Master go test run: Expert Tips & Tricks
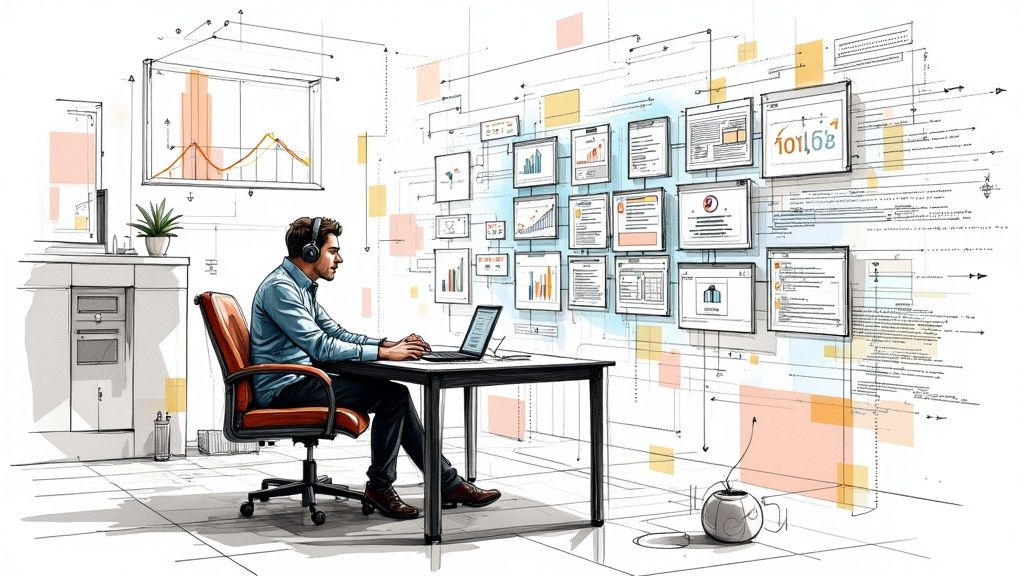
Getting Started with Go Test Run
The go test
command with the run
flag is essential for any Go developer. It lets you run specific tests rather than your entire test suite. This is especially helpful when you need to check a single function after making changes - you can quickly test just that part instead of running everything.
Using the go test run
Command
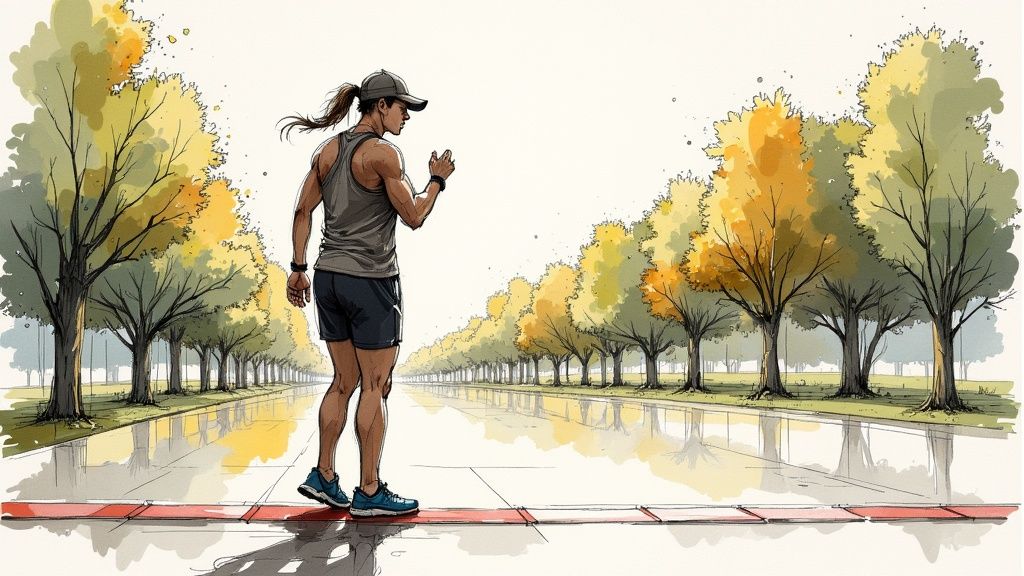
To use the command, add the run
flag followed by a regular expression matching the test names you want to run. For example, go test run CalculateSum
will run any test functions in your *_test.go
files that include "CalculateSum" in their name. This precise control is especially useful in larger projects where running all tests takes significant time.
The run
flag also helps you target tests based on naming patterns. By using consistent names like TestAPI_EndpointName
, you can easily run all API tests with go test run TestAPI_
. This makes organizing and running related tests much simpler.
The Go testing package enhances these capabilities with powerful built-in features. As of Go 1.24, it includes a new Loop
function for benchmarks that handles timing automatically and excludes setup code from measurements. This makes benchmark writing easier and results more accurate.
Practical Examples of go test run
Here are some common ways to use the command:
- Test a specific function:
go test run MyFunction
- Test a particular module:
go test run moduleName_
- Test by keyword:
go test run IntegrationTest
These examples show how go test run
helps in different testing scenarios. The ability to quickly run specific tests speeds up development and debugging. This becomes more valuable as your project grows and testing needs become more complex.
Building Effective Test Organization
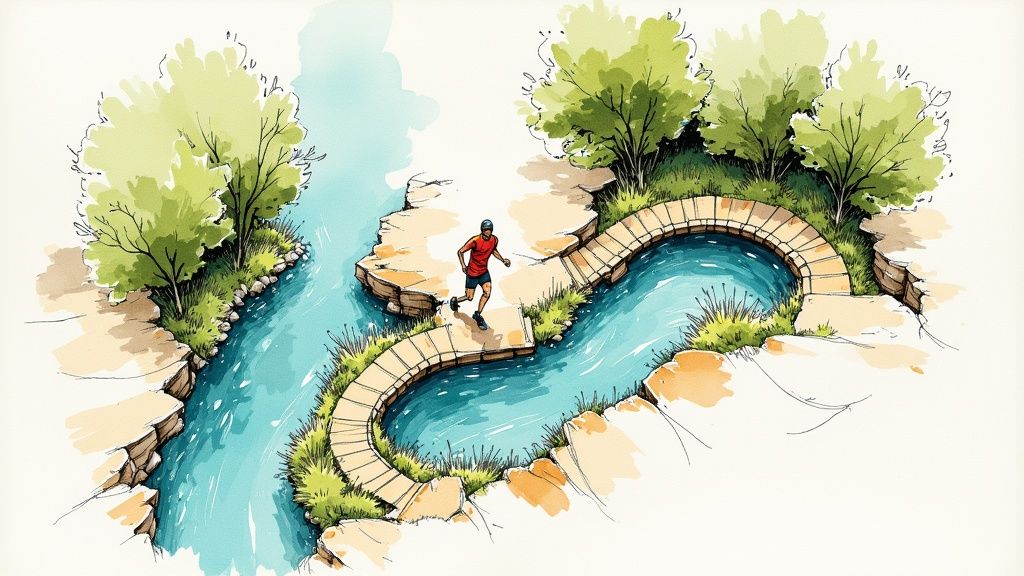
Good test organization makes your codebase easier to maintain and understand, especially when working with go test run
. When you structure tests well, you can quickly find, execute, and update specific tests - saving valuable time as your project grows.
Establishing a Clear Test Hierarchy
Think of your test files like folders on your computer. Set up test directories that match your project's package structure. For example, a users
package should have a matching users_test
package in the same location. This clear organization helps you efficiently use go test run
to target specific package tests.
Managing Test Dependencies Effectively
Many tests need common setup and cleanup steps. Instead of copying this code across tests, create helper functions to handle it. For example, if several tests need a database connection, write one function to open and close it. This approach keeps your tests clean and consistent, which is especially helpful when running specific tests with go test run
.
Implementing Descriptive Naming Conventions
Good test names make it simple to pick which tests to run with go test run
. Use a standard format like Test[FunctionName]_[Scenario]
- for example, TestCalculateSum_PositiveNumbers
. This clear naming helps you use regular expressions with go test run
to find specific test cases.
Leveraging Table-Driven Tests
Table-driven tests let you check multiple inputs and outputs in one test function. This cuts down on duplicate code while making sure you test thoroughly. When used with go test run
, you can easily debug specific test cases using regular expressions.
Here's an example:
func TestCalculateArea(t *testing.T) { testCases := []struct { length int width int area int }{ {2, 3, 6}, {5, 5, 25}, {0, 10, 0}, }
for _, tc := range testCases {
t.Run(fmt.Sprintf("Length:%d_Width:%d", tc.length, tc.width), func(t *testing.T) {
result := calculateArea(tc.length, tc.width)
if result != tc.area {
t.Errorf("Expected %d, got %d", tc.area, result)
}
})
}
}
To run just one case: go test run TestCalculateArea/Length:2_Width:3
These organizational practices help create better tests that work smoothly with go test run
. When your tests are well-structured, you can find and fix issues faster, making your development work more efficient.
Maximizing Your IDE Testing Workflow
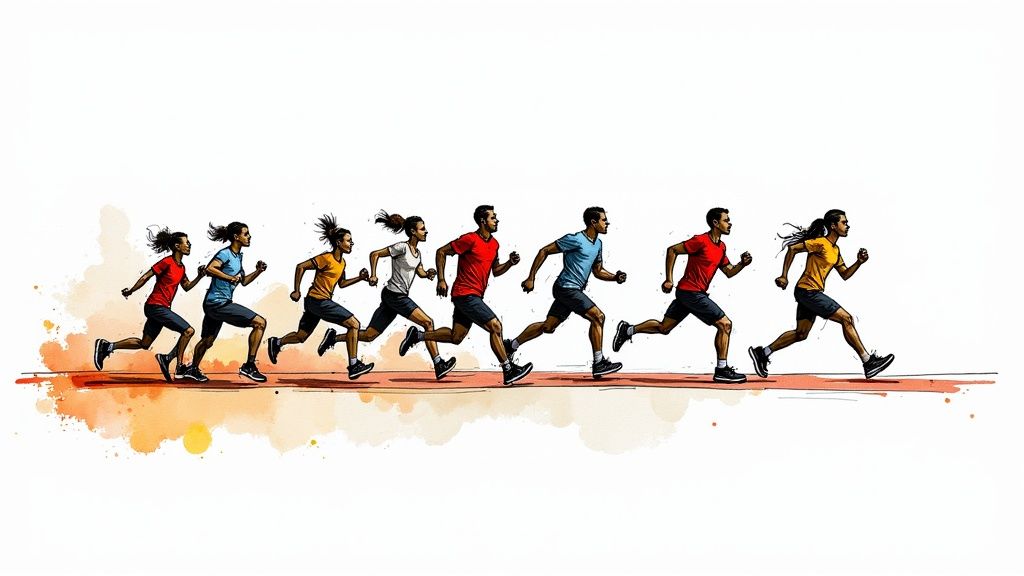
Using a good IDE makes running Go tests much easier and faster. With the right IDE setup, you can spend less time managing test runs and more time writing quality tests and fixing issues. Let's explore how to get the most out of your IDE for Go testing.
Key IDE Features for Go Testing
IDEs provide several essential features that make Go testing more efficient. Let's compare how top IDEs handle key testing capabilities:
Feature | GoLand | VSCode | Atom |
---|---|---|---|
Test Runner | Built-in runner with advanced options | Go extension with basic runner | Basic Go test support |
Debugging | Full debugging toolkit | Debug via Delve integration | Limited debugging |
Code Coverage | Real-time visualization | Coverage via extension | Basic coverage reporting |
Test Results | Detailed test analytics | Simple pass/fail display | Basic results view |
Refactoring | Advanced refactoring tools | Basic refactoring support | Minimal refactoring |
The main features that help with testing include:
- Test Runners: Run tests directly in your IDE instead of switching to the terminal
- Debugging Tools: Set breakpoints, step through code, and check variables to find test failures
- Coverage Tools: See which code is tested right in your editor
- Results Display: Get clear test results and error messages to fix issues faster
- Code Updates: Change code while keeping tests working using built-in refactoring
Optimizing Your IDE for Go Test Run
Here's how to set up your IDE for better testing:
- Learn keyboard shortcuts for running and debugging tests
- Set up test configurations that match your project structure
- Add code coverage tracking to watch test coverage as you code
- Install plugins that help with test visualization and reporting
Choosing the Right IDE
Popular IDEs for Go development include:
- GoLand: Full-featured Go IDE with excellent debugging
- VS Code: Lightweight editor with strong Go support through extensions
- Atom: Simple but capable editor with a growing Go ecosystem
Pick the IDE that fits your workflow best. The right IDE can help you write better tests faster and produce higher quality Go code. By using these testing features effectively, you'll catch bugs earlier and build more reliable applications.
Mastering Advanced Testing Techniques
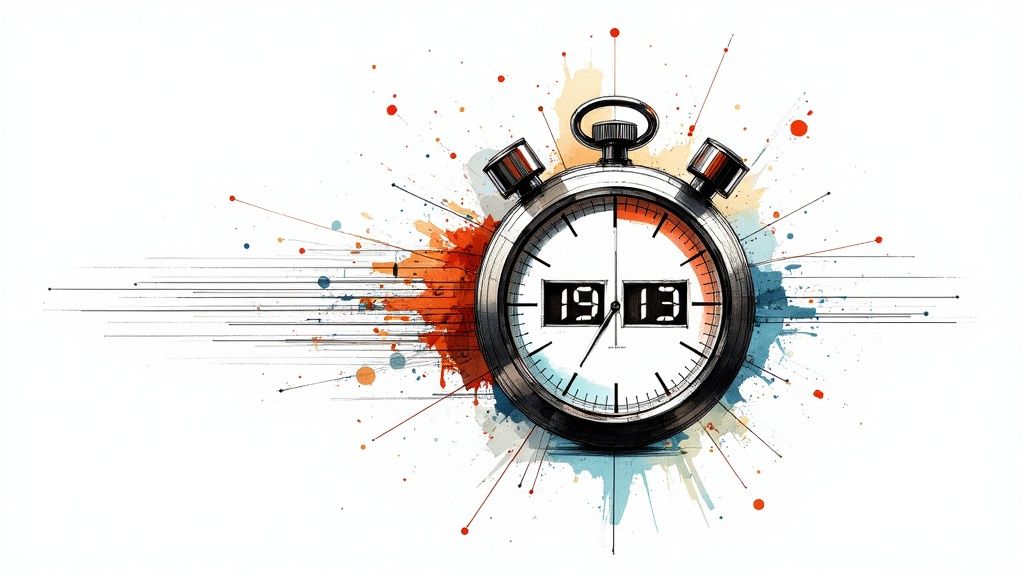
Writing effective tests is crucial for building reliable Go applications. Let's explore key testing strategies used by Go developers to get the most out of go test run
. These approaches help isolate specific test cases, making debugging and development more efficient.
Table-Driven Tests
Table-driven tests organize multiple test cases into a structured format. By arranging inputs and expected outputs in a table or slice, you can easily add or modify test cases without major code changes. This works especially well with go test run
since you can target specific scenarios.
For example, when testing email validation, you might set up a table with various email formats and their expected validation results. Using go test run
with a regex pattern lets you focus on testing just one format at a time. This precision helps quickly identify issues in complex validation logic.
Mocking for Isolation
Mocking creates controlled substitutes for external dependencies like databases or APIs. This isolation helps determine if test failures come from your code or from interactions with external systems. With mock objects, go test run
becomes more powerful - you can test function logic directly without setting up real dependencies.
This focused approach speeds up testing since you don't need to wait for external systems. Developers can quickly verify code behavior and make improvements without infrastructure delays.
Concurrent Testing Patterns
Many modern apps use concurrent code, which can lead to race conditions and hard-to-find bugs. Concurrent testing verifies that code works correctly when running in parallel. The go test
command includes built-in race detection to help catch these issues.
Testing concurrent code requires specific strategies beyond basic race detection. You need carefully designed tests that trigger different combinations of goroutine execution to find concurrency bugs. Using go test run
helps target these complex scenarios, making it easier to isolate and fix threading issues.
Practical Test Implementation Guide
Here's an overview of common testing patterns and when to use them:
Pattern | Use Case | Example | Benefits |
---|---|---|---|
Table-Driven Tests | Testing multiple inputs/outputs | Testing various email formats | Improved code organization, easier test case management |
Mocking | Isolating unit under test | Simulating a database connection | Clearer test results, easier debugging |
Concurrent Testing | Verifying concurrent behavior | Testing a function with multiple goroutines | Identifies race conditions, ensures robustness in concurrent environments |
By applying these techniques and using go test run
effectively, you can build comprehensive test suites that catch bugs early and ensure your code works reliably. The combination of smart testing strategies and targeted test execution helps create stable, high-quality Go applications.
Monitoring and Managing Test Performance
Effective test monitoring keeps your go test run
commands valuable and reliable as your codebase grows. By tracking performance metrics and consistency, you can catch issues early and maintain test quality.
Tracking Test Execution Time
Your test suite will naturally take longer to run as your project grows. By regularly measuring execution time, you can spot any concerning spikes that may indicate inefficient tests or underlying code issues. Regular monitoring helps keep your development feedback loop fast and effective.
Identifying and Addressing Bottlenecks
Individual tests can sometimes become performance bottlenecks that slow down your entire test suite. Common causes include excessive network calls, database operations, or resource-heavy test logic. Using profiling tools helps pinpoint exactly where tests are spending the most time, so you can focus your optimization efforts.
Maintaining Consistent Test Results
Your tests need to produce reliable results across development, staging and production environments. Issues often stem from environment-specific settings, external services, or test data inconsistencies. Some effective strategies include:
- Using containerized test environments
- Mocking external dependencies
- Carefully managing test data across environments
Tools like QAComplete can help track test history and identify inconsistencies over time.
Strategies for Long-Term Test Health
Keep your test suite healthy and effective with these key practices:
- Review and refactor tests regularly - Tests need maintenance just like application code
- Establish clear test ownership - Ensure tests stay updated as code changes
- Monitor test performance in CI/CD - Get automated feedback on every code change
- Use coverage tools strategically - Focus on testing critical application components
By actively monitoring and managing your tests, you'll get lasting value from your go test run
commands. This practical approach leads to more stable and maintainable tests that serve your development process well.
Essential Testing Best Practices
Good testing is fundamental to building reliable Go applications. Let's explore key practices that can help you create better tests and build stronger, more maintainable code.
Writing Effective Test Cases
Start with unit tests - they help you test individual components in isolation. When using go test run
, break down complex functions into smaller testable pieces. For instance, if you're testing a price calculator, create separate tests for standard pricing, special discounts, and edge cases like zero or negative values.
Table-driven tests are a powerful way to organize multiple test scenarios. Think of them like a spreadsheet - each row represents a unique test case with specific inputs and expected outputs. This format works seamlessly with go test run
and lets you filter tests using pattern matching.
Integrating Mocking and Dependency Injection
Mocking helps isolate the code you're testing from external systems. For example, instead of connecting to a real database during tests, create a mock that simulates database behavior. This makes your go test run
commands faster and produces consistent results.
Dependency injection makes testing easier by letting you swap real dependencies with test versions. Rather than hardcoding dependencies, pass them in as parameters. This makes your code more flexible and easier to test.
Implementing a Robust Testing Workflow
Make testing part of your daily development process. Set up automated testing in your CI/CD pipeline to catch problems early. Running tests on every code change helps prevent bugs from reaching production.
Track test coverage to find gaps in your testing. While 100% coverage isn't always needed, focus on testing critical features and complex logic. Use go test -cover
to measure and improve your coverage over time.
Emphasizing Test Maintainability
Keep your tests clean and up-to-date as your code evolves. Apply the same quality standards to tests as you do to production code. Review tests regularly to ensure they remain useful and accurate.
Build a strong testing culture in your team. During code reviews, examine both the main code and its tests. Share testing knowledge and best practices with teammates.
These practices help turn testing from a chore into a valuable tool for building better Go applications. go test run
becomes an essential part of how you work, helping you ship quality code with confidence.
Want to improve your development process? Check out Mergify to automate pull requests and strengthen your CI/CD pipeline.