Pytest in Python: Essential Guide to Modern Testing for Real-World Projects
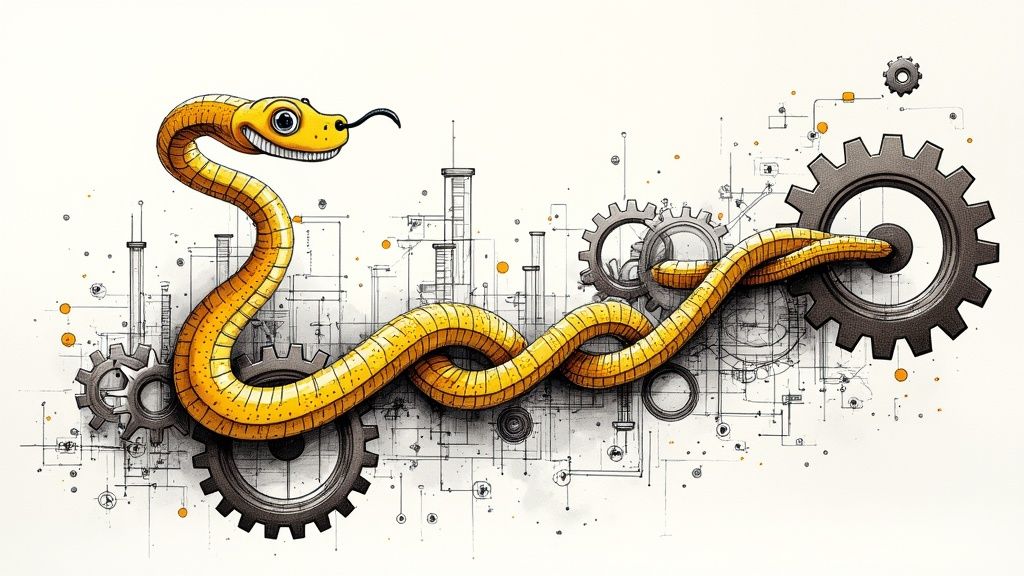
Getting Started with Pytest: Beyond Basic Testing
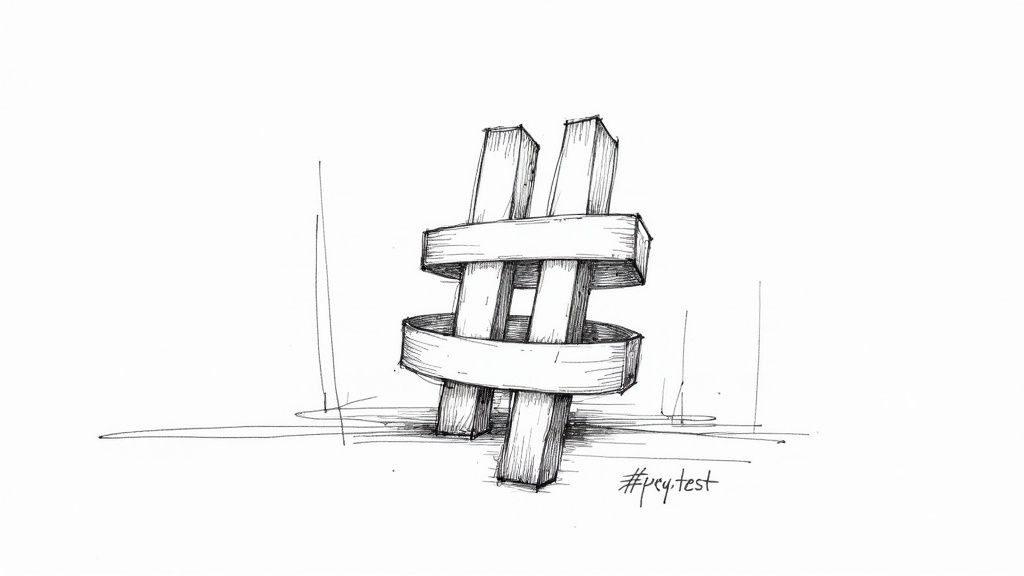
Let's explore how to use Pytest effectively to build reliable tests for your Python projects. We'll look at practical ways to set up, configure, and write tests that give you confidence in your code.
Installing and Configuring Pytest for Real Projects
Getting started with Pytest is straightforward - just run pip install pytest
. But for real projects, you'll want to think about where to put your test files and how to organize them. Most teams create a dedicated tests
directory and use configuration files to specify test locations and settings.
The key is making Pytest work smoothly with your existing project structure. Consider factors like:
- Where test files should live
- Which plugins you need
- How to set up test environments
- What naming conventions to follow
Writing Effective Tests with Pytest's Intuitive Syntax
One of Pytest's best features is its clean, simple syntax that uses standard Python assert statements. Here's a basic example:
def test_addition(): assert 2 + 2 == 4
This approach means less setup code and more focus on actual test logic. Compared to Python's built-in unittest
module, Pytest tests are shorter and easier to read. Want to learn more? Check out this in-depth Pytest tutorial.
Addressing Common Testing Challenges with Pytest
Many developers find testing daunting, especially when tests become hard to maintain or debug. Pytest helps by providing clear error messages that point directly to failed assertions. It also offers a rich collection of plugins for tasks like:
- Measuring code coverage
- Creating test doubles (mocks)
- Testing database code
- Generating test reports
Advanced Features for Enhanced Testing
Two powerful Pytest features are fixtures and parameterized tests. Fixtures let you create reusable setup code that multiple tests can share. This reduces duplication and keeps your test suite clean.
Parameterized testing allows running the same test with different inputs. Instead of writing separate test functions for each case, you can test multiple scenarios in one place. This improves both test coverage and maintainability.
Test-Driven Development: Building Reliable Python Applications
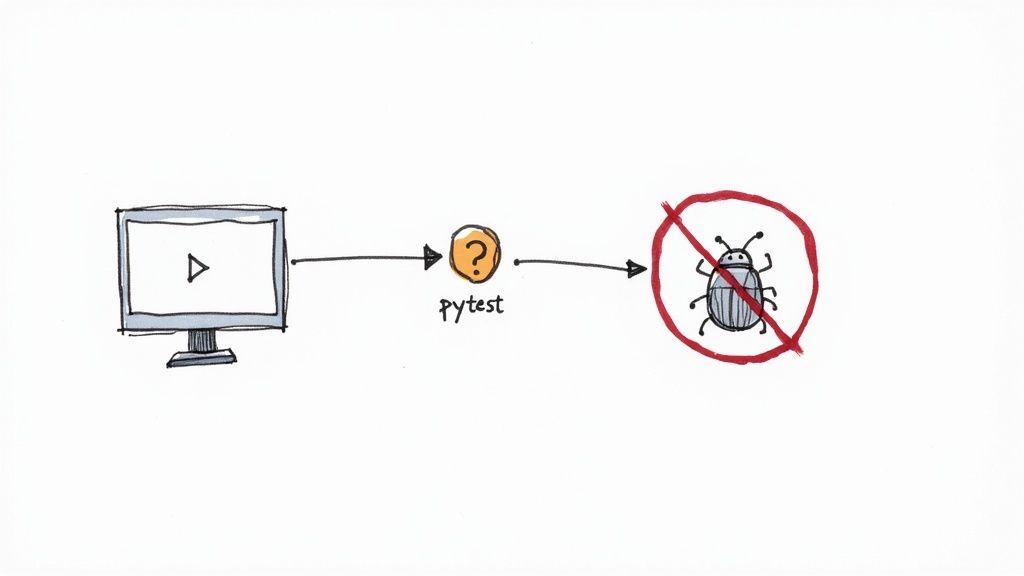
Test-Driven Development (TDD) combined with Pytest helps you build better Python applications by putting tests first. Instead of writing code and then testing it later, TDD flips the process - you write tests before writing any code. This simple change makes a big difference in how reliable your applications become.
Key Steps of the TDD Cycle Using Pytest
When practicing TDD, you follow these basic steps:
- Write a Test: Create a specific test case that shows exactly what you want your code to do
- Run the Test: Run it knowing it will fail (since you haven't written the code yet)
- Write Minimal Code: Add just enough code to make the test pass
- Clean Up: Make your code cleaner and more efficient while keeping the tests green
Using Pytest for TDD helps catch bugs early and gives you confidence when improving your code. Want to learn more about getting started with TDD and Pytest? Check out this detailed guide on Pytest testing.
Benefits of Pytest in TDD
Pytest makes TDD easier and more effective with several key features:
- Clear Syntax: Simple assert statements help you focus on what matters - testing your code's behavior
- Helpful Plugins: Add tools like
pytest-cov
for code coverage andpytest-mock
for mocking with minimal setup - Better Error Messages: When tests fail, Pytest shows exactly what went wrong and where
Real-World TDD with Pytest
Many development teams have found success with TDD and Pytest by keeping their test suites focused and well-organized. Think of it like building a house - you wouldn't start putting up walls before laying a solid foundation. Similarly, writing tests first gives you a blueprint that keeps your code stable and reliable.
Adding TDD to your workflow isn't always easy at first, but the payoff is worth it. Your code becomes more reliable, bugs become less common, and maintaining your application gets much easier over time.
Mastering Advanced Pytest Features for Complex Applications
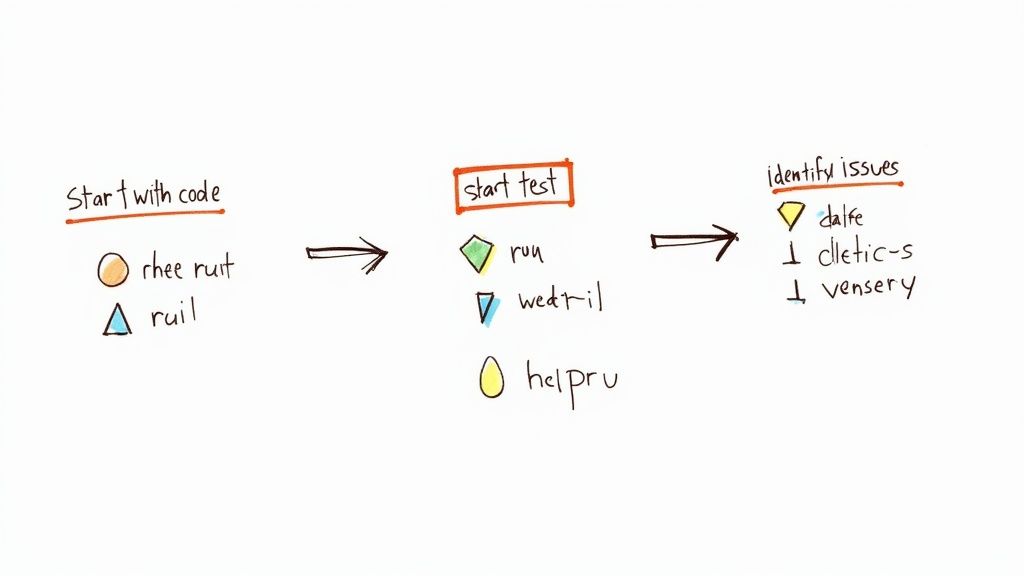
Now that you're comfortable with basic testing and TDD in Pytest, let's explore the advanced features that make it truly powerful for complex testing needs. These capabilities help you build better, more maintainable test suites as your projects grow.
Fixtures: Managing Test Dependencies and State
Fixtures are essential tools in Pytest that handle test setup, dependencies, and cleanup. Think of them as helpers that prepare and clean up your test environment. For example, when testing database operations, you don't want to create and close connections in every test.
A simple database fixture could look like this: @pytest.fixture def database(): conn = create_connection() yield conn conn.close()
Parameterization: Testing Multiple Scenarios Efficiently
Writing separate tests for different input scenarios is tedious and prone to mistakes. Pytest's parameterization lets you run the same test with multiple inputs. This is perfect for testing input validation, edge cases, and various data combinations.
Key benefits of parameterization:
- Write one test function instead of many similar ones
- Easily spot patterns in test failures
- Maintain test cases in a clear, organized way
Markers: Organizing and Selecting Tests
As your test suite grows, you need ways to organize and run specific groups of tests. Markers let you tag tests with labels and run them selectively. This helps manage large test suites effectively.
Common uses for markers:
- Label slow tests to skip during development
- Group related tests by feature or component
- Mark tests that need special setup or conditions
Plugins: Extending Pytest's Capabilities
The Pytest ecosystem includes many useful plugins that add extra features. Some popular ones include:
- pytest-cov: Track code coverage
- pytest-mock: Simplify mocking in tests
- pytest-xdist: Run tests in parallel
To get started with plugins:
- Install what you need with pip
- Add configuration to your pytest.ini file
- Use the new features in your tests
By mastering these features, you'll write better tests that catch bugs earlier and make maintenance easier. Remember to check the Pytest documentation for detailed examples and best practices as you build your test suites.
Building a Stronger Testing Community Through Pytest
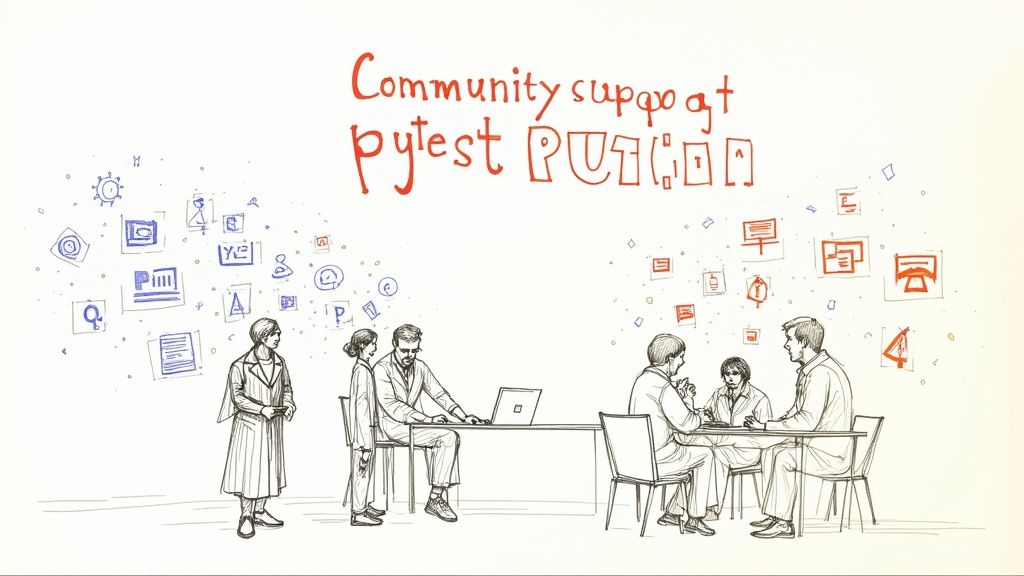
Pytest in Python has grown thanks to its active and supportive community of developers. The combined efforts of core contributors and regular users have shaped Pytest into the powerful testing tool it is today. A great example of this community spirit was 'adopt pytest month' in April 2015, where experienced users dedicated time to help open source projects adopt pytest effectively. This mentoring approach helped teams build confidence and skill with the framework. Learn more about adopt pytest month.
Community Resources and Success Stories
The Pytest community excels at knowledge sharing through detailed documentation, active forums, and practical tutorials. These resources help both new users and experienced developers improve their testing practices. Real teams have seen impressive results - many report a 40% decrease in debugging time after fully implementing Pytest in their workflow.
Contributing to the Ecosystem
Anyone can help improve Pytest. Whether you write plugins, update docs, or share feedback, every contribution makes a difference. Take Pytest-cov as an example - this widely-used code coverage plugin came from community members wanting to help others test better. It shows how sharing solutions benefits everyone.
Practical Community Solutions
Many companies have improved their testing by using community-created tools and techniques. You can do the same for your projects - for instance, platforms like Mergify help teams run tests more efficiently as part of their workflow.
By participating in this community, you join developers worldwide who use Pytest to write better tests and improve code quality. The ongoing exchange of ideas and solutions keeps Pytest growing and adapting to new testing needs in Python development.
Effective Debugging Strategies with Pytest's Toolkit
Pytest does more than just run tests - it gives developers powerful tools to debug and fix issues quickly. Let's explore how teams use Pytest's debugging capabilities to speed up their development process.
Interpreting Test Failures: Pytest's Detailed Reporting
When a test fails, Pytest provides rich error details that help pinpoint the exact issue. You get the specific line where the assertion failed, a comparison of expected vs actual values, and a traceback showing the sequence of function calls. For example, when testing a discount calculator, Pytest clearly shows the test inputs, expected discount amount, and what was actually calculated - making it easy to spot calculation errors.
Utilizing Advanced Debug Options: Beyond Basic Assertions
Pytest offers several options for deeper debugging:
- The
-s
flag lets you see print statements during test execution - The
--pdb
flag drops you into the Python debugger when a test fails - The debugger lets you inspect variables and step through code line-by-line
- You can examine the application state at the exact moment of failure
These tools give you precise control to diagnose complex issues.
Debugging Complex Test Suites: Strategies and Tools
For large test suites, Pytest provides ways to manage complexity:
- Use markers to categorize tests (e.g.
@pytest.mark.database
) - Run specific test categories with the
-m
flag - Focus debugging on relevant test subsets
- Remove noise from unrelated test cases
The Pytest plugin ecosystem adds even more capabilities. pytest-xdist enables parallel test execution to speed up feedback cycles. pytest-check allows multiple assertions per test for better error detection.
These debugging features help teams quickly understand and fix issues. For managing tests in CI/CD pipelines, tools like Mergify can automate test execution and merging. The combination of Pytest's debugging tools with automation makes it easier to maintain complex projects while keeping code quality high.
Streamlining CI/CD Workflows with Pytest Integration
Running automated tests efficiently is crucial for any modern development workflow. Let's explore how to effectively integrate Pytest with your continuous integration pipeline to catch bugs early and ship code confidently.
Configuring Pytest for Automated Environments
Getting Pytest working smoothly in CI requires some upfront configuration. First, specify where your test files live and what command-line flags you need in your CI config. Store standard settings and plugin configurations in a pytest.ini
or pyproject.toml
file to ensure tests run consistently across different environments.
Making Tests Run Faster with Parallelization
When test suites grow large, running them sequentially becomes a bottleneck. The pytest-xdist plugin lets you distribute tests across CPU cores or machines in parallel. This simple change can dramatically speed up your test runs, getting you feedback faster so you can keep coding.
Creating Clear Test Reports
Good test reports help teams understand results and fix problems quickly. Pytest pairs well with plugins like pytest-html
to generate detailed reports showing what passed, what failed, and why. Add pytest-cov
to track code coverage and identify undertested areas. These insights guide improvements to your test suite over time.
Real Examples of CI/CD Integration
Many development teams run Pytest automatically on every code push using cloud CI platforms like CircleCI. This catches problems before they reach main branches. Tools like GitHub Actions make it easy to run tests right in your repository workflow.
Keeping Tests Reliable at Scale
As projects grow, flaky tests become more common. Use fixtures to properly isolate tests and markers to organize them into logical groups. This prevents failures from cascading and keeps results consistent across environments - especially important for large codebases.
Solid test automation with Pytest helps teams ship quality code confidently. The time invested in good testing practices pays off in fewer bugs and more time for building new features.
Want to take your testing workflow further? Mergify integrates seamlessly with Pytest-based CI/CD pipelines to automate merging and deployment. Check out how Mergify can help streamline your development process.