Master the go test command for Enhanced Go App Testing
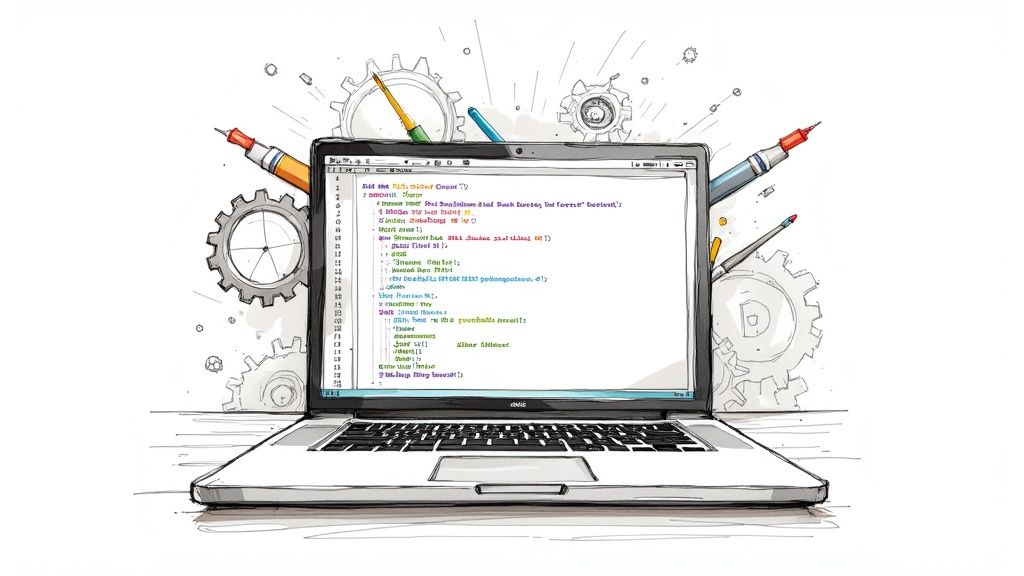
Getting Started with the Go Test Command
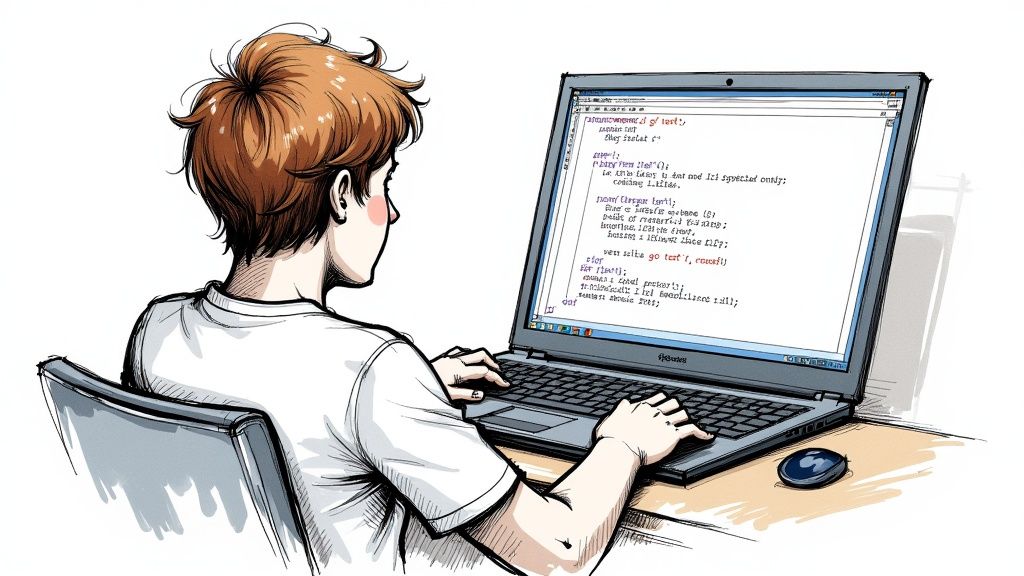
Learning how to use the go test
command is essential for writing reliable Go applications. This built-in tool helps you write and run tests to verify your code works correctly. Let's walk through the key steps to create your first test suite.
Structuring Test Files
Go test files must end with _test.go
. Inside these files, you'll write test functions that start with Test
followed by the name of what you're testing (which must begin with a capital letter). For example, to test a CalculateSum
function, you'd name the test TestCalculateSum
. This naming pattern helps the test runner find and execute your tests.
Implementing Basic Test Cases
Your tests will use Go's testing
package to check if your code behaves as expected. When writing tests, you'll use functions like t.Errorf
or t.Fatalf
to report when something goes wrong. For instance, if CalculateSum
returns the wrong answer, t.Errorf
tells you exactly what went wrong and where to look.
Interpreting Test Results
Running go test
shows you whether tests passed or failed. Successful tests appear in green, while failures show up in red with helpful error messages. These messages tell you what went wrong - like when an expected value doesn't match what your code actually produced.
The go test
command has been part of Go since the language began. One of its most useful features is the ability to generate test coverage reports. Just add the -cover
flag to see what percentage of your code is tested. Check out more testing tips in this Comprehensive Guide to Testing in Go.
Common Pitfalls and Solutions
Many developers run into similar challenges when they start testing in Go. Two big ones are not handling errors properly in tests and writing overly complex test cases. Keep your tests simple and focused on checking one specific thing at a time. This makes them easier to maintain and more effective at catching bugs.
Mastering Test Command Flags and Options
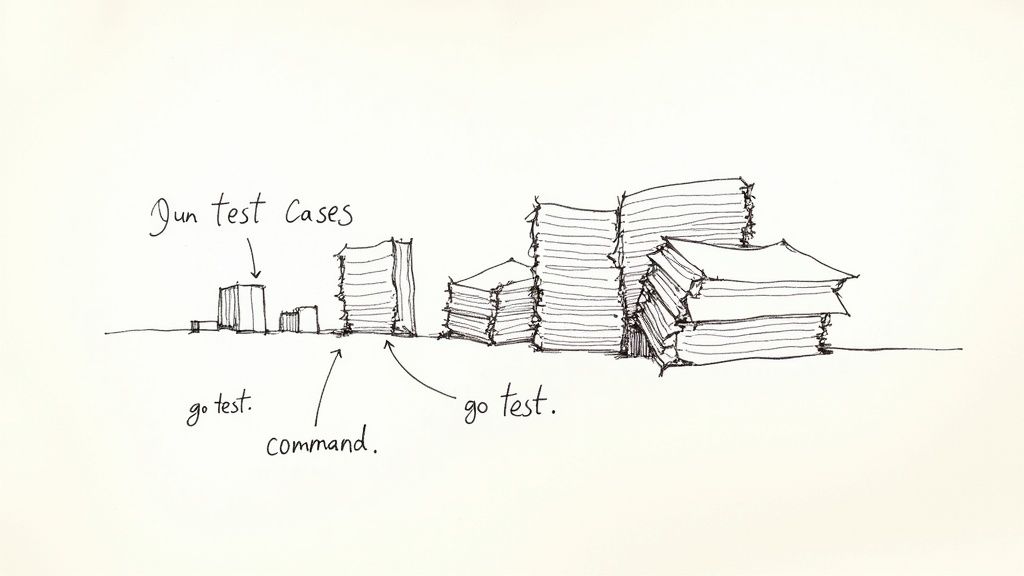
The go test
command gives you powerful control over how you run tests in Go. Understanding these flags helps you test more effectively and get better insights into your test results.
Common Go Test Command Flags
Let's explore the most frequently used test flags to help you get started. Here's a handy reference table of essential flags you'll use often:
Flag | Purpose | Example Usage |
---|---|---|
-v | Shows detailed test output | go test -v |
-run | Runs specific tests matching a pattern | go test -run TestLogin |
-short | Runs tests in quick mode | go test -short |
-failfast | Stops after first test failure | go test -failfast |
-count | Repeats tests multiple times | go test -count=3 |
The -v (verbose) flag is particularly helpful during debugging as it shows each test's progress and results. When you need to focus on specific tests, the -run flag lets you use regular expressions to select exactly which tests to run.
Managing Test Output
When dealing with many tests, the output can get overwhelming. Several tools can help make test results more readable:
- rakyll/gotest - Adds color coding to test output
- spectest - Shows only failed tests and summary stats
The -json flag outputs results in JSON format, which works great when you need to process test results in CI/CD pipelines or other tools.
Advanced Testing Options
For larger projects, you can use advanced flags to speed up testing and get more insights:
- -parallel lets you run multiple tests at once
- -timeout prevents tests from running too long
- -coverprofile generates reports showing which code your tests cover
These options help you find the right balance between thorough testing and fast feedback in your development workflow.
By picking the right combination of these flags, you can create a testing setup that matches your needs while keeping your tests reliable and maintainable.
Conquering Advanced Testing and Race Detection
The go test
command offers powerful features beyond basic testing. Let's explore some advanced techniques that will help you write better tests and catch tricky bugs early.
Parallel Testing with go test
Speed up your test runs by running tests in parallel with the -parallel
flag. For example, running go test -parallel 4
executes tests using up to 4 parallel processes. This simple change can dramatically reduce test execution time for large test suites. If you don't specify a number, the command uses your GOMAXPROCS
value.
Organizing Tests with Subtests
Group related tests together using subtests to keep your test code clean and organized. The t.Run
function lets you create subtests within a single test function. This makes it easier to:
- Share setup and teardown code
- Group related test cases
- Get clearer test output when failures occur
- Run specific subtest groups
Benchmarking Your Go Code
Want to know if your code changes made things faster or slower? Go's built-in benchmarking helps you measure performance. Here's how it works:
- Create functions starting with
Benchmark
- Use the
b *testing.B
parameter - Let
b.N
control iteration count - Run with
go test -bench
The benchmarking system automatically determines how many iterations to run to get reliable timing data.
Detecting Race Conditions
Race conditions can cause mysterious bugs in concurrent code that are hard to reproduce. The go test
command includes a powerful race detector - just add the -race
flag when running tests. This tool helps catch data races where multiple goroutines access shared data without proper synchronization. Learn more about race detection in the Go testing documentation.
Balancing Testing and Maintainability
Good tests need to be both thorough and maintainable. Focus on writing clear, focused test cases that:
- Test one specific thing at a time
- Have descriptive names
- Avoid unnecessary complexity
- Are easy to debug when they fail
By keeping tests simple and focused while using Go's advanced testing features, you can build a test suite that catches bugs early while remaining easy to maintain as your codebase grows.
Maximizing Test Coverage and Quality
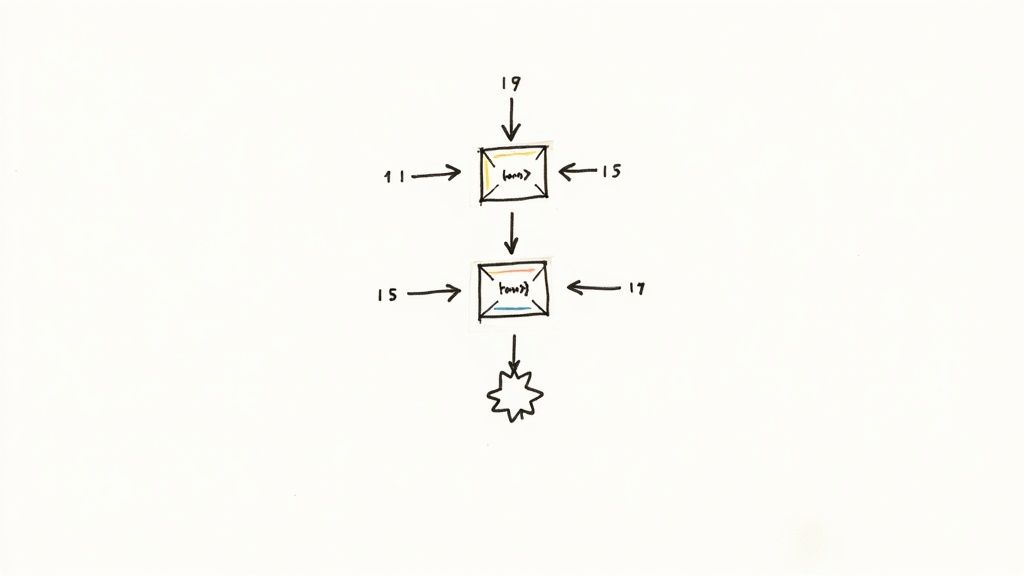
Now that we understand how to use go test
, let's focus on making our tests more thorough and effective. We'll look at smart ways to test important code paths and make sense of coverage data.
Understanding Test Coverage
Test coverage shows how much code your tests actually run. While higher percentages often mean better testing, the numbers don't tell the whole story. Writing meaningful tests matters more than chasing high coverage numbers. For instance, testing simple getter/setter methods might boost your coverage stats but won't help catch real bugs. Focus instead on testing complex logic and edge cases where problems are more likely to occur.
Strategies for Increasing Test Coverage
Start by identifying the critical code paths in your application - the core functions that absolutely must work correctly. Use the reports from go test -cover
to find gaps in your testing. This lets you focus your efforts where they matter most, rather than trying to test everything equally.
Interpreting Coverage Metrics
Here's how to make sense of different types of test coverage:
Metric Type | Description | Target Range |
---|---|---|
Statement Coverage | How many lines of code your tests run | 80-90% |
Branch Coverage | How many if/else and switch paths are tested | 70-85% |
Function Coverage | How many functions get called during tests | 75-90% |
While 100% coverage might sound great, it's often impractical and unnecessary. Focus on writing tests that actually prove your code works, even if that means slightly lower coverage numbers.
Maintaining High-Quality Tests
Good tests need regular attention. Update them as your code changes to keep them relevant. Write clear, simple tests that other developers can understand. Well-written tests not only catch bugs but also show others how the code should work.
Beyond Coverage: Evaluating Test Effectiveness
Coverage is just one way to measure test quality. Also consider:
- Test speed: Fast tests let you get quick feedback while coding
- Test reliability: Remove tests that sometimes pass and sometimes fail
- Mutation testing: Check if your tests can spot when code changes break things
These factors help you build tests that truly protect your code quality. Making good use of go test
is key - not just running the command, but understanding what the results mean and using them to improve your testing approach.
Building Scalable Test Organization Patterns
Building a clean, efficient test suite becomes essential as Go projects expand. Without clear organization, tests quickly become hard to navigate and maintain. Here's how successful Go teams structure their tests for long-term success.
Organizing Test Helpers
Test helpers reduce code duplication, but they need proper organization. Place helper functions in a dedicated testutils_test.go
file within your test package. This keeps common test utilities like setupTestDatabase()
or createTestUser()
in one accessible location while separating them from production code. With this structure, running tests via go test
becomes more straightforward.
Implementing Mocks Effectively
Mocks play a key role in isolating code units during testing. Create interfaces that represent your code's dependencies to keep tests focused and decoupled. For example, instead of directly using a database connection, define a DataStore
interface that you can easily mock using tools like testify/mock. This approach makes managing test dependencies much simpler.
Establishing Conventions
Clear test conventions help teams work together efficiently. Set standard patterns for:
- Test file naming (always use
_test.go
suffix) - Test function naming (start with
Test
prefix) - Helper function usage
- Mock implementation approaches
Following these patterns keeps tests predictable and easier to understand across the team.
Documenting Tests for Future Team Members
While good code is important, explaining test purpose and design is crucial. Add clear comments that describe:
- The specific scenarios being tested
- Expected outcomes
- Any complex test setup or edge cases
- Overall testing strategy and conventions
This documentation helps new team members understand the test suite quickly and ensures tests remain maintainable as the codebase grows. When used with go test
, well-documented tests provide clear insights into test failures and expected behavior.
Optimizing Test Performance and Reliability
Running tests effectively means having them complete quickly and consistently. When tests are slow or unreliable, they can slow down development and make teams lose faith in their test suite. Let's explore practical ways to improve how your go test
command runs to achieve both speed and reliability.
Identifying and Fixing Flaky Tests
Flaky tests - those that pass sometimes and fail other times without code changes - can be incredibly frustrating. Finding them requires watching test runs carefully, especially in CI/CD systems. Common causes include:
- Test Order Dependency: Tests need to work independently without relying on other test side effects
- Resource Leaks: Not closing files or network connections properly
- Concurrency Issues: Multiple tests accessing shared resources without proper synchronization. Use
go test -race
to spot these problems - Timeouts: Tests with external services may need adjusted timeout settings. Try
go test -timeout 30s
to set suite-wide timeouts - External Dependencies: Tests depending on external systems are prone to failure. Consider using test doubles instead
Reducing Test Execution Time
Slow tests bog down development. Here's how to speed up your test runs:
- Parallelization: Run tests concurrently with
go test -parallel
for better performance on multi-core systems - Profiling: Find slow spots using Go's profiling tools - run
go test -cpuprofile cpu.prof
and analyze withgo tool pprof
- Caching: Save and reuse expensive setup steps across multiple tests
- Targeted Testing: Use
go test -run
with regex patterns to run only relevant tests while working on specific features
Implementing Effective Debugging Practices
When tests fail, good debugging is key:
- Verbose Output: See detailed test info with
go test -v
- Debugging Tools: Step through code using Delve to inspect variables and find errors
- Logging: Add strategic log statements to understand the sequence leading to failures
Maintaining Consistent Test Reliability in CI/CD
Keeping tests stable in CI/CD requires:
- Isolated Environments: Run tests in clean, separate environments to avoid build conflicts
- Regular Test Runs: Test on every code change
- Test Result Analysis: Monitor results over time to spot trends and areas needing improvement
By applying these approaches and using go test
effectively, you'll build faster, more reliable tests that support quality code and smooth development.
Want simpler development workflows and better CI/CD? Check out Mergify for smart merge automation.