How to Use pytest: A Quick Guide to Python Testing
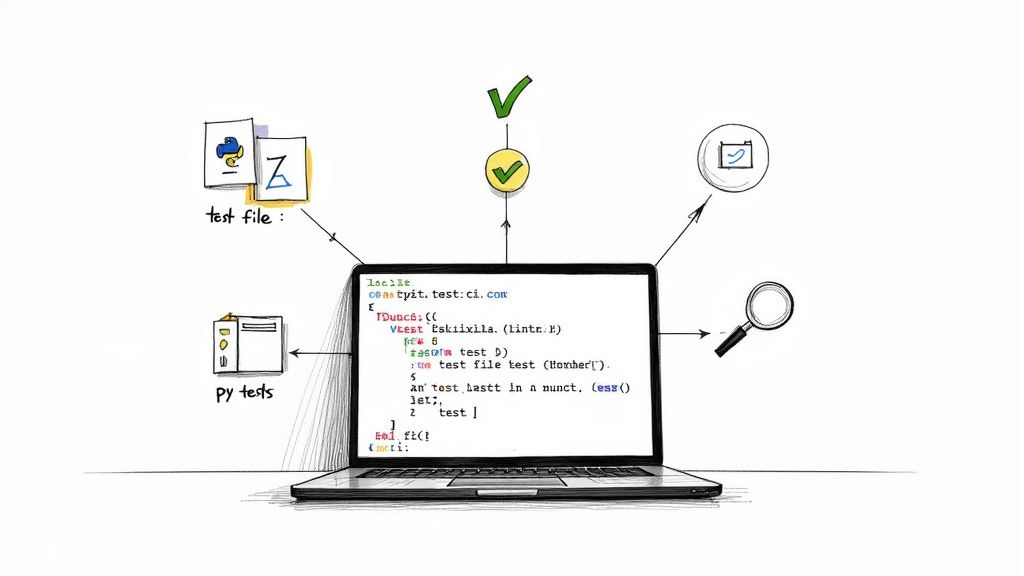
Why Pytest Is Transforming Python Testing
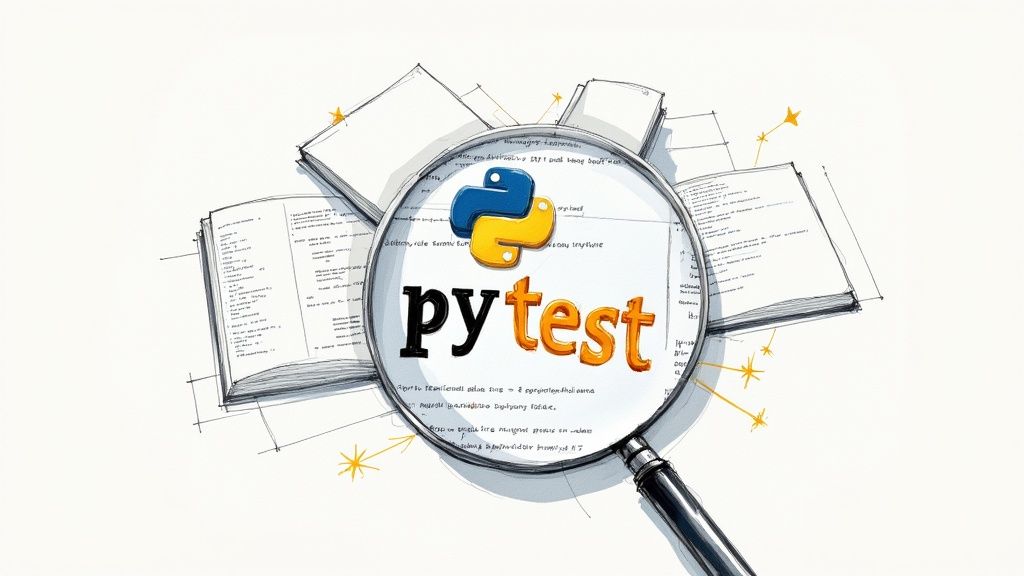
Pytest has rapidly become the go-to testing framework for many Python developers. This isn't a passing trend; it's a fundamental shift. Pytest simplifies what used to be a complicated and often tedious process. Unlike older frameworks like unittest
and nose
, pytest requires far less boilerplate code.
This allows developers to concentrate on crafting effective tests instead of getting bogged down in complex setup procedures. This simplified approach makes testing more approachable for beginners while also increasing productivity for experienced developers.
Pytest’s Simple Yet Powerful Design
One of pytest’s greatest strengths lies in its intuitive design. Tests are written as straightforward functions, making them easy to read and comprehend. This clean structure significantly improves maintainability and team collaboration.
For instance, assert statements are written naturally using Python's built-in assert
keyword. This avoids the need for specialized assertion methods. This direct approach ensures that tests accurately reflect the intended code behavior. Plus, pytest's automatic test discovery eliminates manual test registration, saving developers time and effort. This automation streamlines the process of running tests, creating a more efficient workflow.
The Fixture Advantage and Plugin Ecosystem
Pytest's fixture system offers a robust mechanism for handling test dependencies and setups. Fixtures enable developers to create reusable components. These components can be used for setting up test environments, accessing resources, or generating test data.
This eliminates code duplication and makes managing complex test scenarios easier. A fixture might, for example, set up a database connection or create a mock API client. This guarantees that tests are run in a consistent and controlled setting. This level of control makes pytest suitable for projects of any scale. Furthermore, pytest's widespread adoption is impressive. The framework is used in over 81 countries across diverse industries. This global usage demonstrates its versatility and effectiveness in maintaining software quality. Learn more about Pytest here.
A Thriving Community and Continued Growth
The extensive plugin ecosystem around pytest is another factor driving its popularity. Supported by a large and active community, pytest offers a wide array of plugins. These plugins extend pytest's functionality in many ways.
This empowers developers to customize their testing environment to meet specific project needs. This vibrant community fuels continuous improvement and innovation within the pytest ecosystem. It solidifies pytest’s position as a top testing framework within the Python community. This strong community and ongoing development are essential for the framework’s long-term viability.
From Zero to Testing Hero: Setting Up Your First Pytest
Starting with pytest is surprisingly simple. Let's walk through the installation process and create your very first test. You might be interested in: How to master Pytest setup.
Installation and Test Discovery
Pytest is easily installed using pip
, the standard package installer for Python. Simply open your terminal or command prompt and run pip install pytest
.
This command installs pytest and its core dependencies, making it ready to use in your projects. Pytest's intelligent test discovery automatically finds tests without complicated configurations. It searches for files named test_*.py
or *_test.py
and runs functions prefixed with test_
. This convention keeps your tests organized and readily identifiable.
Writing Your First Test
Creating a test with pytest is straightforward. Let's create a simple function to add two numbers:
def add(x, y): return x + y
Now, let's write a pytest function to test this add
function:
def test_add(): assert add(2, 3) == 5
This simple test verifies that add(2, 3)
returns the expected value, 5. When diving into setting up pytest, understanding basic testing principles is key. The Bug Testing Template provides a helpful resource for structuring your testing process.
Running Pytest
To run your test, simply navigate to the directory containing your test file in the terminal and execute pytest
. Pytest will discover and run all the test functions, providing a report of the results. The following infographic visualizes this three-step process of creating a test file, writing an assertion, and running pytest.
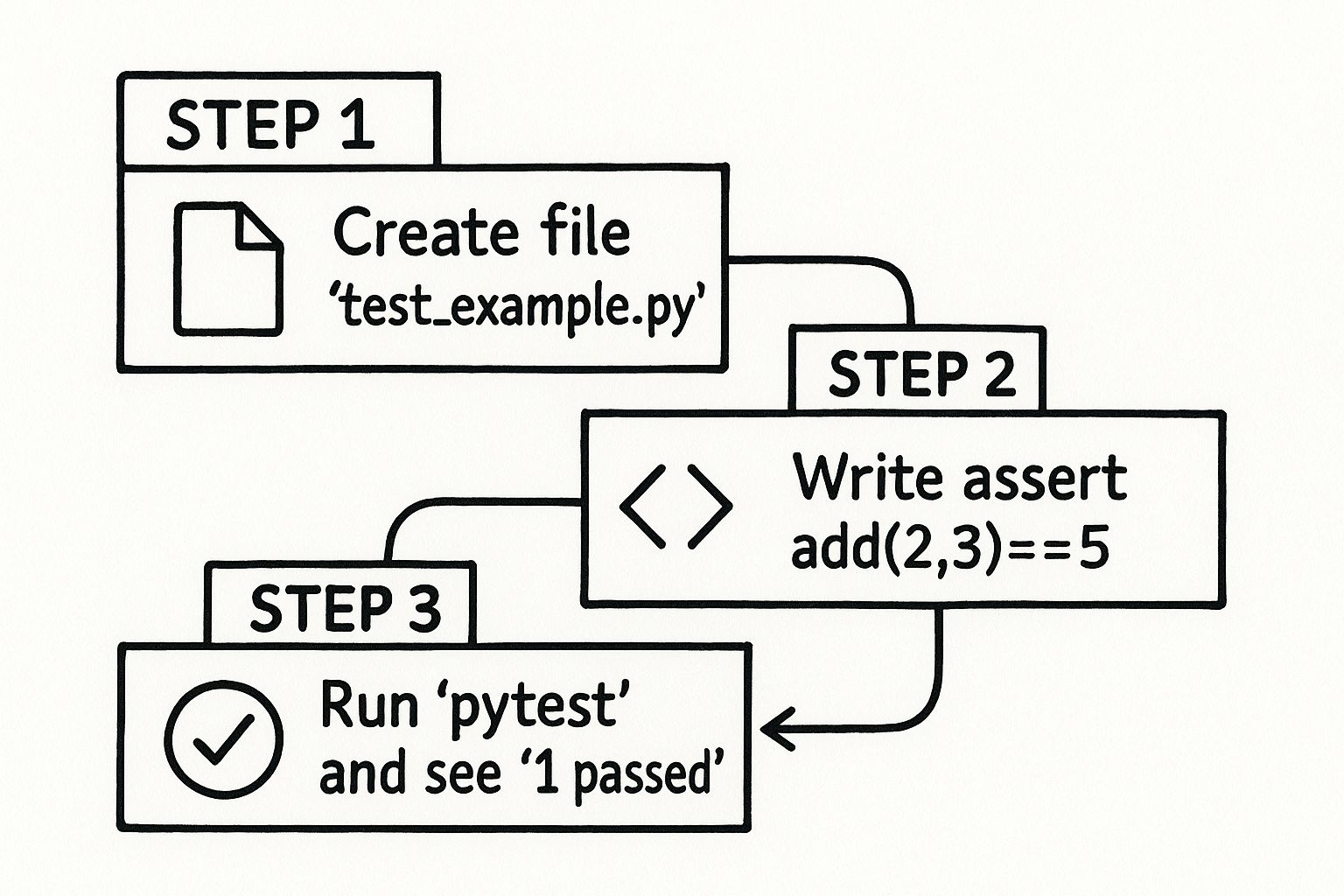
As shown in the infographic, if the assertion passes, pytest outputs a "1 passed" message, indicating a successful test. This simple workflow enables you to quickly write and run tests, ensuring the correctness of your code from the start.
Testing Different Python Constructs
Pytest is highly versatile and can test various Python constructs. This includes testing functions, classes, and even exception handling. Let's consider a function that raises an exception:
def raise_exception(): raise ValueError("Invalid input")
You can test this function using pytest.raises
as follows:
def test_exception(): with pytest.raises(ValueError) as excinfo: raise_exception() assert str(excinfo.value) == "Invalid input"
This ensures that your error handling logic works correctly, preventing unexpected behavior.
To help illustrate pytest's advantages, let's look at a comparison table:
The following table compares pytest with other popular Python testing frameworks:
"Pytest vs. Other Python Testing Frameworks" "Comparison of pytest features against other popular Python testing frameworks"
Feature | pytest | unittest | nose | doctest |
---|---|---|---|---|
Test Discovery | Automatic | Explicit | Automatic | Embedded in Docstrings |
Fixtures | Yes | setUp/tearDown | Limited | No |
Assertions | assert statement |
Specific assertion methods | assert statement |
assert statement |
Plugins | Rich ecosystem | Fewer plugins | Moderate number | Limited |
Key takeaways from the table: pytest shines with its automatic test discovery and the powerful fixture system. While unittest
requires explicit test definitions, pytest simplifies this process. The use of the standard assert
statement further streamlines test writing in pytest and nose
. Finally, pytest's extensive plugin ecosystem provides unmatched flexibility. This introductory look at how to use pytest showcases its straightforward nature, paving the way for you to write more comprehensive and efficient tests as you dive deeper into Python testing.
Harnessing the Power of Pytest Fixtures
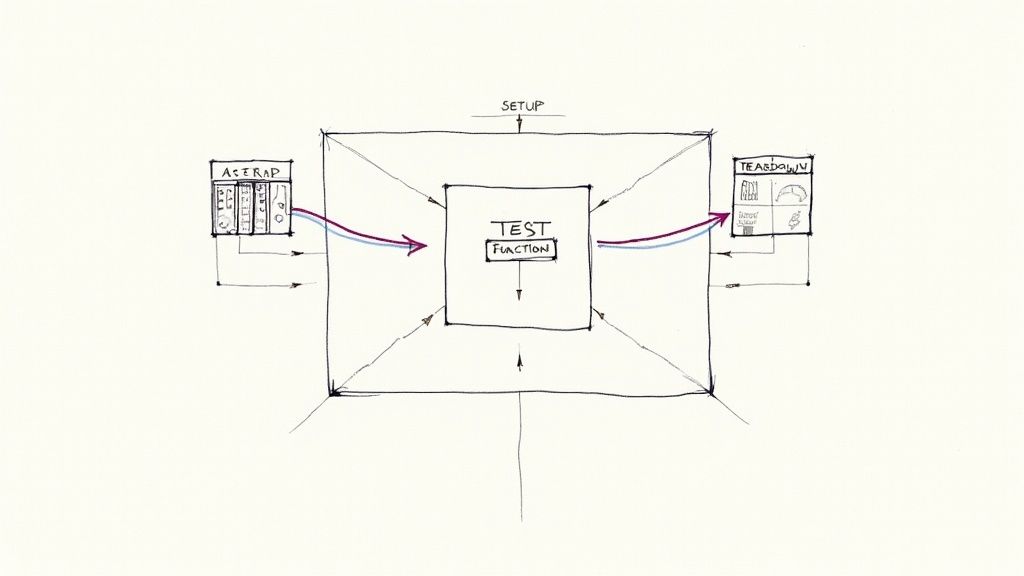
Pytest fixtures are essential for writing efficient and maintainable tests. They provide a robust mechanism for managing test dependencies, setting up preconditions, and sharing resources across your tests. This helps keep your test code clean and organized. For a deeper dive, check out this helpful guide on how to master pytest fixtures.
Understanding Fixture Scopes
Scopes in pytest fixtures define how often a fixture is executed, giving you fine-grained control over test setup. Choosing the right scope optimizes performance and ensures you're not wasting resources.
- Function Scope: This is the default scope. The fixture runs for each test function, providing excellent isolation.
- Class Scope: The fixture is executed once for every test class. This is perfect for resources shared by all methods within a class.
- Module Scope: If you have expensive operations or resources used across a whole module, this scope is your best bet. It runs the fixture once per module.
- Session Scope: This runs the fixture only once for the entire test session, making it ideal for setting up resources like database connections that are needed across multiple modules.
Creating Fixtures for Complex Dependencies
Fixtures truly shine when managing intricate dependencies. Consider testing database interactions. A fixture can streamline the database connection setup and teardown, ensuring a clean slate for each test.
import pytest
@pytest.fixture(scope="module") def db_connection(): # Setup database connection connection = connect_to_database() yield connection # Provide the connection to tests # Teardown database connection connection.close()
def test_database_query(db_connection): # Use db_connection to execute queries result = db_connection.execute("SELECT * FROM users") # ... assert on result ...
This example isolates each test with a fresh database instance. Fixtures can handle various dependencies like API clients, test data generation, and more. Centralizing these setup steps reduces code duplication and ensures consistency across your tests. It’s worth noting that Python's growing popularity fuels the expansion of the pytest framework. The global Python market is projected to reach an estimated $100.6 million by 2030, a significant jump from $3.6 million in 2021. Learn more about the growing Python market here.
Advanced Fixture Techniques
Pytest fixtures offer even more powerful features:
- Fixture Parametrization: Run the same test logic with different input values, maximizing test coverage without writing repetitive code. This creates variations of the same fixture, each with different parameters.
- Factory Fixtures: Generate dynamic test data or environments for each test, guaranteeing isolation and allowing for flexible test scenarios.
- Fixture Composition: Combine simpler fixtures to build complex ones. This promotes reusability and modularity in your test setup. You can create a hierarchy of fixtures, leveraging pytest's dependency injection.
These advanced techniques enable you to create robust and scalable test suites in pytest, effectively handling even the most demanding testing scenarios. They empower you to build more comprehensive and efficient tests.
Beyond Basic Testing: Advanced Pytest Techniques
Building upon the fundamentals of Pytest, let's explore advanced techniques that empower experienced developers to create robust and efficient test suites. These techniques will elevate your testing from simple verification to a comprehensive quality assurance process.
Mastering Pytest's Built-in Assertions
Pytest’s assertion system is remarkably powerful, providing detailed error messages without requiring special syntax. This simplicity allows you to focus on the logic of your tests, rather than wrestling with complex assertion methods. You can easily compare complex objects, floating-point values, and even handle expected exceptions with precision.
For example, when comparing floating-point numbers, you can use pytest.approx
to account for potential rounding errors:
def test_floating_point_equality(): assert 0.1 + 0.2 == pytest.approx(0.3)
This ensures that minor discrepancies due to floating-point representation don’t lead to false test failures. Additionally, using pytest.raises
lets you verify that your code correctly handles expected exceptions:
def test_expected_exception(): with pytest.raises(ValueError) as excinfo: # Code that raises ValueError raise ValueError("Specific error message") assert str(excinfo.value) == "Specific error message"
This confirms that the exception is not only raised but also carries the correct message.
Organizing Growing Test Suites
As your projects grow, maintaining a well-structured test suite is crucial. Pytest provides several ways to organize your tests for improved scalability and maintainability. You can group tests into classes, modules, and packages.
- Classes: Grouping related tests within classes helps improve code organization. This can be particularly useful when sharing setup logic among multiple tests using class-level fixtures.
- Modules: Modules provide a broader organizational structure, separating tests for different parts of your application.
- Packages: For very large projects, using packages to organize your test code becomes even more important. This allows you to break down your tests into smaller, more manageable units.
This structured approach makes it easier to locate and manage tests as your project evolves.
Test Selection and Filtering
Pytest offers powerful features for selecting and filtering the tests you want to run, saving valuable development time. This becomes particularly beneficial when dealing with large test suites where running every test during development can be slow and inefficient. You can select tests based on names, markers, or file paths.
- Name-based selection: Run tests whose names match a specific pattern. For example, use
pytest -k "test_api"
to run tests containing "test_api" in their name. - Marker-based selection: Apply markers to categorize tests and then run only the tests with specific markers. As an example,
pytest -m "integration"
would run tests marked “integration”. - Path-based selection: Specify the files or directories containing the tests you want to run.
pytest tests/api
would run tests within thetests/api
directory.
This precise control over test execution helps improve development efficiency.
Parametrized Testing
Parametrized testing in pytest increases code coverage without repeating test logic. This is particularly useful when testing functions or methods that need to work with various inputs. By using the @pytest.mark.parametrize
decorator, you can specify multiple inputs and expected outputs for a single test function.
The following table demonstrates essential command line options for controlling test execution and output in Pytest.
Pytest Command Line Options
Option | Description | Example Usage | Common Use Case |
---|---|---|---|
-k EXPRESSION |
Selects tests based on name, using a substring expression | pytest -k "test_api" |
Run only API-related tests |
-m MARKEXPR |
Selects tests based on markers | pytest -m "integration" |
Run only integration tests |
tests/PATH |
Specify a file or directory to run tests from | pytest tests/api |
Run tests within a specific directory |
-v |
Enables verbose output | pytest -v |
Get detailed information on each test being run |
-s |
Disable output capturing. Shows print statements | pytest -s |
Useful for debugging |
This table summarizes some of the frequently used Pytest command-line options that contribute to efficient test selection and filtering.
For example:
@pytest.mark.parametrize("input, expected", [(1, 2), (2, 3), (3, 4)]) def test_increment(input, expected): assert input + 1 == expected # Simulate increment function for demonstration
This single test function will run three times, once for each input-output pair, checking each scenario without code duplication. This technique reduces code and makes it easier to add new test cases. By combining these advanced techniques, you can build robust test suites in pytest that scale with your project and improve development efficiency. These advanced techniques enhance your software quality assurance process.
Supercharging Your Testing With Pytest Plugins
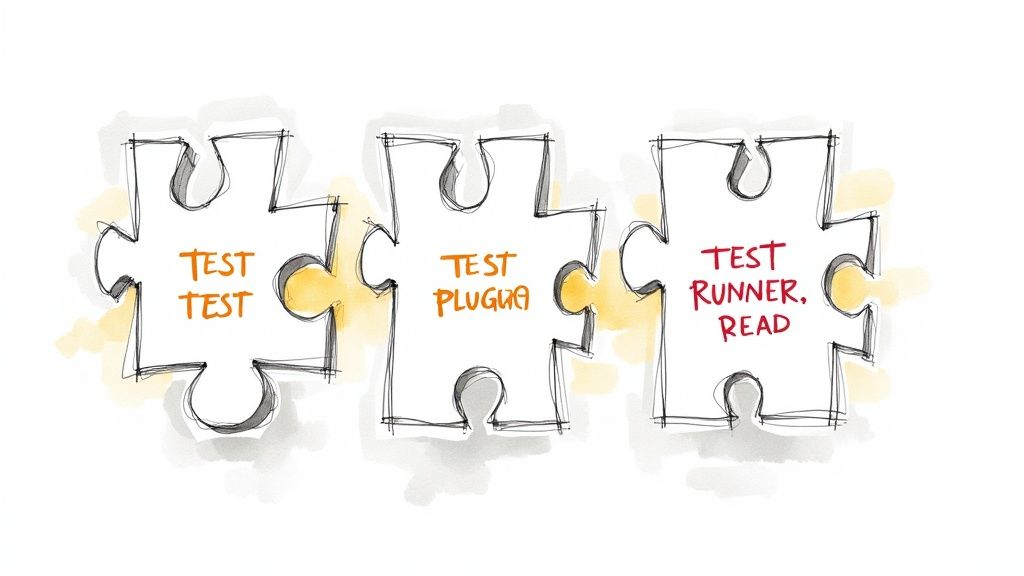
Pytest's real strength lies in its extensibility. A diverse range of plugins enhances pytest, streamlining common testing tasks. This lets you tailor your testing environment to your project's specific needs.
Essential Pytest Plugins
Several key plugins significantly improve the testing process:
- pytest-cov: This plugin provides detailed code coverage reports, highlighting untested code. This helps identify gaps in your test suite and improve overall coverage, ensuring a reliable codebase.
- pytest-mock: Pytest-mock simplifies the creation of test doubles like mocks and stubs. It isolates code units during testing, facilitating tests of complex interactions.
- pytest-xdist: For larger projects, pytest-xdist enables parallel test execution across multiple CPUs. This significantly reduces testing time and accelerates the development workflow.
- pytest-bdd: If you're using Behavior-Driven Development (BDD), pytest-bdd is a must-have. It uses a Gherkin-like syntax to define tests, bridging the gap between technical and non-technical stakeholders.
These are just a few examples; numerous other plugins address various testing needs, from performance evaluation to database integration.
Implementing Plugins: Real-World Benefits
Using these plugins is simple. After installation (pip install pytest-cov pytest-mock pytest-xdist pytest-bdd
), they integrate seamlessly with pytest. Running pytest --cov=my_package
generates a coverage report for my_package
. Pytest-mock requires minimal setup within test functions, making it easy to mock dependencies.
The demand for efficient software testing is rising, driving the adoption of tools like pytest. The global software testing services market is projected to grow by $90 billion between 2021 and 2030, with a CAGR of 12.39%. This growth highlights the increasing need for robust testing tools. Find more statistics here.
Discovering and Configuring Pytest Plugins
Finding suitable plugins is easy, thanks to the extensive pytest documentation and online communities. Search by keyword or functionality to find plugins that match your project’s specific requirements. Each plugin has its own documentation, which offers clear guidance on configuration and usage, often with practical examples.
Configuring plugins typically involves command-line options or configuration files, offering flexibility and seamless integration into your testing process. This allows for fine-tuning of your testing environment. By exploring the rich pytest plugin ecosystem, you can significantly improve your testing workflow and enhance the quality of your Python projects.
Creating a Sustainable Testing Culture With Pytest
Building a successful testing culture with Pytest involves more than just understanding the framework. It requires establishing practices and habits that encourage your team to consistently write high-quality, maintainable tests. This section explores strategies for creating a thriving testing environment that evolves alongside your codebase. Check out our guide on how to master Python testing with pytest.
Organizing Test Code for Clarity
New team members should easily grasp and contribute to your test suite. Intuitive naming conventions and folder structures are crucial for this. Consistent naming makes it simple to understand the purpose of each test. A well-defined folder structure mirroring your application’s architecture provides a clear connection between tests and the code they verify.
For instance, if your application has a users
module, a corresponding tests/users
directory containing related tests makes sense. Within this directory, you could organize tests by feature, such as test_user_registration.py
or test_user_authentication.py
.
Maintaining Test Isolation and Preventing Flaky Tests
Test isolation ensures each test runs independently, preventing unintended interactions between them. This avoids flaky tests, which pass or fail intermittently without code changes, reducing confidence in your suite.
Use fixtures to set up and tear down resources like database connections or mock objects for each test. This gives each test a clean slate.
Managing Test Dependencies and Handling Slow Tests
As projects grow, test dependencies become more complex. Well-structured fixtures are vital for managing them. Different fixture scopes (function, class, module, session) efficiently manage resources and optimize test execution.
Identify and isolate slow tests. Run these less often or optimize them to improve overall testing speed.
For projects involving other tools and systems, effective access management is important. For information about how Pytest integrates with other tools like Jira, check out resources like this one about Jira Access Management.
Balancing Test Coverage and Development Velocity
Aim for high test coverage, but 100% coverage isn't always necessary. Focus on critical paths and core functionality. Prioritize tests offering the most value in risk mitigation and confidence. This ensures thorough testing without hindering development.
Regularly review your test suite for redundant or outdated tests to maintain focus and efficiency.
Specialized Testing Approaches
Different applications need different testing strategies:
- Web Applications: Emphasize end-to-end, UI, and integration testing across browsers and devices.
- APIs: Prioritize testing endpoints, request/response structures, and error handling.
- Data Processing Pipelines: Test data transformations, integrity, and performance at each stage.
- Microservices: Use integration testing and contract testing to ensure proper service interaction.
Tailoring your approach creates a more targeted testing strategy.
By following these practices, you'll build a strong testing foundation that supports your evolving codebase. This fosters a culture of quality, benefiting the entire development process.
Ready to improve your CI/CD pipeline? Learn how Mergify can help automate merge processes, reduce CI costs, and boost code quality.