Python Testing Success: The Ultimate Guide to Pytest Mastery
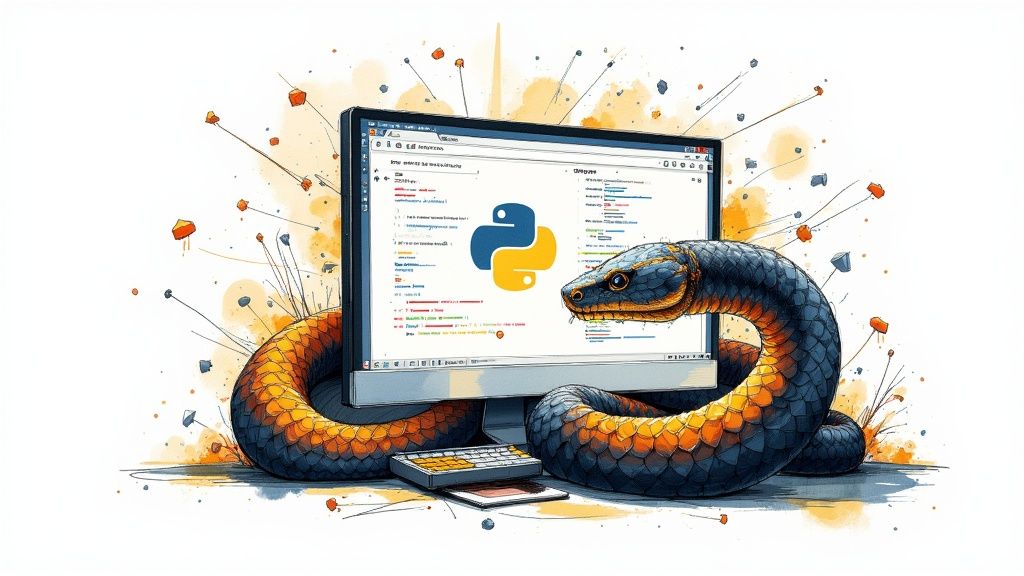
Understanding the Power of Modern Pytest
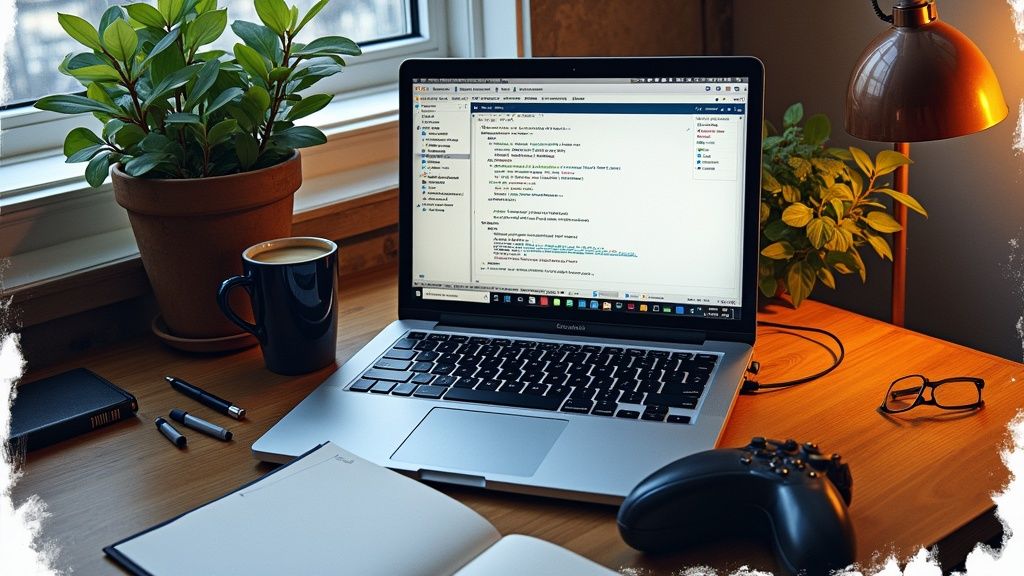
Software testing can be challenging, but Python Pytest makes it straightforward and effective. Many Python developers are choosing Pytest because it helps them write better tests more easily. Teams at growing tech companies have found that Pytest's simple approach helps them test their code more thoroughly while spending less time on test maintenance.
Simplifying Testing with Assertion-Based Approach
The beauty of Pytest lies in how it removes unnecessary complexity from testing. Unlike Python's built-in unittest
framework, which requires lots of setup code, Pytest lets developers write tests using regular functions and simple assert
statements. This means tests are easier to write and understand. For teams looking to improve their testing process, this simpler approach is a major advantage. Learn more about Pytest basics.
Pytest Features Boosting Productivity
Here's why development teams find Pytest so helpful:
- Fixtures: Make it easy to set up test data and clean up afterwards, helping developers focus on testing the actual code
- Markers: Let you group and organize tests, so you can run specific test categories when needed
- Parameterization: Run the same test with different inputs, which means better test coverage without writing more code
Real-World Success Stories
Teams that switch to Pytest often see concrete improvements in their testing. For example, one development team cut their test execution time by 30% after moving to Pytest. They found that features like parameterization helped them run tests more efficiently while maintaining thorough coverage.
Conclusion
Pytest excels at making testing more manageable - whether you're writing simple unit tests or complex test suites. Its clear error messages and debugging tools help developers find and fix issues faster. As more teams discover these benefits, Pytest continues to gain popularity, showing how the right tools can make testing both easier and more effective.
Building Your Optimal Testing Environment
Testing Python code effectively requires thoughtful setup and organization. By creating a well-structured environment and properly configuring your tools, you can make testing smoother and more maintainable over time.
Structuring Your Test Directories
Good test organization makes it easy to find and maintain tests as your project grows. Start by creating a dedicated "tests" directory at your project's root. Then, mirror your application's structure within that tests folder. For example, if you have a file at app/my_module.py
, put its tests in tests/app/test_my_module.py
. This makes it simple to locate tests for specific functionality and keeps everything neatly organized.
Configuring pytest.ini for Better Control
The pytest.ini
file gives you fine-grained control over how pytest behaves. You can set up key preferences like:
- Which files pytest should look for when finding tests
- Custom markers to label and group related tests
- Project-specific settings and configurations
This central configuration file helps maintain consistency across your testing setup.
Isolating Dependencies with Virtual Environments
Using virtual environments prevents dependency conflicts between projects. Tools like venv or conda create isolated spaces where you can install specific package versions your tests need. This ensures your tests run reliably no matter what other Python projects you have on your system.
Seamless IDE Integration
Most modern IDEs work smoothly with pytest out of the box. Popular editors like PyCharm and VS Code let you:
- Run tests directly in the editor
- See results instantly
- Debug failing tests step by step
These integrations speed up the test-debug cycle significantly.
Troubleshooting Common Setup Issues
Even with careful setup, you may run into some common challenges. If pytest isn't finding your tests, check that your test files follow the standard naming pattern (starting with "test_" or ending in "_test.py"). For dependency problems, make sure you're using a fresh virtual environment with the correct package versions installed. Catching these issues early helps maintain a smooth testing workflow.
Advanced Features and Plugin Ecosystem
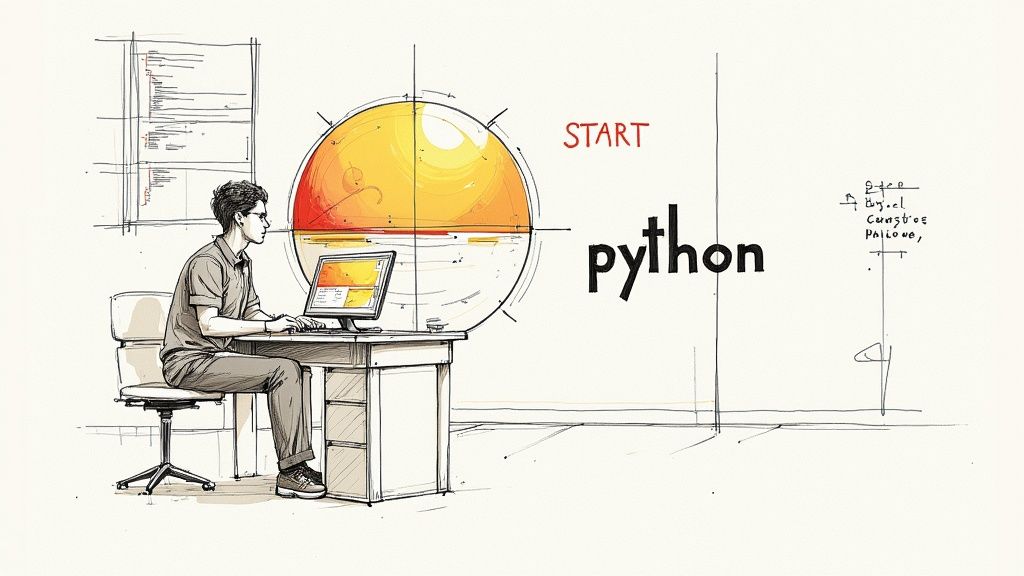
Python Pytest becomes even more powerful when you explore beyond its basic capabilities. The framework's extensive collection of plugins helps solve specific testing challenges while making testing more efficient. Take the widely-used pytest-cov plugin - with over 47 million downloads, it has become essential for measuring code coverage and getting clear insights into code quality.
Finding the Right Plugins
To make your testing more effective, consider these proven plugins that many developers rely on:
- pytest-xdist: Speeds up testing by running tests in parallel across multiple CPUs - perfect for large test suites
- pytest-html: Creates clear, visual test reports that team members and stakeholders can easily understand
- pytest-mock: Makes it simpler to write mocked tests by providing helpful wrappers around Python's mock package
Each plugin serves a specific purpose, helping you build a testing setup that fits your project's exact needs.
Success Stories from Development Teams
Real teams are seeing concrete benefits from combining Pytest with strategic plugin choices. For example, one development team solved their slow test execution by adding pytest-xdist
to run tests in parallel. This simple change led to a 20% faster CI/CD pipeline, showing how the right plugins can make a real difference.
Best Practices for Adding Plugins
Here's how to effectively bring these tools into your testing workflow:
- Begin gradually: Add and test one plugin at a time to understand its impact
- Track performance: Use plugins like pytest-system-statistics to monitor CPU and memory usage during test runs. Install it with
python -m pip install pytest-system-statistics
and use various flags for detailed metrics. Learn more about system statistics - Keep tests organized: Check your test suite regularly as plugins update to ensure everything works together smoothly
By carefully selecting and implementing plugins, you can create a more powerful and efficient testing environment. Start small, measure the results, and build up to a robust testing system that serves your team's needs.
Mastering Command-line Options for Flexible Testing
Managing test suites effectively requires a solid grasp of Python Pytest's command-line options. These options give development teams precise control over test execution and help them adapt testing strategies based on specific needs. Let's explore the key features that make Pytest such a powerful testing tool.
Essential Command-line Options
The most useful Pytest command-line options help teams run tests more efficiently and get better insights from test results:
- Test Selection (-k): Choose specific tests by matching keywords in test names
- Test Groups (-m): Run groups of related tests using markers
- Output Control: Use
-q
for quiet mode or-v
for detailed test output
These options help teams save time by running exactly the tests they need, when they need them.
Extending Pytest with Custom Options
Teams can add their own command-line options using pytest_addoption
. This feature lets you pass custom arguments to change how tests behave at runtime. For instance, you can create options that modify test parameters based on different environments or testing scenarios. Learn more about implementing custom command-line arguments to fit your testing needs.
Practical Benefits in Daily Use
Think about a development team running tests across different environments as part of their CI/CD pipeline. By setting up environment-specific options in pytest.ini
and using custom arguments, they ensure tests work consistently no matter where they run. This approach reduces errors and makes test maintenance easier.
Key Benefits:
- Command-line options give you precise control over test execution
- Custom arguments with
pytest_addoption
help adapt tests to different scenarios - Environment-specific settings make tests more reliable across different systems
Pytest's command-line features help teams write better tests and run them more efficiently. This combination of flexibility and control makes testing less frustrating and more productive for development teams of all sizes.
Creating Test Suites That Stand the Test of Time
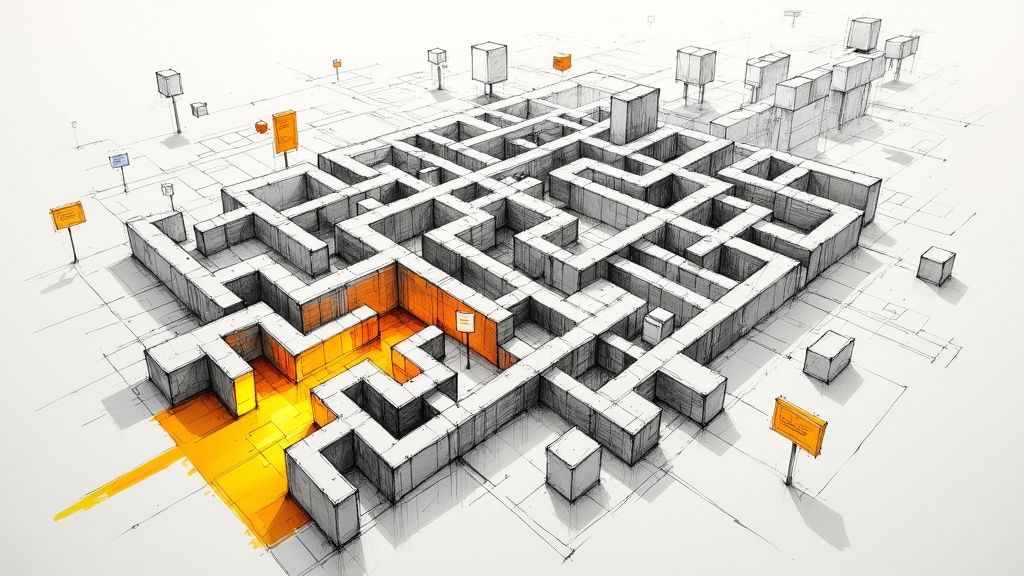
Building effective test suites with Python Pytest takes careful planning beyond just writing individual tests. By focusing on organization, clear naming, and good documentation from the start, you can create tests that remain useful and maintainable as your project grows.
Organizing Tests for Maximum Maintainability
The foundation of a good test suite is its structure. One proven approach is to mirror your application's directory layout within your tests
folder. This creates a direct mapping that makes tests easy to find and update. For example, if you have code in app/module.py
, its tests would go in tests/app/test_module.py
. This simple organization pays off as your codebase expands.
Clear Naming Conventions for Easy Understanding
Test names should clearly communicate what they verify. Using a format like test_[function_name]_[scenario]
makes each test's purpose immediately obvious. A test named test_calculate_total_valid_input
tells you exactly what function it tests and under what conditions. This clarity helps both current and future developers understand the test suite.
Documentation: Turning Tests into Living Documentation
Well-written tests serve as examples of how code should work, but adding clear docstrings makes them even more valuable. These docstrings should explain what the test verifies, any important setup or context, and why certain choices were made. This documentation helps others maintain and extend the tests confidently.
Avoiding Brittle Tests: Best Practices
Tests that break with minor code changes become a maintenance headache. The key is focusing on testing behavior rather than implementation details. This means verifying what your code does (its outputs and effects) rather than exactly how it achieves those results. This approach creates more stable tests that continue working even as implementation details change.
Framework-Agnostic Principles for Test Design
Good test design principles work across any testing framework. The FIRST principles provide a solid foundation:
- Fast: Tests should run quickly
- Independent: Tests shouldn't depend on each other
- Repeatable: Tests should give consistent results
- Self-Validating: Tests should clearly pass or fail
- Thorough: Tests should cover important cases
Pytest Fixtures: Enhancing Test Reusability
Fixtures in Pytest provide a clean way to handle test setup and cleanup. For example, a database fixture can create test data and clean it up automatically for any test that needs it. This removes duplicate setup code and ensures consistent test environments. When multiple tests need the same resources or starting conditions, fixtures keep your code DRY and easier to maintain.
Parameterization for Comprehensive Testing
Pytest's parametrize feature lets you run the same test logic with different inputs and expected results. Instead of writing separate test functions for each case, you can define one test with multiple sets of parameters. This is perfect for testing edge cases, boundary conditions, and different valid inputs while keeping your test code concise and clear.
By combining these practices - thoughtful organization, clear names, good documentation, and Pytest's powerful features - you create test suites that help rather than hinder development. This foundation helps ensure your tests remain valuable tools for maintaining code quality over time.
Optimizing Performance and Solving Common Challenges
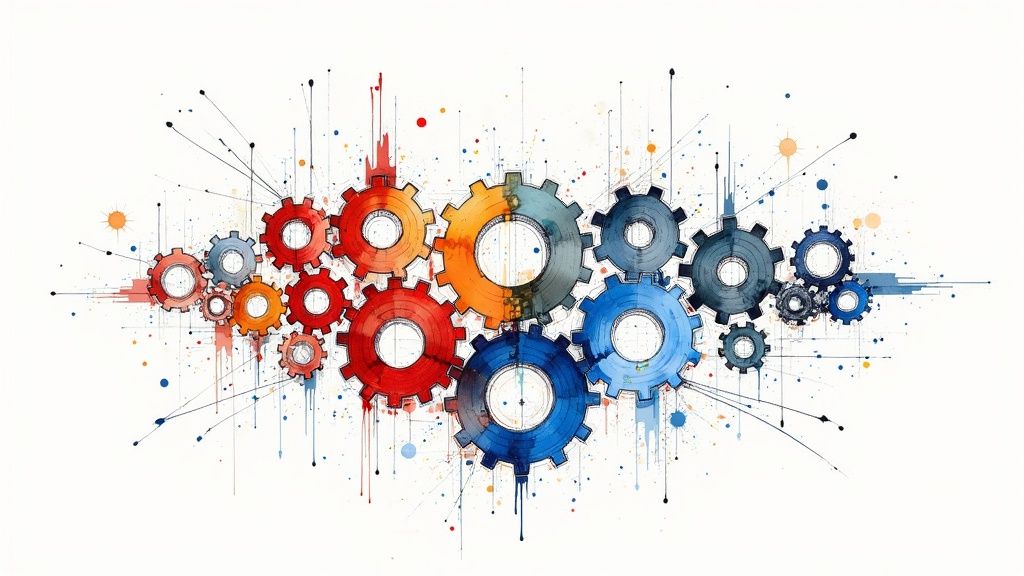
Growing test suites can strain your testing workflow. When your project expands, keeping tests fast and reliable becomes essential. Let's explore practical solutions to speed up testing and solve common issues that teams face when working with Python Pytest.
Parallel Test Execution for Faster Results
Running tests one after another takes time, especially with hundreds of tests. That's where Pytest-xdist comes in - with over 18 million downloads, this plugin lets you run tests across multiple CPU cores at once. Think of it like having several cooks in the kitchen instead of just one. For large projects, parallel testing can cut run times dramatically, helping you catch bugs and ship fixes faster.
Selective Test Running for Targeted Testing
You don't always need to run every test in your suite. Pytest has built-in options to run specific tests that matter right now. The -k
flag lets you filter by test name - for example, pytest -k "database"
runs only database-related tests. You can also use markers to group and run tests by feature. This focused approach saves time during development and debugging by running just the tests you need.
Performance Profiling to Identify Bottlenecks
Individual tests can become slow over time. Pytest plugins help you find exactly where tests are getting bogged down. Like a mechanic's diagnostic tools, profiling shows which parts of your test code are running slowly so you can fix the specific issues slowing things down.
Debugging Failing Tests: A Practical Approach
Clear error messages make all the difference when tests fail. Pytest provides detailed failure reports with stack traces and assertion details. Most modern code editors integrate smoothly with Pytest, letting you debug tests right in your development environment. Setting breakpoints and checking variables helps pinpoint issues quickly so you can get tests passing again.
Monitoring and Early Issue Detection
Catching problems early prevents bigger headaches later. Adding monitoring to your CI/CD pipeline helps you spot concerning patterns in your tests. Track key metrics like test run times, success rates, and code coverage to find areas that need attention. Like regular health checkups, monitoring your test suite helps catch and fix small issues before they grow into major problems.
Mergify can help speed up your development process by automating merges and managing pull requests efficiently. Their platform reduces CI costs while maintaining high code quality standards. Learn how Mergify can transform your workflow.