Essential rspec in ruby: Boost Your Testing Skills
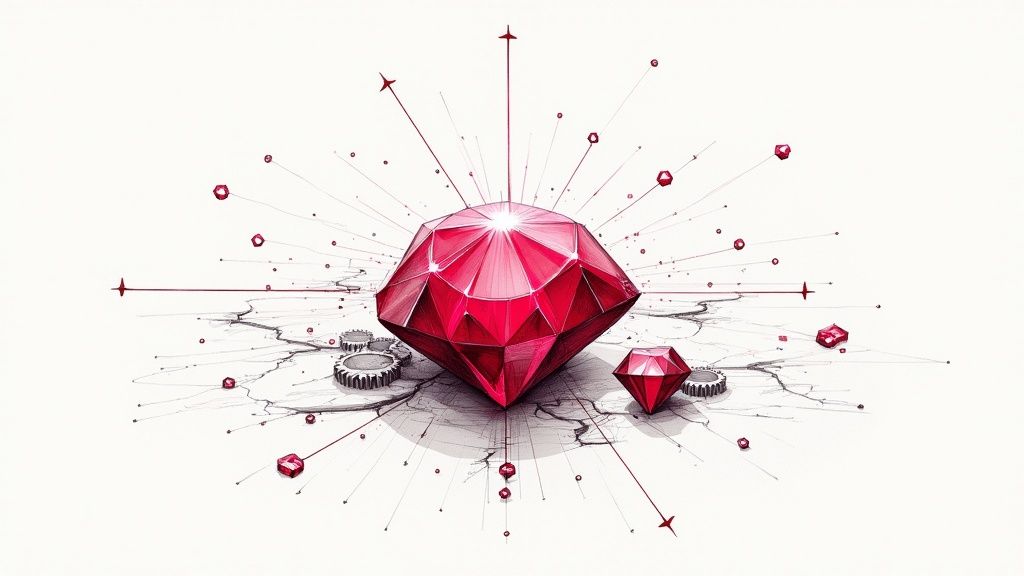
The Power Behind RSpec in Ruby
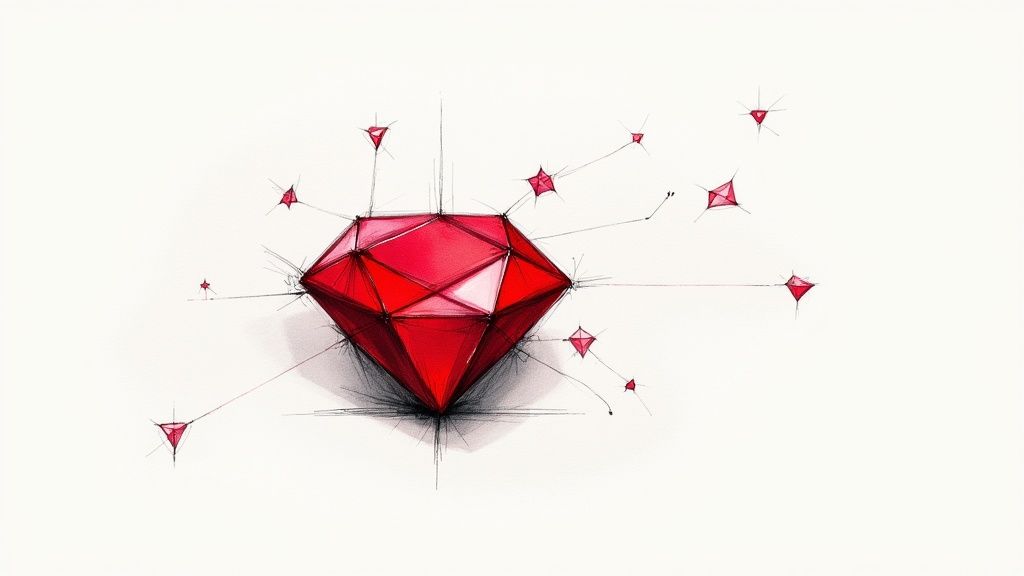
RSpec has become a core component of Ruby development. It has transformed testing from a chore into a valuable practice. Its elegant design and tight integration with Ruby make it feel like a natural extension of the language itself. This seamless fit is a key reason why developers, from startups to large corporations, have adopted RSpec.
The Behavior-Driven Development Approach
One of RSpec's major strengths is its support for behavior-driven development (BDD). BDD focuses on describing how code should behave in a way that's easy to understand.
This emphasis on behavior improves communication between developers, testers, and even non-technical stakeholders. Tests become a shared understanding of the system's functionality. This shared understanding fosters collaboration and minimizes misinterpretations.
RSpec's prominence isn't new. Since its 2006 release by Steven Baker, David Chelimsky, and Pat Maddox, it has been a cornerstone of the Ruby testing community. It has evolved into one of the most popular testing tools in the Ruby ecosystem, thanks largely to its BDD capabilities. These capabilities empower developers to write more comprehensible and maintainable tests. Learn more about RSpec here to discover more about its history and impact.
The RSpec Domain-Specific Language (DSL)
RSpec's domain-specific language (DSL) makes this natural language approach possible. The DSL uses keywords like describe
, context
, and it
to structure tests in a way that reflects how developers discuss code behavior.
For example, a test might begin with describe User
to identify what's being tested. Then, context "with a valid email"
sets up a specific scenario. Inside this context, individual expectations are expressed with it "should save successfully"
. This structure creates tests that read like a story, clearly explaining the intent and expected behavior of the code.
Benefits of Using RSpec
Using RSpec offers advantages beyond better testing. By clearly defining the desired behavior, RSpec can influence code design. Developers start thinking about functionality in terms of how it should work, which leads to more modular and testable code.
Furthermore, the clear, descriptive nature of RSpec tests makes them excellent documentation. They serve as a living document of the system's behavior. This helps new team members quickly understand the functionality and purpose of different parts of the system. This means RSpec is more than a testing tool; it's a valuable tool for better communication, design, and documentation within a Ruby project.
From Zero to Testing Hero With RSpec in Ruby
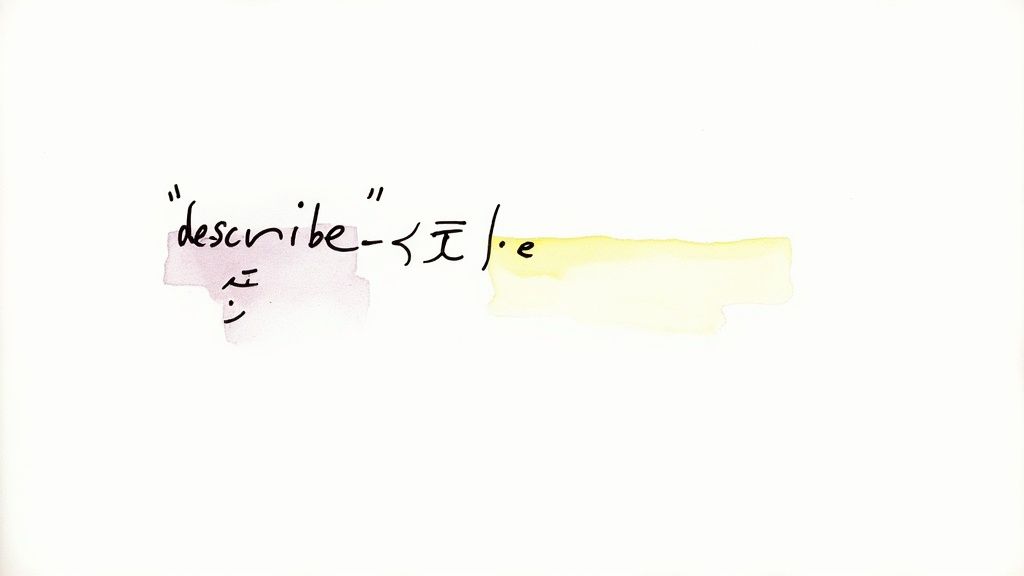
So, you're ready to add the power of RSpec to your Ruby projects? Great! This section will guide you through setting up your RSpec environment and offer insights into how experienced Ruby teams structure their tests.
Setting Up Your RSpec Environment
Getting started with RSpec is pretty straightforward. First, add the rspec
gem to your project's Gemfile
and run bundle install
. This installs the necessary library for writing and running your tests.
Next, create a directory named spec
at the root of your project. This is the standard location for RSpec tests. Within this spec
directory, you'll create individual Ruby files for your tests, often mirroring the structure of your application's code. This organization helps keep your tests manageable as your project grows.
Structuring Your Tests for Success
Experienced Ruby teams often organize their RSpec tests with describe
and context
blocks. describe
blocks group tests related to a specific class or module. context
blocks, on the other hand, define different scenarios or conditions within those groups.
For instance, you might have a describe User
block containing contexts like context "with a valid email"
and context "with an invalid email"
. This allows you to logically separate different test cases for the same class.
Within these blocks, individual tests are defined using it
blocks. Each it
block describes a specific behavior or expectation you're testing. This structured approach not only verifies your code's functionality but also acts as living documentation for your project.
Let's take a look at a comparison of different RSpec setup approaches. This table summarizes the pros and cons of each method to help you choose the best fit for your needs.
RSpec Setup Approaches Compared Find the perfect RSpec setup method for your project's specific needs
Setup Method | Difficulty | Best For | Limitations |
---|---|---|---|
before(:each) |
Easy | Setting up instance variables for each test | Can lead to redundant setup if not carefully managed |
let |
Easy | Defining memoized helper methods for test data | Can be confusing for complex setups |
subject |
Medium | Implicitly defining the object under test | Less flexible than explicit setup |
Shared Examples | Advanced | Reusing test code across multiple contexts | Requires careful design to avoid coupling tests too tightly |
As you can see, each approach has its strengths and weaknesses. Choosing the right one depends on the specific needs of your project and the complexity of your tests. Consider the trade-offs between simplicity and flexibility when making your decision.
Avoiding Common RSpec Pitfalls
One common mistake is overusing mocks and stubs. While these tools are useful for isolating units of code, over-reliance on them can create brittle tests that don't accurately reflect real-world interactions. Focus on testing actual interactions whenever possible.
Another pitfall is neglecting to organize tests effectively. As your project grows, a disorganized test suite becomes difficult to maintain. Adhering to established conventions and structuring your tests thoughtfully prevents this problem.
Example RSpec Test
Here's a simple example of an RSpec test:
describe User do context "with a valid email" do let(:user) { User.new(email: "test@example.com") }
it "is valid" do
expect(user).to be_valid
end
end end
This example demonstrates the core components of an RSpec test. It shows how to use describe
, context
, and it
blocks to define a clear and understandable test case.
By following these principles and learning from experienced Ruby teams, you can write effective tests that improve the quality of your software.
Speaking the Language of RSpec in Ruby
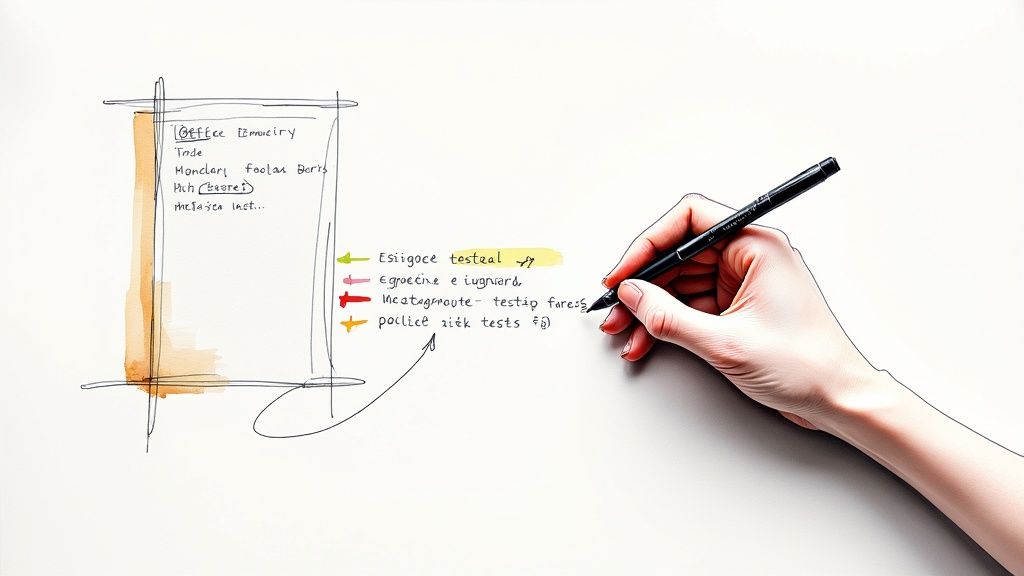
RSpec's effectiveness hinges on its intuitive syntax. It employs a domain-specific language (DSL), allowing tests to resemble natural language. This DSL comprises several core components that bring structure and clarity to your test suite.
Core Components of the RSpec DSL
The foundation of RSpec's DSL rests on describe
, context
, and it
blocks. These blocks create a structured narrative for the code under test. The describe
block defines the subject, usually a class or module. For example, describe User
signifies that the enclosed tests relate to the User
class.
Context
blocks, nested within describe
blocks, add further detail by specifying scenarios or conditions. For example, context "with a valid email"
refines the User
tests to instances with valid email addresses. This nesting organizes the tests logically, making them easier to follow.
Finally, it
blocks, nested within describe
or context
blocks, outline the expectations for each scenario. For instance, within the "with a valid email" context, you might find it "should save successfully"
. This structure embodies behavior-driven development. RSpec emphasizes describing expected code behavior. This makes tests easier to grasp, even for non-technical individuals. Learn more about this approach.
Matchers and Hooks: Enhancing Test Expressiveness
RSpec offers an array of matchers. These are methods that compare a code execution's actual result with its expected result. Matchers such as eq
, be_valid
, and include
enhance the expressiveness of tests. expect(user.email).to eq("test@example.com")
clearly illustrates the expectation for a user's email address.
RSpec also incorporates hooks – code blocks executed before, after, or around individual tests or groups of tests. Hooks are especially useful for setting up common test conditions or cleaning up afterward. The before(:each)
hook, for example, might create test data before each test, ensuring consistent starting conditions.
Living Documentation: Tests as a Communication Tool
Using RSpec's DSL, matchers, and hooks, Ruby teams can create test suites that become living documentation. This not only verifies functionality but also communicates the code's purpose.
The clarity of RSpec tests helps new team members quickly grasp the codebase. Furthermore, RSpec tests effectively illustrate edge cases and complex behaviors. Each context
block can set up unique scenarios, and it
blocks explicitly define expected outcomes. This transparency simplifies understanding of the system's behavior. When tests fail, RSpec’s descriptive nature helps pinpoint the problem’s source.
Writing Effective Tests With RSpec
RSpec encourages modularity and logical test groupings, fostering organization. This structure simplifies understanding, debugging, and maintenance. Combined with features like metadata, teams can create highly specialized testing strategies. Resources like the Red Canary blog post on using RSpec metadata offer helpful insights. This ultimately contributes to robust and maintainable Ruby applications.
Advanced RSpec Techniques That Actually Deliver
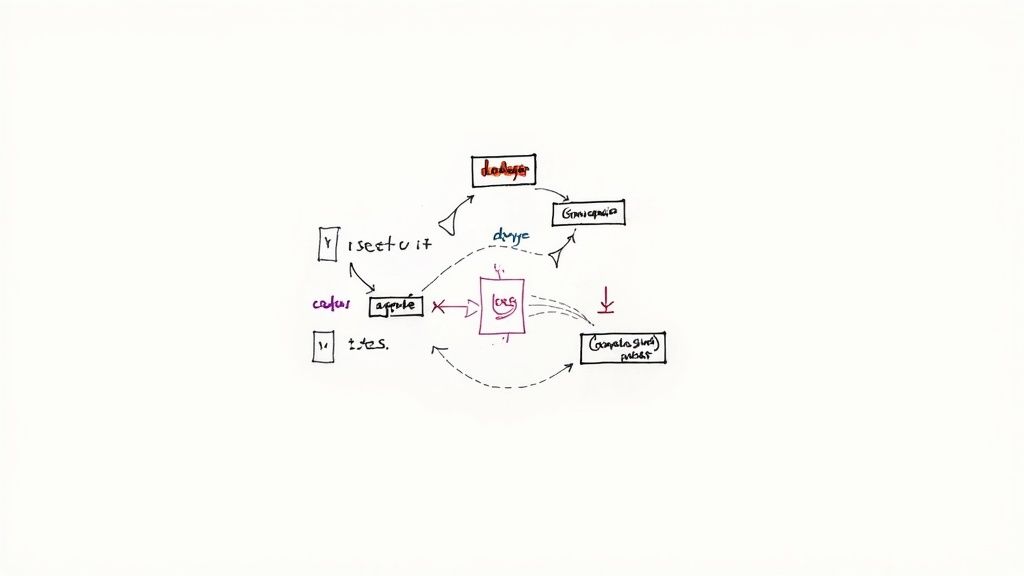
Building upon the basics of RSpec in Ruby, let's delve into advanced techniques to enhance your testing process. These methods can streamline your workflow and improve code quality, leading to a more robust and maintainable application.
Test Doubles: Mocks, Stubs, and Spies
Test doubles are crucial for isolating components and accelerating test execution. They simulate the behavior of dependencies without requiring their presence, particularly useful when dealing with external services or databases.
- Mocks: Mocks define expectations for method calls and verify these expectations are met, ensuring specific interactions during testing. For instance, mocking an email service confirms the
send_email
method is called with the correct arguments upon user signup. - Stubs: Stubs return pre-defined responses for method calls, controlling the data returned by dependencies. This simulates various scenarios, such as stubbing a database query to return specific users for a test.
- Spies: Spies combine recording interactions with a double while allowing the original method call. This checks both method calls and their effects, like confirming a caching mechanism is used before retrieving data from a database.
Shared Examples: DRY Up Your Tests
Shared examples eliminate duplication in your tests. Encapsulate similar logic across contexts within a shared example, then include it where needed. This promotes consistency and reduces errors from copy-pasting.
Let's explore how this works with a practical example:
shared_examples "a model with a name" do it { is_expected.to validate_presence_of(:name) } end
Then, incorporate it into your model specs:
RSpec.describe User, type: :model do it_behaves_like "a model with a name" end
RSpec.describe Product, type: :model do it_behaves_like "a model with a name" end
Custom Matchers: Increase Readability
Custom matchers enhance readability, particularly with complex conditions. They express expectations in a domain-specific language. For example, to verify a user's role:
RSpec::Matchers.define :have_role do |expected_role| match do |user| user.role == expected_role end end
This simplifies tests to expect(user).to have_role("admin")
, clarifying the test's intent.
The following table summarizes the different RSpec testing approaches discussed:
RSpec Testing Approaches That Work
Choose the right testing approach for different Ruby development scenarios
Technique | When to Use | Benefits | Potential Issues |
---|---|---|---|
Mocks | Verifying interactions with dependencies | Ensures specific methods are called with the correct arguments | Over-specification can make tests brittle |
Stubs | Controlling the return values of dependencies | Simplifies testing by isolating components | Can mask issues in the real implementation |
Spies | Observing interactions while allowing the original method to execute | Provides insights into method calls and their effects | Can be more complex to set up than mocks or stubs |
Shared Examples | Testing similar logic across multiple contexts | Reduces code duplication and improves consistency | Can be challenging to manage when shared examples become too complex |
Custom Matchers | Expressing complex expectations in a readable way | Improves test clarity and maintainability | Requires defining custom matcher logic |
These advanced techniques, coupled with core RSpec features, enhance your testing strategy. They create cleaner, more expressive tests, improving maintainability and reducing development time.
Rails + RSpec in Ruby: A Perfect Testing Partnership
When Ruby on Rails and RSpec meet, testing reaches a new level of effectiveness. This section explores how successful Rails teams use these tools to test everything from complex models to API endpoints.
Integrating RSpec With the Rails Ecosystem
RSpec integrates seamlessly with the Rails ecosystem, using tools like factories and fixtures. Factories dynamically create test data, offering more flexibility than fixtures. For example, when testing user authentication, factories can generate users with varying roles and permissions for broader test coverage.
Specialized gems further enhance this integration, simplifying the testing process. Gems like factory_bot_rails
streamline the creation and management of factories. This synergy lets developers concentrate on writing meaningful tests instead of struggling with test data setup.
Testing Key Rails Components
Testing Active Record relationships is more straightforward with RSpec's clear syntax. You can define the expected association behavior using matchers like belong_to
and have_many
, ensuring correct model relationships.
Controller actions are thoroughly testable with RSpec's request specs. These specs simulate real HTTP requests, checking the controller's response and any model layer changes, ensuring user interactions are handled correctly.
Even testing view components, often challenging, becomes easier with RSpec's helper methods. You can test the rendered HTML, verifying data display for user interface consistency.
Optimizing Test Organization and Performance
Well-organized tests are crucial, especially in larger Rails applications. Structuring tests by application architecture and using descriptive names helps developers quickly locate and understand each test. This reduces test execution time by focusing tests on specific areas.
Quickly identifying failures is vital for efficiency. RSpec's clear error messages and stack traces pinpoint the cause of failing tests without extensive debugging. This rapid feedback loop allows for faster iterations and quicker bug fixes. RSpec's adoption also contributed to Ruby's overall popularity. As a language emphasizing rapid prototyping, Ruby's tools like RSpec enhance developer productivity. Find more statistics here: Discover more insights about Ruby's popularity.
Maintaining RSpec Test Suites
Maintaining RSpec test suites during Rails upgrades and feature evolution requires a strategic approach. Using shared examples and custom matchers minimizes code duplication and maintains test suite consistency. This simplifies updates when the Rails framework changes and promotes maintainability. These practices allow Rails teams to adapt their RSpec suites effectively, ensuring consistent and reliable testing throughout the application's lifespan. Insights from experienced RSpec teams guarantee robust testing across a Rails application’s entire lifecycle.
Performance Testing With RSpec in Ruby That Matters
Performance testing often gets overlooked. It's just as vital as checking that your code functions correctly. Don't just ask if it works, but how well it works. Smart Ruby teams use RSpec to ensure their applications not only function as designed but also perform optimally under pressure.
Introducing rspec-benchmark
The rspec-benchmark
gem extends RSpec, enabling developers to write tests that measure execution time and overall code performance. This gem ensures code optimizations haven't accidentally degraded performance and quickly highlights bottlenecks. It provides a robust method for integrating performance expectations directly into your testing.
This proactive approach to performance testing can prevent those late-night calls about slow servers. Catch regressions before they affect users by including performance metrics in your tests. This results in a more stable and positive experience for everyone.
rspec-benchmark
also lets you set execution limits. For instance, you can specify that a method should perform_under(1).ms
. This creates a clear performance expectation and flags any regressions exceeding that limit. For a more detailed look at rspec-benchmark
, explore further readings on performance assertions with RSpec. Discover more insights about performance testing.
Writing Meaningful Performance Tests
Effective performance tests require more than just measuring execution time. Your tests must be resilient against database or infrastructure changes. Focus on testing the relative performance of your code rather than absolute timings.
For example, when optimizing a database query, measure the percentage improvement rather than the raw query time. This reduces the test's sensitivity to environmental variations in your testing setup. This method gives more accurate insights into the true impact of your code changes.
Integrating Performance Expectations Into Your Workflow
The secret to consistently good performance is integration. Make performance testing a regular part of your development process, not something you tack on at the end. Include performance tests right alongside your functional tests.
Run these performance tests as part of your Continuous Integration (CI) process. This lets you track performance trends and catch regressions early. This proactive strategy ensures your Ruby applications remain responsive and efficient. Integrating performance testing into your daily routine keeps your applications fast, even as they grow more complex.
Real-World Examples and Case Studies
Imagine an e-commerce platform with slow product searches during peak traffic. By implementing performance tests with RSpec, the team can identify the bottlenecks in their search algorithm. They can then establish a performance baseline and track improvements as they optimize their code. This ensures searches remain fast and responsive, even under high load.
Another example is a social media application aiming to reduce the time it takes to display user feeds. Performance tests can pinpoint areas for optimization, resulting in a smoother, faster user experience. Prioritizing performance improves user engagement and reduces user churn.
Ready to elevate your team’s development process and significantly reduce integration friction? Explore Mergify today! Enhance your CI/CD pipeline with Mergify.