Git Fetch vs Pull: A Strategic Guide for Modern Developers
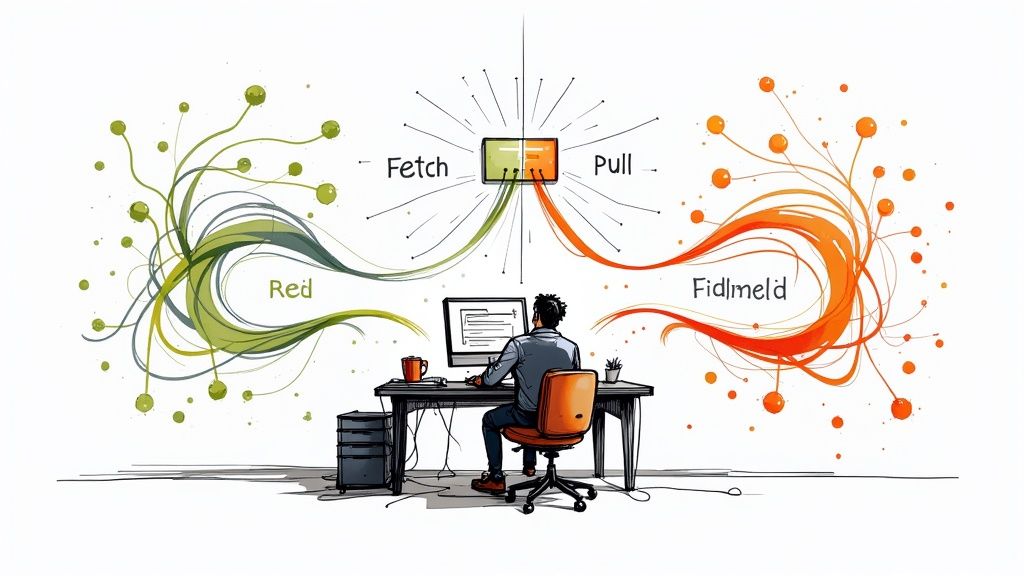
Understanding Git Operations Without The Confusion
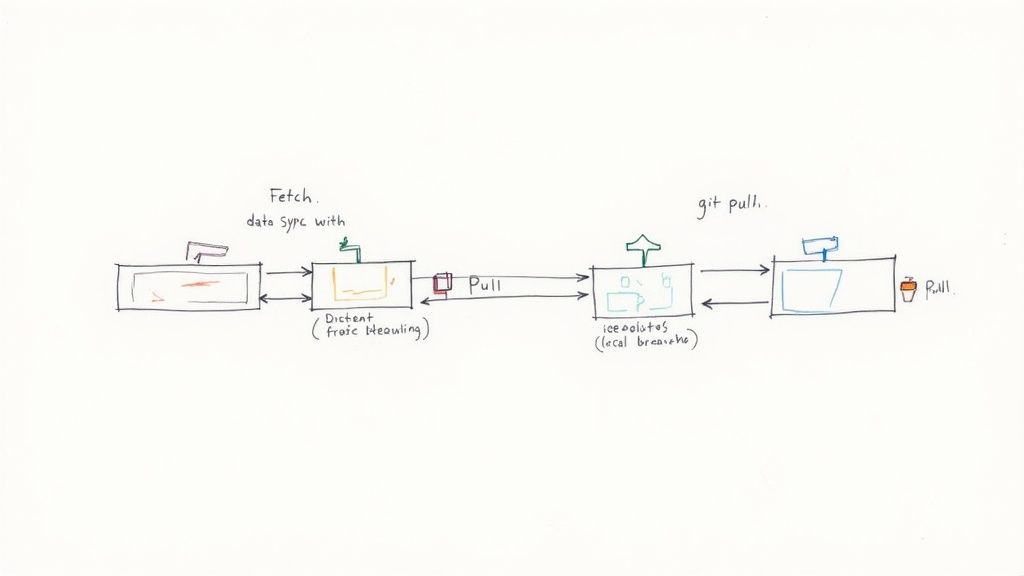
When working with Git, choosing between git fetch
and git pull
can seem confusing at first. But understanding the key differences between these commands helps you work more effectively and avoid common pitfalls. Let's break down how each command works and when to use them in your daily coding workflow.
Fetching: A Safe Preview of Remote Changes
Think of git fetch
like checking your mailbox - you're just looking at what mail has arrived, without opening or reading anything yet. This command safely downloads updates from your remote repository without changing your local files. For example, when your team members push changes to the main branch while you're working on a feature, git fetch
lets you see their updates without disrupting your work. This separation keeps your local environment stable as you review incoming changes.
This cautious approach really shines when working on larger teams. By fetching first, you can check what changes are coming and plan how to integrate them smoothly. You might spot potential conflicts early or realize you need to adjust your own code before merging. This helps prevent messy merge conflicts and keeps your project's history clean and organized.
Pulling: Direct Integration, Potential Conflicts
On the other hand, git pull
is like getting your mail and immediately opening everything at once. It combines two steps: fetching remote changes and merging them straight into your local branch. While this quick update is handy when working alone or on simple changes, it can lead to merge conflicts if you're not careful - especially when your local changes overlap with incoming updates.
Picture git pull
as ordering takeout delivery - it's fast and convenient, but you might get something unexpected. This direct approach works well when you're confident about merging, like on a personal feature branch. But for shared branches or complex projects, many developers prefer checking changes with git fetch
before pulling. This extra step helps avoid surprises and keeps work flowing smoothly.
Choosing the Right Command: A Strategic Decision
Your choice between git fetch
and git pull
should match your current needs and comfort level. git fetch
offers more control and safety, making it ideal for collaboration on shared codebases. This becomes especially important as projects grow larger, since bigger repositories take longer to process updates.
While git pull
saves time with its all-in-one approach, it's less predictable in team settings. Many developers follow a simple pattern: first using git fetch
to preview changes, reviewing what's new, then running git pull
once they're ready to integrate updates. This method lets you spot potential issues before they become problems, helping maintain a smooth development process. By understanding these differences, you can pick the right command for each situation and work more confidently with your team's code.
Mastering Performance In Large-Scale Projects
When handling large repositories, the choice between git fetch
and git pull
becomes crucial for your team's efficiency. What starts as a few seconds delay can snowball into hours of lost development time across a large project. Let's examine how repository size affects performance and look at proven strategies teams use to maintain smooth operations.
Why Size Matters: The Impact on git fetch
and git pull
Repository size directly affects how much data git fetch
needs to process. Since git fetch
downloads all new commits, files, and references from the remote, large projects naturally take longer to fetch than smaller ones. Studies comparing command-line Git and JGit (a Java implementation of Git) show that command-line Git typically performs better when fetching from large repositories. This highlights how your choice of Git implementation can make a real difference at scale.
While having many branches and tags does slow things down somewhat, the main factor affecting git fetch
speed is the size of objects in your repository. This means that even with excellent branch organization, repositories containing many large files will experience slower fetch times. Since git pull
includes a fetch operation, it faces these same challenges - especially when merging large changes or handling conflicts in big projects.
Optimizing Your Workflow for Large Repositories
How do teams stay efficient when working with large repositories? One key approach is to be selective with git fetch
. Rather than pulling constantly, developers can fetch updates strategically, review them locally using git log --oneline --graph --decorate --all
, and only pull when needed. This reduces unnecessary merges and keeps local operations quick.
Managing binary files effectively is also essential. These files can quickly bloat your repository and slow down every Git operation. Using Git Large File Storage (LFS) to store binary files externally while keeping lightweight references in your repository can make a big difference. This is particularly helpful for projects with lots of media files, datasets, or other binary content.
Managing Multiple Remotes and Distributed Teams
Working with multiple remotes or distributed teams adds another layer of complexity to performance considerations. When fetching from several remotes, performance issues can stack up quickly. Be strategic about which remotes you fetch from and when, focusing on those most relevant to your current work. Tools like Mergify can help by automating and optimizing merge processes, reducing the overhead of managing multiple remotes and pull requests in large repositories.
Remember that keeping performance in check requires ongoing attention. Make it a habit to review your repository's size and structure regularly. Clean out unnecessary files, implement clear branching practices that limit long-lived branches, and encourage frequent merges. These consistent efforts will help maintain an efficient and responsive Git workflow as your project grows.
Building Cross-Platform Excellence
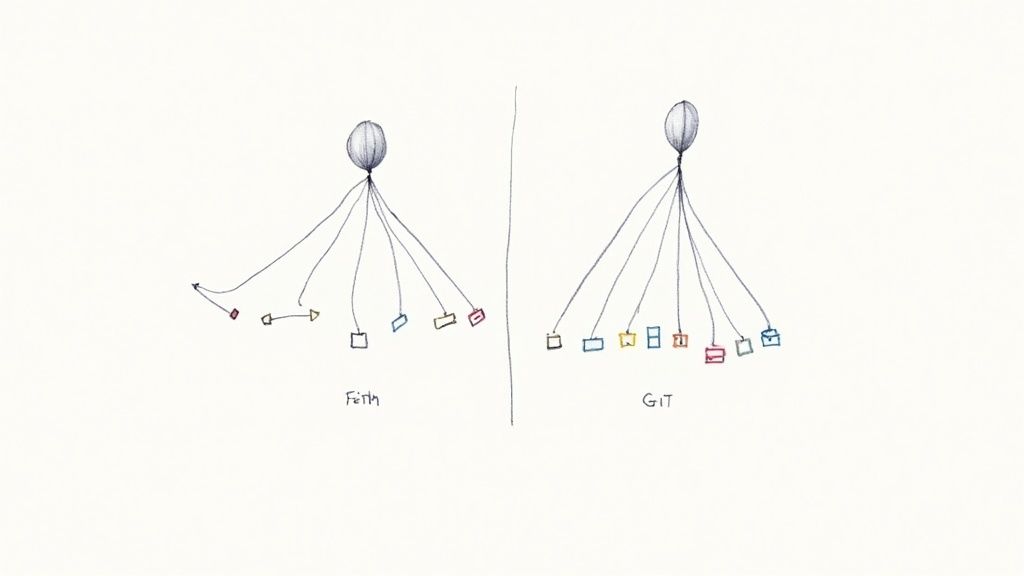
When development teams work across different operating systems, maintaining a consistent Git workflow becomes essential. Teams need Git commands like git fetch
and git pull
to work reliably whether someone is using Windows, macOS, or Linux. Let's look at practical ways to build a reliable cross-platform Git workflow that helps teams collaborate smoothly.
Addressing Platform-Specific Quirks
While Git works on any platform, some key differences between operating systems can cause issues. One common challenge is how different systems handle line endings - Windows uses CRLF line endings, while macOS and Linux use LF. Without proper configuration, this can lead to Git tracking unnecessary changes. The solution is to configure Git's line ending behavior using the core.autocrlf
setting:
- For Windows users, set
core.autocrlf
totrue
- this converts CRLF to LF when committing and back to CRLF when checking out files - For macOS and Linux users, set
core.autocrlf
toinput
- this ensures only LF endings are used in the repository
This configuration prevents line ending differences from causing problems. Using a shared .gitignore
file across the team also helps by excluding platform-specific files that shouldn't be in the repository.
Ensuring Consistent Tooling
The tools your team uses to interact with Git also impact cross-platform work. While Git's command line interface stays mostly consistent across systems, graphical interfaces can vary significantly. Consider having your team standardize on either the command line or a specific GUI client. This creates familiarity and reduces errors from using unfamiliar tools. For large repositories, test how different Git clients handle commands like git fetch
and git pull
- performance can vary depending on the implementation.
Practical Strategies for Cross-Platform Collaboration
Beyond technical setup, certain practices make cross-platform collaboration smoother. Clear branching strategies and standard commit message formats help maintain consistency regardless of operating system. This becomes especially important when using git fetch
to review changes or git pull
to integrate work - well-written commit messages provide crucial context across platforms. Regular team communication also plays a key role - sharing platform-specific tips and solutions helps prevent and quickly resolve issues. Following these strategies creates an environment where teams can work effectively together across any platform.
Crafting A Conflict-Free Workflow
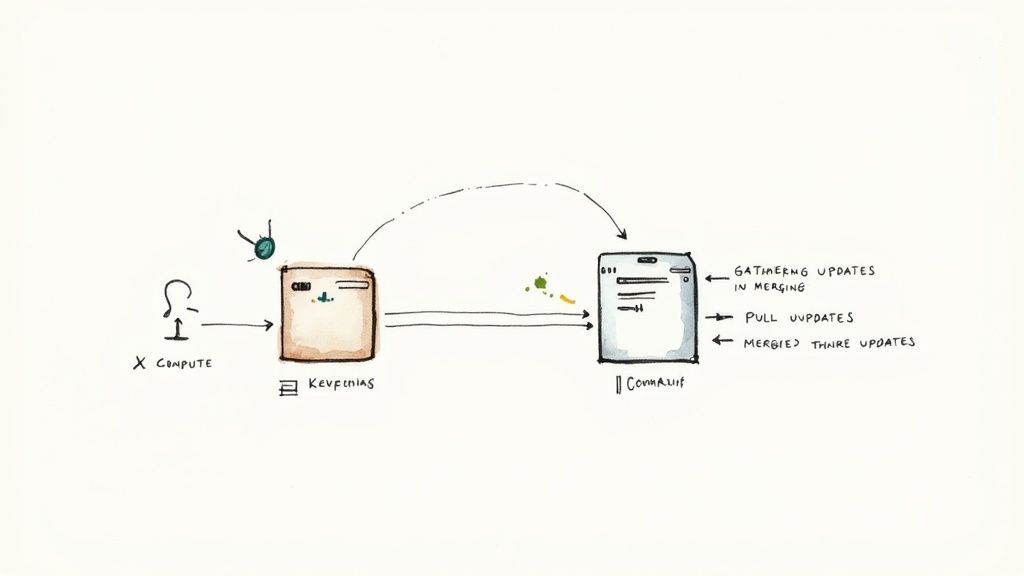
Every developer has experienced the headache of merge conflicts. But by making smart choices about when to use git fetch
versus git pull
, teams can work together smoothly while keeping conflicts to a minimum. Let's look at some practical ways to manage remote changes, keep your commit history clean, and handle conflicts when they do pop up.
Previewing Changes With git fetch
The best way to avoid conflicts is to look before you leap. That's where git fetch
comes in handy. Unlike git pull
, which immediately merges remote changes into your work, git fetch
lets you download and inspect updates first. This gives you a chance to see how incoming changes might affect your code before committing to them. For instance, if you're working on a feature while your teammates update the main branch, fetching first lets you spot potential issues early.
Understanding the Impact of Remote Changes
After fetching remote changes, you'll want to understand exactly what's different. One helpful approach is to map out the branch structure using git log --oneline --graph --decorate --all
. This gives you a clear picture of how commits relate to each other and where branches have split off. With this view, you can easily spot where your local work differs from the remote version and what kind of changes are coming in. This insight helps you plan the best way to combine everything.
Integrating Changes Strategically With git pull
or git merge
Once you've reviewed the changes, you can choose how to bring them in. For simple updates where you're sure there won't be conflicts, git pull
makes it quick and easy by fetching and merging in one step. But when things are more complex or you spot potential issues, git merge
gives you more control. You can merge specific branches or commits one at a time, dealing with any conflicts step by step. Many experienced teams prefer this careful approach, especially on shared branches or bigger projects.
Resolving Conflicts When They Occur
Even with careful planning, conflicts sometimes happen. When they do, having a clear process makes all the difference. Git points out exactly which parts of the code conflict, so you can edit those sections to fix things. You might need to pick one version over another, combine both changes, or write something new that works better. Tools like Mergify can help automate this process, which is especially useful for bigger projects or teams handling lots of pull requests.
Establishing a Team Workflow
At its core, avoiding conflicts depends on good teamwork. Teams need clear rules about how to branch, write commit messages, and keep each other in the loop. When everyone communicates well and takes care with code integration, you can minimize disruptions and keep work flowing smoothly, even with complex code and frequent updates. By focusing on preventing conflicts rather than just reacting to them, teams can spend more time building and less time untangling code.
Taking Control Of Complex Repositories
Every Git project starts simple but grows more complex as teams expand and requirements evolve. Once you've mastered the basics of git fetch
and git pull
, you'll need strategies to effectively manage growing codebases, especially when coordinating multiple remotes, feature branches, and team members.
Branching Models: The Foundation of Organized Development
A well-planned branching strategy is essential for coordinating development work, much like how construction crews need organized blueprints and workflows. Two common approaches stand out: The Gitflow model uses separate branches for development, features, releases, and fixes to keep changes isolated. In contrast, Trunk-Based Development focuses on frequent commits to a main branch with short-lived feature branches.
Your choice of branching model directly affects how efficiently you can use git fetch
and git pull
. Rather than pulling all changes every time, developers can target specific branches they need, reducing both network load and potential conflicts. This selective approach becomes especially important as repositories grow larger.
Managing Multiple Remotes: Expanding Your Collaboration Horizons
Working with multiple remote repositories adds complexity, particularly in open-source projects. Just as checking multiple physical mailboxes across town would be inefficient, fetching from numerous remotes can slow down your work. The key is being strategic - use git remote -v
to review your remotes and only fetch from the ones relevant to your current task. For example, when working on a feature based on an upstream project, focus on fetching from that upstream remote rather than your team's origin.
By combining focused remote management with clear branching rules, you can effectively handle complex projects. This approach helps separate experimental code from stable releases while maintaining clear records of who contributed what - especially valuable for large teams and open-source collaboration.
Maintaining a Clean History: The Art of Rewriting
Even with solid planning, codebases need occasional cleanup. Feature branches often accumulate experimental commits that could be simplified before merging. While detailed history has value, a clean, well-organized history makes code review and understanding easier. Interactive rebasing serves as your editing tool - like revising a draft before publication. You can combine multiple commits, change their order, or update commit messages to create a more readable history.
Tools like Mergify can help manage complex repositories by automating merges and enforcing branch rules as projects grow. For debugging, git bisect
helps pinpoint issues by efficiently searching commit history. These techniques and tools form the foundation for successful collaboration on large-scale projects.
Solving Real-World Git Challenges
Let's explore how git fetch
and git pull
help solve common Git challenges that developers face daily. From network issues to merge conflicts, understanding how to handle these situations will help you maintain smooth code collaboration.
Network Issues: Staying Productive Offline
Picture this: You're deep in coding when your internet drops. With git pull
, you'd be stuck since it needs a connection to fetch and merge remote changes. But if you've already used git fetch
to download remote updates, you can keep working locally. You can review changes against fetched remote branches and create new branches based on that state - all without an internet connection. Once you're back online, running git pull
will sync everything up.
The Dreaded Merge Conflict: Understanding and Resolution
Nobody likes merge conflicts, but they're part of collaborative development. While using git fetch
to preview changes helps spot potential issues early, sometimes conflicts are inevitable. They typically happen when you and a teammate modify the same code lines. When merging branches, Git marks these overlapping changes in the files. Your job is to carefully review and decide what to keep - your changes, their changes, or a mix of both. Taking time to resolve conflicts properly keeps your codebase clean.
Lost Commits: Recovering From the Abyss
Accidentally deleting commits can feel like a disaster, but Git provides several recovery options. If you've recently fetched changes, the remote branch might still have your lost work. The reflog command (git reflog
) shows your local Git history, including commits removed by rebase or reset operations. This history can help you find and restore lost work, especially if you fetched remote changes that provide a backup reference.
Large Teams and Continuous Integration: Strategies for Success
When working with big teams and CI systems, coordinating frequent changes becomes critical. Running git fetch
before pushing lets developers check and integrate updates locally first, reducing CI pipeline issues. Combined with good branching practices and regular integration using tools like Mergify, this approach helps maintain a stable main branch.
Are you spending too much time managing pull requests and resolving conflicts? Mergify automates your PR workflow with features like automatic merging and merge queues. This lets your team focus on writing great code instead of juggling merges. Visit Mergify to learn more about streamlining your development process.