Top java testing libraries for 2025
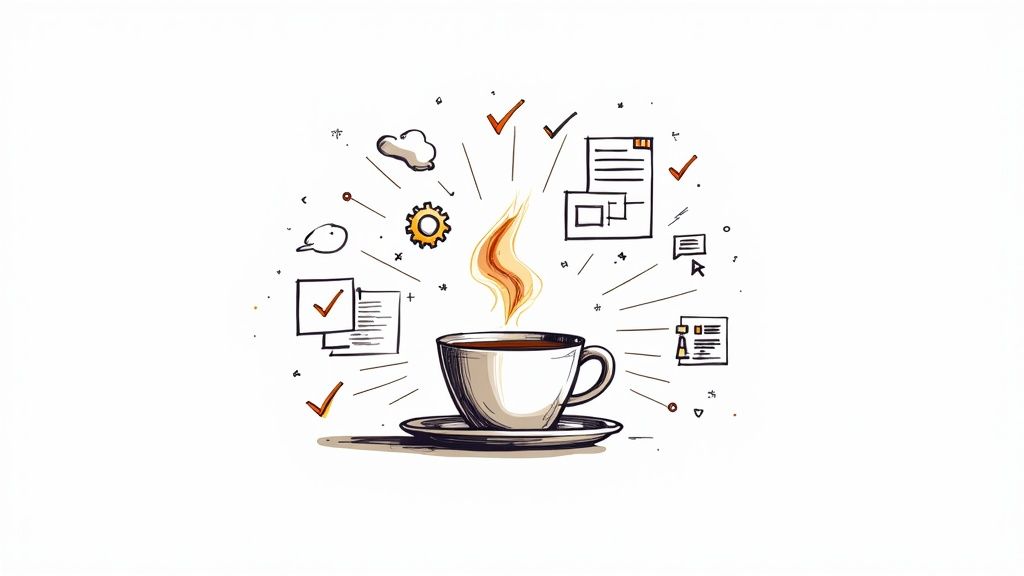
Supercharge Your Java Testing: A Deep Dive into the Top Libraries
Ensuring the quality and reliability of Java code is critical for any software project. Thorough testing is the cornerstone of this process, and selecting the right Java testing libraries can significantly improve your workflow and the overall health of your applications.
Whether you're working on a large enterprise system or a smaller project, the appropriate testing tools are essential. They help catch bugs early, minimize development costs, and speed up the time it takes to get your product to market.
Key Factors in Choosing a Testing Library
When selecting a Java testing library, several key factors come into play:
- Ease of Use: How quickly can your team learn and effectively use the library?
- Community Support: A strong community provides valuable resources, troubleshooting assistance, and ongoing development.
- Integration: Seamless integration with your existing development environment—including IDEs like IntelliJ IDEA, Eclipse, and NetBeans, build tools such as Maven and Gradle, and CI/CD pipelines—is crucial for a smooth workflow.
- Specific Testing Needs: Consider whether the library supports your specific needs, such as mocking with tools like Mockito, assertions with libraries like JUnit, and behavior-driven development (BDD) with frameworks like Cucumber.
An effective Java testing library simplifies the process of creating tests, delivers valuable insights through detailed reports, and ultimately contributes to the development of robust and dependable software.
Exploring the Options
Some Java testing libraries are open-source and freely available, while others offer commercial versions with additional features and support. Understanding both the technical capabilities and the pricing models is important for making an informed decision.
In this guide, we'll explore eight prominent Java testing libraries that can empower you to build resilient, maintainable, and high-performing applications. We'll delve into their strengths, weaknesses, and ideal use cases to help you determine the best fit for your Java development needs.
1. JUnit 5
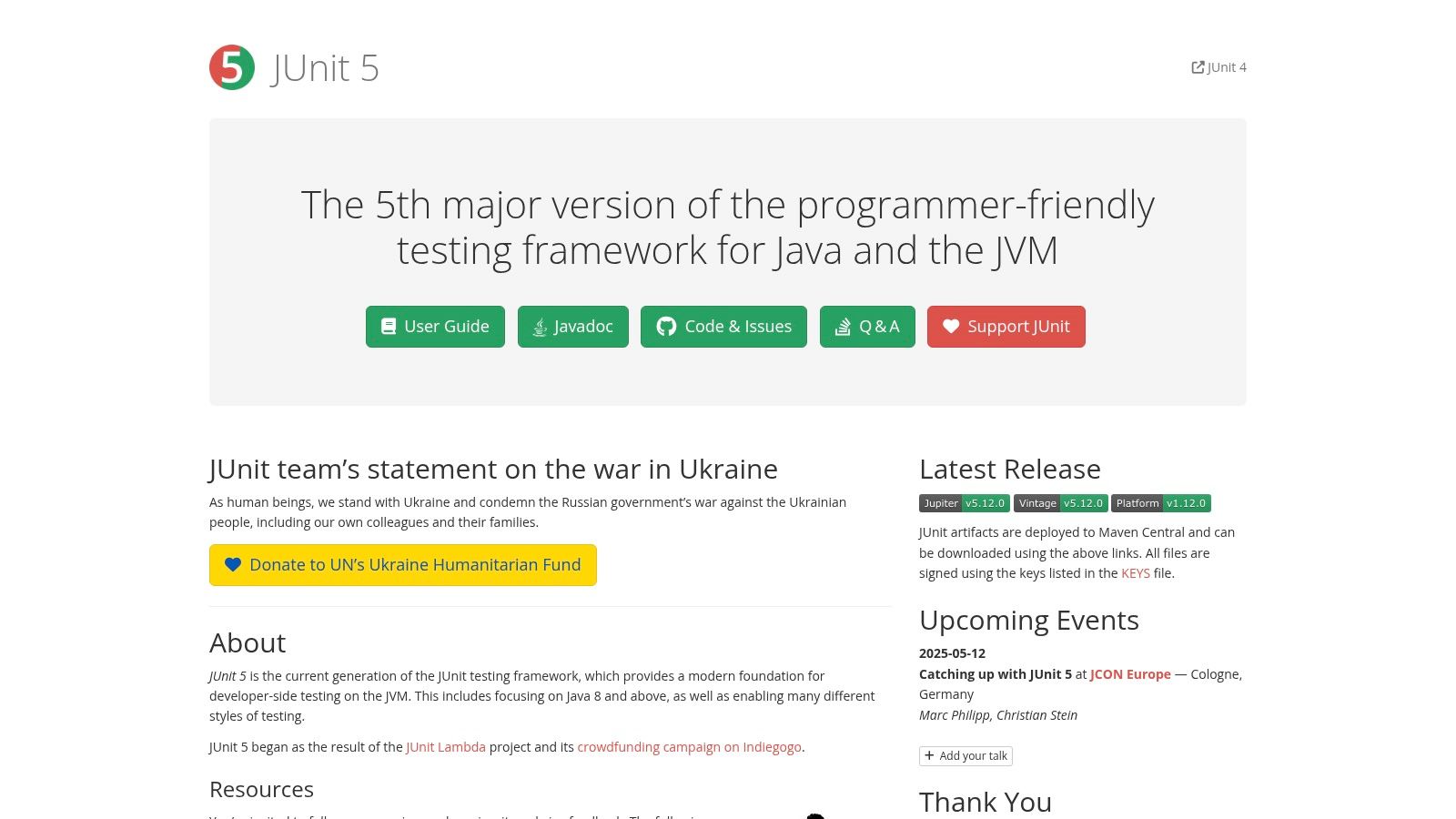
JUnit 5 stands as the leading Java testing library, the industry standard for unit testing in the Java world. It offers a robust, adaptable framework for developers building reliable and resilient code. Composed of three core modules – JUnit Platform, JUnit Jupiter, and JUnit Vintage – it covers a spectrum of testing needs, from basic unit tests to more involved integration tests.
JUnit 5 takes advantage of Java 8 and later versions, incorporating features like lambda expressions and streams for more concise tests. This results in cleaner, more readable test code. For example, assertions become more descriptive with lambdas:
assertTrue(() -> list.size() > 0, "List should not be empty");
Parameterization is a significant strength, allowing a single test to run with multiple inputs for comprehensive coverage. Arguments can be pulled from methods, CSV files, or even enums, simplifying the process of testing diverse scenarios. Nested tests promote better organization by grouping related tests within a class, improving readability and maintainability.
Extending JUnit 5
The extensible architecture of JUnit 5 allows customization and extension to match specific testing needs. This is especially valuable for integrating with other tools and frameworks within your CI/CD pipeline. The ability to tag tests allows for targeted execution, focusing on particular areas during development or debugging. For example, tag tests related to a specific feature and then execute only those tagged tests.
Features and Benefits of JUnit 5
Here’s a quick rundown of the key features and benefits:
Features:
- Support for Java 8 features (lambdas and streams)
- Parameterized tests (various argument sources)
- Nested test classes
- Extension model
- Tag filtering
Pros:
- Industry standard with strong IDE integration
- Excellent documentation and community support
- JUnit 4 backward compatibility (via Vintage engine)
- Wide range of extensions
Cons:
- Java 8+ requirement
- Code changes needed for migration from JUnit 4
- Verbosity compared to newer frameworks
Technical Requirements and Resources
JUnit 5 requires Java 8 or higher and is free and open-source. You can explore Mergify Blog Posts for further insights into development best practices.
While newer testing frameworks are emerging, the maturity, extensive features, and community support of JUnit 5 make it indispensable for Java development teams. Its seamless integration with popular IDEs like IntelliJ IDEA and Eclipse and build tools like Maven and Gradle strengthens its position as the top Java testing framework. For robust, reliable, and maintainable Java code, particularly in larger projects where maintainability and extensibility are paramount, JUnit 5 is an essential choice.
2. TestNG
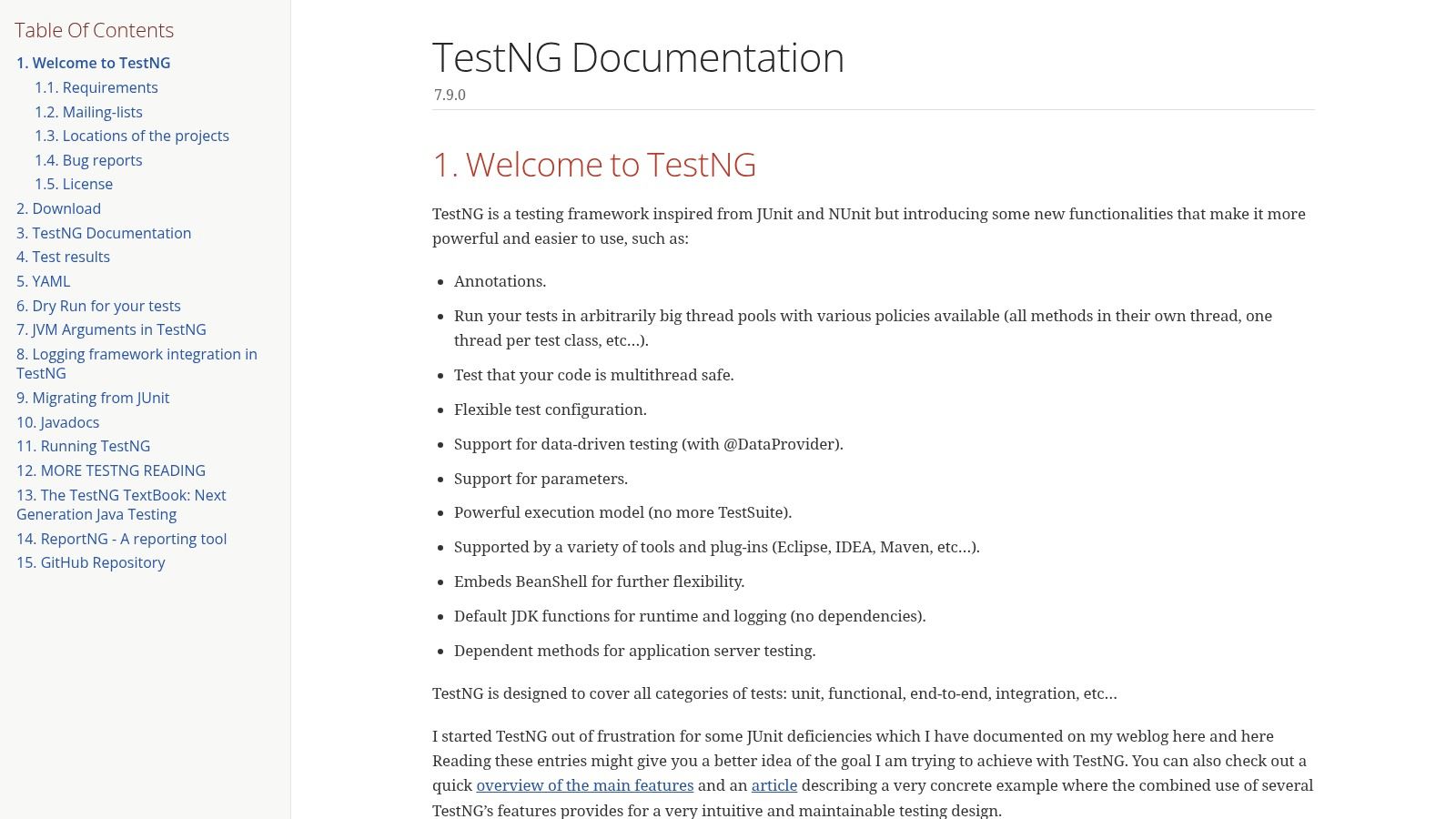
TestNG is a robust testing framework particularly suited for complex scenarios. Inspired by JUnit and NUnit, it surpasses their capabilities with advanced features for enterprise-level testing and intricate integrations. It’s a solid choice for teams handling large projects requiring adaptable and strong testing solutions.
TestNG covers the full spectrum of testing needs, from unit and functional tests to complex integration and end-to-end tests. It addresses many JUnit limitations with a richer feature set, streamlining the testing process, especially in large and complex projects.
Key Features and Benefits
TestNG offers several advantages for developers seeking a comprehensive testing solution.
- Flexible Test Configuration With Annotations: TestNG uses annotations like
@Test
,@BeforeMethod
, and@AfterMethod
to clearly define test methods, setup, and teardown actions. This organized approach makes tests easier to understand and maintain. - Advanced Grouping Concepts: Group tests using XML configurations to run specific sets based on criteria like features, functionalities, or modules. This is incredibly helpful for targeted testing and continuous integration pipelines.
- Built-in Support for Data-Driven Testing: Integrate seamlessly with data providers to run the same test with various input data sets, simplifying data-driven testing and reducing code duplication.
- Parallel Execution Support: TestNG excels at parallel test execution, shrinking test execution time, especially for large test suites. Fast feedback cycles are crucial in agile development.
- Dependency Testing: Define dependencies between test methods, ensuring tests run in the correct sequence when one test relies on the successful completion of another.
Pros of Using TestNG
- Excellent for Complex Integration and End-to-End Testing: Features like dependency injection, data providers, and parallel execution make TestNG perfect for complex testing.
- Powerful XML Configuration for Test Suites: Control test execution and configuration with XML files, allowing you to create flexible and customized test suites.
- Better Reporting Capabilities than JUnit: Generate comprehensive HTML reports detailing test execution results, simplifying failure analysis.
- Strong Support for Multi-threaded Testing: Robust multi-threading support improves efficiency when testing concurrent applications, reducing test execution time.
Cons of Using TestNG
- Steeper Learning Curve Compared to JUnit: The wealth of features can initially make TestNG slightly more challenging to learn, especially for developers familiar with JUnit.
- Less IDE Integration Compared to JUnit: While improving, TestNG’s IDE integration might not be as seamless as JUnit’s in some IDEs.
- More Complex Setup for Basic Testing Needs: For very simple projects, JUnit may be a simpler option, as TestNG’s advanced features might be overkill.
Implementation/Setup Tips
- Use a build tool like Maven or Gradle to manage TestNG dependencies.
- Consult the official TestNG documentation and explore example projects to grasp core concepts.
- Utilize the XML configuration capabilities to structure and manage test suites.
Pricing and Technical Requirements
TestNG is open-source and free to use. It requires Java to be installed.
Website
TestNG is a valuable tool for any Java developer, especially those working on complex projects needing integration testing. While JUnit suits simpler projects, TestNG provides the advanced capabilities and flexibility crucial for robust, comprehensive testing in larger, enterprise-level applications. It empowers teams to build high-quality software with confidence.
3. Mockito
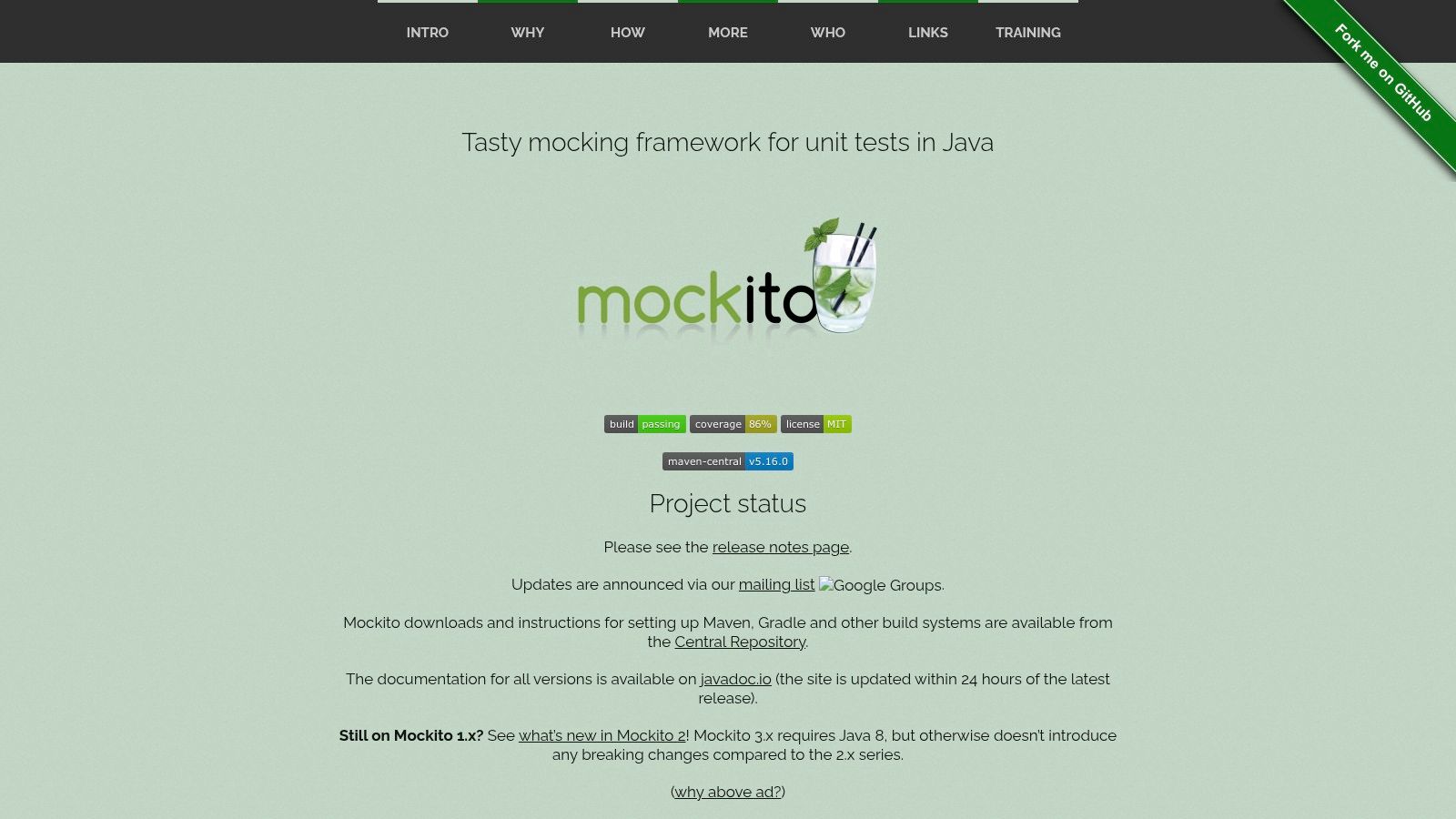
Mockito is a highly popular mocking framework within the Java ecosystem. Its clean API and emphasis on behavior verification make it a valuable tool for building robust and maintainable unit tests. By allowing developers to create and configure mock objects, Mockito simplifies the process of isolating units of code. These mock objects act as stand-ins for real dependencies, enabling testing without the complications of a fully integrated system.
Mockito is particularly well-suited for behavior-driven development (BDD). Its intuitive syntax enables developers to specify how their code interacts with its dependencies, rather than focusing on the dependencies' internal state. This results in more readable and expressive tests that clearly convey the intended behavior.
Key Features and Benefits
- Simple API for Creating and Stubbing Mocks: Creating mocks is straightforward with methods like
mock()
andwhen()
. Stubbing allows developers to define specific return values or exceptions for method calls. - Verification of Mock Interactions: The
verify()
method ensures that expected interactions occurred between the code and the mock objects, confirming that dependencies are used correctly. - Annotation-Based Mock Creation: Annotations like
@Mock
simplify test setup by automatically creating mock objects. - Spying on Real Objects: The spy functionality lets developers partially mock real objects, mocking select methods while preserving the actual behavior of others.
- Support for BDD-Style Testing: Mockito's syntax naturally aligns with BDD principles, facilitating tests that describe the desired behavior.
Practical Applications
- Testing Service Layers: Mockito is invaluable for isolating service layer logic by mocking data access objects or external dependencies. This enables focused testing of business logic without database connections or external API calls.
- Testing Controllers: In web applications, Mockito helps mock service layer components during controller testing. This isolates the controller's handling of requests and responses.
- Testing Complex Interactions: Mockito simplifies testing scenarios involving multiple components. Mocking dependencies allows for simulation of various situations and edge cases without needing complex test environments.
Pros
- Intuitive API with Minimal Setup: Mockito is easy to start using, thanks to its well-designed API and minimal boilerplate code.
- Excellent Integration with JUnit and TestNG: Mockito integrates seamlessly with popular testing frameworks.
- Very Good Documentation and Community Support: Ample resources and support are available through comprehensive documentation and a large, active community.
- Focuses on Behavior Verification: Mockito's focus on behavior verification promotes robust and meaningful tests.
Cons
- Limitations with Mocking Final and Static Methods: Mocking final classes, methods, and static methods can be challenging within the core library, although workarounds and extensions like PowerMockito exist.
- Sometimes Creates Vague Error Messages: Occasionally, error messages can lack detail, which can slightly complicate debugging.
Technical Requirements
Mockito requires Java (version 7 or higher).
Comparison with Similar Tools
While alternatives like EasyMock and JMockit exist, Mockito's popularity stems from its simple API, emphasis on behavior verification, and excellent documentation.
Implementation/Setup Tips
- Use dependency injection to inject mock objects into your code under test.
- Use annotations like
@Mock
and@InjectMocks
for streamlined test setup. - Familiarize yourself with Mockito's matchers (e.g.,
any()
,eq()
) for flexible argument matching.
Website
Mockito is a valuable tool for any Java development team aiming for high-quality, well-tested software. Its ease of use, powerful features, and focus on behavior verification contribute significantly to writing more effective and maintainable tests.
4. AssertJ
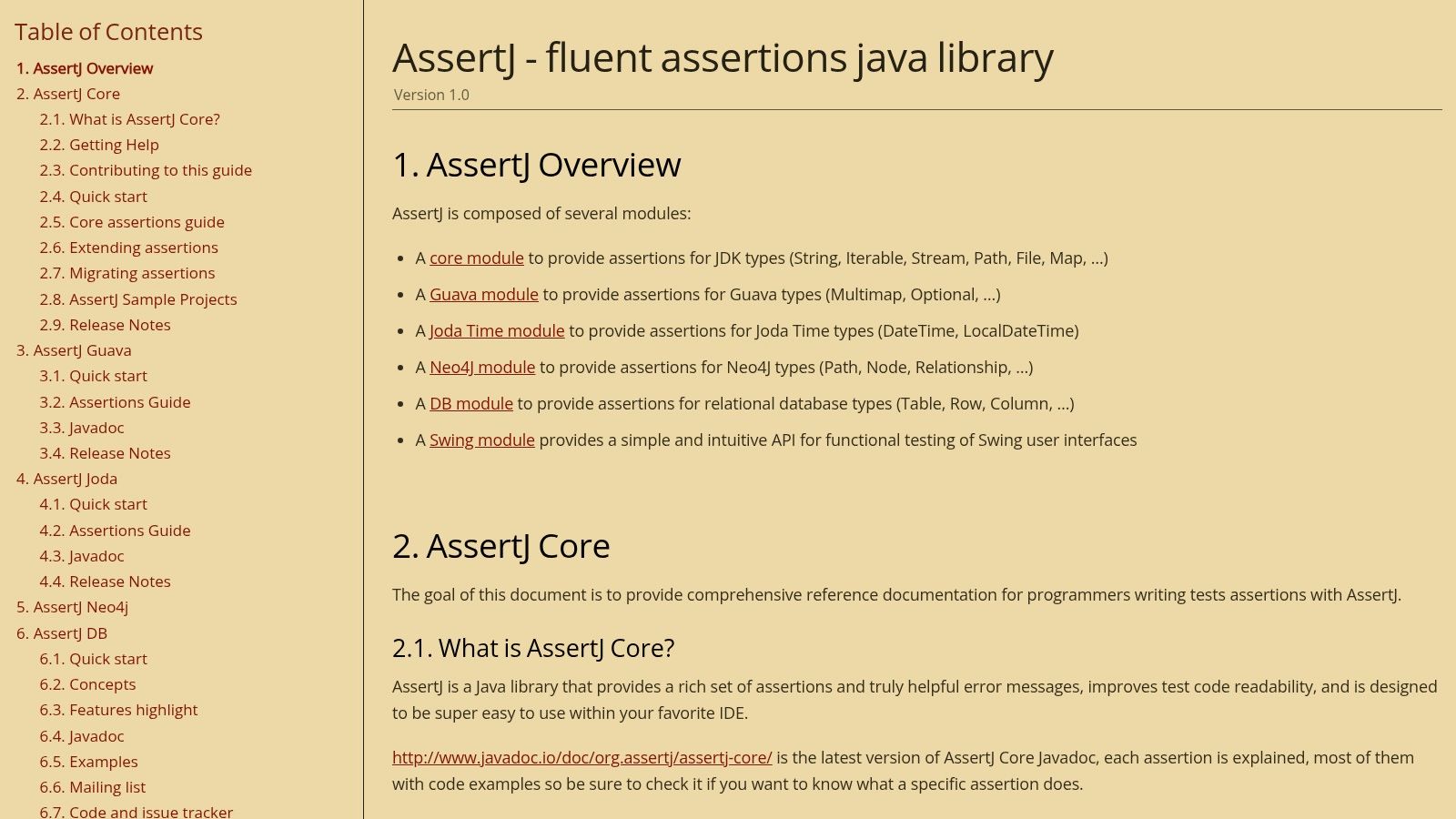
AssertJ stands out for its fluent API, which makes test assertions significantly easier to read. While basic JUnit assertions are fine for simple checks, AssertJ truly shines when working with complex objects and detailed comparisons. This leads to test suites that are more maintainable and understandable.
AssertJ offers a rich and fluent API, enabling developers to chain assertions together for improved readability. This "fluent" style, along with IDE auto-completion, makes writing assertions faster and reduces errors. Comparing the properties of a complex object can be cumbersome in JUnit. AssertJ simplifies this process with chained assertions.
// JUnit assertEquals(expected.getName(), actual.getName()); assertEquals(expected.getAge(), actual.getAge()); assertEquals(expected.getAddress(), actual.getAddress());
// AssertJ assertThat(actual).isEqualToComparingFieldByField(expected);
This example highlights how AssertJ reduces boilerplate and enhances clarity. The chained syntax also helps with debugging by precisely identifying the failure point within a complex assertion.
Beyond simple comparisons, AssertJ offers special assertions for different data structures like lists, maps, and sets. It also extends support to popular libraries such as Guava, Joda Time, and Neo4j, covering a wide array of project requirements. Developers can write assertions specific to these libraries, making tests even more expressive.
Another key feature is AssertJ's soft assertions. Unlike standard assertions that stop at the first failure, soft assertions gather all failing assertions and report them together at the end. This is particularly helpful for complicated scenarios where you want to uncover multiple issues within a single test.
Features
- Rich, fluent API for assertions with code completion support
- Detailed error messages with clear failure descriptions
- Ability to generate custom assertions
- Soft assertions to gather multiple failures
- Extensive set of string, collection, and object assertions
Pros
- Readable chained syntax with IDE auto-completion
- Descriptive error messages for effective debugging
- Simplifies complex object comparisons
- Active development with new features and community support
Cons
- Requires an initial learning curve compared to JUnit
- Can be verbose for simple assertions
- May introduce project dependency on AssertJ’s style
Website
https://assertj.github.io/doc/
Implementation/Setup Tips
Adding AssertJ to a project is easy with Maven, Gradle, or other dependency management tools. Just add the core AssertJ dependency. For specific modules, include the corresponding dependencies (like Guava or Joda Time support). Once added, import the assertThat
static method to use AssertJ in your test classes.
AssertJ offers substantial benefits over traditional assertion methods, especially when dealing with complex objects or requiring clear error messages. The initial learning curve is offset by enhanced readability and maintainability of tests. It's a valuable tool for any team focused on improving test quality and effectiveness.
5. JMockit
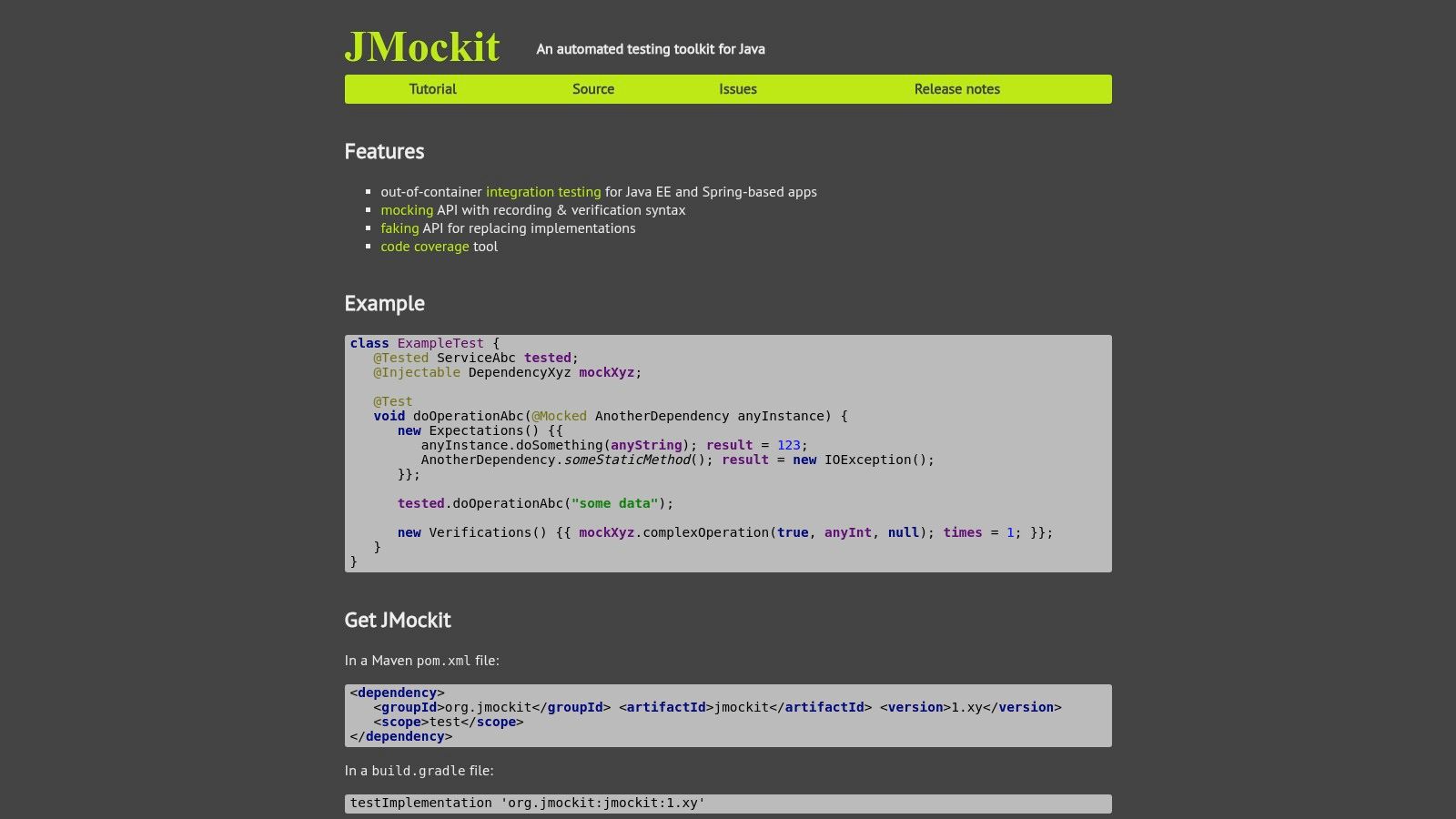
JMockit stands out for its ability to mock almost anything in Java. Where other mocking frameworks struggle with static methods, final classes, or constructors, JMockit handles them seamlessly. This makes it particularly useful for testing legacy code or complex systems where these constructs are common.
JMockit is a Java mocking framework that provides advanced capabilities beyond many other tools. It supports mocking based on interfaces or classes, including final classes and methods, static methods, constructors, and even private methods. It achieves this through runtime bytecode manipulation, giving it more power than proxy-based frameworks like Mockito, but with a more complex API.
Features
- Mocking of Static Methods, Constructors, and Final Classes: This is JMockit's key strength. It overcomes limitations in other frameworks, enabling thorough testing of difficult code.
- Powerful Expectations Recording and Verification Syntax: JMockit offers a flexible and expressive syntax for defining expectations on mocked objects.
- Code Coverage Tool Integration: JMockit seamlessly integrates with code coverage tools, helping identify gaps in test suites.
- Data-Driven Testing Support: Run tests with various inputs from data sources.
- State-Based and Behavior-Based Verification: Verify expected state changes and method invocations on mocks.
Practical Applications
- Legacy Code Testing: The ability to mock final and static methods makes JMockit invaluable for testing legacy systems not designed with testability in mind.
- Testing Complex Interactions: JMockit excels when handling intricate code involving static dependencies or final classes, where other frameworks might struggle.
- Unit Testing Code With Limited Testability: For code difficult to isolate, JMockit provides the tools to mock dependencies effectively, facilitating focused unit tests.
Pros
- Extensive Mocking Capabilities: JMockit significantly expands the possibilities of mocking in Java.
- Mocks Virtually Any Java Construct: Including final and static methods, setting it apart from other mocking frameworks.
- Built-in Support for Code Coverage: Streamlines the process of measuring test coverage.
- Effective for Legacy Code Testing: Offers solutions for testing code not inherently designed for testability.
Cons
- Steeper Learning Curve Than Mockito: The more powerful API comes with increased complexity.
- Less Intuitive API Compared to Mockito: Requires time to become comfortable with its conventions.
- Less Active Development: Potentially a concern for long-term support.
- Challenging Documentation for Beginners: Assumes familiarity with mocking concepts.
Implementation/Setup Tips
Add the JMockit dependency to your project using Maven or Gradle. Ensure the JMockit JAR file precedes the JUnit JAR in the classpath. This order is essential for correct bytecode manipulation.
Comparison With Mockito
While Mockito is generally preferred for its simplicity, JMockit addresses Mockito's limitations in mocking static methods, final classes, and constructors. Mockito might suffice for well-designed, testable code. However, JMockit’s power becomes crucial with legacy systems or complex dependencies.
Website: https://jmockit.github.io/
JMockit offers powerful mocking capabilities, despite its steeper learning curve. Its ability to overcome limitations in other frameworks makes it a valuable tool for challenging testing scenarios, especially with legacy code or complex systems. It's a powerful asset for any Java developer's testing toolkit.
6. Spock Framework
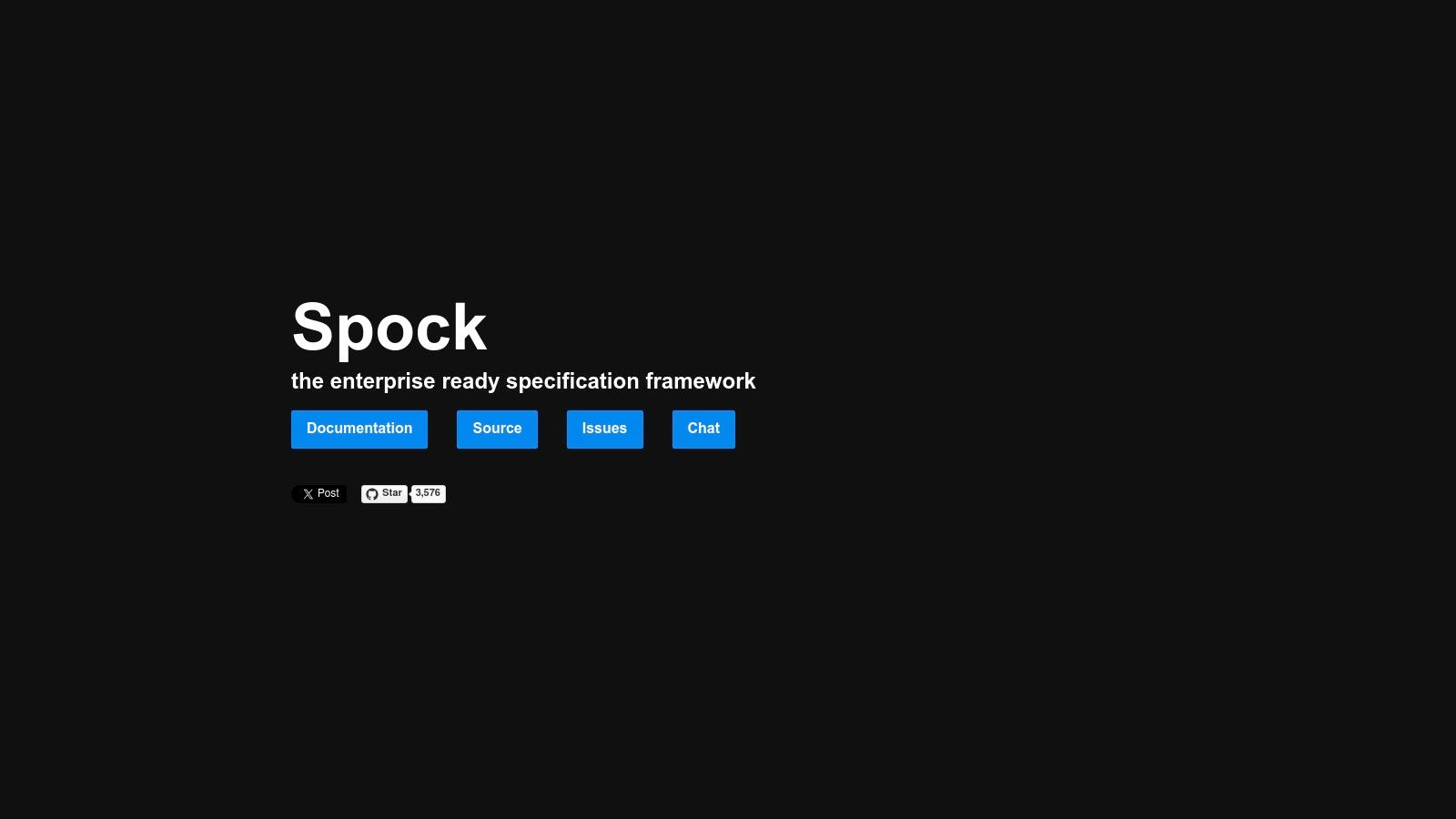
Spock is a powerful and expressive testing framework for Java and Groovy applications. It stands out for its readability and ease of use, making it a compelling alternative to traditional Java testing frameworks. By leveraging Groovy's dynamic nature, Spock allows developers to write concise and descriptive tests, leading to improved maintainability and faster debugging.
Spock offers a unique blend of power and simplicity. While based on Groovy, it seamlessly integrates with Java projects. This offers teams a new approach to testing, particularly beneficial when dealing with complex scenarios that can make traditional JUnit tests verbose and difficult to follow.
Key Features of Spock
Here's a breakdown of some of Spock's most useful features:
- Expressive Specification Language: Spock uses a Groovy-based specification language, allowing tests to be written in a more natural and human-readable format. The structure, with its
given
,when
, andthen
blocks, clearly separates the setup, execution, and assertion phases of each test. - Built-in Mocking: Creating test doubles is simplified with Spock's robust mocking framework. This allows for effective isolation of code units, enabling testing in controlled environments.
- Data-Driven Testing: Running the same test with various inputs and expected outputs is made concise and efficient through Spock's support of data-driven testing with data tables.
- Powerful Assertions: Spock boasts a powerful and expressive assertion framework. Its helpful error messages make diagnosing and fixing issues a much smoother process.
Pros and Cons of Using Spock
Like any tool, Spock has its advantages and disadvantages:
Pros:
- Readability: The
given/when/then
blocks combined with descriptive method names make tests significantly more readable and easier to understand. This contributes greatly to maintainability and team collaboration. - Conciseness: Spock significantly reduces the amount of code required compared to traditional Java testing frameworks, resulting in more compact and manageable tests.
- All-in-One Framework: Spock handles both mocking and testing, minimizing the need for multiple libraries and simplifying project dependencies.
- Versatile Testing: Spock's flexibility makes it suitable for both unit and integration testing, covering a broad spectrum of testing needs.
Cons:
- Groovy Requirement: Teams unfamiliar with Groovy will require some initial training.
- Groovy Dependency: Projects using Spock will have a dependency on the Groovy runtime.
- IDE Support: While IDE support has improved, it may not be as comprehensive as for pure Java frameworks.
- Team Resistance: Introducing Groovy into a Java-only project might encounter resistance.
Implementation and Setup
Adding Spock is straightforward. Include the Spock dependency in your build tool (Maven, Gradle, etc.) and ensure your IDE supports Groovy. Start with simple tests and gradually explore more advanced features like data tables and mocking. For more on structuring resources and documentation, check out this article on Mergify Sitemap.
Pricing and Website
Spock is open-source and free to use. You can find more information and documentation on the official website: https://spockframework.org/
7. REST Assured
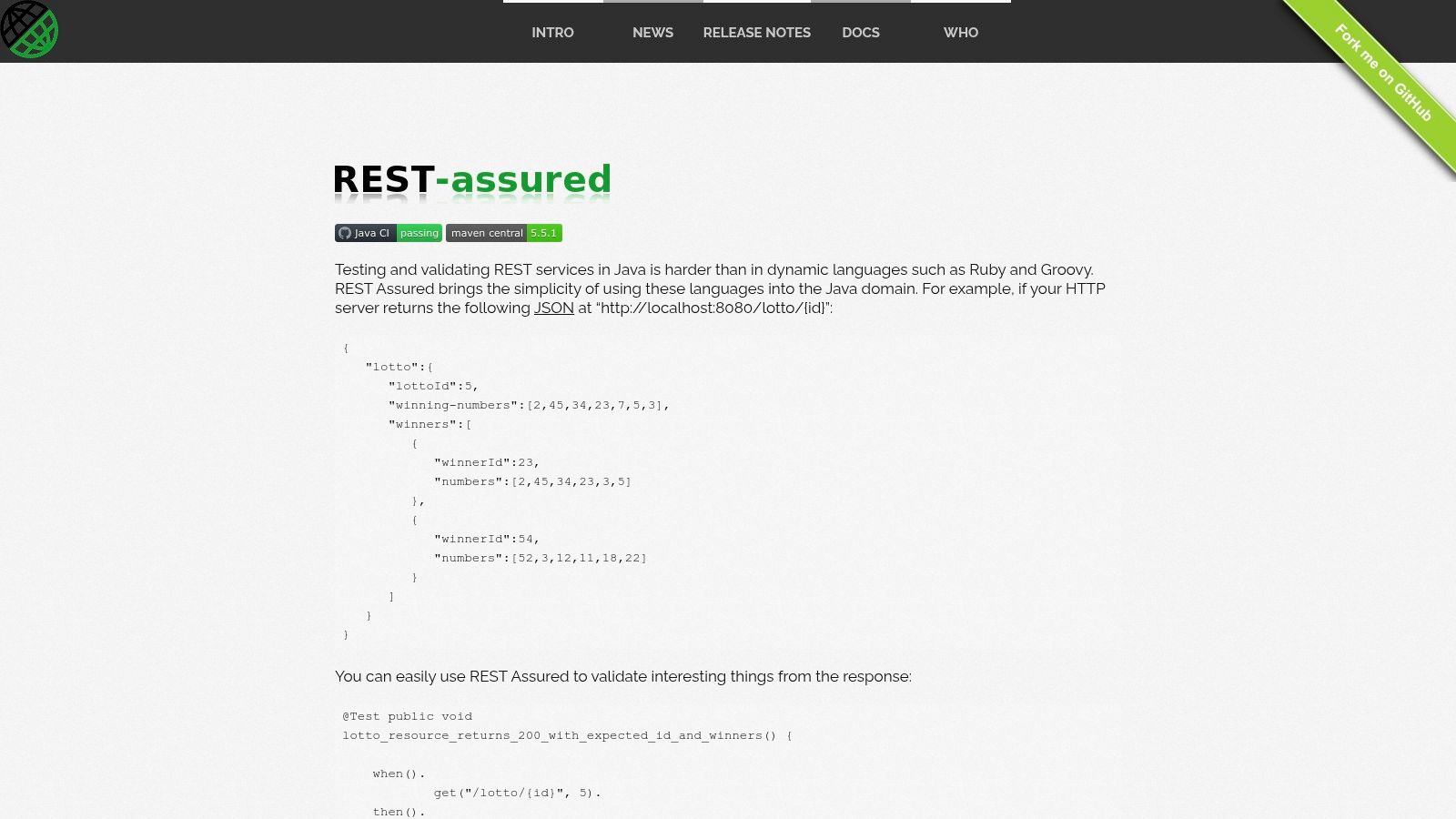
REST Assured simplifies REST API testing in Java. It offers a fluent, readable Domain Specific Language (DSL) that lets developers write clear tests without getting lost in the complexities of HTTP client libraries. This makes it a valuable tool for teams working with RESTful services.
REST Assured acts as a bridge between HTTP and your Java test code. Instead of manually building HTTP requests and parsing responses, you can use its intuitive syntax. Specify request parameters, headers, and body content easily. Then validate the response status code, body, headers, and cookies using effective matchers.
Key Features and Benefits
- Fluent API for Request and Response Handling: REST Assured’s DSL makes working with requests and responses feel natural. This results in tests that are easier to read and maintain. Checking a JSON response becomes simple:
given(). when(). get("/users"). then(). statusCode(200). body("size()", is(5));
- Seamless JSON and XML Handling: REST Assured integrates with libraries like Jackson and JAXB for handling JSON and XML. This removes the need for manual parsing and allows direct data validation.
- Integration with Testing Frameworks: REST Assured works well with JUnit and TestNG, integrating API tests into your existing test suites.
- Support for Authentication and other HTTP Features: REST Assured supports various authentication types (Basic, OAuth, etc.) and other HTTP features. This makes testing secure and complex APIs easier.
- Extensive Logging Capabilities: Detailed request and response logging helps with debugging and troubleshooting.
Pros
- Simplifies REST API testing significantly.
- Intuitive DSL enhances test readability.
- Excellent support for JSON and XML.
- Integrates seamlessly with common testing frameworks.
Cons
- Designed specifically for REST APIs, not a general testing tool.
- Can be verbose for complex validations, especially with nested JSON.
- Some performance overhead compared to lower-level clients, though rarely significant in testing.
Technical Requirements
REST Assured needs Java 7 or later. Dependencies are managed with Maven or Gradle.
Comparison with Similar Tools
Other API testing libraries exist (e.g., Apache HttpClient), but REST Assured's DSL provides a higher level of abstraction. This results in cleaner, more maintainable tests tailored for REST APIs. It simplifies common RESTful testing tasks.
Implementation/Setup Tips
Add the REST Assured dependency to your pom.xml
(Maven) or build.gradle
(Gradle) file. The official website provides examples and documentation.
Website: https://rest-assured.io/
REST Assured enables teams to create comprehensive and maintainable API tests. Its focus and powerful features make it valuable for ensuring the quality of RESTful services. Its simplified approach, along with robust JSON and XML support, helps catch errors early and maintain high quality. REST Assured is recommended for anyone working extensively with RESTful APIs in Java.
8. Cucumber JVM
Cucumber JVM brings the power of Behavior-Driven Development (BDD) to the Java ecosystem. It lets you write acceptance tests in Gherkin, a human-readable language designed to bridge the communication gap between business stakeholders and development teams. These tests, written in plain text using a Given-When-Then structure, describe how the application should behave from a user's perspective. This approach ensures that everyone understands what's being built and tested.
Cucumber JVM executes these Gherkin feature files by mapping them to Java code using step definitions. This automation effectively turns your documentation into living tests. It integrates seamlessly with popular testing frameworks like JUnit, TestNG, and Spock. This flexibility makes Cucumber JVM a versatile tool for any testing toolkit.
Features of Cucumber JVM
- Human-Readable Feature Files (Gherkin Syntax): Tests become understandable even for non-technical team members.
- Multi-Language Support: While frequently used with English, Gherkin supports many other languages.
- Reusable Step Definitions: Write Java code once and reuse it across numerous scenarios.
- Integration with Popular Testing Frameworks: Seamless operation with JUnit, TestNG, and Spock.
- Rich Reporting Capabilities: Provides detailed reports on test execution, including steps, results, and timings.
Pros and Cons
Here's a quick look at the advantages and disadvantages of using Cucumber JVM:
Pros | Cons |
---|---|
Improved communication between stakeholders | Added complexity for simple unit tests |
Living documentation, reducing overhead | Step definition maintenance can be difficult |
Focus on user behavior and acceptance | Performance overhead compared to unit tests |
Ensures alignment with business needs | Requires team discipline for Gherkin/Java mapping |
Practical Applications
Cucumber JVM is especially well-suited for projects with complex business logic that require crystal-clear communication between business and development teams. Some examples include:
- Web Application Testing: Define user journeys and verify acceptance criteria.
- API Testing: Describe API interactions and the expected responses.
- Regression Testing: Ensure existing functionality continues to work correctly.
- User Acceptance Testing (UAT): Facilitate user feedback and validation.
You might also find valuable information on Authors Sitemap for additional developer insights.
Implementation Tips
- Define clear feature files that describe the desired behavior.
- Keep step definitions concise, focusing on a single action.
- Organize step definitions logically to improve maintainability.
- Utilize Cucumber's reporting tools for valuable insights into test execution.
Website: https://cucumber.io/docs/cucumber/api-java/
Cucumber JVM transforms user stories into executable tests, making it a valuable asset for teams practicing BDD. While there is a learning curve and some maintenance involved, the benefits of improved communication and living documentation make it a worthwhile addition, especially for larger projects with complex requirements.
Side-by-Side Comparison: Top 8 Java Testing Libraries
Tool | Core Features ★ | Experience 🏆 | Audience 👥 | Unique ✨ | Value 💰 |
---|---|---|---|---|---|
JUnit 5 | Modular design; supports lambdas, parameterized & nested tests | Excellent IDE integration and broad community support | Java developers | Backward compatibility with JUnit 4 (Vintage) | Free & robust ecosystem |
TestNG | Flexible annotations; grouping; parallel execution | Advanced reporting; steeper learning curve | Enterprise & integration test teams | Dependency testing for method interactions | Free & powerful for complex tests |
Mockito | Simple API for mocks; stubbing & verification | Intuitive integration with JUnit/TestNG | Developers focusing on behavior verification | Clean, minimal setup API | Free & widely supported |
AssertJ | Fluent, rich assertions; detailed error messages | Highly readable chains with IDE auto-completion | Developers needing expressive assertions | Chained syntax enhancing clarity | Free & improves test clarity |
JMockit | Advanced mocking: static, final, constructors, private methods | Extremely powerful but a steeper learning curve | Experts in legacy or complex code testing | Can mock nearly any Java construct | Free & covers hard-to-mock cases |
Spock Framework | Groovy-based DSL; data-driven tests; clear given/when/then blocks | Concise and expressive; readable test structures | Teams open to Groovy integrations | Unified framework for testing and mocking | Free & reduces boilerplate |
REST Assured | DSL for HTTP requests; JSON/XML validation; logging | Natural language style; simplifies REST API validations | API testers and developers | Seamless integration for RESTful service testing | Free & streamlines API testing |
Cucumber JVM | BDD with Gherkin syntax; reusable steps; rich reporting | Enhances collaboration; living documentation through tests | Cross-functional teams (tech & business) | Bridges gap between non-technical & technical teams | Free & fosters clear requirements |
Ready to Test? Choosing The Right Java Testing Library
Selecting the right Java testing libraries is crucial for building high-quality software. From established frameworks like JUnit and TestNG to versatile mocking tools like Mockito and specialized libraries for REST API testing (REST Assured) and BDD (Cucumber JVM and Spock Framework), the options are plentiful. This guide has provided a comprehensive overview of eight leading Java testing libraries, equipping you with the knowledge to make informed decisions for your projects.
When choosing your testing toolkit, consider the following:
- Project Size and Complexity: For smaller projects, JUnit might suffice. Larger projects might benefit from TestNG's advanced features or Spock's descriptive syntax.
- Testing Requirements: Focus on the type of testing. Unit testing? JUnit, TestNG, and mocking tools are essential. Integration or API testing? Look into REST Assured. BDD? Consider Cucumber JVM or Spock Framework.
- Team Familiarity: Adopting a new tool requires a learning curve. Choose libraries that align with your team's existing skills or those easy to learn.
- Integration and Compatibility: Ensure the chosen libraries seamlessly integrate with your IDE, build tools (Maven and Gradle), and CI/CD pipeline. Check for compatibility with other libraries already in use.
- Budget and Resources: While many libraries are open-source and free, consider the cost of training, support, and potential tooling needed for integration.
Getting Started
Most libraries offer comprehensive documentation and getting started guides. Begin with a small, isolated project to experiment and understand the library's core concepts before integrating it into your main project.
Key Takeaways
- JUnit and TestNG: Foundational testing frameworks providing structure and assertions.
- Mockito and JMockit: Powerful mocking tools for isolating units of code.
- AssertJ: Fluent assertions for enhanced readability and debugging.
- Spock Framework: A BDD framework with a concise and expressive syntax.
- REST Assured: Simplified REST API testing.
- Cucumber JVM: A BDD framework for collaboration and acceptance testing.
Effective testing is a cornerstone of successful software development. By carefully selecting and implementing the appropriate Java testing libraries, you can significantly improve code quality, reduce defects, and accelerate the development process.
Streamlining your testing process is only one piece of the puzzle. To truly optimize your development lifecycle and reduce CI costs while enhancing code quality, consider integrating Mergify into your workflow. Mergify offers sophisticated merge queue and merge protection features, ensuring automatic PR updates, strategic CI batching, and even AI-powered CI issue identification. This helps minimize merge conflicts, expedite critical updates, and free your developers from tedious manual tasks. From startups to large enterprises, Mergify empowers engineering teams to manage complex development environments effortlessly. Learn more and improve your CI/CD pipeline at https://mergify.com.