Master RSpec Ruby: Proven Testing Techniques
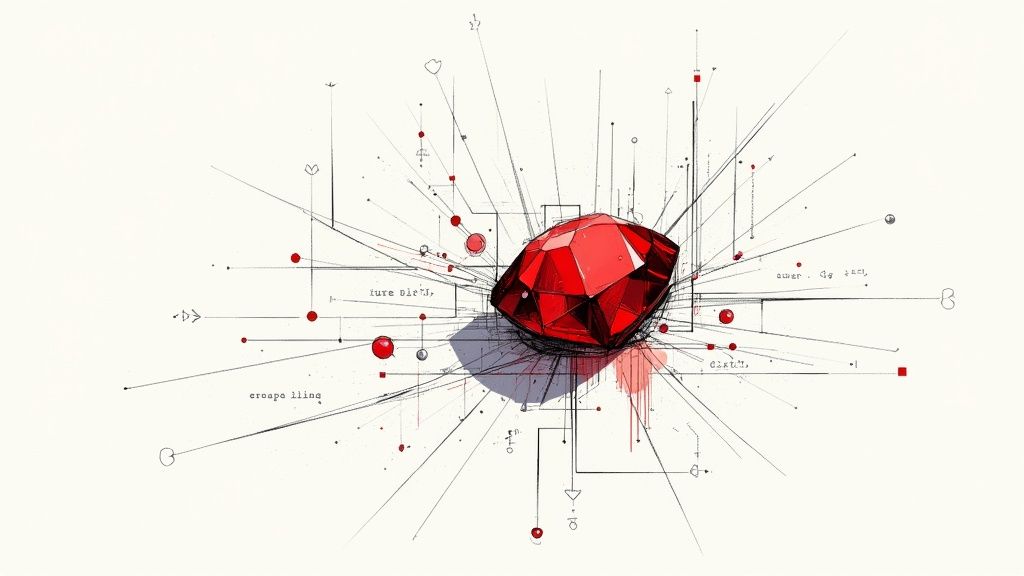
Getting Started with RSpec Ruby
Feeling overwhelmed by testing? You're not alone. This section breaks down the fundamentals of RSpec for Ruby, guiding you toward building confidence in your testing process. We'll explore installation and configuration with practical examples, highlighting why RSpec is a favored choice for behavior-driven development (BDD). You'll learn how to structure your first test suite and grasp the core principles behind RSpec's power and accessibility. By the end of this section, you'll be prepared to write tests that not only verify functionality but also act as clear documentation.
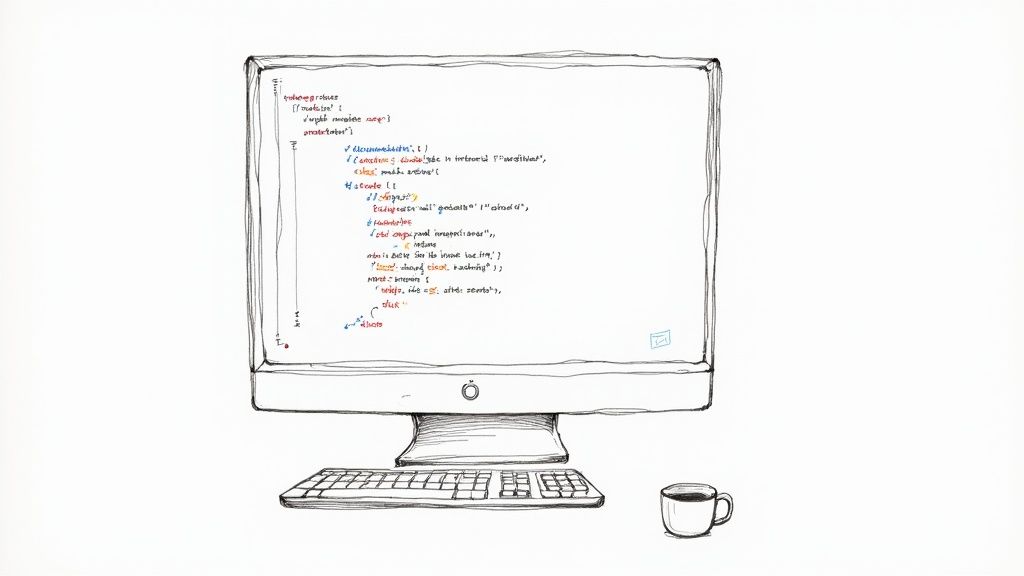
Installing and Configuring RSpec
Before writing tests, you need to prepare your environment. This generally involves incorporating RSpec into your project's Gemfile
and then installing it.
- Add
gem 'rspec'
to your Gemfile. - Run
bundle install
in your terminal. - Initialize RSpec in your project with
rspec --init
. This generates necessary files like.rspec
andspec/spec_helper.rb
.
This lays the groundwork for crafting your first tests. RSpec is recognized for its simple installation, making it easy to incorporate into existing Ruby projects. This initial setup empowers you to begin writing tests almost instantly.
Your First RSpec Test
Let's illustrate with a straightforward example. Suppose you have a User
class equipped with a full_name
method. You want to verify that this method accurately combines the first and last names.
user.rb
class User attr_reader :first_name, :last_name
def initialize(first_name, last_name) @first_name = first_name @last_name = last_name end
def full_name "#{first_name} #{last_name}" end end
spec/user_spec.rb
require 'user'
RSpec.describe User do it "returns the full name" do user = User.new("Jane", "Doe") expect(user.full_name).to eq("Jane Doe") end end
In this example, RSpec.describe User
designates the subject under test. The it
block outlines the anticipated behavior. The expect(user.full_name).to eq("Jane Doe")
line performs the actual assertion. Executing rspec
in your terminal will run this test.
RSpec is widely used for testing Ruby applications in production. This prevalence underscores its significance in BDD. Its simplicity and effectiveness make it an important resource for Ruby developers striving for robust and dependable code. The framework's strength lies in its readable DSL, which promotes maintainable test suites. Learn more about RSpec here.
Structuring Tests With Describe and Context
As your test suite expands, maintaining organization is key. RSpec offers describe
and context
blocks for arranging related tests. describe
groups tests associated with a specific class or module, while context
provides further grouping based on diverse scenarios or conditions.
For instance, you could utilize context
to test the full_name
method with various inputs:
RSpec.describe User do context "with valid first and last names" do # ... tests ... end
context "with an empty first name" do # ... tests ... end end
This structured approach simplifies understanding and maintenance as your application evolves, resulting in more resilient and thoroughly tested code. This organization also makes pinpointing specific test cases more intuitive. This clear framework prepares you for more advanced RSpec techniques.
To help you get started with RSpec in your Ruby projects, here’s a table outlining the setup requirements:
RSpec Ruby Setup Requirements
Component | Version | Purpose | Installation Command |
---|---|---|---|
Ruby | 2.5+ | Programming Language | apt-get install ruby or similar |
Bundler | Latest | Gem dependency manager | gem install bundler |
RSpec | 3.0+ | Testing framework | gem install rspec |
This table outlines the necessary components, their versions, and the commands to install them. Ensure you have Ruby and Bundler installed before proceeding with RSpec. This will equip you with the fundamental tools needed to start using RSpec effectively.
Core RSpec Ruby Patterns That Actually Work
Building a maintainable test suite is crucial for any successful software project. A well-designed suite makes development enjoyable, while a poorly structured one can become a major headache. This section explores practical RSpec patterns for creating tests that are easy to understand, modify, and expand.
The Describe-Context-It Structure
RSpec offers a clear structure for organizing tests: describe
, context
, and it
. describe
blocks define what's being tested, often a class or module. context
blocks, nested within describe
blocks, outline specific scenarios or conditions. it
blocks contain the individual test cases.
For example, consider testing a ShoppingCart
class:
RSpec.describe ShoppingCart do context "when empty" do it "has a total of zero" do # ... test logic ... end end
context "with items" do it "calculates the total price correctly" do # ... test logic ... end end end
This structure improves readability, especially as the test suite grows. Each it
block should test one specific behavior.
Expectations and Matchers
Expectations and matchers are central to RSpec tests. Expectations define the expected outcome of an action. Matchers express these expectations clearly.
Here's an example:
expect(user.full_name).to eq("Jane Doe")
expect(user.full_name)
sets the expectation, and to eq("Jane Doe")
is the matcher. RSpec provides many matchers, from simple equality (eq
, be
) to more complex comparisons (include
, match
). This allows for precise assertions about expected behavior.
Test Doubles: Mocks, Stubs, and Spies
Testing interactions between different parts of your application can be tricky. Test doubles – mocks, stubs, and spies – simplify this by simulating dependencies.
- Mocks: Verify that specific methods are called with the expected arguments.
- Stubs: Force methods to return predefined values, bypassing their actual implementation.
- Spies: Monitor method calls and arguments without modifying the original method's behavior.
The best choice depends on your testing scenario. Using test doubles effectively improves test clarity and efficiency, ensuring you test individual code units in isolation.
Hooks: Before and After
RSpec provides hooks – before
and after
– to run code before or after test blocks. These are especially useful for setting up and tearing down common test fixtures.
For instance, use a before
hook to create a database record needed for several tests within a describe
block. This reduces code duplication and improves consistency. RSpec also offers advanced features like composable matchers, which allow for nesting and expressing more complex assertions.
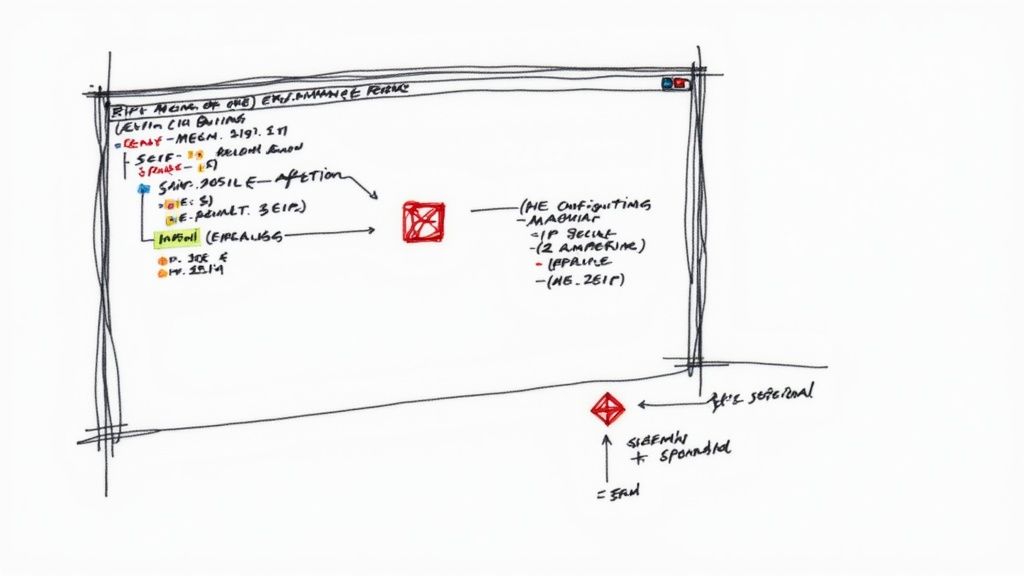
By mastering these RSpec Ruby patterns, you can build a robust and maintainable test suite. These practices improve code quality, boost developer confidence, catch regressions early, and maintain high code quality, saving time and resources.
Advanced RSpec Ruby Techniques Worth Mastering
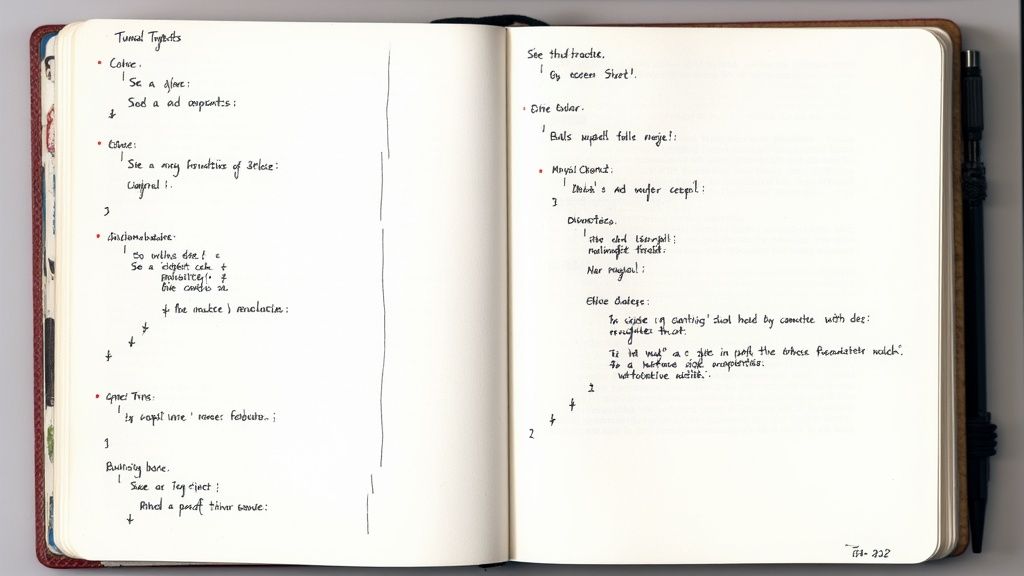
Building upon core RSpec patterns, this section delves into advanced techniques to elevate your Ruby test suite. These methods promote expressive tests, helping you identify subtle bugs before they reach your users.
Shared Examples and Contexts for DRY Tests
Repetitive test code often indicates inefficiency. RSpec's shared examples and shared contexts offer a solution for reusing code, adhering to the DRY (Don't Repeat Yourself) principle and boosting consistency.
Consider multiple classes implementing a similar interface, such as a Validatable
module. You can define shared examples for validation rules and apply them to each class. This minimizes redundancy and ensures consistent interface adherence.
For example:
RSpec.shared_examples "a validatable object" do it "is valid with valid attributes" do expect(subject).to be_valid end
it "is invalid without a name" do subject.name = nil expect(subject).to_not be_valid end end
RSpec.describe User do include_examples "a validatable object" end
RSpec.describe Product do include_examples "a validatable object" end
This concise approach eliminates rewriting identical tests for each class, improving maintainability.
Custom Matchers for Domain-Specific Assertions
While RSpec's built-in matchers are extensive, your application might require more specific assertions. Custom matchers extend RSpec's DSL, allowing you to create expectations tailored to your domain. This enhances readability and clarifies test failures.
For example, frequent email validation checks benefit from a be_a_valid_email
matcher:
RSpec::Matchers.define :be_a_valid_email do match do |email| # Regular expression or other validation logic email =~ /\A[\w+-.]+@[a-z\d-]+(.[a-z\d-]+)*.[a-z]+\z/i end end
expect(user.email).to be_a_valid_email
This concisely expresses complex expectations within your tests.
Mastering Metadata for Fine-Grained Control
RSpec uses metadata to tag and manage test execution. This allows categorizing, running subsets, or skipping tests under specific conditions. This is invaluable for large projects requiring efficient test management.
You can tag tests with key-value pairs:
it "performs some action", :slow => true do
Time-consuming test logic
end
Then, execute specific tests using the --tag
option:
rspec --tag slow
As your testing needs expand, tools like let
, subject
, and context blocks become crucial. The let
method offers lazy evaluation for optimized test setup. Using subject
clarifies the object under test, and super()
within context blocks allows modification of previously defined values for increased flexibility. Learn more about these helpful features here: Discover more insights about RSpec Ruby. This allows for complex test setups with minimal code, improving efficiency and maintainability. Mastering these techniques will not only improve your test suite but also make testing more effective. This contributes to a robust and reliable codebase.
RSpec Ruby Performance Testing That Delivers
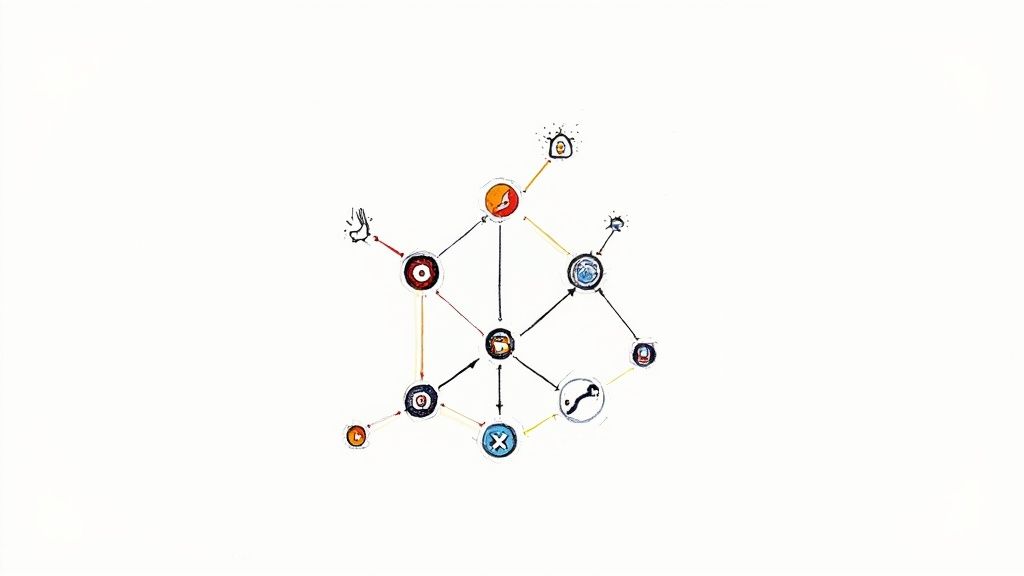
Ensuring your Ruby application functions correctly is paramount. But speed is just as important for a positive user experience. This section focuses on effectively using RSpec to test the performance of your Ruby code. We'll explore building a robust performance testing strategy, preventing performance regressions, and integrating these tests seamlessly into your development workflow.
Establishing Performance Baselines
Before improving performance, you need a baseline. This means measuring the current execution time, memory consumption, and database interaction speeds of key parts of your application. RSpec, combined with tools like the built-in benchmark
or the rspec-benchmark gem, allows you to capture these metrics within your test suite. This establishes a clear starting point for your optimization efforts.
Writing Performance Expectations
With a baseline established, you can define performance expectations. These set limits on acceptable execution times, memory usage, or database query counts. For example, you might specify that a particular method should execute within 100 milliseconds. RSpec lets you express these expectations clearly and maintainably, helping catch performance regressions early.
Measuring Execution Time
Execution time is a common performance metric. RSpec's benchmark
helps you accurately measure how long specific code blocks take to run.
RSpec.describe "Performance of my_method" do it "executes within an acceptable timeframe" do expect { my_method }.to perform_under(100).ms end end
This test ensures my_method
completes within the specified timeframe.
Memory Usage and Database Efficiency
Beyond execution time, memory usage and database efficiency are crucial. While more complex to measure within RSpec, tools like the memory_profiler gem and database profiling tools (like rack-mini-profiler) can integrate into your tests. You can then set expectations for maximum memory allocation or database query counts, ensuring your application remains efficient under load.
Integrating Performance Testing into CI/CD
For maximum impact, integrate performance tests into your Continuous Integration/Continuous Delivery (CI/CD) pipeline. This automates performance checks with every code change, preventing regressions. Tools like Mergify can automate this integration, triggering performance tests during pull request reviews.
Case Studies and Best Practices
Real-world examples offer invaluable insights. Analyzing case studies of applications that maintain both correctness and speed can reveal effective strategies. This includes learning how to identify bottlenecks and optimize for maximum efficiency. Following best practices for organizing and managing performance tests keeps your testing process streamlined and effective.
The following table provides a quick overview of several popular RSpec performance testing tools and their characteristics.
RSpec Performance Testing Tools Comparison
Tool/Gem | Key Features | Best Use Cases | Limitations |
---|---|---|---|
benchmark (built-in) |
Simple time-based benchmarking | Quick performance checks, basic comparisons | Limited detailed metrics |
rspec-benchmark |
Operations per second (ips), detailed comparisons, trend analysis | Focused performance tests, regression detection | Requires additional setup |
memory_profiler |
Memory allocation analysis, detailed reporting | Identifying memory leaks and excessive allocation | Can impact test execution speed |
Database Profilers (e.g., rack-mini-profiler) | Database query analysis, performance insights | Optimizing database interactions, identifying slow queries | Requires specific database support |
This table summarizes the key features, ideal use cases, and potential drawbacks of each tool, allowing you to choose the best fit for your needs.
By implementing these techniques, you can create a comprehensive strategy for ensuring optimal Ruby code performance in real-world scenarios. This proactive approach improves user experience and enhances application stability.
RSpec Ruby in Rails: Strategies That Scale
Testing Rails applications presents unique challenges. This section explores how successful teams leverage RSpec Ruby to overcome these hurdles. Through practical examples, we'll examine testing strategies for each Rails component: models, controllers, views, and API endpoints. This knowledge will empower you to build a robust test suite, boosting confidence with every deployment.
Testing Models and ActiveRecord Relationships
When testing models, prioritize validating core business logic and ActiveRecord relationships. Continuously querying the database during tests, however, slows down the process. To mitigate this, learn how to efficiently use factories and test doubles. FactoryBot allows for the rapid and consistent creation of test data without database interaction. Test doubles isolate model logic from the database, leading to faster, more focused tests. This approach ensures test efficiency while thoroughly verifying data integrity and relational logic.
Controller Testing: Verifying Behavior Without Coupling
Controller tests should verify behavior without getting entangled in implementation details. Avoid testing internal logic better suited for model or unit tests. Instead, focus on the interaction between the controller and the view, HTTP responses, and redirects. Verify the correct data is passed to the view and the controller responds appropriately to different HTTP requests. This approach simplifies testing and reduces the risk of brittle tests breaking with minor code revisions.
Streamlined View Testing with RSpec and Capybara
Testing views in Rails often involves verifying HTML output and user interactions. RSpec seamlessly integrates with Capybara, a library for simulating user actions in a web browser. Capybara allows testing of user workflows, form interactions, and the presence or absence of specific page content. This enables comprehensive UI testing, ensuring the application functions and appears as expected. Capybara facilitates tests that mirror real user actions, leading to more realistic and effective UI testing.
API Endpoint Testing for Robust Integrations
Many modern Rails applications expose API endpoints. Testing these endpoints requires verifying data formats (like JSON or XML), status codes, and authentication. RSpec provides tools to create HTTP requests to your API endpoints and examine responses. You can test different HTTP methods (GET, POST, PUT, DELETE) with various data and headers, ensuring each endpoint adheres to its specifications. These tests are essential for ensuring the stability and reliability of any integrations relying on your API.
Managing Test Data with Factories
As applications grow, so does test data. Factory strategies are crucial for creating and managing realistic test data without cluttering tests. FactoryBot simplifies this process by defining reusable factories for models. You can easily create model variations with specific attributes for different test scenarios. This streamlines test setup and enhances test readability and maintainability. Organized test data management simplifies testing and minimizes test failures due to data inconsistencies.
Real-World Example: Testing User Authentication
Consider a user authentication flow. You might start by testing the user model's validation rules, ensuring password encryption and unique usernames. Next, move to controller tests, verifying successful and failed login attempts, redirects, and session management. Finally, use Capybara to test the entire user flow in a browser, from credential entry to viewing protected content. This thorough approach helps catch regressions across the application's layers.
These combined techniques create a robust and scalable testing strategy for your Rails application. Mastering these techniques allows you to build a test suite that provides confidence with every deployment, letting you focus on feature development instead of bug fixes. For further improvement, consider integrating tools like Mergify into your workflow. Mergify's automated merge queue and CI features significantly reduce the time to run your test suite, especially for larger projects. You can learn more about how Mergify optimizes your CI/CD pipeline here.
RSpec Ruby Best Practices From The Trenches
What separates truly great RSpec Ruby test suites from the merely adequate? This exploration draws upon the wisdom of teams who've successfully navigated the complexities of building and maintaining large-scale RSpec test suites. We'll explore practical organizational techniques, effective naming strategies, and proven methods for managing test data to prevent unreliable tests and minimize the burden of maintenance. By understanding common testing pitfalls, you'll learn how to avoid issues that can undermine confidence in your test results. Finally, we'll discuss how to strike the right balance between test coverage and rapid development to maximize the return on your testing investment.
Organizing Your Test Suite for Scalability
As your project expands, so too will your test suite. Maintaining a well-organized structure is paramount for long-term maintainability. Consider your test suite as a well-curated library where easy navigation is paramount. Organize tests according to feature or domain, mirroring the structure of your application. This allows developers to quickly pinpoint and modify tests related to specific parts of the codebase. Furthermore, use descriptive directory and file names that clearly convey the purpose of each test file. This reduces cognitive load when working within the test suite.
Naming Conventions for Readability and Documentation
Descriptive test names improve readability and serve as valuable living documentation. Choose names that clearly articulate the specific behavior under test and the expected outcome. Steer clear of generic names like "test_method_1". Instead, embrace specific and descriptive names such as "user_can_login_with_valid_credentials" or "product_cannot_be_added_to_cart_if_out_of_stock". This clarifies the purpose of each test and simplifies the process of understanding test failures, ultimately making your tests easier to understand and maintain.
Managing Test Data Effectively
Test data can either be a powerful tool or a significant source of headaches. Poorly managed test data leads to inconsistent and flaky tests, eroding confidence in your entire test suite. Employ factories (FactoryBot) to generate realistic and reusable test data, reducing the need for repetitive setup code. This centralizes data creation logic and simplifies updating test data. Additionally, consider implementing database cleaning strategies between tests to ensure isolation and prevent unintended side effects. This guarantees tests begin with a clean slate and don't interfere with each other.
Avoiding Common Testing Pitfalls
Certain testing practices can be counterproductive, hindering more than they help. One common anti-pattern is testing implementation details rather than behavior. Overly coupled tests become brittle, breaking with even minor code modifications. Concentrate on testing what the code does, not how it does it. This enhances the resilience of your tests to refactoring and implementation changes. Another pitfall is overlooking edge cases and boundary conditions. Thorough testing encompasses scenarios such as empty inputs, invalid data, and unexpected user actions.
Ruby, the language RSpec is built on, has seen its popularity fluctuate. While it remains a popular choice for web development, particularly when compared to Java, factors like developer preference and availability can influence its adoption. Learn more about discussions around Ruby and Rails: Explore this topic further.
Balancing Coverage and Development Speed
While comprehensive test coverage is a worthy goal, it shouldn't impede development velocity. Aim for a balance that provides sufficient confidence without hindering progress. Prioritize testing critical paths and user-facing functionalities first. Less critical areas can have less extensive coverage. Regularly review and refactor your tests, eliminating redundancy and ensuring they remain relevant as the codebase evolves. This pragmatic approach ensures you derive maximum value from your testing efforts.
These proven practices will enable you to build RSpec Ruby test suites that not only verify the correctness of your code but also facilitate sustainable development for the long term. A well-maintained test suite becomes an invaluable asset, boosting confidence and empowering your team to move faster. For enhanced efficiency in managing and running your test suite, consider leveraging Mergify. Mergify can automate your merge queue and CI processes, significantly reducing test execution time, especially in large projects. Learn more: Optimize your CI/CD with Mergify.