Master pytest mock: Tips to Improve Your Python Tests
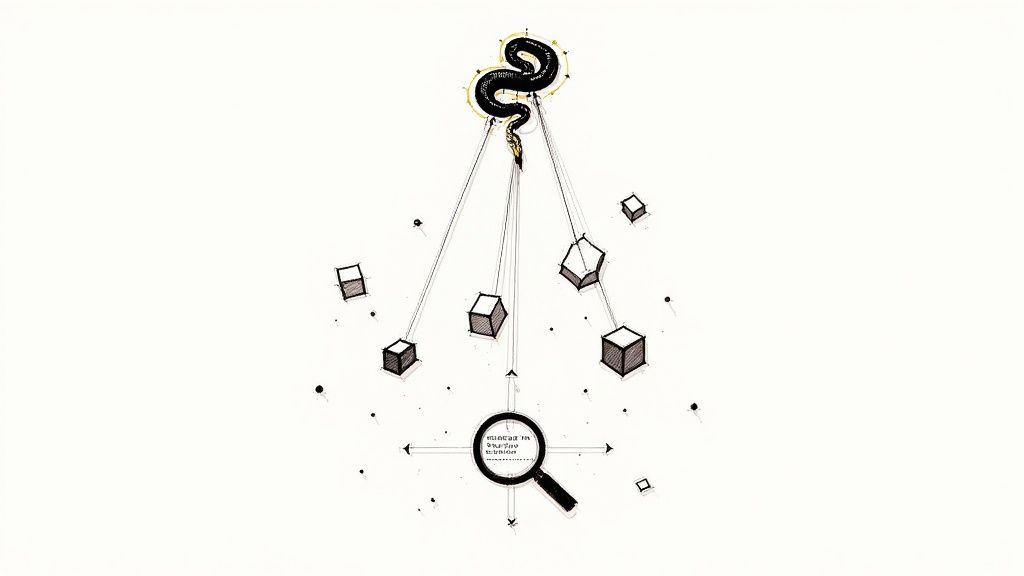
The Pytest Mock Advantage: Why Smart Developers Use It
Behind every dependable Python application lies a solid testing strategy. A core element of this strategy is effective dependency isolation, and that's where pytest mock excels. The pytest-mock
plugin offers a streamlined method for mocking objects and functions, simplifying isolated code testing. This allows developers to concentrate on their code's logic without the distractions of external systems or dependencies.
Isolating Dependencies for Predictable Tests
Think of your code as a car engine. You wouldn't test its performance while connected to the transmission, wheels, and the rest of the car. pytest-mock
helps "detach" code components for individual testing. This isolation is vital for predictable, repeatable tests. For instance, when testing a function that sends data to a database, pytest-mock
simulates the interaction without a real connection, preventing database-related test failures and speeding up the process.
Integrating Seamlessly With Pytest Fixtures
pytest-mock
integrates smoothly with pytest's robust fixture system. Fixtures preconfigure tests and enable reuse across multiple tests, simplifying complex testing scenarios. Imagine mocking multiple database calls within various test functions. With fixtures, define the mock behavior once and inject it into each relevant test, reducing code duplication and ensuring consistency.
Boosting Performance Through Mocking
Mocking's impact on test performance is often overlooked. Isolating dependencies significantly reduces the overhead of interacting with external resources, resulting in faster test execution, quicker feedback, and faster development cycles. The data chart below visualizes mocking's effect on execution time in various scenarios.
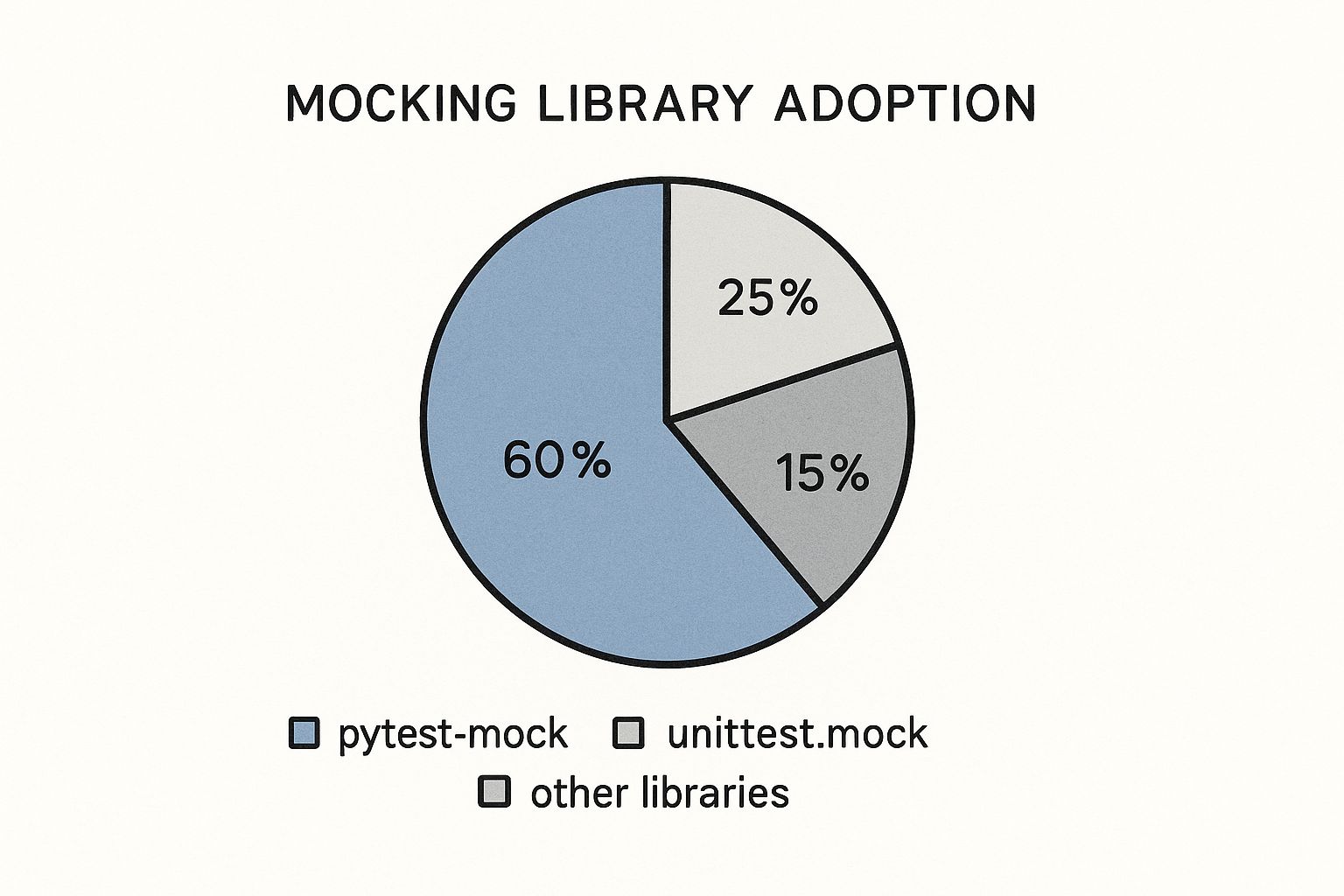
This data chart demonstrates the impact of using mocking on test execution times in several common situations including interacting with databases, external APIs, and file system operations. As you can see, mocking significantly speeds up tests, especially with resource-intensive operations like database interactions.
To further illustrate pytest-mock
's advantages, let's compare it to other popular Python mocking libraries. The following table highlights key features and differences.
Pytest Mock vs Other Mocking Libraries
Feature | pytest-mock | unittest.mock | mock | mockito |
---|---|---|---|---|
Fixture Integration | Yes | Yes | No | No |
Pytest Specific | Yes | No | No | No |
Ease of Use | High | Medium | Medium | High |
This table showcases how pytest-mock
integrates specifically with pytest and offers high ease of use compared to some other mocking libraries. Its tight integration with the pytest framework makes it a powerful tool.
The wide adoption of pytest and pytest-mock
speaks to their effectiveness. While specific adoption metrics for pytest's mocking are unavailable, pytest’s overall dominance in Python testing is clear. Over 80% of Python developers use pytest as of 2023, and pytest-mock
has over 50 million downloads from PyPI as of 2024, demonstrating widespread use of its patching features through monkeypatch
and mocker
fixtures. More detailed statistics can be found here: JetBrains Pytest vs. Unittest Blog Post. Mastering pytest mock is a valuable asset for any Python developer aiming for efficient, reliable tests.
Setting Up Pytest Mock Without the Headaches
Configuration issues can be a real pain when you're trying to get your testing up and running. This section offers a straightforward setup process for pytest-mock that works well for projects of all sizes. We'll share best practices from seasoned Python developers to help you smoothly integrate pytest-mock into your existing workflow.
Installation and Initial Configuration
The first step is making sure you have pytest-mock installed. Use pip for a quick installation:
pip install pytest-mock
This installs the plugin and the crucial mocker
fixture, which we'll dive into later. It's essential to verify the installation. A simple test function can confirm everything works:
import pytest
def test_mocker_installed(mocker): assert mocker is not None
Running pytest
should execute this test without a hitch, confirming pytest-mock is ready to go. For teams using Google Workspace, checking out their project management strategies can be a valuable resource: Google Workspace project management strategies.
Structuring Your Test Files for Maintainability
As your test suite expands, good organization is key. Keep your tests manageable by organizing them into separate files or directories dedicated to particular modules or features. This makes your code clearer and simplifies finding and updating tests.
- Create dedicated test directories (e.g.,
tests/
) - Group tests by module or feature
- Use clear, descriptive filenames (e.g.,
test_api_client.py
)
This structured approach, combined with consistent naming, will save you significant time and effort on debugging and maintenance as your project grows.
IDE Integrations for Increased Efficiency
Modern IDEs often have integrations that can supercharge your pytest-mock experience. Features like autocompletion, code navigation, and debugging tools can make your testing significantly more efficient.
- Configure your IDE to recognize
pytest
andpytest-mock
- Take advantage of autocompletion for mock methods and attributes
- Use debugging tools to step through tests and check how mocks behave
These integrations streamline testing, making it simpler to write, run, and debug.
Onboarding New Team Members
Bringing new developers onto your team smoothly involves clear documentation and consistent coding practices. Set guidelines for writing tests with pytest-mock, and provide examples and resources for common scenarios. This initial effort will help prevent inconsistencies and improve the overall quality of your tests, saving time in the long run. It also helps reduce the time spent answering common questions during onboarding.
By following these tips, your team can adopt pytest-mock effectively. This standardized approach leads to better code organization, maintainability, and long-term efficiency.
Pytest Mock Techniques That Actually Work
Before diving into the world of mocking, it's helpful to understand the context of your tests. For a good overview of testing web applications, check out this resource: Web Application Testing: An Introduction. This section explores practical pytest-mock
patterns for real-world scenarios. We'll cover how to effectively use the mocker
fixture and patch
decorators to create predictable mock objects, leading to robust and reliable tests.
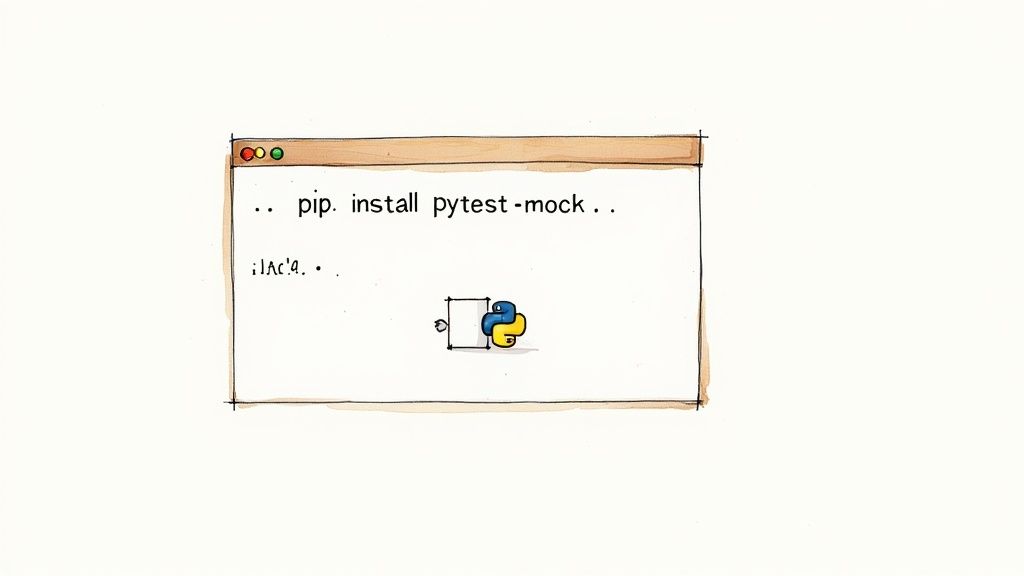
Mastering the Mocker Fixture
The mocker
fixture is your go-to tool for creating and managing mocks within your pytest tests. It offers a clean and intuitive interface for substituting real objects with controlled stand-ins. Think about testing a function that interacts with a database. With mocker
, you can simulate database responses without an actual connection.
This isolation provides two key benefits: faster tests and prevention of database-related errors.
- Use
mocker.patch
to replace functions, methods, or attributes. - Define return values, side effects, or exceptions for your mocks.
This controlled environment ensures predictable test outcomes. It also simplifies the process of simulating various scenarios. Furthermore, the mocker
fixture integrates seamlessly with pytest's fixture system, promoting reusability across multiple tests and reducing code duplication.
Strategic Use of Patch Decorators
While mocker
shines for creating mocks within test functions, patch
decorators offer a more declarative approach, especially when managing numerous dependencies. These decorators allow you to define mocks directly above your test function, making your substitutions explicit.
- Use
@patch
for simple mocks with preset return values. - Use
@patch.object
for more complex mocks targeting class methods or attributes.
This declarative style improves readability and clarifies your testing strategy. However, excessive use of patch
can sometimes hinder understanding. Strategic application is key. For intricate tests, a combined approach using both mocker
and patch
often provides the best balance of clarity and control.
Mocking Complex Interactions
Pytest mock goes beyond simple return values, enabling sophisticated behavior simulation. You can define sequences of return values, raise exceptions on specific calls, and even verify call arguments with assert_called_with
.
- Use
return_value=[value1, value2]
for sequential responses. - Use
side_effect=Exception("Error")
to simulate failures. - Use
assert_called_with(arg1, arg2)
to verify interactions.
These advanced techniques allow you to thoroughly test complex code paths, edge cases, and error handling. This becomes especially important when working with external services, databases, or other components with intricate interactions. Case studies in enterprise environments have shown that pytest's mocking capabilities can reduce test suite maintenance costs by 30-50%. A 2022 analysis revealed that pytest-mock
was used in 58% of Python projects employing advanced testing practices. This reflects the growing complexity of modern testing, evidenced by a 400% increase in mocking-related API references between 2018 and 2022. More detailed statistics can be found here: Pytest Documentation. By strategically utilizing pytest mock, you can build a more robust and maintainable test suite for your Python projects.
Monkeypatch vs. Mocker: Choosing Your Perfect Tool
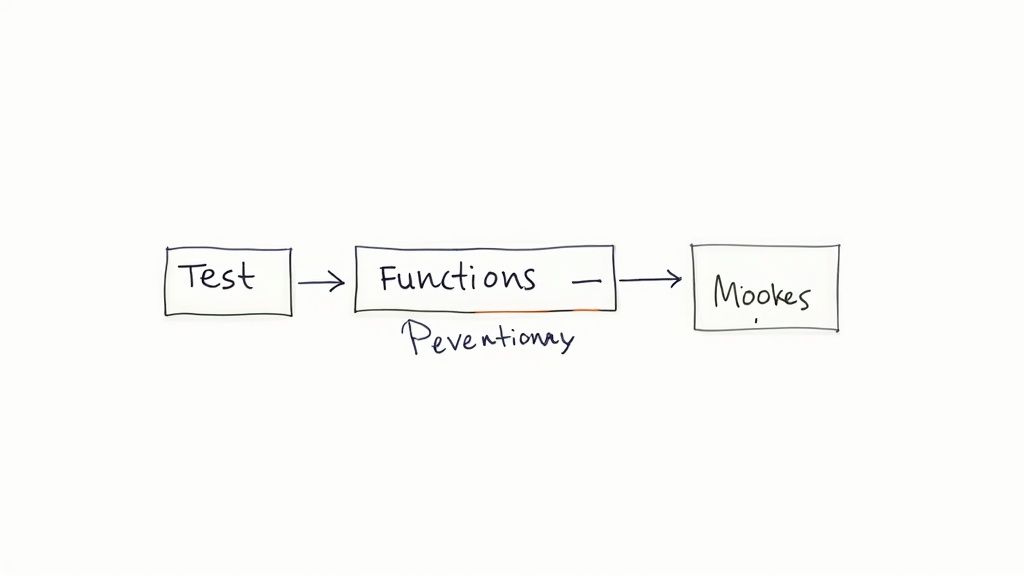
Pytest offers two powerful tools for mocking: monkeypatch
and mocker
. Both help isolate dependencies during testing, but they address different needs. Choosing the right tool is essential for efficient and effective pytest mock tests. Selecting the wrong one can lead to complex debugging and wasted time. This section clarifies the strengths and weaknesses of each, helping you decide which tool best fits your testing scenario.
Monkeypatch: Surgical Precision for Attributes and Environments
The monkeypatch
fixture is a powerful yet straightforward way to modify attributes, environment variables, and other system aspects during testing. Think of it as a surgeon's scalpel, enabling precise, targeted changes without the overhead of full mock objects. This makes it ideal for temporarily altering the behavior of existing functions or modules.
Some common use cases for monkeypatch
include:
- Setting environment variables specifically for a test
- Modifying dictionary entries or list items within a module
- Replacing a function's implementation with a simpler stub
For example, if your code depends on an environment variable, monkeypatch
lets you set its value only within the test's scope. This ensures clean isolation and avoids unintended side effects in other tests or your production environment. This targeted approach simplifies test setup and maintenance compared to creating full mock objects with mocker
.
Mocker: The Swiss Army Knife for Comprehensive Mocking
The mocker
fixture, provided by the pytest-mock plugin, offers more comprehensive mocking. It provides a broader range of features than monkeypatch
, allowing for the creation of complex mock objects and detailed behavior verification. This makes it the go-to choice for intricate interactions between your code and its dependencies.
mocker
excels in these situations:
- Creating mock objects with custom attributes and methods
- Defining return values, side effects, and exceptions
- Verifying call counts, arguments, and interaction order
This flexibility lets you meticulously simulate dependencies, set expectations for their usage, and ensure your code interacts with them correctly. For instance, if your code makes multiple calls to an external API, mocker
helps you create a mock that returns specific responses for each call, enabling thorough testing of various scenarios.
Choosing Your Tool: A Practical Decision Framework
Choosing between monkeypatch
and mocker
depends on your testing needs' complexity. The following table summarizes the key differences and helps you choose the right tool:
To help you make the best decision, we've compiled a comparison table:
Monkeypatch vs. Mocker Feature Comparison Detailed comparison of capabilities and use cases for pytest's two main mocking approaches
Feature | Monkeypatch | Mocker (pytest-mock) | Best For |
---|---|---|---|
Modifying environment variables | Yes | Yes | monkeypatch for simplicity |
Setting attributes | Yes | Yes | monkeypatch for direct modification |
Complex interaction verification | Limited | Yes | mocker for detailed assertions |
Stubbing out functions | Yes | Yes | monkeypatch for quick replacements |
Mocking entire objects | No | Yes | mocker for complex simulations |
This table highlights the core strengths of each approach. monkeypatch
shines in its simplicity for direct modifications, while mocker
provides the robust features needed for complex scenarios.
Sometimes, combining monkeypatch
and mocker
offers the most powerful approach. You might use monkeypatch
to set environment variables and mocker
to simulate database interactions within the same test. This combined approach empowers you to create truly robust and comprehensive test suites. Understanding each tool's strengths allows you to use pytest mock effectively to enhance your testing process.
Advanced Pytest Mock: Taming Complex Systems
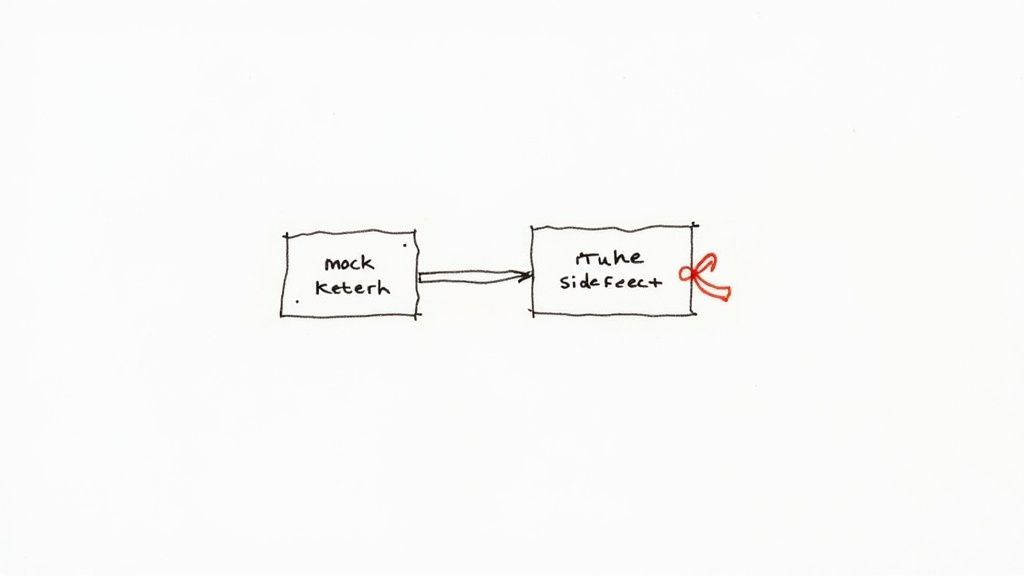
Testing individual functions is usually pretty straightforward. But when you're dealing with interconnected systems, things get more complicated. Think microservices, databases, third-party APIs, and asynchronous operations – these require a robust testing strategy. This section explores advanced pytest mock
techniques that experienced Python developers use to build reliable tests.
Mocking Entire Subsystems for Clarity
Imagine testing a service that interacts with a database. Instead of mocking each database call individually, you can mock the entire database interaction layer. This makes your tests easier to read and understand. You abstract away the low-level details and focus on the service's core behavior.
For example, if your service uses a DatabaseConnector
class, you can mock the entire class:
import pytest
@pytest.fixture def mock_database_connector(mocker): return mocker.patch('your_module.DatabaseConnector')
def test_my_service(mock_database_connector): # Configure the mock connector's behavior mock_database_connector.return_value.connect.return_value = True # ... your test logic ...
This approach simplifies tests and makes them less fragile when the database interaction implementation changes.
Reusable Mock Fixtures for Complex Dependencies
When you have many intricate dependencies, reusable mock fixtures become essential. They reduce code duplication and improve consistency across tests. Think of them as pre-built components you can easily slot into your tests.
Let's say multiple services use a third-party API client. You can create a fixture to mock this client:
import pytest
@pytest.fixture def mock_api_client(mocker): mock_client = mocker.patch('your_module.APIClient') mock_client.return_value.get_data.return_value = {'status': 'ok'} return mock_client
Now use the mock_api_client fixture in multiple tests
def test_service_1(mock_api_client): # ... test logic ...
def test_service_2(mock_api_client): # ... test logic ...
This ensures consistent mock behavior across all tests interacting with the API client.
Partial Mocking for Preserving Key Behaviors
Sometimes, fully mocking an object hides behavior you need to test. Partial mocking lets you mock specific methods while keeping the original implementation of others. This offers a balance between control and realism.
For example, you might want to test the error handling of a method that calls a logging function. Mock the logging function to control its output, but leave the rest of the method untouched. This isolates the error handling logic for testing.
Taming Asynchronous Workflows and Exceptions
Testing asynchronous operations with async
and await
requires special handling. Pytest-mock seamlessly supports mocking coroutines, making it easy to test these complex flows. You can also simulate exceptions to verify your system's resilience.
Building Realistic Test Doubles
Effective tests rely on realistic test doubles (mocks). This means understanding how your system interacts with its dependencies, including external services and databases. By carefully designing your mocks, you create a realistic test environment that uncovers potential problems. These advanced pytest mock
techniques help Python developers build robust and comprehensive tests, ultimately leading to higher quality code and more confident deployments.
Pytest Mock Pitfalls: What Experts Wish They'd Known
Even seasoned Python developers can sometimes trip up when using pytest-mock
. These pitfalls often lead to tests that are unreliable (flaky tests), give false positives, or become debugging nightmares. Based on conversations with testing experts, this post highlights common mistakes and offers practical solutions to improve your testing process.
Mocking the Wrong Thing: A Recipe for Flaky Tests
One common mistake is mocking the wrong part of your code. Imagine, for example, mocking an internal function instead of an external dependency. This can lead to tests that pass even when the external dependency would fail in a real-world scenario, creating a false sense of security. Key takeaway: Always mock at the system's boundary, isolating external dependencies, not internal functions.
Overly Complex Mocks: The Maintainability Nightmare
Creating overly complex mocks can make tests difficult to understand and maintain. If a mock requires a lot of setup or has many conditional behaviors, it becomes a burden rather than a helpful tool. Focus on simplicity: Mocks should be as straightforward as possible. If a mock becomes too complex, consider refactoring the code you're testing or extracting reusable mock fixtures.
Mocking Too Much or Too Little: Finding the Right Balance
Mocking too much leads to brittle tests that break easily with small code changes. Mocking too little, on the other hand, allows external factors to influence your tests, making them unreliable. Finding the right balance is essential. Strive for focused mocking: Mock only what's absolutely necessary to isolate the code under test. This improves test stability while ensuring important interactions are still verified.
Scope Problems With Fixtures: Unintended Consequences
Pytest fixtures are powerful for setting up mocks, but incorrect scoping can create unexpected issues. For instance, a fixture scoped to a module might unintentionally affect other tests in that module. Control fixture scope: Carefully consider the appropriate scope for each fixture. Prefer function-scoped fixtures when possible to minimize interactions between different tests.
Deciphering Pytest Error Messages: A Debugging Challenge
Pytest's error messages related to mocking can sometimes be difficult to understand. Deciphering these messages is crucial for efficient debugging. Tip: Carefully examine the traceback and inspect the mock objects to understand the problem. The pytest-mock
documentation is a valuable resource.
Preventing Test Interdependence: Avoiding Hidden Dependencies
Tests should be independent and able to run in any order. Mocking helps achieve this, but be mindful of shared mocks or state between tests. Isolate test setup: Ensure each test has its own isolated setup, including creating new mock objects for each test function.
Practical Solutions and Real-World Examples
The table below demonstrates common pytest-mock
pitfalls and their solutions with simple code examples:
Pitfall | Problematic Approach | Solution |
---|---|---|
Mocking the Wrong Thing | mocker.patch('my_module.internal_function') |
mocker.patch('my_module.external_dependency') |
Overly Complex Mock | Multiple return_value and side_effect conditions in one mock |
Extract reusable mock fixtures with specific behaviors |
Mocking Too Much | Mocking all dependencies, even irrelevant ones | Mock only dependencies directly used by the code under test |
By understanding these common pitfalls and implementing the suggested solutions, you can write more effective and maintainable tests using pytest-mock
. This ultimately improves code quality and simplifies your development workflow.
Pytest Mock in Action: Real-World Success Stories
Theory is essential, but practical application truly solidifies understanding. This section explores real-world scenarios where pytest-mock
significantly improved testing outcomes in diverse Python projects. We'll see how various teams used this tool to overcome unique challenges, providing practical examples you can adapt to your own work.
Web Application: Streamlining Authentication Flow Tests
Testing authentication flows in web applications often involves complex interactions with external identity providers like OAuth 2.0. Mocking these interactions is key for achieving fast, isolated tests. Consider a web application using OAuth 2.0 for login. Instead of contacting the actual provider during testing, mocking the OAuth client greatly simplifies the process.
- Mock the
get_user_info
method of the OAuth 2.0 client to return predefined user data. - This eliminates the dependence on a live OAuth server during testing.
- Tests run faster and become independent of network conditions and provider availability.
By mocking this external dependency, tests can focus solely on the application's logic, ensuring proper handling of different user data scenarios.
Data Pipeline: Isolating Complex Dependencies
Data pipelines often have multiple stages with intertwined dependencies. Imagine a pipeline extracting data from a database, transforming it, and loading it into a data warehouse. Testing these stages independently requires mocking the connections and interactions between them.
- Mock the database connection to return specific datasets for each test case.
- Mock the data warehouse connection to verify data loading without actually writing to the warehouse.
This isolation simplifies debugging and boosts test reliability by preventing cascading failures from other pipeline stages.
CLI Tool: Simplifying Filesystem Interaction Tests
Command-line interface (CLI) tools often interact with the file system. Directly testing these interactions can be slow and may cause unintended side effects. Mocking file system interactions provides a cleaner approach.
- Use
mocker.patch
to replace theopen
function, preventing actual file access. - Return predefined file content from the mock to simulate various file system states.
This enables thorough testing without modifying or deleting actual files. It also allows easy simulation of file system errors for improved edge case coverage.
API Service: Handling External Integrations
API services frequently integrate with other APIs or external systems. For instance, an e-commerce API might connect to a payment gateway. Directly testing these integrations can be slow and unreliable. pytest-mock
enables effective isolation.
- Mock the payment gateway client to simulate both successful and failed transactions.
- This ensures the API service correctly handles various payment gateway responses.
This isolation allows testing of specific error handling logic without interacting with the live payment gateway, saving time and avoiding potential transaction fees.
Ready to optimize your CI/CD pipeline and empower your development team? Explore how Mergify can enhance your workflow and alleviate infrastructure burdens. Mergify offers advanced merge automation and management, allowing your team to concentrate on building exceptional software.