Minitest Ruby: Fast & Reliable Testing
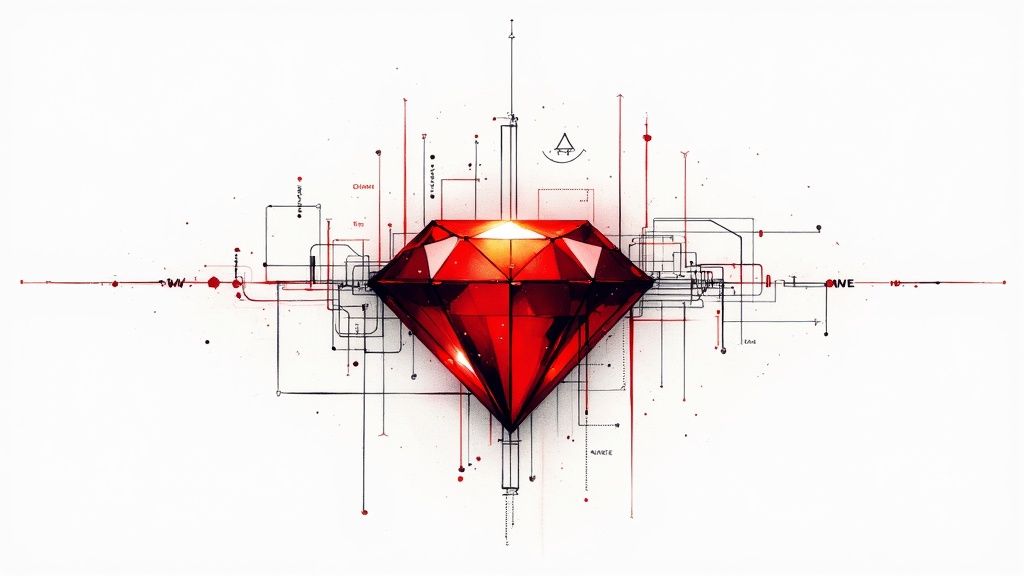
Why Minitest Ruby Wins the Testing Framework Battle
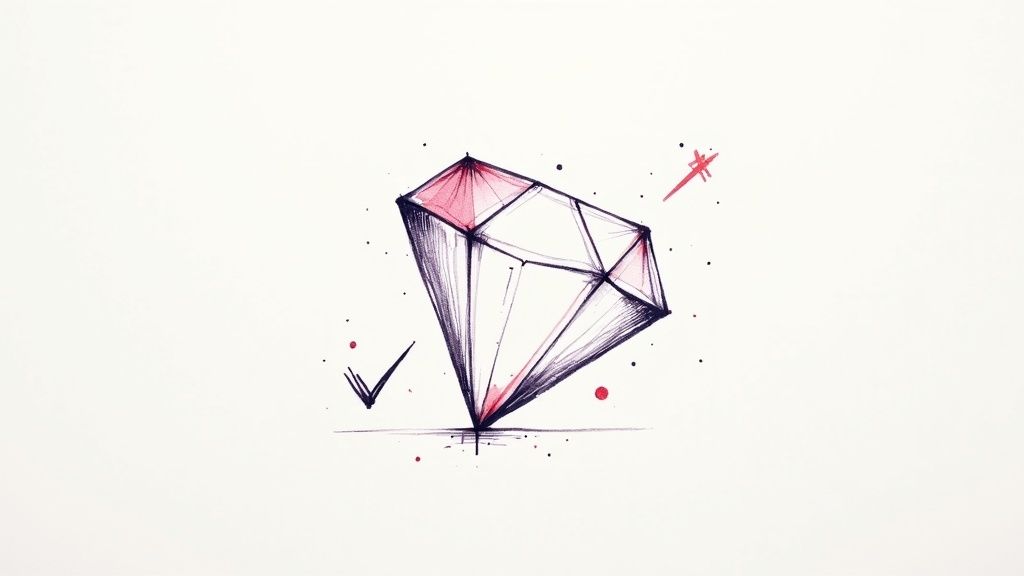
When building reliable and maintainable Ruby applications, choosing the right testing framework is paramount. While many options are available, Minitest Ruby stands out, solidifying its position as the default testing framework for Ruby on Rails. But what makes it so popular?
One key factor is its simplicity. Minitest has a minimalist design, offering a clear and straightforward API. This allows developers to write tests efficiently, concentrating on their code's logic without getting lost in complex framework details. This straightforward approach also means a shorter learning curve, allowing new team members to become proficient quickly.
Minitest's effectiveness also comes from its close integration with Ruby. It follows Ruby's "principle of least surprise," meaning it behaves predictably and aligns with the language's conventions. This makes Minitest code easy to read, understand, and maintain, even in larger projects. This compatibility lets developers use their existing Ruby knowledge, reducing the effort required to learn a new framework.
Furthermore, Minitest offers comprehensive testing capabilities for Ruby and is included with Rails by default. It supports testing for Ruby versions as old as 2.5 thanks to Rails' backward compatibility. This broad support ensures developers using older versions can still rely on Minitest. Minitest supports both assertion-style and spec-style tests, catering to a variety of testing preferences. Discover more insights about Minitest here.
The Benefits of Speed and Maintainability
Minitest's lightweight design results in faster test execution. This speed is crucial for a rapid development cycle, providing developers with quick feedback on changes. Faster tests encourage more frequent testing, leading to earlier bug detection and, ultimately, better code quality.
Embraced by the Community
Minitest's position as the default testing framework for Rails further cements its place in the Ruby ecosystem. This widespread use means abundant resources, tutorials, and community support are available, providing a solid foundation for developers seeking help or guidance on best practices.
From Zero to Testing Hero With Minitest Ruby
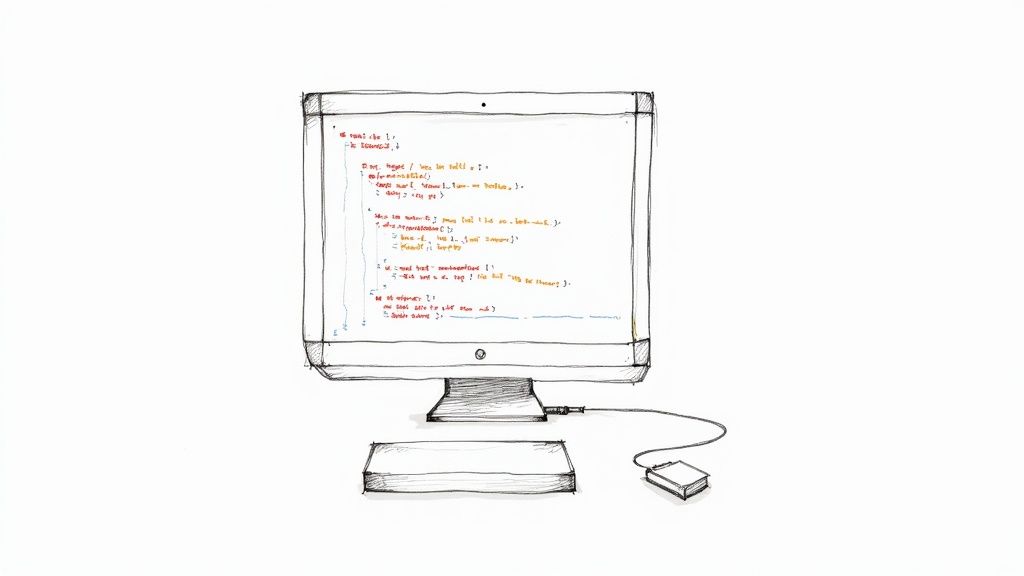
This section offers a practical guide to implementing Minitest Ruby. We'll explore real-world setup examples for both standalone Ruby applications and Rails projects, giving you the tools and techniques to improve your testing effectiveness.
Setting Up Minitest for Standalone Ruby Applications
Setting up Minitest for projects outside of Rails is surprisingly straightforward. Begin by ensuring the minitest
gem is installed. You can add it to your Gemfile
within the :development
and :test
groups, or install it directly using the command gem install minitest
.
Once the gem is installed, create a test file. The convention is to name it test_your_class.rb
. Inside this file, require minitest/autorun
.
require 'minitest/autorun'
class MyTests < Minitest::Test def test_my_method # Your assertions here end end
Using minitest/autorun
allows for automatic test discovery and execution. This streamlined approach allows you to start testing quickly and efficiently. Next, let's look at integrating Minitest into a Rails project.
Integrating Minitest in Rails Projects
Minitest is the default testing framework in Rails. Tests reside in the test
directory, categorized into several types: unit, integration, system, and others. This structure helps organize your tests by scope and purpose.
For example, a unit test for a User
model would typically reside in test/models/user_test.rb
. Rails's built-in structure and conventions provide a strong foundation for building a comprehensive test suite.
Organizing Your Tests: Structure and Naming
Organizing your tests effectively is vital, particularly as your project grows. Descriptive file names and class names that clearly reflect the component under test are crucial. For instance, tests for the User
model should use the filename user_test.rb
and the class name UserTest
.
Inside each test class, group related tests together within methods. If you're testing validations on the User
model, group email validation tests together and username validation tests together. Clear naming and grouping enhance readability and maintainability.
Running and Troubleshooting Minitest Tests
Running your tests is simple. From the project's root directory, execute the command rails test
. Minitest provides detailed output, showing the status of each test – whether it passed, failed, or was skipped – and any error messages. This information helps you quickly identify and resolve issues.
Troubleshooting test failures involves analyzing the error messages and backtraces that Minitest provides. This information pinpoints the failing line of code and explains the reason for the failure. Understanding these reports is key to efficient debugging.
By following these practices, you'll be well on your way to building a robust and maintainable test suite using Minitest Ruby, ultimately ensuring the quality and reliability of your Ruby applications. This solid foundation in Minitest will boost your testing confidence and efficiency.
Minitest vs RSpec: The Truth Beyond the Hype
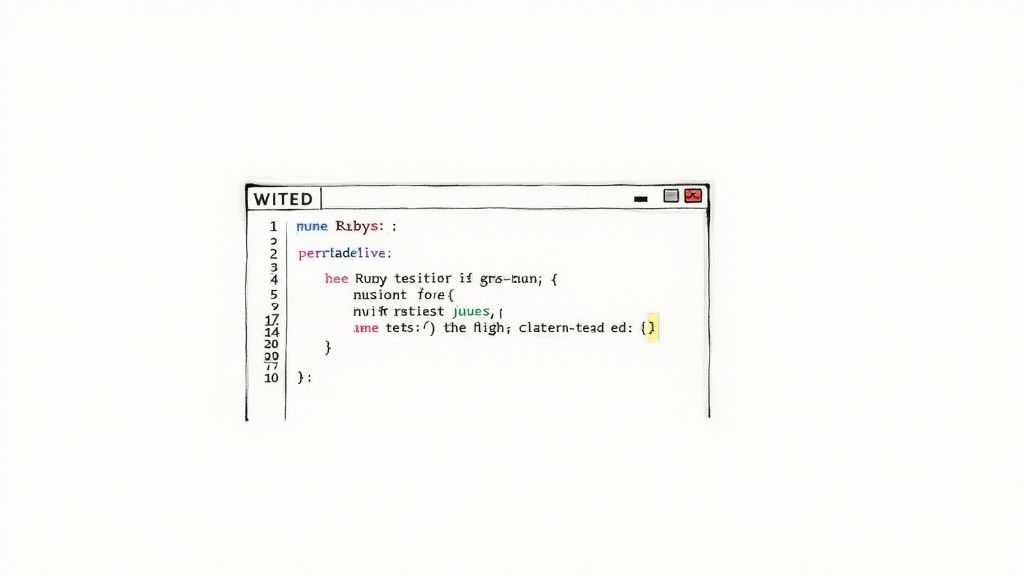
Choosing the right testing framework for your Ruby projects can be tricky. Two popular contenders, Minitest and RSpec, often spark debate among developers. This section provides a practical comparison to help you decide which framework best suits your team and project.
Core Differences: Simplicity vs. Robustness
Minitest is known for its simplicity. It encourages a lean testing approach, leading to faster test execution and quicker feedback during development. Its smaller codebase also simplifies debugging and maintenance. However, this simplicity can be a double-edged sword, sometimes feeling limited when faced with complex testing scenarios.
RSpec, conversely, is known for its rich features and descriptive syntax. RSpec tests are highly readable and expressive, often resembling natural language. This is particularly beneficial for complex projects using behavior-driven development (BDD). This robustness, however, can introduce complexity and potentially slower test execution.
Performance Considerations: Speed vs. Features
Minitest's efficiency stems from its compact size – around 1500 lines of code. This is significantly less than RSpec's over 12,000 lines. This lean codebase contributes to its speed, making it a popular choice for developers who prioritize rapid feedback. This is invaluable for teams running tests constantly throughout development. Despite its smaller size, Minitest still supports essential features like mocking and benchmarking, crucial for test-driven development (TDD). For more detailed Minitest statistics, check out this resource.
RSpec's extensive features, while enabling detailed testing, can lead to slower execution times, especially as the test suite grows. This performance difference becomes more noticeable as your project scales. However, if test readability and thoroughness outweigh raw speed, RSpec's features might be the preferred trade-off.
To further illustrate the key differences, let's look at a comparison table:
The following table provides a detailed comparison of key features, syntax differences, and performance metrics between Minitest and RSpec.
Feature | Minitest | RSpec | Winner |
---|---|---|---|
Syntax | Simple, traditional | Descriptive, domain-specific language (DSL) | RSpec |
Speed | Faster | Slower | Minitest |
Size | Smaller (~1500 lines of code) | Larger (~12,000 lines of code) | Minitest |
Learning Curve | Easier | Steeper | Minitest |
Features | Fewer, focused on core testing needs | More extensive, supports BDD | RSpec |
Flexibility | Less flexible | More flexible | RSpec |
As this table highlights, Minitest excels in speed and simplicity while RSpec offers a richer feature set and more expressive syntax.
Practical Implications: Team Dynamics and Project Scale
Onboarding new team members is generally faster with Minitest due to its simpler API. This is a significant advantage for teams with high turnover. Maintaining a Minitest suite is also often easier thanks to its reduced complexity. However, RSpec's detailed syntax can make understanding test intent easier, particularly in larger projects.
As projects grow, RSpec’s descriptive syntax and features can help manage complexity. While Minitest’s simplicity shines in smaller projects, maintaining its effectiveness in larger projects requires more discipline and organization. The best choice ultimately depends on your team, project size, and specific requirements.
Advanced Minitest Ruby Techniques That Actually Work
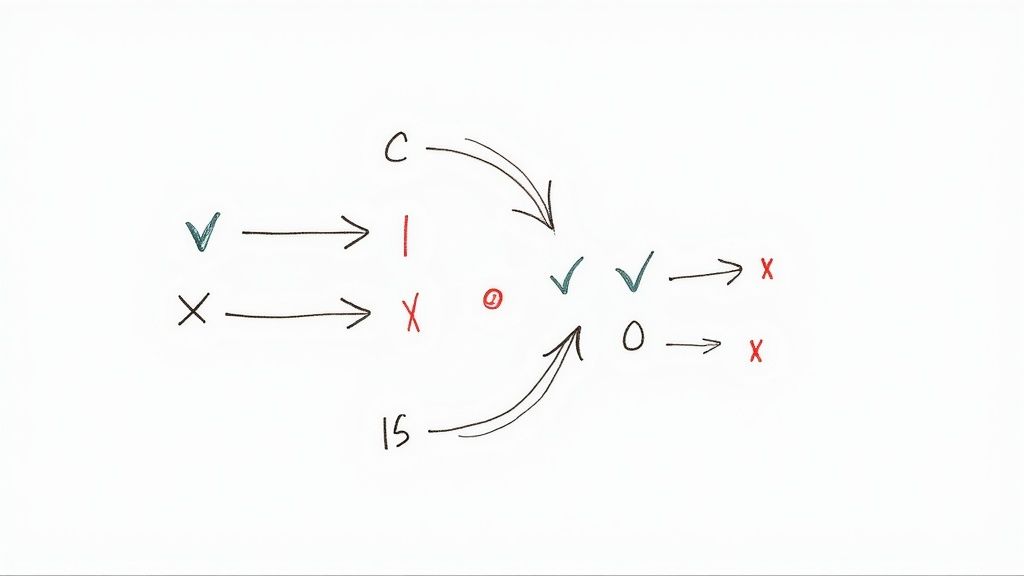
Building on the basics of Minitest Ruby, let's explore some advanced techniques for creating robust and efficient test suites. These techniques, commonly used by seasoned Ruby developers, help tackle complex testing scenarios, simplify debugging, and improve test performance.
Mastering Asynchronous Operations Testing
Testing asynchronous operations in Ruby can present unique challenges. Fortunately, Minitest Ruby provides tools to streamline this process. A common method is using Minitest::Mock to simulate responses from asynchronous calls. This effectively isolates the code being tested, ensuring predictable and controlled testing.
For instance, when testing code that interacts with external APIs, mocking the API responses eliminates dependencies on network availability or API stability. This isolation boosts test execution speed and leads to more reliable results.
Managing Database State Effectively
Consistent database state during testing is critical to avoid unintended consequences. One effective strategy is using database transactions. Wrapping your tests within a transaction ensures that any database changes are rolled back after each test is completed. This keeps your database clean and ensures each test begins with a known state.
Furthermore, tools like database_cleaner integrate seamlessly with Minitest to further improve database state management. These tools provide finer control over the cleanup process, allowing for efficient and reliable cleanup between tests.
Validating Complex Object Relationships
Testing complex object relationships frequently requires validating the integrity of associations and dependencies. Minitest offers a variety of assertions to simplify this task. You can assert the existence or absence of particular associations, ensuring your models interact correctly.
Consider using custom assertions to encapsulate common validation logic. This enhances code readability and makes tests more descriptive. This, in turn, makes the project easier to maintain as it evolves and object interactions increase in complexity.
Crafting Custom Assertions and Matchers
Minitest’s flexibility allows you to create custom assertions and matchers. Custom assertions help encapsulate specific validation logic for your application. This improves test clarity and maintainability.
Matchers provide a more expressive way to define expected outcomes. They are especially helpful when working with complex data structures or comparisons. This avoids complex conditional logic within your tests. Mocking is a key Minitest feature, vital for isolating dependencies during unit tests. Minitest’s mocking capabilities allow you to simulate responses from external services, ensuring test independence. This is especially useful in web development when integrating with external APIs. For example, you can test a payment gateway integration without interacting with the actual gateway by mocking its responses. Learn more about this here.
Leveraging Minitest Plugins Strategically
Minitest plugins extend core functionality for specific testing needs. However, choose plugins wisely. While they can add value, excessive use can lead to unnecessary complexity and dependencies.
Focus on areas where plugins provide significant benefits, such as improving test output, adding specialized assertions, or integrating with other tools. Carefully assess the potential impact on your test suite’s maintainability and performance before incorporating any plugin.
Optimizing Test Performance
As your test suite grows, performance becomes increasingly important. Minitest offers several techniques to optimize test execution speed. One method is parallelizing tests, allowing them to run concurrently. This drastically reduces overall testing time, especially for large suites.
By using these advanced techniques, you can significantly improve your Minitest Ruby skills. You'll be able to create test suites that are not only comprehensive and effective but also maintainable and performant. This results in higher quality code, quicker development cycles, and a more reliable development process.
Supercharging CI/CD Pipelines With Minitest Ruby
Integrating Minitest Ruby into your Continuous Integration/Continuous Delivery (CI/CD) pipeline is key to a smooth development workflow and high-quality code. This goes beyond simply running tests; it involves optimizing Minitest for speed, creating useful reports, and automating quality checks.
Parallelizing Tests for Faster Feedback
A crucial optimization technique for your CI/CD pipeline is test parallelization. By running multiple tests at the same time, you can significantly reduce build times. Minitest supports parallelization, distributing tests across multiple processes or threads. This is especially helpful for large test suites, where running tests one after another can be slow. However, be mindful of test flakiness—tests that intermittently fail due to dependencies or shared resources. Proper setup and isolation are essential for reliable parallel testing.
Generating Actionable Test Reports
Understanding test results and pinpointing problems in your codebase requires effective reporting. Minitest offers various reporting options, including detailed reports in formats like HTML or XML. These reports offer insights into test execution time, failure rates, and code coverage, letting your team monitor quality trends. You can also integrate custom reporters designed for your specific CI/CD environment. For example, create reports optimized for web dashboards or send results directly into CI platforms like Jenkins. This reporting flexibility allows Minitest to fit different workflows. The Minitest::StatisticsReporter class further enhances this flexibility. While not a direct report generator, it collects crucial test run statistics. Extend this class to create custom outputs like HTML for dashboards or XML for Jenkins. Learn more about the Minitest::StatisticsReporter here.
Automating Quality Gates for Enhanced Reliability
To maintain code quality and prevent regressions, implement automated quality gates in your CI/CD pipeline. These gates are checkpoints, ensuring that specific criteria are met before merging or deploying code. Minitest lets you configure gates based on various metrics:
- Code Coverage: Require a minimum level of test coverage to avoid shipping untested code.
- Performance Metrics: Set acceptable limits for test execution time to catch performance slowdowns early.
- Regression Detection: Automatically identify and flag failing tests that suggest newly introduced bugs.
Automating these checks makes releases more reliable and improves the overall development process.
Structuring Test Suites for Optimal Feedback
How you structure your Minitest test suites greatly affects the feedback you receive. Organize tests by scope and purpose—create separate suites for unit tests, integration tests, and system tests. This allows you to focus on specific parts of your codebase and understand how individual components and their interactions behave. Strategically placing tests within your pipeline gives you the most relevant information at each stage of development. For instance, running fast unit tests early provides rapid feedback during development, while more thorough integration tests, performed later, validate overall system behavior. This layered approach ensures efficient identification and resolution of issues. Organizing tests by code modules or functionality improves the maintainability of your test suite as your project grows.
Minitest Ruby Best Practices From The Trenches
Building a robust test suite with Minitest Ruby isn't just about writing tests; it's about building a maintainable and efficient system that scales with your codebase. This involves applying best practices learned from developers managing large Minitest suites.
Organizing Tests for Scalability
A well-organized test suite is essential for long-term maintainability. Think of your tests like a library: easy navigation is key. Use a consistent folder structure that mirrors your application's architecture. For example, model tests reside in test/models
, controllers in test/controllers
, and so on. This makes it easier to find the tests related to your code changes. Furthermore, descriptive file and class names clearly identify the component under test. A User
model should have a user_test.rb
file and a UserTest
class. This clarity helps you quickly understand each test file's purpose.
Managing Test Data Effectively
Managing test data properly is crucial for reliability. Test pollution, where one test affects another's outcome, is a common problem. Isolate your tests using techniques like database transactions or tools like Database Cleaner. This ensures a clean slate for every test, minimizing unexpected side effects. Think of it like cleaning lab equipment between experiments – it ensures accurate results.
Keep your setup code concise and focused. Abstract common setup logic into helper methods or shared contexts to reduce code duplication and simplify test maintenance.
Writing Tests That Validate Behavior, Not Implementation
Testing should validate your code's behavior, not its implementation. This allows your code to evolve without constantly rewriting tests. Focus on testing the public interface of your classes and methods, not the internal workings. Avoid directly testing private methods, as these are more likely to change. This keeps your tests relevant even when the underlying implementation details change.
Balancing Coverage and Maintainability
While high test coverage is desirable, it shouldn't compromise maintainability. 100% coverage might seem ideal, but a brittle and hard-to-update test suite loses its value. Focus on testing critical paths and complex logic. Consider the trade-offs between coverage and maintenance time. A smaller, well-maintained test suite is often more effective than a large, unwieldy one.
Leveraging Minitest Tools and Plugins
Minitest offers built-in tools and a growing ecosystem of plugins. Use mocks and stubs to isolate dependencies and control external behavior. Use plugins judiciously, ensuring they add value without unnecessary complexity. Choose tools that streamline your workflow and improve test quality.
To summarize the key takeaways, here's a table highlighting Minitest best practices:
A detailed table outlining Minitest best practices can help consolidate this information:
Table: Minitest Ruby Best Practices Description: Summary of key best practices for writing effective Minitest Ruby tests, organized by category
Category | Practice | Why It Matters | Implementation Example |
---|---|---|---|
Organization | Mirror application structure | Easy navigation, finding tests quickly | test/models/user_test.rb |
Data Management | Isolate tests (transactions, database_cleaner ) |
Prevent test pollution, reliable results | setup { DatabaseCleaner.start } |
Test Focus | Validate behavior, not implementation | Resilient tests, allows code evolution | Test public interfaces |
Coverage vs. Maintainability | Prioritize critical paths | Avoid brittle tests, maintain a healthy test suite | Focus on complex logic |
Tooling | Use mocks, stubs, plugins | Isolate dependencies, enhance workflow | mock.expects(:some_method) |
This table provides a quick reference guide for implementing these best practices. By following these guidelines, you can create a more robust and maintainable Minitest suite.
By following these best practices, you can create a Minitest suite that not only catches bugs effectively but also acts as a valuable asset in your development process. A well-crafted test suite provides a safety net, allowing you to make code changes with confidence, ultimately leading to a more robust and reliable application.
Ready to improve your CI/CD pipeline? Mergify streamlines your code integration process, optimizing CI costs and reducing developer frustration. Learn more and see how it can benefit your team.