How to pytest show print statements During Tests
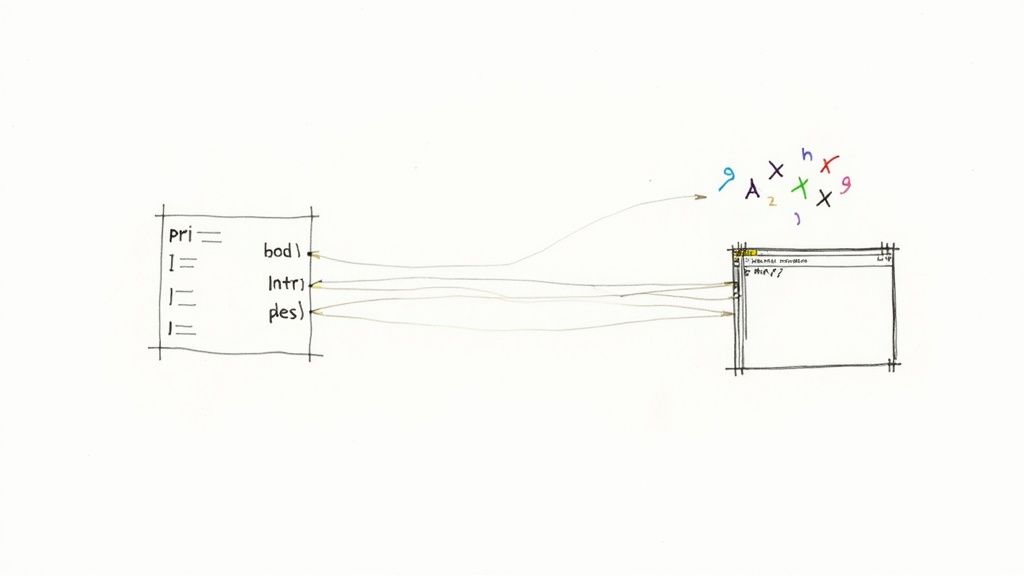
Understanding How Pytest Handles Print Output
Pytest, the popular Python testing framework, handles print statements in a specific way, which is crucial for effective debugging. Understanding this behavior will help you interpret and utilize those helpful debug messages during your tests. Let's explore why your print statements might seem to disappear and how this characteristic actually benefits your testing process.
Why Pytest Captures Print Statements
Pytest captures print statements by default. This means they won't immediately show up in your console. This isn't an error, but a deliberate design choice. Pytest prioritizes clean, concise test results, highlighting pass/fail outcomes rather than the internal workings of your code. This keeps the output focused, especially useful when running extensive test suites. Imagine the clutter if hundreds of tests were all printing debug information!
This capture mechanism prevents information overload. This default behavior leads to more organized test results, improving your development workflow. However, during debugging, access to those print statements becomes essential. This is where understanding Pytest's flexibility comes in handy. Historically, print statements have been captured by default in Pytest. This keeps the output clean, focusing on the test results. However, developers often need to see these print statements for debugging. Even in 2024, online communities continue to discuss solutions for displaying print statements. Using specific flags or plugins can help achieve this visibility. The pytest-print plugin is becoming increasingly popular, providing a convenient way to report sub-steps in long-running tests or provide additional debug information. This points towards a demand for customizable output in testing frameworks like Pytest. Learn more on this topic at the LambdaTest Community.
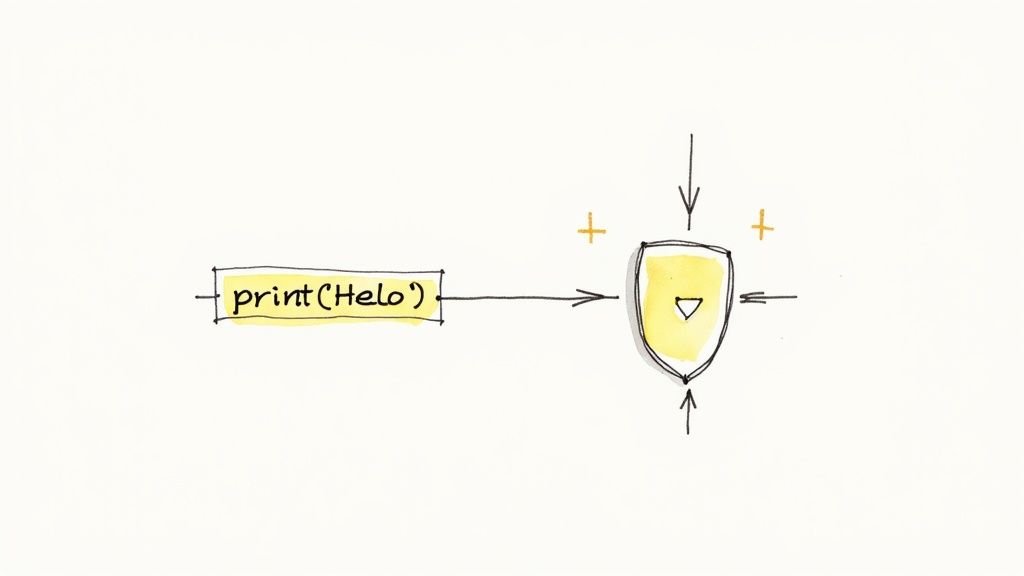
Unveiling Hidden Output
Pytest offers multiple ways to reveal these captured print statements when you need them. Command-line flags, configuration files, and plugins give you back control of your output. This targeted approach maintains clean output for general testing while giving you necessary details during debugging. These options let developers balance conciseness with informational richness. We’ll explore these techniques further in later sections.
Command-Line Strategies for Revealing Print Statements
Controlling the visibility of print statements in your pytest output is essential for efficient debugging. It's a balancing act: keeping test reports clean while having the detailed information you need when things go wrong. Pytest's command-line options give you the control to achieve this balance.
Using the -s Flag
The -s
flag (short for --capture=no
) is invaluable for displaying print statements during your test runs. Normally, pytest captures standard output and standard error, hiding print statements from your console. The -s
flag disables this, letting you see print statements in real-time as tests run. This immediate feedback is crucial for identifying issues during development or dissecting a failing test's logic.
Verbosity Options: -v and -vv
While -s
reveals all print statements, verbosity flags offer more refined control. The -v
flag (verbose) provides additional test details, showing the names of individual test functions as they execute. When debugging, it's also important to ensure your website works across different browsers; you might want to test my website on different browsers. The -vv
flag (very verbose) provides even more granularity, which can be helpful for complex test suites or intricate test interactions. Use -vv
strategically, though, as excessive output can become overwhelming.
To help illustrate how these flags work, let's take a look at the following table:
To understand how pytest handles output, and the specific roles of the -s
flag and verbosity flags like -v
and -vv
, refer to the following table. It provides a clear comparison of these options and their effects.
Option | Description | Use Case | Effect on Test Output |
---|---|---|---|
(No flag) | Default: Captures stdout/stderr | Clean test reports, hides print statements | Only shows test results (pass/fail), errors, and warnings |
-s |
Disables capturing | Debugging, viewing print statements | Displays all print statements during test execution |
-v |
Verbose output | More detailed test information | Shows individual test function names, additional test details |
-vv |
Very verbose output | Granular test information (can be overwhelming) | Highly detailed output for complex tests and suites |
This table summarizes how default capturing affects print statement visibility and how the -s
flag alters this behavior. The verbosity flags add layers of detail, assisting in more complex debugging situations.
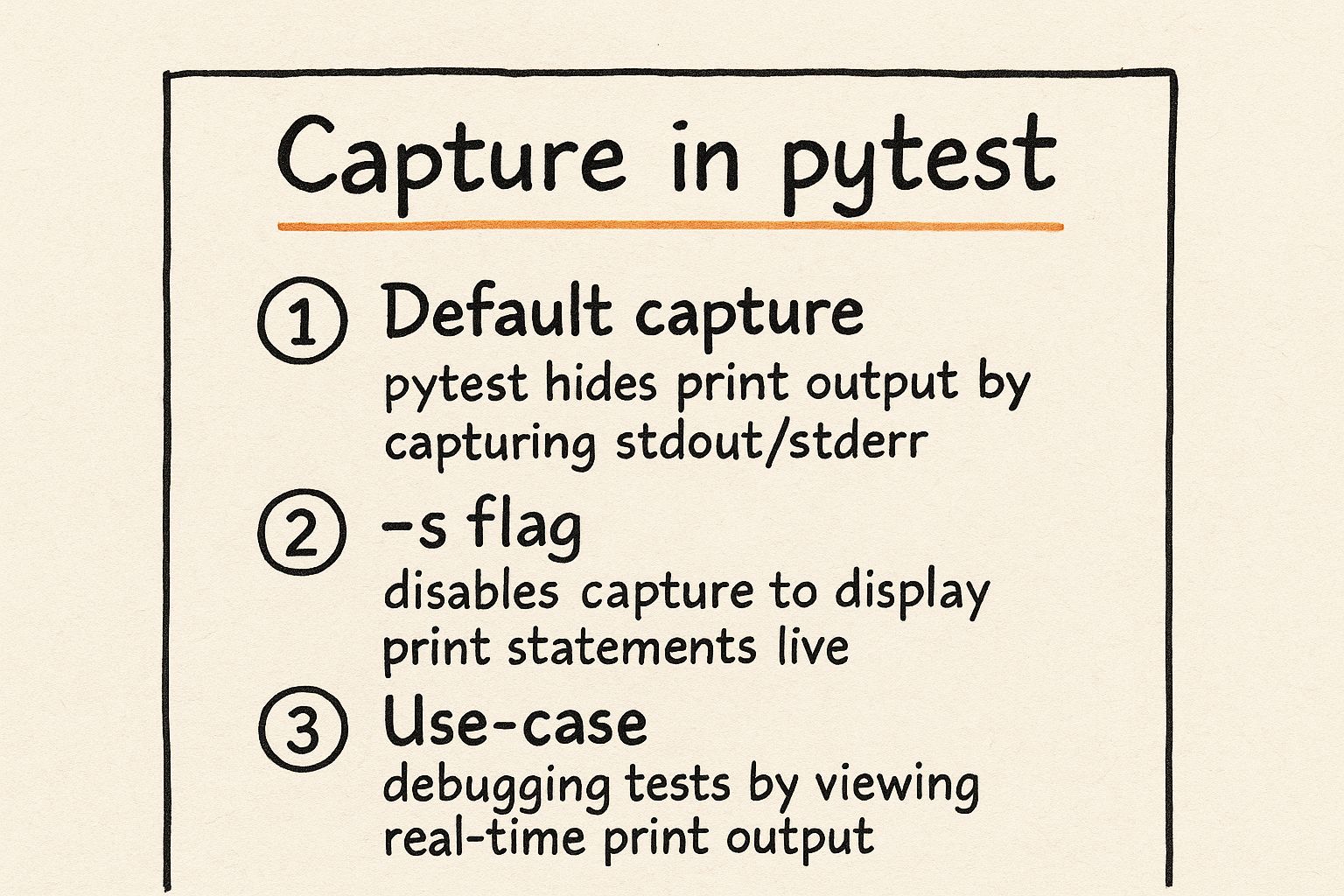
This image visually represents how -s
disrupts default output capturing, allowing print statements to appear during debugging. This allows developers to use print statements effectively for debugging without compromising clean test results under normal operation.
Combining Flags
For comprehensive debugging, combine -s
with verbosity flags. -s -v
shows all print statements along with detailed test information. -s -vv
shows print statements with the most detailed output. This combination gives a complete view of test execution and internal state. The best combination depends on your tests' complexity and the specific information you need. Experiment to find what works best for you. For more in-depth information, you can refer to the official pytest documentation. Effective use of print statements is a key part of debugging with pytest. Pytest offers flexibility and control over output verbosity with flags like -v
and -vv
, providing granular control over detail.
Mastering Fixtures for Strategic Output Control
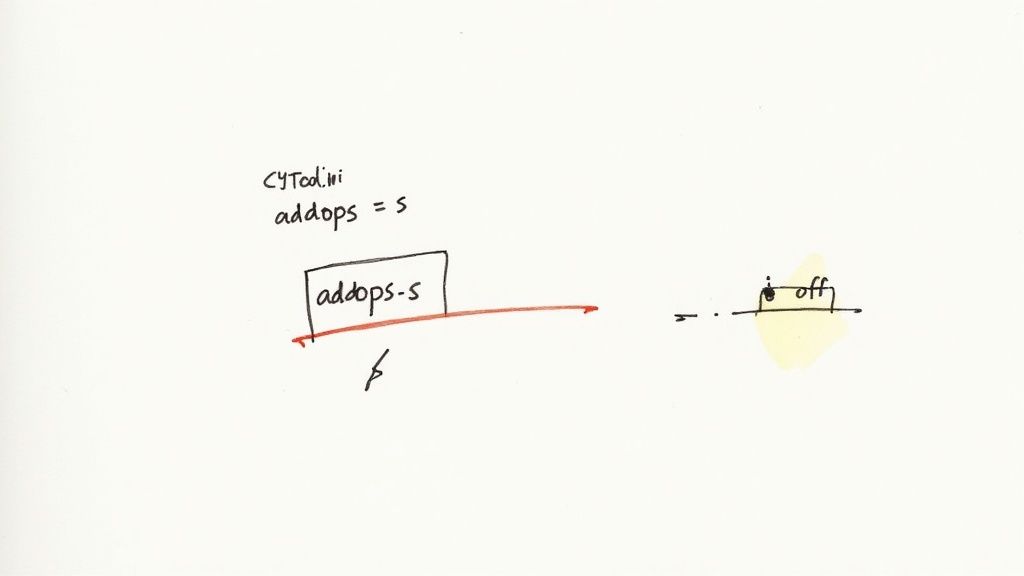
Pytest fixtures provide a robust mechanism for managing print statements during testing. This gives developers precise control over when and where these statements appear, balancing clean test reports with access to crucial debugging information. Experienced Python developers frequently leverage fixtures to refine their debugging workflows.
Creating a Fixture for Print Statement Control
A pytest fixture can be designed to temporarily adjust how pytest captures output. This allows you to selectively enable print statements for specific tests or even entire modules, while maintaining clean output for the rest of your test suite. This targeted approach is particularly helpful when debugging complex scenarios.
Here's how to implement a fixture for displaying print statements:
import pytest
@pytest.fixture def show_prints(capsys): print("This print statement will be shown") yield # The test using the fixture will execute here captured = capsys.readouterr() # Process the captured output if necessary print(f"Captured Output:\n{captured.out}") # print captured output after fixture scope
def test_one(show_prints): print("Print statement inside test_one") assert 1 == 1
def test_two(): print("Print statement inside test_two (will not show without the fixture)") assert 2 == 2
In this example, test_one
utilizes the show_prints
fixture, making its print statements (and those within the fixture itself) visible. test_two
does not use the fixture, so its print statement is captured by pytest's standard behavior.
Asserting Against Print Statements
Fixtures also enable capturing and asserting against print output. This lets you verify not only the results of your code, but also the information it outputs during execution, which is especially beneficial for testing logging or error messages. This is often accomplished with tools like capsys. To ensure your testing practices remain efficient, consider optimizing your workflow, perhaps by implementing strategies for effective communication, such as those discussed in Effective Standup Meetings.
Integrating with CI/CD
Fixture-based print control integrates smoothly with CI/CD pipelines. You retain clean output for CI runs by default but can activate the fixture when needed for debugging or troubleshooting.
Fixture Parameterization
Fixtures can be parameterized to offer various output control strategies depending on the test context. You might, for example, create separate fixtures for verbose logging, debugging particular modules, or integrating with external monitoring systems. This fine-grained control over print statement visibility significantly enhances your debugging workflow and contributes to a more effective testing process. This modular approach keeps tests clean and addresses varying levels of detail required during development and integration testing.
Leveraging Pytest-Print for Enhanced Debug Insights
As your test suites expand, effectively managing print statements becomes essential for efficient debugging. While the core pytest framework offers some output control, the pytest-print plugin provides a more structured and insightful approach. This plugin transforms standard print debugging into an organized system seamlessly integrated with pytest. It's gaining popularity among Python teams who recognize the value of turning print statements into actionable debug information.
Timestamped Output and Hierarchical Debug Information
A key feature of pytest-print is its generation of timestamped output. This allows you to precisely track the execution flow of your tests. This makes pinpointing errors or unexpected behavior much simpler, especially in complex, lengthy tests. Furthermore, pytest-print provides hierarchical debug information that reflects the structure of your tests.
This structured logging significantly simplifies interpreting debug output, particularly when dealing with multiple layers of functions and test cases. Imagine debugging a test that spans multiple classes and methods. Pytest-print structures the output to clearly associate each print statement with its position in the test hierarchy, making it easier to trace the execution path and identify the root cause of a problem.
Color-Coded Logs and Documentation of Complex Test Steps
Pytest-print also offers color-coded logs, making crucial information instantly recognizable within large volumes of output. Assigning different colors to various log levels (like warnings, errors, or info) lets you quickly focus on the most relevant information. The growth of pytest and related tools, like pytest-print, indicates a trend toward more advanced testing practices.
Beyond immediate debugging benefits, pytest-print helps document complex test steps. By including descriptive print statements in your tests, you automatically generate a detailed execution log. This log serves as live documentation for your test suite, beneficial for onboarding new team members, understanding test case rationale, or creating audit trails.
Communicating Test Progress and Creating Audit Trails
For long-running test suites, pytest-print facilitates communication about test progress. Real-time updates through structured print statements provide visibility into the current execution stage. This transparency is especially valuable for stakeholders who may not have direct access to detailed test output. The increasing popularity of pytest-print underscores its ability to effectively handle print statements. You can find more detailed statistics here.
Many development teams also use pytest-print to create audit trails. Detailed logs of test execution are invaluable for tracking changes, identifying regressions, and demonstrating compliance with regulatory requirements. Mastering pytest-print transforms print debugging from an ad-hoc practice into a powerful tool for gaining deep insights into test execution and improving the overall quality of your Python code.
Creating Consistent Output With Pytest.ini Configuration
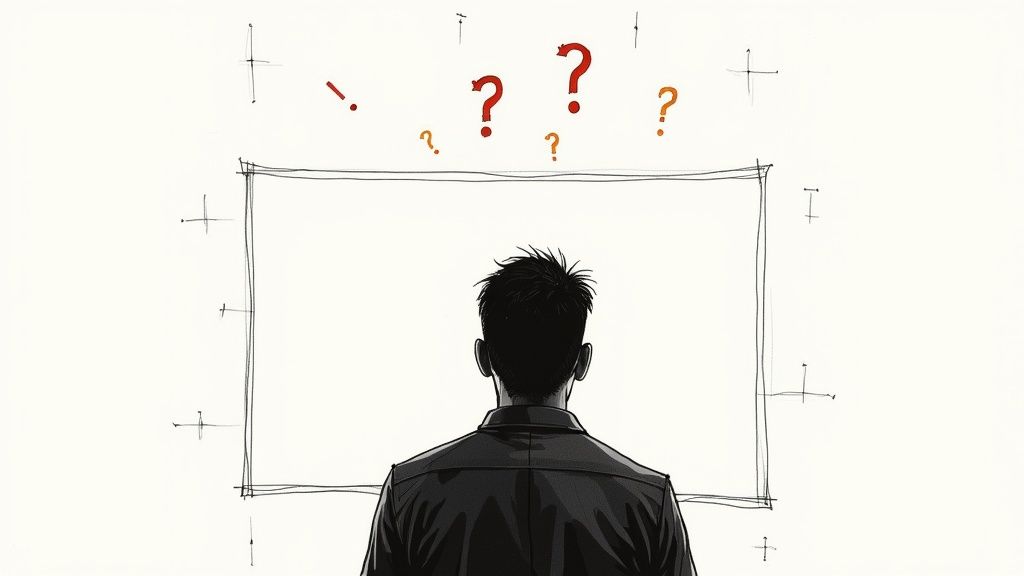
Consistent output is vital for effective teamwork and reliable continuous integration. The pytest.ini
file provides a central point for managing how print statements and other test outputs behave across your project. This avoids repeatedly specifying command-line options and ensures a standardized debugging experience for the whole team.
Setting Global Output Options
The pytest.ini
file lets you set default behaviors for your Pytest runs. You can configure options controlling print statement visibility, log file creation, and more. This is particularly useful for setting team-wide standards.
For example, you can ensure everyone sees print statements during local testing by setting capture=no
in pytest.ini
. This means no one has to remember the -s
command-line flag. This streamlined approach simplifies the testing process and minimizes potential confusion.
Creating Output Profiles for Different Environments
A key advantage of pytest.ini
is the ability to tailor output for different environments. You might want detailed debugging information locally, but a concise output for your CI environment. pytest.ini
makes this easy.
You can create sections within the file for specific environments.
[pytest] addopts = -ra -v
[pytest:ci] addopts = -ra --capture=no
This example uses the verbose option -v
and a report on all tests -ra
for regular runs, while disabling output capturing (--capture=no
) for CI runs, demonstrating the flexibility of pytest.ini
.
Routing Print Statements to Log Files
pytest.ini
also allows routing print statements and other test output to log files. This keeps detailed debug information available without cluttering the console. This is especially helpful for tracking intermittent bugs or analyzing test failures.
You can configure log file locations, formats, and verbosity levels within pytest.ini
for a flexible logging strategy. This allows for a high degree of customization, ensuring the log files contain precisely the information needed.
The following table details the common configuration parameters within pytest.ini
related to output and logging.
Pytest.ini Configuration Options for Output Control
Common pytest.ini settings that affect print statement visibility and overall test output
Configuration Parameter | Default Value | Purpose | Example Setting |
---|---|---|---|
log_cli |
false |
Enables logging to the console | log_cli=true |
log_cli_level |
INFO |
Sets the logging level for the console | log_cli_level=DEBUG |
log_cli_format |
Specific format string | Defines the format of console log messages | log_cli_format=%(asctime)s %(levelname)s %(message)s |
log_file |
None | Specifies the path to a log file | log_file=pytest.log |
log_file_level |
INFO |
Sets the logging level for the file | log_file_level=DEBUG |
log_file_format |
Specific format string | Defines the format of file log messages | log_file_format=%(asctime)s %(levelname)s %(message)s |
This table provides a quick reference for customizing the output and logging behavior of Pytest, offering a wide range of configuration options.
By properly configuring pytest.ini
, teams can build a streamlined and efficient workflow for managing print statements and all test output. This centralized approach improves collaboration, simplifies debugging, and promotes consistent output across all environments. Integration with CI tools like Mergify ensures consistent reporting during automated builds and merges. This is particularly helpful for Mergify users needing clear insight into test output during complex merge scenarios.
Strategic Print Debugging: When, Where, and How
Effective debugging is crucial for any Python developer. Print statements remain a valuable tool, especially when used strategically within the pytest framework. This section explores maximizing the impact of print debugging while keeping test suites clean and efficient.
Crafting Effective Print Statements
Print statements should be concise yet informative. They should act like targeted messages within your code, revealing its state. Instead of simply printing a variable's value, add context. For example: print(f"Variable 'x' in function 'calculate': {x}")
. This clarifies the value's location and meaning, making it easier to find problems in complex codebases.
Strategic Placement for Optimal Information Flow
Consider where print statements offer the most valuable insights. Placing them at critical junctures – before loops, after function calls, or within conditional branches – tracks execution flow and data transformations. Imagine a recursive function. Printing the input parameters at each call's start clarifies the recursion's progress. Strategic placement minimizes the number of print statements while maximizing information.
Formatting for Instant Recognition
Well-formatted print statements are vital in complex output. Visual cues, like separators, enhance readability. For example: print("------> Important Value:", value)
. This highlights essential data. Consider a helper function to format output consistently. This simplifies analysis, particularly across a team.
Print Statements as Execution Breadcrumbs
In complex scenarios, like multi-threaded applications, print statements can act as execution breadcrumbs. They trace execution paths and reveal potential concurrency or branching issues. Imagine a workflow across different modules. Print statements within each module's functions illuminate the exact path. This technique is especially helpful for timing bugs in asynchronous systems.
Troubleshooting Intermittent Failures and Integration Issues
Print statements excel at diagnosing intermittent errors, often linked to timing or environment. These are notoriously hard to reproduce with a debugger. Strategic print statements reveal the execution flow during these failures, helping identify the root cause. When debugging integrations, print statements on both sides of an interaction expose data exchange, often revealing the failure point. Capturing system state before and after a problematic function call isolates specific interactions that might lead to failure.
Evolving Beyond Print Statements
Mature test suites often transition toward structured logging and debuggers. However, print statements remain valuable for quick checks and initial investigations. A balanced debugging ecosystem utilizes each technique’s strengths: print statements for immediate feedback, logs for detailed analysis, and debuggers for complex logic. Print statements are quick and easy. Logging provides persistent records. Debuggers offer deep introspection, but can disrupt flow in complex systems. Combining these approaches optimizes debugging throughout the development lifecycle.
For a more streamlined CI/CD pipeline, consider Mergify. Mergify automates pull request workflows, including automatic updates and merge queue management. This frees developers to focus on writing and debugging code.