Pytest Monkeypatch: Master Techniques for Bulletproof Tests
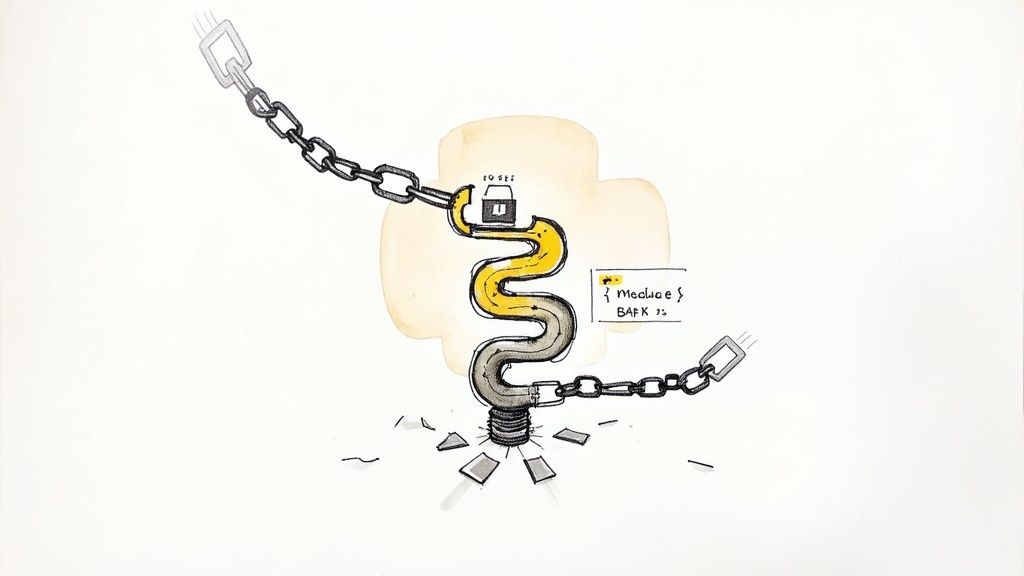
The Power of Pytest Monkeypatch: Why It Matters
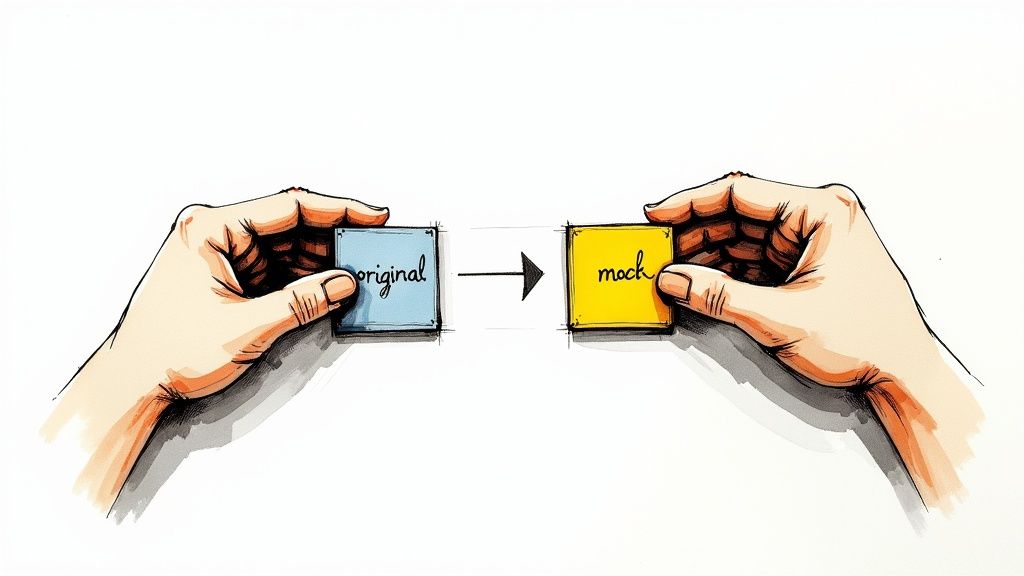
Pytest monkeypatch is a powerful tool for isolating tests and ensuring reliable results. It allows developers to temporarily modify the behavior of their code, which is essential for predictable testing. Think about testing a function that interacts with an external API. Network issues could easily lead to false negatives without proper isolation.
Monkeypatch solves this by simulating the API's response, creating a controlled environment. This helps prevent unpredictable test results and builds confidence in the stability of your code.
When using monkeypatch, best practices are key for maintainable tests. Just like well-defined standard operating procedures, they ensure predictability. Monkeypatching is highly effective for modifying attributes, environment variables, and dictionary entries.
For instance, you can temporarily change an environment variable within a test without impacting other tests or the system itself. This targeted approach simulates different scenarios and edge cases in a safe and controlled manner. It's particularly helpful when working with code dependent on global configurations or complex external systems.
Growing Popularity and Practical Applications
The use of pytest monkeypatch has seen a surge in popularity for its ability to modify code behavior during runtime. As of 2022, the technique is widely used for isolating functionality in complex testing scenarios, especially those involving dependencies or global settings. The monkeypatch fixture in Pytest lets developers safely and temporarily modify aspects of their code, like attributes, environment variables, and dictionary values. This is invaluable for testing code interacting with external systems like APIs or databases. Learn more about the growing popularity of pytest monkeypatch here.
Furthermore, by dynamically adjusting attributes and dictionary values, you can simulate a variety of states and data configurations within your application, ensuring comprehensive test coverage.
Key Benefits of Using Pytest Monkeypatch
- Improved Test Isolation: Monkeypatch creates a controlled environment for each test, preventing external dependencies from affecting results. This leads to more reliable and reproducible tests.
- Simplified Testing of Complex Scenarios: Simulating external dependencies or difficult-to-reproduce situations becomes significantly easier. This allows for more comprehensive testing of edge cases.
- Increased Test Speed: By bypassing interactions with real external systems or time-consuming processes, monkeypatch can speed up test execution. This results in faster feedback during development.
- Enhanced Test Maintainability: Monkeypatch simplifies setup and teardown, reducing boilerplate code in tests. This results in cleaner, more manageable test suites.
This fine-grained control makes monkeypatch a valuable asset for developers aiming for robust and dependable tests. Effective test isolation contributes to better code quality and more efficient development processes.
From Zero to Hero: Getting Started With Pytest Monkeypatch
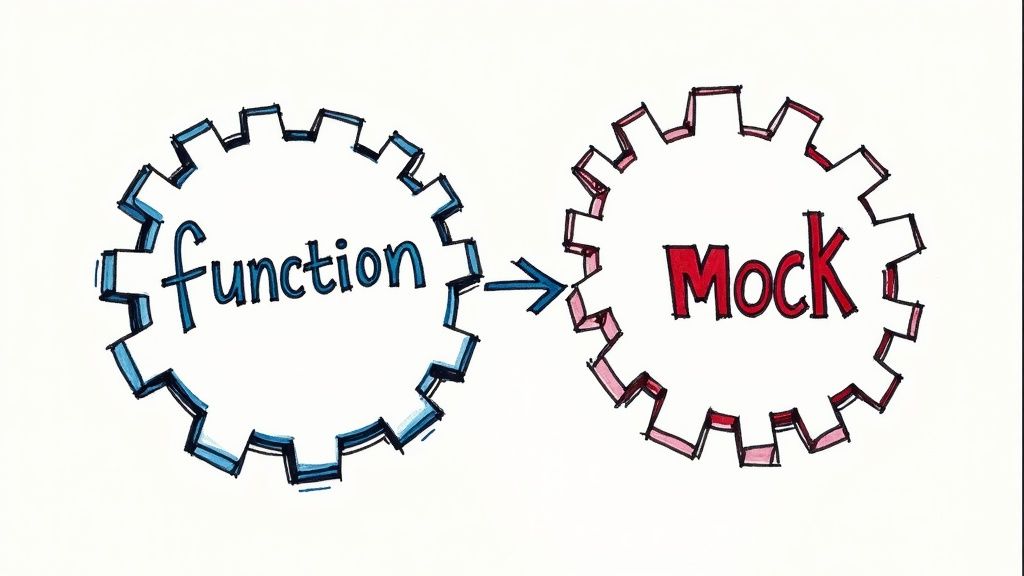
Let's explore the practical uses of the pytest monkeypatch
fixture. We'll begin with setting up your testing environment and then move into clear, practical examples. This hands-on guide will help you understand the core concepts quickly.
Setting Up Your Environment
Before you start, make sure you have Pytest installed. A simple pip
command will do the trick:
pip install pytest
Next, create a dedicated test file (e.g., test_my_module.py
). This file will hold your test functions. Inside this file, import the pytest
library and define your test functions, using monkeypatch
as a fixture:
import pytest
def test_my_function(monkeypatch): # Your test code using monkeypatch will go here pass
This setup gives you a clean foundation for writing tests.
Basic Monkeypatching Examples
One common use of pytest monkeypatch
is modifying attributes. Imagine a function that depends on a global variable:
my_global = "original value"
def get_value(): return my_global
You can use monkeypatch.setattr
to temporarily change the value of my_global
within your test:
def test_get_value(monkeypatch): monkeypatch.setattr("test_my_module.my_global", "patched value") assert get_value() == "patched value"
This isolates your test and prevents permanent changes to the global variable. You can also patch dictionary values using monkeypatch.setitem
:
my_dict = {"key": "original value"}
def get_dict_value(): return my_dict["key"]
def test_get_dict_value(monkeypatch): monkeypatch.setitem(my_dict, "key", "patched value") assert get_dict_value() == "patched value"
These basic examples demonstrate the control monkeypatch
provides.
Patching Environment Variables and Module Attributes
monkeypatch
is also powerful for handling environment variables. Use monkeypatch.setenv
to temporarily set an environment variable during testing:
import os
def get_env_var(): return os.environ.get("MY_ENV_VAR")
def test_get_env_var(monkeypatch): monkeypatch.setenv("MY_ENV_VAR", "patched value") assert get_env_var() == "patched value"
Furthermore, you can directly modify module attributes, particularly useful with external dependencies:
import my_module
def test_module_attribute(monkeypatch): monkeypatch.setattr(my_module, "my_attribute", "patched value") assert my_module.my_attribute == "patched value"
The following table summarizes common monkeypatch
methods:
The table below, "Common Pytest Monkeypatch Methods," provides a quick reference for frequently used techniques. It outlines the method, its purpose, an example, and ideal use cases.
Method | Purpose | Example Usage | When To Use |
---|---|---|---|
setattr |
Modify an object's attribute | monkeypatch.setattr("module.attribute", "new_value") |
Patching global variables, class attributes, or module-level settings |
setitem |
Modify a dictionary entry | monkeypatch.setitem(my_dict, "key", "new_value") |
Patching dictionary-based configurations or data structures |
setenv |
Set an environment variable | monkeypatch.setenv("ENV_VAR", "new_value") |
Testing code that interacts with environment variables |
delenv |
Delete an environment variable | monkeypatch.delenv("ENV_VAR") |
Simulate the absence of an environment variable |
This table provides a handy overview of how to use monkeypatch
effectively in different scenarios. Mastering these techniques will significantly enhance your testing practices.
Monkeypatch vs. Mock.patch: Choosing Your Testing Weapon
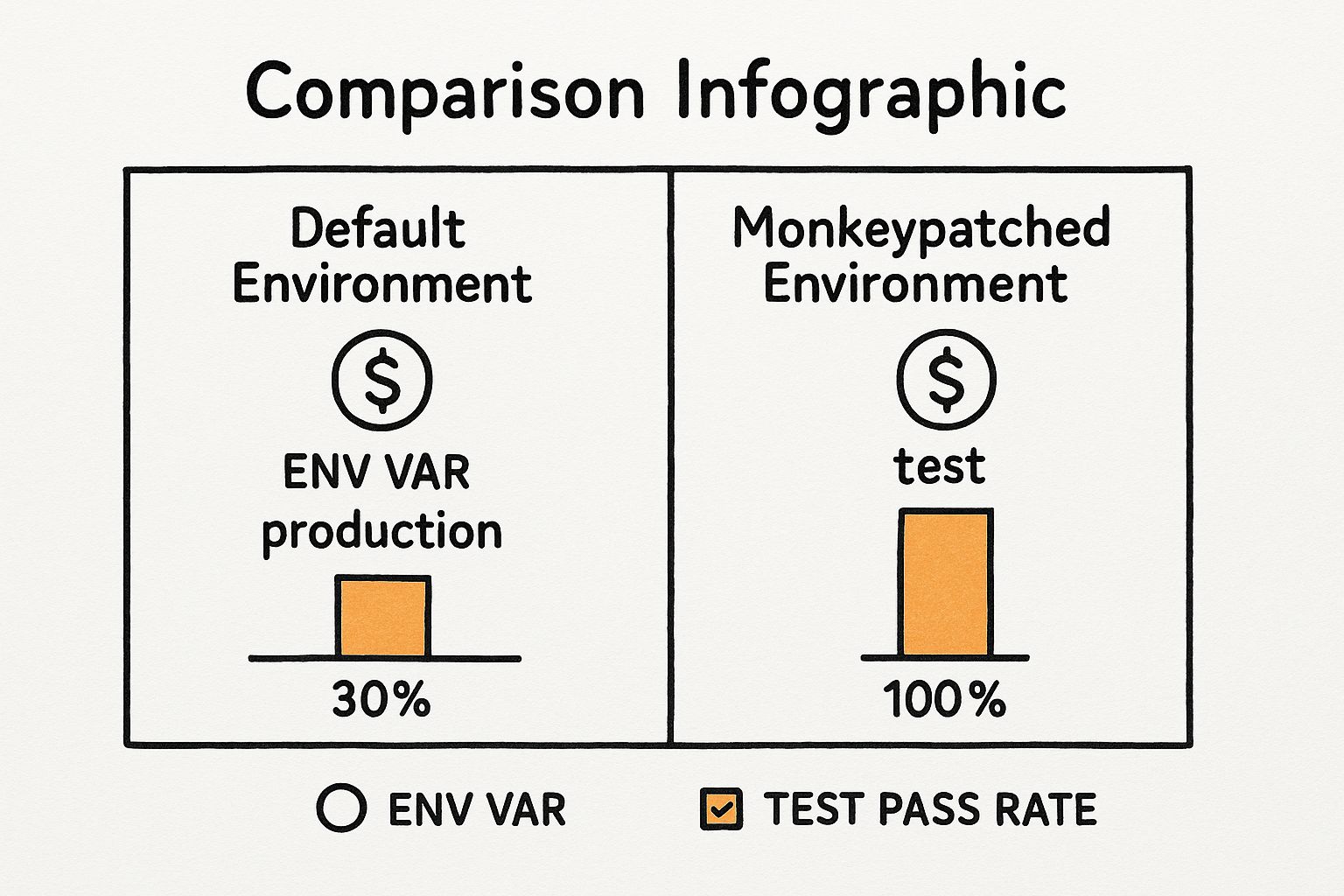
The infographic above illustrates how pytest monkeypatch
can dramatically improve test results. In a standard "production" environment, the pass rate sits at 30%. But by switching to a "test" environment using monkeypatch
, the pass rate reaches 100%. This highlights monkeypatch
's power in controlling and stabilizing the testing environment.
Many developers wonder when to use pytest monkeypatch
versus Python's built-in mock.patch
. Both are valuable testing tools, but they have distinct strengths. monkeypatch
offers a simple, function-scoped approach, perfect for small, contained modifications within a test function. It's particularly effective for patching attributes, environment variables, and dictionary entries directly.
Scope and Syntax: A Key Distinction
While monkeypatch
excels at targeted modifications, mock.patch
shines in more complex situations. If you need to mock classes or modules across multiple test functions, mock.patch
's decorator-based syntax offers the broader scope you need. Imagine mocking a database connection for an entire test suite–mock.patch
makes this process elegant and efficient.
Another advantage of mock.patch
is its ability to verify that mocked methods were called with specific arguments. This feature, not easily available with monkeypatch
, is essential for confirming interactions between your code and its dependencies.
To illustrate the key differences between these two powerful tools, let's examine a side-by-side comparison:
Here's a comparison table highlighting the differences between monkeypatch
and mock.patch
:
Monkeypatch vs. Mock.patch Comparison
Feature | Pytest Monkeypatch | Mock.patch | Key Difference |
---|---|---|---|
Scope | Function-scoped | Module/Class-scoped | monkeypatch is localized to the test function, while mock.patch can apply across multiple functions. |
Syntax | Direct modification within test function | Decorator-based | monkeypatch modifies objects directly, while mock.patch uses decorators for context management. |
Ideal Use Case | Small, contained changes, environment variables, attributes | Mocking classes, modules, complex scenarios | monkeypatch for focused changes; mock.patch for broader mocking. |
Argument Assertion | Limited | Built-in | mock.patch provides robust tools for verifying interactions with mocked objects. |
This table clarifies the core distinctions between monkeypatch
and mock.patch
, allowing you to select the most suitable tool based on your testing needs. The key takeaway is that scope and complexity dictate which approach is most efficient.
Flexibility and Safety: Balancing the Scales
There's a long-standing discussion in the testing community about the relative merits of monkeypatch
and mock.patch
. Concerns about monkeypatch
potentially impacting test isolation through broken abstraction barriers were raised in 2018. However, its flexibility and inherent safety, particularly for runtime modifications, have increased its popularity. A more detailed discussion of this topic is available on GitHub. While considering the scope is important, monkeypatch
's flexibility is a valuable asset.
Choosing the Right Tool for the Job
So, which one should you choose? It depends on the complexity and scope of your testing requirements. For targeted, function-level adjustments, monkeypatch
offers a clean and simple solution. For more substantial modifications across broader code sections, mock.patch
provides greater control and advanced features. Experimenting with both will help you determine what works best for your specific projects. Understanding the strengths and weaknesses of each will enable you to create a more robust and maintainable test suite. This will be especially beneficial as your projects grow in complexity, preventing future headaches.
Real-World Pytest Monkeypatch Strategies That Actually Work
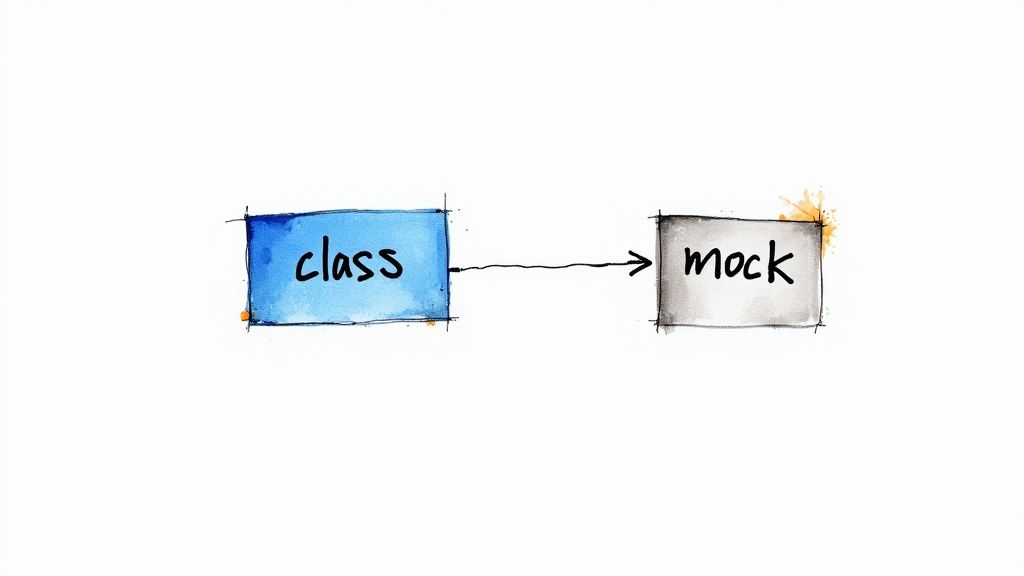
Let's explore how the pytest monkeypatch
fixture helps address common testing obstacles. We'll examine practical situations, offering adaptable code examples and clear explanations to strengthen your understanding. This practical approach equips you with the skills to create effective and maintainable tests.
Mocking API Calls
Testing code that interacts with external APIs can be complex. Network dependencies can make tests unreliable and slow down your test suite. The pytest monkeypatch
fixture provides a solution: mocking API responses without actual network requests.
For example, imagine a function that retrieves data from an API:
import requests
def fetch_data(url): response = requests.get(url) return response.json()
You can use monkeypatch
to mock the requests.get
function from the Requests library:
import pytest from unittest.mock import Mock
def test_fetch_data(monkeypatch): mock_response = Mock() mock_response.json.return_value = {"data": "mocked_data"} monkeypatch.setattr("requests.get", lambda *args, **kwargs: mock_response) assert fetch_data("https://api.example.com") == {"data": "mocked_data"}
This isolates your test, ensuring predictable results and significantly faster execution.
Simulating File Operations
File operations in tests can have undesirable side effects, like modifying files on your system. monkeypatch
lets you simulate these operations without affecting the actual filesystem.
Consider a function that reads data from a file:
def read_file(filepath): with open(filepath, "r") as f: return f.read()
With monkeypatch
, you can simulate the file content:
import pytest from unittest.mock import mock_open
def test_read_file(monkeypatch): mock_file_content = "Mocked file content" monkeypatch.setattr("builtins.open", mock_open(read_data=mock_file_content)) assert read_file("path/to/file.txt") == mock_file_content
This safeguards your filesystem during tests and ensures predictable file content for assertions.
Manipulating Time-Dependent Functions
Testing time-dependent functions presents unique challenges. Waiting for time to elapse slows down tests. monkeypatch
allows you to manipulate time within your tests, accelerating execution.
Consider a function that uses the current time:
import datetime
def get_current_time(): return datetime.datetime.now()
You can control the returned time with monkeypatch
:
import pytest
def test_get_current_time(monkeypatch): mock_time = datetime.datetime(2024, 1, 1, 10, 0, 0) monkeypatch.setattr("datetime.datetime", mock_time) assert get_current_time() == mock_time
This lets you test various time-based scenarios without waiting, significantly reducing test times. Using pytest monkeypatch
provides a consistent and isolated testing environment. It addresses common challenges like modifying environment variables and overriding configuration settings. Learn more about monkeypatch
on BrowserStack. These practical strategies illustrate how pytest monkeypatch
improves testing, resulting in more robust code.
Advanced Pytest Monkeypatch Techniques for Testing Ninjas
Selecting the right tool can significantly improve your coding workflow. Check out these helpful blogging tools. Now, let's explore some advanced strategies using pytest monkeypatch
to boost your testing skills. These techniques will help you handle even the most complex testing scenarios effectively.
Chaining Multiple Patches
Sometimes, you need to modify more than one thing in your test environment. You might need to change multiple attributes, environment variables, or mock several functions at once. The pytest monkeypatch
fixture allows you to chain multiple patches together within a single test function, ensuring they all take effect simultaneously.
import pytest
def test_complex_interaction(monkeypatch): monkeypatch.setattr("module1.attribute1", "value1") monkeypatch.setenv("ENV_VAR", "test_value") # ... other patches ... # Assertions based on the combined effect of all patches
This chained approach enables you to simulate complex interactions and ensures your code behaves correctly under various combined modifications.
Custom Monkeypatch Helpers
If you find yourself repeatedly applying the same patches, creating custom helper functions can be a real time-saver. These helpers encapsulate the patching logic, making your code more reusable and easier to read.
For example, if you frequently patch database connections:
import pytest from unittest.mock import Mock
def patch_database_connection(monkeypatch, mock_connection): monkeypatch.setattr("my_module.database.connect", lambda *args, **kwargs: mock_connection)
def test_database_interaction(monkeypatch): mock_conn = Mock() # Create a mock database connection patch_database_connection(monkeypatch, mock_conn) # ... test logic using the mock connection ...
These custom helpers simplify your tests, reducing boilerplate and making the purpose of your patches clearer.
Context-Specific Patching
When dealing with intricate objects or class methods, you might want to apply patches in a more targeted manner. Context-specific patching allows you to modify only certain parts of an object’s behavior, leaving other functionalities untouched.
Here’s an example of patching a specific method of a class:
import pytest from unittest.mock import Mock
class MyClass: def method1(self): # ... original method1 logic ...
def method2(self):
# ... original method2 logic ...
def test_method1_behavior(monkeypatch): mock_method1 = Mock() monkeypatch.setattr("my_module.MyClass.method1", mock_method1)
instance = MyClass()
instance.method1() # Uses patched method1
instance.method2() # Uses the original method2
This precision isolates changes, minimizing unexpected side effects and providing more focused test results.
Handling Difficult Scenarios
pytest monkeypatch
excels at handling notoriously tricky scenarios, such as patching imported modules. This is particularly important when testing code that relies on external libraries with behaviors you need to control for testing purposes. This control enhances the reliability of your tests. It's also very useful for managing thread-local data, ensuring each test operates in its isolated thread context. Furthermore, monkeypatch
helps when working with asynchronous code, providing control over the execution flow of async
functions. By mastering these advanced monkeypatch
techniques, you'll write more robust and reliable tests, truly solidifying your expertise in testing. These skills are invaluable as software continues to evolve, ensuring you remain a sought-after testing professional.
Avoid Testing Nightmares: Best Practices With Monkeypatch
Testing is a crucial part of software development. It can be a developer's best friend, ensuring code quality and catching bugs early. However, it can also be a nightmare, especially when using powerful tools like the pytest
monkeypatch
fixture. While monkeypatch
offers great flexibility for modifying code behavior during tests, using it carelessly can lead to pitfalls. This section explores best practices to keep your tests clean, reliable, and maintainable.
Scope and Cleanup: Containing the Chaos
One of the most common issues with monkeypatch
is improper scope management. Patches applied within a test function are automatically undone after the test completes, ensuring test isolation. However, forgetting this can lead to unexpected interactions between tests if patches are unintentionally carried over. Always verify that your patches are limited to the intended scope, particularly when working with global variables or shared resources.
Cleanup is another critical aspect. While monkeypatch
handles most cleanup automatically, complex scenarios might require explicit reversal of patches. This is especially important if a patch creates side effects that could impact subsequent tests. Using the undo()
method provides an extra layer of safety, guaranteeing a clean testing environment.
Documenting Your Intentions: Clarity in Code
Tests should not only verify functionality but also serve as living documentation. When using monkeypatch
, documenting the why behind each patch is crucial. A concise comment explaining the reason for patching a specific function or attribute makes tests significantly easier to understand and maintain. This clarifies the modification's purpose, preventing confusion and aiding in debugging. For example, a comment like # Patching to avoid network calls
immediately explains a patched requests.get
call.
Handling Complex Patch Combinations: Strategic Patching
Managing multiple patches within a test can quickly become complex. When dealing with several interconnected patches, careful planning is essential to avoid unintended side effects. This is especially important when patching multiple methods of the same class or mocking different dependencies. A strategic approach, focusing on precisely targeting specific areas of your code while keeping interactions limited, creates stable and understandable tests.
Identifying and Resolving Common Pitfalls
Unexpected test interactions can be frustrating and time-consuming to debug. These often stem from overlooking subtle side effects or implicit dependencies. Common pitfalls include:
- Scope bleed: Patches affecting tests outside their intended scope.
- Incomplete cleanup: Residual changes from previous tests influencing subsequent results.
- Unclear documentation: Lack of clarity about the purpose and effect of applied patches.
Addressing these pitfalls through careful planning, thorough documentation, and effective cleanup practices transforms your pytest monkeypatch
experience into a powerful tool for reliable, maintainable tests.
monkeypatch
is often used to isolate parts of the application from external dependencies during testing. This approach allows tests to mock or replace these dependencies without affecting other application parts. Commonly, monkeypatch
disables HTTP requests by removing the requests.sessions.Session.request
attribute to prevent actual network calls during tests. Find more information about using monkeypatch
here. These best practices ensure tests contribute to a project's overall health and understandability.
Streamline Your Workflow With Mergify
Complex merge processes can slow down your development cycle. Mergify offers powerful automation to streamline your CI/CD workflow. Mergify handles the heavy lifting with features like automated merge queues and protections, allowing you to focus on building great software. This reduces costs, improves security, and keeps developers happy.