Mastering Pytest: A Comprehensive Guide to Python Testing Excellence
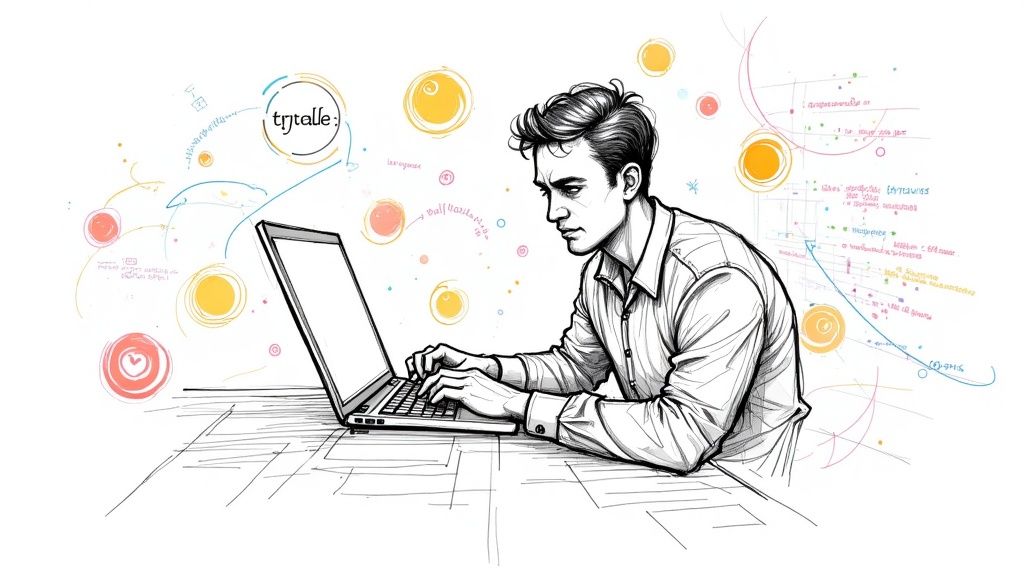
Understanding Pytest's Evolution and Impact
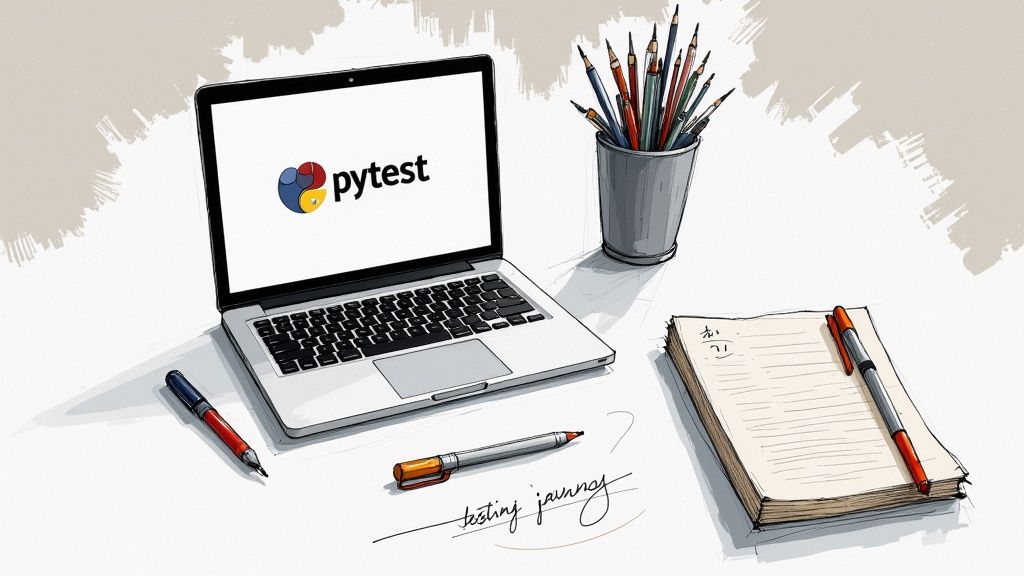
The story of Pytest's rise to become one of Python's most important testing tools is worth exploring. This popular framework has deep roots in Python's testing history and continues to shape how developers approach software quality today.
From PyPy to Pytest: A Testing-Focused Beginning
The seeds of Pytest were planted in the PyPy project, which created an alternative Python implementation. PyPy's developers put testing at the heart of their work from day one, using unit tests for new code, regression tests for bug fixes, and integration tests that worked with CPython's test suite.
What started as a simple tool called utest
in the early 2000s grew into py.test
and finally became the Pytest we use today. This steady evolution helped establish Pytest as a standalone framework with its own identity and growing community of users.
Simplicity and Power: The Pytest Philosophy
Pytest stands out by making testing both easy and powerful. Its clean, straightforward syntax means new developers can write their first tests quickly, while its advanced features give experienced teams the tools they need for complex testing scenarios.
Real-World Impact: Efficiency and Best Practices
Teams using Pytest often see immediate benefits in their development process. The framework's design helps developers create thorough test suites with less effort, leading to better code coverage and fewer bugs making it to production. This means teams can move faster while maintaining high quality standards.
Shaping Modern Development: The Continuing Influence of Pytest
Modern Pytest features like fixtures, parameterization, and plugins help teams build better testing practices into their daily work. The framework keeps improving through community contributions, ensuring it stays relevant as Python development evolves. By making testing more accessible and effective, Pytest helps teams write better code and work together more smoothly.
Getting Started With Pytest Fundamentals
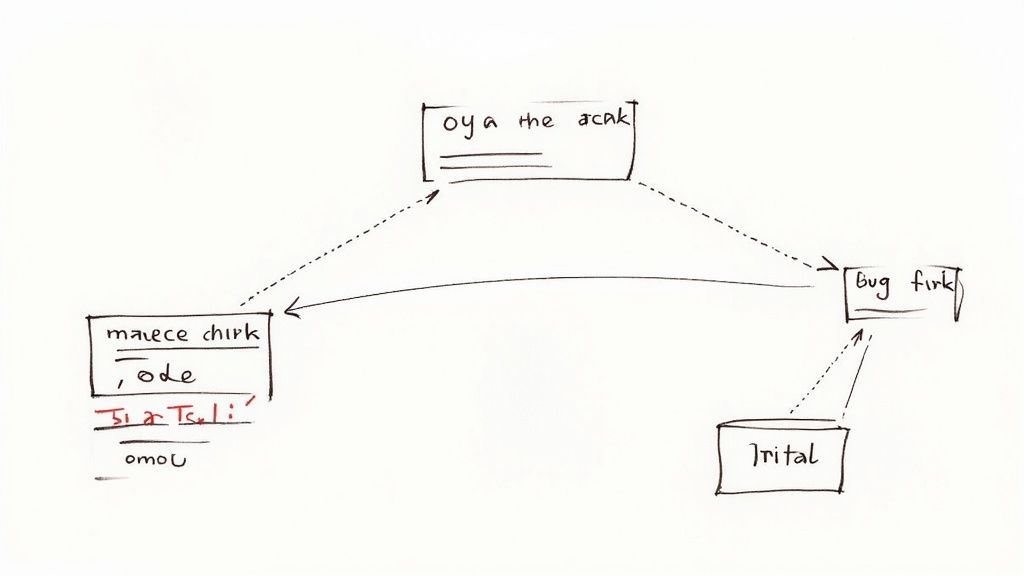
Testing your Python code doesn't have to be complicated. Pytest makes it easy to write and run tests that help catch bugs early. Let's explore how to set up pytest, write your first tests, and make the most of its powerful features.
Setting Up Your Pytest Environment
Getting pytest running is quick and straightforward. First, make sure you have Python installed on your system. Then install pytest using pip with this simple command:
pip install pytest
That's all you need to start testing! The simple setup process is one reason why so many developers choose pytest for their projects.
Writing Your First Pytest Test
With pytest, you write tests as regular Python functions - no complex classes needed. Just name your functions starting with test_
and use Python's built-in assert
statements to check if your code works as expected.
Here's a basic example:
def test_addition(): assert 1 + 1 == 2
To run your tests, open your terminal, go to your project folder and type pytest
. The tool will automatically find and run any functions that start with test_
. When tests fail, pytest shows exactly what went wrong and why.
Understanding Pytest's Clean Syntax
Pytest keeps things simple by using Python's standard assert
statement. This makes tests easy to read and understand, even for developers new to testing. The clean, minimal syntax encourages teams to write more tests, which leads to better code quality.
Pytest stands out from other testing tools because it needs less setup code than alternatives like Python's built-in unittest module. When tests fail, pytest provides detailed error messages that help you track down issues quickly. Learn more about pytest's key features.
Why Choose Pytest?
Pytest shines in several important ways. The error messages are clear and helpful for debugging. Fixtures let you reuse setup code across multiple tests, keeping your test suite organized and DRY (Don't Repeat Yourself). These features make it easier to test your code regularly and catch problems early. Plus, pytest's plugin system lets you add exactly the testing capabilities your project needs.
Mastering Advanced Pytest Features
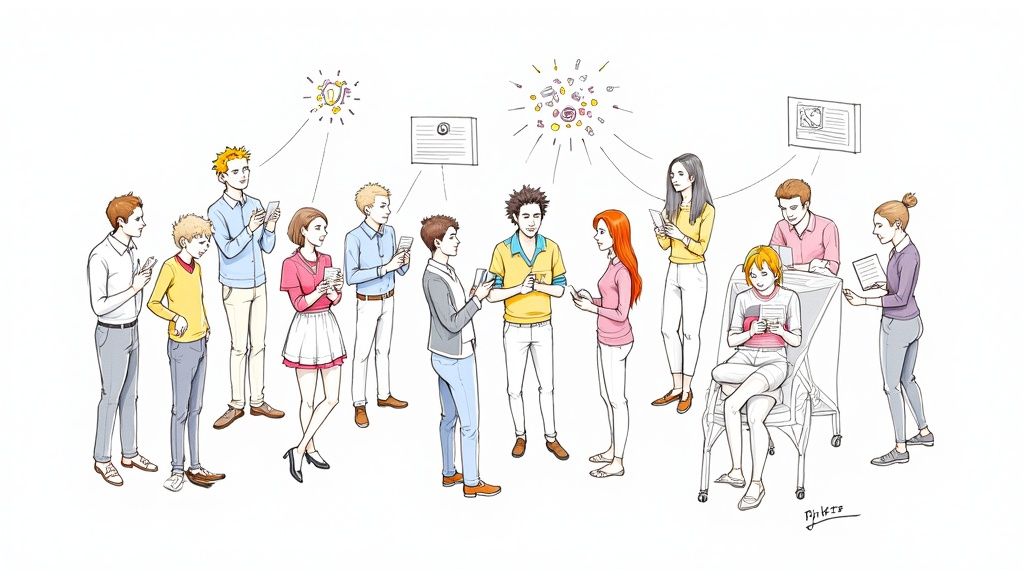
Pytest comes packed with advanced features that make testing Python code more effective. Let's explore the key capabilities that will help you write better tests: fixtures, parameterization, markers, and plugins.
Fixtures: Managing Test Resources and Dependencies
Fixtures are reusable components that handle test setup and cleanup. Think of them as helpers that prepare everything your test needs - like setting up a database connection before running tests and cleaning it up afterward.
Here's what makes fixtures so useful:
- They keep your test code clean by separating setup logic
- You can reuse them across multiple test files
- They make tests easier to read and maintain
- They eliminate repetitive setup code
Parameterization: Running Tests with Different Inputs
Parameterization lets you run the same test multiple times with different input values. This is perfect for testing how your code handles various scenarios without writing duplicate tests.
Common uses include:
- Testing boundary conditions and edge cases
- Verifying behavior with different data types
- Running the same checks across multiple input values
Markers: Categorizing and Selecting Tests
Markers help you organize and filter which tests to run. You can tag tests as "slow", "integration", or "regression" and then choose to run only specific types. Research shows that smart use of markers can cut test runtime by 20-30% by running only relevant tests.
Key ways to use markers:
- Group tests by feature or functionality
- Separate quick tests from slow ones
- Tag tests for different environments (dev, staging, prod)
Plugins: Extending Pytest Functionality
The pytest plugin ecosystem adds powerful features to your testing toolkit. Two standout examples:
- pytest-cov tracks code coverage
- pytest-xdist enables parallel testing, cutting test time by up to 50%
Here are some essential pytest plugins:
Plugin | Purpose |
---|---|
pytest-cov | Code coverage reporting |
pytest-xdist | Parallel test execution |
pytest-mock | Test mocking support |
pytest-html | HTML test reports |
Check out more recommended plugins here.
By using these advanced features effectively, you'll write more reliable tests and catch bugs earlier. Your test suite will run faster and provide better insights into your code quality.
Learning From Industry Leaders Using Pytest
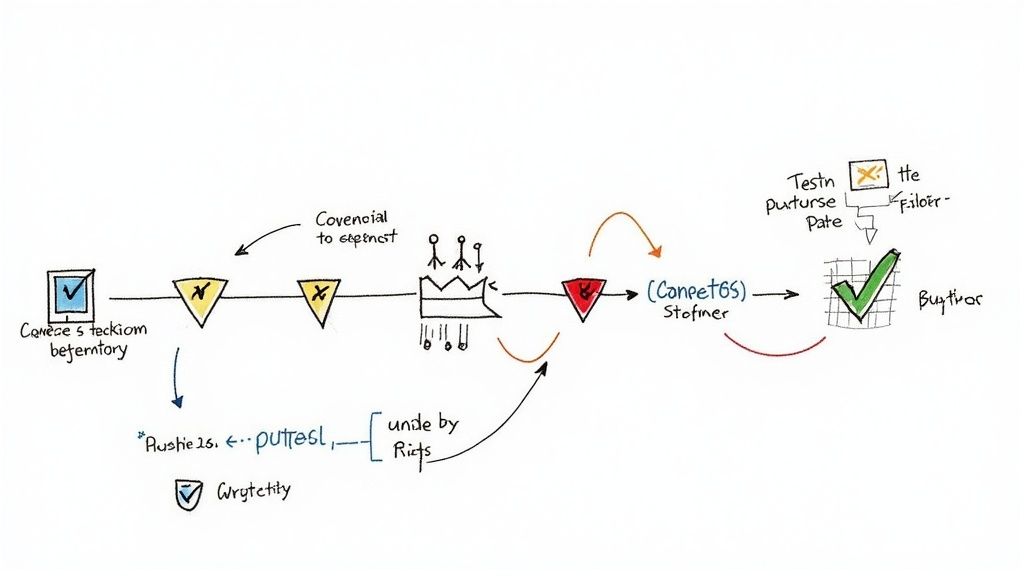
Most companies know how to use pytest's basic functions, but seeing real examples from industry leaders shows how powerful it can be in practice. Let's look at how successful teams are using pytest to build better testing workflows and maintain high code quality.
Scaling Testing With Pytest: Large Codebases and Distributed Teams
Big companies often struggle to manage testing across large codebases and teams working in different locations. This is where pytest's fixtures and markers become essential tools. Fixtures offer a consistent way to set up and clean up test resources across different environments. Markers help teams organize tests by type - like integration tests, performance tests, or database tests. This makes it easy to run specific test groups at different stages of development.
Maintaining High-Quality Standards: Integration With CI/CD and Code Review
Many teams integrate pytest directly into their continuous integration pipeline. This helps catch bugs early before they reach production code. Pytest also plays a key role in code reviews - developers can quickly run tests related to their changes to ensure new code works properly. This focus on early testing helps teams maintain reliable, high-quality code.
Making Development More Efficient With Pytest
Teams use pytest's plugins to speed up their testing process. Popular choices include pytest-cov
for checking code coverage and pytest-xdist
for running tests in parallel. Because pytest makes writing tests straightforward, developers tend to write more of them. Recent research shows that 65.19% of Python machine learning projects on GitHub use testing libraries, with pytest being very popular. Another study found that 61.65% of over 20,000 open-source projects include tests, averaging 41 tests per Python project. These numbers show how important testing tools like pytest have become. Learn more about this research here.
Adapting Pytest to Unique Needs
Each company uses pytest differently based on their specific requirements. Some build custom plugins to add new features, while others use existing plugins to work with their preferred tools. This flexibility makes pytest useful for many different types of projects and teams. By studying these real-world examples, other teams can learn how to make pytest work best for their needs.
Building a Sustainable Testing Culture With Pytest
A solid testing foundation is essential for any software project's long-term success. Pytest offers the tools and features needed to build this foundation effectively. Let's explore practical ways to integrate pytest into your team's workflow and create a testing culture that lasts.
Integrating Pytest Into Your CI/CD Pipeline
Adding pytest to your continuous integration workflow helps catch issues early. When you configure your CI system to run pytest after each code change, you get immediate feedback on whether new code works as expected. This quick feedback prevents bugs from reaching production.
Setting up automated test reporting also gives you clear insights into your code quality over time. Teams can track trends and identify areas that need more testing attention.
Establishing Effective Code Review Processes With Pytest
Good tests make code reviews more productive. When reviewers see comprehensive pytest tests backing up code changes, they can focus on design and logic rather than manually testing functionality. This speeds up reviews while maintaining quality.
During reviews, team members can quickly run related pytest tests to verify behavior. This builds confidence in the changes and helps spot subtle issues before they cause problems.
Maintaining Comprehensive Test Coverage
Writing thorough tests is key for reliable software. Pytest makes test creation straightforward, which encourages developers to write more tests covering different scenarios. The simpler it is to write tests, the more likely your team will maintain good coverage.
Tools like pytest-cov
help by showing exactly which parts of your code need more tests. These coverage reports guide testing efforts to the most important areas.
Fostering Team Collaboration Through Pytest
Using pytest as your shared testing framework helps teams work better together. When everyone follows the same testing approach, it's easier to share knowledge and collaborate on testing.
For example, well-designed pytest fixtures can be reused across different parts of your project. This ensures consistent test setups and reduces duplicate work. Shared fixtures also make it simpler for team members to understand and contribute to each other's tests.
Training and Maintaining Testing Standards
As your team grows, keeping consistent testing practices becomes more important. Create clear guidelines for new team members that explain your pytest best practices and standards.
Regular test reviews and knowledge sharing sessions help reinforce good testing habits. When the whole team understands and values thorough testing, it becomes a natural part of your development process.
Optimizing Test Performance and Debugging
Improving test speed and debugging is essential to keep development flowing smoothly. Let's explore practical ways to optimize pytest tests and build effective debugging skills so your test suite provides fast, reliable feedback.
Identifying Performance Bottlenecks
Finding slow tests is the first step to fixing them. The -vv
flag in pytest shows how long each test takes to run. Tools like pytest-timeout
help catch tests that are taking too long by automatically failing them after a set time limit.
Techniques for Optimizing Pytest Performance
The key to faster tests is identifying what's slowing them down and applying targeted fixes. Two common speed issues are inefficient fixtures and slow external dependencies. With fixtures, use function scope by default since broader scopes can add overhead. For external systems like databases or APIs, tools like pytest-mock
let you replace real calls with quick simulated responses.
Here are key ways to speed up your tests:
- Smart Fixture Usage: Stick to function-scoped fixtures unless broader scope is truly needed
- Mock External Systems: Use
pytest-mock
to replace slow database/API calls with fast simulated responses - Run Tests in Parallel: For larger projects,
pytest-xdist
can run tests across multiple CPU cores to get results faster
Mastering Pytest Debugging Techniques
Strong debugging skills help you quickly find and fix test issues. The --pdb
flag in pytest automatically starts the debugger when tests fail, letting you inspect variables and program state. Most IDEs also integrate well with pytest's debugging features, giving you breakpoints and variable inspection right in your development environment.
Handling Flaky Tests
Tests that pass sometimes and fail others without code changes slow down development and reduce confidence. While tools like pytest-rerunfailures
can retry failed tests, this only masks the real issues. It's better to track down and fix the root causes, which often relate to timing or concurrency problems.
Scaling Your Testing Approach
As projects grow, testing needs change. Use pytest markers to group tests by feature or speed so you can run specific subsets during development. This focused approach keeps feedback quick while maintaining thorough test coverage.
For smoother test automation, consider using Mergify in your CI/CD pipeline. It helps manage pull requests and automates merging, letting you focus on writing solid tests and code.