Master Golang Test Command: Code Testing Tips
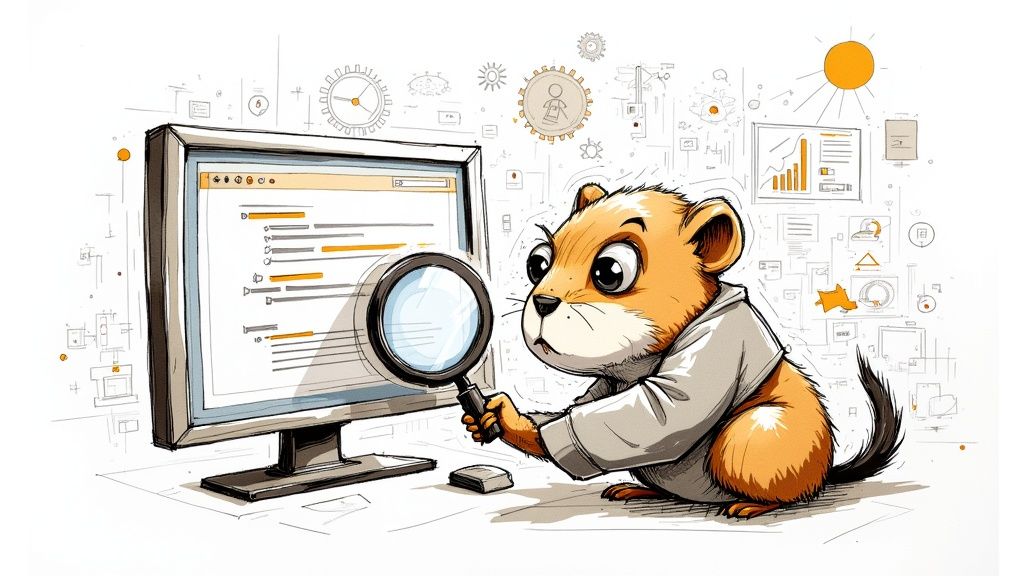
Getting Started With Go Testing Success
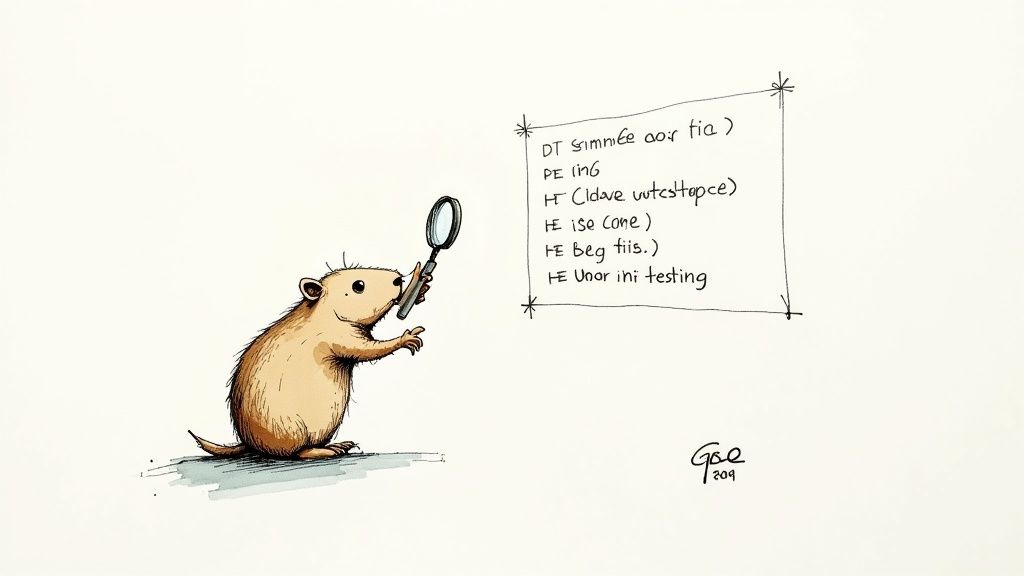
Testing is essential for building stable and reliable Go applications. Go provides a straightforward testing framework through the go test
command that helps catch bugs early. The testing tools are built right into the language, making it natural to add tests as you develop.
Understanding the go test
Command
The go test
command is your main tool for running tests in Go. It automatically finds and executes test files in your project. Here's what you need to know to get started:
- Name test files with
_test.go
suffix - Begin test function names with
Test
(example:TestMyFunction
) - Include
*testing.T
parameter to manage test execution - Tests run in the same package as the code they test
For instance, to test an Add
function, create TestAdd
in add_test.go
. This naming pattern helps Go find and run your tests automatically.
Practical Example
Here's a simple example showing how to write and run tests in Go:
// add.go package main
func Add(x, y int) int { return x + y }
// add_test.go package main
import "testing"
func TestAdd(t *testing.T) { result := Add(2, 3) if result != 5 { t.Errorf("Expected 5, got %d", result) } }
Run go test
in your project directory to execute the tests. The command shows whether tests passed or failed. As of 2022, Go's testing package enables unit testing, benchmarking, and code analysis. Learn more in this Guide to Testing in Go.
Writing Effective Tests
Good tests check both normal cases and edge cases. Consider different inputs and expected outputs to ensure solid test coverage. Table-driven tests help test multiple scenarios cleanly. These practices create reliable tests that catch issues before they reach production.
Unlocking Test Execution Power
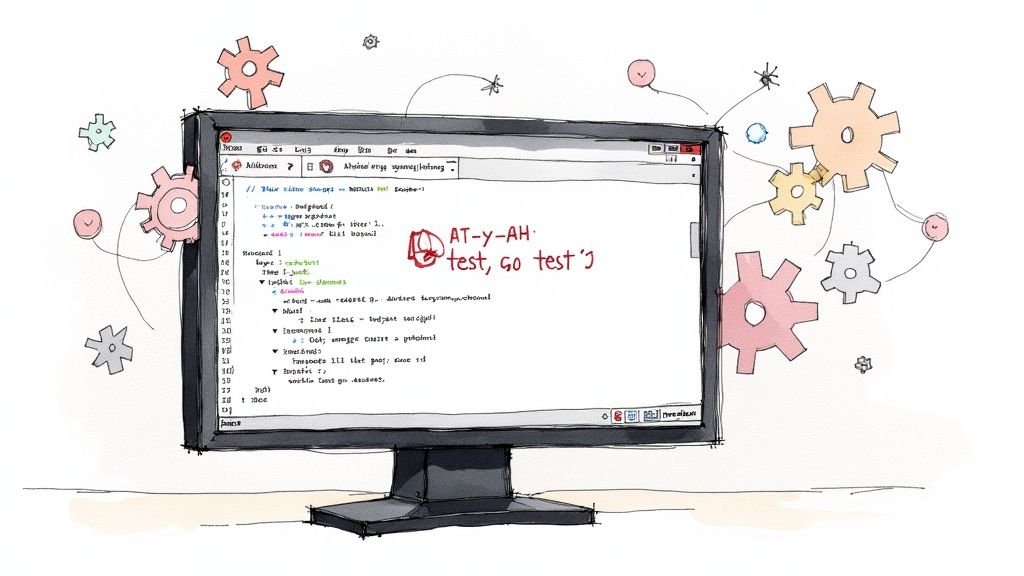
The go test
command is powerful but often underutilized. Getting the most out of testing requires understanding the different execution modes and options available. When used effectively, these features help catch bugs early and speed up the development process.
Understanding Test Modes
Go offers two main ways to run tests - let's look at how they compare:
Feature | Local Directory Mode | Package List Mode |
---|---|---|
Scope | Current directory only | Multiple specified packages |
Caching | No caching | Results are cached |
Best For | Quick development testing | Full project testing |
Speed | Fast for small tests | Efficient for large test suites |
Command | go test |
go test ./... |
The mode you choose depends on your needs. Local directory mode works well for quick checks during development, while package list mode helps test entire projects systematically. For more details on test execution, check out the Go testing documentation.
Leveraging Test Caching
Running tests repeatedly wastes time when code hasn't changed. Go's built-in test caching skips previously passed tests, making subsequent runs much faster. This becomes especially helpful in CI/CD pipelines where quick feedback matters most.
Local vs. Package Testing Strategies
Local testing in a single directory provides rapid feedback when working on specific components. Package testing across multiple packages ensures broader test coverage and catches integration issues. Pick the approach that matches your current task - quick iterations or thorough validation.
Optimizing Test Execution
To speed up your testing workflow:
- Use
-short
to skip slow tests during development - Run tests in parallel with
-p
on multi-core systems - Target specific test functions with
-run
- Filter test output with
-v
for more detail
The key is finding the right balance of speed and thoroughness for your project. Master these options, and you'll build more reliable software while keeping development moving quickly.
Creating Crystal-Clear Test Reports
Getting the most out of the golang test command
requires making sense of the results. A failed test suite can dump a confusing wall of text that's hard to parse. Clear and actionable test reports are essential for quick debugging and maintaining high code quality. Let's explore how to make test output work better for you and your team.
Customizing Output Formats
While the basic golang test command
output gives you core information, you can make it much more useful. The -v
flag shows details for each test run, including test names and results. Want machine-readable data? The -json
flag outputs everything in JSON format. This lets you feed results into dashboards or reporting tools to track testing trends over time.
Implementing Effective Logging
Smart logging in your tests provides crucial context when things go wrong. Adding targeted log statements shows you variable values and execution flow leading up to failures. This gives you much more to work with than just pass/fail results.
The standard log
package works well for basic needs, but specialized logging libraries offer more features. Good logging helps you quickly find where things broke instead of guessing. For example, when testing complex functions, log key values at each step to spot exactly where calculations went wrong.
Organizing and Interpreting Test Results
Large test suites generate lots of output that needs organizing. Group tests logically, tackle failures first, and set clear processes for handling test issues. Tools that gather and display results visually can save hours of log reading. They often spot patterns in failures that point to deeper code problems.
The Go community actively works on making test results more readable. Tools like spectest
and gotest
improve on the basic go test
output by highlighting failures and providing stats. Learn more about better test output formatting.
Tools and Techniques for Easier Debugging
Several tools complement golang test command
to make debugging smoother. The Delve debugger lets you step through code, check variables, and watch how tests run. This gives deeper insights than logs alone can provide. Adding tests to your CI pipeline automates running tests and notifying the team about failures, catching problems early.
By applying these approaches, you can turn golang test command
output from a source of confusion into a powerful debugging aid. Good logging and clear reports help everyone on the team understand and fix issues faster, leading to more stable code.
Advanced Testing Strategies That Work
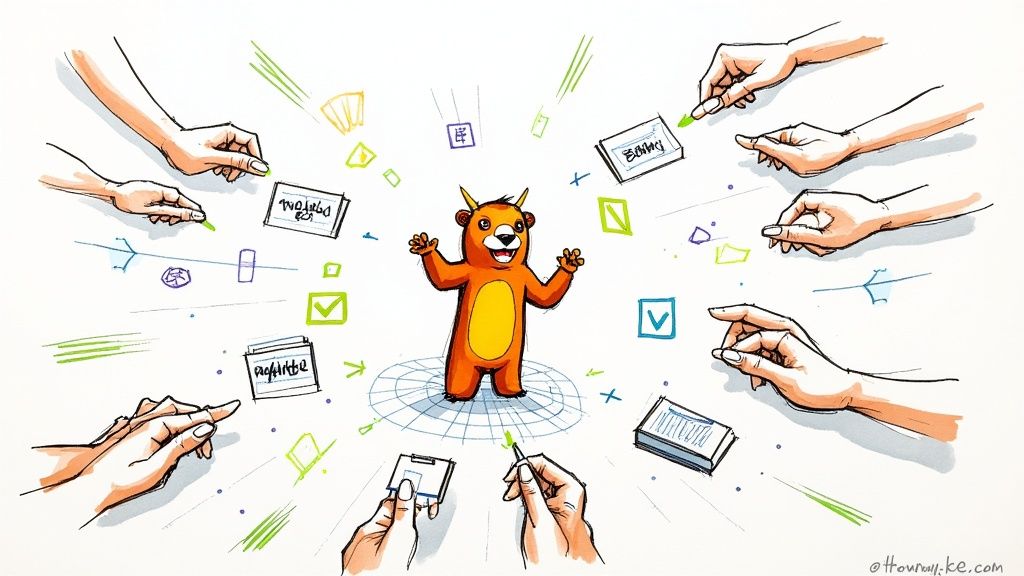
Let's explore proven testing techniques that build on the core golang test command
. These approaches help Go development teams tackle complex scenarios and build reliable, production-quality code. We'll cover strategies that enable you to effectively test challenging use cases as your codebase grows.
Let's start with a comparison of key testing features:
Feature | Use Case | Benefits | Considerations |
---|---|---|---|
Table-Driven Tests | Multiple input/output scenarios | Easy to add test cases, improved readability | Can become complex with many test cases |
Dependency Mocking | External service interactions | Isolates test units, controls behavior | Requires careful mock design |
Race Detection | Concurrent code testing | Catches timing issues early | May slow test execution |
Test Fixtures | Consistent test environment | Reduces test setup duplication | Must be maintained alongside code |
Table-Driven Tests for Comprehensive Coverage
Table-driven tests give you a clear way to verify function behavior across many inputs and expected outputs. This works especially well for functions that need to handle different data formats or edge cases. A good example is email validation - you can easily test various valid and invalid addresses in one organized test.
Here's a practical table-driven test example:
func TestValidateEmail(t *testing.T) { testCases := []struct { input string expected bool }{ {"test@example.com", true}, {"invalid-email", false}, {"another@test.net", true}, }
for _, tc := range testCases {
t.Run(tc.input, func(t *testing.T) {
actual := validateEmail(tc.input)
if actual != tc.expected {
t.Errorf("Input: %s, Expected: %t, Got: %t", tc.input, tc.expected, actual)
}
})
}
}
Mastering Dependency Mocking
As your code grows more complex, managing test dependencies becomes crucial. Mocking lets you test components in isolation by replacing real dependencies with controlled test versions. This helps when testing database operations or external API calls - you can simulate responses without needing the actual services running.
Handling Concurrency and Edge Cases
Testing concurrent Go code requires special attention to race conditions and timing issues. The built-in race detector (run tests with -race
) helps catch these problems early. It's also important to test edge cases thoroughly - check boundary values, invalid inputs, and error conditions. For example, test how your code handles empty strings, very large numbers, or network timeouts.
Managing Test Fixtures and Performance
Test fixtures provide consistent test data and environments. As your test suite grows, organize fixtures using helper functions or dedicated setup/teardown code. Keep an eye on test performance - use Go's benchmarking tools to identify slow tests before they impact your development speed. Regular profiling helps catch performance issues early and keeps your test suite running efficiently.
Maximizing Test Coverage Impact
Effective testing in Go extends beyond just hitting high coverage numbers. The real value comes from writing tests that verify essential functionality and prevent actual bugs from appearing. Let's explore how to make your testing efforts count.
Setting Realistic Coverage Goals
While 100% test coverage might seem like an ideal target, it's not always the best use of resources. Smart Go teams identify and focus on testing the code that matters most:
- Critical business logic
- Error-prone areas
- Complex algorithms
- High-risk functionality
- Core features
A payment processing system needs much more thorough testing than basic utility functions.
Implementing Effective Testing Strategies
A balanced testing approach combines several methods:
- Unit tests check individual components using the
golang test command
- Integration tests verify how components work together
- End-to-end tests validate complete user workflows
For example, testing a database operation might involve:
- Unit tests for query logic
- Integration tests for database connections
- End-to-end tests for full data workflows
Ensuring Tests Protect Against Regressions
Good tests catch bugs before they reach production. Key practices include:
- Testing edge cases and error conditions
- Using table-driven tests for checking multiple scenarios
- Validating error handling paths
- Testing boundary conditions
- Including regression tests for fixed bugs
Tools and Techniques for Measuring and Improving Coverage
Go includes powerful testing tools:
go test -cover
generates coverage reportsgo tool cover
helps visualize untested code- Coverage analysis shows which code paths need attention
For instance, these tools might reveal that your error handling code lacks proper test coverage.
The most valuable tests focus on protecting critical functionality rather than just increasing coverage numbers. By targeting the right areas with thorough testing, you build more reliable Go applications that users can depend on. Remember to regularly run your tests using the golang test command
to catch issues early.
Proven Testing Best Practices
When working with Go tests, there are key practices that help create reliable, maintainable test suites that stand the test of time. Let's explore specific approaches that make tests more effective and easier to manage.
Writing Maintainable Tests
Good tests should be clear and simple to understand. Here are the essential elements:
- Clear Test Cases: Each test should check one specific behavior. Keep tests focused and avoid complex logic within a single test.
- Meaningful Names: Choose test names that describe what's being tested. For example, use
TestAddPositiveNumbers
instead ofTestAdd1
. - Independent Tests: Design tests to run on their own without depending on other tests. Shared state between tests often leads to flaky results.
Rather than creating large tests that verify multiple things, break them into smaller, targeted tests. This makes it much easier to identify and fix issues when they occur.
Organizing Test Suites Effectively
As projects grow larger, test organization becomes crucial:
- Package Organization: Keep related tests in the same package for logical grouping and easy navigation
- File Naming: Use the
_test.go
suffix and descriptive prefixes likeauth_test.go
andauth_integration_test.go
- Table-Driven Tests: Use table-driven testing for functions that need multiple input/output combinations. This makes tests more compact and easier to maintain.
This structure helps developers quickly find relevant tests and makes the codebase more manageable over time.
Ensuring Long-Term Test Reliability
Reliable tests catch bugs before they reach production:
- Regular Updates: Review and improve test code just like production code - eliminate duplication and maintain clarity
- Dynamic Test Data: Use helper functions or variables for test data instead of hardcoding values
- Mixed Testing Levels: Combine unit tests with integration tests using the
golang test command
to verify both individual components and their interactions
Make testing part of your regular development workflow to keep tests current and effective.
Actionable Tips for Test Performance and Data Management
Fast, efficient tests help developers stay productive:
- Run Tests in Parallel: Use the
-p
flag to execute tests concurrently and speed up test runs - Focus Testing: Apply
-short
and-run
flags to run specific tests during development - Clean Test Data: Use separate test databases or reset data between tests to prevent interference
These practices help maintain fast feedback loops as your test suite grows.
Want to improve how your team handles testing and code reviews? Check out Mergify today! to see how our automation can help streamline your development process.