Java Test Framework: Top 7 Picks for 2025
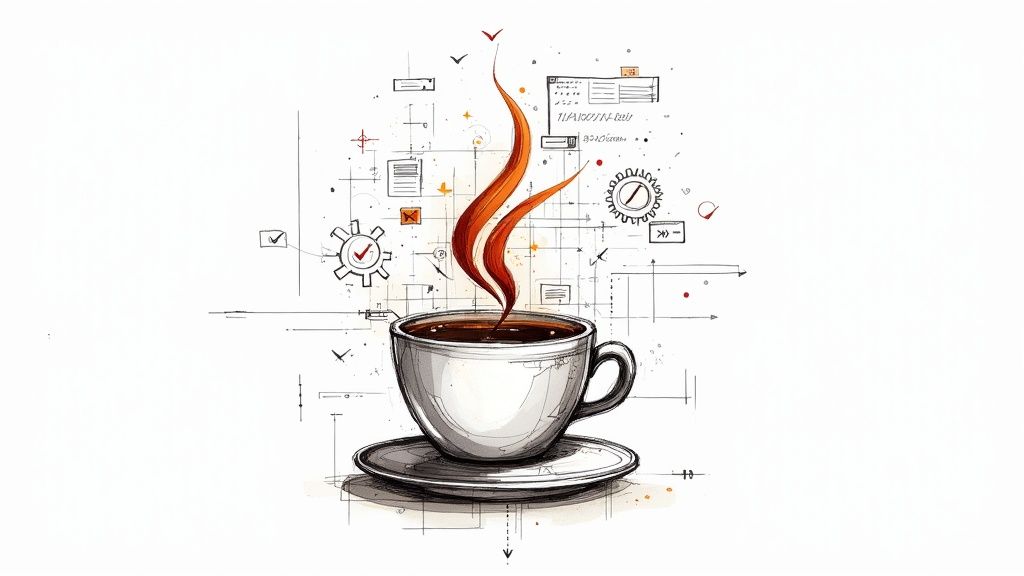
Building Rock-Solid Java Apps: Choosing the Right Test Framework
Ensuring the quality and reliability of your Java applications is critical. A robust Java test framework is essential for catching bugs early, maintaining code integrity, and delivering high-quality software. From unit tests to integration tests and even UI tests, the right framework can significantly impact your development lifecycle. But with so many options, how do you choose the best fit for your project?
Choosing an effective Java testing framework requires considering several key factors. Think about the scope of your testing needs. Do you primarily need unit testing, or are you looking for end-to-end testing capabilities? Seamless integration with your existing CI/CD pipeline is another important factor. How important is the readability and maintainability of your test code?
Technical considerations also play a vital role. These include support for different testing styles (like behavior-driven development), ease of mocking dependencies, and the availability of built-in assertion libraries. Some frameworks, like JUnit, offer commercial support and advanced features. Others, such as TestNG, are open-source and community-driven. These differences can impact both cost and available resources.
This article explores seven leading Java test frameworks to help you navigate this critical decision.
Key Framework Considerations
- JUnit: A widely-used framework known for its simplicity and extensive community support.
- TestNG: A powerful framework offering advanced features like annotations, parallel testing, and data-driven testing.
- Mockito: A popular mocking framework that simplifies the process of isolating units of code for testing.
- Spock: A testing and specification framework that combines features of JUnit and Mockito with a Groovy-based DSL.
- Cucumber: A framework that supports behavior-driven development (BDD) and allows writing tests in plain language.
- Robot Framework: A generic test automation framework that can be used for various types of testing, including web testing and API testing.
- Serenity BDD: A framework built on top of Selenium and WebDriver that focuses on creating living documentation for your tests.
We’ll explore the strengths and weaknesses of each framework and examine their ideal use cases. We’ll also compare their features to help you choose the perfect testing companion for your next Java project. By understanding these frameworks, you'll be equipped to build rock-solid Java applications with confidence.
1. JUnit 5
JUnit 5 stands as the modern standard for developer-side testing in Java. Originally created in 2002, JUnit has continually evolved. JUnit 5 represents a significant advancement, blending the best of JUnit 4 with new features and a more flexible architecture. This makes it a vital tool for Software Development Teams, DevOps Engineers, QA Engineers, and anyone building robust Java applications.
JUnit 5 comprises three sub-projects:
- JUnit Platform: This serves as the base for launching testing frameworks on the JVM. It's designed to be engine-agnostic, supporting various testing frameworks, not just JUnit.
- JUnit Jupiter: This component offers the new programming and extension models for writing tests. Key features include lambda expressions for cleaner test declarations, nested test classes for enhanced organization, and parameterized tests for diverse inputs.
- JUnit Vintage: This sub-project maintains backward compatibility. You can run JUnit 3 and 4 tests alongside your JUnit 5 tests, which is essential for teams transitioning from older versions.
Extensibility and Parameterized Tests
One of JUnit 5's most powerful features is its extensibility. The Extensions API lets developers customize the testing lifecycle, from adding custom annotations to controlling test execution. For example, you can create extensions to run tests based on the operating system, Java version, or even system resources. This flexibility is a major improvement over previous JUnit versions.
Parameterized tests are another key advantage. Instead of writing multiple test methods for different inputs, you define a single method and provide it with various data sources. This reduces code duplication and improves test coverage.
Pros and Cons of JUnit 5
Here's a quick look at the advantages and disadvantages of using JUnit 5:
Pros:
- Industry Standard: Widespread adoption means ample resources and community support are available.
- Seamless Integration: Works well with popular IDEs like IntelliJ IDEA and Eclipse, and build tools like Maven and Gradle.
- Excellent Documentation: Comprehensive documentation and many examples simplify getting started and troubleshooting.
- Modular Architecture: Extensions allow for highly customized testing.
Cons:
- Java 8+ Requirement: JUnit 5 requires Java 8 or later.
- Migration Effort: Migrating from JUnit 4 may require code changes, although JUnit Vintage helps.
- Increased Complexity: The modular architecture adds some complexity compared to earlier versions.
Practical Applications of JUnit 5
JUnit 5 excels in a variety of testing scenarios:
- Unit Testing: Verify individual code units, like methods or classes.
- Integration Testing: Test the interaction between different application components.
- Component Testing: Test larger application parts in isolation.
- Test-Driven Development (TDD): The flexible API supports a TDD workflow.
You might find more development-related content on our Mergify Blog Sitemap.
JUnit 5 is open-source. For more information and downloads, visit the official JUnit 5 website. Its modern features, community support, and integration with the Java ecosystem make JUnit 5 an essential tool for any Java developer striving for high-quality code.
2. TestNG
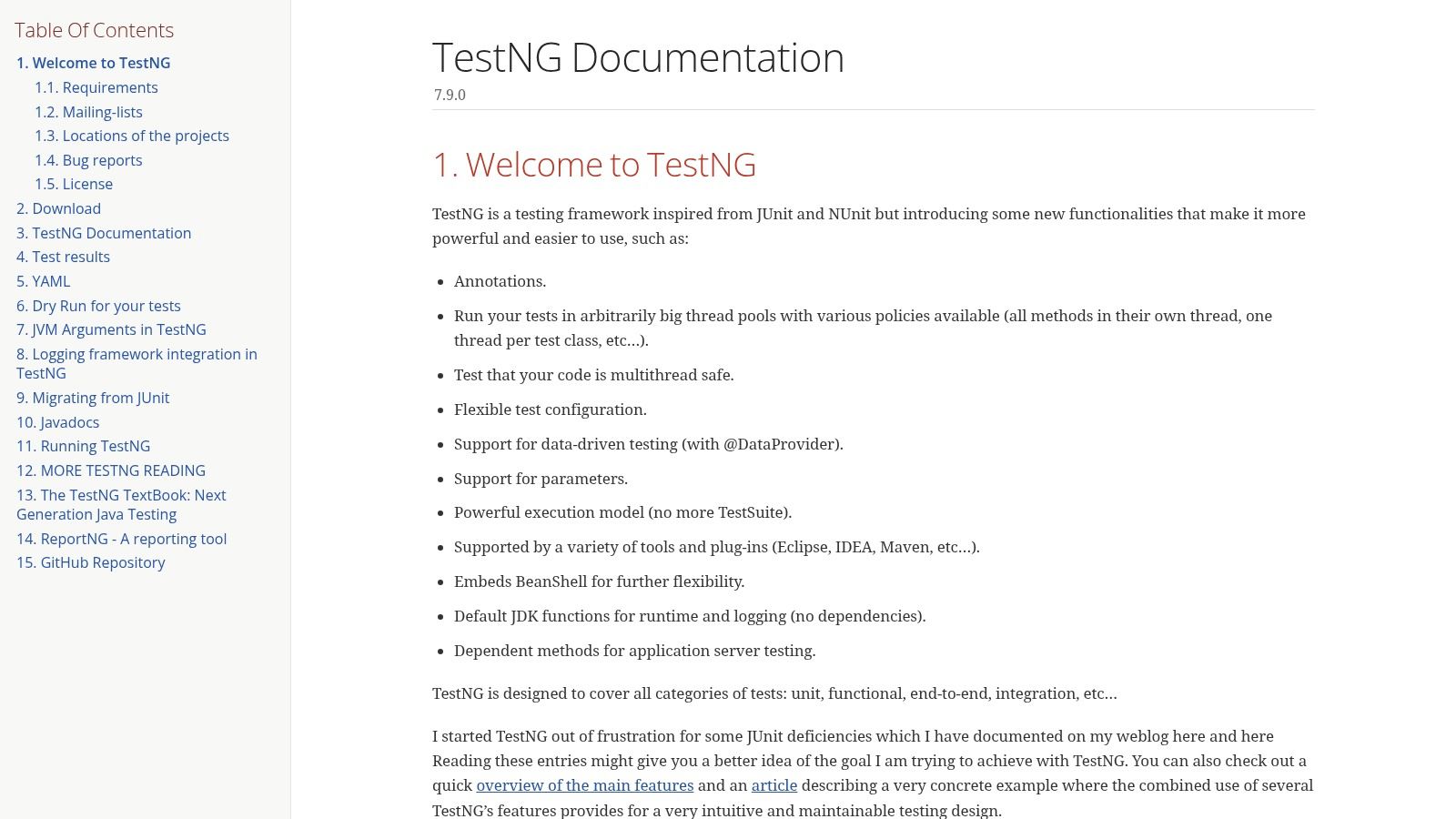
TestNG is a robust Java testing framework that offers more than just basic unit testing. Building upon the foundations of JUnit and NUnit, it provides a richer set of features, making it suitable for a wider range of testing needs, from unit and functional testing to integration and end-to-end testing. This makes it a compelling choice for projects of any size, especially those with increased complexity.
One of TestNG's standout features is its flexible test execution model. Using annotations like @BeforeClass
, @AfterClass
, @BeforeMethod
, @AfterMethod
, @BeforeSuite
, and @AfterSuite
, developers gain precise control over test setup and teardown processes. This granular control is particularly valuable when dealing with complex interdependencies within test methods and suites. For instance, @BeforeClass
ensures specific setup logic runs only once before any test methods in a class are executed.
TestNG also simplifies test grouping and ordering. With XML configuration files, you can easily categorize tests into groups like "smoke," "regression," or "sanity." You can also establish dependencies between these groups. This facilitates sophisticated test execution strategies. You could, for example, execute only the "smoke" tests for a quick build verification. Or you could mandate that integration tests run only after successful unit tests.
Data-driven testing is another area where TestNG shines. The @DataProvider
annotation empowers you to supply test methods with various data sets. This helps reduce code duplication and significantly improves test coverage. It's particularly useful for testing with different inputs and scenarios without creating separate test methods for each.
Parallel test execution is a key feature for efficiency. TestNG allows running tests concurrently across multiple threads. This dramatically reduces overall test execution time, a crucial advantage for large projects with extensive test suites and fast-paced CI/CD pipelines.
Migrating from JUnit to TestNG is generally smooth. This makes it a practical option for teams wanting to upgrade their testing capabilities. While TestNG may initially present a slightly steeper learning curve compared to JUnit, the added features and flexibility make it a worthwhile investment for handling complex testing scenarios.
Features
- Powerful configuration annotations (
@BeforeClass
,@AfterSuite
, etc.) - Flexible test grouping and execution ordering
- Built-in support for data-driven testing
- Parallel test execution
- Integrated support for test dependencies
Pros
- More comprehensive than JUnit for complex testing scenarios
- Better support for integration and system testing
- Built-in HTML reports
- Straightforward migration from JUnit
Cons
- Steeper learning curve than JUnit
- Less IDE integration than JUnit
- Smaller community than JUnit
You might be interested in: Mergify Authors for further insights.
Website: https://testng.org/
Implementation Tip
Start using TestNG for new test cases. Gradually migrate existing JUnit tests to take advantage of TestNG’s advanced features like grouping and parallel execution. Explore the XML configuration options to create custom test suites and execution strategies tailored to your specific project needs.
3. Mockito
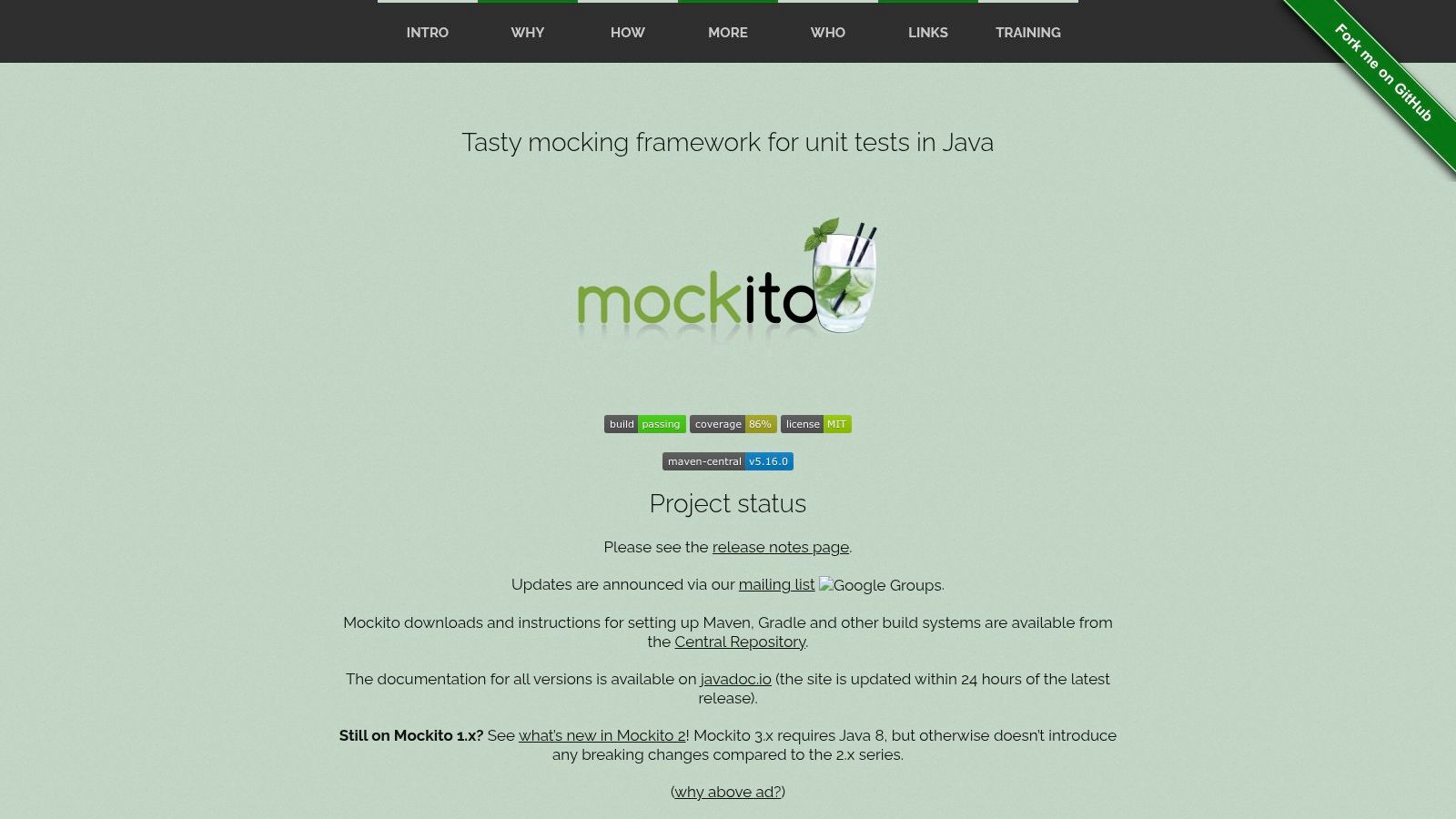
Mockito is a prominent mocking framework within the Java environment, simplifying the creation of reliable unit tests. Its straightforward API makes it a popular choice for developers looking to isolate code units and verify their behavior independently of external systems or complex object relationships. Mockito's core function is creating test doubles, such as mocks and spies, which simulate the actions of real objects – a vital aspect of effective unit testing.
What sets Mockito apart? It excels at crafting realistic simulations of object interactions. Developers can define expected mock behavior using a fluent API, eliminating the need for complicated setup procedures. This enhances the readability and maintainability of tests. You can effortlessly confirm a method call with specific arguments or configure a method to return a preset value. This allows developers to concentrate on testing the unit's logic without the distraction of external dependencies.
Key Features and Benefits
- Simple and Readable API: Mockito's intuitive API streamlines the creation of mock objects and defines their behavior concisely, reducing boilerplate code and improving test clarity.
- Behavior Verification: Confirm that specific methods were called on a mock object with the correct arguments, ensuring intended object interactions.
- Stubbing Method Calls: Specify custom return values for method calls on mock objects to simulate different scenarios and test various code paths.
- Argument Matchers: Employ adaptable matchers to verify interactions based on argument patterns, leading to more robust and less fragile tests.
- Spy Objects: Partially mock real objects, overriding specific methods while preserving the original behavior of others. This is particularly helpful when testing legacy code or intricate situations.
Pros
- Intuitive API with Minimal Learning Curve: Developers can quickly master Mockito’s syntax and begin writing effective tests.
- Excellent IDE Integration: Features like auto-completion within IDEs boost productivity and minimize errors.
- Clear Error Messages: Mockito delivers helpful feedback when tests fail, simplifying debugging and issue resolution.
- Actively Maintained: Mockito is a well-supported project with a strong community and consistent updates.
Cons
- Not a Comprehensive Testing Framework: Mockito is designed to work in conjunction with testing frameworks like JUnit or TestNG.
- Limitations with Final Classes and Static Methods: Mocking these elements often requires supplementary libraries like PowerMock or alternative solutions.
- Complex Mocking Scenarios: While generally simplifying testing, highly complex interactions might necessitate more involved setup.
Implementation/Setup Tips
- Include the Mockito dependency in your project's build file (e.g., Maven's
pom.xml
or Gradle’sbuild.gradle
). - Use the
@RunWith(MockitoJUnitRunner.class)
annotation in your test class (with JUnit) to activate Mockito annotations. - Use
@Mock
to create mock objects. - Use
when(mock.methodCall()).thenReturn(value)
to stub method calls. - Use
verify(mock).methodCall()
to verify interactions.
Comparison with Similar Tools
Mockito is frequently compared to EasyMock and JMockit. While all provide mocking capabilities, Mockito’s simplified API and focus on behavior verification make it a preferred option. EasyMock tends to be more verbose, while JMockit, though powerful, has a steeper learning curve.
Website: https://site.mockito.org/
Pricing: Mockito is open-source and free to use.
Mockito’s user-friendliness, combined with its powerful features, makes it a valuable tool for Java developers employing test-driven development (TDD) and aiming for high-quality, well-tested code. It simplifies the complexities of unit testing, allowing developers to focus on verifying core application logic and ensuring robust software.
4. Spock Framework
Spock Framework is a powerful and expressive testing framework designed for Java and Groovy applications. By leveraging Groovy's dynamic nature, Spock enables developers to write concise and readable tests that often resemble natural language. This readability is particularly beneficial for teams looking to improve collaboration between technical and non-technical stakeholders.
Spock's strength lies in its structured approach to testing. It uses blocks like given
, when
, then
, expect
, and cleanup
to clearly define the different phases of a test case. This structure, combined with Groovy's expressive syntax, results in highly maintainable and self-documenting tests.
Practical Applications and Use Cases
- Unit Testing: Ideal for testing individual units of code. Spock's built-in mocking and stubbing capabilities help isolate dependencies. For example, mocking a database connection allows testing data access logic without a real database.
- Integration Testing: Verify the interactions between different application components. Spock's structured approach clearly defines the setup, execution, and verification steps.
- Data-Driven Testing: Spock's data tables enable running the same test with multiple sets of input data, efficiently testing various scenarios. This is especially useful for validating input validation logic or testing edge cases.
- BDD (Behavior-Driven Development): Spock's natural language-like syntax makes it well-suited for BDD, improving communication between developers, testers, and business analysts.
Features and Benefits
- Expressive Specification Language: The Groovy-based syntax allows for more concise and readable tests than traditional Java testing frameworks.
- Built-in Mocking and Stubbing: Create mock objects and define their behavior with ease, simplifying dependency management during testing.
- Powerful Assertion Framework: Offers a rich set of assertions to verify expected outcomes.
- Data Tables for Data-Driven Testing: Execute tests with multiple input data sets for increased testing efficiency.
- Structured Test Phases (given, when, then, expect, cleanup): Enhance test readability and maintainability.
Pros
- Extremely Readable Test Code: Acts as living documentation, easily understood by all team members.
- Concise Syntax: Requires less boilerplate code than JUnit, leading to faster test development.
- Comprehensive Failure Reporting: Provides detailed diagnostics for simpler debugging.
- Seamless Integration with Java Code: Works well within existing Java projects.
Cons
- Groovy Requirement: Requires Java developers to learn Groovy, which presents a learning curve. However, the benefits of Groovy's expressiveness often outweigh this.
- Slower Adoption in Pure Java Projects: Although Spock integrates with Java, it’s more common in projects already using Groovy.
- Potential Performance Overhead: Groovy's dynamic nature may introduce a minor performance overhead compared to pure Java frameworks. This is usually negligible.
Comparison with JUnit
While JUnit remains a widely used Java testing framework, Spock provides improved readability and conciseness, particularly for complex tests. The structured approach and built-in mocking capabilities increase its attractiveness.
Implementation/Setup Tips
- Use a build tool like Gradle or Maven to manage dependencies and include the Spock library.
- Use IDE integrations (like IntelliJ IDEA) for enhanced code completion and debugging.
- Start with smaller unit tests and gradually explore advanced features.
Pricing and Technical Requirements
Spock is open-source and freely available. It requires a Java Development Kit (JDK) and a Groovy environment.
Website: https://spockframework.org/
Spock Framework is a worthwhile addition to this list. It offers a compelling alternative to traditional Java testing frameworks. Its focus on readability, combined with features like built-in mocking and data-driven testing, makes it a valuable tool for development teams aiming for high-quality, maintainable code. The Groovy requirement, while initially a hurdle, is often outweighed by the benefits of test clarity and conciseness, especially for complex projects.
5. Selenium WebDriver
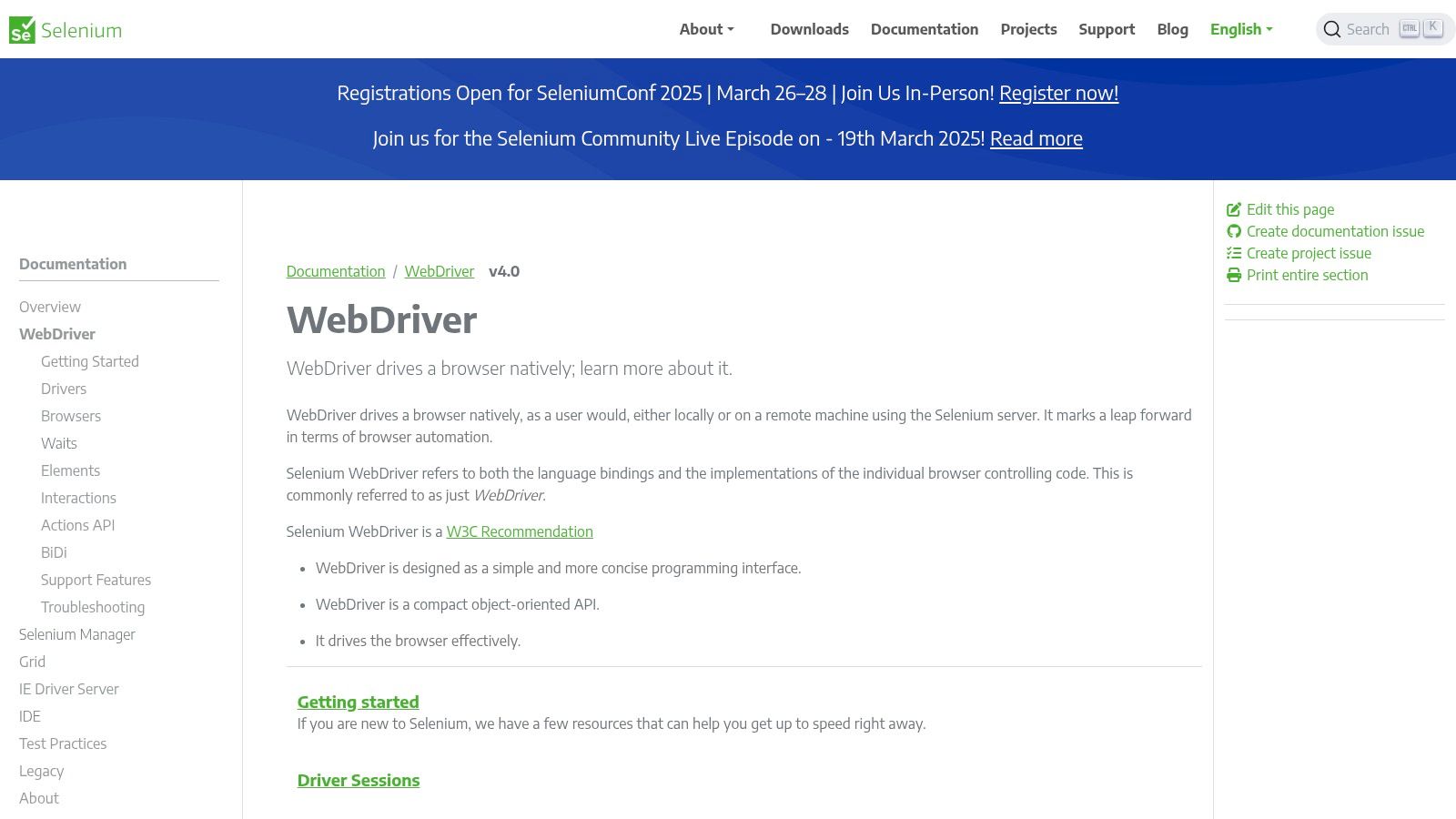
Selenium WebDriver is the industry standard for browser automation. It's a critical tool for end-to-end testing of web applications. With Selenium, you can simulate user interactions within a web browser, ensuring your application works correctly from the user's perspective. This helps verify key workflows and catch bugs before they impact users.
Selenium offers a robust programming interface in several languages, including Java, empowering developers to build comprehensive browser-based regression automation suites. It supports a wide array of browsers like Chrome, Firefox, Safari, and Edge, enabling thorough cross-browser testing.
Key Features and Benefits
Here's a breakdown of what makes Selenium WebDriver so powerful:
- Cross-Browser Compatibility: Ensure a consistent experience across various browsers.
- User Interaction Simulation: Test real-world scenarios by simulating clicks, form submissions, and navigation.
- Advanced Interactions: Perform complex actions such as drag-and-drop and keyboard input.
- Asynchronous Web Element Handling: Manage dynamic elements with wait mechanisms, leading to more reliable tests.
- Integration with Testing Frameworks: Works seamlessly with popular Java testing frameworks like JUnit and TestNG.
- Headless Browser Testing: Run tests without a visible browser window for faster execution in CI/CD pipelines.
- Open-Source and Free: Easily accessible and free to use.
You might be interested in: Mergify Sitemaps for more information related to development best practices.
Pros and Cons
Like any tool, Selenium has its strengths and weaknesses:
Pros:
- Industry Standard: Widely adopted and recognized for browser automation.
- Large Community and Ecosystem: Benefit from ample documentation, support forums, and numerous tools.
- Supports Headless Testing: Perfect for automated CI/CD integration.
Cons:
- Test Brittleness: UI changes can require frequent test maintenance.
- Slower Execution: Browser automation is inherently slower than other testing types.
- Learning Curve: Handling complex interactions and asynchronous elements can be challenging.
- Browser Driver Management: Managing browser-specific drivers adds complexity.
Implementation Tips
Here are some tips for effective Selenium usage:
- Page Object Model (POM): Organize your test code and minimize duplication.
- Explicit Waits: Handle asynchronous elements and improve test reliability.
- Browser Developer Tools: Inspect elements and find locators efficiently.
- WebDriverManager: Simplify driver setup and management.
Selenium WebDriver is a vital tool for any Java team focused on delivering high-quality web applications. While there's a learning curve and ongoing maintenance, the advantages of comprehensive browser automation outweigh the challenges. Using Selenium strengthens the reliability and stability of your web applications.
6. AssertJ
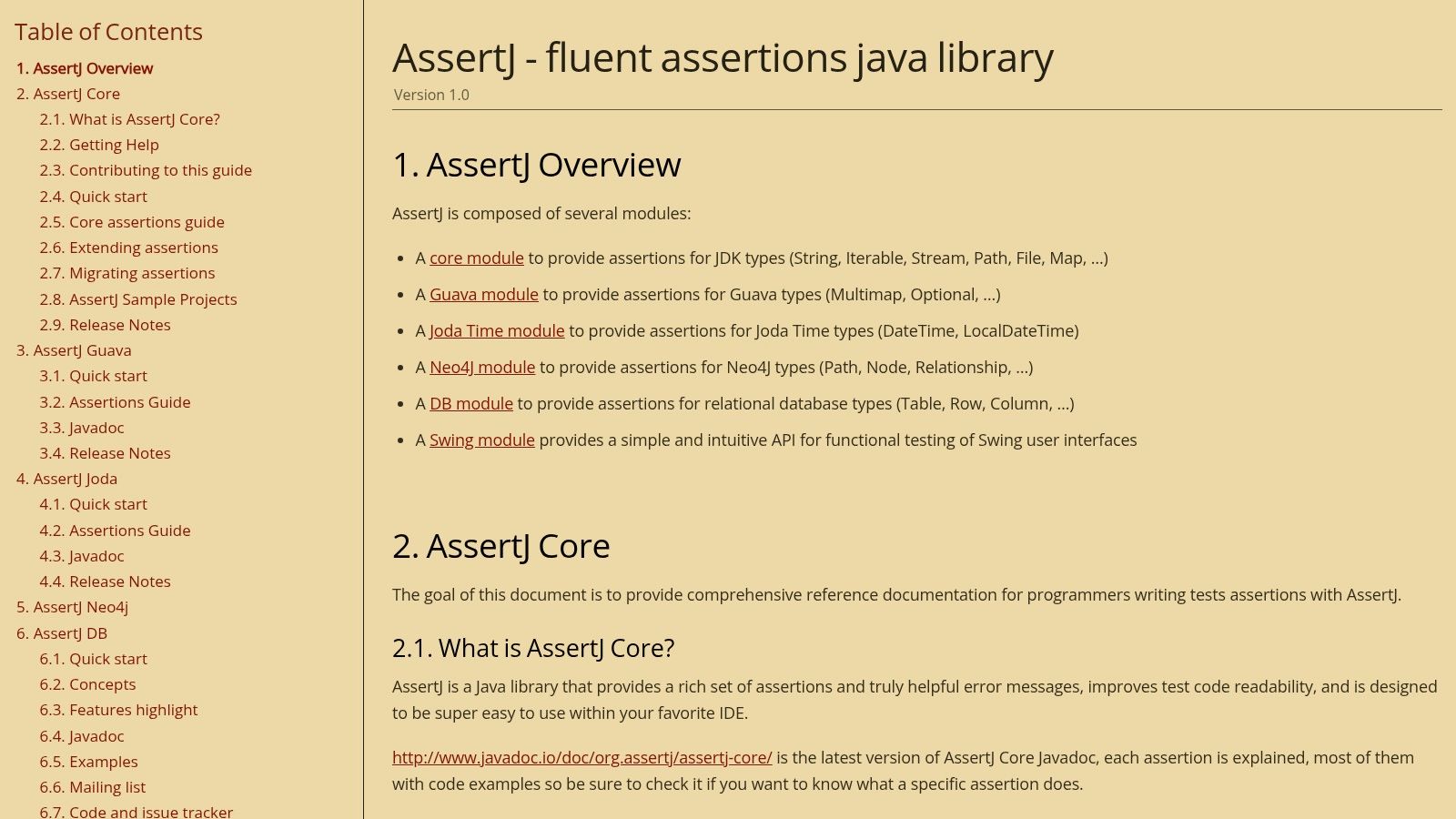
AssertJ stands out for its ability to make Java test code more readable and maintainable. It's not a testing framework on its own, but it integrates smoothly with popular frameworks like JUnit and TestNG to improve your assertions. This is especially useful for teams that value clean code and want to make debugging easier. Forget deciphering confusing error messages. AssertJ provides clear, descriptive feedback, saving you valuable debugging time.
AssertJ's power comes from its fluent API, which allows assertions to read like natural language. This improves code readability and makes tests easier to understand, particularly in complex scenarios. Let's look at a simple example comparing a standard JUnit assertion with AssertJ.
JUnit vs. AssertJ: A Simple Comparison
JUnit:
assertEquals("Expected string value", actualString);
AssertJ:
assertThat(actualString).isEqualTo("Expected string value");
The difference is subtle, but the AssertJ version is more expressive and easier to follow. The real advantage comes when you chain assertions together, like when you're checking multiple properties of an object.
assertThat(user) .isNotNull() .hasFieldOrPropertyWithValue("name", "John Doe") .hasFieldOrPropertyWithValue("age", 30);
This fluent style creates more maintainable tests and allows team members to easily understand the purpose of each assertion.
Key Features and Benefits
Here's a breakdown of what AssertJ offers:
- Fluent API with Method Chaining: This is the heart of AssertJ, creating readable and expressive assertions.
- Rich Set of Assertions: AssertJ provides a wide range of assertions covering various Java types, including collections, strings, dates, and more.
- Comprehensive Error Messages: When tests fail, AssertJ gives you detailed error messages, helping you quickly identify the problem.
- Soft Assertions: Instead of stopping at the first failed assertion, soft assertions gather all failures, providing a more complete picture of the test results.
- Custom Assertion Extensions: For specialized needs, AssertJ lets you create your own custom assertions tailored to your specific objects.
These features translate to tangible benefits:
- Highly Readable Test Code: Improved clarity reduces maintenance time and effort.
- Detailed Failure Messages: Debugging becomes faster and more efficient.
- Actively Maintained: Regular updates ensure compatibility and access to new features.
- Easy Integration: Works seamlessly with existing Java testing frameworks.
Potential Drawbacks
While AssertJ is powerful, it's important to be aware of potential drawbacks:
- Not a Standalone Framework: Requires a separate testing framework like JUnit.
- Learning Curve: Mastering the extensive API takes time and practice.
- Potential Verbosity: In very simple cases, the fluent style might add a little extra code.
Pricing, Technical Requirements, and Implementation
AssertJ is open-source and free. It works with Java 8 and later and can be easily added to your project using build tools like Maven or Gradle. Here are a few implementation tips:
- Add the AssertJ dependency to your project's
pom.xml
(Maven) orbuild.gradle
(Gradle). - Import the necessary static methods from
org.assertj.core.api.Assertions
to useassertThat
and other assertion methods. - Take advantage of IDE auto-completion to explore the available assertions.
- Use soft assertions when you need a complete view of multiple failures within a test.
Comparison and Final Thoughts
Other assertion libraries like Hamcrest and Truth exist, but AssertJ’s fluent API and rich feature set make it a compelling choice. The emphasis on readability and detailed error messages makes it particularly suitable for projects with complex testing requirements. By using AssertJ, you're investing in more expressive and maintainable tests, ultimately improving code quality and debugging efficiency in your Java project. For more information, visit the official AssertJ website.
7. REST Assured
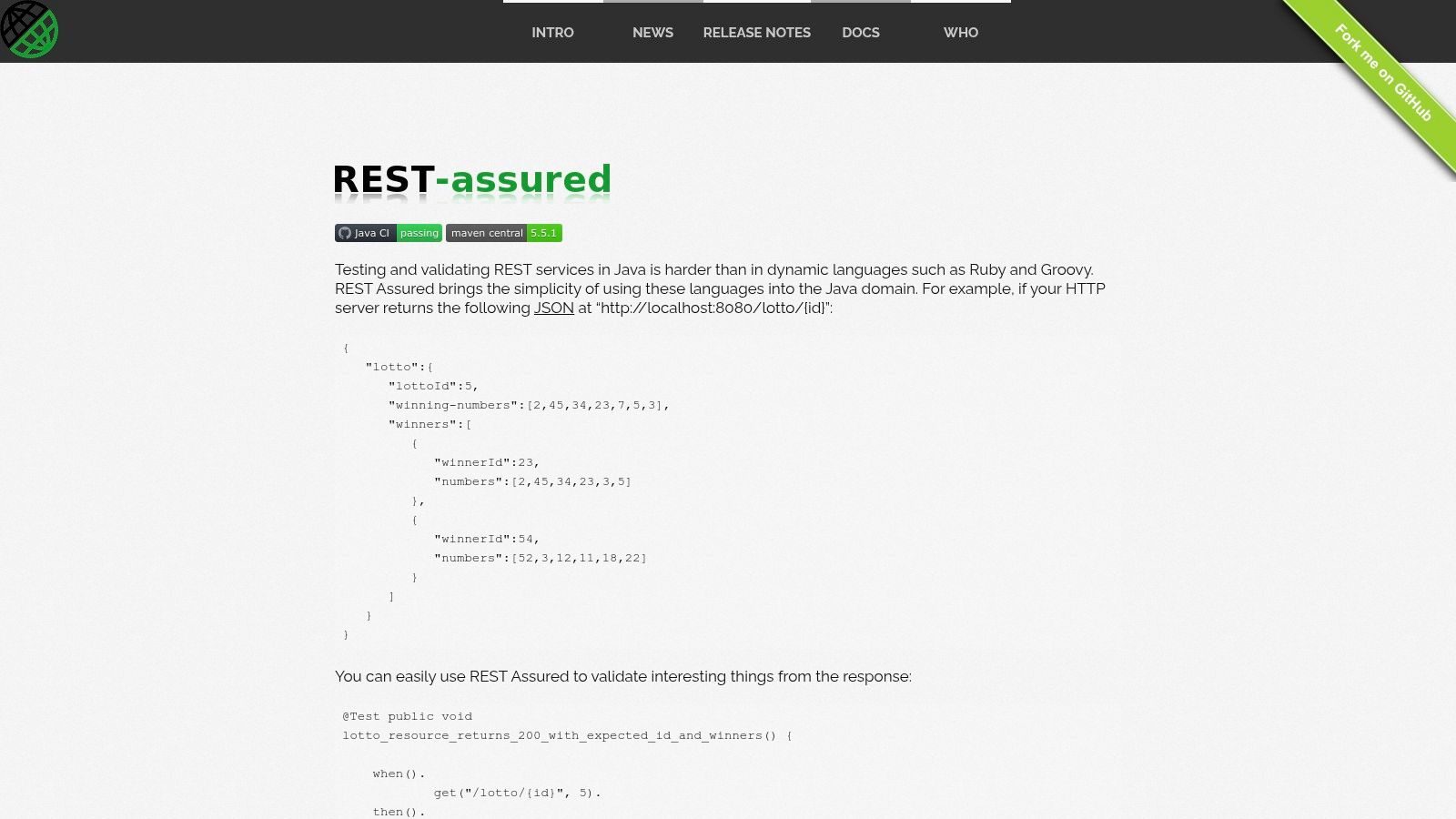
REST Assured is a valuable Java library designed for simplifying REST API testing. Its Domain-Specific Language (DSL) helps developers write clear and understandable tests that mirror the structure of HTTP requests and responses. This makes it a useful tool for software development teams, DevOps engineers, QA engineers, and anyone involved in ensuring the reliability of RESTful web services.
REST Assured is particularly helpful when you need to thoroughly test API endpoints, validate different response aspects, and integrate these tests smoothly into your current testing setup.
Common Use Cases
Let's look at some practical applications of REST Assured:
- Validating Response Codes: Confirm your API returns the correct HTTP status codes (like 200 OK or 400 Bad Request).
- Verifying Response Body Content: Use XML and JSON path expressions to check specific elements within the response body. For example, verify a JSON response has the right key-value pairs.
- Testing Authentication: Implement and test security measures like Basic Authentication or OAuth 2.0. REST Assured has built-in support for these mechanisms.
- Reusing Specifications: Define reusable specifications for common request headers, parameters, or response structures to maintain consistency and reduce redundant code.
- Integration With Testing Frameworks: Combine REST Assured with popular testing frameworks such as JUnit and TestNG for features like parameterized tests and reporting.
Features and Benefits
Here’s a breakdown of REST Assured's key features and their advantages:
- Fluent API: Write tests in a more natural and readable style.
- XML and JSON Support: Easily handle both XML and JSON request and response data.
- Authentication Support: Simplify security testing with built-in authentication support.
- Specification Reuse: Promote consistency and reduce code duplication in tests.
- JUnit/TestNG Integration: Enable structured testing and detailed reporting.
Pros and Cons
Like any tool, REST Assured has strengths and weaknesses:
Pros:
- Simplified API Testing: Much easier than using lower-level HTTP clients.
- Readable Tests: Clear syntax for maintainable tests.
- Good Documentation: Plenty of resources and examples to guide you.
- Thorough Validation: Supports a wide range of validation scenarios.
Cons:
- REST API Focus: Limited to testing RESTful services.
- Potential Verbosity: Can get lengthy for very complex requests or responses.
- Advanced Features: Requires some effort to master the more advanced functionalities.
- Not for Performance: Not designed for performance testing or load testing.
Getting Started
REST Assured is open-source and free to use. To get started, add the REST Assured dependency to your project's pom.xml
(Maven) or build.gradle
(Gradle). Check out the official documentation (https://rest-assured.io/) for tutorials and examples, beginning with simple GET requests and gradually exploring more advanced features as you need them. You can also find numerous online resources to help you quickly become proficient with REST Assured.
7 Java Testing Frameworks: Side-by-Side Comparison
Tool | Core Features (✨) | User Experience (★) | Unique Benefits (🏆) | Target Audience (👥) |
---|---|---|---|---|
JUnit 5 | Lambda expressions, nested & parameterized tests, extensions | Seamless IDE integration, excellent documentation | Industry standard, modular architecture | Java developers seeking modern unit testing |
TestNG | Flexible configuration, test grouping, parallel execution | Built-in HTML reports, comprehensive integration support | Advanced dependency management and diverse test types | Teams handling unit, integration and system tests |
Mockito | Simple API for mocks, behavior verification, stubbing | Intuitive, minimal learning curve, IDE friendly | Focused mocking framework with clear error messages | Developers needing robust behavior verification |
Spock Framework | Groovy DSL, built-in mocking, data-driven tests, structured phases | Concise, highly readable tests that serve as documentation | Natural language style and expressive specification | Developers open to using Groovy in Java environments |
Selenium WebDriver | Cross-browser automation, user interactions, wait mechanisms | Robust ecosystem, strong community support | Industry standard for UI testing with CI/CD integration | QA engineers and web testers |
AssertJ | Fluent assertions, rich error messages, soft assertions | High readability and excellent IDE auto-completion | Enhances clarity and debuggability in test assertions | Java teams refining existing testing frameworks |
REST Assured | DSL for HTTP requests, JSON/XML validation, authentication | Expressive syntax with extensive examples | Simplifies API testing with reusable specifications | Developers focused on REST API validation |
Equipping Your Java Arsenal: The Power of Effective Testing
Choosing the right Java testing framework is crucial for building high-quality, reliable applications. Whether your priority is simplicity (like JUnit 5), flexibility (like TestNG), mocking (like Mockito), behavior-driven development (BDD) (like Spock), UI testing (like Selenium), fluent assertions (like AssertJ), or API testing (like REST Assured), the options available offer a robust toolkit. These tools ensure your Java code can withstand real-world use.
When selecting a framework, consider your project's specific needs. For unit testing, JUnit 5 is often the default choice. TestNG excels in more complex integration testing scenarios. If mocking is essential, Mockito is indispensable.
For BDD, Spock provides a readable and expressive syntax. Selenium is the industry standard for UI testing, while AssertJ enhances the readability of your assertions. REST Assured simplifies API testing.
Getting started with these frameworks is usually straightforward. Ample documentation and community support are readily available. Most integrate seamlessly with popular build tools like Maven and Gradle, and IDEs provide extensive support for running and debugging tests.
Budget is rarely a concern, as these frameworks are open-source. However, consider the resources needed for team training and implementing best practices. Your chosen framework should integrate with your existing CI/CD pipeline. Compatibility with other tools in your development environment is also key.
Key Takeaways
- Choose the Right Tool for the Job: Select the framework that best meets your specific testing requirements. Don't over-engineer your testing process.
- Prioritize Testability: Design your code with testing in mind from the outset. This will save time and effort in the long run.
- Embrace Automation: Integrate your tests into your CI/CD pipeline for continuous feedback and faster development cycles.
- Invest in Training: A well-trained team can leverage the full potential of your chosen framework.
By choosing the right framework, you can create software that is not only functional but also resilient and maintainable. Managing complex CI/CD pipelines and ensuring smooth code integration can still be a challenge, even with a robust testing framework. Mergify can streamline your workflow and boost your development process. Reduce CI costs, improve code security, and minimize developer frustration with automated merge queues and intelligent merge strategies. Explore how Mergify can transform your development workflow.