Py Testing: A Professional's Guide to Mastering Python Testing
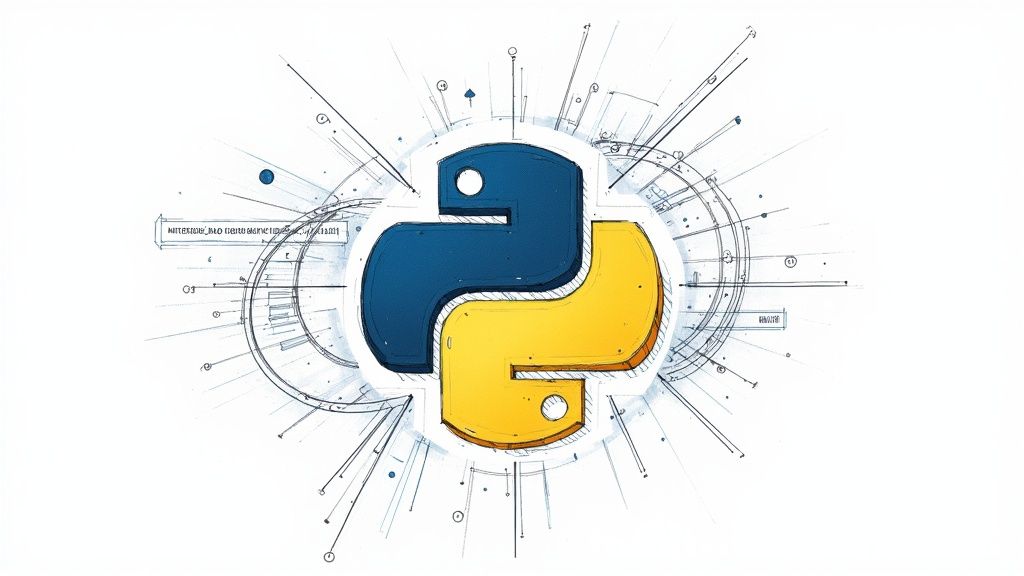
Mastering the Foundations of Py Testing
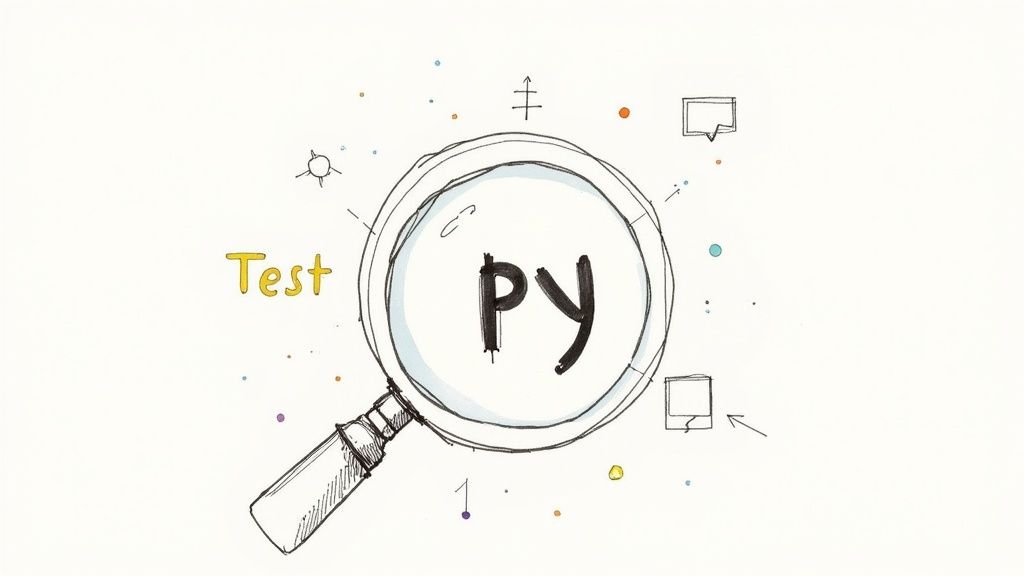
Writing good tests is essential for building reliable Python applications. It goes beyond just writing test code - you need to understand core testing principles and apply them consistently throughout development.
Building a Solid Test Case Strategy
Good test cases are focused and catch bugs before they reach users. Start by mapping out what could go wrong in your application. For an online store, you'd want to test things like order processing, inventory tracking, and payment handling.
Your test cases should include:
- Positive Tests: Check that features work correctly with valid inputs
- Negative Tests: Verify proper handling of invalid data and errors
- Edge Cases: Test boundary conditions and extreme scenarios
Structuring Your Py Testing Workflow
A clear testing process helps teams work together effectively, especially on bigger projects. Many developers use pytest for testing because it's straightforward and finds tests automatically based on naming patterns. This saves time compared to manual test configuration, particularly for complex codebases. To learn more about testing frameworks, check out this comparison of Pytest vs. Unittest.
A basic workflow includes:
- Test Setup: Preparing test data and environments
- Test Execution: Running the test suite
- Result Analysis: Investigating any failures
- Bug Fixing: Correcting issues and retesting
Maintaining Testing Discipline
Keeping up good testing habits gets harder as projects grow. Here are ways to stay consistent:
- Make Testing Part of Development: Write tests alongside code instead of as an afterthought. Test-Driven Development (TDD) can help build this habit.
- Use Continuous Integration: Set up automated testing when code changes. This catches problems quickly.
- Review Tests: Check test quality during code reviews to ensure proper test coverage.
By getting these basics right, you'll build a strong testing practice that improves code quality and reduces bugs. This foundation makes it easier to add more advanced testing approaches as your needs grow.
Building Your Professional Testing Environment
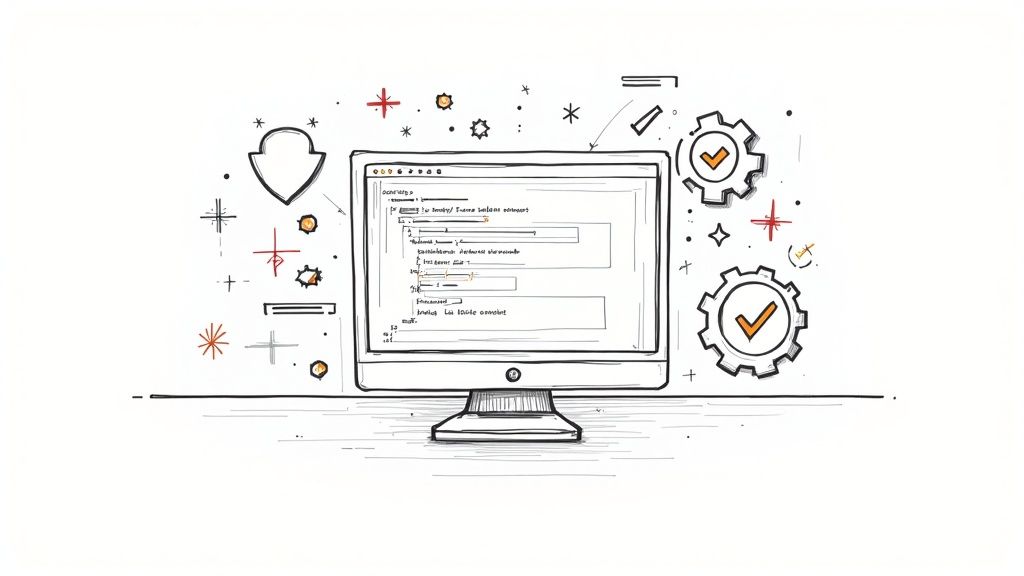
Setting up a solid Python testing environment requires more than just writing test cases. You need to establish practices that ensure your tests run consistently and reliably across different setups. Let's explore the key components of a professional testing environment.
Virtual Environments: Your Testing Sandbox
Think of virtual environments as clean rooms for your Python projects. They prevent package conflicts by keeping each project's dependencies separate. You can use Python's built-in venv or the popular virtualenv tool to create these isolated spaces. This separation helps you maintain a clean testing environment where updates to one project won't affect others.
Dependency Management: Keeping it Consistent
Managing project dependencies is crucial for reliable testing. Pip, Python's package installer, lets you specify exact versions of required libraries. Create a requirements.txt
file to list all dependencies - this simple text file helps team members recreate the exact same environment on their machines. It also makes setting up continuous integration pipelines much easier.
Cross-Platform Compatibility: Testing Everywhere
Most software needs to work on different operating systems. Docker containers let you test your code in environments that match your production setup. For example, you can run tests in a Linux container even while developing on Windows or Mac. This catches platform-specific bugs early, before they reach users.
Pytest: A Robust Foundation
Pytest stands out as a powerful testing framework for Python projects. It offers simple syntax, rich features, and many useful plugins. The framework requires Python 3.7 or newer, ensuring access to modern language features. From automatic test discovery to detailed error reports, pytest makes testing more efficient and helps catch bugs earlier. Learn more in the official pytest documentation.
Crafting Tests That Tell Your Code's Story
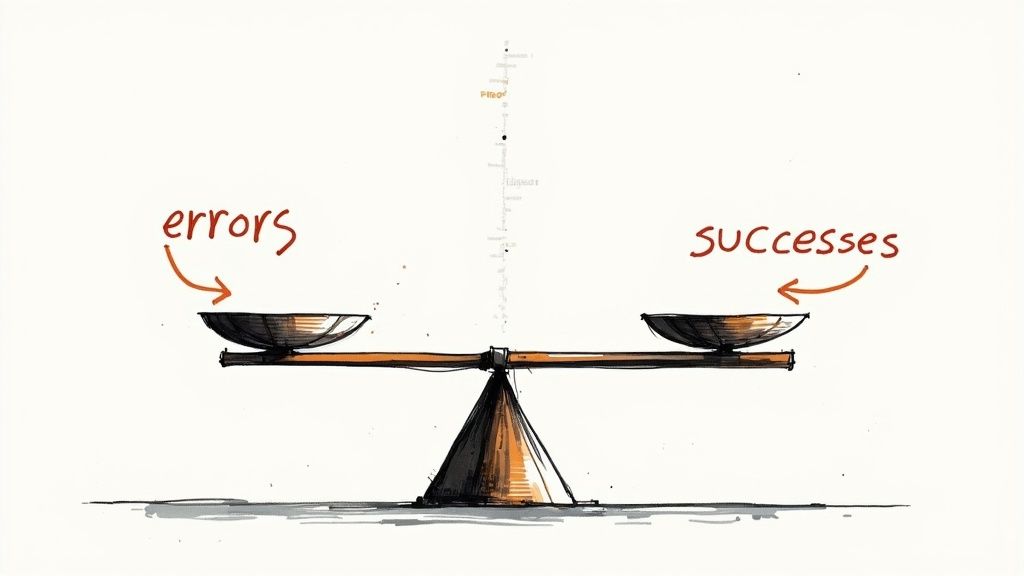
A good test suite does more than catch bugs - it shows other developers exactly how your code works. When you write tests thoughtfully, they become clear documentation that helps future maintainers (including yourself!) understand what each piece of code should do. This mindset makes testing feel less like a burden and more like an investment in your codebase.
Structuring Test Cases for Clarity
Good tests are like small stories that demonstrate one specific behavior at a time. Break each test into three clear parts:
- Arrange: Set up everything needed for the test - create objects, set variables, mock dependencies
- Act: Run the actual code you want to test
- Assert: Check if you got the results you expected, using tools like
assert
or pytest helpers
Here's a practical example testing a discount calculator:
def calculate_discount(price, discount_percentage):
... function logic ...
A clear test might look like:
def test_calculate_discount_positive(): # Arrange price = 100 discount_percentage = 10
# Act
discounted_price = calculate_discount(price, discount_percentage)
# Assert
assert discounted_price == 90
Managing Test Data Effectively
Using realistic test data helps catch real-world issues. Here are the key ways to handle test data well:
- Parameterization: Use pytest to run the same test with different inputs
- Fixtures: Create reusable test data setups
- Data Factories: Build helper functions to generate complex test objects
Writing Maintainable Assertions
Good assertions are specific and clear. They form the foundation of your test suite:
- Write precise checks that target exactly what you want to verify
- Don't repeat the same checks - move common assertions into helper functions
- Add helpful error messages - instead of
assert x == y
, writeassert x == y, f"Expected {x}, but got {y}"
Handling Edge Cases and Complex Scenarios
The trickiest bugs often hide in edge cases. Test these carefully:
- Check boundary values like zero, empty strings, and maximum sizes
- Verify error handling works correctly
- Use mocking with tools like unittest.mock to test complex interactions
Following these practices helps create a test suite that truly documents your code's behavior while catching bugs early. This makes your codebase much easier to maintain and update over time.
Leveling Up Your Testing Strategy
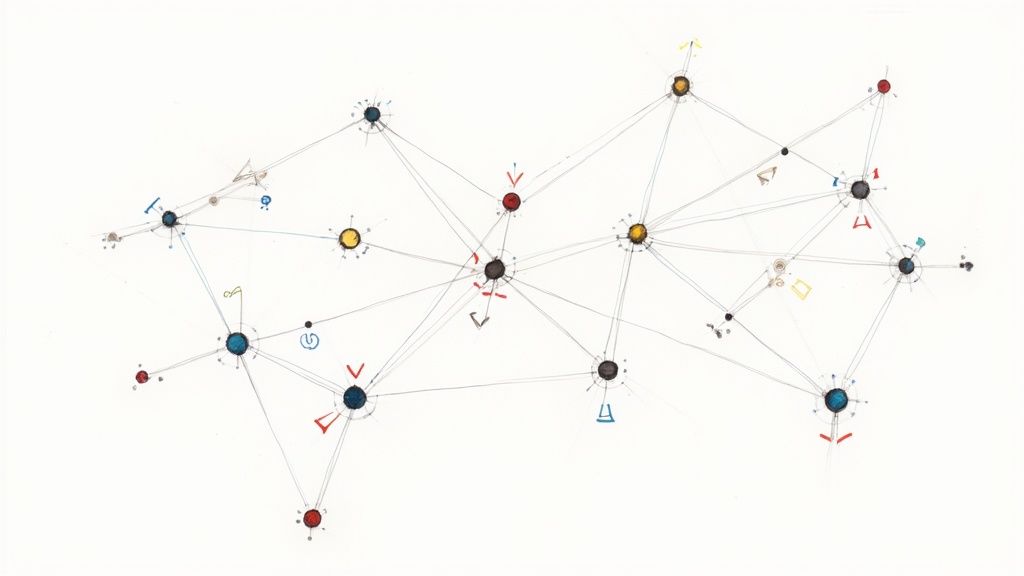
You've got the basics of Python testing down. Now let's explore some advanced techniques that will help you handle complex real-world testing scenarios more effectively.
Parameterized Testing: Exploring All Possibilities
When you need to test a function with many different inputs, writing separate test functions gets messy fast. Parameterized testing lets you run the same test with multiple values using Pytest's @pytest.mark.parametrize
decorator. Here's a simple example testing email validation:
import pytest
@pytest.mark.parametrize("email", [ "test@example.com", "invalid.email", "another@test.net" ]) def test_validate_email(email):
... test logic using the 'email' parameter ...
This approach tests three email formats in one clean function. Speaking of clean code, let's look at fixtures.
Fixtures: Setting the Stage for Success
Fixtures are like building blocks for your tests - they handle setup and cleanup so you don't have to repeat yourself. Need a database connection? Test data? Mocked services? Fixtures make it happen consistently. Your tests stay focused on what matters instead of getting bogged down in setup code.
Mocking: Isolating Components for Deeper Insights
External dependencies can make testing tricky. They might be slow, unreliable, or just hard to control. Mocking lets you simulate these dependencies so you can focus on testing your actual code. For example, rather than making real HTTP calls, you can mock the requests to test how your code handles different responses. Python's built-in unittest.mock
makes this straightforward.
Asynchronous Testing: Embracing Modern Python
If you're working with modern Python, you'll encounter lots of async
and await
code. Testing async functions needs special handling, but Pytest makes it simple with features like pytest.mark.asyncio
. This keeps your async tests flowing naturally with the rest of your test suite.
Continuous Testing: Integrating Testing into Your Workflow
Testing works best when it's part of your regular workflow. Continuous testing means running your tests automatically whenever code changes. Tools like Jenkins or GitLab CI can trigger your test suite with each push, catching issues early when they're easiest to fix.
Maintaining Performance at Scale
Big test suites can get slow. Pytest offers solutions like pytest-xdist
for parallel test execution, which can seriously speed things up. Popular plugins like pytest-split
help by dividing tests into balanced groups based on how long they take to run. Fast tests mean quick feedback, which encourages more regular testing and better code quality.
Implementing Testing Patterns That Scale
Building effective tests for growing Python projects requires careful planning and structure. As your codebase expands, you need test suites that can handle new requirements and increased complexity. Let's explore how successful teams build scalable testing approaches.
Test-Driven Development (TDD): A Design Philosophy
Test-driven development flips the traditional coding approach on its head - you write tests before writing any code. While this may seem backward, it pushes you to deeply consider your code's purpose from the start. Research from Microsoft found that TDD reduced defects by 15-35%.
Here's how TDD works with pytest:
- Write a test that defines the desired behavior (it will fail initially)
- Write just enough code to make the test pass
- Clean up and improve the code while keeping tests green
This cycle continues for each feature, building up your test suite naturally alongside your application.
Behavior-Driven Development (BDD): Making Tests Human-Readable
Behavior-driven development takes TDD further by focusing on user behavior. Tools like Behave and pytest-bdd let you write tests in plain English that describe how your application should work. This helps teams communicate better about testing needs.
Here's a BDD test example:
Scenario: User adds item to cart Given a user is on the product page When they click "Add to cart" Then the cart item count should increase by 1
Finding the Right Coverage Balance
While 100% test coverage sounds great, it's often impractical and costly for large projects. Focus your testing efforts on high-risk areas and core features that could seriously impact users if they break. Use coverage tools to spot gaps and guide your testing strategy.
Keeping Tests Current
Your tests need to adapt as your requirements change. Build flexibility into your test suite from the start. Using fixtures and parameterized tests helps make updates easier when needed. Remember that maintainable tests are just as important as good test coverage.
Adding Tests to Existing Code
Starting testing on an established project can feel overwhelming. Begin with the most critical code sections. Use coverage tools to identify where tests are most needed. Add TDD or BDD practices gradually as you build new features or fix bugs. Over time, this steady approach will improve your application's reliability.
Optimizing Your Testing Workflow
Testing should help you develop better code faster and with greater confidence. Building a testing workflow that runs smoothly and grows with your project is key. Here's how experienced teams achieve this.
Identifying and Fixing Performance Bottlenecks
A slow test suite can be a major roadblock. When tests take too long to run, developers avoid running them frequently. Start by finding what's causing delays. Tools like pytest-cov help spot untested code that might be slowing things down, while pytest-split groups tests by runtime to help identify slow performers.
Look for patterns in slow tests. Database tests often need better connection handling or query optimization. Tests calling external services usually speed up dramatically with proper mocking.
Managing Test Dependencies Effectively
Keeping dependencies in check is crucial for reliable testing. Use pip and maintain a clear requirements.txt
file so everyone runs tests with matching library versions. This prevents confusing errors from mismatched dependencies.
Virtual environments keep your project's dependencies separate from other projects and system packages. This is especially helpful when working with multiple Python versions.
Maintaining Reliable Test Suites at Scale
As your codebase grows, keeping tests reliable becomes harder. Flaky tests that pass and fail randomly are especially frustrating - they waste time and erode trust in your test suite. Tools like pytest-rerunfailures can help spot inconsistent tests, but you need to fix the root cause.
Practical Approaches for Handling Flaky Tests
Take a methodical approach to fix flaky tests:
- Reproduce the failure consistently
- Check for timing issues in async code
- Look for resource leaks
- Test external service dependencies
- Add detailed logging
- Use debuggers when needed
- Profile performance bottlenecks
Efficient Debugging and Optimized Execution Times
Good debugging tools make a huge difference. Strategic print statements or logging help track variable values and code flow. pytest's error messages often point directly to the problem. To speed up test runs:
- Write efficient test code
- Use smart data structures
- Run tests in parallel with pytest-xdist
With these improvements, testing becomes an asset rather than a burden. A well-tuned test suite leads to better code, faster development, and more confident releases.
Want to improve your development workflow even more? Mergify automates your merge process to reduce CI costs and save developer time.