Accelerate Your Testing with Go Go Test Strategies
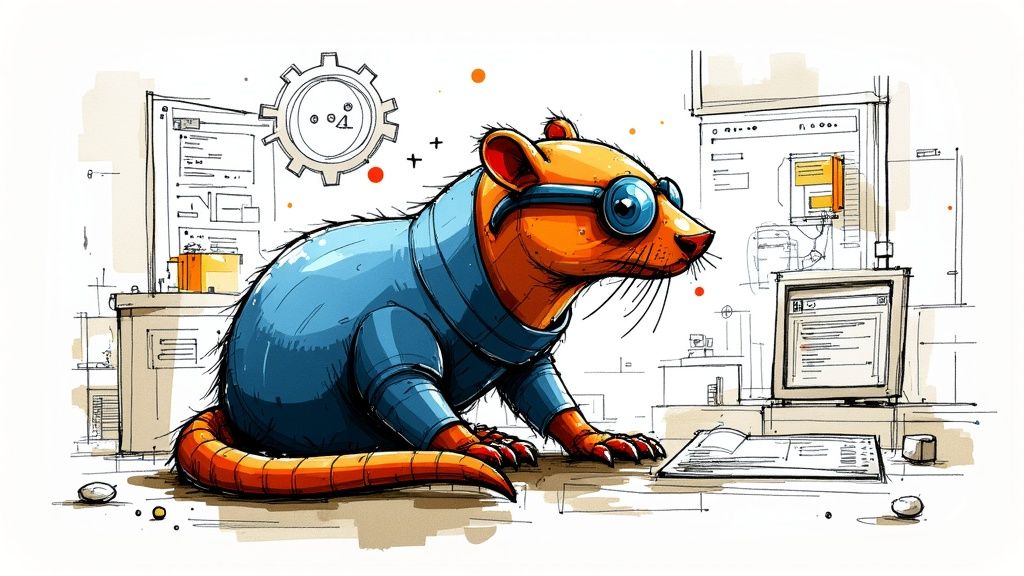
Demystifying Go Go Test For Real-World Success
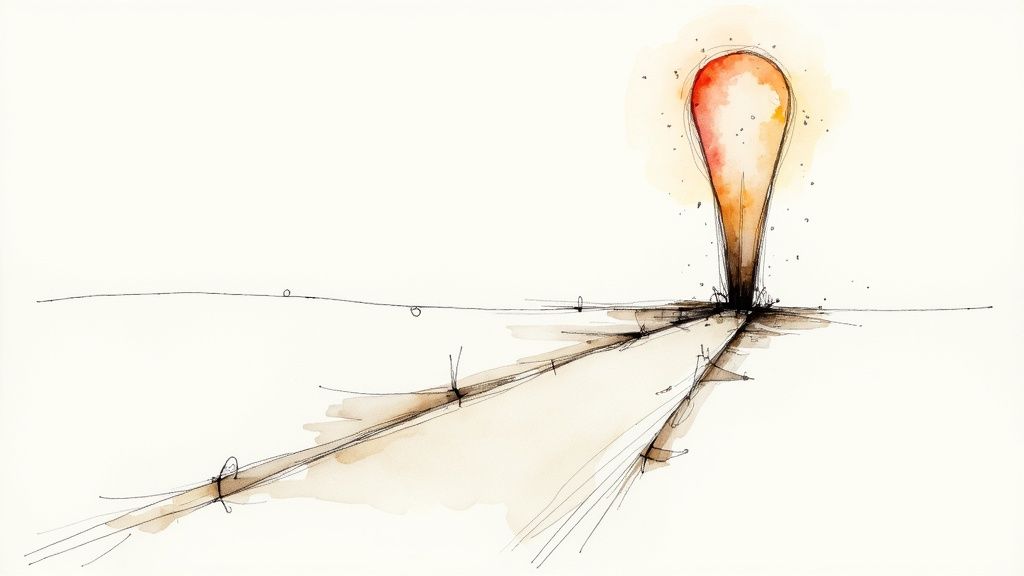
The go test
command is essential for building reliable Go applications. It does more than just run basic tests - it's a powerful tool that lets you analyze code performance through benchmarks. These benchmark functions follow the format func BenchmarkXxx(*testing.B)
and provide detailed performance data when you use the -bench
flag. Learn more about Go testing at pkg.go.dev.
Understanding the Basics of Go Go Test
Getting started with go test
is straightforward - create test files with names ending in _test.go
in your project. Your test functions should follow the pattern TestXxx(*testing.T)
, where Xxx
describes what you're testing. The tool automatically finds and runs these test functions, making the testing process simple and efficient.
Moving Beyond the Basics: Table-Driven Tests
Table-driven tests help you test multiple scenarios without repeating code. By using a slice of structs or arrays, each element represents a different test case. This makes it easy to:
- Add new test cases quickly
- Maintain existing tests
- Get better test coverage
- Keep test code organized
Best Practices For Real-World Success
Good testing needs more than just technical knowledge. Here are key practices that make testing work better:
- Group related tests together in logical files
- Use clear, descriptive names for test functions
- Create helper functions to reduce duplicate code
- Keep test code clean and readable
The final piece is connecting your tests to your CI/CD pipeline. Running tests automatically helps catch problems early and keeps your code stable.
Crafting Test Reports That Drive Decision Making
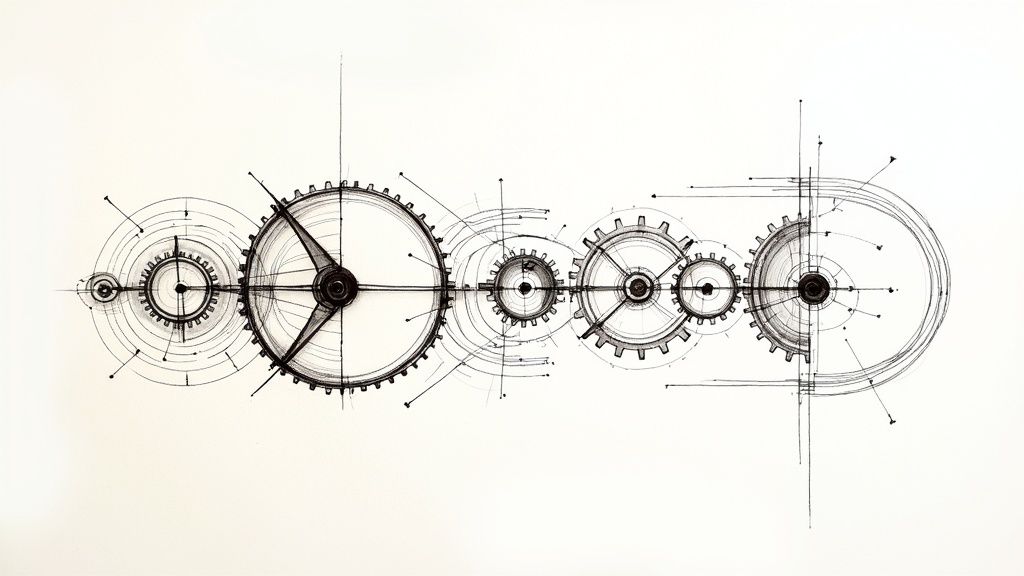
Testing with go test
requires more than just pass/fail results - it needs clear insights teams can act on. The raw output often presents a wall of text that makes finding key issues difficult. Well-designed test reports help teams spot problems faster and make better decisions about code quality.
Transforming Raw Data Into Clear Insights
Let's examine how test reporting tools can help teams get more value from their test results. Here's a comparison of key reporting features:
Feature | Basic Go Test | Enhanced Report Tools | Benefits |
---|---|---|---|
Results Format | Plain text output | Interactive HTML/JSON | Easier analysis and sharing |
Error Details | Basic error messages | Stack traces and context | Faster debugging |
Statistics | Pass/fail counts | Trends and patterns | Better decision making |
Organization | Chronological only | Grouped by test type | Improved navigation |
The standard go test
output works for basic needs, but larger projects need better organization and insights. Think of it like reading complex code - you need proper formatting and structure to understand what's happening.
Making Reports Work for Different Teams
Each team member needs different information from test results. Developers want detailed error messages and traces, while managers need high-level metrics. Custom reports help meet these varied needs effectively.
When critical bugs appear, good reports help teams:
- Quickly identify the failing tests
- See exact error conditions
- Track fix progress
- Share status updates
Using Tools for Better Reports
The go-test-report tool enhances basic test output with:
- Clean HTML reports
- Test result grouping
- Color-coded status indicators
- Summary statistics
Many CI platforms also offer built-in reporting that works with Go tests. These often include:
- Custom dashboards
- Historical data
- Automated notifications
- Trend analysis
Automating Result Analysis
Smart automation helps teams work faster by:
- Finding patterns in failures
- Flagging performance issues
- Tracking code coverage gaps
- Suggesting test improvements
Good reports turn raw test data into actionable insights. This helps teams spend less time digging through logs and more time improving code quality.
Mastering Performance Testing That Matters
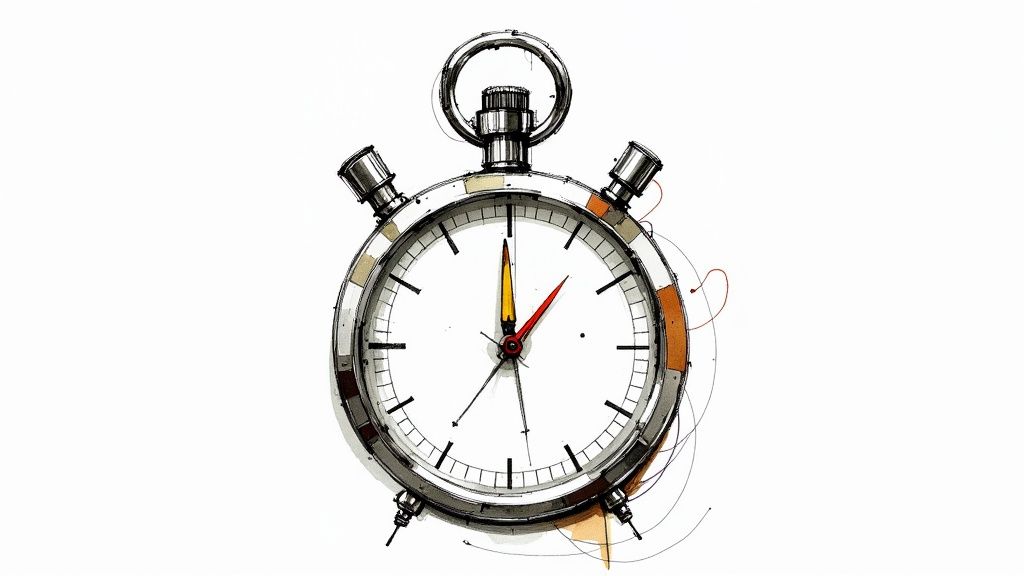
Getting real value from performance testing with go test
requires careful planning and execution. The key is creating tests that match how your application works in the real world, then using those results to make smart improvements.
Designing Effective Benchmarks
Smart teams build their go test
benchmarks to match real usage patterns. This means testing complete workflows - including database calls and network requests - instead of just isolated functions. They also test with different data sizes and concurrent users to find problems that only show up under heavy load.
Identifying Key Performance Metrics
Different metrics matter for different applications. Throughput and latency often have the biggest impact on users. For a real-time app, keeping latency low is critical. But if you're building a batch processing system, high throughput might be your main goal. Focus your go test
efforts on the numbers that truly matter for your specific case.
Analyzing Results and Making Optimization Decisions
Reading go test
results takes careful attention. Watch for patterns that show where performance drops as load increases. A jump in response times might point to database issues. Use these findings to guide your improvements - whether that means rewriting code, tuning your database, or changing your system design.
Avoiding Common Benchmarking Pitfalls
Watch out for these common go test
mistakes:
- Running tests that don't match real usage
- Forgetting about external factors like network delays
- Making decisions based on too little data
Run your tests multiple times with different settings to get reliable results. Good benchmarking combines careful setup, thoughtful analysis, and ongoing testing to keep improving performance.
Building Test Suites That Scale With Your Code
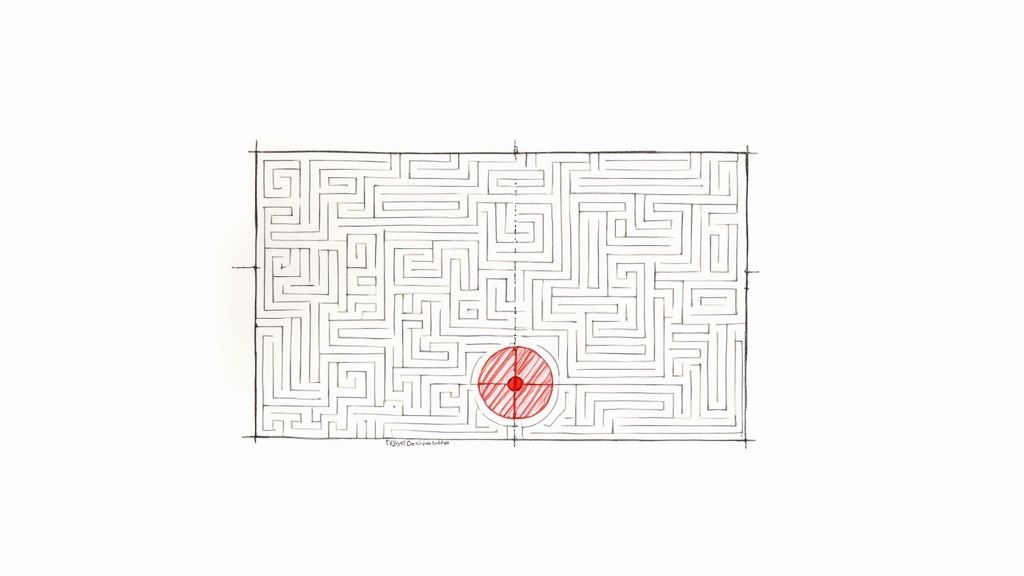
A growing Go project needs a well-organized test suite to remain maintainable. When tests become messy, they slow down development and make your CI/CD pipeline drag. Let's explore practical ways to build go test
suites that grow smoothly with your code.
Organizing Tests for Clarity and Maintainability
Just like a well-planned city needs clear street signs, your test suite needs thoughtful organization. Here's how to structure your go test
files so they stay manageable:
- Package Layout: Match your test structure to your main code. For a
users
package, create a matchingusers_test
package to keep related tests together - Clear File Names: Follow Go's standard
*_test.go
naming pattern - it helps thego test
command find your tests - Descriptive Test Names: Name tests to show what they check.
TestUserLogin
tells more than justTest1
Strategies for Managing Test Complexity at Scale
As projects grow, you need smarter ways to handle more tests. Try these go test
approaches:
- Use Subtests: Group related checks with
t.Run()
. This creates easy-to-read test output and helps pinpoint failures - Create Helper Functions: Put common setup code in helpers to avoid repetition and keep tests consistent
- Write Table Tests: When testing many similar cases, use table-driven tests. One test function can handle many scenarios using a slice of test cases
Real-World Examples: Structuring Your Go Test Suite
Take user authentication testing as an example. Instead of one big test function, break it into focused subtests:
t.Run("ValidCredentials", ...)
- Check successful login workst.Run("InvalidCredentials", ...)
- Verify wrong passwords are rejectedt.Run("LockedAccount", ...)
- Test locked account handling
For input validation, table tests let you check many cases clearly. This approach keeps tests organized and efficient while making changes easier. With good test structure, you can confidently update your code knowing your tests will catch problems.
Using Go Go Test in CI/CD Pipelines
Adding go go test
to CI/CD pipelines helps catch bugs early and maintain code quality. Learn how to configure test runs, monitor results, and optimize performance to make testing an asset rather than a bottleneck.
Setting Up Efficient Test Execution
Running tests efficiently starts with parallel execution. The go test
command's -p
flag runs multiple tests simultaneously, reducing overall run time. For large codebases, this is like having several workers tackling tasks at once instead of one doing everything sequentially.
Test dependency caching also speeds up runs by avoiding repeat downloads. This matters most when dealing with lots of external packages.
Environment Setup for Testing
The right environment setup ensures consistent testing. Start by specifying your Go version in the CI/CD config file. This keeps behavior predictable across different systems.
Next, add any environment variables your tests need, like database connections or API keys. Having the correct setup is like preparing a stage before a performance - it enables tests to run smoothly.
Result Tracking and Feedback
Beyond just running tests, getting actionable results matters. Most CI platforms display test outcomes clearly, making it easy to spot failures and analyze patterns.
Quick developer alerts about test failures help catch issues fast. Like a smoke detector warning of danger, prompt notifications let teams address problems immediately.
Real Implementation Examples
Teams integrate go go test
in different ways. Some connect with specialized testing services, while others use custom scripts tailored to their needs.
Here are key metrics for successful test integration:
Metric | Target Value | Impact |
---|---|---|
Test Runtime | < 5 minutes | Faster feedback loops |
Code Coverage | > 80% | Better code reliability |
Failure Rate | < 1% | Fewer regressions |
By optimizing your CI/CD pipeline for quick, reliable testing with clear feedback, go go test
becomes a powerful quality tool. This testing approach helps teams catch issues early and ship better code.
Solving Real-World Testing Challenges
Testing with go test
can present real obstacles, even with carefully crafted tests. Let's look at common problems Go developers face and practical ways to solve them.
Diagnosing Test Failures
When your tests fail, understanding why is crucial. Start by carefully reading the error messages - they usually show exactly where and why things went wrong. If you need more detail, run go test
with the -v
flag for expanded output. Like a debugger, this shows you step-by-step what happened during the test.
Managing Flaky Tests
Flaky tests that randomly pass or fail make it hard to trust your test results and spot real issues. Here's how to tackle them:
- Reproduce the Issue: Run the problematic test multiple times to see if you can spot patterns in when it fails
- Test in Isolation: Run just that one test by itself to check if other tests are affecting it
- Find the Root Cause: Look closely at timing issues, race conditions, and external services the test depends on. For instance, tests that rely on strict timing can fail when system performance varies
Maintaining Testing Efficiency at Scale
As your codebase grows, keeping tests running smoothly takes work. Here are key approaches:
- Focus Your Testing: Use build tags or the
-run
flag to test just what you need. This saves significant time on large projects - Run Tests in Parallel: Use
go test
's parallel testing features to speed things up, especially on machines with multiple cores - Watch Resource Usage: Keep an eye on memory and CPU use. Make sure tests clean up after themselves - like deleting test files or database records - so they don't slow down other tests
Case Study: Debugging a Complex Integration Test
Consider a test that connects to multiple services and fails occasionally. The error points to database connection problems. Using go test -v
reveals that sometimes the test tries connecting before the database is ready. The fix? Add a short wait or retry mechanism to ensure the database is available before the test continues.
These practical strategies help you build reliable tests that grow with your project. Good tests catch bugs early and help you ship code confidently.
Ready to improve your development process? Try Mergify's smart merge automation. Cut CI costs and help developers work more efficiently. Check out Mergify today.