How to Start Using Python Typing?
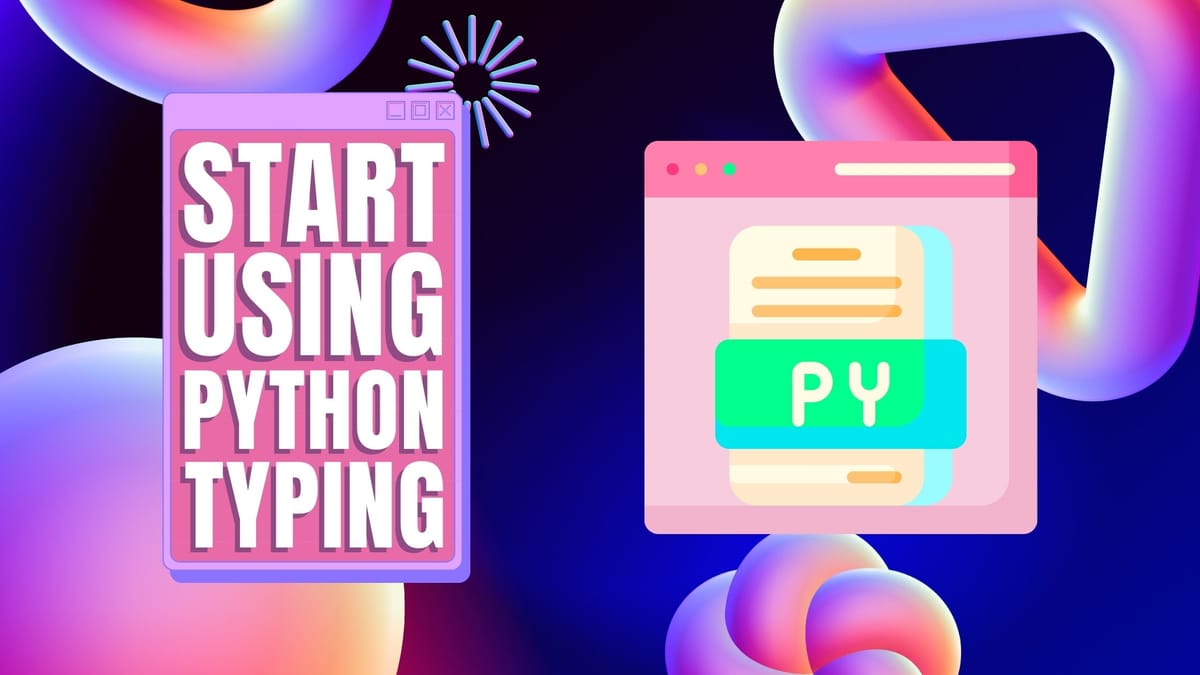
Most programming languages are either statically or dynamically typed.
With static typing, developers need to define the type of data used, like in the example below in the C language:
int example_function(int number, char *string)
From the very first look at this function, you know that it takes an int
and a char *
as parameters, and you know it will return an int
. This makes it significant for humans and computers to understand what's expected.
In that regard, static typing makes the code safer. A compiler can detect inconsistencies with types and warns (or errors out) if you try to pass a variable with the wrong type as a parameter.
On the other hand, defining every type of variable, argument, and return type of every function can be pretty tedious. You have to be 100% sure of every variable type, even when trying to do "debugs-prints."
This is one of the strengths of dynamic programming languages such as Python. If you want to see what's inside a variable, you can just run print(variable)
without specifying if it's a string, a number, or a piece of cheese.
➡️ This makes your code more concise and faster to write.
With the introduction of type hints, Python allows you to choose whether you want your code to be typed. You don't have to specify the types by default, but a typed code is more robust and easier to debug.
For example, your code could crash because of a variable not storing data of the type you're expecting, and you would have to know the type of every variable to find out which one is not doing as expected.
You can also choose to have untyped variables in a typed code. This is only useful in a few cases, but you can still do that if needed.
How to start using typing? 🏁
In Python, all you have to do to declare the type of a variable is to add :
followed by the type of the variable.
mynumber: int
This is especially useful in functions where you want to know what types your variables are. You need to know exactly what goes inside and out of your function.
This way, you can use functions without having to read their code to understand what variables you have to pass as parameters for them to work.
def example_function(number: int, string: str) -> int
This function takes exactly the same kind of parameters and has the same return type as the C function above: it takes an int
and a str
and returns an int
.
You can also create new types with the NewType
helper to fit your needs.
The typing makes your function far less ambiguous for humans on default Python (for example, if you had a code
variable, maybe you wouldn't know immediately if it was a string or a number).
In other languages like C, if you try to pass a char *
in a function expecting an int
, you'll get an error, and you won't be able to compile your code at all.
This is not the case in default Python, but you can use tools such as mypy to do the same check as a C compiler. More than that, you can specify which modules or expressions should be checked by mypy: you can mix static and dynamic typing.
You also have the option to use Any
as a type, which will be considered as a type compatible with every other type, meaning you can assign Any
kind of value to this type.
Why typing? 🧐
Depending on your use case, typing Python can be very useful — or annoying.
Using Python typing is a good idea when you're working on a large-scale project to ensure that your code doesn't have inconsistencies and make it more explicit for other developers.
On the other hand, it does take more time to type everything, which could not be worth it on smaller projects or also when you find yourself constantly using Any
or mixing non-typed Python (e.g., when you're manipulating a lot of data with different types and you want to process this data with the same few functions).
Remember: sometimes it's better to use the simplest and most boring solution for a problem, and remember that “boring” should not be conflated with “bad” or “technical debt.” Don't use Python typing just to use it; adapt it to your use case.