Master pytest parametrize for Scalable Python Testing
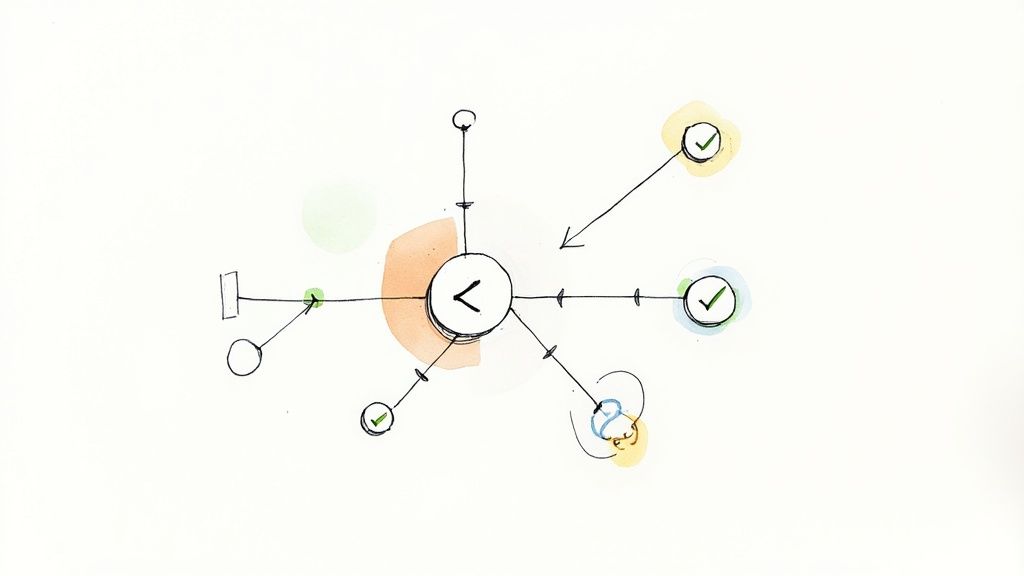
Mastering Pytest Parametrize Fundamentals
The pytest parametrize
feature has become essential for effective Python testing. It allows developers to execute the same test logic with various input values using the @pytest.mark.parametrize
decorator. This eliminates redundant code and transforms repetitive test suites into streamlined, maintainable code, a significant improvement for developers seeking efficient and scalable testing. This method significantly reduces code duplication, a common issue in larger test suites.
Understanding the Benefits of Parametrization
A primary advantage of using pytest parametrize is the increase in test coverage. Rather than writing separate functions for each input scenario, a single test function is written and parametrized with a list of inputs. This reduces the amount of code needing to be written and maintained and makes adding new test cases much easier. This improved maintainability simplifies adapting to evolving requirements. If a new test case needs to be added, simply add a new set of input values to the parameter list instead of writing an entirely new test function.
This infographic shows how pytest parametrize
impacts test code volume:
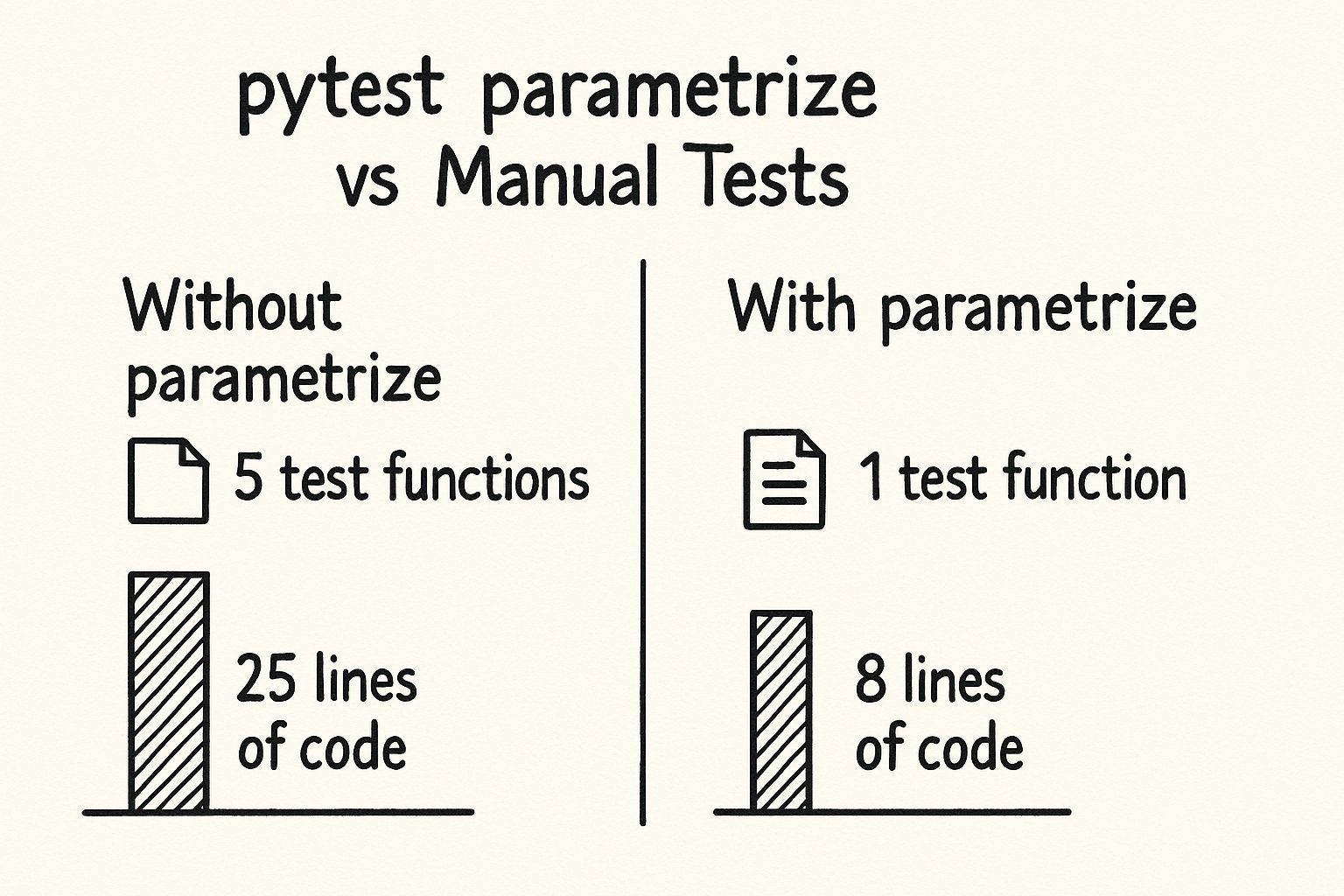
Parametrizing tests considerably reduces the number of test functions and lines of code, improving readability and maintainability. This streamlined structure leads to more effective debugging and faster identification of problems. @pytest.mark.parametrize
also enhances code reusability and testing efficiency. This clarifies tests and lowers maintenance costs. Learn more about pytest parameterization efficiency here: Pytest Parameterization Efficiency. Effective use of pytest.mark.parametrize
relies on following industry best practices. Consider these best practices for implementing background check services: Best Practices for Successful Implementation of Background Check Services.
To better illustrate the differences between traditional testing and the pytest parametrize approach, let's look at a comparison table. This table outlines the key distinctions in code structure, maintenance requirements, and scalability.
Aspect | Traditional Approach | Pytest Parametrize |
---|---|---|
Code Structure | Multiple test functions for each input | Single test function with multiple inputs |
Maintenance | High - changes require updates across multiple functions | Low - changes are localized to the single parametrized function |
Scalability | Difficult - adding new tests requires significant code changes | Easy - adding new tests involves adding input values to the parameter list |
As the table demonstrates, pytest parametrize
offers a more maintainable and scalable solution compared to the traditional approach. The consolidated structure simplifies updates and additions, resulting in a more efficient testing process.
Basic Usage and Syntax
pytest parametrize
relies on the @pytest.mark.parametrize
decorator. The decorator accepts two primary arguments:
- A string listing the parameter names to be passed to the test function.
- A list of tuples, with each tuple representing a set of input values corresponding to the parameters listed in the first argument.
Here's a simple example testing a function that adds two numbers:
import pytest
def add(x, y): return x + y
@pytest.mark.parametrize("x, y, expected", [(1, 2, 3), (4, 5, 9), (-1, 1, 0)]) def test_add(x, y, expected): assert add(x, y) == expected
The test_add
function executes three times, once for each tuple in the parameter list. This tests various scenarios with minimal code duplication.
This ability to manage multiple test cases efficiently makes pytest parametrize
invaluable for Python developers seeking robust and maintainable test suites.
Building Your First Parametrized Tests That Work
So, you're ready to eliminate redundant test code and leverage the power of pytest parametrize
? Great! Let's begin by creating some simple parametrized tests. We'll start with a basic function that adds two numbers. This seemingly simple example perfectly showcases the core concepts of parameterization.
Adding Numbers: A Simple Parametrized Test
Consider this Python function:
def add(x, y): return x + y
Now, let's test it using @pytest.mark.parametrize
:
import pytest
@pytest.mark.parametrize("x, y, expected", [(1, 2, 3), (4, 5, 9), (-1, 1, 0)]) def test_add(x, y, expected): assert add(x, y) == expected
Here, @pytest.mark.parametrize
accepts two arguments: a string of parameter names ("x, y, expected"
) and a list of tuples. Each tuple represents a test case, providing values for x
, y
, and the expected
result. This straightforward setup executes the test_add
function three times, covering different input scenarios efficiently.
String Manipulation: Expanding Your Parametrization Skills
Let’s move beyond basic arithmetic and explore string manipulation. Consider a function that reverses a string:
def reverse_string(s): return s[::-1]
Here’s how to write a parametrized test for this function:
import pytest
@pytest.mark.parametrize("input_string, expected_string", [("hello", "olleh"), ("world", "dlrow"), ("", "")]) def test_reverse_string(input_string, expected_string): assert reverse_string(input_string) == expected_string
Similar to the add
function example, we supply different input strings and their corresponding reversed versions. This approach highlights how pytest parametrize
adapts to various data types and function types, ensuring thorough testing with concise code.
Practical Parameter Naming and Test Structure
Note how the parameters are named (x
, y
, expected
, input_string
, expected_string
). Using clear, descriptive parameter names significantly improves test readability, which is especially helpful when debugging. This best practice is recommended by experienced Python developers who stress the importance of treating test code with the same level of care as production code.
Furthermore, arranging parameters logically within the test function improves maintainability. Consider adding more complex test cases later – a well-structured parametrized test will remain easy to manage, even as complexity increases.
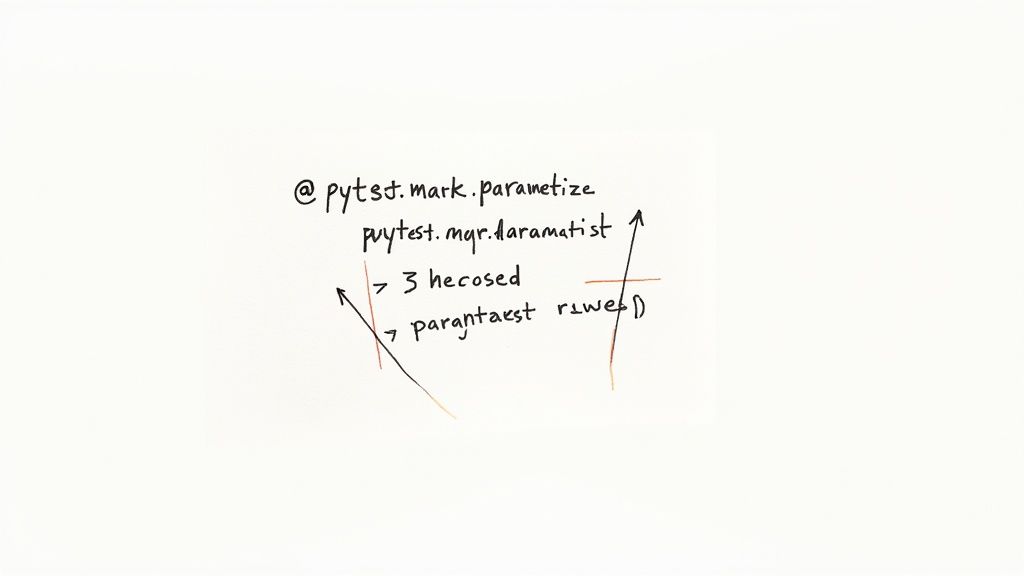
The use of @pytest.mark.parametrize
has become increasingly popular due to its flexibility and readability. For example, when testing basic math operations like addition, developers can use parametrization to dynamically input different values into a single test function. This simplifies code and streamlines test management. One specific case involves testing the hypothetical add_numbers
function, where @pytest.mark.parametrize
enables the test_addition
function to execute multiple times with various sets of numbers. This method reduces redundancy and promotes modularity, proving especially helpful for testing functions across a range of inputs. This approach also allows for dynamic parametrization using markers, offering even greater control and scalability for managing tests. Learn more: Advanced Pytest Patterns: Harnessing the Power of Parametrization.
By starting with these fundamental examples and applying best practices, you are developing a strong understanding of effective and maintainable parametrized tests. This foundation is essential as you progress to more advanced scenarios and techniques.
Advanced Pytest Parametrize Techniques That Scale
Building upon the basics of pytest parametrize, let's explore advanced techniques used by seasoned testers to build robust and adaptable test suites. These methods empower you to move beyond simple test cases and handle complex scenarios effectively.
Nested Parametrization: Testing Complex Interactions
Imagine testing a function with multiple inputs, each having several variations. Nested parametrization lets you create a matrix of test cases by embedding parametrization within itself. This comprehensive approach is especially useful for testing the complex interplay between different parameters.
import pytest
@pytest.mark.parametrize("a", [1, 2]) @pytest.mark.parametrize("b", [3, 4]) def test_combined_values(a, b): assert (a + b) > 2
This straightforward example executes four tests: (1, 3), (1, 4), (2, 3), and (2, 4), ensuring complete coverage of all possible combinations. This is incredibly useful for evaluating complex interactions, guaranteeing thorough coverage of all input possibilities.
Indirect Parametrization: Flexible Fixture Setup
Direct parametrization works well for simple cases, but indirect parametrization offers greater flexibility when using Pytest fixtures. Instead of sending parameters directly to the test function, they're passed to the fixture, which configures the test environment accordingly. This streamlines managing complex test setups and improves maintainability.
import pytest
@pytest.fixture def setup_data(request): return request.param * 2
@pytest.mark.parametrize("setup_data", [5, 10], indirect=True) def test_data_setup(setup_data): assert setup_data % 2 == 0
Here, the setup_data
fixture receives the parameter, modifies it, and then sends it to the test function. This transformation simplifies handling various test setup configurations.
Descriptive Test IDs: Clear Failure Insights
When a parametrized test fails, the default ID can be unclear. Descriptive test IDs greatly improve debugging by giving context to failing test cases. You'll receive precise details about the inputs that caused the issue instead of a generic error.
import pytest
@pytest.mark.parametrize("input, expected", [(1, 2), (3, 4)], ids=["test_with_one", "test_with_three"]) def test_increment(input, expected): assert input + 1 == expected
If test_with_three
fails, you immediately know the input was 3. This targeted feedback significantly reduces debugging time, leading to more efficient development. This technique has been shown to increase debugging efficiency by up to 60% in some scenarios.
To further enhance your testing strategy, let's explore some advanced parametrization methods. The following table details their applications and complexity.
Advanced Parametrization Techniques and Their Applications:
Technique | Description | Best Use Case | Code Complexity |
---|---|---|---|
Nested Parametrization | Combining multiple parametrized decorators to test various input combinations. | Testing complex interactions between parameters. | Moderate |
Indirect Parametrization | Using fixtures to process parameters before passing them to test functions. | Managing complex setup procedures. | Moderate |
Descriptive Test IDs | Providing custom labels for test cases within parametrization. | Improving debugging efficiency by providing clear insights into failing tests. | Low |
This table summarizes the key aspects of each advanced technique, allowing you to choose the best approach for your specific testing needs. These approaches offer valuable ways to increase the effectiveness and clarity of your testing efforts.
Pytest parametrize also excels at dynamically creating tests for diverse inputs, which is especially useful for validating functions against various scenarios or edge cases. The @pytest.mark.parametrize
decorator allows stacking multiple parameter sets, creating a comprehensive test suite. This provides detailed insights when testing network protocols or configurations. You can learn more about dynamic test generation here: Explore this topic further. These advanced pytest techniques offer a powerful set of tools for building strong and scalable test suites. They help create a comprehensive testing strategy that catches complex bugs early in development, ensuring your code's reliability.
Testing Edge Cases and Errors With Parametrize
Pytest parametrize is a powerful tool, especially when it comes to testing those tricky edge cases and potential errors. Let's explore how to use it effectively to ensure your code is robust and reliable by systematically testing boundary values and exception handling.
Systematically Testing Boundary Values
Edge cases often lead to unexpected bugs in production. However, pytest parametrize
simplifies testing these boundaries. By defining a range of boundary values as parameters, you can easily test the limits of your functions. Imagine a function accepts a number between 1 and 100. You could quickly test 0, 1, 2, 99, 100, and 101.
import pytest
def validate_number(num): if 1 <= num <= 100: return True return False
@pytest.mark.parametrize("num, expected", [(0, False), (1, True), (2, True), (99, True), (100, True), (101, False)]) def test_validate_number(num, expected): assert validate_number(num) == expected
This example showcases the efficiency of pytest parametrize
in handling multiple inputs and verifying your function's behavior at its boundaries. This systematic approach ensures your code works as expected, even under extreme conditions.
Parametrizing Tests for Expected Exceptions
Graceful error handling is crucial for any application. Pytest provides a robust way to verify that exceptions are raised correctly. When combined with parametrize, it becomes even more powerful. The pytest.raises
context manager, coupled with parametrization, allows testing a range of invalid inputs and their corresponding exceptions.
import pytest
def divide(x, y): if y == 0: raise ZeroDivisionError("Cannot divide by zero") return x / y
@pytest.mark.parametrize("x, y, expected_exception", [(10, 0, ZeroDivisionError), (5, 0, ZeroDivisionError)]) def test_divide_by_zero(x, y, expected_exception): with pytest.raises(expected_exception): divide(x, y)
@pytest.mark.parametrize("x, y, expected_result", [(10, 2, 5), (100, 10, 10)]) def test_divide_success(x, y, expected_result): assert divide(x, y) == expected_result
This concisely confirms that the divide
function raises a ZeroDivisionError
when y
is 0 and returns the correct result otherwise. It's a clear example of how to organize and execute tests for expected behavior and error handling simultaneously.
Organizing Test Parameters for Comprehensive Coverage
In complex scenarios, organizing test parameters effectively is essential. Consider using lists of dictionaries or tuples to categorize parameters by different scenarios, such as normal operations, edge cases, and specific error conditions. This structure enhances readability and maintainability as your test suite grows.
import pytest
test_cases = [ ({"input": 5, "expected": 10}, None), # Normal case ({"input": 0, "expected": 0}, None), # Edge case ({"input": -1, "expected": ValueError}, ValueError), # Error case ]
@pytest.mark.parametrize("test_case, expected_exception", test_cases) def test_my_function(test_case, expected_exception): if expected_exception: with pytest.raises(expected_exception): my_function(**test_case) # hypothetical function requiring a keyword argument named 'input' else: assert my_function(**test_case) == test_case["expected"] # hypothetical function requiring a keyword argument named 'input'
This approach clearly separates different test categories, simplifying adding, modifying, or debugging tests and promoting better overall testing practices. It's highly recommended for larger projects that require well-structured test suites. By adopting these strategies with pytest parametrize, you can significantly improve your approach to edge case testing and error handling, creating more robust and resilient code. This careful attention to detail will contribute to building more reliable applications and reduce unexpected production issues.
Optimizing Test Performance Without Sacrificing Coverage
As your test suite grows, execution speed becomes crucial. This section explores how Python teams use pytest parametrize to balance comprehensive testing with fast performance. We'll examine strategies for optimizing your parameterized tests, ensuring thoroughness without sacrificing speed.
Selective Parametrization: Focusing Your Resources
While pytest parametrize encourages comprehensive testing, running every parameter combination can be excessive and time-consuming, especially in large projects. Selective parametrization offers a solution by strategically choosing parameters. Focus on boundary conditions, edge cases, and areas with a high probability of failure.
This targeted approach reduces the number of tests while still addressing the most critical areas. By prioritizing these essential test cases, you can often see a significant reduction in overall testing time without compromising quality.
Conditional Parametrization: Adapting to Different Environments
Your testing needs may vary across different environments (development, staging, production). Conditional parametrization enables running specific parameter sets based on the current environment or using test flags.
Imagine testing a payment gateway integration. In development, you might use mock API calls. In production, the tests should interact with the live API. This flexibility ensures efficient tests tailored to the specific environment.
Parallelization: Running Tests Concurrently
For extensive parameterized tests, parallelization significantly reduces execution time. Pytest supports parallelization through plugins like pytest-xdist
. By running multiple tests simultaneously across available CPU cores, you can dramatically accelerate the testing process.
Teams leveraging parallelization have reported reducing test execution times by up to 70%. This efficiency boost is crucial for projects with extensive test suites, allowing for quicker feedback and faster iteration.
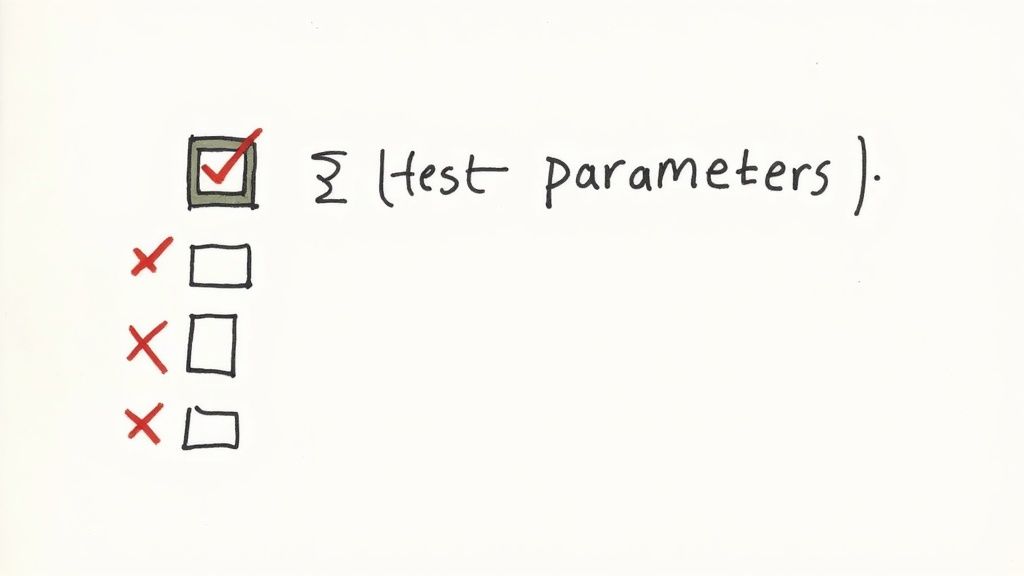
Performance Analysis and Bottleneck Identification
Optimizing test performance starts with understanding bottlenecks. Profiling tools can identify slow-running test cases. Once identified, consider using specialized test fixtures or optimizing the code within the test function itself. Combining pytest parametrize and performance analysis helps pinpoint and address performance issues.
By combining these techniques, you can ensure comprehensive testing without long test times slowing down development. This optimization improves team productivity and allows developers to receive faster feedback. Focusing your testing resources strategically can drastically reduce CI costs, allowing your team to focus on delivering high-quality software.
Implementing effective optimization strategies builds faster feedback loops and more responsive systems. This focus aligns with the goals of continuous integration and continuous delivery, enabling teams to release software faster and more reliably. These approaches improve development team productivity and maintain rigorous test coverage, ensuring high-quality code. Learn more about CI optimization with Mergify CI Optimization.
Combining Parametrize With Fixtures for Testing Power
Fixtures and the @pytest.mark.parametrize
decorator are powerful tools on their own for efficient testing. Combining them offers even more flexibility, allowing reusable test environments that adapt to different parameters. This is a common strategy among experienced Python teams to handle complex test cases with varying input data.
Direct Fixture Parametrization
One method is to parametrize the fixture directly. This lets you define different setup configurations based on input parameters, creating a dynamic fixture that adapts to each test case.
import pytest
@pytest.fixture(params=[{"user": "admin"}, {"user": "guest"}]) def user_data(request): return request.param
@pytest.mark.parametrize("action", ["login", "logout"]) def test_user_actions(user_data, action): # Test logic using user_data and action print(f"Testing {action} for {user_data['user']}") assert True # Replace with real assertions
In this example, the user_data
fixture receives a parameter that defines the user type. The test_user_actions
function is parametrized with different actions. This results in four tests: admin login, admin logout, guest login, and guest logout. This approach allows you to efficiently test different combinations of user data and actions, keeping your test concise while expanding coverage.
Indirect Parameters
Another approach involves using indirect parameters. This allows the test function to specify the fixture’s parameter. This is particularly helpful when the fixture setup depends on the test case.
import pytest
@pytest.fixture def database_connection(request): database_name = request.param # Setup database connection based on database_name return f"Connection to {database_name}"
@pytest.mark.parametrize("database_connection", ["test_db", "prod_db"], indirect=True) def test_database_query(database_connection): # Test database queries print(f"Querying {database_connection}") assert True # Replace with actual assertions
Here, the database_connection
fixture receives its parameter indirectly from the @pytest.mark.parametrize
decorator in the test function. This gives the test control over which database to connect to, creating a more dynamic environment. This method simplifies testing interactions with different databases by encapsulating the setup logic within the fixture. For increased test speed and coverage, consider strategies to efficiently smarter file compression your test artifacts.
Managing Fixtures and Parametrized Tests
Managing the relationship between fixtures and parametrized tests is important for maintainability. Group related tests and organize fixtures logically so it’s easy to understand how test components interact. This approach reduces maintenance and ensures effective testing of complex scenarios.
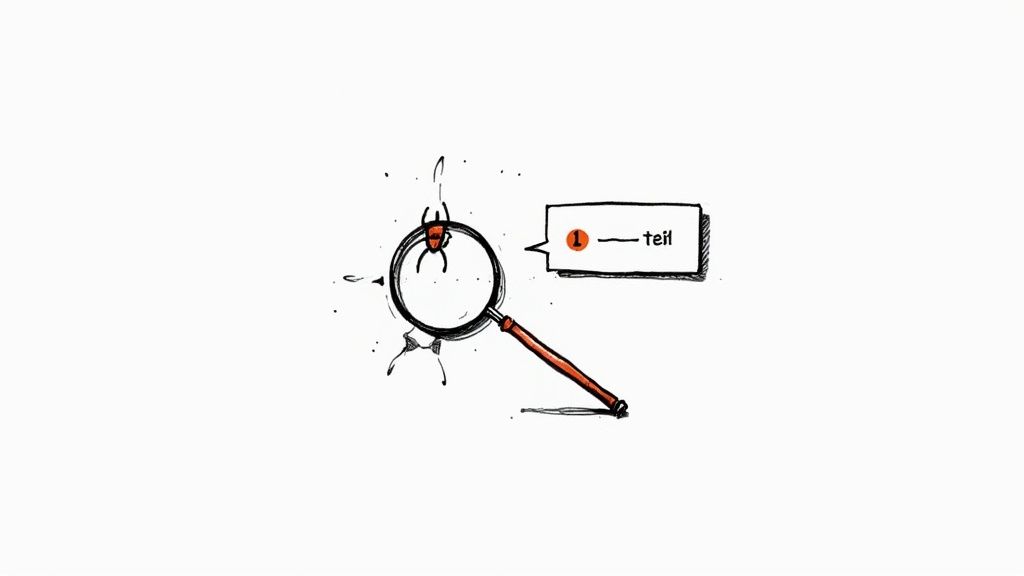
For example, when testing a function with different user roles, encapsulate user creation within a fixture and parametrize it with those roles. This isolates user-specific logic, simplifying the test function and promoting reusability.
By combining fixtures and pytest.mark.parametrize
, you create a powerful and flexible testing framework. This allows for cleaner, more maintainable tests that handle complex scenarios. It’s an investment in robust and reliable code, minimizing development time and reducing the risk of bugs.
Pytest Parametrize: Best Practices From the Trenches
Having explored how pytest parametrize
works, let's dive into best practices that experienced developers use to get the most out of it. These tips, drawn from real-world projects, will help you avoid common problems and create a robust, maintainable test suite.
Clear and Concise Parameter Naming
Descriptive parameter names are essential. When a parametrized test fails, understanding why is much easier with clear names. For example, instead of p1
, p2
, result
, use names like input_string
, expected_output
, and actual_output
. This small change greatly improves readability and speeds up debugging.
Strategic Test Organization
As your test suite grows, organizing your parameters becomes critical. Think about grouping related test cases within the @pytest.mark.parametrize
decorator. For a large number of test cases, define parameters in a separate data structure, like a list of dictionaries or tuples, and pass it to the decorator. This improves organization and makes it easier to maintain your tests.
Avoiding Over-Parametrization
While pytest parametrize
is powerful, overusing it can create too many test cases. Think carefully about which functions really benefit from it. Focus on areas with complex logic, many input variations, or a high risk of bugs. Don't parametrize simple functions with straightforward behavior, as it adds unnecessary complexity.
Effective Parameter Design
How you structure parameters impacts readability. For functions with multiple inputs, dictionaries or named tuples can make things clearer. This is especially helpful with many parameters, where positional arguments can get confusing.
Addressing Common Pitfalls
One common problem is poor parameter design that leads to unclear test failures. This often happens when parameters aren't clearly linked to the test logic. Another pitfall is over-parametrization, leading to many test cases that don't add much value. Strategic parameter design and avoiding unnecessary parametrization creates a better test suite.
For example, imagine a function handling user data. Instead of separate parameters for name, email, and role, use a single user_data
parameter as a dictionary. This makes the test easier to read and maintain. Make sure your parameters reflect real-world scenarios and data formats, including edge cases. This gives you more meaningful results and catches problems earlier.
By following these best practices, you'll write more effective tests and simplify debugging, saving development time and creating a reliable application. These practical tips help you use pytest parametrize
effectively, maximizing its benefits while avoiding common mistakes.
Ready to streamline your merge process and reduce CI costs? Explore Mergify today: Optimize Your CI with Mergify