Master Minitest: Essential Ruby Testing Techniques
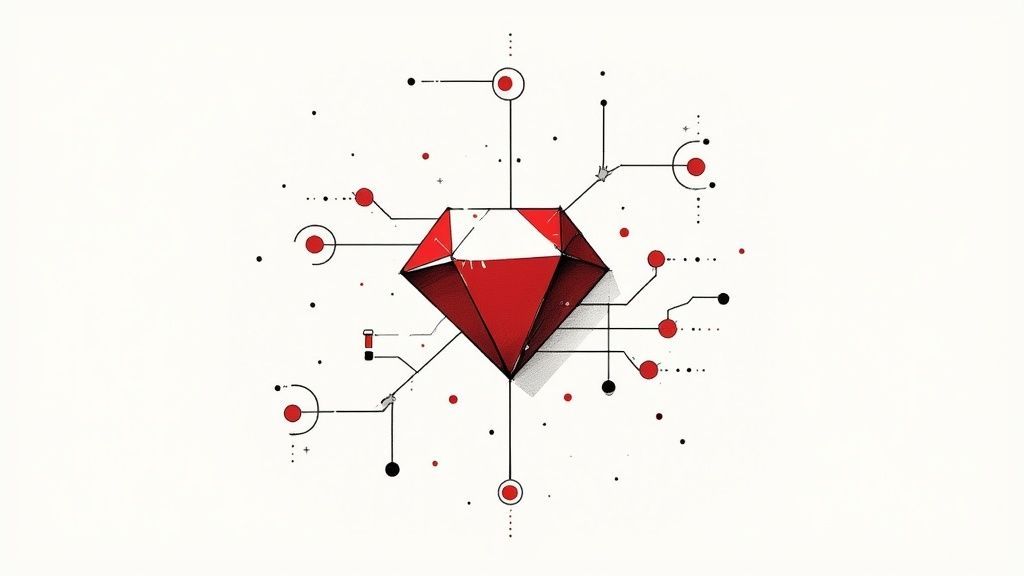
Why Minitest Wins the Ruby Testing Game
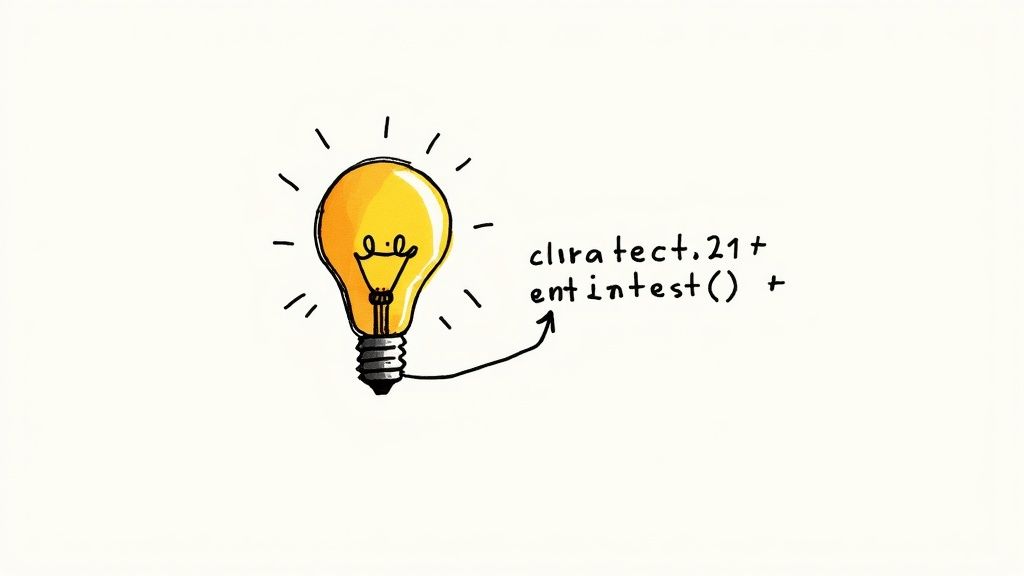
In the world of Ruby development, efficient testing is crucial. Minitest excels in this area. Its minimalist design offers considerable benefits, allowing developers to write effective tests rapidly. Many experienced Ruby developers appreciate Minitest's straightforward approach, particularly when managing large test suites. This efficiency leads to faster development cycles and more immediate feedback. Understanding how testing saves money underscores Minitest's importance in your workflow. For a deeper look at this, check out this resource on Software Testing.
Minitest's speed and simplicity aren't its only advantages. This framework also enjoys widespread adoption across various projects, from nimble startups to large-scale Rails applications. Many developers who try other testing frameworks often return to the familiar efficiency and clarity of Minitest. Its continued popularity demonstrates its effectiveness and ease of use. Moreover, Minitest's core philosophy aligns perfectly with sustainable test-driven development.
Minitest: A Deep Dive into Simplicity and Speed
Minitest achieves its speed and simplicity through several key design choices. First, it offers a comprehensive set of assertions that are both powerful and easy to grasp. This lets developers write concise and readable tests, reducing time spent deciphering complex testing logic.
Secondly, Minitest is lightweight with minimal dependencies. This minimizes overhead and contributes to its quick execution speed, vital for rapid feedback during development. Finally, Minitest is included in Ruby’s standard library. This means it’s readily available without requiring additional installations.
Minitest is a fast and simple testing framework for Ruby, providing a robust set of assertions for clean, readable tests. It supports both unit tests and specifications, with features like mocking and benchmarking. The 5.25.1 release on August 16, 2024, addressed incompatibility issues and reverted some changes for improved compatibility. This release demonstrates Minitest's ongoing commitment to providing stable and compatible testing solutions for Ruby developers. You can delve into its history here. Minitest's extensive use in Ruby projects highlights its effectiveness in Test-Driven Development (TDD) and Behavior-Driven Development (BDD). For instance, its inclusion in Ruby's standard library makes it a popular choice for testing Ruby applications.
Why Developers Choose Minitest
- Reduced Cognitive Load: Minitest's straightforward syntax and clear assertions make tests easier to write, read, and understand. This simplifies maintaining a comprehensive test suite.
- Fast Execution: Minitest's lightweight design results in quicker test execution, providing faster feedback and development cycles.
- Seamless Integration: Being part of the Ruby standard library ensures Minitest integrates seamlessly into Ruby projects, streamlining setup and minimizing dependencies.
- Sustainable TDD: Minitest's design supports sustainable test-driven development practices, simplifying writing tests first and maintaining them over time.
Minitest's focus on simplicity and speed doesn't compromise its capabilities. Its powerful features allow developers to write effective tests for various scenarios, leading to higher-quality code and a more efficient development process.
From Zero to Testing Hero With Minitest
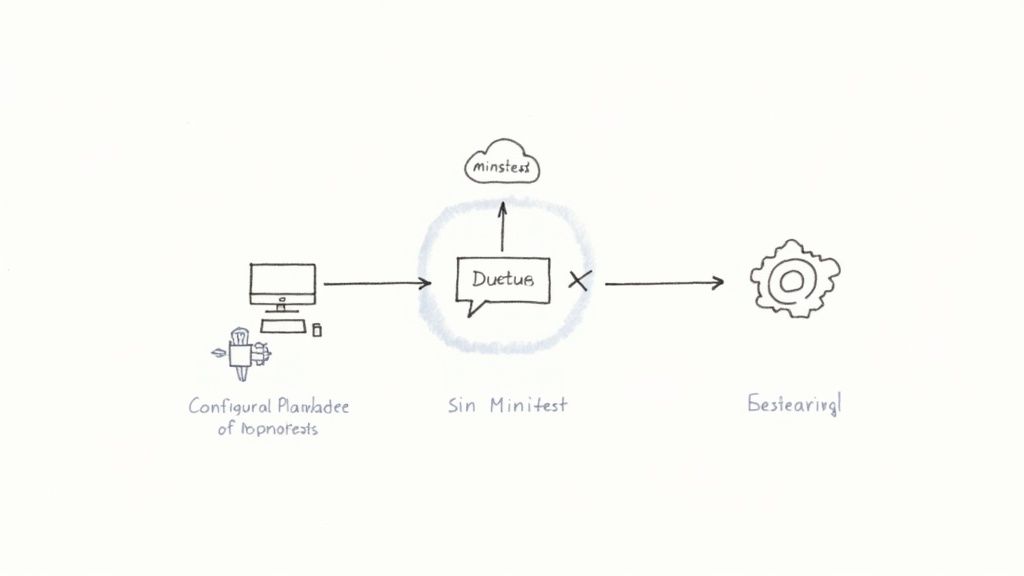
Starting with a new codebase and implementing tests can feel overwhelming. This section will guide you through using Minitest, from installation to building a robust test suite. You'll learn how to structure tests effectively and write meaningful tests that clearly communicate their purpose. These skills are crucial for maintainable code and efficient collaboration.
Installing and Setting Up Minitest
One of the best things about getting started with Minitest is its built-in inclusion with Ruby. This makes the initial setup incredibly convenient. However, if you're working with an older project, it's a good idea to check your Minitest version to make sure it's up-to-date. This ensures you have the latest features and bug fixes. Run gem list minitest
for a quick version check.
Organizing Your Minitest Files
Maintaining a well-organized structure for your test files becomes vital as your project expands. Experienced Ruby developers often mirror their application's structure within their test directory. This mirroring approach enhances navigation and maintainability. For example, tests for the User
model would typically reside in test/models/user_test.rb
. This logical structure makes locating and managing your tests much easier as your project grows.
Writing Your First Minitest
Let’s dive into writing your first test. Minitest offers a straightforward yet powerful syntax. You'll create a test file (for instance, user_test.rb
) and define a class that inherits from Minitest::Test
. Within this class, you define your test methods, each prefixed with test_
. These methods will contain assertions to verify your code's behavior.
require 'minitest/autorun'
class UserTest < Minitest::Test def test_user_creation user = User.new(name: "John Doe") assert_equal "John Doe", user.name end end
Test/Unit vs. Spec Style: Choosing Your Approach
Minitest supports two main testing styles: test/unit and spec. Test/unit focuses on testing individual components of your code in isolation. Spec style, influenced by RSpec, emphasizes behavior-driven development. The best choice depends on your team's preferences and project requirements. Understanding both allows you to select the style that best fits your needs.
Effective Test Naming: Communicating Your Intent
Test names should clearly convey the behavior under test. Descriptive names make it easier for other developers (and your future self) to understand each test's purpose. This clarity simplifies debugging and maintenance. For instance, test_user_is_valid_with_valid_attributes
is much more informative than test_1
.
The following table summarizes several common Minitest assertion methods:
Minitest Assertion Methods Comparison
This table provides a comparison of common assertion methods in Minitest, showing their syntax, purpose, and example usage to help developers choose the right assertions for their tests.
Assertion Method | Purpose | Example | When to Use |
---|---|---|---|
assert_equal(expected, actual) |
Checks if two values are equal. | assert_equal 2, 1 + 1 |
Verifying calculations, return values, etc. |
assert_nil(object) |
Checks if an object is nil. | assert_nil User.find_by(id: 999) |
Testing for non-existent records, etc. |
assert_includes(collection, object) |
Checks if a collection includes an object. | assert_includes [1, 2, 3], 2 |
Verifying membership in arrays, etc. |
assert_raises(exception_class) { ... } |
Checks if a specific exception is raised. | assert_raises(ActiveRecord::RecordNotFound) { User.find(999) } |
Testing exception handling. |
assert_instance_of(klass, object) |
Check if an object is an instance of a given class. | assert_instance_of User, User.new |
Verifying object types. |
This table provides a handy reference for common Minitest assertions. Choosing the right assertion makes your tests more readable and effective.
Establishing Sustainable Testing Patterns
Building sustainable testing patterns from the outset is essential. Tests should evolve alongside your codebase, not become a burden. Adopting clear naming conventions, organized file structures, and a suitable testing style can ensure your tests remain a valuable asset throughout your project's lifecycle. This proactive approach helps prevent technical debt and promotes a healthier, more maintainable project.
Minitest vs. RSpec: The Testing Framework Showdown
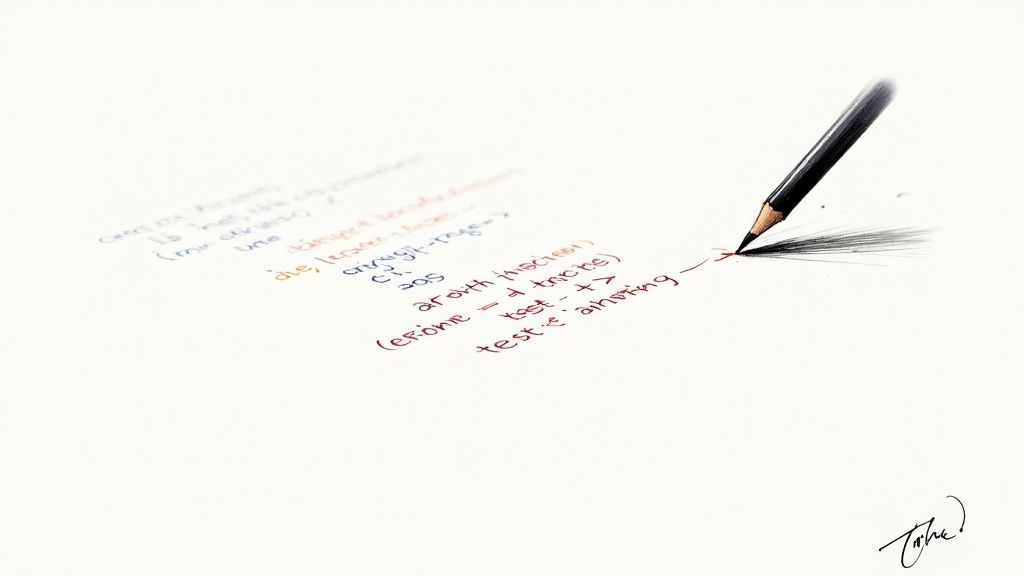
While our previous discussion focused on using Minitest in practice, let's broaden our view of Ruby testing. We'll compare Minitest and RSpec, another popular framework, to help you select the right tool for your projects. This comparison goes beyond mere syntax differences, exploring the core philosophies and long-term impacts of each.
Performance and Scalability
As your projects expand, your test suite's performance becomes crucial. Minitest's minimalist design often results in faster execution speeds. This difference is particularly noticeable in large projects.
RSpec, however, offers a rich feature set and descriptive syntax for more comprehensive testing. In some situations, thorough testing might outweigh raw speed. The key is understanding your project's specific needs and priorities.
Maintenance and Learning Curve
Minitest's simplicity often means a shorter learning curve and easier maintenance. Its straightforward syntax and minimal dependencies make it easy to grasp and modify.
RSpec, while powerful, has a steeper initial learning curve. Its domain-specific language (DSL) takes time to master. However, once understood, it can lead to more readable and expressive tests.
Community and Ecosystem
Both Minitest and RSpec boast active communities and robust ecosystems. Minitest, being part of the Ruby standard library, enjoys wide availability and compatibility. Ruby ensures that Minitest is readily accessible.
RSpec has a large and dedicated community, offering a wealth of extensions and resources. This active community ensures strong support for the framework. RSpec boasts a vibrant and helpful community.
Choosing the Right Framework
Choosing between Minitest and RSpec isn't about declaring a winner. The decision hinges on factors like your project's size, team experience, testing philosophy, and performance needs.
For example, a smaller project prioritizing speed might benefit from Minitest’s efficiency. A larger project with an RSpec-experienced team might prefer its descriptive capabilities. Ultimately, the best choice aligns with your specific needs.
To help summarize the key differences, let's look at a comparison table:
The following table, "Minitest vs. RSpec Feature Comparison," provides a comprehensive look at the features, syntax, performance, and ecosystem support of both frameworks. This information is designed to help developers make informed decisions when choosing the right testing tool for their project.
Feature | Minitest | RSpec | Notes |
---|---|---|---|
Syntax | Simple, Ruby-like | Domain-Specific Language (DSL) | Minitest feels more like native Ruby code. RSpec has its own distinct syntax. |
Performance | Generally faster | Can be slower for large test suites | Minitest's simplicity often results in quicker execution times. |
Learning Curve | Easier to learn | Steeper initial learning curve | RSpec's DSL requires more time and effort to master. |
Community | Part of Ruby standard library | Large and active community | Both frameworks enjoy robust support ecosystems. |
Maintenance | Simpler to maintain | Can require more effort for larger test suites | Minitest's straightforward nature contributes to its ease of maintenance. |
As the table illustrates, both Minitest and RSpec offer distinct advantages. Minitest excels in simplicity and speed, while RSpec shines in expressiveness and community support.
Minitest vs. RSpec: A Summary
The key takeaway is to select the most effective framework for your specific needs and team dynamics. The goal isn’t picking the "best" framework, but the one that best suits your particular project.
Minitest Power Techniques You're Not Using Yet
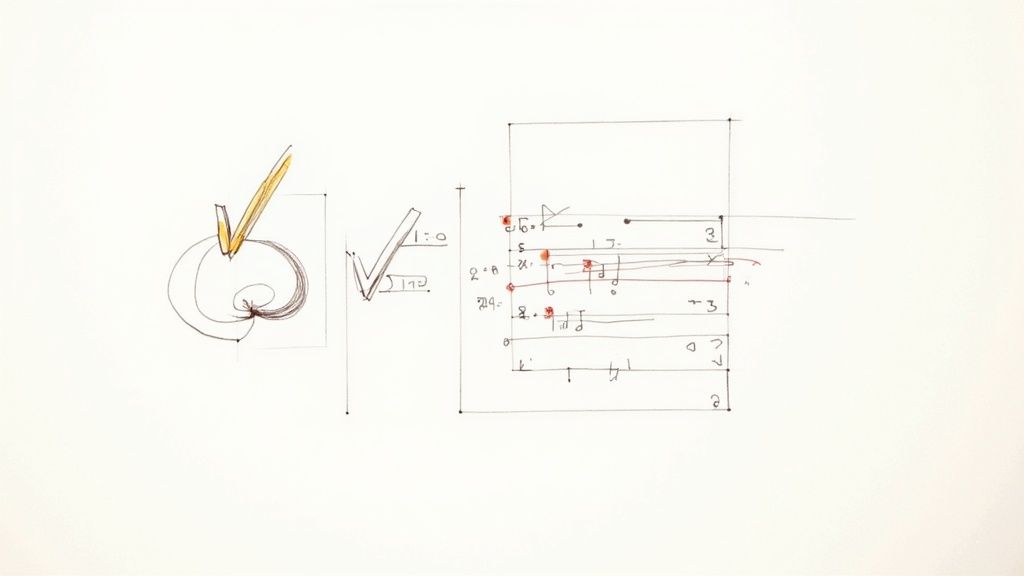
While Minitest's core features provide a solid testing base, many developers overlook its advanced capabilities. These techniques can significantly boost your testing efficiency, especially for complex projects. Integrating tools like Mergify can further enhance these Minitest strategies, optimizing your entire development workflow.
Parameterized Testing: Reducing Code Duplication
Parameterized testing lets you run the same test logic against different input sets without rewriting the entire test. This drastically reduces code duplication and boosts test coverage. Imagine testing a validation function. Instead of separate tests for each valid and invalid input, parameterized testing covers a range of scenarios with a single method.
require 'minitest/autorun'
class ValidationTest < Minitest::Test def test_valid_inputs [1, 10, 100].each do |input| assert_equal true, validate(input) end end
def test_invalid_inputs [-1, 0, nil].each do |input| assert_equal false, validate(input) end end
private
def validate(input) input.is_a?(Integer) && input > 0 end
end
This approach streamlines your tests and ensures comprehensive coverage across various input combinations.
Custom Assertions: Encoding Business Logic
Custom assertions integrate business logic directly into your tests, improving expressiveness and maintainability. They create a powerful abstraction, clarifying test intent. For example, instead of scattering complex validation logic, encapsulate it within a custom assertion, like assert_valid_user
. This cleans up individual tests and ensures consistent validation across your test suite.
Mocking and Stubbing: Isolating Components
Isolating components is crucial for robust tests. Minitest excels at mocking and stubbing. These techniques isolate the code under test by controlling its dependencies. Mocking verifies interactions between system parts, while stubbing replaces complex dependencies with simpler versions, preventing brittle tests and leading to more stable test suites.
Testing Asynchronous Code: Avoiding Random Failures
Testing asynchronous code can be tricky. Minitest offers solutions to avoid random failures. Using wait
and resume
controls asynchronous operation flow during testing. Another approach employs gems like mocha, to mock or stub asynchronous methods, simplifying the process.
Parallel Test Execution: Speeding Up Your CI Pipeline
Parallel test execution significantly reduces CI pipeline times, saving valuable development time. This is especially beneficial for large test suites. Parallelization techniques can achieve noticeable improvements, with some developers reporting CI time reductions of 60% or more. This speed boost, combined with services like Mergify's Merge Queue, streamlines code integration, leading to faster feedback and quicker deployments.
Custom Reporting: Making Minitest Speak Your Language
Standard test output often lacks the depth and clarity needed for effective team communication. Leading development teams customize minitest reporting to gain targeted insights that better suit their workflows. This transforms raw test data into actionable information.
Beyond the Basics: Tailoring Minitest Output
Customizing reports starts with understanding your team's needs. Are you trying to improve communication with non-technical stakeholders? Do you need specific outputs for your CI environment? Minitest’s flexibility accommodates a wide range of reporting requirements.
For example, visually appealing HTML reports can bridge the communication gap between developers and business teams. These reports translate technical details into an easily digestible format, making test coverage understandable for everyone. Also, consider platforms like Mergify, which offer CI insights that use AI to quickly identify and resolve infrastructure problems, further improving workflow efficiency.
Targeting CI Environments: From GitHub Actions to Jenkins
Different CI environments often require specific output formats. Minitest lets you create targeted reports for platforms like GitHub Actions and Jenkins, enabling seamless integration and automated analysis. This ensures your CI pipeline receives the necessary information in the correct format.
The Minitest::StatisticsReporter
class gathers statistics about test runs. It serves as the parent class for custom reporters, such as HTML or CI outputs. This lets developers create reports in various formats. For instance, generating XML output for Jenkins CI allows easy integration and tracking of key test metrics. Learn more about StatisticsReporter here. A Jenkins reporter might print XML tags like <testsuite tests='#{count}' failures='#{failures}' ...>
for smooth integration with the CI environment. This customization is crucial in complex testing scenarios.
Implementing Custom Formatters: A Step-by-Step Approach
Implementing custom formatters in minitest ranges from simple adjustments to building comprehensive solutions. Start by understanding the existing formatter structure. Then, gradually introduce changes to tailor the output to your specific requirements. This iterative process helps create a robust and personalized reporting system.
From Cryptic Output to Valuable Communication Tools
Well-designed reporting customization transforms minitest results from purely technical data into valuable communication tools. This closes the gap between technical and business teams, fostering better collaboration and shared understanding.
Levels of Customization
- Simple Modifications: Adjust the existing output format, adding details like timestamps or specific error messages.
- Custom Formatters: Create new formatters for specific needs like generating JSON or HTML reports.
- Integration with CI: Design formatters that integrate directly with your CI environment, automating analysis and reporting.
By customizing your minitest reporting, you provide your team with clear, actionable insights, leading to improved code quality and a more efficient development process. This proactive testing approach not only improves code reliability but also improves collaboration and communication across the team.
Mastering Rails Testing With Minitest
Rails and Minitest offer a powerful testing combination, although it's often underutilized. This exploration dives into how effective teams leverage Rails-specific helpers within Minitest to significantly enhance both test readability and coverage. We'll examine strategies for testing every layer of your Rails application—models, controllers, and even those tricky user interactions—using Minitest's concise syntax.
Streamlined Testing With Rails and Minitest
Minitest’s integration with Rails offers specialized helpers that streamline testing common Rails features. For instance, testing Active Record associations becomes significantly easier with built-in assertions. Rather than manually verifying foreign keys, you can directly test relationships between models. This enhances clarity and reduces boilerplate code.
Testing Active Record callbacks is similarly simplified. You can directly assert that callbacks like before_save
or after_create
are executed correctly and perform the expected actions. This straightforward approach clarifies the test's purpose, making it simpler to understand and maintain.
Managing Test Data Effectively
A frequent testing challenge is managing test data. Creating and cleaning up data for every test can create performance bottlenecks. Minitest, combined with tools like fixtures and factories, provides effective solutions. Fixtures offer a pre-defined data set, while factories allow for more dynamic data generation. The optimal strategy depends on your specific testing needs.
Imagine testing a complex user interaction involving multiple models. Factories enable you to quickly generate the required objects with specific attributes, keeping your tests focused and efficient.
Structuring Your Test Suite for Rapid Iteration
A well-structured test suite is crucial for supporting rapid iteration in Rails development. Organizing tests into logical groups—models, controllers, helpers, etc.—improves maintainability and simplifies finding and running specific tests. Clear, concise test names reflecting the tested behavior are also vital.
Think of your test suite like a well-organized library. Each book (test file) belongs to a category (model, controller, etc.), and each chapter (test) has a clear title describing its content. This structure facilitates easy navigation.
Testing Complex User Interactions
Testing complex user interactions that span multiple requests and actions can be difficult. Minitest, paired with tools like Capybara, allows you to simulate user behavior and test these interactions end-to-end. Capybara lets you interact with your application like a real user, ensuring all components work together seamlessly.
Minitest: Your Partner in Rails Development
Minitest, with its straightforward syntax and powerful Rails integrations, is an invaluable asset for building robust and maintainable Rails applications. Mastering these techniques can boost your testing efficiency and confidence, leading to faster development cycles and higher-quality code. Explore Mergify to discover how its automated merge strategies can optimize your CI/CD pipeline.