Master Python Parametrize: Write Efficient, Clean Tests
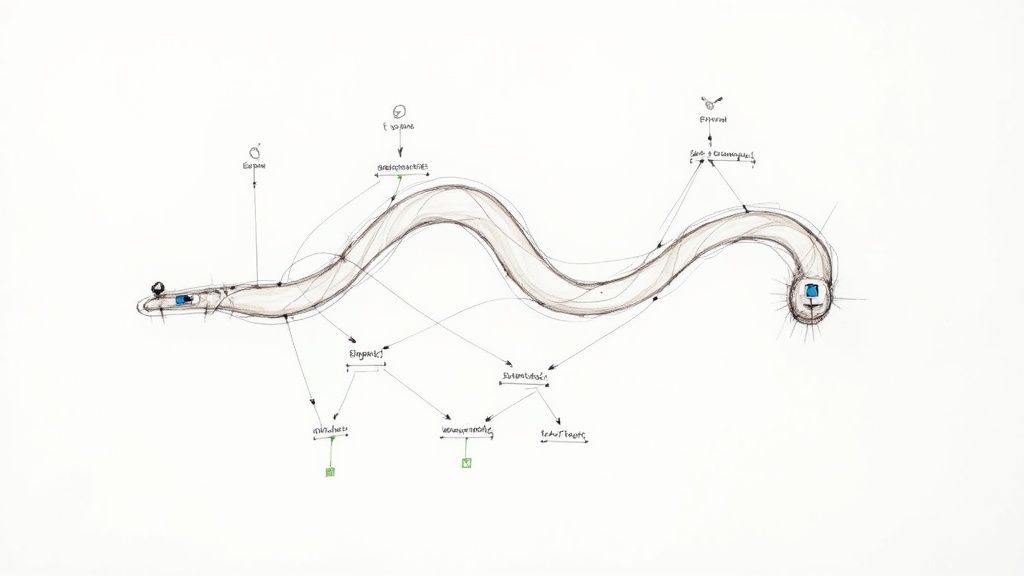
Why Python Parametrize Is Changing the Testing Game
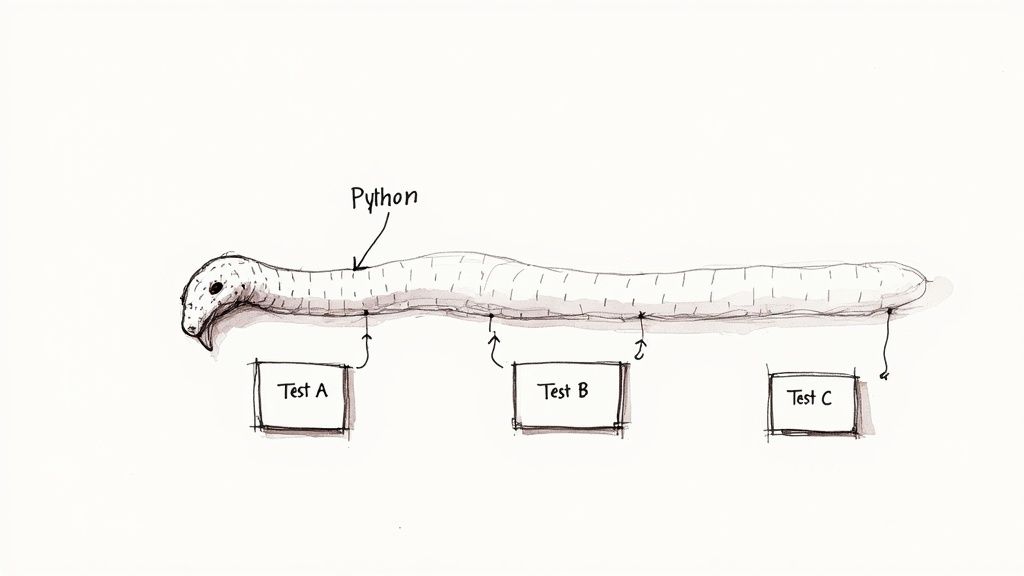
Writing repetitive tests is a familiar headache for developers. It's time-consuming, error-prone, and makes maintaining a large test suite a real challenge. This is where Python parametrize, and more specifically pytest.mark.parametrize
, enters the scene. It offers a much-needed improvement to testing workflows.
This seemingly simple decorator lets developers execute the same test function with various sets of input values, fundamentally altering how we approach test design and execution. Instead of crafting separate test functions for each scenario, you write one function and parametrize the inputs.
This move toward parametrized testing provides several key benefits. It drastically cuts down on code duplication, resulting in tests that are easier to read, understand, and maintain.
For example, consider testing a function that calculates discounts. Instead of writing separate tests for 10%, 20%, and 30% discounts, you could create a single parametrized test covering all three scenarios.
Additionally, much like the need for rigorous testing of phishing emails, your Python tests also demand robust strategies. To improve your testing security, think about integrating phishing email testing strategies. This focus on streamlined and efficient processes underlines the importance of solid testing methods in software development.
The Impact of Pytest
Python's parametrize feature has significantly impacted how developers approach test automation. As of 2023, pytest stands as one of the most widely used testing frameworks for Python.
Its capacity to parametrize tests allows developers to run the same test with diverse inputs, saving time and boosting test coverage by automatically producing various test scenarios. For example, when performing cross-browser testing with Selenium, parametrization helps in testing multiple browser and OS combinations with minimal code duplication.
Articles highlight the scalability and efficiency of this kind of testing, stating that teams can decrease test execution times by up to 70% using methods such as parallelization. Learn more about pytest.mark.parametrize
here. This efficiency makes parametrized testing an invaluable asset in building reliable software with fewer resources.
Mastering Python Parametrize Syntax That Actually Works
Building upon the foundations of pytestpytest.mark.parametrize
, let's explore its sometimes intricate syntax. We'll use practical examples to illustrate how to structure your parameter names, efficiently organize test data, and create tests that clearly communicate their purpose. This section will also address common pitfalls that even experienced developers encounter and offer practical solutions to avoid them.
Understanding the Core Syntax
The core of pytest.mark.parametrize
lies in its decorator format. It accepts at least two arguments: a comma-separated string of parameter names and a list of values. This structure enables pytest
to inject these values into your test function.
- Parameter Names: These names are used within your test function to access the provided values. Choose descriptive names that reflect the data's purpose.
- Values List: This list contains the data your test will use. Each item in the list corresponds to a single test execution.
For example:
import pytest
@pytest.mark.parametrize("input, expected", [(1, 2), (3, 4), (5, 6)]) def test_increment(input, expected): assert input + 1 == expected
In this example, input
and expected
are the parameter names, and [(1, 2), (3, 4), (5, 6)]
is the list of value pairs. The test will run three times, once for each tuple.
Handling Complex Data Structures
pytest.mark.parametrize
isn't limited to simple values. It can handle complex data structures like dictionaries and lists, enabling you to test with more realistic scenarios.
import pytest
@pytest.mark.parametrize("user_data", [ {"name": "Alice", "age": 30}, {"name": "Bob", "age": 25} ]) def test_user_registration(user_data): assert "name" in user_data assert "age" in user_data
This example demonstrates how to parametrize a test with dictionaries, making it easier to test various user registration scenarios.
Debugging Parametrized Tests
Debugging parametrized tests might initially appear challenging, but pytest
offers tools to simplify this process. When a parametrized test fails, pytest
clearly identifies the specific parameter combination that caused the error. Writing meaningful error messages within your tests is crucial here, providing valuable context and accelerating debugging.
Building Maintainable Tests With Python Parametrize
As your test suite grows, maintainability becomes essential. By centralizing test data within the pytest.mark.parametrize
decorator, you eliminate code duplication and establish a single source of truth for your test parameters. This simplifies updating test cases and ensures consistency across different tests.
Let's explore some different ways to use the pytest.mark.parametrize
decorator. The following table summarizes key syntax variations and options.
Python Parametrize Syntax Options
Syntax Pattern | Use Case | Example | Notes |
---|---|---|---|
@pytest.mark.parametrize("param1, param2", [(val1, val2), (val3, val4)]) |
Multiple parameters | @pytest.mark.parametrize("input, expected", [(1, 2), (3, 4)]) |
Useful for testing functions with multiple inputs. |
@pytest.mark.parametrize("param", [val1, val2, val3]) |
Single parameter | @pytest.mark.parametrize("input", [1, 3, 5]) |
Simplifies tests with single inputs. |
@pytest.mark.parametrize("param", [{"key": val1}, {"key": val2}]) |
Dictionaries | @pytest.mark.parametrize("user", [{"name": "Alice"}, {"name": "Bob"}]) |
Effective for passing complex data structures. |
This table highlights how you can use pytest.mark.parametrize
with various data types and parameter combinations, allowing for flexible and maintainable testing scenarios. This flexibility is especially valuable in large projects with evolving requirements, making your tests more robust and adaptable to changes.
Advanced Python Parametrize Techniques That Scale
As your testing suite expands, basic parametrization might not suffice. This is where advanced techniques become invaluable, enabling you to manage complex scenarios without creating excessively large and difficult-to-manage tests. These techniques empower testing professionals to efficiently handle multi-dimensional test spaces.
Indirect Parametrization
Indirect parametrization allows for dynamic test environment setup. Instead of directly inputting values into your test function, you provide a string identifier. pytest
then uses this identifier to retrieve the actual parameters. This is especially helpful when your test setup involves external resources or complicated configurations.
import pytest
@pytest.fixture(params=["small", "large"]) def dataset(request): if request.param == "small": return [1, 2, 3] else: return list(range(1000))
@pytest.mark.parametrize("size_id", ["small", "large"]) def test_dataset_size(dataset, size_id): if size_id == "small": assert len(dataset) == 3 else: assert len(dataset) == 1000
This approach separates your test data from the test logic, which improves readability and simplifies maintenance.
Nested Parametrization
Nested parametrization ensures thorough test coverage by combining multiple parameter sets. This can be achieved by nesting pytest.mark.parametrize
decorators, effectively creating a matrix of all possible combinations.
import pytest
@pytest.mark.parametrize("x", [1, 2]) @pytest.mark.parametrize("y", [3, 4]) def test_combinations(x, y): assert isinstance(x * y, int)
This example generates four tests, covering every combination of x
and y
.
Conditional Parameters
Conditional parameters provide a way to bypass unnecessary test combinations. This is done by programmatically generating your parameter list based on specific conditions. This is especially beneficial when not all parameter combinations are valid or required.
import pytest
test_data = [] for os in ["windows", "linux"]: for browser in ["chrome", "firefox"]: if os == "linux" and browser == "chrome": test_data.append((os, browser))
@pytest.mark.parametrize("os, browser", test_data) def test_browser_compatibility(os, browser): # Test logic here pass
In this scenario, the test only runs for Chrome on Linux.
The infographic below illustrates the differences between standard tests and using the pytest.mark.parametrize
decorator for managing multiple test scenarios.
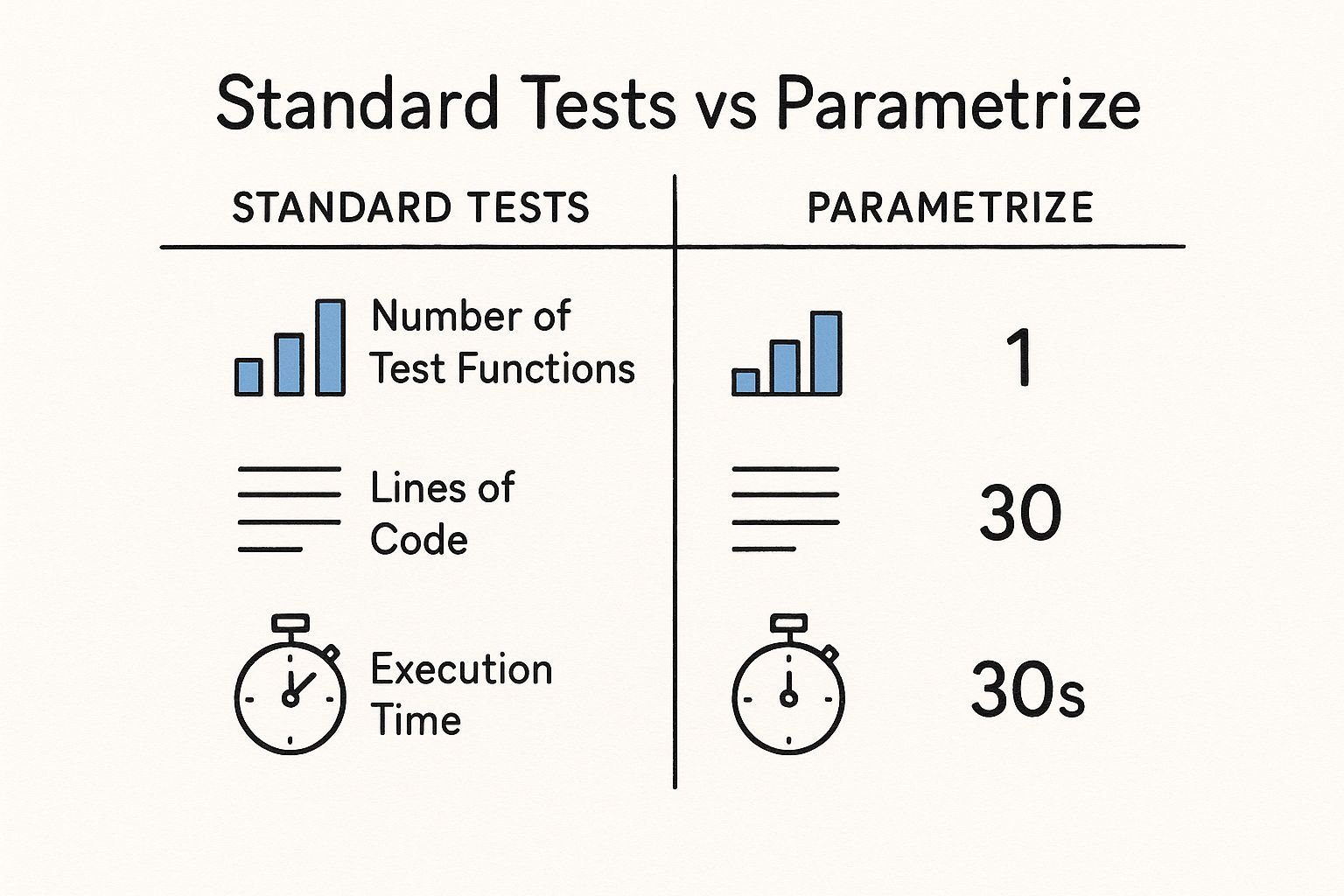
As shown, using parametrize
noticeably reduces code complexity and execution time while still providing comprehensive testing. The use of pytest.mark.parametrize
has steadily increased since its introduction. It simplifies testing by allowing a single test function to run with multiple input sets. This is particularly valuable for data-heavy applications that require numerous tests. Learn more about Python parametrize here.
Organizing and Generating Parameters
Managing extensive parameter sets demands careful organization. Using lists, dictionaries, or even external files can help keep your parameters manageable and readable. Parameters can also be generated programmatically, allowing for adaptation to evolving requirements. This dynamic generation is especially useful when test data needs to be created or modified during runtime. These advanced techniques enable efficient test scaling and adaptation to new application needs.
To further illustrate the differences between basic and advanced parametrization, let's look at a comparison table:
To help illustrate these concepts, let's examine a comparison table.
Comparing Basic vs. Advanced Parametrization Techniques A comparison of different parametrization approaches showing their benefits and limitations
Technique | Complexity | Use Cases | Advantages | Limitations |
---|---|---|---|---|
Basic Parametrization | Low | Simple input variations | Easy to implement, improves code reuse | Limited to direct input values, can become cumbersome with many parameters |
Indirect Parametrization | Medium | Dynamic test setup, complex configurations | Separates test data from logic, improves maintainability | Requires fixtures, adds a layer of indirection |
Nested Parametrization | Medium | Testing all combinations of multiple parameters | Comprehensive coverage | Can lead to a large number of tests |
Conditional Parametrization | Medium | Skipping irrelevant test combinations | Efficiently tests specific scenarios | Requires programmatic parameter generation |
This table highlights the trade-offs between simplicity and flexibility offered by different parametrization approaches. Choosing the right technique depends on the specific needs of your testing scenario.
Supercharging Test Performance With Python Parametrize
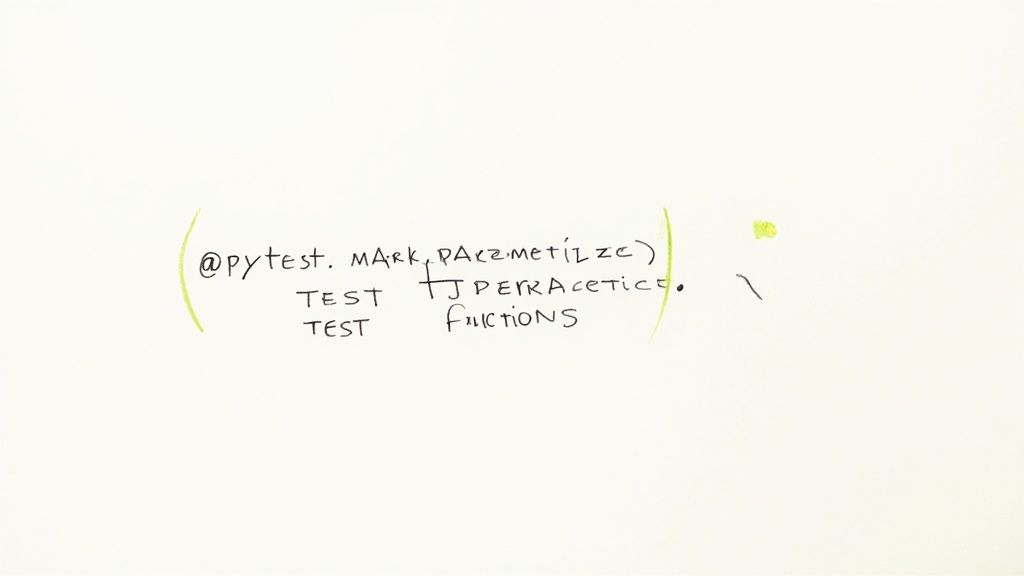
As your test suites grow, execution time can become a real bottleneck. This section explores how you can structure pytest parameterized tests for optimal performance without sacrificing thoroughness. We'll dive into techniques used by top-performing teams to significantly reduce testing time.
Optimizing for Parallelization
One of the best ways to speed up testing is through parallelization. Simply using pytest-xdist isn't enough, though. How you organize your parameters significantly impacts parallel efficiency.
Grouping related tests within a single parametrize
call can often improve how well tests run in parallel. This lets pytest distribute related test chunks to different workers, minimizing overhead and maximizing throughput.
Targeted Testing Subsets
Comprehensive test coverage is vital for your Continuous Integration (CI) pipeline. However, running every test during development can be slow.
By strategically choosing parameter combinations, you can create targeted test subsets. This lets you execute a smaller, faster group of tests during development while still ensuring full coverage in your CI runs. This approach dramatically speeds up the development feedback loop. Consider creating separate parameter sets for "smoke tests" and "regression tests" targeting specific features.
Profiling for Bottlenecks
Profiling your parameterized tests helps identify performance bottlenecks. Tools like pytest-profiling can pinpoint slow test functions or parameter combinations.
This data allows you to focus your optimization efforts where they'll have the most impact. You might discover that certain database queries or network requests take up a large chunk of the execution time. Addressing these bottlenecks can yield substantial performance gains without a major test suite overhaul.
Optimizations for Speed
After identifying bottlenecks, several strategies can boost test speed. Consider mocking external dependencies to isolate your test logic and avoid slow interactions with external systems.
Carefully managing test fixtures and setup/teardown processes can also minimize overhead. Even minor optimizations, like using efficient data structures or algorithms within your tests, can accumulate into considerable performance improvements. This focus on practical improvements ensures fast tests without compromising accuracy or value.
Python Parametrize: Enhancing Web and UI Testing
Web and UI testing presents unique difficulties. Fortunately, Python's pytest.mark.parametrize
offers elegant solutions. Leading QA teams are leveraging parametrization to significantly boost the efficiency of their web and UI testing. Let's explore how they achieve this.
Streamlining Cross-Browser and Cross-Device Testing
Testing across various browsers, devices, and viewport sizes can quickly become a maintenance nightmare due to code duplication. Parametrization offers a way out. By parametrizing tests with browser names, screen resolutions, or device types, you can execute the same test logic across multiple configurations. This reduces code bloat and simplifies maintenance.
import pytest from selenium import webdriver
@pytest.mark.parametrize("browser", ["chrome", "firefox", "safari"]) def test_login(browser): if browser == "chrome": driver = webdriver.Chrome() elif browser == "firefox": driver = webdriver.Firefox() # ... other browser setup ... driver.get("https://www.example.com/login") # ... test login functionality ... driver.quit()
This example demonstrates how a single test can cover multiple browsers using Selenium.
Efficient Form Validation Testing
Form validation is another area where parametrization excels. Imagine a form with many input fields and validation rules. Testing each combination manually is tedious and time-consuming. Using pytest.mark.parametrize
, you can define a set of input values and expected outcomes for each field.
@pytest.mark.parametrize("username, password, expected_result", [ ("valid_user", "correct_password", "success"), ("", "password", "username_required"), ("user", "", "password_required"), ]) def test_login_form(username, password, expected_result): # ... submit form with username and password ... # ... assert result matches expected_result ...
This approach ensures comprehensive form validation with minimal code.
Handling Timing-Sensitive UI Tests
UI tests often involve time-sensitive actions, such as waiting for elements to load or animations to complete. Parametrization helps manage these scenarios by allowing you to adjust wait times based on different browser or network conditions. You could, for example, parametrize the timeout duration for an element to appear, ensuring your tests remain robust across different network speeds and browser performance.
Managing Browser-Specific Quirks
Different browsers can exhibit quirks that affect how web pages render and function, making cross-browser testing a challenge. With Python parametrization, you can incorporate browser-specific logic or workarounds into tests without cluttering the main test code. You could use conditional logic within your test based on the browser parameter to handle specific browser behaviors.
Real-World Implementation Examples
Popular testing frameworks integrate seamlessly with pytest.mark.parametrize
.
- Selenium: As shown previously, Selenium is easily parametrized for cross-browser testing.
- Playwright: Playwright also supports parametrization for efficient cross-browser and cross-device testing.
By implementing these strategies, you can harness the power of Python parametrize to simplify web and UI testing. The result is more reliable and maintainable tests, ultimately leading to improved software quality and faster release cycles.
Real-World Python Parametrize Success Stories
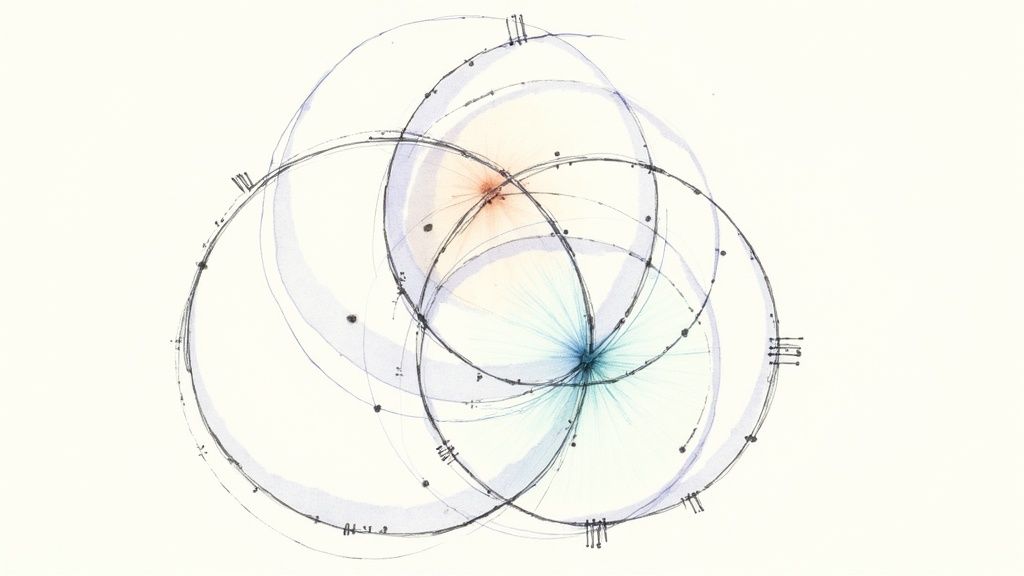
Practical examples are often the best way to understand the power of a new technique. This section dives into how several companies successfully integrated Python's parametrize
function to bolster their software testing strategies. We'll explore the initial challenges they faced and how parameterized testing provided effective solutions.
API Testing at Scale: Streamlining With Parametrization
A rapidly expanding fintech startup found itself struggling to keep up with the demands of testing its increasingly complex API. Hundreds of endpoints, combined with a multitude of data input combinations, made maintaining a comprehensive test suite a significant burden. The solution? Python's parametrize
function.
- Challenge: Maintaining a large and intricate API test suite consumed valuable resources and slowed down development progress.
- Solution: Using Pytest
pytest.mark.parametrize
, the team condensed hundreds of individual test functions into a much smaller, more manageable set of parameterized tests. This significantly reduced code duplication and improved the overall maintainability of the test suite. - Results: The company saw a dramatic 60% reduction in test code and a substantial 40% decrease in test execution time.
Data-Intensive Scientific Applications: Enhancing Test Coverage
A research team dealing with large datasets faced a constant battle to ensure their code performed reliably across diverse data inputs. Manually creating test cases for every possible scenario was an impossible task. Python's parametrize
offered a practical solution.
- Challenge: Testing code with numerous data variations proved impractical, leading to gaps in test coverage and potential vulnerabilities.
- Solution: The team implemented
parametrize
to automatically generate tests, covering a wide range of data combinations. This automated approach allowed them to explore edge cases and uncover scenarios that had previously gone untested. - Results: They observed a significant increase in test coverage, which, in turn, led to the discovery and correction of critical bugs. This greatly enhanced the reliability and trustworthiness of their research results.
Integrating Parametrized Testing into CI/CD: A Case Study
A major e-commerce company aimed to integrate parameterized testing into their CI/CD pipeline to speed up testing without compromising quality. However, they initially encountered resistance from team members unfamiliar with the method.
- Challenge: Integrating new testing methodologies into established workflows can be challenging due to internal resistance and the need for team training.
- Solution: The company addressed this by investing in training focused on Python
parametrize
. They demonstrated its advantages through small, focused pilot projects, building confidence and encouraging wider adoption across the development team. They also integratedpytest
into their CI/CD pipeline, automating test execution with every code change. - Results: Integrating parameterized tests into the CI/CD pipeline drastically reduced overall testing time, enabling faster release cycles and fewer regressions.
Lessons Learned and Best Practices
These case studies highlight valuable lessons about using Python parametrize
in real-world scenarios. Here are some key takeaways:
- Start Small: Begin with a small pilot project to showcase the benefits of parameterized testing.
- Provide Training: Invest in training to empower your team with the skills to use
parametrize
effectively. - Integrate with CI/CD: Automate test execution through
pytest
integration with your CI/CD pipeline. - Organize Parameter Data: As your test suite expands, manage parameter data efficiently using external files or programmatic generation.
By following these best practices, teams can unlock the full power of Python parametrize
to improve test quality, accelerate development, and ultimately deliver better software.
Streamline your team's development process and experience the benefits of efficient, automated code integration with Mergify. Mergify's robust features, such as Merge Queue and CI Insights, simplify your workflow and reduce CI costs, allowing your team to concentrate on building exceptional software.