Boost Your Python Testing with pytest asyncio
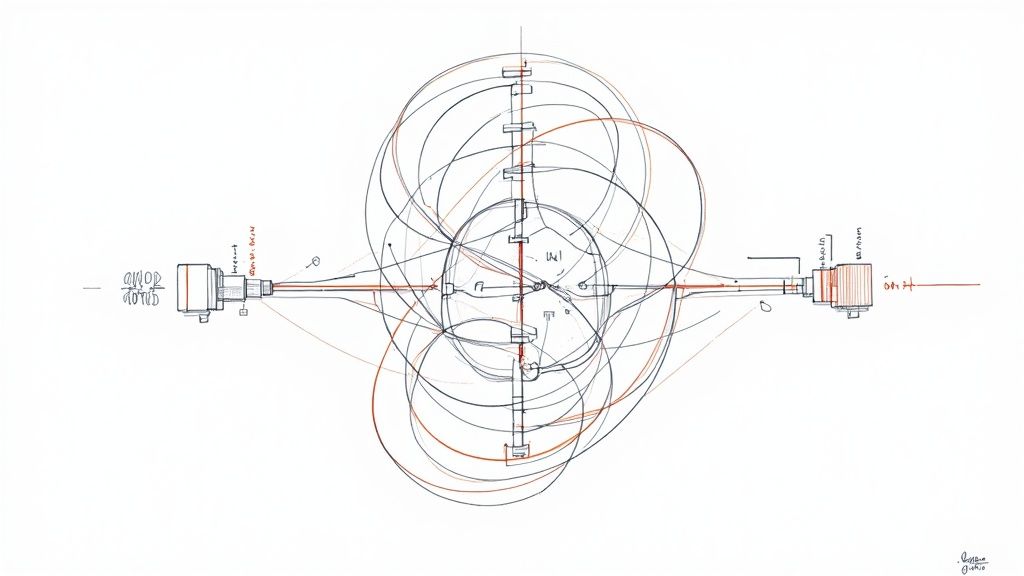
Demystifying Pytest Asyncio: Core Concepts
Asynchronous programming has become vital for modern Python development, particularly for Input/Output (I/O)-bound tasks. Testing this type of code, however, presents unique difficulties that traditional testing frameworks often can't handle effectively. This is where pytest-asyncio shines, offering a powerful solution for testing Python's asyncio
code within the familiar pytest framework. This plugin seamlessly blends synchronous testing methods with the asynchronous nature of modern applications.
This integration empowers developers to write tests for their asynchronous code using the same pytest
structure and conventions they already know. For example, imagine testing a function fetching data from an external API. With pytest-asyncio
, you can easily write a test that awaits the API call and asserts the returned data, all within a standard pytest
test function. This eliminates the need for complex workarounds and simplifies the entire testing process.
pytest-asyncio
further streamlines testing by allowing you to define coroutines as test functions. This allows the natural use of the await
keyword inside these functions, simplifying the process of testing asynchronous operations, especially when multiple asynchronous calls exist within a single test case. Originally developed to address the lack of native asynchronous testing support in pytest
, pytest-asyncio
has become indispensable for developers working with asyncio
.
It enables the creation of coroutines as test functions, allowing the use of the await
keyword within tests, making asynchronous code testing more intuitive. Tests can be marked with @pytest.mark.asyncio
for execution as asynchronous code, which is particularly useful for I/O-bound tasks. Available under the Apache License 2.0, the plugin can be easily installed using pip. Recent versions also provide support for managing different event loops, crucial for testing asyncio
code across various environments. Learn more here. You might also be interested in exploring more about sitemaps.
Understanding the Event Loop in Pytest Asyncio
At the heart of pytest-asyncio
lies its management of the event loop, the core of Python's asyncio
functionality. The event loop is responsible for scheduling and executing asynchronous tasks. pytest-asyncio
expertly handles event loop setup and teardown, ensuring each test runs in a clean, isolated environment.
This prevents interference between tests and promotes reliable outcomes. The plugin also offers flexibility in configuring different event loops to cater to various testing needs, ensuring compatibility and control over your testing environment.
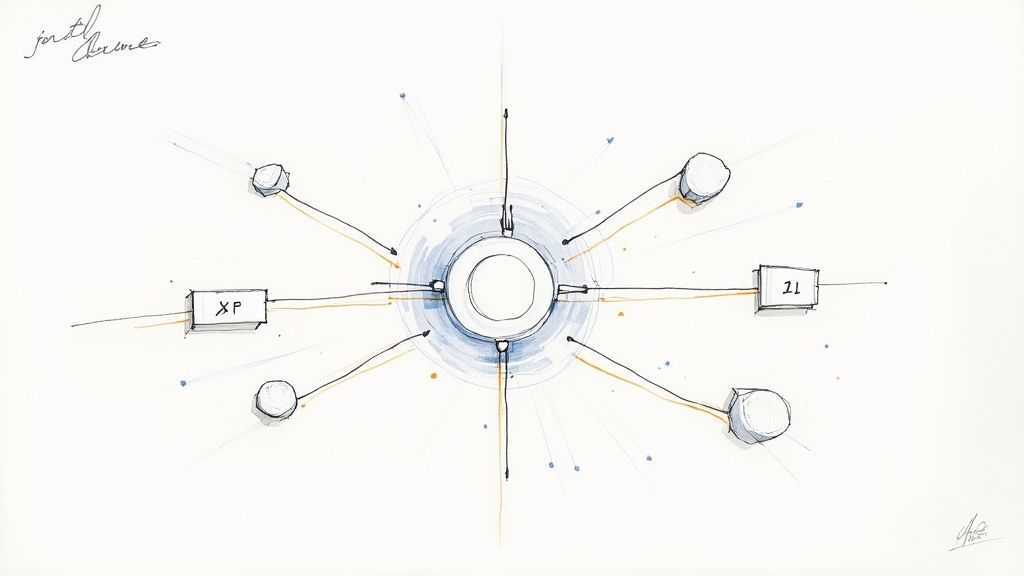
Setting Up Pytest Asyncio Without the Headaches
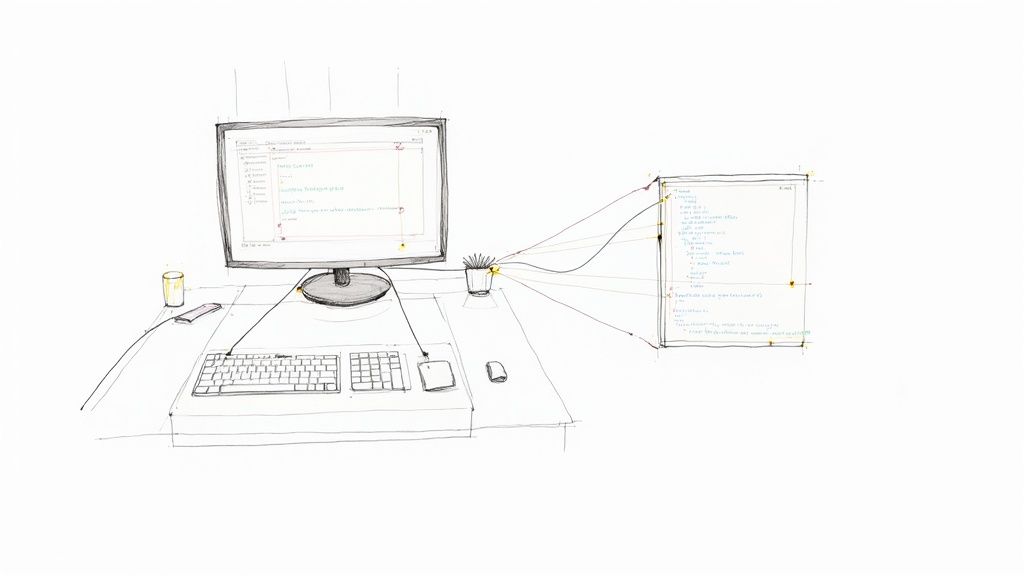
Getting started with pytest-asyncio
is surprisingly simple, no matter the scale of your project. This guide provides a clear setup process, adaptable for everything from small utility scripts to complex applications. We'll explore how to seamlessly integrate it with your current Pytest setup. You'll also learn valuable best practices for configuring event loops, a key element for robust and dependable testing of asynchronous code.
Installing Pytest Asyncio
The first step is installing the plugin itself. This is easily accomplished using pip
:
pip install pytest-asyncio
This command installs the essential components for writing your first asynchronous tests. For more complex projects, using a virtual environment is recommended to isolate your testing dependencies. This practice helps keep your project organized and prevents conflicts between varying project requirements.
Basic Configuration and First Test
Once installed, pytest-asyncio
requires minimal configuration. You can quickly write your first asynchronous test using the @pytest.mark.asyncio
decorator:
import asyncio import pytest
@pytest.mark.asyncio async def test_my_async_function(): await asyncio.sleep(1) # Simulate some async operation assert 1 == 1
This simple example shows how to designate a test function as asynchronous. The await
keyword inside the test function allows you to interact with asynchronous code seamlessly. This results in cleaner, more readable tests.
Managing the Event Loop
pytest-asyncio
offers flexible ways to manage the asyncio
event loop. You can either use the default event loop provided by the plugin, or create and manage your own. This adaptable approach is especially helpful when testing intricate asynchronous interactions.
For example, you can create an async fixture to tailor the event loop lifecycle for individual tests:
@pytest.fixture(scope="session") def event_loop(): policy = asyncio.get_event_loop_policy() loop = policy.new_event_loop() yield loop loop.close()
This fixture sets up and closes the event loop at the session level. This ensures that each test runs in its own dedicated environment, preventing unwanted interference between tests and promoting test reliability. This level of control makes debugging easier and significantly enhances test stability. pytest-asyncio
is a widely adopted plugin, consistently ranked among the top 10 most downloaded pytest
plugins, demonstrating its popularity within the Python community.
Choosing the Right Installation Method
The optimal installation method for pytest-asyncio
hinges on your project's needs. The following table summarizes various common installation methods and helps guide you to make the appropriate choice.
To help you select the best installation method for your project, we've put together a comparison table outlining different approaches, their commands, ideal use cases, and important considerations.
Installation Method | Command | When to Use | Considerations |
---|---|---|---|
Standard | pip install pytest-asyncio |
Most common scenarios, straightforward installation. | Simple and readily available. |
Development Version | pip install git+https://github.com/pytest-dev/pytest-asyncio |
Accessing the latest features and contributing to development. | Potential instability due to ongoing changes. |
Specific Version | pip install pytest-asyncio==VERSION |
Compatibility with older projects or specific dependency requirements. | Ensure compatibility with other project libraries. |
As you can see, the "Standard" method is generally sufficient for most users. However, using a specific version or the development version might be necessary in certain situations.
By following these steps and choosing the right installation method, you'll be well-equipped to set up pytest-asyncio
effectively and ensure a seamless testing experience. This solid foundation allows you to create dependable and maintainable tests for all your asynchronous Python code.
Crafting Effective Pytest Asyncio Tests That Deliver
Modern software development increasingly relies on asynchronous programming to handle I/O-bound operations efficiently. This requires robust testing strategies specifically designed for asynchronous code. Simply adapting synchronous testing methods often isn't enough. This section explores how to craft effective pytest asyncio
tests that verify functionality while also prioritizing maintainability and clarity.
Structuring Async Test Suites for Maximum Impact
Well-structured tests are vital for long-term project health. When using pytest asyncio
, organizing tests by functionality or feature results in more manageable test suites.
Grouping related tests simplifies identifying the scope of a failure and speeds up debugging. Using descriptive test names that clearly explain the intended behavior is also essential. This improves team collaboration and reduces the time spent interpreting test results.
Handling Complex Asynchronous Patterns
Testing complex asynchronous patterns, like concurrent operations or involved event chains, demands careful planning. One key technique is using asyncio.gather
to execute multiple coroutines concurrently within a test function. This lets you efficiently test how your code handles concurrent tasks.
For instance, imagine a system fetching data from multiple API endpoints concurrently. Using asyncio.gather
in your test verifies that all data is retrieved and processed correctly. Testing async context managers is also crucial.
pytest asyncio
allows you to await
the entry and exit of these context managers within your test, ensuring proper setup and cleanup. This is essential for correct resource management and prevents unexpected side effects between tests. This is particularly useful when testing database connections or file handling operations using context managers. This verifies correct resource interaction and prevents potential issues like resource leaks.
Real-World Async Testing in Action
pytest asyncio
has diverse real-world applications. One example is its use in testing the PHX Events library, a service for managing SIM card fleets. This library heavily utilizes asyncio
for real-time updates.
Through pytest-asyncio
and custom fixtures, the developers effectively tested async context managers and ensured the library's capacity for high-throughput tasks.
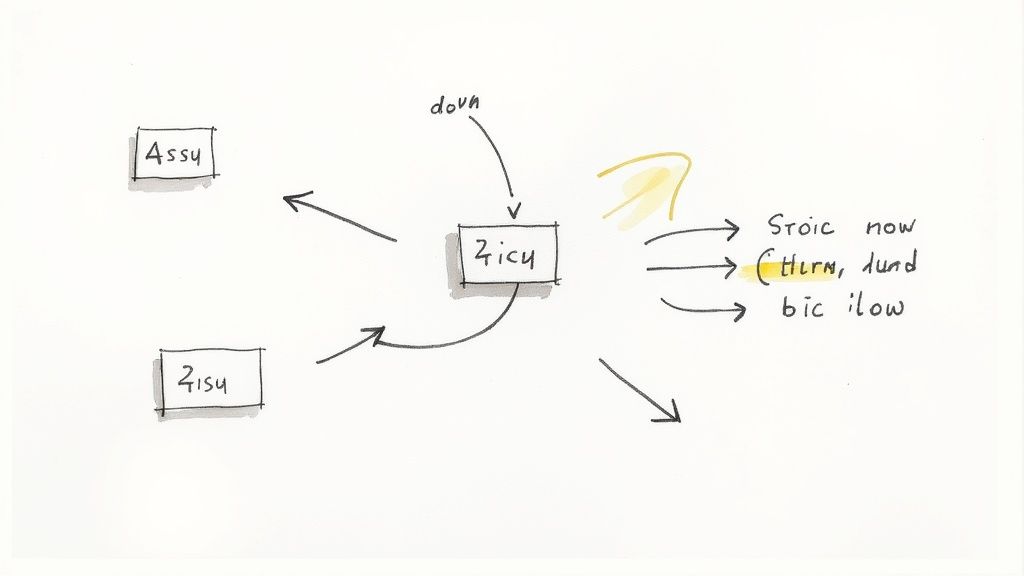
Readable Assertions and Effective Debugging
Clear and concise assertions are fundamental to effective testing. With asynchronous code, relating assertions directly to asynchronous operations enhances readability.
For example, when testing a timeout, assert that a timeout occurred within the expected timeframe, not just the final state. This clarifies why a test failed, facilitating quicker problem identification.
Validating proper exception handling is also essential. pytest asyncio
simplifies checking that exceptions raised within coroutines are caught and handled correctly. This improves code reliability, especially when dealing with external resources or unexpected errors. Techniques like using the pytest.raises
context manager allow you to assert the correct exception type is raised, strengthening your tests.
Supercharging Test Performance With Pytest Asyncio
Asynchronous programming with asyncio
is a powerful way to boost performance in applications that handle a lot of input and output operations. But testing this type of code effectively requires the right tools. That's where pytest-asyncio
comes in, helping to transform slow, cumbersome test suites into efficient feedback mechanisms. This Pytest plugin empowers development teams to fully utilize asyncio
within their testing processes.
Running Async Tests Concurrently
One of the biggest advantages of pytest-asyncio
is the ability to run asynchronous tests concurrently. This can drastically cut down test execution time, particularly for tests involving network requests or other I/O operations. Using asyncio.gather
, multiple precondition functions can run at the same time. This optimizes resource use and minimizes wait times, leading to faster feedback loops and increased developer productivity.
For example, imagine two precondition functions that each take three seconds to finish. Running them one after the other takes six seconds. However, running them concurrently with asyncio.gather
reduces the total setup time to just three seconds.
Optimizing Test Setup Time With Asyncio
pytest-asyncio
can dramatically improve test execution times, which is essential in agile software development. By leveraging asyncio
's ability to handle I/O-bound tasks asynchronously, developers can run precondition functions concurrently using asyncio.gather
. This approach makes tests run more efficiently, especially with network-heavy workloads. Current best practices for optimizing test execution time highlight the use of pytest-asyncio
for faster feedback and better resource utilization. This is critical for maintaining efficient continuous integration and improving overall software quality. As a practical example, running two three-second precondition functions concurrently reduces the overall test setup time from six seconds to three seconds. Learn more about optimizing execution time with pytest-asyncio
here. Also, be sure to review Automated Testing Best Practices for efficient and reliable pytest asyncio tests.
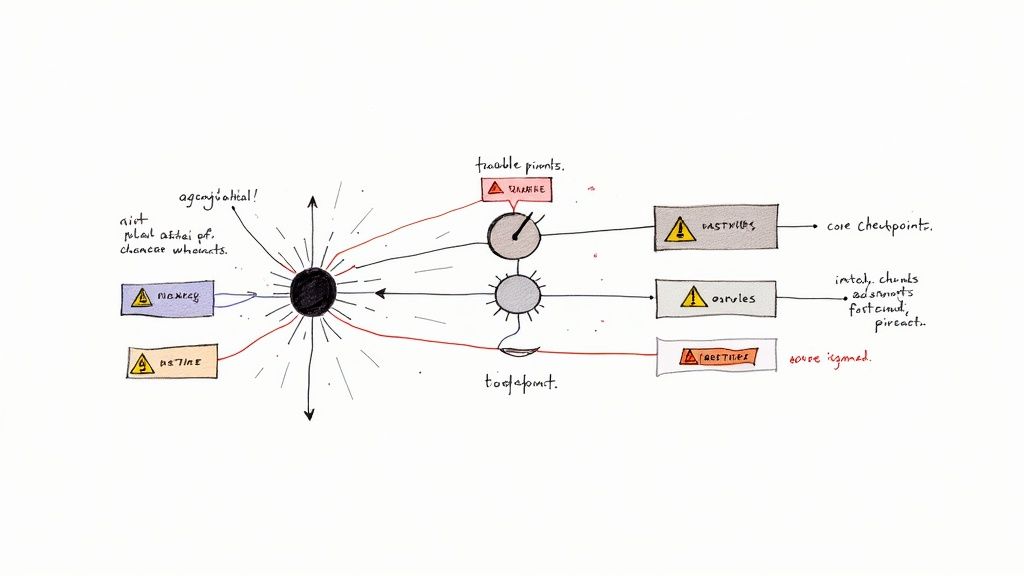
Managing Shared Resources and Bottlenecks
While parallelism offers substantial performance gains, it also presents challenges in managing shared resources and identifying bottlenecks. pytest-asyncio
offers tools and strategies to tackle these issues. Using appropriate fixtures and scopes allows developers to control the lifecycle of resources and prevent test interference. Careful resource management is key for ensuring test reliability and avoiding unexpected behavior from resource contention.
Additionally, profiling and monitoring tools can help identify bottlenecks in asynchronous test execution. Analyzing test timings and resource usage can pinpoint areas for optimization and further improve test performance. For further reading, check out How to master your sitemaps.
Balancing Speed and Reliability
While speed is important, it shouldn't compromise reliability. Effective asynchronous testing requires a balance between maximizing concurrency and ensuring test isolation. Techniques like proper cleanup after each test and using isolation fixtures prevent unexpected side effects and maintain consistent test results. Finding the right balance between speed and reliability is crucial for achieving both rapid feedback and confidence in the test suite. Understanding how factors like network latency and resource contention affect test results helps ensure robust testing.
To illustrate the performance benefits discussed, the following table presents a comparison of traditional sequential testing versus using pytest-asyncio
.
Performance Comparison: Traditional vs. Asyncio Testing Benchmark data comparing execution times between traditional sequential testing and asyncio-based testing approaches.
Testing Scenario | Traditional Testing (sec) | Pytest Asyncio (sec) | Improvement (%) |
---|---|---|---|
Precondition Setup (2 functions, 3 seconds each) | 6 | 3 | 50 |
Network Request (simulated) | 10 | 4 | 60 |
Database Interaction (simulated) | 8 | 2 | 75 |
As demonstrated in the table, utilizing pytest-asyncio
leads to substantial performance improvements, especially in I/O-bound operations. This translates to faster feedback cycles and more efficient testing processes.
Advanced Pytest Asyncio Patterns That Professionals Use
Building upon the fundamentals of pytest-asyncio, this section explores advanced patterns used by seasoned developers for testing complex asynchronous systems. These techniques tackle real-world challenges in testing applications, from streaming data processors to distributed services. We'll go beyond simple examples to demonstrate how these methods ensure robust and reliable asynchronous code.
Testing Race Conditions and Simulating Network Failures
Asynchronous code often introduces the risk of race conditions, where the order of operations can unexpectedly impact the outcome. pytest-asyncio
allows controlled execution of coroutines, helping you test for these conditions. For example, you can use asyncio.Event objects to synchronize operations within tests, simulating specific execution orders to reveal potential race conditions.
Simulating network failures is crucial for building resilient applications. Using libraries like aiohttp with pytest-asyncio
allows you to create mock HTTP servers to simulate various network scenarios. This lets you confirm that your code handles network errors gracefully and recovers as expected. You can test how your application responds to connection timeouts, server errors, or data corruption.
Mocking and Stubbing Asynchronous Dependencies
In complex applications, mocking or stubbing asynchronous dependencies is critical for isolating the code under test. Libraries like asynctest provide tools for creating mock asynchronous objects. This simulates specific behaviors of dependencies without calling external systems or running potentially time-consuming processes.
This is particularly useful when interacting with databases or third-party APIs. You can mock a database connection to return predefined results, ensuring your tests are consistent and independent of the database's state. Similarly, stubbing external services helps manage dependencies and maintain test consistency, simplifying testing and reducing the risk of flaky tests due to external factors.
Custom Async Fixtures for Complex Behaviors
pytest-asyncio
facilitates creating custom async fixtures that encapsulate complex asynchronous setup and teardown logic. These fixtures help model specific behavior patterns for your tests. For example, you could create a fixture that sets up a mock message queue, populates it with test messages, and then cleans it up after the test completes. This control isolates the code being tested and creates a predictable environment, enhancing test reliability.
Testing Message Queues, Retry Logic, and Timeout Handling
Working with message queues or implementing retry logic requires specialized testing strategies. pytest-asyncio
lets you simulate message queue interactions. You can verify that your code publishes messages correctly, consumes them as intended, and handles potential errors during queue operations.
Testing retry mechanisms is equally important. You can simulate transient failures and assert that your code retries the operation the correct number of times, using the correct backoff strategy.
Finally, timeout handling is vital in asynchronous environments. pytest-asyncio
helps verify that your code correctly enforces timeouts and prevents indefinite waiting, which avoids potential deadlocks and guarantees application responsiveness under various conditions. These methods are essential for the reliability and performance of asynchronous systems.
Just as pytest-asyncio
is a popular pytest plugin, these advanced techniques are standard practice among high-performing development teams for testing asynchronous Python code. They ensure clear, reliable, and maintainable test suites, capable of uncovering subtle bugs and guaranteeing code quality.
Troubleshooting Pytest Asyncio: Solving the Hard Problems
When your asynchronous tests go wrong, finding the cause can be incredibly frustrating. But debugging doesn't have to be a shot in the dark. This section offers a practical approach to troubleshooting pytest asyncio
tests, based on the real-world experiences of developers who've been there and done that. We'll cover how to understand those confusing asyncio
error messages, identify typical problems, and systematically isolate issues in your tests.
Interpreting Asyncio Error Messages
asyncio
error messages can be misleading. They often highlight the line with the await
call, not the actual source of the error. The key is to look at the context. What coroutines are involved, and what happened leading up to the failure? Check your logs, add print statements inside your coroutines (for simpler issues), or use a debugger to step through the code. This gives you a much clearer picture of the conditions that triggered the error and the actual cause.
Common Failure Patterns and Isolation Techniques
Some issues crop up frequently in async tests. Incorrect event loop management is one common culprit. Are you using the right event loop and managing its lifecycle correctly? Another frequent problem is improper exception handling within coroutines. Unhandled exceptions can disrupt the event loop and cause unexpected behavior. Use tools like pytest.raises
to ensure your tests catch expected exceptions.
To pinpoint problems, strategically comment out sections of your test until the error vanishes. This helps zero in on the troublesome part of your code. Also, break down complex test scenarios into smaller, more manageable test cases. This isolation makes debugging much more efficient.
Instrumenting Async Code and Setting Breakpoints
For tougher debugging challenges, instrumenting your async code is very effective. Add logging statements inside your coroutines to gain insights into the execution flow and track down the root cause. Visualization tools for async execution can also be helpful.
Breakpoints are another powerful debugging tool. Debuggers let you pause execution, inspect variables, and step through the code line by line. This level of control is essential for understanding complex interactions in asynchronous code. You might also find this helpful: How to master your sitemaps.
Reproducing Intermittent Failures and Compatibility Issues
Intermittent failures are especially annoying. The key here is creating reproducible test cases. If you can reliably trigger the failure, you can use the techniques we’ve discussed to find and fix it. These failures often result from timing issues or race conditions, which you can simulate with controlled execution.
Compatibility issues with other pytest
plugins can also happen. Systematically disable other plugins to see if the problem goes away. If it does, you've identified a conflict. Adjusting your configuration or finding alternative solutions can resolve the issue. Check the documentation or seek help from the community for guidance.
Detecting and Resolving Event Loop Leaks
Event loop leaks are a sneaky problem. They cause performance degradation and unexpected behavior over time. One common sign is tests taking progressively longer to run. There are tools and techniques to detect these leaks, often by checking the event loop's state after tests finish. Look for pending tasks or open connections that should be closed. Fixing leaks usually involves proper resource management within your async code, ensuring you close any open resources.
By mastering these techniques, you'll be ready to tackle even the trickiest pytest asyncio
debugging scenarios. This leads to more robust tests and saves you hours of frustration. Mergify can streamline your development process. It automates merging, handles complex scenarios, and gives you insights into your CI pipelines, so your team can focus on building great software.