Pytest Timeout Strategies to Speed Up Testing
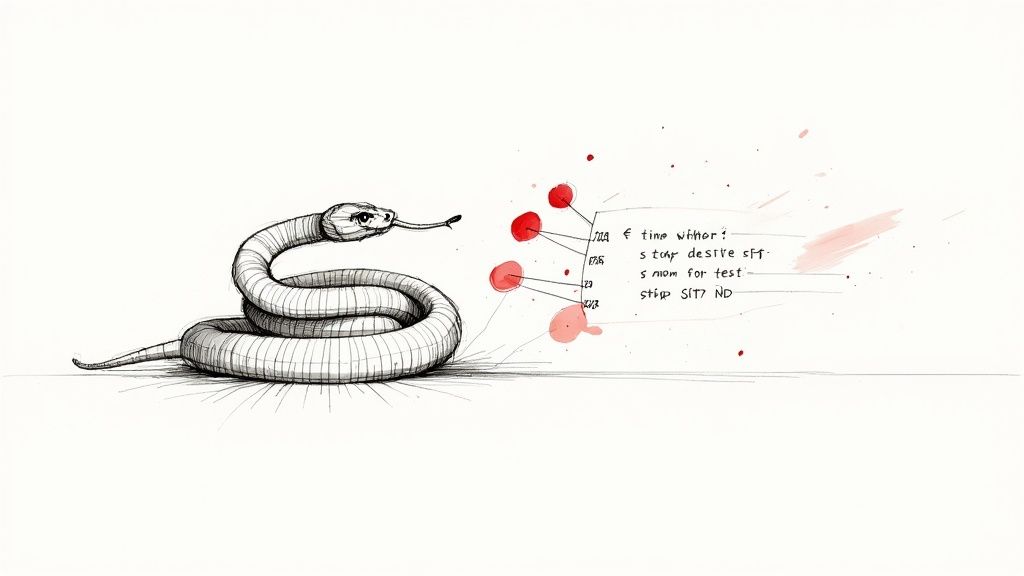
Why Pytest Timeout Matters: Beyond Basic Test Efficiency
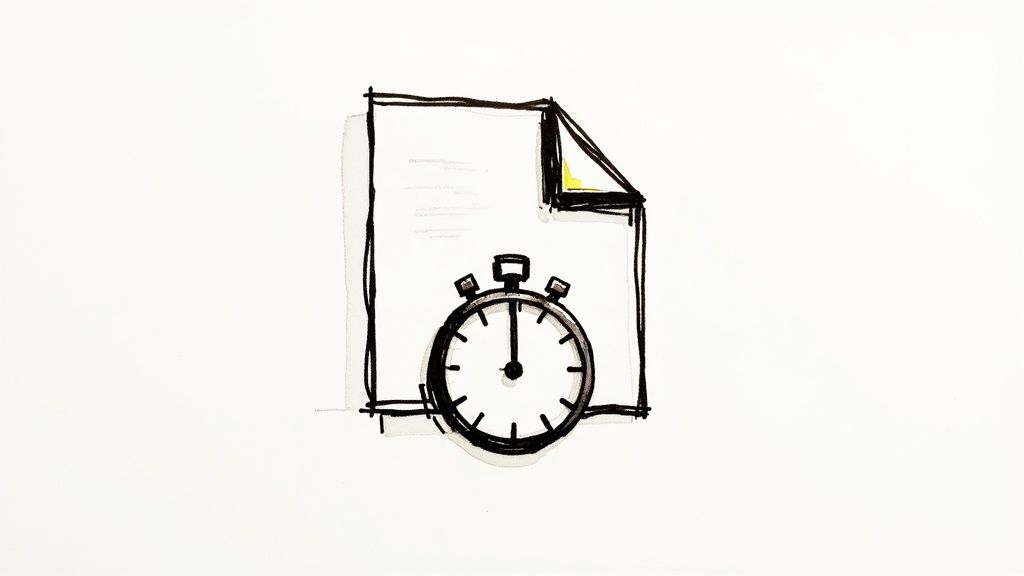
Runaway tests can quietly drain your development resources and time. They stall pipelines and lead to team frustration. This is precisely where pytest timeout becomes essential. It acts as a safeguard, stopping tests from running endlessly and preventing bottlenecks in your workflow. This goes beyond simple test efficiency; it's about protecting your development momentum and your team's well-being.
Understanding The Need For Timeouts
Even code that appears straightforward can contain hidden problems. A minor logic error, for instance, can accidentally trigger an infinite loop, trapping a test indefinitely. Similarly, deadlocks, situations where processes are stuck waiting for each other, can also halt test execution. Pytest timeouts help prevent these issues from disrupting your development process.
How Pytest Timeout Works: A Protective Shield
The pytest-timeout
plugin addresses long-running tests by imposing time limits. Tested on Python 3.6 and above, including PyPy3, this plugin ensures wide compatibility. You can set timeouts globally, such as using pytest --timeout=300
for a 300-second limit on all tests. You can also configure timeouts in your pytest.ini
file. This prevents indefinite hangs, especially valuable in resource-limited CI environments. For more granular control, @pytest.mark.timeout()
allows per-test timeouts, accommodating tests of varying lengths. To ensure consistency with your pytest timeout setup, implementing solid documentation and control procedures is crucial. You can learn more about this in this article on Documentation Control Procedures.
The Impact of Unmanaged Timeouts
Without effective timeout management, one problematic test can create a domino effect. Consider a CI pipeline delayed for hours due to a single hung test. This slows down feedback, blocks other tests, and ultimately hinders your entire development cycle. Implementing pytest timeouts ensures tests finish within a defined timeframe, maintaining a smooth development process. You might find this interesting: More content related to development.
Beyond Preventing Hangs: Proactive Performance Improvement
Pytest timeouts aren't just about preventing major hangs. They can also be used proactively to enhance code quality. By setting challenging, but realistic timeouts, you encourage yourself to address and optimize inefficient code. A test consistently hitting a timeout suggests a potential performance bottleneck that requires attention. This helps you identify and remove slow code paths, creating more efficient and robust software.
Getting Started: Pytest Timeout Installation and Setup
Stop watching your test suite crawl along—let's get pytest timeout working for you. We'll walk through installation, providing clear examples for various environments, from local development to containerized setups. You'll learn how seasoned developers strategically choose between thread-based and signal-based timeout methods. We'll also cover helpful configuration patterns that encourage consistency across development teams. Finally, we’ll explore how to verify your timeout setup and troubleshoot typical problems that can create a false sense of security.
Installing Pytest Timeout
The first step is installing the pytest-timeout plugin. You can easily install it with pip
:
pip install pytest-timeout
This command installs the most recent plugin version. If you need a specific version for compatibility reasons, use:
pip install pytest-timeout==
This targeted approach ensures your project's dependencies work harmoniously.
Choosing Your Timeout Method
pytest-timeout
provides two main methods for enforcing timeouts: thread-based and signal-based. The thread-based method is generally preferred for its broad compatibility across different operating systems. It operates by running each test in its own thread, monitoring it for timeout violations. However, this method might not be suitable for tests that utilize C extensions which release the GIL. The signal-based method, while potentially more efficient, can be less dependable across different operating systems. Understanding these differences helps you choose the best approach for your needs.
Basic Configuration
After installing the plugin, configure your timeout globally in your pytest.ini
file:
[pytest] timeout = 300
This establishes a default timeout of 300 seconds for every test. You can also specify the timeout via the command line:
pytest --timeout=300
This provides flexibility, allowing you to override the default setting for specific testing scenarios.
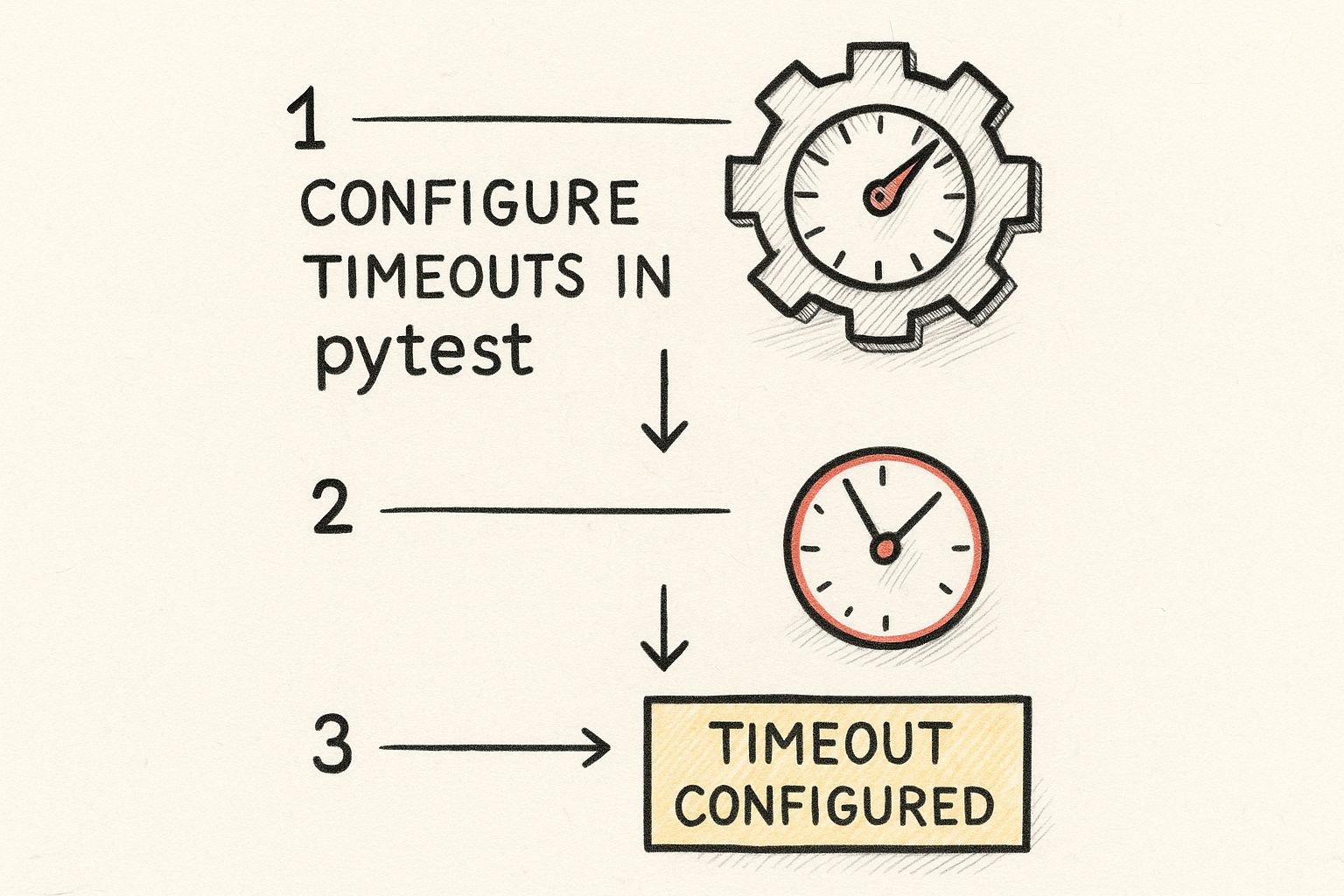
The infographic above illustrates configuring timeouts in pytest, emphasizing the relationship between the timer and the overall settings. This visualization highlights that configuring pytest timeouts involves both setting the duration and integrating it into the pytest framework.
Let's take a look at different installation methods and their comparison:
The following table outlines the various ways to install and utilize the pytest-timeout
plugin, highlighting their strengths and weaknesses. This comparison should help you choose the method best suited for your project.
Installation Method | Command | Advantages | Limitations |
---|---|---|---|
Using pip (Latest Version) | pip install pytest-timeout |
Simple, installs the most up-to-date version. | May introduce compatibility issues with existing project dependencies if a newer version has breaking changes. |
Using pip (Specific Version) | pip install pytest-timeout==<version_number> |
Ensures compatibility by installing a designated version. | Requires knowing the compatible version beforehand. |
As you can see, selecting a specific version offers greater control over compatibility, while installing the latest version simplifies the process but introduces a potential risk of incompatibility. Choose the method that aligns with your project's stability and update frequency.
Verifying Your Setup
To ensure pytest-timeout
is working as expected, create a test designed to exceed the specified timeout:
import pytest import time
@pytest.mark.timeout(5) # Setting a 5-second timeout for this specific test def test_timeout(): time.sleep(10) # This will induce a timeout
Running this test should trigger a pytest.fail.Exception
, signaling that the test timed out. This confirms your setup is active and correctly interrupting tests exceeding the allowed time. This important verification step ensures your tests are properly monitored and you’re not working under a false sense of security. Now you're ready to explore more advanced pytest timeout configurations.
Advanced Timeout Techniques That Actually Work
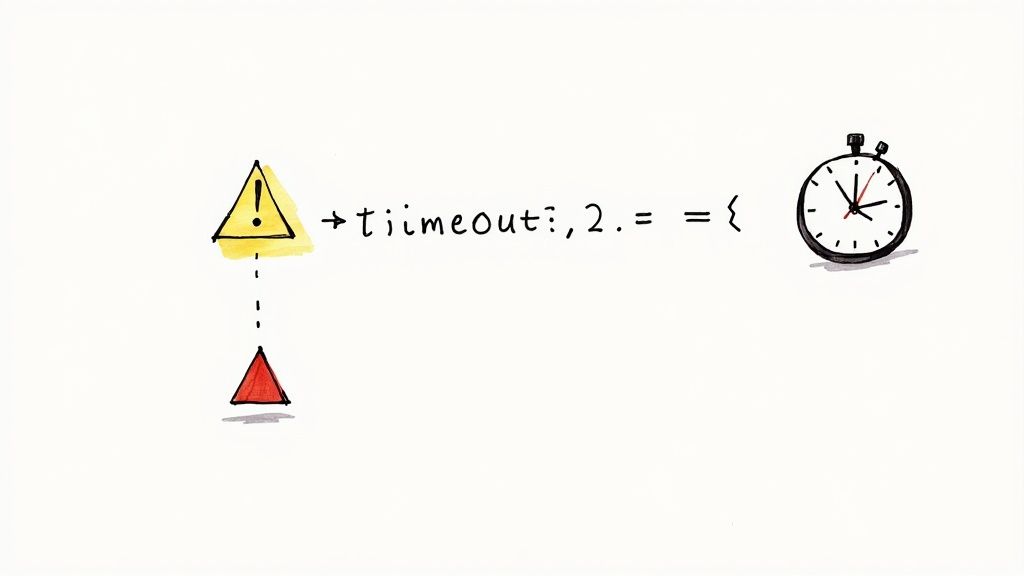
Beyond a basic global timeout, Pytest offers powerful tools for fine-tuning timeout control. This allows you to create a robust and adaptable timeout strategy, ensuring efficient test runs while accurately identifying problematic code. Let's delve into these advanced techniques.
Test-Specific Timeouts
Not all tests are the same. Some inherently take longer due to complexity or resource consumption. With the @pytest.mark.timeout()
decorator, you can customize the timeout duration for individual tests.
import pytest import time
@pytest.mark.timeout(10) def test_long_running_operation(): time.sleep(8) # Simulate a long operation assert True
@pytest.mark.timeout(1) def test_quick_check(): assert True
This gives long-running operations ample time while monitoring quicker checks for unexpected delays, preventing false positives where long but valid tests are incorrectly flagged as timeouts.
Dynamic Timeout Policies
For greater flexibility, implement dynamic timeout policies. This adjusts timeout duration based on factors like the testing environment, test category, or even historical test performance. For instance, longer timeouts might be suitable for development environments compared to resource-constrained continuous integration systems.
Use environment variables or configuration files to set different timeout values based on context:
import pytest import os
timeout_duration = int(os.environ.get("TEST_TIMEOUT", "5")) # Default to 5 seconds
@pytest.mark.timeout(timeout_duration) def test_environment_dependent_operation(): # ... test code ... pass
This approach allows your timeout strategy to adapt, optimizing efficiency without sacrificing test coverage or accuracy. Refining timeouts based on observed performance improves overall test suite speed. Strategic timeout usage catches errors and encourages more efficient code, mitigating potential deadlocks or uncovering inefficient implementations.
The following table provides a summary of different timeout configuration methods within Pytest:
Timeout Configuration Methods in Pytest
A comprehensive overview of different ways to configure timeouts in pytest, showing syntax examples and use cases.
Method | Syntax | Scope | Use Case | Priority Order |
---|---|---|---|---|
Command-line option | pytest --timeout=300 |
Global | Sets a timeout for all tests | Lowest |
Configuration file (pytest.ini ) |
[pytest]\ntimeout = 300 |
Global | Configures timeout globally | Second lowest |
Marker decorator | @pytest.mark.timeout(10) |
Test/Module/Class | Applies timeout to specific tests, modules, or classes | Highest |
Dynamic timeout | timeout_duration = int(os.environ.get("TEST_TIMEOUT", "5"))\n@pytest.mark.timeout(timeout_duration) |
Test/Module/Class | Allows for context-dependent timeouts based on environment variables or configuration settings | Highest (when combined with marker decorator) |
This table outlines the various timeout configuration methods, their scope, typical use cases, and their order of precedence. Understanding these methods empowers you to craft a comprehensive and efficient timeout strategy tailored to your project's specific needs.
Gradually Tightening Timeouts
One of the most effective ways to optimize long-running tests is by gradually tightening timeouts. Start generously and progressively reduce it while monitoring performance. This iterative approach identifies performance bottlenecks without premature test failures. It’s especially useful for finding and fixing slow tests without causing false failures.
By combining these advanced timeout techniques—test-specific timeouts, dynamic timeout policies, and gradual tightening—you create a sophisticated strategy that harmonizes with your workflow. This not only increases test suite efficiency but also proactively contributes to better code quality. For more insights into pytest timeouts and best practices, check out this helpful resource: Explore this topic further.
Integrating Pytest Timeout in Your CI/CD Pipeline
Integrating timeout strategies within your Continuous Integration/Continuous Deployment (CI/CD) pipeline is crucial for maintaining a fast and efficient workflow. Unattended tests in a CI environment can easily stall the entire build process if they encounter unexpected delays or infinite loops. This is where pytest-timeout
plays a vital role, ensuring your pipeline remains healthy and responsive. You can learn more about CI/CD integration in this article.
Environment-Specific Configurations
CI environments often have stricter time constraints than local development setups. You might need more aggressive timeout settings in your CI pipeline. Using environment variables allows you to fine-tune timeout durations based on the specific environment.
For example, you could set a shorter timeout for your CI builds using an environment variable like CI_TIMEOUT
. This allows your local tests to run with more lenient timeouts while keeping your CI builds fast.
Analyzing Timeout Patterns
Tracking timeout occurrences across builds can help identify recurring issues and problematic tests. If a particular test frequently times out in your CI, it indicates a potential performance bottleneck or underlying code problem. This data empowers you to proactively address performance issues and optimize your tests.
By analyzing these trends, you can target your optimization efforts and improve the overall efficiency of your test suite. Effectively managing timeouts in pytest has become increasingly vital as CI/CD adoption grows. Prolonged test execution can cause significant deployment delays. The widespread use of the pytest-timeout
plugin, evidenced by its thousands of downloads on PyPI, demonstrates its importance in managing test times and ensuring efficient software delivery. Discover more insights about Pytest timeouts in this article.
Balancing Speed and Flexibility
While aggressive timeouts keep your CI pipeline moving, it's important to maintain some flexibility. Some tests, especially those involving external dependencies or resource-intensive operations, may legitimately require longer execution times.
For such tests, consider using higher, test-specific timeouts. This ensures that legitimate variations in test execution time don't lead to false failures, while still guarding against truly problematic tests.
Practical Example: Configuring Timeouts in a CI Environment
Let's consider a simple example using environment variables within your CI configuration file:
.gitlab-ci.yml (or similar CI configuration)
variables: CI_TIMEOUT: "60" # Timeout in seconds for CI environment
stages:
- test
test: image: python:latest script: - pip install pytest pytest-timeout - pytest --timeout=$CI_TIMEOUT
This configuration sets the timeout to 60 seconds specifically for your CI runs. This example demonstrates how environment variables inject context-specific timeout values, providing the control and flexibility needed to optimize your CI pipeline's performance. This approach guarantees that timeouts are appropriately set based on the environment and the unique demands of your CI/CD workflow.
Troubleshooting Real-World Pytest Timeout Challenges
Let's be honest: implementing timeouts can be tricky. While setting up pytest-timeout might seem initially straightforward, real-world scenarios often present more complex hurdles. This section explores these common timeout-related issues and offers practical solutions for navigating them.
Distinguishing Between Code Problems and Environmental Factors
A test timing out doesn't automatically point to faulty code. Sometimes, external factors are to blame. A slow network, overloaded servers, or resource contention within a CI/CD pipeline can cause perfectly valid tests to exceed their allotted time. Before you start debugging code, consider whether outside factors might be playing a role. For more insights into CI/CD and how external factors influence pipelines, check out this guide on Capacitor OTA updates CI/CD integration.
Debugging Intermittent Timeout Issues
Intermittent timeouts are especially frustrating. These tests pass sometimes and fail others due to variations in timing. Identifying the root cause requires careful investigation. Start by increasing verbosity in your pytest output with the -v
flag. This gives detailed information about each test's execution, potentially revealing hidden clues. If the problem seems connected to particular test data, try isolating and repeatedly running those cases to reliably reproduce the timeout. Tools like logging and profiling can pinpoint bottlenecks in your test code or dependencies contributing to these intermittent slowdowns.
Handling Resource-Intensive Tests
Some tests legitimately take longer due to their inherent complexity or resource usage. Simply increasing the global timeout is not the answer. This can mask genuine problems elsewhere in your suite. Instead, use test-specific timeouts with the @pytest.mark.timeout()
decorator. This grants more time where needed without weakening your overall timeout strategy.
Example: Debugging a Database Interaction Timeout
Imagine a test interacting with a database. It consistently times out in your CI/CD environment but passes locally. Checking the CI/CD server’s resource utilization reveals the database server is under heavy load during test execution. The solution? Either optimize the database queries within the test or allocate more resources to the database server in your CI/CD environment. This highlights how important context is for effective troubleshooting.
Strategies for Preventing False Alarms
To minimize false alarms, create clear timeout policies within your team. Document the reasons behind specific timeout values, and review and adjust them as your codebase grows. Consider implementing a tiered timeout system, with shorter timeouts for unit tests and longer ones for integration tests. This provides quick feedback from fast tests while giving more complex tests sufficient time to complete. Adopting these strategies builds confidence in your test results and supports a more productive development workflow.
Pytest Timeout Best Practices: What Actually Works
Don't settle for basic pytest timeout configurations. Successful testing teams go beyond default settings, using timeouts as dynamic tools that adapt to their code. Let's explore what sets these teams apart.
Determining Appropriate Timeout Values
Effective teams use data, not guesswork, to determine timeout durations. They analyze historical test execution times, considering factors like network latency and external service dependencies. For example, a test consistently finishing in 10 seconds might have a 15-second timeout, allowing for fluctuations.
This data-driven approach ensures realistic timeouts, catching real issues without false alarms.
Implementing Tiered Timeout Strategies
Different tests have different needs. High-performing teams use tiered timeout strategies, assigning shorter timeouts to quick unit tests for rapid feedback and longer timeouts for complex integration tests. This targeted approach balances efficiency and thoroughness.
Continuous Monitoring and Refinement
Timeout values should evolve with your code. Leading teams monitor timeout patterns, tracking test durations and identifying trends using tools and dashboards. A sudden increase in a test's execution time could signal a performance regression requiring attention. This keeps tests effective and informative. For further resources on Python testing, explore our sitemap pages related to Python testing.
Documentation and Communication
Top teams document their timeout decisions clearly, explaining the logic behind specific values and sharing best practices. This shared understanding fosters collaboration and ensures consistent timeout strategies across the team.
Building a Robust and Adaptive Timeout Strategy
These best practices transform pytest timeout from a basic safety net into a proactive tool for better code. You'll build a robust, adaptive strategy that catches real problems while minimizing distractions, letting your team focus on building great software.
Ready to improve your development process? Learn how Mergify automates pull request workflows and integrates with your testing process.