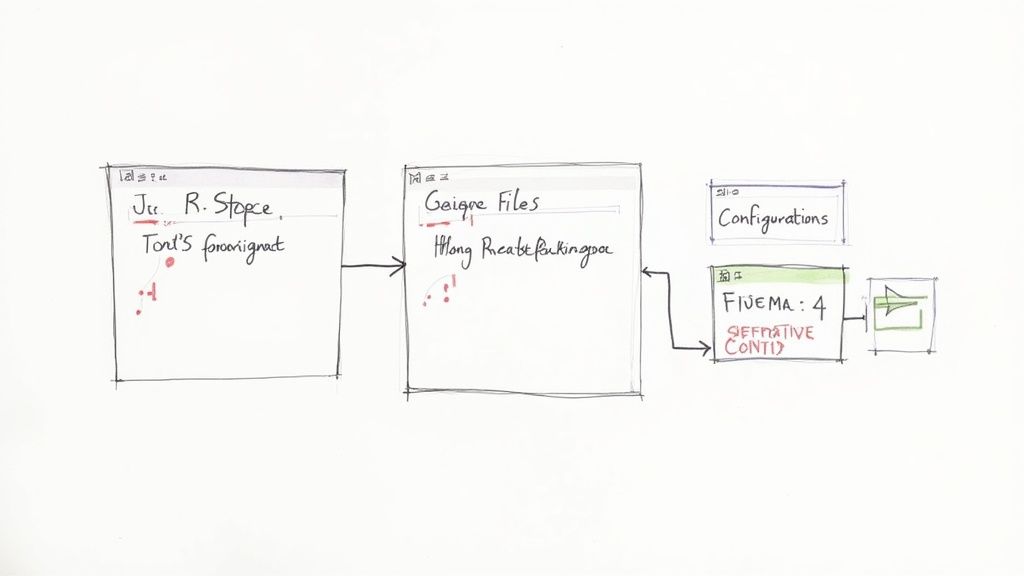
Knowing the basic syntax of RSpec is just the first step. The real power lies in writing tests that are both effective and easy to maintain. This means understanding how to structure your rspec tests
to act as living documentation while simultaneously catching real bugs. Finding the right balance between comprehensiveness and maintainability is the key to a healthy test suite.
Avoiding Brittle Tests
One of the most common testing pitfalls is creating brittle tests. These tests break easily with small code changes, even if the core functionality still works correctly. Brittle tests often stem from testing implementation details instead of behavior.
For example, a brittle test might check the precise format of an internal data structure. This structure could change without affecting how the user interacts with the software. Instead, concentrate on testing the public interface and the observable behavior of your code.
This approach makes your tests more resilient to implementation changes, reducing maintenance overhead and boosting developer productivity. It also allows for more flexibility when refactoring and improving your codebase.
The Pitfalls of Excessive Mocking
Another frequent issue is excessive mocking. While mocking is a valuable tool for isolating units of code, overusing it can tightly couple your tests to the implementation. This makes refactoring or changing your code difficult without breaking tests.
Aim for a balanced approach. Mock external dependencies and collaborators to isolate the unit under test, but avoid mocking the object you're actually testing. Concentrate on verifying interactions with those collaborators and ensuring the expected behavior of your unit.
Balancing DRY and DAMP Principles
Finding the sweet spot between DRY (Don't Repeat Yourself) and DAMP (Descriptive And Meaningful Phrases) is essential. DRY principles encourage minimizing code duplication. However, sometimes a little redundancy in tests improves readability and clarity.
Following best practices when writing RSpec tests is crucial for maintaining efficient, readable, and reliable test suites. A key principle is balancing DRY and DAMP, sometimes duplicating code to enhance readability. For instance, using let
statements instead of before
blocks ensures variables are created only when needed, speeding up test execution. Also, avoid stubbing the object under test; mock collaborators instead. This ensures each test is independent and relevant, building confidence in the codebase. Learn more about this: Discover more insights about balancing DRY and DAMP in RSpec tests.
Testing Edge Cases Effectively
Finally, think about which edge cases truly matter. Don't spend time testing scenarios unlikely to occur in real-world use. Instead, focus on edge cases relevant to the core functionality and those that could cause major bugs or security vulnerabilities.
Prioritizing these critical edge cases ensures your rspec tests
provide maximum value and effectively protect your codebase. This focused approach ensures your test suite is lean, efficient, and targets real-world problems.
By following these strategies, your RSpec tests will become robust safeguards, protecting your code and improving your development process. The result? A more robust and reliable application built on a foundation of well-crafted rspec tests
.
Supercharging Your RSpec Tests Performance
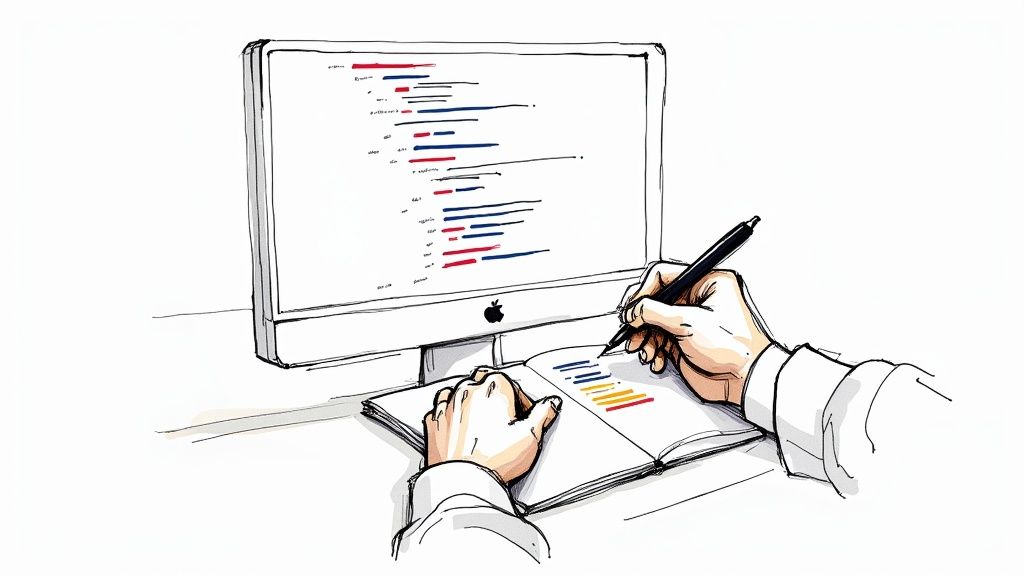
Slow rspec tests
can significantly impact your development workflow. They can turn a safety net into a frustrating bottleneck. This section explores practical techniques to optimize your RSpec test suite, transforming it from a blocker into a productivity booster. We'll examine real-world strategies that deliver real performance gains.
Identifying Performance Bottlenecks
Before optimizing, it's crucial to pinpoint the bottlenecks in your test suite. Identifying these slow points focuses your efforts for maximum impact. Are your tests spending too much time interacting with the database? Are there too many external API calls? Perhaps the setup and teardown phases are too complex.
Understanding the source of the slowdown is the first step toward a faster, more efficient test suite. This allows for targeted optimization, maximizing performance improvements with minimal effort.
Optimizing Test Data Management
Efficient test data management is essential for optimal performance. Consider using tools like Factory Bot's build_stubbed
to create lightweight objects without hitting the database. This avoids the overhead of database operations, significantly speeding up test execution, especially with complex object graphs.
Also, avoid excessive data creation within your tests. If tests don't need unique data, reuse existing data or create shared examples to reduce redundant setup. This reduces database load and improves test runtime.
Leveraging Parallel Testing
Running tests in parallel can drastically reduce execution time. Tools like parallel_tests distribute tests across multiple CPU cores, maximizing hardware usage. This is a game-changer for large test suites, allowing for faster feedback and quicker iteration cycles. Teams can run tests concurrently, drastically reducing overall testing time.
However, ensure your tests are isolated to avoid conflicts when running in parallel. Proper test isolation is crucial to prevent unexpected behavior and ensure accurate results when running tests concurrently. This often involves carefully managing shared resources and avoiding global state changes within tests.
The following table summarizes some key RSpec test optimization techniques:
RSpec Tests Performance Optimization Techniques
Optimization Technique | Implementation Complexity | Potential Speed Improvement | Best Used For |
---|---|---|---|
Using build_stubbed |
Low | High | Tests not requiring database persistence |
Reusing test data | Low | Moderate | Tests with similar data requirements |
Parallel testing with parallel_tests |
Moderate | High | Large test suites |
Avoiding loading the full Rails environment | Low | Moderate | Tests not using the entire Rails stack |
This table provides a quick overview of various optimization strategies, their complexity, and potential benefits. Choosing the right technique depends on the specific needs and characteristics of your test suite.
Fine-Tuning RSpec Configuration
Small configuration changes can significantly impact RSpec performance. If you're not using the full Rails stack in your tests, configure RSpec to avoid loading the entire Rails environment. This can significantly speed up individual test runs.
Experiment with different configurations to find what works best for your project. Profiling tools can be helpful for understanding the impact of various configuration options. They provide insights into where time is being spent during test execution, allowing for data-driven optimization.
The Importance of Continuous Monitoring
Optimizing your rspec tests
is an ongoing process. Regularly monitor your test suite's performance to identify regressions and areas for improvement. Set up performance benchmarks and track execution times over time.
This proactive approach helps you maintain a fast and efficient test suite. It prevents slowdowns and ensures that your tests remain a valuable asset in your development workflow. Consistent monitoring enables proactive optimization, catching performance regressions early and minimizing their impact. It ensures your test suite remains valuable, providing rapid feedback without hindering workflow.
Taking Your RSpec Tests to the Next Level
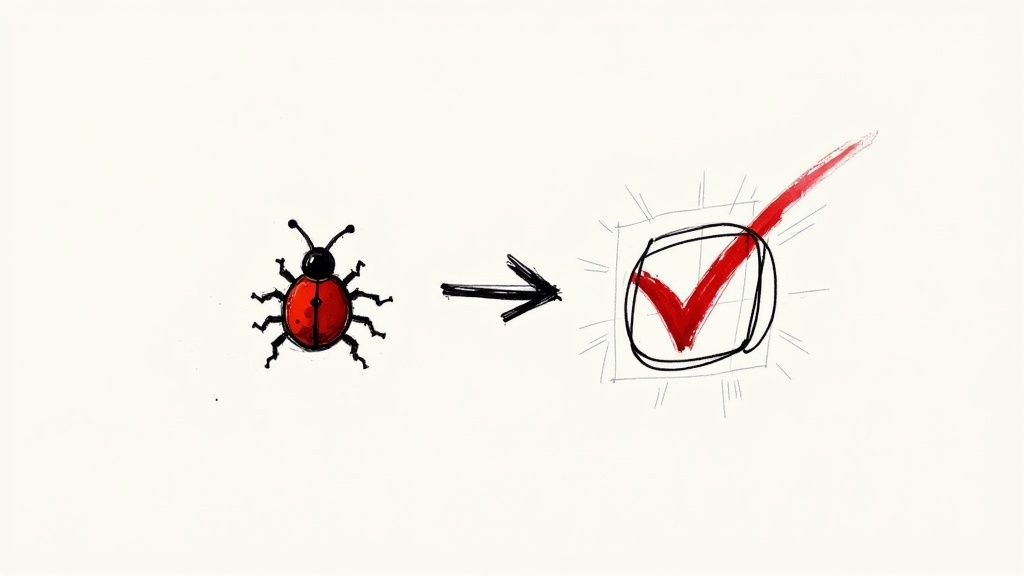
So you've got the basics of rspec tests
down and are writing tests that truly make a difference. Excellent! Now, let's explore how to take your testing to the next level with advanced techniques that set truly great test suites apart. This section explores how experienced Ruby teams handle tricky testing situations, going beyond the fundamentals to achieve testing mastery.
Conquering Complex Testing Scenarios
Seasoned Ruby developers know that real-world applications often present complexities like asynchronous operations, intricate state management, and essential third-party integrations. These situations require specific testing approaches.
For example, testing asynchronous operations might involve libraries like rspec-mocks. You can use this to stub or mock time-dependent functions, ensuring predictable test behavior.
Similarly, managing complex state requires careful setup and teardown procedures. This ensures test isolation and avoids unwanted side effects.
Testing third-party integrations presents its own set of challenges. It's crucial to carefully mock external services to keep your tests fast and dependable. Mocking external API calls avoids slowdowns and removes external dependencies during testing, while also letting you test diverse scenarios.
The Art of Custom Matchers
One of RSpec's strengths is its support for custom matchers. Creating these allows you to express complex expectations clearly and concisely. This makes your rspec tests
more readable and easier to maintain.
Imagine frequently testing a string against a specific regular expression. Instead of writing the same code repeatedly, you can encapsulate this logic within a custom matcher. This simplifies your test code and makes its purpose more evident. This not only boosts readability but also promotes a more declarative and expressive testing style.
Leveraging Shared Contexts and Metadata
Shared contexts offer a powerful way to group common setup and helper methods. This encourages DRY (Don't Repeat Yourself) principles within your test suite. However, use them wisely to avoid overly complex and confusing tests. Keep shared contexts focused and relevant to a specific functional area, making sure they clarify rather than obscure.
Metadata, on the other hand, lets you tag tests with descriptive information. This allows for powerful filtering and focused test runs. For example, you can tag tests related to specific features, allowing you to execute only those tests during development. This focused approach streamlines your workflow and allows for a more efficient use of testing resources.
Performance Testing with RSpec-Benchmark
As applications grow more complex, the demand for both functional and performance reliability intensifies. RSpec-Benchmark provides a powerful way to integrate performance metrics into your RSpec tests. It allows you to set expectations for how quickly your code executes and how many iterations per second it handles.
The perform_under
matcher lets you specify expected execution times, for example, requiring code to complete in under 6 milliseconds. This becomes especially critical for high-performance applications, where even small code changes can subtly impact execution speed. This early identification of performance regressions allows for proactive optimization.
These advanced techniques aren't just theoretical concepts—they're practical solutions derived from real-world testing challenges. By incorporating these strategies, your rspec tests
become a valuable asset, assuring code quality, maintainability, and ultimately, project success. These are the skills that elevate test suites from effective to exceptional, ensuring code quality and long-term project health.
Understanding What Your 20.8% RSpec Tests Are Really Doing
Beneath the surface of your rspec tests
lies a treasure trove of information about your codebase's health. This goes beyond simply checking whether tests pass or fail. It's about understanding how your tests perform and what they reveal about the underlying code. Top Ruby teams use profiling tools to gain deep insights into their tests' behavior, catching problems that traditional testing often misses.
Unveiling Hidden Bottlenecks
A key benefit of profiling is its ability to uncover hidden bottlenecks. These often stem from inefficient database interactions, memory leaks, or unforeseen external dependencies. Imagine a test retrieving a large dataset from the database. Without profiling, pinpointing whether the retrieval itself or the subsequent data processing is the bottleneck can be difficult.
Profiling offers granular data, highlighting which code lines or methods consume the most time. This targeted insight allows you to focus your optimization efforts precisely where needed, maximizing performance gains.
Identifying Memory Issues and Test Interdependencies
Profiling also helps identify memory issues. Tests that consume excessive memory or create leaks can impact your entire test suite's performance and even affect your application. Profiling tools expose these issues, allowing you to optimize memory management and prevent problems in production.
Moreover, profiling can uncover test interdependencies. Sometimes tests unintentionally rely on side effects from earlier tests, causing unpredictable and flaky behavior. Profiling helps identify these dependencies, so you can refactor your tests for better isolation and reliability.
Case Studies and Real-World Examples
Real-world examples clearly demonstrate the value of profiling. Tools like StackProf and rblineprof have proven instrumental in optimizing RSpec tests. They pinpoint slow parts of the test suite, letting developers concentrate on areas like database interactions, which often consume the most execution time. A common scenario is that database statements like ActiveRecord::ConnectionAdapters::PostgreSQL::DatabaseStatements#execute
account for a substantial amount of test time, sometimes up to 20.8% of total execution samples. By profiling rspec tests
, developers can uncover surprises like memory leaks or unexpected HTTP requests that might otherwise go unnoticed. Tools like rblineprof offer line-by-line data, showing which methods are the most time-consuming and helping developers streamline tests by removing unnecessary computations or optimizing database access. More statistics can be found here: Explore this topic further.
For example, one team found a seemingly simple test was making hundreds of unnecessary database queries. By optimizing the test to retrieve the data with a single query, they decreased its execution time by 90%. These performance gains significantly impact developers' daily work.
To further illustrate the comparison of different profiling tools, the following table highlights key features and use cases.
RSpec Tests Profiling Tools Comparison Evaluation of different profiling tools for RSpec tests with their features and use cases
Tool | Focus Area | Integration Complexity | Output Detail | Ideal Use Case |
---|---|---|---|---|
StackProf | Overall CPU time | Low | Method-level | Identifying performance bottlenecks in general |
rblineprof | Line-by-line | Medium | Line-level | Pinpointing slow lines within specific methods |
This table summarizes the strengths of each tool, helping you choose the best one for your specific needs. StackProf provides a broad overview of performance, while rblineprof offers a more granular view, ideal for deep dives into particular methods.
Establishing Effective Monitoring Systems
Finally, profiling provides the data needed to set up effective monitoring systems. By tracking key metrics over time, you can identify performance regressions and prevent your test suite from slowing down. This proactive approach helps maintain a fast and efficient test suite, ensuring that your tests remain a valuable asset in your development workflow. Continuous monitoring guarantees that your investment in testing pays off by preventing issues from accumulating silently and hindering your development process.
Making RSpec Tests Work in Your Team's Workflow
The most comprehensive rspec tests
are useless if they don't integrate into your team's workflow. This section explores practical strategies for incorporating RSpec into modern CI/CD pipelines and development processes. We'll see how successful teams balance thorough testing with rapid delivery.
Integrating RSpec Tests into CI/CD Pipelines
Continuous Integration and Continuous Delivery (CI/CD) pipelines depend on automated tests. RSpec tests should be a core part of this automation. Configure your CI/CD system, such as Jenkins, to run your RSpec suite after every code change. This provides immediate feedback and catches regressions early.
Effective CI integration requires fast test execution. Use optimization techniques to minimize test runtime. This ensures quick feedback loops and prevents CI from becoming a bottleneck. Fast tests are crucial for efficient CI/CD.
Structuring Tests for Collaboration
How you structure your rspec tests
significantly impacts team collaboration. Organize tests by feature or component, mirroring your application code's structure. This makes it easier for team members to find and modify relevant tests.
Establish clear conventions for test naming, setup, and teardown. Consistent practices improve code readability and reduce errors. This simplifies code reviews and promotes knowledge sharing.
Handling Flaky Tests
Flaky tests—those that pass or fail intermittently without code changes—are a common problem. They erode trust in the test suite and slow down development.
Address flaky tests proactively. Tools like Buildkite can help identify and track them. Prioritize fixing these tests, as they can be a significant source of frustration and wasted time. Investigate the root cause of flakiness, which could be anything from timing issues to external dependencies.
Establishing Team Conventions
Effective testing requires shared responsibility. Establish clear conventions about who writes tests and when. Some teams use Test-Driven Development (TDD), writing tests before the code. Others write tests alongside or after implementation.
Regardless of your approach, ensure everyone understands their testing role. Define expectations and provide training as needed. This shared understanding promotes a culture of quality and integrates testing into every development stage.
Improving Test Coverage in Legacy Codebases
Introducing rspec tests
to a legacy codebase can feel overwhelming. Start small, focusing on critical areas or new features. Gradually expand test coverage over time.
Prioritize testing code prone to bugs or frequent changes. Don't aim for 100% coverage immediately. Focus on meaningful coverage that provides real value and confidence in the codebase. Gradual improvement is key to successful test integration in legacy projects.
By integrating these strategies, your team can utilize the full power of RSpec. Your tests become more than just checks; they become integral to your workflow, ensuring code quality, facilitating collaboration, and leading to better software. These practical steps improve team efficiency and confidence.
Ready to streamline your team's merge workflow and improve CI efficiency? Explore how Mergify can help.