Master pytest assert raises for Effective Exception Testing
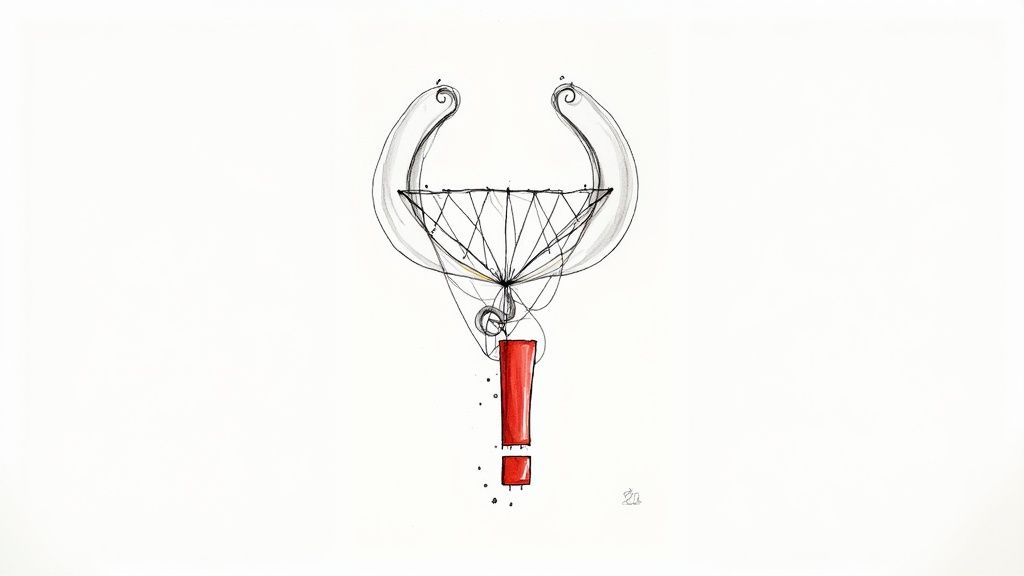
The Power of Pytest Assert Raises: Why It Matters
Exception handling is crucial for creating robust software. Well-handled exceptions prevent crashes and provide valuable debugging information. Testing these mechanisms, however, can be challenging. That's where pytest assert raises
comes in, simplifying and streamlining exception testing.
Imagine testing code that should raise a ValueError
for invalid user input. Traditional methods might involve clunky try/except
blocks in your test functions. pytest assert raises
offers a more elegant solution. You can directly specify the expected exception and the triggering code, resulting in concise and readable tests.
This approach has become popular among Python developers. For a deeper dive into Pytest, check out this resource: How to master Pytest. The shift towards pytest assert raises
reflects a broader movement in Python testing towards clearer, more maintainable code. Using the pytest.raises
context manager, pytest assert raises
allows tests to explicitly define the anticipated exception. This method gained popularity around 2013-2014 alongside the rise of Pytest as a major testing tool. By 2023, Pytest became the most-used Python testing framework, surpassing unittest, partially thanks to features like pytest.raises
which simplify error condition testing. Learn more about assertions in pytest here.
Why Proper Exception Testing Matters
Effective exception testing, facilitated by tools like pytest assert raises
, significantly boosts code reliability. It ensures your error handling logic works as intended, preventing unexpected crashes and providing helpful error messages. This is especially critical for production applications where unexpected errors can have severe consequences.
Furthermore, pytest assert raises
encourages better code design. By prompting developers to consider potential exceptions early on, it fosters more robust and resilient code. This proactive approach to error handling minimizes technical debt and simplifies future maintenance.
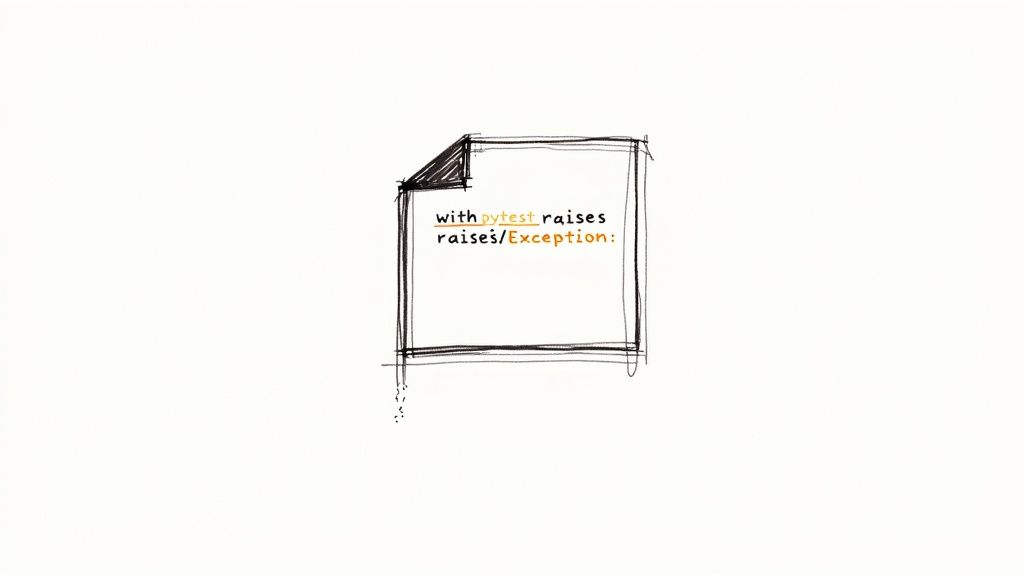
Experienced developers understand the importance of comprehensive exception testing. They recognize that gracefully handling errors is as crucial as implementing core application functionality. Using pytest assert raises
allows them to build code that is both functional and resilient to unexpected inputs and edge cases. This proactive approach ultimately leads to more stable and maintainable software.
Mastering Pytest Assert Raises: Syntax That Works
Building upon the basics of exception testing, let's delve into the practical uses of pytest assert raises
. This feature transforms exception handling from a cumbersome chore into a streamlined process. We'll explore both the context manager and decorator methods, highlighting their advantages in different situations.
Context Manager: The Standard Approach
The context manager is the most common way to utilize pytest assert raises
. It clearly defines the code block where the exception is anticipated, enhancing readability and pinpointing the precise location of the expected error.
Consider a function designed to raise a ValueError
when provided with invalid input:
import pytest
def validate_input(input): if input < 0: raise ValueError("Input must be non-negative")
def test_validate_input_negative(): with pytest.raises(ValueError): validate_input(-1)
This example showcases how pytest assert raises
isolates the exception, preventing it from disrupting other tests. This controlled environment simplifies the management and interpretation of test results.
Decorator Method: For Expected Exceptions in Test Functions
Alternatively, the pytest.mark.raises
decorator offers another approach. This method is particularly beneficial when an entire test function centers around the expectation of a specific exception. It's important to note, however, that this focuses the test on the exception itself rather than the preceding behavior.
import pytest
@pytest.mark.raises(ValueError) def test_validate_input_negative_decorator(): validate_input(-1)
Understanding various testing types, like Web Application Testing, broadens your perspective on the utility of pytest.
Visualizing Key Aspects of pytest.raises
The following infographic summarizes the essential elements of using pytest.raises
: Exception Type, the match
parameter, and Code Block Scope. These three components provide granular control over your exception tests.
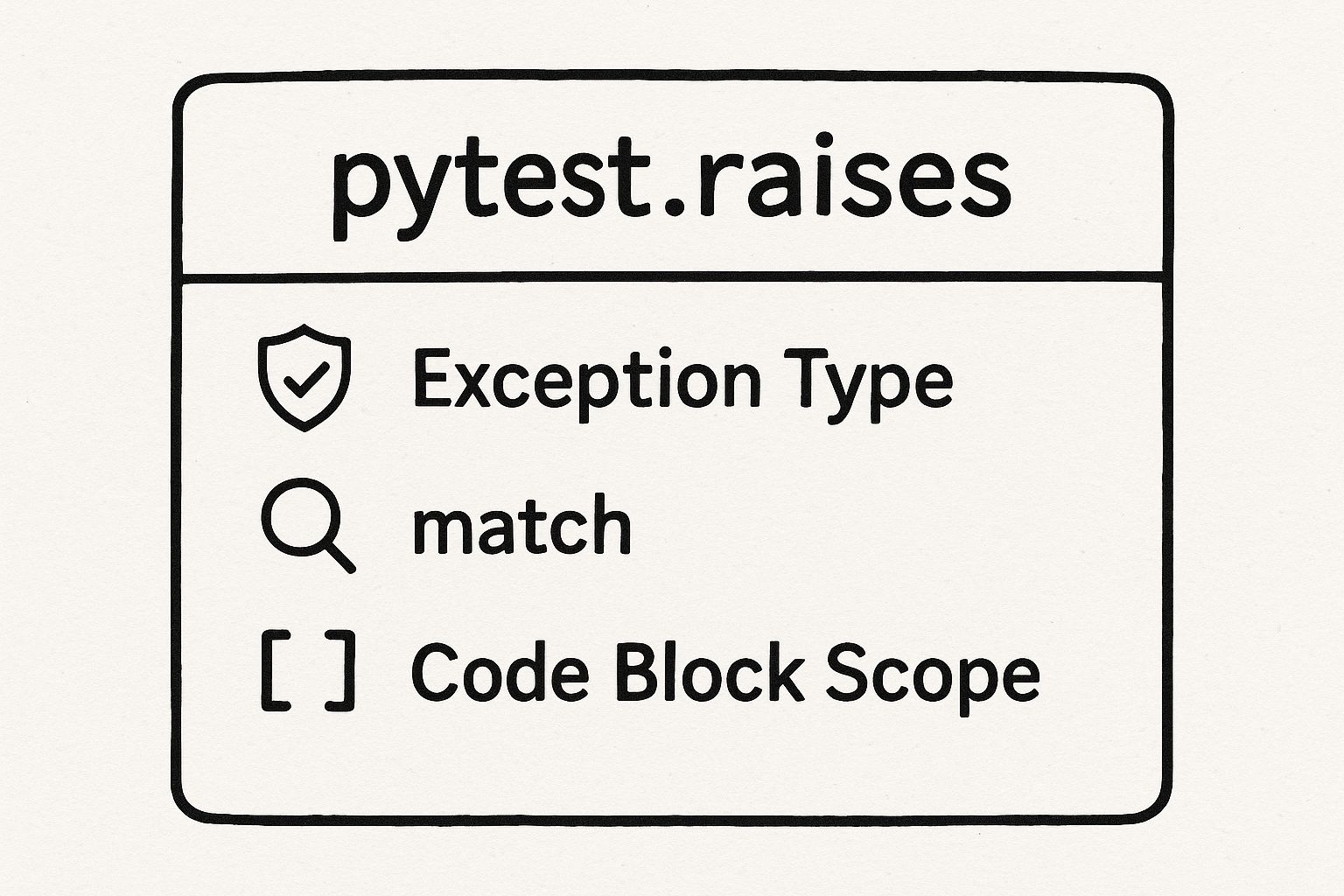
This visualization emphasizes the significance of specifying the correct exception type and employing the match
parameter for accurate error message verification. The Code Block Scope highlights the targeted nature of exception testing within a defined context.
Testing Custom Exceptions
Beyond standard Python exceptions, pytest assert raises
seamlessly integrates with custom exception classes. This feature is especially valuable when working with complex applications that define their own error hierarchies, enabling highly specific error condition testing and improving diagnostic clarity.
class CustomError(Exception): pass
def raise_custom_error(): raise CustomError("Something went wrong")
def test_custom_error(): with pytest.raises(CustomError): raise_custom_error()
Choosing the Right Approach: Context Manager vs. Decorator
The choice between the context manager and decorator hinges on your specific testing needs. The context manager affords more precise control, while the decorator provides a more succinct syntax when the entire test function focuses on a single exception.
To further illustrate the differences, let's look at a comparison table:
Pytest Assert Raises Syntax Comparison
This table compares different syntax approaches for testing exceptions with pytest.
Syntax Approach | Code Example | Best Used When | Limitations |
---|---|---|---|
Context Manager | with pytest.raises(ValueError): validate_input(-1) |
Need granular control over the code block where the exception is expected. Useful for testing specific parts of a function. | More verbose than the decorator. |
Decorator | @pytest.mark.raises(ValueError) def test_validate_input_negative_decorator(): validate_input(-1) |
The entire test function focuses on a single expected exception. | Less control over the specific location where the exception is raised within the test function. |
Key takeaway: the context manager provides more precise control, while the decorator offers conciseness.
The adaptability of pytest assert raises
empowers developers to select the most suitable method for their testing scenario. Mastering these approaches enables confident testing of both standard and custom exceptions, ultimately bolstering application robustness.
Beyond Basics: Advanced Pytest Assert Raises Techniques
Advanced techniques with pytest.raises
can truly set apart experienced Python developers. This section explores how to use the exception information object (excinfo
) for detailed validations of exception properties, messages, and attributes. This deeper level of testing leads to more comprehensive error handling and more robust code.
Leveraging the Exception Information Object (excinfo)
The excinfo
object, accessible within the pytest.raises
context manager, offers valuable insights into raised exceptions. It goes beyond simply checking if an exception occurred; it lets you verify why and how. This is essential for effective debugging and ensures your code behaves as expected in error conditions.
For example, consider validating a user's age:
import pytest
def validate_age(age): if age < 0: raise ValueError("Age cannot be negative")
def test_validate_age_negative(): with pytest.raises(ValueError) as excinfo: validate_age(-1) assert str(excinfo.value) == "Age cannot be negative"
This example shows how excinfo.value
accesses the exception's message, allowing for precise validation. This granular control strengthens your tests and prevents silent failures due to misleading error messages.
Handling Complex Scenarios: Multiple and Nested Exceptions
Real-world applications often involve multiple potential exceptions or even nested errors. pytest.raises
handles these complexities with ease. You can test for different exception types within a single block or use nested pytest.raises
contexts for intricate error scenarios.
Furthermore, combining pytest.raises
with parameterized testing lets you efficiently check diverse error cases, eliminating code duplication. This approach streamlines test maintenance and is particularly beneficial as your project grows. For more pytest tips and tricks, check out this helpful resource on how to master pytest-monkeypatch.
Advanced Validation Techniques with excinfo
Beyond just the message, excinfo
provides access to other exception attributes, enabling more in-depth analysis. You can inspect specific properties like error codes or custom attributes defined within your exception classes. Examining the traceback with excinfo.traceback
helps pinpoint the exact source of the error within your function.
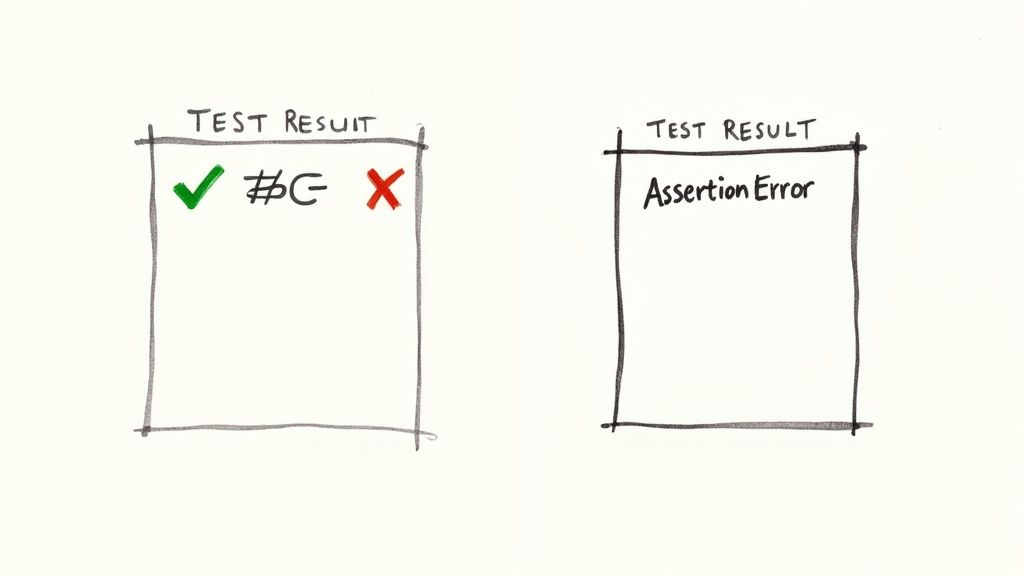
Understanding the underlying processes in your code is key. Consider applying structured documentation practices like those outlined in this guide on how to write a standard operating procedure. This approach leads to more organized and effective testing methodologies.
Practical Examples: Putting It All Together
Consider a function that might raise either a ValueError
or a TypeError
:
import pytest
def process_data(data): if not isinstance(data, int): raise TypeError("Data must be an integer") if data < 0: raise ValueError("Data must be positive")
def test_process_data_invalid_type(): with pytest.raises(TypeError) as excinfo: process_data("invalid") assert str(excinfo.value) == "Data must be an integer"
def test_process_data_negative_value(): with pytest.raises(ValueError) as excinfo: process_data(-1) assert str(excinfo.value) == "Data must be positive"
These examples demonstrate how to test for multiple exception types within a single test function. Checking both the type and the message provides greater confidence in the robustness of your error handling. This attention to detail is fundamental for building resilient and reliable software.
Pytest Assert Raises in Practice: Patterns That Scale
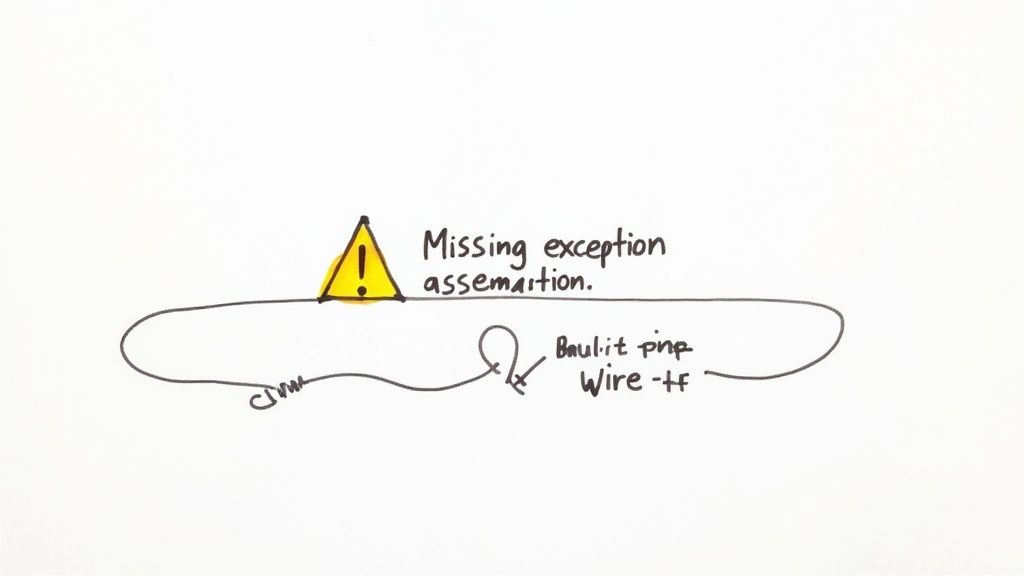
This section explores practical, proven methods for using the pytest assert raises
functionality in larger projects. Through real-world examples, we'll see how development teams can achieve thorough test coverage. This balance is struck by testing both expected behavior (the "happy path") and potential exceptions.
Maintaining Exception Tests in Evolving Codebases
As your project grows, maintaining your tests becomes increasingly important. Exception tests can be particularly fragile. One common issue is the brittleness introduced by changing error messages. A simple wording change can break a test, even if the underlying logic remains correct. Constantly updating tests for minor message tweaks can become a significant maintenance burden.
One effective approach is to test only the key parts of the error message. Instead of checking the entire string, verify the presence of a specific keyword or phrase. This approach offers flexibility while ensuring the correct error type is raised.
Precise Scoping: Avoiding False Positives
Accurate scoping of pytest.raises
blocks is crucial for scalable exception testing. If the block covers too much code, you risk catching unintended exceptions, leading to false positives. This can mask real bugs and reduce the effectiveness of your test suite.
Consider a function with multiple potential ValueError
exceptions. Wrapping the entire function in a single pytest.raises(ValueError)
block won't pinpoint the error's origin. Instead, use individual pytest.raises
blocks around each potential failure point. This granular approach significantly improves debugging and future maintenance.
Balancing Happy Path and Exception Path Testing
Effective testing involves balancing tests for expected behavior ("happy path") with those for exceptions. While focusing on the happy path is tempting, exception tests are essential for robust code.
Think about testing an API endpoint. You should test successful requests and various error responses. This provides comprehensive coverage, allowing you to identify and address potential disruptions to normal application operations.
Practical Strategies for Exception Testing
The following table, "Exception Testing Best Practices," summarizes recommended approaches for different exception testing scenarios. These techniques offer practical, easy-to-implement solutions for projects of any size.
To help you navigate the complexities of exception testing, we've compiled a table summarizing best practices. This table clarifies the recommended approaches, highlights anti-patterns to avoid, and provides concrete examples.
Exception Testing Best Practices
Scenario | Recommended Approach | Anti-Pattern to Avoid | Example |
---|---|---|---|
Changing error messages | Match against a key phrase within the message. | Matching the entire error string. | assert "Invalid input" in str(excinfo.value) |
Multiple potential exceptions | Use separate pytest.raises blocks for each possible exception. |
Enclosing everything in a single broad pytest.raises . |
See examples in the "Advanced Pytest Assert Raises Techniques" section. |
Nested exceptions | Use nested pytest.raises blocks. |
Ignoring or flattening nested exception structures. | with pytest.raises(OuterError): with pytest.raises(InnerError): ... |
These practices, derived from real-world development experience, help establish a robust exception testing strategy that scales with your project. Addressing common challenges and emphasizing precision and maintainability leads to a more resilient and scalable test suite. This ultimately results in higher code quality and fewer production errors.
Pytest Assert Raises vs. Alternative Approaches
Why is pytest.raises
the preferred method for testing exceptions in Python? This section compares pytest
's approach to other methods, such as the assertRaises
method in the unittest
framework, manual try/except
blocks, and similar features in other testing frameworks. We'll explore the differences in readability, maintainability, and functionality that have contributed to pytest
's popularity. We’ll also look at situations where other methods might still be relevant while emphasizing why pytest.raises
is the common choice for many Python developers. This comparison will improve your testing skills and empower you to make informed decisions about exception testing in your projects. See also: Unittest vs Pytest.
Readability and Maintainability
A key advantage of pytest.raises
is its concise and readable syntax. Compared to manual try/except
blocks, using a context manager simplifies test code, improving understanding and maintenance. This clarity lets developers concentrate on the test logic instead of deciphering complex exception handling.
Consider the difference:
Try/Except:
def test_example(): try: function_that_raises_exception() assert False # Should not reach here except ExpectedException: pass # Test passes
pytest.raises:
def test_example(): with pytest.raises(ExpectedException): function_that_raises_exception()
The pytest.raises
example is significantly shorter and more direct. This enhanced readability becomes especially valuable as tests grow in complexity.
Functionality and Flexibility
pytest.raises
provides more than just cleaner syntax. The excinfo
object gives access to the raised exception itself, allowing for in-depth analysis of its attributes, message, and traceback. This level of detail can be challenging to obtain with simpler assertRaises
methods or manual try/except
blocks.
Moreover, pytest.raises
seamlessly integrates with other pytest
features. For example, combining it with parameterized testing allows efficient testing of various exception scenarios without unnecessary code duplication. This flexibility makes pytest.raises
a powerful tool for thorough exception testing.
When to Consider Alternatives
While pytest.raises
is excellent in most cases, there may be specific situations where alternative approaches are warranted. If you require very precise control over the exception handling process, or if you are working with a legacy codebase heavily dependent on unittest
, using assertRaises
might be a more practical solution. However, for most modern Python projects, pytest.raises
offers the ideal combination of simplicity, functionality, and integration with the overall testing ecosystem.
Making Informed Decisions: Choosing the Right Tool
Choosing between pytest.raises
and its alternatives depends on your project’s specific requirements. If readability, maintainability, and seamless pytest
integration are important, pytest.raises
is generally the preferred option. Understanding the strengths and weaknesses of each method allows you to choose the best tool for your testing strategy, leading to more robust and reliable code.
Real-World Success With Pytest Assert Raises
This section explores how real-world development teams effectively use pytest.raises
in production. By examining open-source projects and drawing from the experience of testing experts, we'll uncover successful exception testing strategies at scale. These insights will help you create robust exception tests that improve code quality without adding to your maintenance burden.
Testing API Error Responses
Many teams rely on pytest.raises
for comprehensive API endpoint testing. Testing both successful requests (the "happy path") and various error responses is crucial for a resilient application. For example, consider an API endpoint designed to return a 400 Bad Request error for invalid input. pytest.raises
can verify the correct HTTP error code and the accompanying error message. This ensures your API handles and reports errors as expected in a production setting.
import pytest import requests
def test_invalid_api_request(): with pytest.raises(requests.exceptions.HTTPError) as excinfo: response = requests.post("your_api_endpoint", json={"invalid": "data"}) response.raise_for_status() # Raise HTTPError for bad responses (4xx or 5xx) assert excinfo.value.response.status_code == 400 assert "Invalid input" in str(excinfo.value.response.content)
Handling Validation Failures
Another common use case for pytest.raises
is validating user input or data integrity within applications. Imagine a function designed to raise a ValueError
for invalid data. pytest.raises
confirms that the exception occurs at the right moment and provides specific details about the failure, such as the invalid value or field. This level of testing prevents unexpected behavior in production.
import pytest
def validate_data(data): if data < 0: raise ValueError(f"Data must be non-negative, received: {data}")
def test_validate_data_negative(): with pytest.raises(ValueError) as excinfo: validate_data(-1) assert "Data must be non-negative, received: -1" in str(excinfo.value)
Testing System-Level Exceptions
pytest.raises
isn't limited to application-specific exceptions. It's equally valuable for testing lower-level system errors. For instance, a file-processing function might raise an IOError
if a file doesn't exist. pytest.raises
ensures these potential problems are handled correctly, preventing crashes or data loss.
import pytest import os
def process_file(filepath): if not os.path.exists(filepath): raise IOError(f"File not found: {filepath}")
def test_process_file_not_found(): with pytest.raises(IOError) as excinfo: process_file("nonexistent_file.txt") assert "File not found" in str(excinfo.value)
Maintaining Readability and Test Performance
High-performing teams prioritize code readability, even in exception tests. They use descriptive test function names and structure their tests logically. This makes it easier for other developers to understand and maintain the tests. These teams also pay close attention to test performance. While thorough exception testing is essential, excessively slow tests can impede development. They optimize their tests for efficiency without compromising comprehensiveness, avoiding redundant assertions or unnecessarily complex setups. The goal is to provide valuable feedback quickly without slowing down the development process. A well-maintained and efficient test suite is an invaluable asset for any software project.
Troubleshooting Pytest Assert Raises: Solutions That Work
Even experienced developers sometimes struggle with testing exceptions. This guide addresses common issues with pytest.raises
, offering practical solutions to streamline your testing process. We'll explore problems like false positives masking real bugs, unexpected exception sources, and the complexities of nested exceptions. You'll learn effective debugging techniques and how to refine your tests for precision and clarity. We'll also touch on performance considerations for large projects. These proven solutions will save you valuable debugging time and lead to more robust tests.
Common Pitfalls and Solutions
One common issue is encountering an unexpected exception type. You anticipate a ValueError
, but a TypeError
appears. This often signals a problem earlier in the code, possibly with input validation. The excinfo
object, available within the pytest.raises
block, is your friend. Inspecting excinfo.type
reveals the actual exception raised, helping pinpoint type mismatches or other underlying issues.
Another frequent problem arises when a test fails because the exception message differs from what’s expected. This can occur when error messages are updated without corresponding test revisions. Instead of matching the entire message, which can be brittle, focus on key phrases within the message:
with pytest.raises(ValueError) as excinfo: # Code that raises ValueError assert "Invalid input" in str(excinfo.value)
This approach allows for message adjustments without breaking your tests.
Debugging Strategies for Exception Tests
A false positive occurs when a pytest.raises
test unexpectedly passes, potentially hiding genuine issues. This happens when the expected exception isn't raised. Carefully examine the code within the pytest.raises
block. Is it actually executing? Using a debugger or print statements (Python documentation on print) can help verify the code path.
Sometimes, an exception is raised, but not from the anticipated source. This often indicates a problem with your test setup or assumptions about code behavior. Thoroughly review your test setup and the code being tested. Are there other potential triggers for the exception? Isolating the code within the pytest.raises
block is crucial for pinpointing the issue.
Nested Exceptions: A Special Case
Nested exceptions arise when an exception is thrown while handling another. This scenario requires specific debugging strategies. Nested pytest.raises
blocks enable capturing and inspecting both exceptions:
with pytest.raises(OuterException) as outer_excinfo: with pytest.raises(InnerException) as inner_excinfo: # Code raising nested exceptions
Examine both outer_excinfo and inner_excinfo
This technique helps unravel the interaction between exceptions and your code's response.
Performance Considerations
Numerous exception tests can impact your test suite's speed. While exception testing is crucial, ensure each test serves a distinct purpose. Avoid redundant tests that check the same conditions. Efficient setup and teardown procedures can minimize overhead. Profiling tools can identify performance bottlenecks in your tests.
Practical Tips for Efficient Testing
Here are key best practices for using pytest.raises
:
- Isolate the expected exception: Use precise code blocks within
pytest.raises
. - Match on keywords: Don't match entire exception messages for flexibility.
- Verify execution: Ensure the code within
pytest.raises
runs as expected. - Check test setup: Review for unexpected exception sources in the test itself.
- Use nested
pytest.raises
for nested exceptions: Examine both inner and outer exceptions. - Optimize test performance: Avoid redundant or slow exception tests.
By implementing these techniques, you can develop thorough and maintainable exception tests, contributing to a more robust and reliable software product. This benefits both developers and end-users.
Streamline your development workflow and minimize CI costs with Mergify. Mergify automates your pull request process, freeing you to focus on writing excellent code—including comprehensive tests with pytest.raises
—while Mergify handles the rest.