Top Java Test Frameworks for 2025: JUnit, TestNG & More
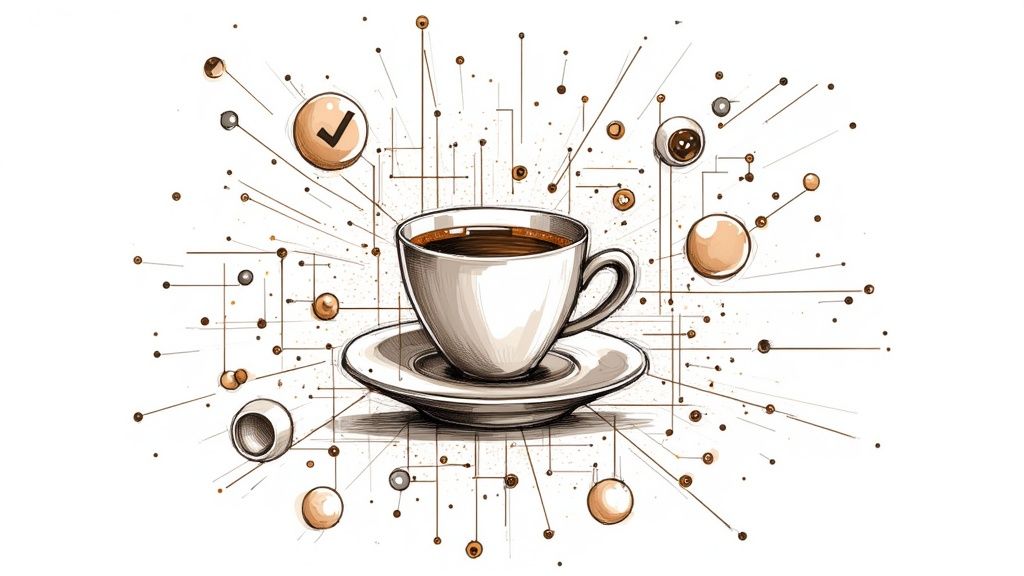
Supercharge Your Java Testing: A Guide to Top Frameworks
Building robust and reliable Java applications requires a solid testing strategy. At the core of this strategy is selecting the right testing framework. In software development, where speed and quality are paramount, the right tools can mean the difference between a smooth launch and a debugging headache. Are slow, fragile tests slowing you down? Is achieving comprehensive test coverage a struggle? Is testing itself bottlenecking your CI/CD pipeline? The correct Java testing framework can tackle these issues and dramatically improve your workflow.
This guide explores eight leading Java test frameworks designed to optimize your testing, whether you're working on unit testing individual components, integration testing complex interactions, or end-to-end UI testing.
Key Framework Considerations
We'll examine the strengths and weaknesses of each framework, considering factors like:
- Ease of use
- Community support
- Integration with other tools (like your CI/CD pipeline)
- Effectiveness in various testing scenarios
We'll also touch on technical aspects such as compatibility with different Java versions and any licensing or pricing models.
Empowering Your Team
By the end of this guide, software development teams, DevOps engineers, QA professionals, and IT leaders will be able to choose the ideal Java testing framework for their projects in 2025 and beyond. This knowledge will lead to higher quality software and faster release cycles.
1. JUnit 5
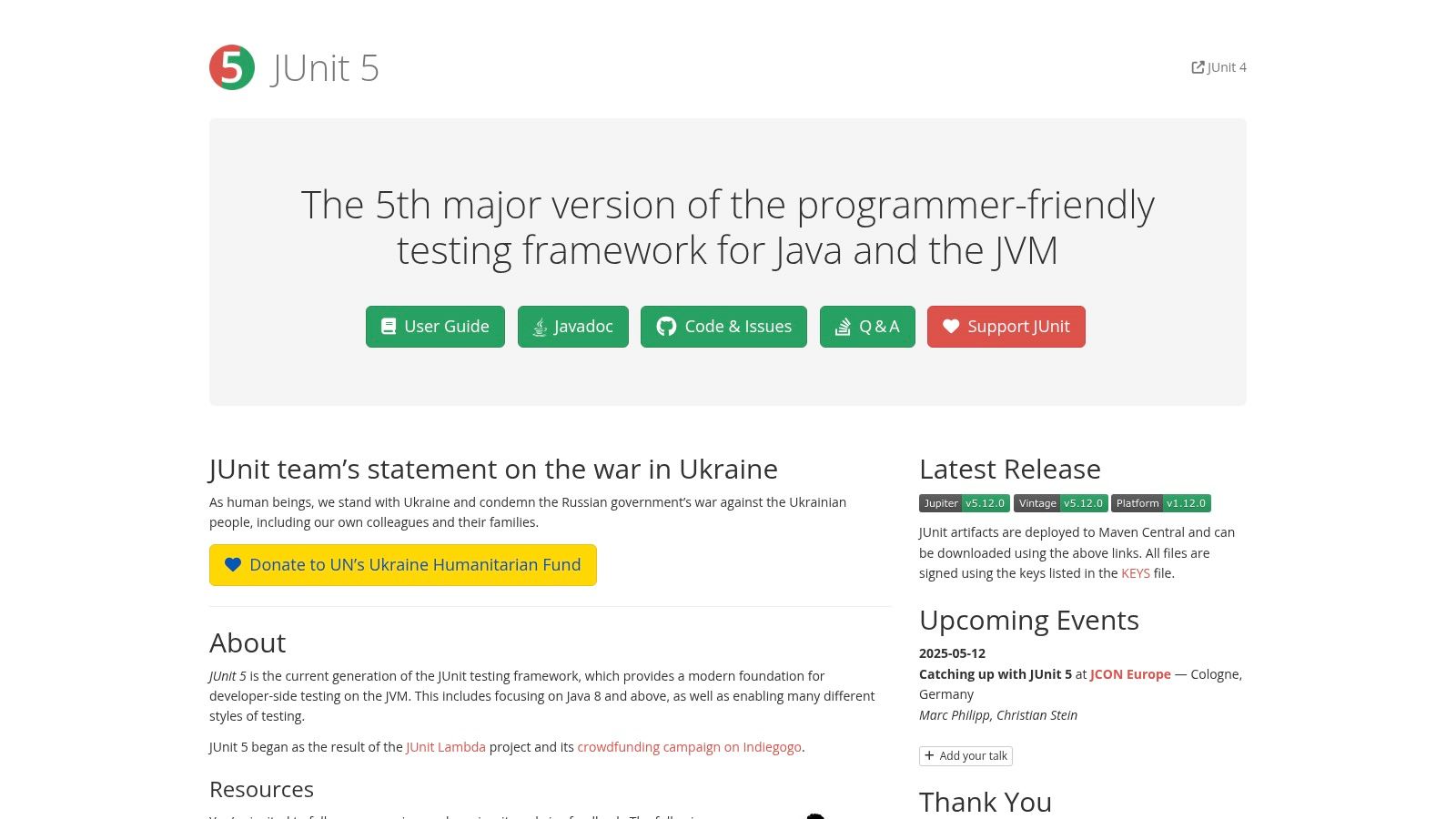
JUnit 5 stands as the leading standard for Java testing. Its widespread adoption, robust features, and active community make it a powerful choice for developers. Building on previous versions, JUnit 5 embraces modern Java development practices.
This latest iteration comprises several key modules. These include the JUnit Platform, the foundation for launching testing frameworks; JUnit Jupiter, offering a new programming and extension model; and JUnit Vintage, allowing you to run existing JUnit 4 tests. This modular design provides flexibility for diverse testing needs. JUnit 5 requires Java 8 or higher, enabling you to use features like lambdas and streams in your tests.
Parameterized Tests and Other Advantages
One of JUnit 5’s most valuable features is parameterized testing. This allows a single test to run multiple times with various inputs, reducing code duplication. You can source these parameters from several locations, including CSV files, methods, and enums.
Furthermore, nested test classes bring logical structure to your test suite, grouping related cases and clarifying their relationships. Test lifecycle callbacks (@BeforeAll
, @AfterAll
, @BeforeEach
, @AfterEach
) offer precise control over test execution, managing setup and teardown operations efficiently.
Migration and Considerations
For developers moving from JUnit 4, JUnit Vintage offers backward compatibility. This lets you run your JUnit 4 tests alongside JUnit 5. However, some code modifications are necessary for a full migration to the JUnit 5 model.
While JUnit 5 is lightweight and focused on core testing, some features present in other frameworks, like TestNG’s built-in data providers, might require extensions in JUnit 5. This can introduce a small learning curve as you become familiar with the new extension model. For further insights on software development, you might find Mergify's Blog Posts helpful.
Key Features:
- Support for Java 8+ language features (lambdas, streams)
- Parameterized tests (CSV, method sources, enums)
- Nested test classes
- Test lifecycle callbacks (
@BeforeAll
,@AfterAll
,@BeforeEach
,@AfterEach
) - Extensible architecture
Pros:
- Excellent IDE and build tool integration
- Large community and extensive documentation
- Backward compatible with JUnit 4 (via JUnit Vintage)
- Lightweight and focused
Cons:
- Some advanced features require extensions
- Migration from JUnit 4 requires code changes
- Learning curve for the extension model
Technical Requirements: Java 8 or higher.
Pricing: Open Source (free).
Website: https://junit.org/junit5/
Implementation Tip: Begin by adding the JUnit 5 dependencies to your project. Explore the @DisplayName
annotation for more descriptive test names. Use parameterized tests to minimize redundant code. Consider using nested test classes to organize your test suite efficiently.
2. TestNG
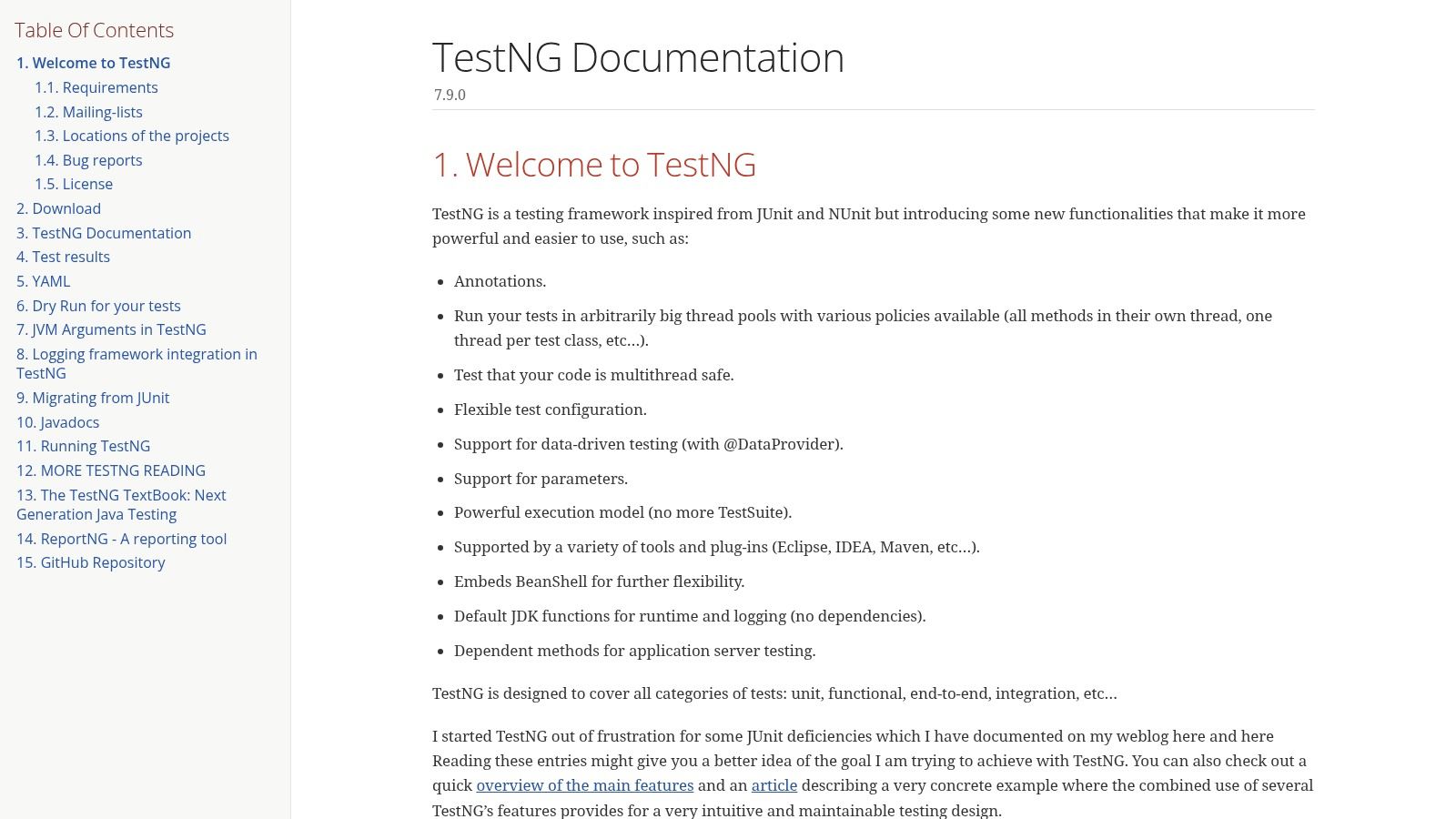
TestNG is a powerful testing framework for Java developers. It builds upon the foundations laid by JUnit and NUnit, adding features designed for more complex testing scenarios. Whether you're working on unit tests, integration tests, or full-blown end-to-end testing, TestNG offers a robust set of tools. It’s particularly well-suited for larger projects and teams that require efficient dependency management and parallel test execution.
TestNG is open-source, so it's free to use and distribute. It does require Java to be installed. You can find the specific version requirements on the official TestNG website.
Key Features and Benefits
TestNG offers a range of features to streamline your testing process:
- Annotations: TestNG uses annotations like
@Test
,@BeforeMethod
, and@AfterMethod
to clearly define test methods and their setup/teardown procedures. This makes tests more readable and easier to maintain. - XML Configuration: While potentially verbose, XML configuration provides granular control over how your tests are executed. You can define test suites, group tests, and specify dependencies. This level of control is essential for complex projects.
- Data-Driven Testing: The
@DataProvider
annotation simplifies data-driven testing, enabling you to run the same test with various inputs without redundant code. This is extremely valuable for thorough testing. - Dependency Testing: TestNG excels at managing dependencies between tests. You can specify that one test must complete successfully before another begins, preventing cascading failures. This is particularly useful for integration testing.
- Parallel Execution: Significantly reduce your overall testing time with parallel test execution. You can configure parallelism at different levels (methods, classes, or suites) for optimal performance.
- Reporting: TestNG generates detailed reports, giving you a comprehensive overview of test execution. This includes pass/fail status, execution time, and any exceptions encountered.
Practical Applications
TestNG has a wide range of applications:
- API Testing: Combine TestNG with libraries like REST Assured for robust testing of RESTful web services.
- UI Automation: Integrate TestNG with Selenium or Appium to automate UI tests and ensure your web and mobile apps function correctly.
- Database Testing: Use TestNG to automate database testing, checking data integrity, validating stored procedures, and more.
Comparison with JUnit
Both TestNG and JUnit are widely-used Java testing frameworks. TestNG offers more built-in features for dependency management, data-driven testing, and parallel execution. While JUnit is often considered simpler for beginners, TestNG's richer feature set makes it more suitable for larger, more complex projects.
Implementation Tips
Here are a few tips for getting started with TestNG:
- Add the TestNG dependency to your project (via Maven, Gradle, or manually).
- Use annotations to define your test methods and setup procedures.
- Utilize XML configuration to manage complex test suites and dependencies.
- Explore the
@DataProvider
annotation for data-driven testing. - Optimize your testing time with parallel execution.
Pros and Cons
Pros:
- Richer set of features than JUnit, minimizing the need for extensions.
- Excellent for complex test suites and dependencies.
- Flexible test configuration through XML.
- Detailed reporting.
Cons:
- XML configuration can become verbose and complex.
- Steeper learning curve compared to JUnit.
- Potentially less seamless IDE integration than JUnit in certain cases.
Website
TestNG's robust features, especially its support for dependencies and data-driven testing, make it a compelling choice for teams with sophisticated testing requirements. While the XML configuration may take some time to master, the flexibility and control it offers are invaluable for managing large test suites.
3. Mockito
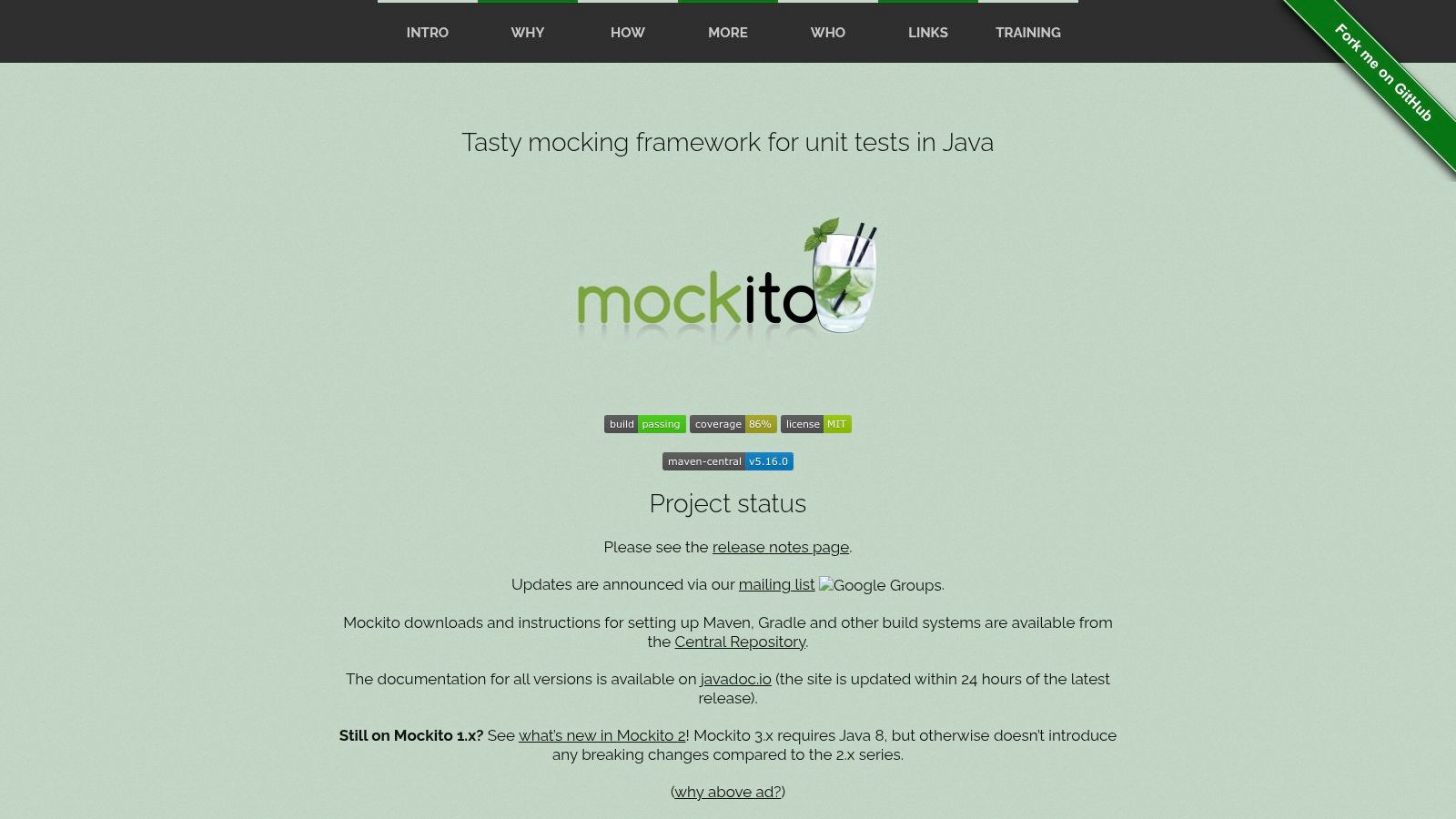
Mockito is a prominent mocking framework within the Java environment. Its clean API and powerful features make it a popular choice for developers. It simplifies the process of creating and managing mock objects, essential components for effective unit testing. By simulating the behavior of real dependencies, Mockito enables developers to isolate code units and test various scenarios without relying on the actual implementation of those dependencies.
This isolation significantly improves code robustness and maintainability.
Mockito offers a streamlined approach to mocking, with an intuitive syntax that simplifies mock creation and configuration. For example, mocking a List
and defining its behavior is straightforward:
List mockedList = mock(List.class); when(mockedList.get(0)).thenReturn("first"); when(mockedList.size()).thenReturn(1);
assertEquals("first", mockedList.get(0)); assertEquals(1, mockedList.size());
Key Features and Benefits
Mockito offers a range of useful features for developers:
- Simple Mock Creation: Creating mock objects is simple with
mock(Class)
, minimizing boilerplate code. - Behavior Verification:
verify(mock).methodCall()
allows you to confirm interactions with the mock. - Flexible Stubbing: Define custom return values for method calls using
when(mock.methodCall()).thenReturn(value)
. Argument matchers likeany()
andeq()
offer precise control over stubbing. - Spy Functionality: Partially mock real objects with
spy(Object)
, overriding specific methods while retaining real behavior for others. This is particularly useful when dealing with complex objects. - Seamless Integration: Mockito integrates smoothly with popular testing frameworks like JUnit and TestNG.
Pros and Cons
Like any tool, Mockito has its strengths and weaknesses.
Pros:
- Intuitive and Readable API: Mockito's syntax is designed for clarity and ease of use, making test code more readable.
- Excellent Integration: It seamlessly integrates with existing testing workflows.
- Comprehensive Documentation: Extensive documentation and a large community provide ample resources.
- Active Development: Mockito is actively maintained and updated.
Cons:
- Limitations with Final: Mocking final classes and methods typically requires extensions like Mockito Inline.
- Static Method Mocking: Similar to final methods and classes, mocking static methods also necessitates using Mockito Inline.
- Complex Scenarios: While Mockito handles most common use cases effectively, some complex scenarios may require workarounds.
Implementation/Setup Tips
Here are a few tips to get started with Mockito:
- Add the Mockito dependency to your project’s
pom.xml
(Maven) orbuild.gradle
(Gradle). - Simplify mock creation and injection with annotations like
@Mock
and@InjectMocks
. - Learn about argument matchers for more flexible and robust stubbing.
Comparison with Similar Tools
Mockito stands out for its simplicity and ease of use compared to other mocking frameworks like EasyMock and JMockit. This often results in cleaner and more maintainable test code.
Pricing and Technical Requirements
Mockito is open-source and free to use. It requires Java and is compatible with various build tools and testing frameworks.
Website and Conclusion
For more information, visit the official Mockito website: https://site.mockito.org/
Mockito is a valuable tool for any Java development team aiming for well-tested and maintainable code. Its user-friendly API, robust features, and strong community support make it a leading choice for mocking in Java projects.
4. Selenium
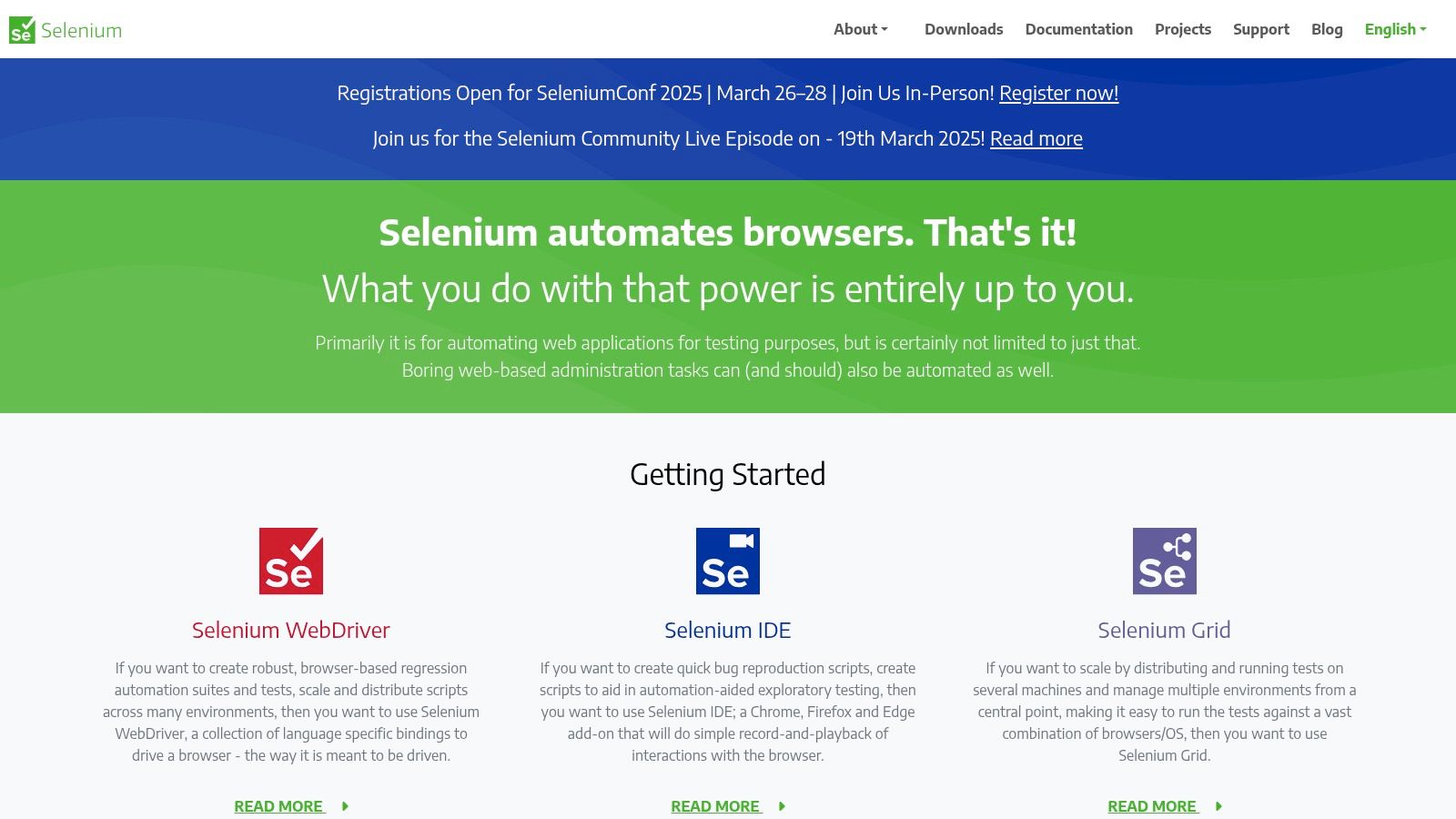
Selenium is a leading tool for web UI testing, setting the industry standard. It's an open-source project with a suite of tools and libraries that enable web browser automation. Selenium offers a powerful way to ensure your web application works as expected across various browsers and platforms.
Whether it's a simple click or a complex user flow involving drag-and-drop actions or form submissions, Selenium can automate it. This versatility makes it an invaluable asset for ensuring a consistent user experience.
In Java development, the Selenium WebDriver is essential for controlling browser behavior. It offers a streamlined and robust API. This API allows interaction with web elements, manages browser windows, and handles asynchronous operations, making it a vital tool for Java-based web projects.
Key Features
- Cross-Browser Compatibility: Test across all major browsers like Chrome, Firefox, Safari, Edge, and even Internet Explorer. This ensures your application works consistently for a broad user base.
- WebDriver API: A powerful API lets you interact with web elements, simulate user actions, and control the browser itself. This provides fine-grained control over the testing process.
- Advanced User Interactions: Selenium supports complex user interactions such as drag-and-drop, multi-select, keyboard inputs, and precise mouse movements. This allows you to simulate real-world user scenarios effectively.
- Wait Mechanisms: Selenium's robust wait mechanisms handle the asynchronous nature of web applications. This minimizes flaky tests caused by timing issues, leading to more reliable results.
- Page Object Model (POM) Support: Selenium facilitates the use of the Page Object Model, which creates a maintainable test architecture. Abstracting web pages as objects promotes code reusability and simplifies maintenance.
Pros and Cons of Using Selenium
Pros:
- Industry Standard: Selenium's widespread adoption makes it easy to find skilled testers and access abundant resources.
- Multi-Browser and Platform Support: Verify your web application functions correctly across various environments, ensuring a consistent user experience.
- Integration with Testing Frameworks: Selenium integrates seamlessly with popular Java testing frameworks like JUnit and TestNG.
- Extensive Community and Resources: A large, active community provides readily available documentation, tutorials, and support, facilitating troubleshooting and knowledge sharing.
Cons:
- Test Brittleness: UI changes can impact test stability, requiring updates and maintenance to keep tests running smoothly.
- Slower Execution: UI tests naturally take longer to execute than unit tests, which can impact the overall testing cycle time.
- Browser Driver Setup: Separate browser drivers are needed for each browser you test, adding a layer of setup and configuration.
- Complexity: Creating reliable and stable tests can be complex, requiring careful planning and consideration of asynchronous behavior.
You might be interested in: Our guide on...
Implementation Tips
- Page Object Model: Use the POM to structure your test code for better maintainability and organization.
- Wait Mechanisms: Implement appropriate wait strategies to handle asynchronous operations and reduce the occurrence of flaky tests.
- Advanced Interactions: Make full use of Selenium's API to simulate complex user interactions, ensuring comprehensive test coverage.
- CI/CD Integration: Integrate Selenium into your CI/CD pipeline for automated testing and continuous feedback.
Selenium is a free, open-source tool available for download from the official website: https://www.selenium.dev/
Selenium's rich feature set, widespread adoption, and powerful API make it an essential tool for Java-based web UI testing. While it has its drawbacks, the advantages significantly outweigh the disadvantages, solidifying its position as a leading Java testing framework. By mastering its capabilities, you can significantly improve the quality and reliability of your web applications.
5. Spock Framework
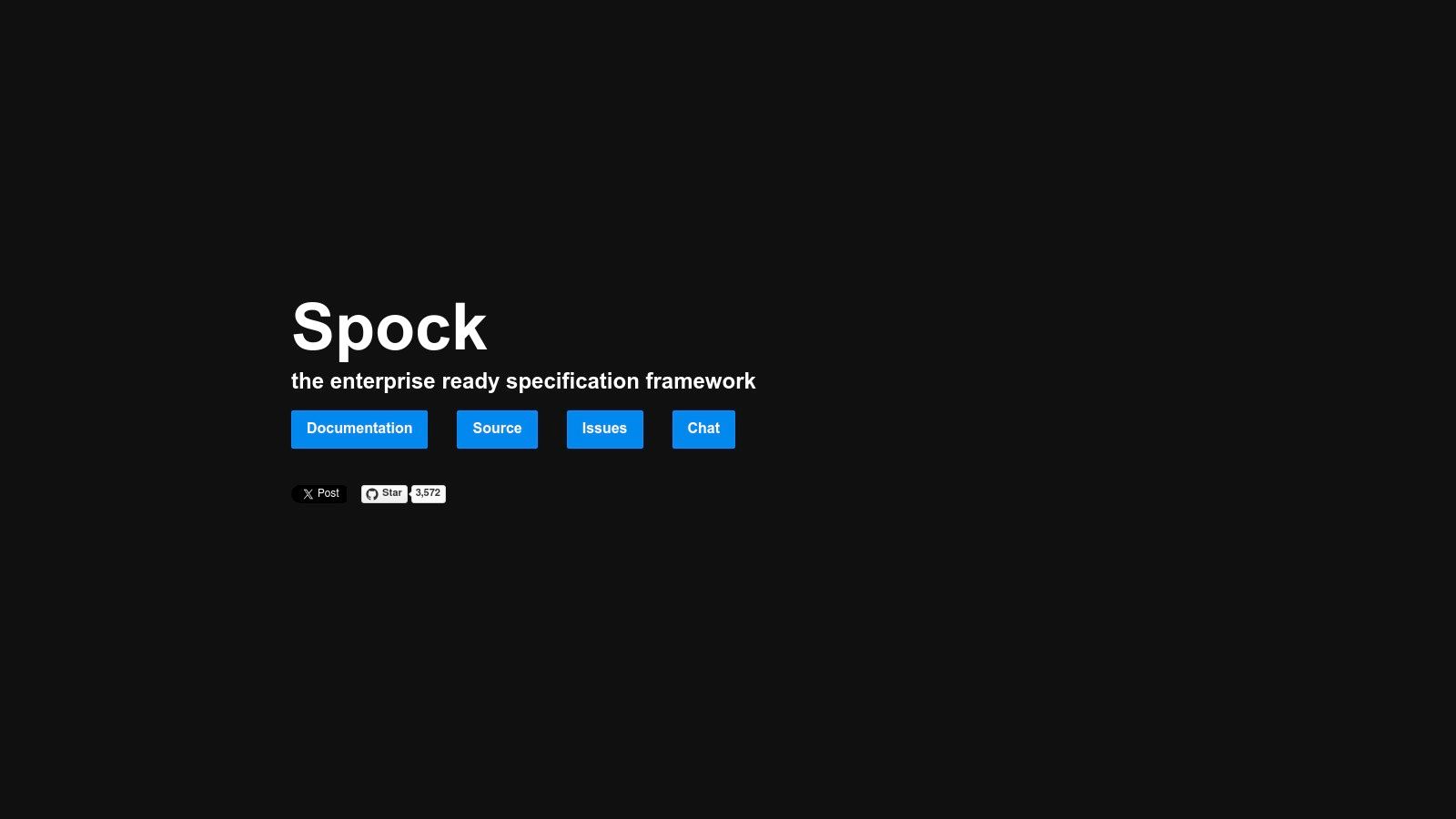
Spock is a testing and specification framework powered by Groovy. Designed for testing Java and Groovy applications, it offers a compelling alternative to traditional Java testing frameworks like JUnit. Its blend of expressiveness, readability, and robust features secures its place on this list. And even though it uses Groovy, Spock integrates seamlessly with JUnit, allowing for its use within existing Java projects.
Spock excels in creating readable tests that also function as documentation. Its expressive specification language uses the familiar given-when-then structure from Behavior-Driven Development (BDD). This structure enables developers to write tests that clearly explain the intended behavior of their code, a valuable asset, especially in complex projects where understanding and maintaining the test suite is critical.
Key Features and Benefits
- Built-in Mocking and Stubbing: Spock simplifies isolating units under test and managing interactions with dependencies thanks to its built-in mocking and stubbing capabilities.
- Data-Driven Testing With Data Tables: Spock shines with data-driven testing. It allows developers to concisely define test cases with various input and output values using data tables. This drastically reduces boilerplate compared to JUnit's parameterized tests.
- Given-When-Then Blocks: The structured approach of given-when-then blocks makes tests easier to read and understand, simplifying the process of following the logic of each test case.
- Detailed Failure Reporting: When tests fail, Spock provides detailed reports, pinpointing the exact cause and speeding up the debugging process.
- Extension System: Spock is highly extensible, allowing developers to customize the framework and integrate it with other tools and libraries.
Practical Applications
- Unit Testing: Spock is perfectly suited for unit testing Java and Groovy code, enabling the creation of concise, expressive tests that clearly define the expected behavior.
- Integration Testing: While designed primarily for unit testing, Spock can be effectively used for integration testing, especially when combined with its mocking capabilities.
- BDD: Spock's given-when-then blocks and focus on readability naturally lend themselves to BDD practices.
Pros
- Highly readable test code that effectively serves as documentation.
- Combines testing, mocking, and BDD in a single framework.
- Concise syntax compared to Java-based alternatives.
- Excellent for testing both Java and Groovy code.
Cons
- Requires some Groovy knowledge.
- Introduces a Groovy dependency to the project.
- Less prevalent in Java-only projects.
- Some Java IDEs might have limited Groovy support.
Technical Requirements
Pricing
Spock is open-source and free to use.
Implementation/Setup Tips
- Manage your Groovy and Spock dependencies with your chosen build tool (Maven or Gradle).
- Consult the official Spock documentation for detailed setup instructions and practical examples: https://spockframework.org/
- Start with simple test cases to familiarize yourself with the Groovy syntax and Spock’s features.
Spock offers a strong alternative to traditional Java testing frameworks. Teams seeking increased readability and the advantages of BDD will find it particularly appealing. While learning Groovy is a prerequisite, the gains in test clarity and maintainability often make the effort worthwhile. Spock's integration with existing Java projects via JUnit makes it a practical option for teams aiming to elevate their testing practices.
6. AssertJ
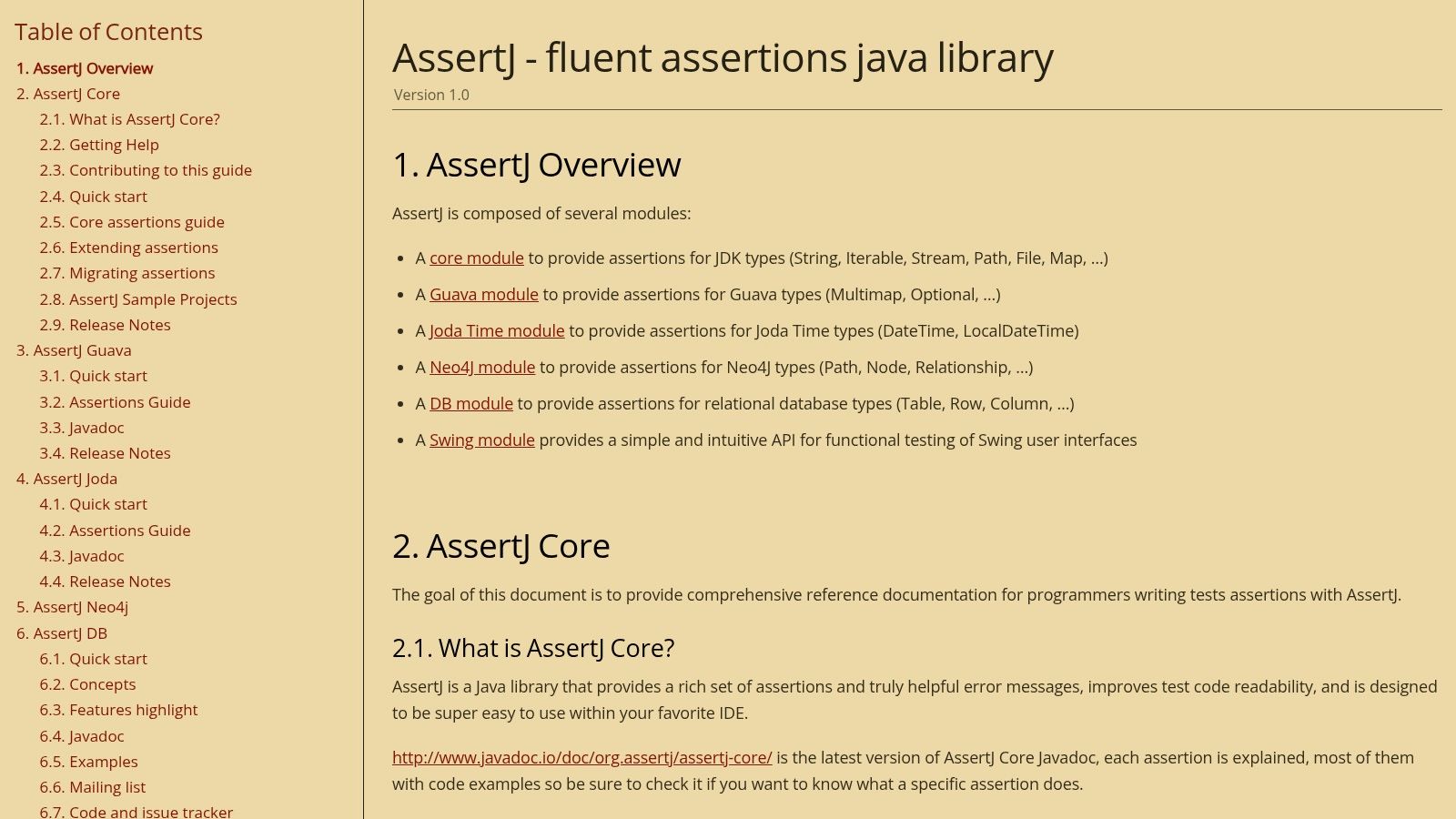
AssertJ stands out thanks to its fluent API, which significantly improves the readability and maintainability of Java test code. While not a standalone framework, it integrates seamlessly with popular testing frameworks like JUnit and TestNG, boosting your assertions with clarity and expressiveness.
AssertJ's main strength is its fluent assertions. Consider verifying multiple properties of an object. With standard JUnit assertions, you'd likely have multiple separate statements. AssertJ allows you to chain these together in a more natural, readable style that resembles plain English.
This is especially helpful when dealing with complex objects and conditions. For example, instead of this:
assertEquals("John Doe", user.getName()); assertTrue(user.isActive()); assertEquals(30, user.getAge());
You can write this:
assertThat(user) .hasName("John Doe") .isActive() .hasAge(30);
This chained approach clarifies your test's intent, especially for new team members or when revisiting older tests. The improved readability leads to better maintainability and less debugging time.
Features
- Fluent Assertion API with IDE Auto-Completion Support: Write assertions in a clear, chained manner with help from your IDE's code completion.
- Rich Set of Assertions: AssertJ offers a comprehensive library of assertions, covering various data types, from primitives and collections to custom objects.
- Detailed Error Messages: Forget cryptic error messages. AssertJ provides descriptive messages that pinpoint exactly where and why assertions failed, simplifying debugging.
- Exception Assertion Support: Easily verify that your code throws the correct exceptions with the expected messages, ensuring robust error handling.
- Custom Assertions: Create custom assertions tailored to your domain objects for more expressive and targeted testing.
Pros
- Highly readable assertions through method chaining.
- Excellent IDE integration and code completion.
- More expressive than standard JUnit assertions.
- Descriptive error messages for easier debugging.
Cons
- Not a standalone testing framework; requires JUnit or TestNG.
- Can involve a learning curve for the extensive API.
- Long assertion chains in complex tests may require breaking them down for readability.
Website: https://assertj.github.io/doc/
Implementation Tips
- Add the AssertJ dependency to your project using your build tool (Maven, Gradle, etc.).
- Familiarize yourself with the core assertion methods (
assertThat
,has*
,contains*
, etc.) and explore the wider API gradually. - Use your IDE's auto-completion to discover available assertions and speed up your test writing.
AssertJ is a valuable tool for any Java developer. By prioritizing readability and giving rich feedback, it helps create cleaner, more maintainable tests. This contributes to better code quality and faster debugging. While there's a learning curve, the benefits outweigh the initial investment. It's especially useful for teams on large projects where clear tests are vital for long-term success. AssertJ is free to use and compatible with any Java version supporting JUnit or TestNG.
7. REST Assured
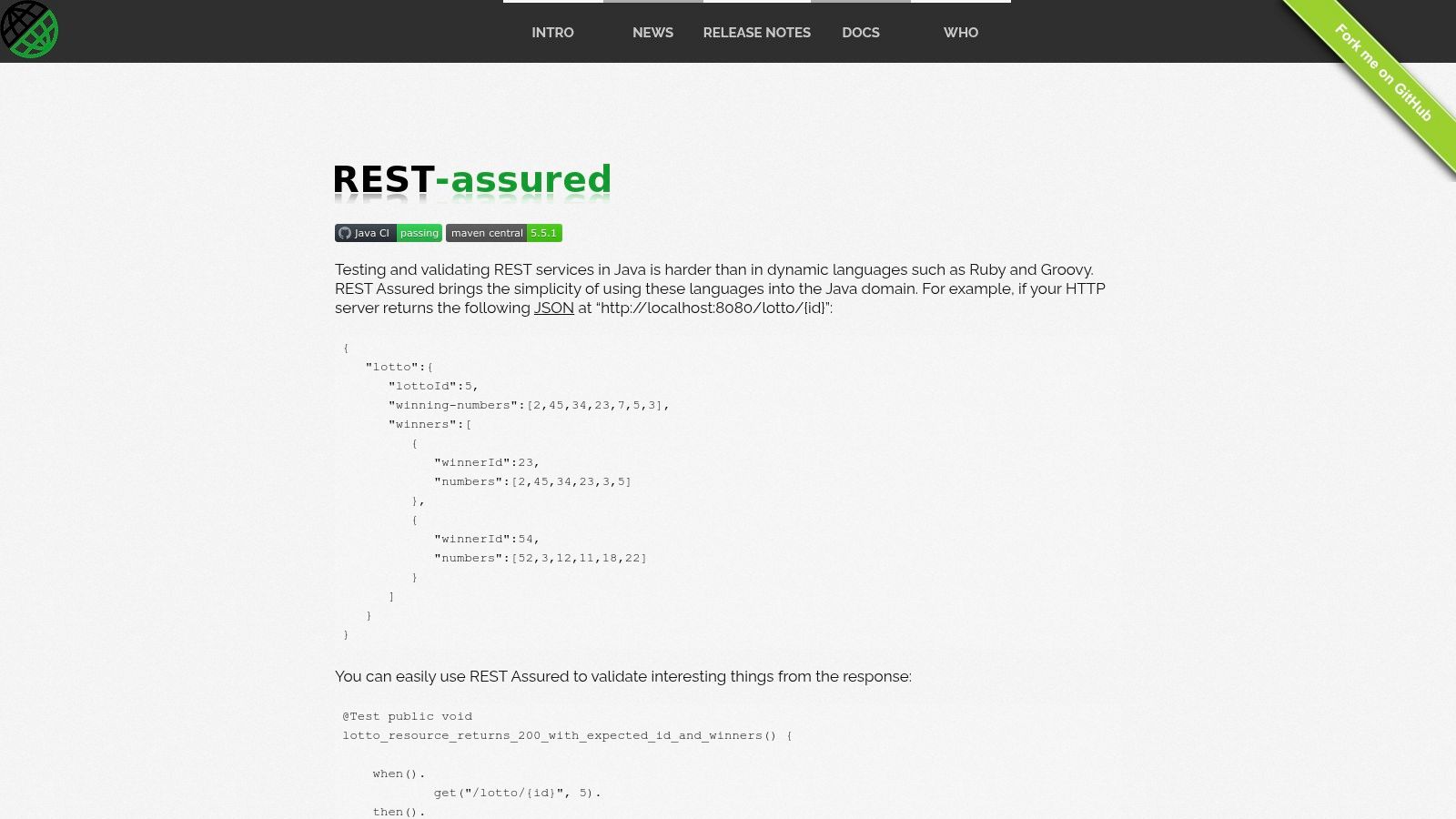
REST Assured simplifies API testing in Java. It combines the ease of dynamic language testing with Java's robust structure, creating a more efficient process. This is especially helpful for teams using agile development and prioritizing fast development cycles.
REST Assured is a Java Domain Specific Language (DSL) that provides a user-friendly API for testing RESTful web services. It works well with Java testing frameworks like JUnit and TestNG, enabling developers to write clear and understandable tests. The tool handles the complexities of HTTP requests, response parsing, and validation, letting developers concentrate on testing the API's core functions.
Key Features and Benefits
- Fluent API: Create HTTP requests (GET, POST, PUT, DELETE, etc.) with a clean and readable syntax. This improves code maintainability and makes it easier to understand. The fluent API design allows for easy chaining of methods to define request parameters, headers, and body content.
- XML and JSON Support: Parse and validate both XML and JSON responses smoothly, accommodating various API formats. REST Assured simplifies extracting key data from responses for validation purposes.
- Built-in Authentication: Handles common authentication methods like Basic, Digest, OAuth, and custom schemes, making security testing more efficient.
- GPath for Response Validation: Use GPath expressions for flexible response validation, enabling checks on complex nested data structures.
- Request/Response Logging: Built-in logging features help with debugging and troubleshooting API interactions by displaying detailed request and response information.
Practical Applications and Use Cases
- Validating API Responses: Confirm that API endpoints return the correct data and status codes. Check for specific values within JSON or XML structures.
- Testing API Authentication and Authorization: Verify secure access to API resources by testing various authentication methods.
- Performance Testing with Integrations: While not a primary performance testing tool, REST Assured integrates with performance testing frameworks to gauge API response times.
- Contract Testing: Validate that APIs comply with defined contracts, ensuring smooth communication between services.
- Integrating with CI/CD Pipelines: REST Assured integrates seamlessly with common CI/CD tools, enabling automated API testing within the development process.
Pros and Cons
Pros:
- Simplifies REST API testing.
- Generates readable and maintainable test code.
- Integrates with standard Java test frameworks.
- Handles intricate response validation scenarios.
Cons:
- Primarily for REST API testing.
- Requires familiarity with the DSL and GPath.
- Can be lengthy for very basic tests.
- May need additional libraries for certain tasks.
Technical Requirements and Setup
- Java Development Kit (JDK): A fundamental requirement for running Java-based applications.
- Dependencies: The REST Assured library, a testing framework (JUnit or TestNG), and any necessary JSON/XML processing libraries. Use Maven or Gradle to manage dependencies. Start with basic tests and progressively learn more advanced features. Utilize the available documentation and online resources.
Comparison with Similar Tools
Compared to tools like Postman or Insomnia, REST Assured offers a more Java-focused approach. While Postman and Insomnia excel at exploratory testing and individual requests, REST Assured is better for integrating API tests into a Java development process and CI/CD pipeline. It provides a structured approach for thorough API testing in Java environments.
REST Assured is a valuable tool for Java developers working with REST APIs. Its intuitive API, extensive features, and smooth integration with the Java environment make it a strong choice for improving API testing.
8. Cucumber-JVM
Cucumber-JVM is the Java implementation of Cucumber, a popular testing tool for Behavior-Driven Development (BDD). It promotes collaboration by allowing test scenarios to be written in Gherkin, a plain-language format easily understood by both technical and non-technical stakeholders. This bridges the communication gap between business analysts, developers, and testers, ensuring everyone understands the project goals.
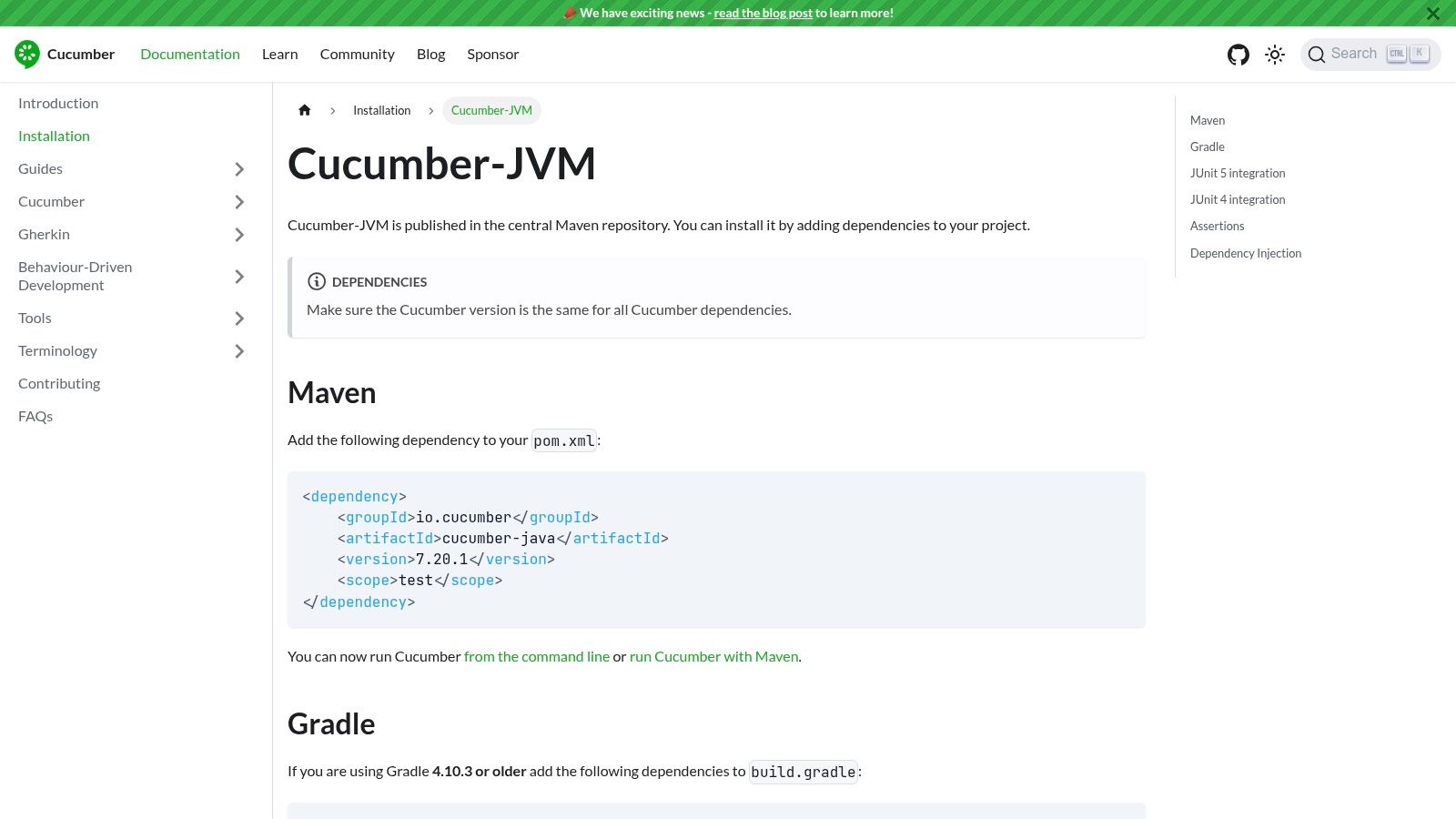
Instead of jumping straight into code, Cucumber-JVM prioritizes defining the application's desired behavior first. This is achieved using feature files written in Gherkin. These files employ a Given-When-Then structure to outline specific scenarios.
For example:
Feature: Login functionality
Scenario: Successful login Given a user is on the login page When the user enters valid credentials And the user clicks the login button Then the user should be redirected to the home page
These Gherkin steps are then connected to Java code through step definitions. This effectively transforms plain-language specifications into executable tests. This not only automates testing but also creates living documentation that reflects the application's current functionality.
Features
Cucumber-JVM offers a range of features:
- Gherkin Language Support: Write tests in an easily readable format.
- Step Definitions: Link Gherkin steps to Java code.
- Cucumber Expressions and Regular Expressions: Offer flexible step matching.
- Data Tables and Doc Strings: Manage complex input data effectively.
- JUnit Integration: Integrate smoothly with JUnit for testing and reports.
- Multilingual Support: Supports multiple languages for broader accessibility.
Pros
Using Cucumber-JVM offers several advantages:
- Improved Communication: Fosters understanding between technical and non-technical team members.
- Living Documentation: Tests become documentation, always up-to-date.
- BDD Promotion: Encourages and supports behavior-driven development.
Cons
There are also some drawbacks to consider:
- Maintenance Overhead: Keeping feature files and step definitions synchronized requires effort.
- Increased Complexity: Setup can be more involved than basic unit testing.
- Potential for Bloat: Step definitions can become unwieldy in larger projects.
- Learning Curve: Gherkin requires some initial learning.
Cucumber-JVM earns its place on this list by addressing a crucial aspect of software development: communication. By establishing a common language for defining and testing software behavior, it promotes collaboration and minimizes misunderstandings. For further reading on software development best practices, consider checking out this resource: Mergify Authors Sitemap.
Implementation Tips
Here are a few tips for implementing Cucumber-JVM effectively:
- Begin with a clear grasp of the feature being tested.
- Write Gherkin scenarios before the step definitions.
- Keep step definitions concise and focused on a single action.
- Utilize data tables and doc strings for complex test data.
Cucumber-JVM is free and integrates easily with Java development environments. It’s a valuable tool for teams adopting BDD and aiming to enhance the quality and maintainability of their software. For installation and documentation, visit the official Cucumber website.
8 Java Testing Frameworks: Side-by-Side Comparison
Item | Core Features ★ | Developer Experience 🏆 | Unique Selling Points ✨ | Target Audience 👥 |
---|---|---|---|---|
JUnit 5 | Modular design with Java 8 support, parameterized tests, nested classes | Excellent IDE/build integration, large community | Backward compatibility via JUnit Vintage | Java developers needing modern testing tools |
TestNG | Annotations, powerful XML configurations, data-driven & dependency testing | Robust for complex suites and flexible test configuration | Built-in features that reduce need for extensions | Developers managing intricate test dependencies |
Mockito | Simple mock creation, stubbing, verification & spy functionality | Intuitive, readable API with great integration with JUnit/TestNG | Lightweight mocking with clear and succinct syntax | Unit testers requiring easy-to-use mocks |
Selenium | WebDriver API, cross-browser automation, advanced user interactions | Industry standard for UI testing with extensive community support | Broad browser/platform compatibility | QA engineers and automation testers |
Spock Framework | Expressive Groovy-based syntax, built-in mocking, data-driven testing, clear BDD blocks | Highly readable and concise tests that double as documentation | Combines testing, mocking, and BDD in one integrated framework | Teams working with both Java and Groovy |
AssertJ | Fluent assertion API with rich, descriptive assertions | Enhances test readability with IDE auto-completion | Detailed error messaging and expressive method chaining | Developers wanting clear and expressive test codes |
REST Assured | DSL for fluent HTTP requests, support for XML/JSON parsing, built-in logging | Simplifies REST API validations with clear and concise syntax | Tailored specifically for REST API testing | API testers and back-end developers |
Cucumber-JVM | Gherkin language for plain language scenarios, step definitions, integration with JUnit | Bridges technical and non-technical teams with readable BDD scenarios | Natural language test scenarios that improve collaboration and clarity | Teams practicing behavior-driven development (BDD) |
Choosing Your Ideal Java Test Framework
Selecting the right Java testing framework hinges on several factors: your project's specific needs, your team's familiarity with different tools, and your overall testing objectives. For example, if unit testing is your primary focus, JUnit 5 or TestNG might be ideal choices.
Need robust mocking? Mockito is a powerful option. For UI testing, Selenium remains the industry standard. Meanwhile, REST Assured excels at API testing.
Other frameworks bring unique advantages to the table. Spock offers a distinctive, Groovy-based approach, while AssertJ streamlines and simplifies assertions. Cucumber-JVM facilitates behavior-driven development (BDD) using its highly readable Gherkin syntax.
Factors to Consider When Choosing a Framework
When making your decision, consider:
- Project size
- Project complexity
- The specific types of tests required (unit, integration, UI, etc.)
Getting Started With Your Chosen Framework
Once you've chosen a framework, start small. Implement a simple, well-defined test case. The framework's documentation should provide detailed setup instructions and best practices – most frameworks offer extensive documentation and active community support. Begin with basic assertions and gradually incorporate more advanced features like mocking, parameterized tests, and test runners.
Budget and Integration
Budget is another key consideration. While many frameworks are open-source and free, integrating them into your CI/CD pipeline might require additional resources, especially for features like parallel test execution and detailed reporting. Evaluate the potential return on investment of a thorough testing strategy against the cost of implementation and maintenance.
Integration and compatibility are also essential. Your chosen framework should integrate seamlessly with your current development environment, including your IDE, build tools (like Maven or Gradle), and CI/CD platform. Confirm compatibility with other libraries and frameworks already in use. Choosing a framework with a strong community and active development will also help future-proof your decision.
By carefully evaluating these eight leading Java testing frameworks and considering factors like project scope, team expertise, budget, and integration needs, you can build a testing strategy that ensures code quality and application stability for years to come.
Streamlining Your Workflow with Mergify
Effective testing is only part of the equation. Managing your code integration workflow efficiently is just as crucial. Mergify provides a powerful solution for seamless code integration, focusing on reducing CI costs, improving code security, and minimizing developer frustration.
Mergify uses tools like Merge Queue and Merge Protections to automate pull request updates and strategically batch CI jobs, minimizing conflicts and promoting stable codebases. The platform's CI Issues feature uses AI to detect and categorize infrastructure problems, which speeds up issue resolution. From intelligent scheduling and prioritization to expedited critical updates and optimized merge timings, Mergify empowers engineering teams to manage complex development environments with ease, saving both time and money. Supercharge your development workflow with Mergify today!