Master RSpec: Expert Testing for Ruby Projects
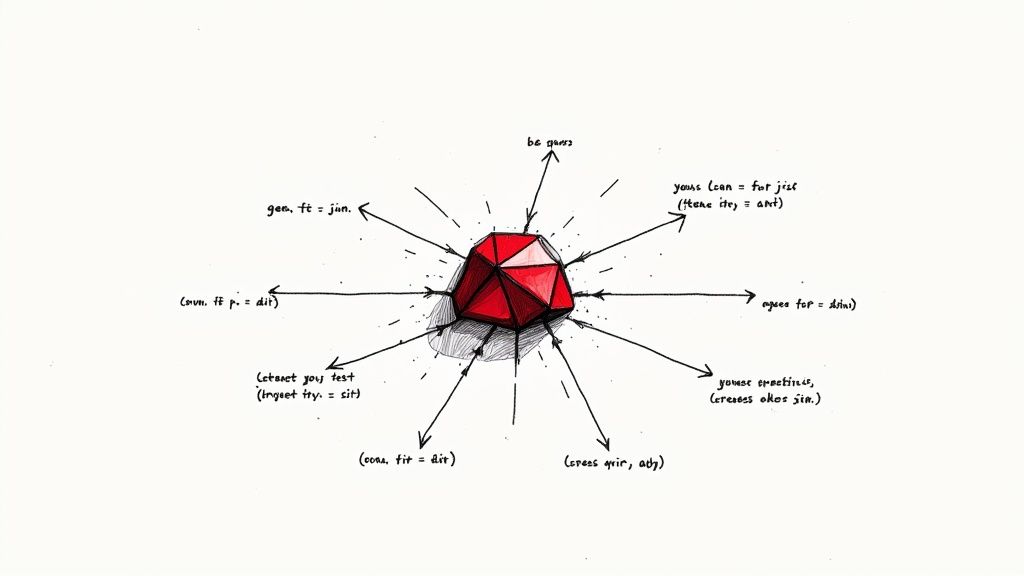
Why RSpec Transforms Ruby Development
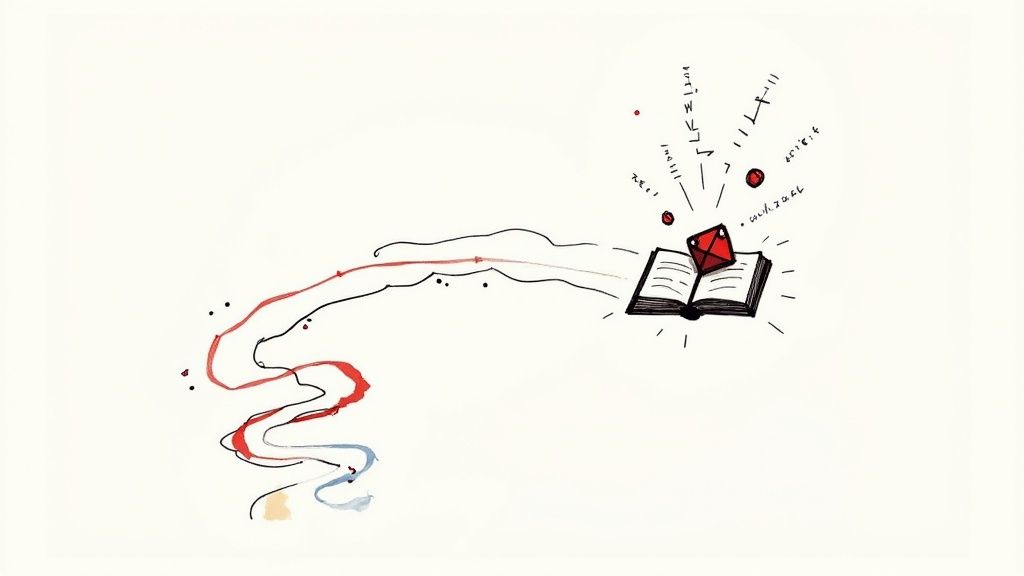
RSpec has become an indispensable tool for Ruby developers. Its unique approach to testing sets it apart. Instead of simply verifying outputs, RSpec encourages developers to consider the behavior of their code. This behavior-driven development (BDD) approach results in more descriptive and understandable tests, fundamentally changing how quality assurance is approached.
Imagine testing a user authentication system. A traditional method might involve checking if the login method returns the correct user object. RSpec, however, lets you describe the intended behavior: "a user with valid credentials should be able to log in." This seemingly small difference produces tests that closely resemble real user stories and business needs.
RSpec, initially introduced in 2005, has become a cornerstone of BDD and Test-Driven Development (TDD) in Ruby. It has continually evolved through various versions, with the latest being version 3.12. This ongoing development demonstrates its wide adoption and dedication to supporting effective test scenarios. For a deeper look into RSpec's history, check out this resource: Learn more about RSpec's history.
Readability and Maintainability in RSpec
Another key benefit of RSpec is its expressive syntax. RSpec tests resemble plain English, making them accessible to developers of all skill levels. This improved readability fosters better team collaboration and simplifies onboarding for new team members.
Maintaining and updating test suites also becomes significantly less challenging. When tests are easy to grasp, identifying and fixing issues is more straightforward. Refactoring code becomes less risky, and the test suite continues to deliver reliable feedback. RSpec's structure, using describe
, context
, and it
blocks, also promotes better test organization.
RSpec and the Ruby Philosophy
RSpec aligns perfectly with Ruby's focus on developer satisfaction. By making tests more readable and maintainable, RSpec reduces the friction often associated with testing. This can result in shorter development cycles and fewer bugs in production.
This also contributes to increased developer productivity. When testing is a positive experience, developers are more inclined to create comprehensive tests, which ultimately enhances the overall quality of the software. Developing strong RSpec skills is a valuable investment in a more efficient and enjoyable development process. Mastering RSpec is therefore a crucial skill for any serious Ruby developer.
Setting Up RSpec For Success From Day One
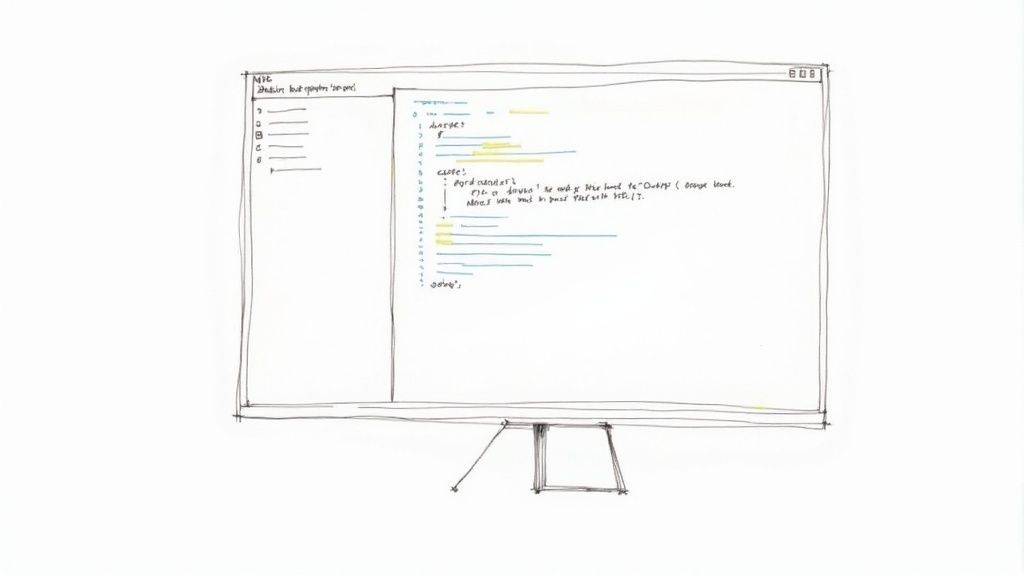
Setting up RSpec effectively is key for efficient testing. This guide will walk you through a straightforward installation process, so you can start testing quickly. We'll explore how to configure RSpec for various project types, from simple Ruby gems to more complex Ruby on Rails applications. This foundational knowledge is essential for building a robust and scalable testing environment.
Installing and Configuring RSpec
First, add the rspec
gem to your project's Gemfile
. Then, run bundle install
to install the necessary dependencies.
After installation, initialize RSpec by running the command rspec --init
. This creates the spec
directory and the crucial spec_helper.rb
file. This file houses the default configurations for your tests and serves as the starting point for customizing your RSpec setup to fit your project's specific needs.
RSpec in Rails
RSpec is a popular choice for testing in Ruby on Rails applications due to its clear syntax and comprehensive features. Several gems contribute to this robust testing framework.
rspec-core
: The core RSpec gem providing the testing structure.rspec-rails
: Provides Rails-specific integrations for RSpec.rspec-mocks
: Facilitates isolating dependencies through techniques like stubbing and mocking, leading to more reliable tests.rspec-expectations
: Offers a user-friendly API for defining expected outcomes.
The combination of these gems makes RSpec a preferred testing framework in the Ruby community. For a deeper dive into using RSpec with Rails, explore this guide on using the RSpec testing framework.
Organizing Your Specs
As your project expands, maintaining a well-organized spec
directory becomes essential. A good practice is to group your spec files by type, such as models, controllers, or services. Alternatively, you can group them by feature.
For instance, put all model specs under spec/models
and controller specs under spec/controllers
. This structure simplifies locating and managing tests as your test suite grows. Using descriptive file names that clearly reflect the tested components further enhances organization.
To provide a clearer view of how these gems contribute to your testing setup, take a look at the table below:
Essential RSpec Gems Comparison
A comparison of the main RSpec gems and what functionality each provides to your testing setup
Gem Name | Purpose | When To Use | Key Features |
---|---|---|---|
rspec-core |
Provides the core RSpec testing framework | Always – this is the foundation of RSpec. | Defines test structure, runs tests, and reports results. |
rspec-rails |
Integrates RSpec with Rails projects | When using RSpec in a Rails application. | Provides Rails-specific helpers and generators. |
rspec-mocks |
Enables mocking and stubbing of dependencies | When isolating units of code for testing or dealing with external dependencies. | Helps create test doubles to control behavior and improve reliability. |
rspec-expectations |
Defines a clear API for expectations | When specifying expected outcomes of tests. | Provides matchers for asserting values and behavior. |
This table outlines the core RSpec gems and their roles, allowing you to tailor your setup to your project's needs. Remember that rspec-core
is fundamental, while others provide specific enhancements depending on your project's complexity and requirements.
Customizing Your Configuration
The spec_helper.rb
file allows for fine-grained control over RSpec's behavior. You can modify default settings, add custom matchers, and incorporate extensions.
This might involve configuring the output format, disabling certain features, or adjusting the mocking framework. This level of control allows you to optimize your testing environment for maximum efficiency and clarity.
Avoiding Common Pitfalls
A frequent mistake is neglecting to configure RSpec correctly for the specific project type. For Rails applications, the rspec-rails
gem is essential for seamless integration and provides helpful generators for creating specs.
Another common issue is poorly organized specs, which become difficult to navigate and maintain as the project grows. By following these tips, you can create a robust and efficient testing environment with RSpec that enhances your workflow and ultimately improves your code quality.
Crafting Your First RSpec Tests That Actually Matter
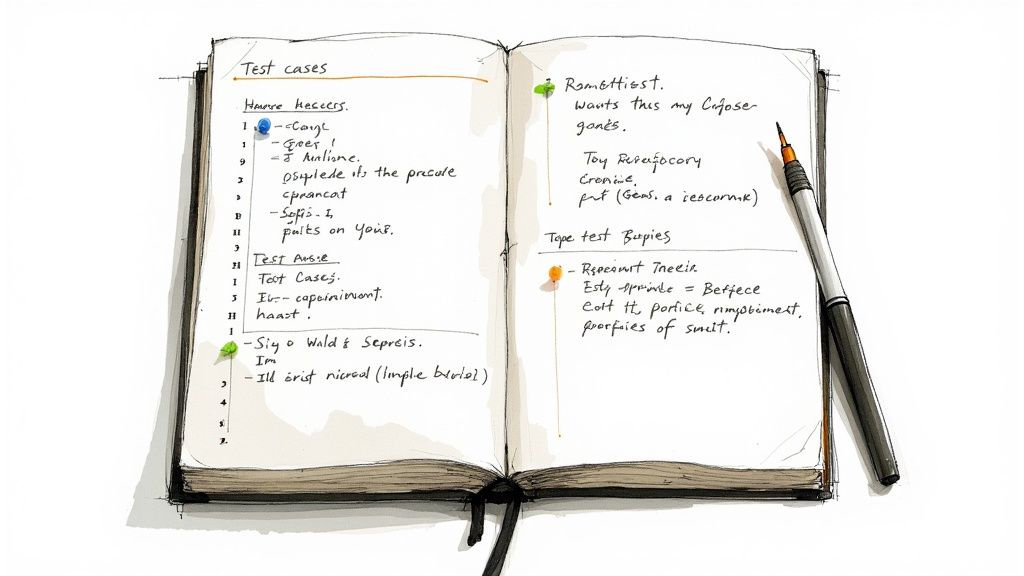
Creating effective tests with RSpec is more than just putting together basic examples. It involves understanding the core components and how they contribute to valuable, readable tests. This exploration dives into the fundamentals of RSpec – describe
, context
, and it
– and how to structure tests that serve as clear documentation. We'll also touch on expectation syntax and best practices for test organization.
The Building Blocks of RSpec: Describe, Context, and It
RSpec tests are structured around three key elements: describe
, context
, and it
.
describe
: This defines the class or module you're testing. It groups related tests and provides context. Think of it as answering the question, "What are we testing?"context
:context
blocks establish specific scenarios within adescribe
block. This helps clarify the conditions under which the code should behave in a particular way. It answers the question, "Under what circumstances?"it
: Theit
block contains the actual test case. Eachit
block should focus on a single, specific behavior or expectation, explaining "how" the code should function in the given context.
For example, consider a User
class with a valid?
method:
describe User do context "with a valid email" do it "is valid" do user = User.new(email: "test@example.com") expect(user).to be_valid end end
context "without an email" do it "is not valid" do user = User.new(email: nil) expect(user).not_to be_valid end end end
This structure makes it clear which part of the User
class is being tested and under what conditions.
Mastering Expectation Syntax
RSpec's expectation syntax clearly defines the expected outcome of a test. The expect
method, combined with matchers like to
and not_to
, forms the core of this syntax.
Matchers offer flexibility in defining expected behavior, checking values, truthiness, presence, and more. Precise matchers make tests more expressive. For instance, expect(user.name).to eq("John Doe")
explicitly states the expected user name.
Organizing Your Tests for Long-Term Success
As projects grow, a well-organized test suite is crucial. Using describe
and context
provides structure, but consistent file organization is equally important.
A best practice is to mirror your application's structure within your spec
folder. Model specs reside in spec/models
, controller specs in spec/controllers
, and so on. This improves navigability in larger projects.
Using Hooks for Efficiency
RSpec provides hooks – before
and after
– to execute code before or after specific tests. This is helpful for setting up common dependencies or cleaning up. For example, a before
hook can establish a database connection if multiple tests require it, reducing code duplication.
By using describe
, context
, it
, expectation syntax, and hooks effectively, your RSpec tests become more than just bug catchers. They evolve into living documentation, supporting a more efficient and reliable development process.
Advanced RSpec Patterns The Pros Actually Use
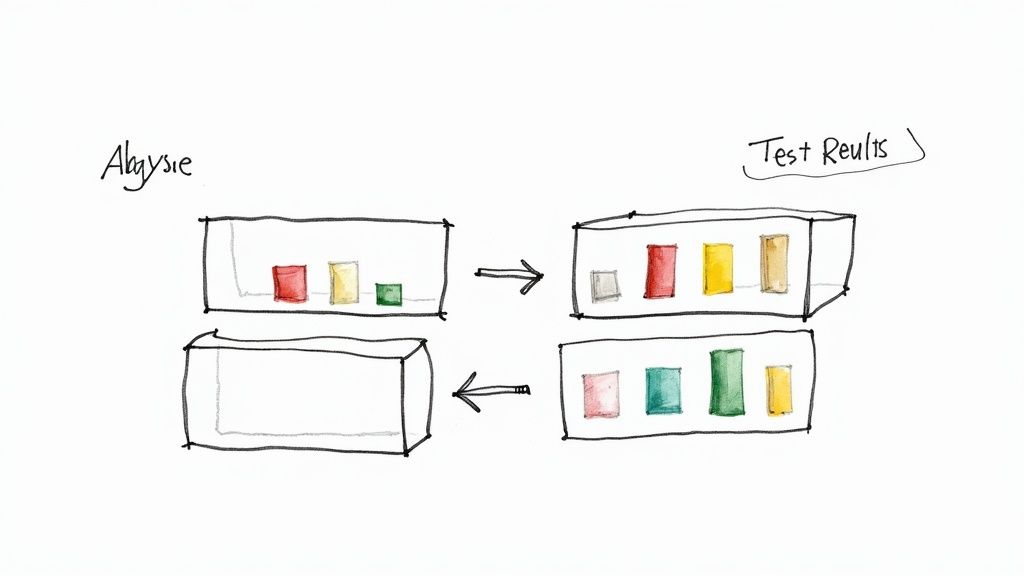
Building upon the foundational concepts of RSpec, this article explores advanced techniques that set experienced testers apart. These patterns enhance clarity and maintainability, leading to more robust and efficient testing. We'll delve into shared examples, custom matchers, and strategies for handling complex scenarios.
Leveraging Shared Examples and Contexts for Reusability
RSpec's shared examples and shared contexts promote code reuse across multiple components. This is especially helpful when testing similar behaviors in different classes or modules. For instance, if several models share validation rules, you can define these rules once and reuse them across your tests.
This not only reduces redundancy but also ensures consistency. Updating a shared example propagates the change everywhere it's used, simplifying maintenance. Shared contexts offer similar benefits, providing reusable setup for your tests.
Custom Matchers: Creating a Domain-Specific Language
Custom matchers extend RSpec's expect
syntax, enabling expectations specific to your application. This creates a domain-specific language (DSL) that improves test readability. Consider email validation: instead of a complex regular expression, a custom matcher like expect(email).to be_a_valid_email
is far more expressive.
This simplifies tests and encapsulates complex logic within the matcher itself. The improved readability also facilitates onboarding and collaboration.
Handling Edge Cases and Error Conditions
Robust tests account for edge cases and potential errors. RSpec provides tools to simulate exceptions and verify error handling. Using expect { code }.to raise_error(SpecificError)
ensures your code raises the correct exception under specific conditions.
This proactive approach catches bugs before they reach users, contributing significantly to application reliability and stability.
Testing Complex Object Interactions
Testing complex object interactions requires careful planning. Break down complex scenarios into smaller, testable units. Focus on isolating dependencies and testing individual interactions.
This simplifies tests and makes debugging easier. By isolating each unit, you can pinpoint issues more efficiently, especially when working with services or classes with multiple dependencies. This approach also strengthens isolated behavioral testing.
By incorporating these advanced RSpec patterns, you can cultivate a more efficient and effective testing process. This empowers you to write tests that not only identify bugs but also enhance the design and maintainability of your Ruby code. These practices are essential for managing complex test scenarios in growing projects.
RSpec Test Doubles: Beyond Basic Mocks and Stubs
Test doubles are essential for effective isolation testing in RSpec. They let you simulate the behavior of dependencies, ensuring your tests focus solely on the unit of code you're examining. This post explores how to use test doubles effectively, going beyond simple mocks and stubs to create robust and maintainable tests. We'll look at various types of doubles, their appropriate uses, and strategies for handling complex testing scenarios.
Understanding the Different Types of Test Doubles
RSpec provides a variety of test doubles:
- Mocks: Mocks verify interactions. They ensure specific methods are called on a dependency with the expected arguments. Use mocks when the interaction with the dependency is a critical part of your code's correct behavior.
- Stubs: Stubs replace a method's actual behavior with a predefined return value. They simplify tests by avoiding complex setup or interactions with external systems. Use stubs to focus on the "happy path" when the dependency's internal workings aren't the primary focus.
- Spies: Spies combine mocks and stubs. They let you stub a method's return value and verify it was called. This is useful when you need to control a dependency's behavior while also ensuring it's being used correctly.
- Object Doubles/Dummy Objects: Dummy objects are stand-ins that satisfy type checking and structural requirements without any real behavior. They're useful when a dependency is required, but its behavior isn't relevant to the test.
Choosing the Right Test Double
Picking the right test double depends on your testing scenario:
- Use stubs to simplify tests and focus on core logic.
- Use mocks when the interaction itself is the key part of the test.
- Use spies when you need both stubbing and interaction verification.
- Use dummy objects when only the dependency's presence matters, not its actions.
For example, if your method sends an email, you might stub the email service to avoid sending actual emails during tests. However, if you're testing the logic deciding when to send an email, mocking would be better to verify the email service is called under the right conditions.
Isolating External Dependencies
Test doubles are invaluable for isolating external dependencies like APIs and databases. By stubbing or mocking these dependencies, your tests become faster, more reliable, and independent of outside factors. This prevents unwanted side effects, like sending real emails or changing production data.
Additionally, RSpec integrates with other tools for enhanced testing analysis. For instance, the rspec_tesults_formatter
gem lets you send results to Tesults, a platform providing detailed reporting and metrics.
Managing State and Complex Interactions
For complex interactions or stateful dependencies, consider using spies or partial doubles. These let you control specific parts of a dependency's behavior while allowing other parts to function normally. This approach balances isolation with realistic testing.
For instance, if a dependency has internal state that affects its behavior, partially stubbing some methods while leaving others intact can simulate realistic interactions. This avoids over-mocking, which can lead to brittle tests tied too closely to implementation details.
Building Maintainable Test Doubles
As your codebase grows, your test doubles must remain relevant. Avoid over-specifying mocks or stubs, which can create brittle tests that break with code changes. Focus on the crucial interactions and behaviors.
Use descriptive names for test doubles and document their purpose clearly. This improves readability and makes it easier to understand your tests. These practices make your tests more robust, maintainable, and valuable over time.
RSpec for Rails: Testing That Actually Catches Bugs
Rails applications often present unique testing challenges. This article explores how to use RSpec-Rails effectively to test every part of your application, from models and controllers to API endpoints. We’ll also discuss how experienced development teams find a balance between unit and integration tests for optimal coverage without sacrificing speed.
Balancing Unit and Integration Tests in Rails
Successful Rails teams understand the value of balancing unit tests and integration tests. Unit tests focus on small, isolated pieces of code, while integration tests verify how different components interact. Unit tests allow you to quickly pinpoint and fix problems in individual methods or classes. Integration tests ensure that the entire system functions as expected, catching errors that unit tests might miss.
For example, unit tests might confirm that a model validates data correctly. An integration test would then ensure a user can submit a form using that model without any issues. Finding the right balance between these two types of tests maximizes coverage and keeps the development cycle efficient.
Testing Controllers, Models, and API Endpoints
RSpec-Rails provides specialized tools for testing various Rails components. For controllers, you can simulate user requests and check the responses. For models, RSpec helps test validations, associations, and callbacks. When testing API endpoints, you can test various request methods (GET, POST, PUT, DELETE) and their respective responses.
This targeted testing approach catches real-world bugs before they impact users. By focusing on the specific characteristics of each Rails component, you create highly effective tests that offer valuable feedback. This ensures your application behaves correctly in diverse scenarios.
Handling Rails-Specific Concerns
RSpec-Rails helps address challenges specific to the Rails framework, such as testing associations, validations, and callbacks. You can test whether model relationships are correctly set up and whether validations perform as expected. You can also test model callbacks to ensure they trigger at the right times.
The key is to avoid creating brittle tests – tests that break easily with changes to the framework. Writing tests that are not overly reliant on Rails internals ensures your tests remain useful as your application grows and evolves.
System Testing User Experiences
System tests verify user interactions across multiple components, simulating real user flows. These tests ensure users can complete tasks like signing up, creating content, or making purchases. While valuable for validating user experiences, system tests can become difficult to maintain.
RSpec-Rails simplifies these tests by providing structure, making them more manageable and less prone to becoming a maintenance burden as your application scales. This helps to guarantee a smooth and functional user experience, critical for delivering a high-quality application.
Let's examine the various RSpec test types available for Rails development. The following table provides a summary of their purpose and application within your projects.
RSpec Test Types for Rails Applications
Overview of different RSpec test types and their applications in Rails projects
Test Type | Purpose | When To Use | Example Scenario |
---|---|---|---|
Model Specs | Test model logic, validations, associations, and callbacks | When ensuring data integrity and business rules are enforced correctly. | Verifying user data is validated before saving. |
Controller Specs | Test controller actions and responses | When ensuring requests are handled appropriately and views are rendered correctly. | Testing a user creation flow through a controller. |
System Specs | Test end-to-end user interactions | When verifying complete user flows and complex behavior across multiple components. | Testing the user signup process, including email verification. |
Request Specs | Test API endpoints | When ensuring API responses are correct and adhere to the expected format. | Verifying API returns correct data for a specific request. |
Helper Specs | Test helper methods | When verifying helper methods work correctly in isolation. | Testing a date formatting helper function. |
Job Specs | Test background jobs | When ensuring jobs perform their intended function correctly. | Testing the job for sending confirmation emails after signup. |
Mailer Specs | Test email functionality | When verifying that emails are sent correctly with the right content and recipients. | Testing email content and delivery for password resets. |
As you can see, RSpec-Rails offers a robust suite of tools to cover all aspects of your Rails application testing. Choosing the right test type depends on the specific functionality you are verifying.
Looking to streamline your CI process and boost your team’s workflow? Mergify offers powerful features like Merge Queues and Merge Protections to automate pull request updates and prevent conflicts. Its AI-powered CI Issues feature even helps identify infrastructure problems. Learn more about how Mergify can help your team.