How to Run Go Tests: Expert Tips & Tricks
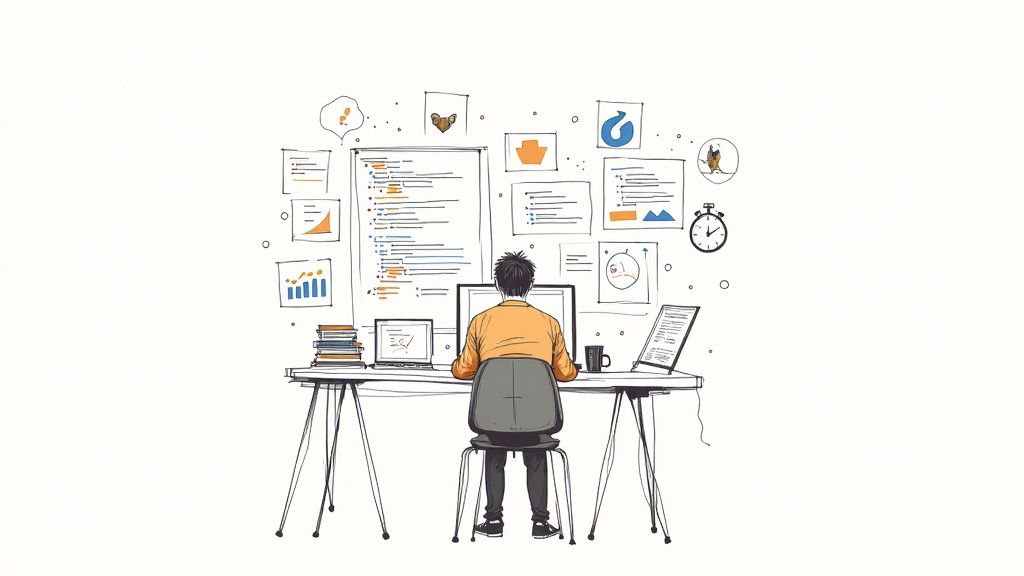
Getting Started with Go Testing Fundamentals
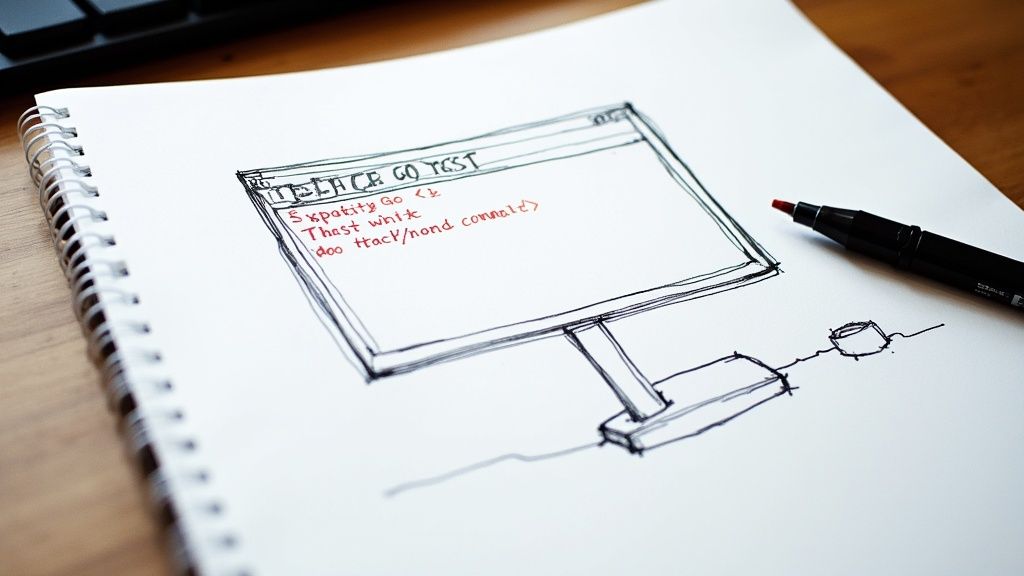
Testing is a crucial skill for writing reliable Go applications. This section covers the essential concepts and practices you need to write effective tests in Go. We'll explore the core testing tools, common patterns, and practical approaches that professional Go developers use.
The Core of Go Testing: The testing
Package
Go's standard library includes the testing
package, which provides everything needed to write and run tests. When writing tests, you'll create files that end in _test.go
. These special files contain test functions that the go test
command will automatically detect and run.
Here's what a basic test function looks like:
import "testing"
func TestMyFunction(t *testing.T) { // Test logic here }
The testing
package also includes TestMain
- a special function for handling setup and cleanup tasks. This is particularly useful when your tests need to create resources like database connections or test files that should be properly managed between test runs.
Essential Testing Concepts: Assertions and Naming Conventions
Writing clear test assertions helps verify your code works correctly. While Go's testing
package includes basic tools like t.Errorf
, many teams use additional testing libraries to make assertions more readable and powerful.
The Go ecosystem offers several excellent testing frameworks that extend the standard library's capabilities. Popular options like Testify and Ginkgo provide features for:
- Easy-to-read assertions
- Test grouping and organization
- Mock object creation
- Custom test runners
Good test names make it easy to understand what's being tested. Name your test functions starting with "Test" followed by a clear description of the functionality being verified. This helps other developers quickly find and run specific tests, which becomes increasingly important as your test suite grows.
Mastering Different Types of Go Tests
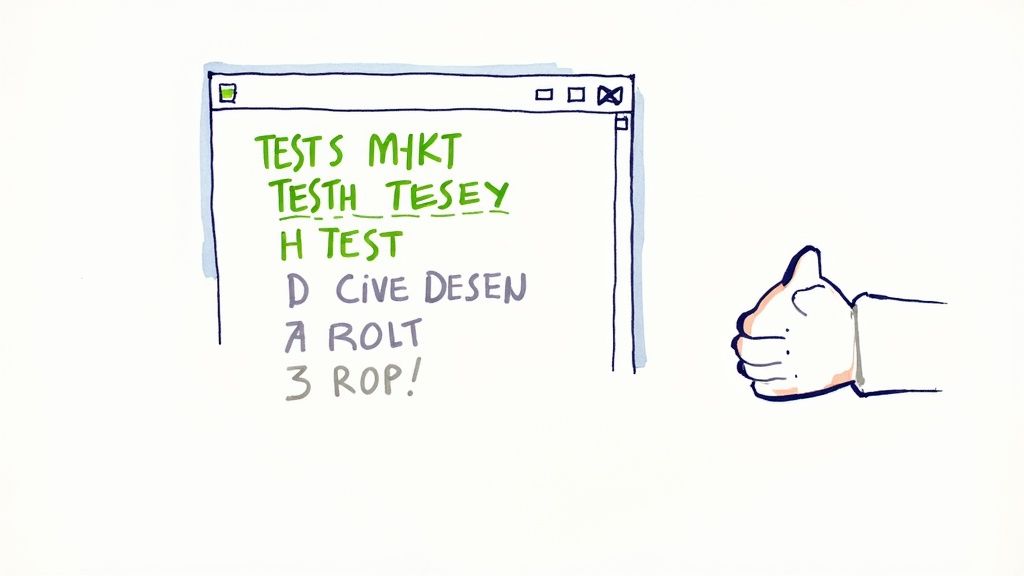
Writing effective tests is crucial for building reliable Go applications. Let's explore the three main types of tests in Go: unit tests, integration tests, and benchmarks. Each type serves a specific purpose and helps ensure your code works as intended.
Unit Tests: The Foundation of Go Testing
Unit tests check individual pieces of code in isolation, like specific functions or methods. Think of them as examining each brick before building a wall - you want to make sure each piece is solid on its own. A good example is testing a function that calculates a circle's area: you verify it returns the right value for a given radius. Keeping unit tests focused makes them easier to maintain and debug.
Integration Tests: Connecting the Dots
Integration tests look at how different parts of your code work together. While unit tests check individual components, integration tests verify the connections between them. For example, you might test if your database layer properly communicates with your business logic. These tests catch issues that unit tests might miss, like data handling problems between modules.
Benchmarks: Measuring Performance
Benchmarks help you understand how fast your code runs. They measure execution time for specific functions, helping you spot slow parts of your application that need improvement. This becomes especially important as your application grows and handles more users or data. The Go testing package includes built-in support for benchmarks - you can run them using the go test -bench
command to see detailed performance metrics. Learn more about Go testing.
Combining Test Types for Comprehensive Coverage
The most effective testing strategy uses all three types together. Start with unit tests as your foundation, add integration tests to verify components work together, and use benchmarks to keep your code fast. By understanding when to use each type, you'll catch more bugs early and keep your application running smoothly in production.
Optimizing Your Test Coverage Strategy
Understanding different types of Go tests is just the first step. The real challenge lies in creating a smart testing strategy that protects your code where it matters most. Instead of chasing high percentages blindly, focus on testing the parts of your code that are most likely to cause problems.
Identifying Coverage Gaps
Go's built-in coverage tools show you exactly which lines of code your tests run and which ones they miss. This helps you spot areas that need more testing attention. Look closely at complex logic flows and error handling - these are often where bugs hide. Pay special attention to code that handles core business tasks or features that users rely on heavily.
Targeted Improvements and Data-Driven Decisions
After finding gaps in your test coverage, it's time for smart improvements. Research shows that good test coverage in Go typically falls between 61% and 80%. While 100% coverage sounds nice, it's rarely worth the effort. Instead, look at the data to decide what to test next. Start with code that could cause the biggest problems if it breaks. Learn more about Go testing practices.
Balancing Comprehensive Testing and Pragmatic Goals
Good testing strikes a balance. Writing and maintaining tests takes time and effort. Too many tests can slow down development without adding much safety. Focus on writing tests that give you the most protection for your effort. This usually means thoroughly testing your core features while accepting some risk in less critical areas.
Prioritizing Tests for Maximum Value
Here's how to think about different levels of test coverage for your code:
Coverage Level | Benefits | Drawbacks | Recommended Use Cases |
---|---|---|---|
High (80%+) | Increased confidence in critical functionality | Higher maintenance overhead | Core business logic, frequently used features, complex algorithms |
Medium (60-80%) | Good balance between coverage and effort | Some risk remains | Standard application logic, less critical features |
Low (Below 60%) | Minimal effort | Higher risk of undetected bugs | Experimental features, rarely used code paths |
By focusing your testing efforts where they matter most, you can build reliable Go applications without wasting time on low-value tests. This approach helps you deliver quality code while keeping development moving at a good pace.
Accelerating Tests with Parallel Execution
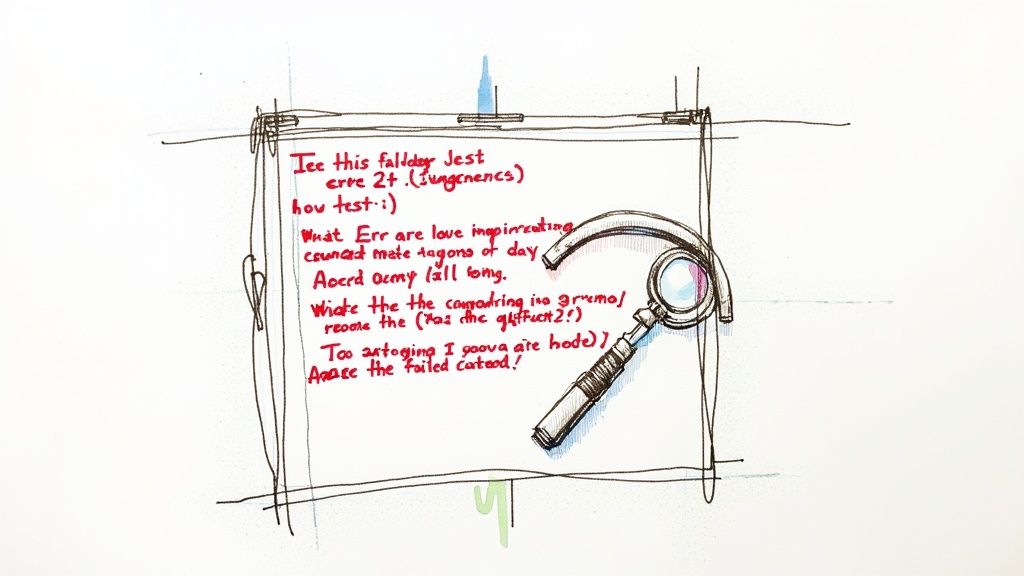
As Go projects expand, test suites naturally grow larger. Running tests one after another can create a major slowdown in development. By running tests in parallel, teams can dramatically cut down testing time and get back to building features. Let's explore how to make the most of parallel testing in Go.
Understanding t.Parallel()
The Go testing
package makes it easy to run tests in parallel using the t.Parallel()
method. By adding this one line to your test function, you tell Go that this test can run at the same time as others. This small change often leads to much faster test runs, especially for larger test suites.
The Power of GOMAXPROCS
The GOMAXPROCS
environment variable controls how many tests can run at once. By default, it matches your computer's CPU core count. This means on a 4-core machine, 4 tests can run simultaneously.
This multi-core approach can seriously speed up testing. For example, a test suite that takes 10 minutes to run normally could finish in about 2.5 minutes on a 4-core machine - a 75% reduction in testing time.
The Go testing framework runs tests one at a time by default. But with t.Parallel()
, tests can run together, often saving significant time. The number of concurrent tests is limited by GOMAXPROCS
, which typically matches your available CPU cores. Learn more about Go testing.
Writing Concurrent-Safe Tests
While parallel testing offers great speed benefits, it requires careful test design. Tests running at the same time must not interfere with each other. Without proper handling, shared resources like global variables can cause tests to fail unpredictably.
Best Practices for Test Isolation
- Keep Tests Independent: Avoid using global variables or shared state between tests. If you must share resources, use mutexes to prevent race conditions.
- Handle External Systems: Keep tests separate from databases and network services. Use mocks to simulate these systems for consistent test results.
- Clean Up After Tests: Always clean up temporary files and connections after tests finish. This gives the next test a fresh start.
Optimizing Parallel Execution
- Choose Tests Carefully: Not every test works well in parallel. Tests that depend on each other or modify shared resources might need to run one at a time. Focus on making independent tests parallel.
- Test and Adjust: Watch how test times change as you add parallel testing. Try different
GOMAXPROCS
values to find what works best for your setup.
Following these guidelines helps you safely speed up your Go tests through parallel execution. The goal is faster testing without sacrificing reliability or accuracy.
Building Maintainable Test Suites
Quality test code needs thoughtful organization to stay manageable as your project grows. Without clear structure and guidelines, tests can become messy and hard to maintain. Let's explore proven approaches that successful Go teams use to build test suites that stand the test of time.
Organizing Test Files
Clear file organization is the foundation of maintainable tests. Follow the standard practice of matching your test file names to source files - for example, pair user.go
with user_test.go
. This makes test locations immediately obvious. Use descriptive test function names like TestCreateUser
or TestGetUserById
to clearly communicate what each test verifies.
Structuring Test Suites
Take advantage of Go's testing
package to create well-structured test suites. Use helper functions to avoid duplicating setup and cleanup code. Group related test cases with t.Run()
subtests to create logical hierarchies. This organization helps developers quickly understand how different test cases fit together.
Implementing Maintenance-Friendly Practices
Focus on writing clear, focused test code that's easy to understand and update. Keep each test case limited to verifying one specific behavior rather than trying to test everything at once. Small, targeted tests are much easier to debug and modify when requirements change. The Go community continues to emphasize the importance of maintainable tests as more developers adopt the language. Check out the latest adoption trends in the Go Developer Survey Results.
Common Test Organization Patterns
Below are proven patterns for organizing Go tests. Choose the approach that best fits your project's needs:
Pattern | Description | Advantages | When to Use |
---|---|---|---|
By Function/Method | Each test file maps to a source file and contains tests for that file's functions | Simple to navigate, clear relationship between tests and code | Standard approach that works well for most projects |
By Feature/Use Case | Tests grouped around user-facing features or workflows | Ensures thorough testing of complete user scenarios | Good for features that span multiple components |
By Test Type | Tests separated by category (unit, integration, benchmark) | Easy to run specific test types in isolation | Best for large test suites with diverse test types |
Pick the pattern that matches your project's size and team workflow. A clean, logical test organization helps ensure your test suite remains a valuable asset as your codebase grows.
Resolving Common Testing Challenges
Writing tests in Go is simple to start with, but can get tricky as your projects grow. Let's look at some frequent testing challenges Go developers encounter and practical ways to solve them.
Debugging Test Failures: A Systematic Approach
When tests fail, stay calm and follow a clear process. The first step is to analyze the error messages from go test
. These messages point to the exact failure location and tell you what went wrong - like incorrect function returns or unexpected crashes.
Go's testing package has built-in tools to help understand failures. Use t.Log()
and t.Errorf()
to print values and custom error messages during test runs. These logs help track how the test runs and find where things break down.
Interpreting Test Output: Beyond Pass and Fail
A simple pass/fail result only tells part of the story. Running go test -v
shows detailed output including which test functions ran and their results, making it easier to spot problematic test cases.
The go test -cover
command reveals test coverage - showing which code lines your tests actually check. This helps find untested code that might have bugs. For instance, if a complex function shows only 50% coverage, you probably need more tests.
Handling Errors in Tests: Expecting the Unexpected
Good tests prepare for errors. Use Go's error handling in your tests to catch problems cleanly. The testing package provides t.Error()
and t.Fatal()
to mark test failures, while defer
statements ensure cleanup happens even if tests crash.
Take a database test as an example. Using defer
to close database connections means resources get freed even when tests fail. This keeps your test environment clean and prevents resource leaks.
Building Resilient Test Suites: Maintaining Order and Isolation
As test suites grow, keeping tests separate and clean becomes vital. Create helper functions for setup and cleanup tasks to ensure consistency between tests.
Keep tests independent by mocking external systems like databases and network services. Using mocks gives you a controlled test environment and prevents flaky results from outside factors. For example, testing with a mock database means you don't need a real database server running. This makes tests faster and more reliable.
These practices help build strong test suites that catch bugs early and give you confidence in your Go code.
Want to improve your code review process and eliminate merge bottlenecks? See how Mergify can help streamline your workflow.