Essential Git Workflow Best Practices: A Complete Guide for Modern Development
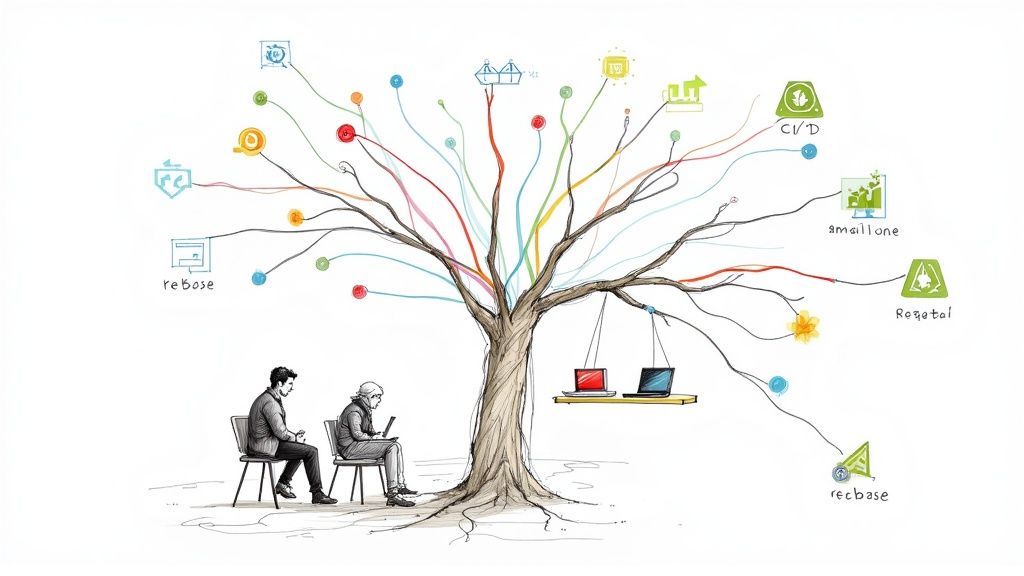
Making Sense of Modern Git Workflows
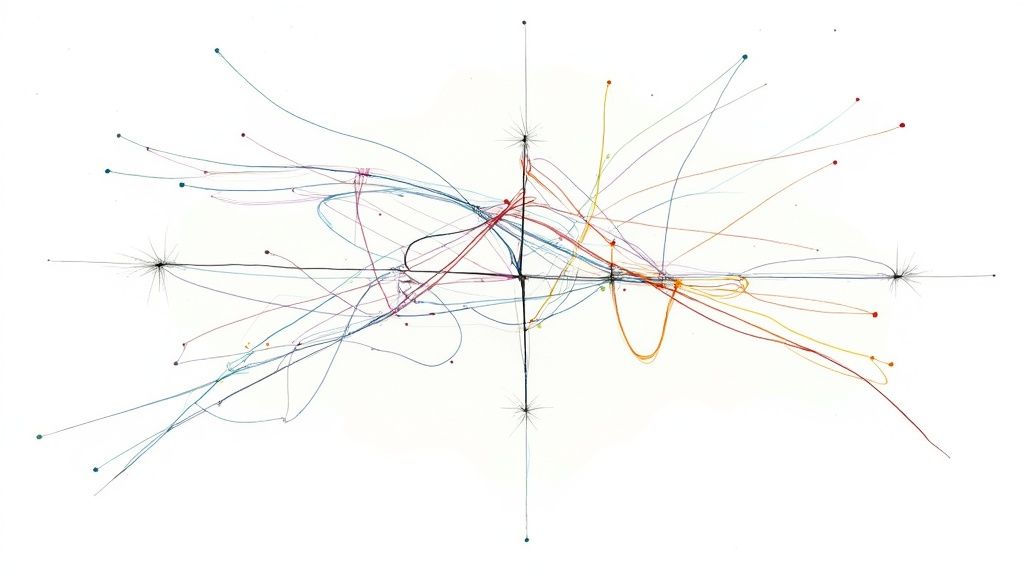
Good software development depends on teams working together smoothly through version control. That's why picking the right Git workflow makes a huge difference in how well a project turns out. When teams find an approach that fits, it helps everyone code better together and produce higher quality work. But with so many different Git workflows out there, it can be tricky to figure out which one to use. Let's look at the most common approaches and how to pick what works best for your team.
Choosing the Right Git Workflow Model
Finding the best Git workflow is like selecting tools for a job - you need to match the approach to your needs. Each model has its own pros and cons that are worth understanding. Here are the key options to consider:
- Gitflow Workflow: This detailed model works well for bigger projects that need carefully planned releases. It uses different branches for new features, releases, and quick fixes to keep everything organized. But for smaller projects, it might be more structure than you need. For example, a small team building a mobile app might find it too restrictive.
- Feature Branch Workflow: Teams often prefer this simpler approach of making new branches for each feature or bug fix. Working on separate branches helps keep code clean and prevents conflicts between developers. It's flexible enough for teams of any size. A web development team, for instance, could use it to work on different parts of a site without getting in each other's way.
- Forking Workflow: This model shines in open-source projects where lots of people contribute code. Each developer works with their own copy of the code and submits changes through pull requests. This setup protects the main codebase while making it easy for anyone to contribute. It's especially good for projects with many outside contributors who shouldn't have direct access to the main code.
Implementing Git Workflow Best Practices Across Models
No matter which workflow you pick, certain practices help teams work better together:
- Meaningful Commit Messages: Clear messages help everyone understand what changed and why. Good messages explain the reasoning behind changes, making it easier to track the project's history and fix issues later.
- Frequent Commits: Making small, regular commits creates a detailed record of changes. This makes it simple to find and fix problems by rolling back to earlier versions when needed.
- Strategic Branching: Creating specific branches for new features or fixes keeps the main code stable. This helps maintain quality and prevents integration headaches down the road.
- Thorough Code Reviews: Reviews catch problems early and help teams share knowledge. Mergify can help automate this process - it handles code integration, reduces testing costs, and keeps code secure. Its features update pull requests automatically, group testing jobs efficiently, and help spot infrastructure issues early.
These practices help teams work better together no matter which workflow they use. They lead to cleaner code that's easier to maintain and help create a more collaborative environment where everyone can do their best work.
Mastering the Art of Feature Branches
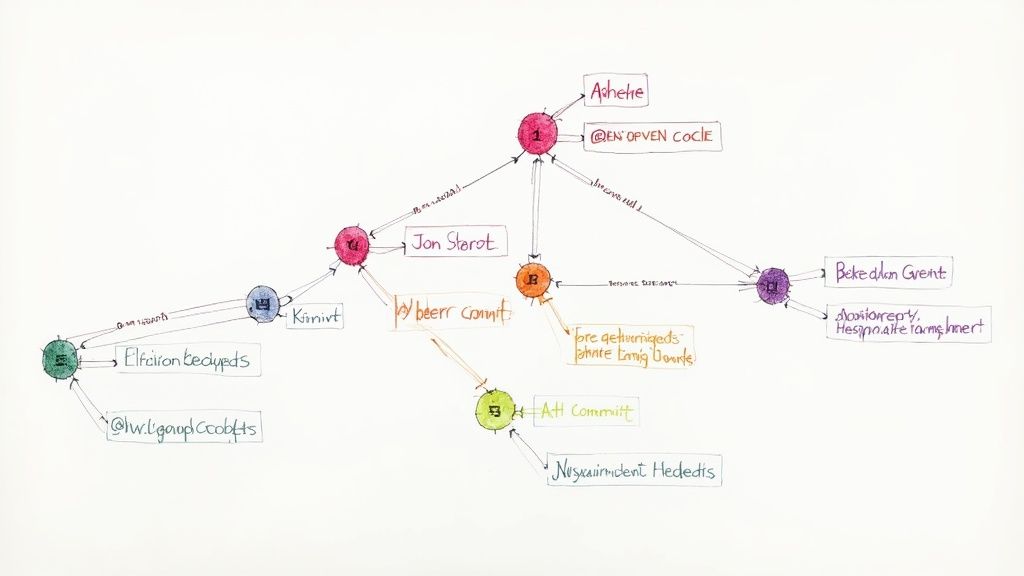
Feature branches form a key part of modern development workflows, allowing teams to work on new features and fixes without affecting the main codebase. Many software teams use feature branching to speed up development while keeping their code stable. By isolating work in separate branches, developers can experiment freely without worrying about breaking production code. Let's explore how to make the most of feature branches for your team.
Naming Conventions for Clarity
Good branch names help everyone understand what work is happening where. Using consistent naming makes it easy to track projects and communicate about changes. Start branch names with prefixes that show their purpose - for example, feat/
for new features, fix/
for bug fixes, and chore/
for maintenance work. After the prefix, add a short description like feat/user-authentication
or fix/login-error-handling
. Clear names make it simple to search through branches and see what each one contains.
Strategic Branch Management
Managing branches well prevents confusion and keeps work flowing smoothly. When starting new work, create your feature branch from the latest version of the main or development branch. This reduces merge conflicts later. Once you finish and test your changes, open a pull request to merge them back. This lets your team review the code and share knowledge. After merging, delete the old feature branch to keep your repository tidy. A clean branch structure makes it easier for everyone to find what they need.
Resolving Conflicts Effectively
When multiple developers work on related code, conflicts will happen during merges. The key is handling them carefully to maintain code quality. First, understand what caused the conflict. Git shows exactly which lines changed in both branches, so you can decide how to combine them correctly. For example, when merging feature work into main, Git marks any overlapping changes. You'll need to edit those files manually to resolve the conflicts and ensure everything works properly. Visual merge tools can help by showing the differences side-by-side. Talk with your team when resolving tricky conflicts - often someone else's perspective leads to better solutions. Good communication during conflict resolution strengthens team collaboration and improves the whole development process.
Building a Commit Strategy That Makes Sense
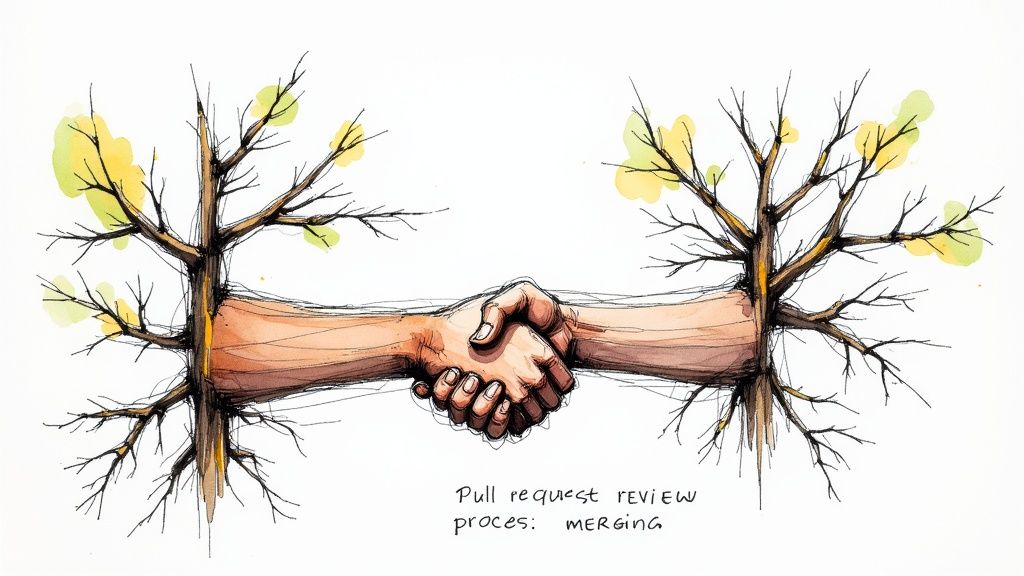
Good commit practices are essential for effective teamwork and code maintenance. When teams follow clear commit guidelines, they can better understand code changes, even when looking back months later. This means writing clear commit messages, committing code at the right frequency, and keeping your Git history organized. Let's explore these key practices and how to use them in your projects.
Writing Effective Commit Messages
A commit message is often the main way developers learn about past code changes. That's why writing clear, informative messages matters so much for team collaboration. Good commit messages should tell you both what changed and why it changed. Just describing the code update isn't enough - you need to explain the reasoning behind it to help with future debugging and code reviews.
Here's an example of the difference it makes: Compare "Fixed bug" to "Fixed bug in user authentication flow caused by incorrect handling of expired session tokens. Added check for token expiry before processing login request." The second message gives your team the full picture - what the bug was, where it occurred, and how it was fixed. This level of detail saves time when investigating issues or reviewing old code changes.
Finding the Right Commit Frequency
How often you commit code has a big impact on your project's Git history and how easy it is to work with. Committing too frequently can fill your history with tiny, unhelpful changes. But waiting too long between commits makes it hard to track how your code evolved. The sweet spot is committing complete units of work - like a finished feature, a bug fix, or one clear step in a larger task.
Small, focused commits also make it easier to undo changes when needed. Think about trying to reverse a huge commit that contains many unrelated updates - it's risky and complex. When commits are focused on one thing, you can easily roll back specific changes without affecting other code. This precise control is crucial for debugging and keeping your codebase stable. For example, Facebook ran into this exact problem with their massive codebase - Git struggled to handle millions of files, leading them to consider switching to Mercurial for better performance.
Keeping Your History Clean: Squashing and Rewriting Commits
A clean Git history makes projects easier to maintain long-term. Tools like squashing let you combine several small, related commits into one meaningful update. This is especially helpful before merging feature branches into your main branch, as it keeps your history clear and easy to follow. Just be careful when rewriting history on shared branches - it can cause problems for other developers, so make sure your team agrees on when and how to use these tools.
By keeping your Git history organized, you create a clear story of how your code developed. This helps other developers understand your project's evolution and makes it easier for teams to work together effectively.
Navigating the Merge vs. Rebase Decision
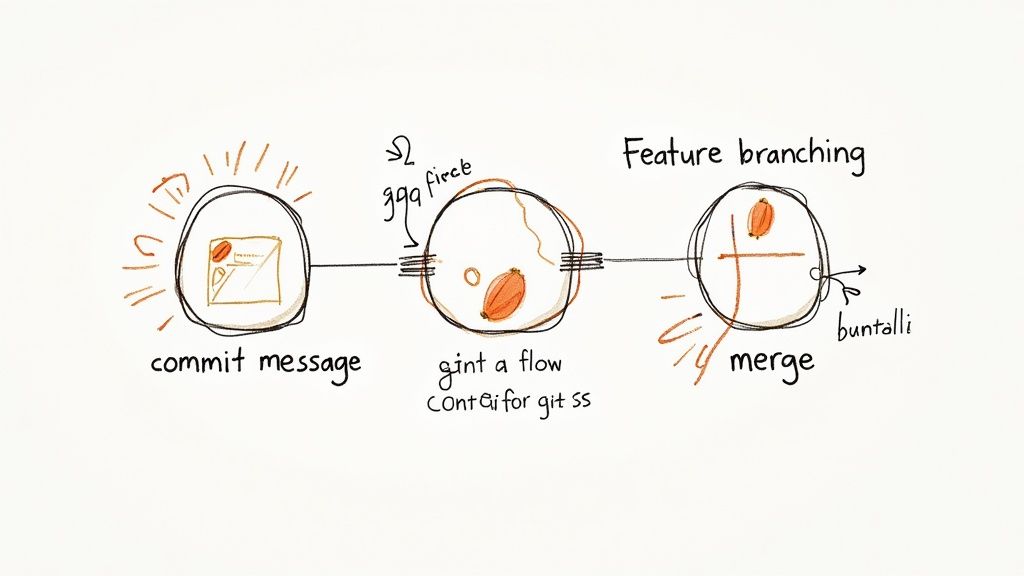
When working with Git branches, developers often face a key decision: whether to merge or rebase their changes. Both approaches help combine code from different branches, but they work differently and serve different purposes. Understanding when to use each method helps teams maintain clear project history and smooth collaboration.
Understanding the Merge Operation
A merge creates a new commit that brings together changes from two branches. Think of it like combining two parallel timelines into one - the merge commit shows exactly when and how the branches came together. For instance, when merging a feature branch into main
, Git creates a commit that preserves both branches' history.
This approach works well when teams need to track how features developed over time. Teams can easily see when different pieces came together and trace the evolution of the codebase. The downside? Active projects with lots of merges can end up with a complex history full of merge commits, which some find harder to follow.
Exploring the Rebase Alternative
Rebasing takes a different approach. Instead of creating a merge commit, it moves commits from one branch and places them at the end of another branch. Picture taking sticky notes from one page and placing them at the end of another - you're rewriting where those changes happened. When you rebase a feature branch onto main
, it looks like you wrote that code directly on top of the latest main
branch.
This creates a simpler, straight-line history that's easy to read. However, there's an important catch - rebasing changes Git's history. If other developers are working with the branch you rebased, they'll run into problems since their version of history no longer matches. For this reason, rebasing shared branches is generally discouraged.
Making the Right Choice: Merge vs. Rebase
The best choice between merging and rebasing depends on your specific situation. When bringing feature branches into main
, merging often makes more sense. It preserves the true story of how the feature developed and provides clear documentation of when changes were integrated. This visibility helps teams track progress and troubleshoot issues.
Rebasing shines when keeping feature branches current with main
. By regularly rebasing your feature branch onto the latest main
, you incorporate new changes as they happen. This reduces the chance of painful merge conflicts later. For example, if you're working on a feature that takes several weeks, rebasing onto main
every few days keeps your code aligned with the rest of the project.
Consider a team working on a large new feature. They might rebase their feature branch onto main
weekly to stay current, then do a proper merge when the feature is complete. This combines the benefits of both approaches - clean development with rebasing, followed by clear history with merging.
Teams that understand both merging and rebasing can use each tool effectively. The key is picking the right approach for each situation, which leads to clearer history and smoother collaboration. These choices form an essential part of any effective Git workflow.
Creating a Code Review Culture That Works
Creating meaningful code reviews is essential for any team striving to maintain high code quality. Well-executed reviews strengthen collaboration, catch potential issues early, and help teams deliver better software. Let's explore how to build an effective code review process through smart use of pull requests, clear feedback structures, and practical tools.
Pull Requests: The Heart of Collaborative Review
Pull requests serve as the central hub where code review happens. They provide a structured space for developers to examine changes together, discuss implementation approaches, and share knowledge across the team. When reviewing a new feature, team members can dive into the technical details, propose alternative solutions, and ensure the code fits the project's architecture. This collaborative discussion improves code quality while helping spread expertise throughout the team. Pull requests also create a clear history of changes, making it easier to understand how the codebase has evolved over time.
Structuring Reviews for Meaningful Feedback
Clear guidelines make code reviews more productive for everyone involved. Teams benefit from having specific checklists and focus areas for reviewers to consider, along with recommendations for static analysis tools to use. For example, reviewers might evaluate not just whether code works correctly, but also how readable and maintainable it is, and how well it performs. When contributors understand exactly how to implement feedback, it reduces back-and-forth discussions and speeds up the revision process.
Balancing Thoroughness and Velocity in Code Reviews
Reviews need to be thorough enough to catch issues but quick enough to maintain development momentum. Many teams tackle this challenge by using automated tests and linters to catch basic errors early, allowing human reviewers to focus on higher-level concerns like design choices and architectural decisions. Setting clear timelines also helps - for instance, aiming to provide initial feedback within 24-48 hours prevents pull requests from becoming bottlenecks. This keeps development flowing while maintaining quality standards.
Tools and Techniques for Effective Code Reviews
The right tools can significantly improve the review process. Mergify helps automate code integration tasks like updating pull requests and enforcing merge rules. Code analysis tools catch common issues before human review begins. This automation lets reviewers spend their time on more complex aspects of the code while speeding up the feedback cycle. When combined with good review practices, these tools help teams deliver quality code efficiently. By focusing on both the technical and human elements of code review, teams can make this process a valuable part of their development workflow.
Integrating CI/CD Into Your Git Workflow
Software development teams need smart automation to work efficiently. By adding Continuous Integration and Continuous Delivery (CI/CD) to your Git workflow, you can automate building, testing, and deploying code while maintaining high quality. Let's look at how development teams are using CI/CD effectively with Git to speed up their work and boost productivity.
Automating Quality Checks With CI
Continuous Integration helps teams automatically check code changes from multiple developers. Think of a team building a web application - whenever someone pushes new code to a branch, the CI system springs into action. It compiles everything, runs tests, analyzes code quality, and can even deploy to a test environment. This automation catches problems early, reduces manual work, and keeps code quality consistent across the team.
Streamlining Deployments With CD
Continuous Delivery takes things further by automating how code moves to production. Once changes pass all CI checks, CD can automatically deploy them to staging or production environments. This speeds up releases while reducing human mistakes. Teams can quickly ship updates based on user feedback, helping them stay ahead of user needs.
Implementing Automated Testing Strategies
Good testing is key to CI/CD success. Automated tests help catch bugs before they reach users. A complete testing approach usually includes:
- Unit Tests: Check individual code components work correctly on their own
- Integration Tests: Verify different parts of the app work well together
- End-to-End Tests: Run through real user scenarios to test the full application
This layered testing gives teams confidence to move quickly while maintaining quality.
Real-World CI/CD Configurations for Git Workflows
Popular platforms like GitLab CI, GitHub Actions, and Jenkins work smoothly with Git. They provide tools to set up CI/CD pipelines and trigger actions based on Git events like pushes and merges. Here's a basic GitLab CI setup example:
Stage | Action |
---|---|
Build | Compile code, run linters |
Test | Execute unit and integration tests |
Deploy | Deploy to staging environment |
This structured process ensures all code changes go through consistent checks before reaching users. Teams find that using CI/CD leads to smoother releases, faster feedback, and better code quality in their Git workflows.
Adding CI/CD to Git is essential for modern development teams. Automated testing and deployment help teams work faster while maintaining high standards. Tools like Mergify can improve this further by automating merges and managing pull requests. When teams combine Git with solid CI/CD practices, they create an efficient, reliable development process that helps them ship better software faster.